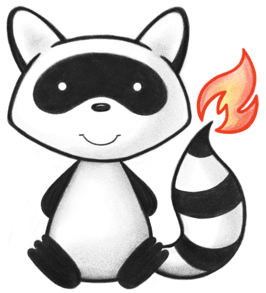
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3AcknowledgementType { 037 038 /** 039 * Receiving application successfully processed message. 040 */ 041 AA, 042 /** 043 * Receiving application found error in processing message. Sending error 044 * response with additional error detail information. 045 */ 046 AE, 047 /** 048 * Receiving application failed to process message for reason unrelated to 049 * content or format. Original message sender must decide on whether to 050 * automatically send message again. 051 */ 052 AR, 053 /** 054 * Receiving message handling service accepts responsibility for passing message 055 * onto receiving application. 056 */ 057 CA, 058 /** 059 * Receiving message handling service cannot accept message for any other reason 060 * (e.g. message sequence number, etc.). 061 */ 062 CE, 063 /** 064 * Receiving message handling service rejects message if interaction identifier, 065 * version or processing mode is incompatible with known receiving application 066 * role information. 067 */ 068 CR, 069 /** 070 * added to help the parsers 071 */ 072 NULL; 073 074 public static V3AcknowledgementType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("AA".equals(codeString)) 078 return AA; 079 if ("AE".equals(codeString)) 080 return AE; 081 if ("AR".equals(codeString)) 082 return AR; 083 if ("CA".equals(codeString)) 084 return CA; 085 if ("CE".equals(codeString)) 086 return CE; 087 if ("CR".equals(codeString)) 088 return CR; 089 throw new FHIRException("Unknown V3AcknowledgementType code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case AA: 095 return "AA"; 096 case AE: 097 return "AE"; 098 case AR: 099 return "AR"; 100 case CA: 101 return "CA"; 102 case CE: 103 return "CE"; 104 case CR: 105 return "CR"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 return "http://terminology.hl7.org/CodeSystem/v3-AcknowledgementType"; 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case AA: 120 return "Receiving application successfully processed message."; 121 case AE: 122 return "Receiving application found error in processing message. Sending error response with additional error detail information."; 123 case AR: 124 return "Receiving application failed to process message for reason unrelated to content or format. Original message sender must decide on whether to automatically send message again."; 125 case CA: 126 return "Receiving message handling service accepts responsibility for passing message onto receiving application."; 127 case CE: 128 return "Receiving message handling service cannot accept message for any other reason (e.g. message sequence number, etc.)."; 129 case CR: 130 return "Receiving message handling service rejects message if interaction identifier, version or processing mode is incompatible with known receiving application role information."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case AA: 141 return "Application Acknowledgement Accept"; 142 case AE: 143 return "Application Acknowledgement Error"; 144 case AR: 145 return "Application Acknowledgement Reject"; 146 case CA: 147 return "Accept Acknowledgement Commit Accept"; 148 case CE: 149 return "Accept Acknowledgement Commit Error"; 150 case CR: 151 return "Accept Acknowledgement Commit Reject"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159}