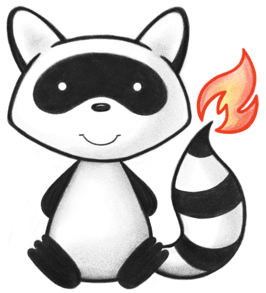
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ActCode { 037 038 /** 039 * An account represents a grouping of financial transactions that are tracked 040 * and reported together with a single balance. Examples of account codes 041 * (types) are Patient billing accounts (collection of charges), Cost centers; 042 * Cash. 043 */ 044 _ACTACCOUNTCODE, 045 /** 046 * An account for collecting charges, reversals, adjustments and payments, 047 * including deductibles, copayments, coinsurance (financial transactions) 048 * credited or debited to the account receivable account for a patient's 049 * encounter. 050 */ 051 ACCTRECEIVABLE, 052 /** 053 * Cash 054 */ 055 CASH, 056 /** 057 * Description: Types of advance payment to be made on a plastic card usually 058 * issued by a financial institution used of purchasing services and/or 059 * products. 060 */ 061 CC, 062 /** 063 * American Express 064 */ 065 AE, 066 /** 067 * Diner's Club 068 */ 069 DN, 070 /** 071 * Discover Card 072 */ 073 DV, 074 /** 075 * Master Card 076 */ 077 MC, 078 /** 079 * Visa 080 */ 081 V, 082 /** 083 * An account representing charges and credits (financial transactions) for a 084 * patient's encounter. 085 */ 086 PBILLACCT, 087 /** 088 * Includes coded responses that will occur as a result of the adjudication of 089 * an electronic invoice at a summary level and provides guidance on 090 * interpretation of the referenced adjudication results. 091 */ 092 _ACTADJUDICATIONCODE, 093 /** 094 * Catagorization of grouping criteria for the associated transactions and/or 095 * summary (totals, subtotals). 096 */ 097 _ACTADJUDICATIONGROUPCODE, 098 /** 099 * Transaction counts and value totals by Contract Identifier. 100 */ 101 CONT, 102 /** 103 * Transaction counts and value totals for each calendar day within the date 104 * range specified. 105 */ 106 DAY, 107 /** 108 * Transaction counts and value totals by service location (e.g clinic). 109 */ 110 LOC, 111 /** 112 * Transaction counts and value totals for each calendar month within the date 113 * range specified. 114 */ 115 MONTH, 116 /** 117 * Transaction counts and value totals for the date range specified. 118 */ 119 PERIOD, 120 /** 121 * Transaction counts and value totals by Provider Identifier. 122 */ 123 PROV, 124 /** 125 * Transaction counts and value totals for each calendar week within the date 126 * range specified. 127 */ 128 WEEK, 129 /** 130 * Transaction counts and value totals for each calendar year within the date 131 * range specified. 132 */ 133 YEAR, 134 /** 135 * The invoice element has been accepted for payment but one or more 136 * adjustment(s) have been made to one or more invoice element line items 137 * (component charges). 138 * 139 * Also includes the concept 'Adjudicate as zero' and items not covered under a 140 * particular Policy. 141 * 142 * Invoice element can be reversed (nullified). 143 * 144 * Recommend that the invoice element is saved for DUR (Drug Utilization 145 * Reporting). 146 */ 147 AA, 148 /** 149 * The invoice element has been accepted for payment but one or more 150 * adjustment(s) have been made to one or more invoice element line items 151 * (component charges) without changing the amount. 152 * 153 * Invoice element can be reversed (nullified). 154 * 155 * Recommend that the invoice element is saved for DUR (Drug Utilization 156 * Reporting). 157 */ 158 ANF, 159 /** 160 * The invoice element has passed through the adjudication process but payment 161 * is refused due to one or more reasons. 162 * 163 * Includes items such as patient not covered, or invoice element is not 164 * constructed according to payer rules (e.g. 'invoice submitted too late'). 165 * 166 * If one invoice element line item in the invoice element structure is 167 * rejected, the remaining line items may not be adjudicated and the complete 168 * group is treated as rejected. 169 * 170 * A refused invoice element can be forwarded to the next payer (for 171 * Coordination of Benefits) or modified and resubmitted to refusing payer. 172 * 173 * Invoice element cannot be reversed (nullified) as there is nothing to 174 * reverse. 175 * 176 * Recommend that the invoice element is not saved for DUR (Drug Utilization 177 * Reporting). 178 */ 179 AR, 180 /** 181 * The invoice element was/will be paid exactly as submitted, without financial 182 * adjustment(s). 183 * 184 * If the dollar amount stays the same, but the billing codes have been amended 185 * or financial adjustments have been applied through the adjudication process, 186 * the invoice element is treated as "Adjudicated with Adjustment". 187 * 188 * If information items are included in the adjudication results that do not 189 * affect the monetary amounts paid, then this is still Adjudicated as Submitted 190 * (e.g. 'reached Plan Maximum on this Claim'). 191 * 192 * Invoice element can be reversed (nullified). 193 * 194 * Recommend that the invoice element is saved for DUR (Drug Utilization 195 * Reporting). 196 */ 197 AS, 198 /** 199 * Actions to be carried out by the recipient of the Adjudication Result 200 * information. 201 */ 202 _ACTADJUDICATIONRESULTACTIONCODE, 203 /** 204 * The adjudication result associated is to be displayed to the receiver of the 205 * adjudication result. 206 */ 207 DISPLAY, 208 /** 209 * The adjudication result associated is to be printed on the specified form, 210 * which is then provided to the covered party. 211 */ 212 FORM, 213 /** 214 * Definition:An identifying modifier code for healthcare interventions or 215 * procedures. 216 */ 217 _ACTBILLABLEMODIFIERCODE, 218 /** 219 * Description:CPT modifier codes are found in Appendix A of CPT 2000 Standard 220 * Edition. 221 */ 222 CPTM, 223 /** 224 * Description:HCPCS Level II (HCFA-assigned) and Carrier-assigned (Level III) 225 * modifiers are reported in Appendix A of CPT 2000 Standard Edition and in the 226 * Medicare Bulletin. 227 */ 228 HCPCSA, 229 /** 230 * The type of provision(s) made for reimbursing for the deliver of healthcare 231 * services and/or goods provided by a Provider, over a specified period. 232 */ 233 _ACTBILLINGARRANGEMENTCODE, 234 /** 235 * A billing arrangement where a Provider charges a lump sum to provide a 236 * prescribed group (volume) of services to a single patient which occur over a 237 * period of time. Services included in the block may vary. 238 * 239 * This billing arrangement is also known as Program of Care for some specific 240 * Payors and Program Fees for other Payors. 241 */ 242 BLK, 243 /** 244 * A billing arrangement where the payment made to a Provider is determined by 245 * analyzing one or more demographic attributes about the persons/patients who 246 * are enrolled with the Provider (in their practice). 247 */ 248 CAP, 249 /** 250 * A billing arrangement where a Provider charges a lump sum to provide a 251 * particular volume of one or more interventions/procedures or groups of 252 * interventions/procedures. 253 */ 254 CONTF, 255 /** 256 * A billing arrangement where a Provider charges for non-clinical items. This 257 * includes interest in arrears, mileage, etc. Clinical content is not included 258 * in Invoices submitted with this type of billing arrangement. 259 */ 260 FINBILL, 261 /** 262 * A billing arrangement where funding is based on a list of individuals 263 * registered as patients of the Provider. 264 */ 265 ROST, 266 /** 267 * A billing arrangement where a Provider charges a sum to provide a group 268 * (volume) of interventions/procedures to one or more patients within a defined 269 * period of time, typically on the same date. Interventions/procedures included 270 * in the session may vary. 271 */ 272 SESS, 273 /** 274 * A billing arrangement where a Provider charges a separate fee for each 275 * intervention/procedure/event or product. 276 * 277 * Fee for Service is used when an individual intervention/procedure/event is 278 * used for billing purposes. In other words, fees are associated with the 279 * intervention/procedure/event. For example, a specific CCI (Canadian 280 * Classification of Interventions) code has an associated fee and is used for 281 * billing purposes. 282 */ 283 FFS, 284 /** 285 * A first fill where the quantity supplied is less than one full repetition of 286 * the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial 287 * fill might be for only 30 tablets.) and also where the strength supplied is 288 * less than the ordered strength (e.g. 10mg for an order of 50mg where a 289 * subsequent fill will dispense 40mg tablets) 290 */ 291 FFPS, 292 /** 293 * A first fill where the quantity supplied is equal to one full repetition of 294 * the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete 295 * fill would be for the full 90 tablets) and also where the strength supplied 296 * is less than the ordered strength (e.g. 10mg for an order of 50mg where a 297 * subsequent fill will dispense 40mg tablets). 298 */ 299 FFCS, 300 /** 301 * A fill where a small portion is provided to allow for determination of the 302 * therapy effectiveness and patient tolerance and also where the strength 303 * supplied is less than the ordered strength (e.g. 10mg for an order of 50mg 304 * where a subsequent fill will dispense 40mg tablets). 305 */ 306 TFS, 307 /** 308 * Type of bounded ROI. 309 */ 310 _ACTBOUNDEDROICODE, 311 /** 312 * A fully specified bounded Region of Interest (ROI) delineates a ROI in which 313 * only those dimensions participate that are specified by boundary criteria, 314 * whereas all other dimensions are excluded. For example a ROI to mark an 315 * episode of "ST elevation" in a subset of the EKG leads V2, V3, and V4 would 316 * include 4 boundaries, one each for time, V2, V3, and V4. 317 */ 318 ROIFS, 319 /** 320 * A partially specified bounded Region of Interest (ROI) specifies a ROI in 321 * which at least all values in the dimensions specified by the boundary 322 * criteria participate. For example, if an episode of ventricular fibrillations 323 * (VFib) is observed, it usually doesn't make sense to exclude any EKG leads 324 * from the observation and the partially specified ROI would contain only one 325 * boundary for time indicating the time interval where VFib was observed. 326 */ 327 ROIPS, 328 /** 329 * Description:The type and scope of responsibility taken-on by the performer of 330 * the Act for a specific subject of care. 331 */ 332 _ACTCAREPROVISIONCODE, 333 /** 334 * Description:The type and scope of legal and/or professional responsibility 335 * taken-on by the performer of the Act for a specific subject of care as 336 * described by a credentialing agency, i.e. government or non-government 337 * agency. Failure in executing this Act may result in loss of credential to the 338 * person or organization who participates as performer of the Act. Excludes 339 * employment agreements. 340 * 341 * 342 * Example:Hospital license; physician license; clinic accreditation. 343 */ 344 _ACTCREDENTIALEDCARECODE, 345 /** 346 * Description:The type and scope of legal and/or professional responsibility 347 * taken-on by the performer of the Act for a specific subject of care as 348 * described by an agency for credentialing individuals. 349 */ 350 _ACTCREDENTIALEDCAREPROVISIONPERSONCODE, 351 /** 352 * Description:Scope of responsibility taken on for specialty care as defined by 353 * the respective Specialty Board. 354 */ 355 CACC, 356 /** 357 * Description:Scope of responsibility taken on for specialty care as defined by 358 * the respective Specialty Board. 359 */ 360 CAIC, 361 /** 362 * Description:Scope of responsibility taken on for specialty care as defined by 363 * the respective Specialty Board. 364 */ 365 CAMC, 366 /** 367 * Description:Scope of responsibility taken on for specialty care as defined by 368 * the respective Specialty Board. 369 */ 370 CANC, 371 /** 372 * Description:Scope of responsibility taken on for specialty care as defined by 373 * the respective Specialty Board. 374 */ 375 CAPC, 376 /** 377 * Description:Scope of responsibility taken on for specialty care as defined by 378 * the respective Specialty Board. 379 */ 380 CBGC, 381 /** 382 * Description:Scope of responsibility taken on for specialty care as defined by 383 * the respective Specialty Board. 384 */ 385 CCCC, 386 /** 387 * Description:Scope of responsibility taken on for specialty care as defined by 388 * the respective Specialty Board. 389 */ 390 CCGC, 391 /** 392 * Description:Scope of responsibility taken on for specialty care as defined by 393 * the respective Specialty Board. 394 */ 395 CCPC, 396 /** 397 * Description:Scope of responsibility taken on for specialty care as defined by 398 * the respective Specialty Board. 399 */ 400 CCSC, 401 /** 402 * Description:Scope of responsibility taken on for specialty care as defined by 403 * the respective Specialty Board. 404 */ 405 CDEC, 406 /** 407 * Description:Scope of responsibility taken on for specialty care as defined by 408 * the respective Specialty Board. 409 */ 410 CDRC, 411 /** 412 * Description:Scope of responsibility taken on for specialty care as defined by 413 * the respective Specialty Board. 414 */ 415 CEMC, 416 /** 417 * Description:Scope of responsibility taken on for specialty care as defined by 418 * the respective Specialty Board. 419 */ 420 CFPC, 421 /** 422 * Description:Scope of responsibility taken on for specialty care as defined by 423 * the respective Specialty Board. 424 */ 425 CIMC, 426 /** 427 * Description:Scope of responsibility taken on for specialty care as defined by 428 * the respective Specialty Board. 429 */ 430 CMGC, 431 /** 432 * Description:Scope of responsibility taken on for specialty care as defined by 433 * the respective Specialty Board 434 */ 435 CNEC, 436 /** 437 * Description:Scope of responsibility taken on for specialty care as defined by 438 * the respective Specialty Board. 439 */ 440 CNMC, 441 /** 442 * Description:Scope of responsibility taken on for specialty care as defined by 443 * the respective Specialty Board. 444 */ 445 CNQC, 446 /** 447 * Description:Scope of responsibility taken on for specialty care as defined by 448 * the respective Specialty Board. 449 */ 450 CNSC, 451 /** 452 * Description:Scope of responsibility taken on for specialty care as defined by 453 * the respective Specialty Board. 454 */ 455 COGC, 456 /** 457 * Description:Scope of responsibility taken on for specialty care as defined by 458 * the respective Specialty Board. 459 */ 460 COMC, 461 /** 462 * Description:Scope of responsibility taken on for specialty care as defined by 463 * the respective Specialty Board. 464 */ 465 COPC, 466 /** 467 * Description:Scope of responsibility taken on for specialty care as defined by 468 * the respective Specialty Board. 469 */ 470 COSC, 471 /** 472 * Description:Scope of responsibility taken on for specialty care as defined by 473 * the respective Specialty Board. 474 */ 475 COTC, 476 /** 477 * Description:Scope of responsibility taken on for specialty care as defined by 478 * the respective Specialty Board. 479 */ 480 CPEC, 481 /** 482 * Description:Scope of responsibility taken on for specialty care as defined by 483 * the respective Specialty Board. 484 */ 485 CPGC, 486 /** 487 * Description:Scope of responsibility taken on for specialty care as defined by 488 * the respective Specialty Board. 489 */ 490 CPHC, 491 /** 492 * Description:Scope of responsibility taken on for specialty care as defined by 493 * the respective Specialty Board. 494 */ 495 CPRC, 496 /** 497 * Description:Scope of responsibility taken on for specialty care as defined by 498 * the respective Specialty Board. 499 */ 500 CPSC, 501 /** 502 * Description:Scope of responsibility taken on for specialty care as defined by 503 * the respective Specialty Board. 504 */ 505 CPYC, 506 /** 507 * Description:Scope of responsibility taken on for specialty care as defined by 508 * the respective Specialty Board. 509 */ 510 CROC, 511 /** 512 * Description:Scope of responsibility taken on for specialty care as defined by 513 * the respective Specialty Board. 514 */ 515 CRPC, 516 /** 517 * Description:Scope of responsibility taken on for specialty care as defined by 518 * the respective Specialty Board. 519 */ 520 CSUC, 521 /** 522 * Description:Scope of responsibility taken on for specialty care as defined by 523 * the respective Specialty Board. 524 */ 525 CTSC, 526 /** 527 * Description:Scope of responsibility taken on for specialty care as defined by 528 * the respective Specialty Board. 529 */ 530 CURC, 531 /** 532 * Description:Scope of responsibility taken on for specialty care as defined by 533 * the respective Specialty Board. 534 */ 535 CVSC, 536 /** 537 * Description:Scope of responsibility taken-on for physician care of a patient 538 * as defined by a governmental licensing agency. 539 */ 540 LGPC, 541 /** 542 * Description:The type and scope of legal and/or professional responsibility 543 * taken-on by the performer of the Act for a specific subject of care as 544 * described by an agency for credentialing programs within organizations. 545 */ 546 _ACTCREDENTIALEDCAREPROVISIONPROGRAMCODE, 547 /** 548 * Description:Scope of responsibility taken on by an organization for care of a 549 * patient as defined by the respective accreditation agency. 550 */ 551 AALC, 552 /** 553 * Description:Scope of responsibility taken on by an organization for care of a 554 * patient as defined by the respective accreditation agency. 555 */ 556 AAMC, 557 /** 558 * Description:Scope of responsibility taken on by an organization for care of a 559 * patient as defined by the respective accreditation agency. 560 */ 561 ABHC, 562 /** 563 * Description:Scope of responsibility taken on by an organization for care of a 564 * patient as defined by the respective accreditation agency. 565 */ 566 ACAC, 567 /** 568 * Description:Scope of responsibility taken on by an organization for care of a 569 * patient as defined by the respective accreditation agency. 570 */ 571 ACHC, 572 /** 573 * Description:Scope of responsibility taken on by an organization for care of a 574 * patient as defined by the respective accreditation agency. 575 */ 576 AHOC, 577 /** 578 * Description:Scope of responsibility taken on by an organization for care of a 579 * patient as defined by the respective accreditation agency. 580 */ 581 ALTC, 582 /** 583 * Description:Scope of responsibility taken on by an organization for care of a 584 * patient as defined by the respective accreditation agency. 585 */ 586 AOSC, 587 /** 588 * Description:Scope of responsibility taken on by an organization for care of a 589 * patient as defined by the disease management certification agency. 590 */ 591 CACS, 592 /** 593 * Description:Scope of responsibility taken on by an organization for care of a 594 * patient as defined by the disease management certification agency. 595 */ 596 CAMI, 597 /** 598 * Description:Scope of responsibility taken on by an organization for care of a 599 * patient as defined by the disease management certification agency. 600 */ 601 CAST, 602 /** 603 * Description:Scope of responsibility taken on by an organization for care of a 604 * patient as defined by the disease management certification agency. 605 */ 606 CBAR, 607 /** 608 * Description:Scope of responsibility taken on by an organization for care of a 609 * patient as defined by the disease management certification agency. 610 */ 611 CCAD, 612 /** 613 * Description:Scope of responsibility taken on by an organization for care of a 614 * patient as defined by the disease management certification agency. 615 */ 616 CCAR, 617 /** 618 * Description:Scope of responsibility taken on by an organization for care of a 619 * patient as defined by the disease management certification agency. 620 */ 621 CDEP, 622 /** 623 * Description:Scope of responsibility taken on by an organization for care of a 624 * patient as defined by the disease management certification agency. 625 */ 626 CDGD, 627 /** 628 * Description:Scope of responsibility taken on by an organization for care of a 629 * patient as defined by the disease management certification agency. 630 */ 631 CDIA, 632 /** 633 * Description:Scope of responsibility taken on by an organization for care of a 634 * patient as defined by the disease management certification agency. 635 */ 636 CEPI, 637 /** 638 * Description:Scope of responsibility taken on by an organization for care of a 639 * patient as defined by the disease management certification agency. 640 */ 641 CFEL, 642 /** 643 * Description:Scope of responsibility taken on by an organization for care of a 644 * patient as defined by the disease management certification agency. 645 */ 646 CHFC, 647 /** 648 * Description:Scope of responsibility taken on by an organization for care of a 649 * patient as defined by the disease management certification agency. 650 */ 651 CHRO, 652 /** 653 * Description:Scope of responsibility taken on by an organization for care of a 654 * patient as defined by the disease management certification agency. 655 */ 656 CHYP, 657 /** 658 * Description:. 659 */ 660 CMIH, 661 /** 662 * Description:Scope of responsibility taken on by an organization for care of a 663 * patient as defined by the disease management certification agency. 664 */ 665 CMSC, 666 /** 667 * Description:Scope of responsibility taken on by an organization for care of a 668 * patient as defined by the disease management certification agency. 669 */ 670 COJR, 671 /** 672 * Description:Scope of responsibility taken on by an organization for care of a 673 * patient as defined by the disease management certification agency. 674 */ 675 CONC, 676 /** 677 * Description:Scope of responsibility taken on by an organization for care of a 678 * patient as defined by the disease management certification agency. 679 */ 680 COPD, 681 /** 682 * Description:Scope of responsibility taken on by an organization for care of a 683 * patient as defined by the disease management certification agency. 684 */ 685 CORT, 686 /** 687 * Description:Scope of responsibility taken on by an organization for care of a 688 * patient as defined by the disease management certification agency. 689 */ 690 CPAD, 691 /** 692 * Description:Scope of responsibility taken on by an organization for care of a 693 * patient as defined by the disease management certification agency. 694 */ 695 CPND, 696 /** 697 * Description:Scope of responsibility taken on by an organization for care of a 698 * patient as defined by the disease management certification agency. 699 */ 700 CPST, 701 /** 702 * Description:Scope of responsibility taken on by an organization for care of a 703 * patient as defined by the disease management certification agency. 704 */ 705 CSDM, 706 /** 707 * Description:Scope of responsibility taken on by an organization for care of a 708 * patient as defined by the disease management certification agency. 709 */ 710 CSIC, 711 /** 712 * Description:Scope of responsibility taken on by an organization for care of a 713 * patient as defined by the disease management certification agency. 714 */ 715 CSLD, 716 /** 717 * Description:Scope of responsibility taken on by an organization for care of a 718 * patient as defined by the disease management certification agency. 719 */ 720 CSPT, 721 /** 722 * Description:Scope of responsibility taken on by an organization for care of a 723 * patient as defined by the disease management certification agency. 724 */ 725 CTBU, 726 /** 727 * Description:Scope of responsibility taken on by an organization for care of a 728 * patient as defined by the disease management certification agency. 729 */ 730 CVDC, 731 /** 732 * Description:Scope of responsibility taken on by an organization for care of a 733 * patient as defined by the disease management certification agency. 734 */ 735 CWMA, 736 /** 737 * Description:Scope of responsibility taken on by an organization for care of a 738 * patient as defined by the disease management certification agency. 739 */ 740 CWOH, 741 /** 742 * Domain provides codes that qualify the ActEncounterClass (ENC) 743 */ 744 _ACTENCOUNTERCODE, 745 /** 746 * A comprehensive term for health care provided in a healthcare facility (e.g. 747 * a practitioneraTMs office, clinic setting, or hospital) on a nonresident 748 * basis. The term ambulatory usually implies that the patient has come to the 749 * location and is not assigned to a bed. Sometimes referred to as an outpatient 750 * encounter. 751 */ 752 AMB, 753 /** 754 * A patient encounter that takes place at a dedicated healthcare service 755 * delivery location where the patient receives immediate evaluation and 756 * treatment, provided until the patient can be discharged or responsibility for 757 * the patient's care is transferred elsewhere (for example, the patient could 758 * be admitted as an inpatient or transferred to another facility.) 759 */ 760 EMER, 761 /** 762 * A patient encounter that takes place both outside a dedicated service 763 * delivery location and outside a patient's residence. Example locations might 764 * include an accident site and at a supermarket. 765 */ 766 FLD, 767 /** 768 * Healthcare encounter that takes place in the residence of the patient or a 769 * designee 770 */ 771 HH, 772 /** 773 * A patient encounter where a patient is admitted by a hospital or equivalent 774 * facility, assigned to a location where patients generally stay at least 775 * overnight and provided with room, board, and continuous nursing service. 776 */ 777 IMP, 778 /** 779 * An acute inpatient encounter. 780 */ 781 ACUTE, 782 /** 783 * Any category of inpatient encounter except 'acute' 784 */ 785 NONAC, 786 /** 787 * An encounter where the patient usually will start in different encounter, 788 * such as one in the emergency department (EMER) but then transition to this 789 * type of encounter because they require a significant period of treatment and 790 * monitoring to determine whether or not their condition warrants an inpatient 791 * admission or discharge. In the majority of cases the decision about admission 792 * or discharge will occur within a time period determined by local, regional or 793 * national regulation, often between 24 and 48 hours. 794 */ 795 OBSENC, 796 /** 797 * A patient encounter where patient is scheduled or planned to receive service 798 * delivery in the future, and the patient is given a pre-admission account 799 * number. When the patient comes back for subsequent service, the pre-admission 800 * encounter is selected and is encapsulated into the service registration, and 801 * a new account number is generated. 802 * 803 * 804 * Usage Note: This is intended to be used in advance of encounter types such as 805 * ambulatory, inpatient encounter, virtual, etc. 806 */ 807 PRENC, 808 /** 809 * An encounter where the patient is admitted to a health care facility for a 810 * predetermined length of time, usually less than 24 hours. 811 */ 812 SS, 813 /** 814 * A patient encounter where the patient and the practitioner(s) are not in the 815 * same physical location. Examples include telephone conference, email 816 * exchange, robotic surgery, and televideo conference. 817 */ 818 VR, 819 /** 820 * General category of medical service provided to the patient during their 821 * encounter. 822 */ 823 _ACTMEDICALSERVICECODE, 824 /** 825 * Provision of Alternate Level of Care to a patient in an acute bed. Patient is 826 * waiting for placement in a long-term care facility and is unable to return 827 * home. 828 */ 829 ALC, 830 /** 831 * Provision of diagnosis and treatment of diseases and disorders affecting the 832 * heart 833 */ 834 CARD, 835 /** 836 * Provision of recurring care for chronic illness. 837 */ 838 CHR, 839 /** 840 * Provision of treatment for oral health and/or dental surgery. 841 */ 842 DNTL, 843 /** 844 * Provision of treatment for drug abuse. 845 */ 846 DRGRHB, 847 /** 848 * General care performed by a general practitioner or family doctor as a 849 * responsible provider for a patient. 850 */ 851 GENRL, 852 /** 853 * Provision of diagnostic and/or therapeutic treatment. 854 */ 855 MED, 856 /** 857 * Provision of care of women during pregnancy, childbirth and immediate 858 * postpartum period. Also known as Maternity. 859 */ 860 OBS, 861 /** 862 * Provision of treatment and/or diagnosis related to tumors and/or cancer. 863 */ 864 ONC, 865 /** 866 * Provision of care for patients who are living or dying from an advanced 867 * illness. 868 */ 869 PALL, 870 /** 871 * Provision of diagnosis and treatment of diseases and disorders affecting 872 * children. 873 */ 874 PED, 875 /** 876 * Pharmaceutical care performed by a pharmacist. 877 */ 878 PHAR, 879 /** 880 * Provision of treatment for physical injury. 881 */ 882 PHYRHB, 883 /** 884 * Provision of treatment of psychiatric disorder relating to mental illness. 885 */ 886 PSYCH, 887 /** 888 * Provision of surgical treatment. 889 */ 890 SURG, 891 /** 892 * Description: Coded types of attachments included to support a healthcare 893 * claim. 894 */ 895 _ACTCLAIMATTACHMENTCATEGORYCODE, 896 /** 897 * Description: Automobile Information Attachment 898 */ 899 AUTOATTCH, 900 /** 901 * Description: Document Attachment 902 */ 903 DOCUMENT, 904 /** 905 * Description: Health Record Attachment 906 */ 907 HEALTHREC, 908 /** 909 * Description: Image Attachment 910 */ 911 IMG, 912 /** 913 * Description: Lab Results Attachment 914 */ 915 LABRESULTS, 916 /** 917 * Description: Digital Model Attachment 918 */ 919 MODEL, 920 /** 921 * Description: Work Injury related additional Information Attachment 922 */ 923 WIATTCH, 924 /** 925 * Description: Digital X-Ray Attachment 926 */ 927 XRAY, 928 /** 929 * Definition: The type of consent directive, e.g., to consent or dissent to 930 * collect, access, or use in specific ways within an EHRS or for health 931 * information exchange; or to disclose health information for purposes such as 932 * research. 933 */ 934 _ACTCONSENTTYPE, 935 /** 936 * Definition: Consent to have healthcare information collected in an electronic 937 * health record. This entails that the information may be used in analysis, 938 * modified, updated. 939 */ 940 ICOL, 941 /** 942 * Definition: Consent to have collected healthcare information disclosed. 943 */ 944 IDSCL, 945 /** 946 * Definition: Consent to access healthcare information. 947 */ 948 INFA, 949 /** 950 * Definition: Consent to access or "read" only, which entails that the 951 * information is not to be copied, screen printed, saved, emailed, stored, 952 * re-disclosed or altered in any way. This level ensures that data which is 953 * masked or to which access is restricted will not be. 954 * 955 * 956 * Example: Opened and then emailed or screen printed for use outside of the 957 * consent directive purpose. 958 */ 959 INFAO, 960 /** 961 * Definition: Consent to access and save only, which entails that access to the 962 * saved copy will remain locked. 963 */ 964 INFASO, 965 /** 966 * Definition: Information re-disclosed without the patient's consent. 967 */ 968 IRDSCL, 969 /** 970 * Definition: Consent to have healthcare information in an electronic health 971 * record accessed for research purposes. 972 */ 973 RESEARCH, 974 /** 975 * Definition: Consent to have de-identified healthcare information in an 976 * electronic health record that is accessed for research purposes, but without 977 * consent to re-identify the information under any circumstance. 978 */ 979 RSDID, 980 /** 981 * Definition: Consent to have de-identified healthcare information in an 982 * electronic health record that is accessed for research purposes re-identified 983 * under specific circumstances outlined in the consent. 984 * 985 * 986 * Example:: Where there is a need to inform the subject of potential health 987 * issues. 988 */ 989 RSREID, 990 /** 991 * Constrains the ActCode to the domain of Container Registration 992 */ 993 _ACTCONTAINERREGISTRATIONCODE, 994 /** 995 * Used by one system to inform another that it has received a container. 996 */ 997 ID, 998 /** 999 * Used by one system to inform another that the container is in position for 1000 * specimen transfer (e.g., container removal from track, pipetting, etc.). 1001 */ 1002 IP, 1003 /** 1004 * Used by one system to inform another that the container has been released 1005 * from that system. 1006 */ 1007 L, 1008 /** 1009 * Used by one system to inform another that the container did not arrive at its 1010 * next expected location. 1011 */ 1012 M, 1013 /** 1014 * Used by one system to inform another that the specific container is being 1015 * processed by the equipment. It is useful as a response to a query about 1016 * Container Status, when the specific step of the process is not relevant. 1017 */ 1018 O, 1019 /** 1020 * Status is used by one system to inform another that the processing has been 1021 * completed, but the container has not been released from that system. 1022 */ 1023 R, 1024 /** 1025 * Used by one system to inform another that the container is no longer 1026 * available within the scope of the system (e.g., tube broken or discarded). 1027 */ 1028 X, 1029 /** 1030 * An observation form that determines parameters or attributes of an Act. 1031 * Examples are the settings of a ventilator machine as parameters of a 1032 * ventilator treatment act; the controls on dillution factors of a chemical 1033 * analyzer as a parameter of a laboratory observation act; the settings of a 1034 * physiologic measurement assembly (e.g., time skew) or the position of the 1035 * body while measuring blood pressure. 1036 * 1037 * Control variables are forms of observations because just as with clinical 1038 * observations, the Observation.code determines the parameter and the 1039 * Observation.value assigns the value. While control variables sometimes can be 1040 * observed (by noting the control settings or an actually measured feedback 1041 * loop) they are not primary observations, in the sense that a control variable 1042 * without a primary act is of no use (e.g., it makes no sense to record a blood 1043 * pressure position without recording a blood pressure, whereas it does make 1044 * sense to record a systolic blood pressure without a diastolic blood 1045 * pressure). 1046 */ 1047 _ACTCONTROLVARIABLE, 1048 /** 1049 * Specifies whether or not automatic repeat testing is to be initiated on 1050 * specimens. 1051 */ 1052 AUTO, 1053 /** 1054 * A baseline value for the measured test that is inherently contained in the 1055 * diluent. In the calculation of the actual result for the measured test, this 1056 * baseline value is normally considered. 1057 */ 1058 ENDC, 1059 /** 1060 * Specifies whether or not further testing may be automatically or manually 1061 * initiated on specimens. 1062 */ 1063 REFLEX, 1064 /** 1065 * Response to an insurance coverage eligibility query or authorization request. 1066 */ 1067 _ACTCOVERAGECONFIRMATIONCODE, 1068 /** 1069 * Indication of authorization for healthcare service(s) and/or product(s). If 1070 * authorization is approved, funds are set aside. 1071 */ 1072 _ACTCOVERAGEAUTHORIZATIONCONFIRMATIONCODE, 1073 /** 1074 * Authorization approved and funds have been set aside to pay for specified 1075 * healthcare service(s) and/or product(s) within defined criteria for the 1076 * authorization. 1077 */ 1078 AUTH, 1079 /** 1080 * Authorization for specified healthcare service(s) and/or product(s) denied. 1081 */ 1082 NAUTH, 1083 /** 1084 * Indication of eligibility coverage for healthcare service(s) and/or 1085 * product(s). 1086 */ 1087 _ACTCOVERAGEELIGIBILITYCONFIRMATIONCODE, 1088 /** 1089 * Insurance coverage is in effect for healthcare service(s) and/or product(s). 1090 */ 1091 ELG, 1092 /** 1093 * Insurance coverage is not in effect for healthcare service(s) and/or 1094 * product(s). May optionally include reasons for the ineligibility. 1095 */ 1096 NELG, 1097 /** 1098 * Criteria that are applicable to the authorized coverage. 1099 */ 1100 _ACTCOVERAGELIMITCODE, 1101 /** 1102 * Maximum amount paid or maximum number of services/products covered; or 1103 * maximum amount or number covered during a specified time period under the 1104 * policy or program. 1105 */ 1106 _ACTCOVERAGEQUANTITYLIMITCODE, 1107 /** 1108 * Codes representing the time period during which coverage is available; or 1109 * financial participation requirements are in effect. 1110 */ 1111 COVPRD, 1112 /** 1113 * Definition: Maximum amount paid by payer or covered party; or maximum number 1114 * of services or products covered under the policy or program during a covered 1115 * party's lifetime. 1116 */ 1117 LFEMX, 1118 /** 1119 * Maximum net amount that will be covered for the product or service specified. 1120 */ 1121 NETAMT, 1122 /** 1123 * Definition: Maximum amount paid by payer or covered party; or maximum number 1124 * of services/products covered under the policy or program by time period 1125 * specified by the effective time on the act. 1126 */ 1127 PRDMX, 1128 /** 1129 * Maximum unit price that will be covered for the authorized product or 1130 * service. 1131 */ 1132 UNITPRICE, 1133 /** 1134 * Maximum number of items that will be covered of the product or service 1135 * specified. 1136 */ 1137 UNITQTY, 1138 /** 1139 * Definition: Codes representing the maximum coverate or financial 1140 * participation requirements. 1141 */ 1142 COVMX, 1143 /** 1144 * Codes representing the types of covered parties that may receive covered 1145 * benefits under a policy or program. 1146 */ 1147 _ACTCOVEREDPARTYLIMITCODE, 1148 /** 1149 * Definition: Set of codes indicating the type of insurance policy or program 1150 * that pays for the cost of benefits provided to covered parties. 1151 */ 1152 _ACTCOVERAGETYPECODE, 1153 /** 1154 * Set of codes indicating the type of insurance policy or other source of funds 1155 * to cover healthcare costs. 1156 */ 1157 _ACTINSURANCEPOLICYCODE, 1158 /** 1159 * Private insurance policy that provides coverage in addition to other policies 1160 * (e.g. in addition to a Public Healthcare insurance policy). 1161 */ 1162 EHCPOL, 1163 /** 1164 * Insurance policy that provides for an allotment of funds replenished on a 1165 * periodic (e.g. annual) basis. The use of the funds under this policy is at 1166 * the discretion of the covered party. 1167 */ 1168 HSAPOL, 1169 /** 1170 * Insurance policy for injuries sustained in an automobile accident. Will also 1171 * typically covered non-named parties to the policy, such as pedestrians and 1172 * passengers. 1173 */ 1174 AUTOPOL, 1175 /** 1176 * Definition: An automobile insurance policy under which the insurance company 1177 * will cover the cost of damages to an automobile owned by the named insured 1178 * that are caused by accident or intentionally by another party. 1179 */ 1180 COL, 1181 /** 1182 * Definition: An automobile insurance policy under which the insurance company 1183 * will indemnify a loss for which another motorist is liable if that motorist 1184 * is unable to pay because he or she is uninsured. Coverage under the policy 1185 * applies to bodily injury damages only. Injuries to the covered party caused 1186 * by a hit-and-run driver are also covered. 1187 */ 1188 UNINSMOT, 1189 /** 1190 * Insurance policy funded by a public health system such as a provincial or 1191 * national health plan. Examples include BC MSP (British Columbia Medical 1192 * Services Plan) OHIP (Ontario Health Insurance Plan), NHS (National Health 1193 * Service). 1194 */ 1195 PUBLICPOL, 1196 /** 1197 * Definition: A public or government health program that administers and funds 1198 * coverage for dental care to assist program eligible who meet financial and 1199 * health status criteria. 1200 */ 1201 DENTPRG, 1202 /** 1203 * Definition: A public or government health program that administers and funds 1204 * coverage for health and social services to assist program eligible who meet 1205 * financial and health status criteria related to a particular disease. 1206 * 1207 * 1208 * Example: Reproductive health, sexually transmitted disease, and end renal 1209 * disease programs. 1210 */ 1211 DISEASEPRG, 1212 /** 1213 * Definition: A program that provides low-income, uninsured, and underserved 1214 * women access to timely, high-quality screening and diagnostic services, to 1215 * detect breast and cervical cancer at the earliest stages. 1216 * 1217 * 1218 * Example: To improve women's access to screening for breast and cervical 1219 * cancers, Congress passed the Breast and Cervical Cancer Mortality Prevention 1220 * Act of 1990, which guided CDC in creating the National Breast and Cervical 1221 * Cancer Early Detection Program (NBCCEDP), which provides access to critical 1222 * breast and cervical cancer screening services for underserved women in the 1223 * United States. An estimated 7 to 10% of U.S. women of screening age are 1224 * eligible to receive NBCCEDP services. Federal guidelines establish an 1225 * eligibility baseline to direct services to uninsured and underinsured women 1226 * at or below 250% of federal poverty level; ages 18 to 64 for cervical 1227 * screening; ages 40 to 64 for breast screening. 1228 */ 1229 CANPRG, 1230 /** 1231 * Definition: A public or government program that administers publicly funded 1232 * coverage of kidney dialysis and kidney transplant services. 1233 * 1234 * Example: In the U.S., the Medicare End-stage Renal Disease program (ESRD), 1235 * the National Kidney Foundation (NKF) American Kidney Fund (AKF) The Organ 1236 * Transplant Fund. 1237 */ 1238 ENDRENAL, 1239 /** 1240 * Definition: Government administered and funded HIV-AIDS program for 1241 * beneficiaries meeting financial and health status criteria. Administration, 1242 * funding levels, eligibility criteria, covered benefits, provider types, and 1243 * financial participation are typically set by a regulatory process. Payer 1244 * responsibilities for administering the program may be delegated to 1245 * contractors. 1246 * 1247 * 1248 * Example: In the U.S., the Ryan White program, which is administered by the 1249 * Health Resources and Services Administration. 1250 */ 1251 HIVAIDS, 1252 /** 1253 * mandatory health program 1254 */ 1255 MANDPOL, 1256 /** 1257 * Definition: Government administered and funded mental health program for 1258 * beneficiaries meeting financial and mental health status criteria. 1259 * Administration, funding levels, eligibility criteria, covered benefits, 1260 * provider types, and financial participation are typically set by a regulatory 1261 * process. Payer responsibilities for administering the program may be 1262 * delegated to contractors. 1263 * 1264 * 1265 * Example: In the U.S., states receive funding for substance use programs from 1266 * the Substance Abuse Mental Health Administration (SAMHSA). 1267 */ 1268 MENTPRG, 1269 /** 1270 * Definition: Government administered and funded program to support provision 1271 * of care to underserved populations through safety net clinics. 1272 * 1273 * 1274 * Example: In the U.S., safety net providers such as federally qualified health 1275 * centers (FQHC) receive funding under PHSA Section 330 grants administered by 1276 * the Health Resources and Services Administration. 1277 */ 1278 SAFNET, 1279 /** 1280 * Definition: Government administered and funded substance use program for 1281 * beneficiaries meeting financial, substance use behavior, and health status 1282 * criteria. Beneficiaries may be required to enroll as a result of legal 1283 * proceedings. Administration, funding levels, eligibility criteria, covered 1284 * benefits, provider types, and financial participation are typically set by a 1285 * regulatory process. Payer responsibilities for administering the program may 1286 * be delegated to contractors. 1287 * 1288 * 1289 * Example: In the U.S., states receive funding for substance use programs from 1290 * the Substance Abuse Mental Health Administration (SAMHSA). 1291 */ 1292 SUBPRG, 1293 /** 1294 * Definition: A government health program that provides coverage for health 1295 * services to persons meeting eligibility criteria such as income, location of 1296 * residence, access to other coverages, health condition, and age, the cost of 1297 * which is to some extent subsidized by public funds. 1298 */ 1299 SUBSIDIZ, 1300 /** 1301 * Definition: A government health program that provides coverage through 1302 * managed care contracts for health services to persons meeting eligibility 1303 * criteria such as income, location of residence, access to other coverages, 1304 * health condition, and age, the cost of which is to some extent subsidized by 1305 * public funds. 1306 * 1307 * 1308 * Discussion: The structure and business processes for underwriting and 1309 * administering a subsidized managed care program is further specified by the 1310 * Underwriter and Payer Role.class and Role.code. 1311 */ 1312 SUBSIDMC, 1313 /** 1314 * Definition: A government health program that provides coverage for health 1315 * services to persons meeting eligibility criteria for a supplemental health 1316 * policy or program such as income, location of residence, access to other 1317 * coverages, health condition, and age, the cost of which is to some extent 1318 * subsidized by public funds. 1319 * 1320 * 1321 * Example: Supplemental health coverage program may cover the cost of a health 1322 * program or policy financial participations, such as the copays and the 1323 * premiums, and may provide coverage for services in addition to those covered 1324 * under the supplemented health program or policy. In the U.S., Medicaid 1325 * programs may pay the premium for a covered party who is also covered under 1326 * the Medicare program or a private health policy. 1327 * 1328 * 1329 * Discussion: The structure and business processes for underwriting and 1330 * administering a subsidized supplemental retiree health program is further 1331 * specified by the Underwriter and Payer Role.class and Role.code. 1332 */ 1333 SUBSUPP, 1334 /** 1335 * Insurance policy for injuries sustained in the work place or in the course of 1336 * employment. 1337 */ 1338 WCBPOL, 1339 /** 1340 * Definition: Set of codes indicating the type of insurance policy. Insurance, 1341 * in law and economics, is a form of risk management primarily used to hedge 1342 * against the risk of potential financial loss. Insurance is defined as the 1343 * equitable transfer of the risk of a potential loss, from one entity to 1344 * another, in exchange for a premium and duty of care. A policy holder is an 1345 * individual or an organization enters into a contract with an underwriter 1346 * which stipulates that, in exchange for payment of a sum of money (a premium), 1347 * one or more covered parties (insureds) is guaranteed compensation for losses 1348 * resulting from certain perils under specified conditions. The underwriter 1349 * analyzes the risk of loss, makes a decision as to whether the risk is 1350 * insurable, and prices the premium accordingly. A policy provides benefits 1351 * that indemnify or cover the cost of a loss incurred by a covered party, and 1352 * may include coverage for services required to remediate a loss. An insurance 1353 * policy contains pertinent facts about the policy holder, the insurance 1354 * coverage, the covered parties, and the insurer. A policy may include 1355 * exemptions and provisions specifying the extent to which the indemnification 1356 * clause cannot be enforced for intentional tortious conduct of a covered 1357 * party, e.g., whether the covered parties are jointly or severably insured. 1358 * 1359 * 1360 * Discussion: In contrast to programs, an insurance policy has one or more 1361 * policy holders, who own the policy. The policy holder may be the covered 1362 * party, a relative of the covered party, a partnership, or a corporation, 1363 * e.g., an employer. A subscriber of a self-insured health insurance policy is 1364 * a policy holder. A subscriber of an employer sponsored health insurance 1365 * policy is holds a certificate of coverage, but is not a policy holder; the 1366 * policy holder is the employer. See CoveredRoleType. 1367 */ 1368 _ACTINSURANCETYPECODE, 1369 /** 1370 * Definition: Set of codes indicating the type of health insurance policy that 1371 * covers health services provided to covered parties. A health insurance policy 1372 * is a written contract for insurance between the insurance company and the 1373 * policyholder, and contains pertinent facts about the policy owner (the policy 1374 * holder), the health insurance coverage, the insured subscribers and 1375 * dependents, and the insurer. Health insurance is typically administered in 1376 * accordance with a plan, which specifies (1) the type of health services and 1377 * health conditions that will be covered under what circumstances (e.g., 1378 * exclusion of a pre-existing condition, service must be deemed medically 1379 * necessary; service must not be experimental; service must provided in 1380 * accordance with a protocol; drug must be on a formulary; service must be 1381 * prior authorized; or be a referral from a primary care provider); (2) the 1382 * type and affiliation of providers (e.g., only allopathic physicians, only in 1383 * network, only providers employed by an HMO); (3) financial participations 1384 * required of covered parties (e.g., co-pays, coinsurance, deductibles, 1385 * out-of-pocket); and (4) the manner in which services will be paid (e.g., 1386 * under indemnity or fee-for-service health plans, the covered party typically 1387 * pays out-of-pocket and then file a claim for reimbursement, while health 1388 * plans that have contractual relationships with providers, i.e., network 1389 * providers, typically do not allow the providers to bill the covered party for 1390 * the cost of the service until after filing a claim with the payer and 1391 * receiving reimbursement). 1392 */ 1393 _ACTHEALTHINSURANCETYPECODE, 1394 /** 1395 * Definition: A health insurance policy that that covers benefits for dental 1396 * services. 1397 */ 1398 DENTAL, 1399 /** 1400 * Definition: A health insurance policy that covers benefits for healthcare 1401 * services provided for named conditions under the policy, e.g., cancer, 1402 * diabetes, or HIV-AIDS. 1403 */ 1404 DISEASE, 1405 /** 1406 * Definition: A health insurance policy that covers benefits for prescription 1407 * drugs, pharmaceuticals, and supplies. 1408 */ 1409 DRUGPOL, 1410 /** 1411 * Definition: A health insurance policy that covers healthcare benefits by 1412 * protecting covered parties from medical expenses arising from health 1413 * conditions, sickness, or accidental injury as well as preventive care. Health 1414 * insurance policies explicitly exclude coverage for losses insured under a 1415 * disability policy, workers' compensation program, liability insurance 1416 * (including automobile insurance); or for medical expenses, coverage for 1417 * on-site medical clinics or for limited dental or vision benefits when these 1418 * are provided under a separate policy. 1419 * 1420 * 1421 * Discussion: Health insurance policies are offered by health insurance plans 1422 * that typically reimburse providers for covered services on a fee-for-service 1423 * basis, that is, a fee that is the allowable amount that a provider may 1424 * charge. This is in contrast to managed care plans, which typically prepay 1425 * providers a per-member/per-month amount or capitation as reimbursement for 1426 * all covered services rendered. Health insurance plans include indemnity and 1427 * healthcare services plans. 1428 */ 1429 HIP, 1430 /** 1431 * Definition: An insurance policy that covers benefits for long-term care 1432 * services people need when they no longer can care for themselves. This may be 1433 * due to an accident, disability, prolonged illness or the simple process of 1434 * aging. Long-term care services assist with activities of daily living 1435 * including: 1436 * 1437 * 1438 * 1439 * Help at home with day-to-day activities, such as cooking, cleaning, bathing 1440 * and dressing 1441 * 1442 * 1443 * 1444 * Care in the community, such as in an adult day care facility 1445 * 1446 * 1447 * 1448 * Supervised care provided in an assisted living facility 1449 * 1450 * 1451 * 1452 * Skilled care provided in a nursing home 1453 */ 1454 LTC, 1455 /** 1456 * Definition: Government mandated program providing coverage, disability 1457 * income, and vocational rehabilitation for injuries sustained in the work 1458 * place or in the course of employment. Employers may either self-fund the 1459 * program, purchase commercial coverage, or pay a premium to a government 1460 * entity that administers the program. Employees may be required to pay 1461 * premiums toward the cost of coverage as well. 1462 * 1463 * Managed care policies specifically exclude coverage for losses insured under 1464 * a disability policy, workers' compensation program, liability insurance 1465 * (including automobile insurance); or for medical expenses, coverage for 1466 * on-site medical clinics or for limited dental or vision benefits when these 1467 * are provided under a separate policy. 1468 * 1469 * 1470 * Discussion: Managed care policies are offered by managed care plans that 1471 * contract with selected providers or health care organizations to provide 1472 * comprehensive health care at a discount to covered parties and coordinate the 1473 * financing and delivery of health care. Managed care uses medical protocols 1474 * and procedures agreed on by the medical profession to be cost effective, also 1475 * known as medical practice guidelines. Providers are typically reimbursed for 1476 * covered services by a capitated amount on a per member per month basis that 1477 * may reflect difference in the health status and level of services anticipated 1478 * to be needed by the member. 1479 */ 1480 MCPOL, 1481 /** 1482 * Definition: A policy for a health plan that has features of both an HMO and a 1483 * FFS plan. Like an HMO, a POS plan encourages the use its HMO network to 1484 * maintain discounted fees with participating providers, but recognizes that 1485 * sometimes covered parties want to choose their own provider. The POS plan 1486 * allows a covered party to use providers who are not part of the HMO network 1487 * (non-participating providers). However, there is a greater cost associated 1488 * with choosing these non-network providers. A covered party will usually pay 1489 * deductibles and coinsurances that are substantially higher than the payments 1490 * when he or she uses a plan provider. Use of non-participating providers often 1491 * requires the covered party to pay the provider directly and then to file a 1492 * claim for reimbursement, like in an FFS plan. 1493 */ 1494 POS, 1495 /** 1496 * Definition: A policy for a health plan that provides coverage for health care 1497 * only through contracted or employed physicians and hospitals located in 1498 * particular geographic or service areas. HMOs emphasize prevention and early 1499 * detection of illness. Eligibility to enroll in an HMO is determined by where 1500 * a covered party lives or works. 1501 */ 1502 HMO, 1503 /** 1504 * Definition: A network-based, managed care plan that allows a covered party to 1505 * choose any health care provider. However, if care is received from a 1506 * "preferred" (participating in-network) provider, there are generally higher 1507 * benefit coverage and lower deductibles. 1508 */ 1509 PPO, 1510 /** 1511 * Definition: A health insurance policy that covers benefits for mental health 1512 * services and prescriptions. 1513 */ 1514 MENTPOL, 1515 /** 1516 * Definition: A health insurance policy that covers benefits for substance use 1517 * services. 1518 */ 1519 SUBPOL, 1520 /** 1521 * Definition: Set of codes for a policy that provides coverage for health care 1522 * expenses arising from vision services. 1523 * 1524 * A health insurance policy that covers benefits for vision care services, 1525 * prescriptions, and products. 1526 */ 1527 VISPOL, 1528 /** 1529 * Definition: An insurance policy that provides a regular payment to compensate 1530 * for income lost due to the covered party's inability to work because of 1531 * illness or injury. 1532 */ 1533 DIS, 1534 /** 1535 * Definition: An insurance policy under a benefit plan run by an employer or 1536 * employee organization for the purpose of providing benefits other than 1537 * pension-related to employees and their families. Typically provides 1538 * health-related benefits, benefits for disability, disease or unemployment, or 1539 * day care and scholarship benefits, among others. An employer sponsored health 1540 * policy includes coverage of health care expenses arising from sickness or 1541 * accidental injury, coverage for on-site medical clinics or for dental or 1542 * vision benefits, which are typically provided under a separate policy. 1543 * Coverage excludes health care expenses covered by accident or disability, 1544 * workers' compensation, liability or automobile insurance. 1545 */ 1546 EWB, 1547 /** 1548 * Definition: An insurance policy that covers qualified benefits under a 1549 * Flexible Benefit plan such as group medical insurance, long and short term 1550 * disability income insurance, group term life insurance for employees only up 1551 * to $50,000 face amount, specified disease coverage such as a cancer policy, 1552 * dental and/or vision insurance, hospital indemnity insurance, accidental 1553 * death and dismemberment insurance, a medical expense reimbursement plan and a 1554 * dependent care reimbursement plan. 1555 * 1556 * 1557 * Discussion: See UnderwriterRoleTypeCode flexible benefit plan which is 1558 * defined as a benefit plan that allows employees to choose from several life, 1559 * health, disability, dental, and other insurance plans according to their 1560 * individual needs. Also known as cafeteria plans. Authorized under Section 125 1561 * of the Revenue Act of 1978. 1562 */ 1563 FLEXP, 1564 /** 1565 * Definition: A policy under which the insurer agrees to pay a sum of money 1566 * upon the occurrence of the covered partys death. In return, the policyholder 1567 * agrees to pay a stipulated amount called a premium at regular intervals. Life 1568 * insurance indemnifies the beneficiary for the loss of the insurable interest 1569 * that a beneficiary has in the life of a covered party. For persons related by 1570 * blood, a substantial interest established through love and affection, and for 1571 * all other persons, a lawful and substantial economic interest in having the 1572 * life of the insured continue. An insurable interest is required when 1573 * purchasing life insurance on another person. Specific exclusions are often 1574 * written into the contract to limit the liability of the insurer; for example 1575 * claims resulting from suicide or relating to war, riot and civil commotion. 1576 * 1577 * 1578 * Discussion:A life insurance policy may be used by the covered party as a 1579 * source of health care coverage in the case of a viatical settlement, which is 1580 * the sale of a life insurance policy by the policy owner, before the policy 1581 * matures. Such a sale, at a price discounted from the face amount of the 1582 * policy but usually in excess of the premiums paid or current cash surrender 1583 * value, provides the seller an immediate cash settlement. Generally, viatical 1584 * settlements involve insured individuals with a life expectancy of less than 1585 * two years. In countries without state-subsidized healthcare and high 1586 * healthcare costs (e.g. United States), this is a practical way to pay 1587 * extremely high health insurance premiums that severely ill people face. Some 1588 * people are also familiar with life settlements, which are similar 1589 * transactions but involve insureds with longer life expectancies (two to 1590 * fifteen years). 1591 */ 1592 LIFE, 1593 /** 1594 * Definition: A policy that, after an initial premium or premiums, pays out a 1595 * sum at pre-determined intervals. 1596 * 1597 * For example, a policy holder may pay $10,000, and in return receive $150 each 1598 * month until he dies; or $1,000 for each of 14 years or death benefits if he 1599 * dies before the full term of the annuity has elapsed. 1600 */ 1601 ANNU, 1602 /** 1603 * Definition: Life insurance under which the benefit is payable only if the 1604 * insured dies during a specified period. If an insured dies during that 1605 * period, the beneficiary receives the death payments. If the insured survives, 1606 * the policy ends and the beneficiary receives nothing. 1607 */ 1608 TLIFE, 1609 /** 1610 * Definition: Life insurance under which the benefit is payable upon the 1611 * insuredaTMs death or diagnosis of a terminal illness. If an insured dies 1612 * during that period, the beneficiary receives the death payments. If the 1613 * insured survives, the policy ends and the beneficiary receives nothing 1614 */ 1615 ULIFE, 1616 /** 1617 * Definition: A type of insurance that covers damage to or loss of the 1618 * policyholderaTMs property by providing payments for damages to property 1619 * damage or the injury or death of living subjects. The terms "casualty" and 1620 * "liability" insurance are often used interchangeably. Both cover the 1621 * policyholder's legal liability for damages caused to other persons and/or 1622 * their property. 1623 */ 1624 PNC, 1625 /** 1626 * Definition: An agreement between two or more insurance companies by which the 1627 * risk of loss is proportioned. Thus the risk of loss is spread and a 1628 * disproportionately large loss under a single policy does not fall on one 1629 * insurance company. Acceptance by an insurer, called a reinsurer, of all or 1630 * part of the risk of loss of another insurance company. 1631 * 1632 * 1633 * Discussion: Reinsurance is a means by which an insurance company can protect 1634 * itself against the risk of losses with other insurance companies. Individuals 1635 * and corporations obtain insurance policies to provide protection for various 1636 * risks (hurricanes, earthquakes, lawsuits, collisions, sickness and death, 1637 * etc.). Reinsurers, in turn, provide insurance to insurance companies. 1638 * 1639 * For example, an HMO may purchase a reinsurance policy to protect itself from 1640 * losing too much money from one insured's particularly expensive health care 1641 * costs. An insurance company issuing an automobile liability policy, with a 1642 * limit of $100,000 per accident may reinsure its liability in excess of 1643 * $10,000. A fire insurance company which issues a large policy generally 1644 * reinsures a portion of the risk with one or several other companies. Also 1645 * called risk control insurance or stop-loss insurance. 1646 */ 1647 REI, 1648 /** 1649 * Definition: 1650 * 1651 * 1652 * 1653 * 1654 * A risk or part of a risk for which there is no normal insurance market 1655 * available. 1656 * 1657 * 1658 * 1659 * Insurance written by unauthorized insurance companies. Surplus lines 1660 * insurance is insurance placed with unauthorized insurance companies through 1661 * licensed surplus lines agents or brokers. 1662 */ 1663 SURPL, 1664 /** 1665 * Definition: A form of insurance protection that provides additional liability 1666 * coverage after the limits of your underlying policy are reached. An umbrella 1667 * liability policy also protects you (the insured) in many situations not 1668 * covered by the usual liability policies. 1669 */ 1670 UMBRL, 1671 /** 1672 * Definition: A set of codes used to indicate coverage under a program. A 1673 * program is an organized structure for administering and funding coverage of a 1674 * benefit package for covered parties meeting eligibility criteria, typically 1675 * related to employment, health, financial, and demographic status. Programs 1676 * are typically established or permitted by legislation with provisions for 1677 * ongoing government oversight. Regulations may mandate the structure of the 1678 * program, the manner in which it is funded and administered, covered benefits, 1679 * provider types, eligibility criteria and financial participation. A 1680 * government agency may be charged with implementing the program in accordance 1681 * to the regulation. Risk of loss under a program in most cases would not meet 1682 * what an underwriter would consider an insurable risk, i.e., the risk is not 1683 * random in nature, not financially measurable, and likely requires 1684 * subsidization with government funds. 1685 * 1686 * 1687 * Discussion: Programs do not have policy holders or subscribers. Program 1688 * eligibles are enrolled based on health status, statutory eligibility, 1689 * financial status, or age. Program eligibles who are covered parties under the 1690 * program may be referred to as members, beneficiaries, eligibles, or 1691 * recipients. Programs risk are underwritten by not for profit organizations 1692 * such as governmental entities, and the beneficiaries typically do not pay for 1693 * any or some portion of the cost of coverage. See CoveredPartyRoleType. 1694 */ 1695 _ACTPROGRAMTYPECODE, 1696 /** 1697 * Definition: A program that covers the cost of services provided directly to a 1698 * beneficiary who typically has no other source of coverage without charge. 1699 */ 1700 CHAR, 1701 /** 1702 * Definition: A program that covers the cost of services provided to crime 1703 * victims for injuries or losses related to the occurrence of a crime. 1704 */ 1705 CRIME, 1706 /** 1707 * Definition: An employee assistance program is run by an employer or employee 1708 * organization for the purpose of providing benefits and covering all or part 1709 * of the cost for employees to receive counseling, referrals, and advice in 1710 * dealing with stressful issues in their lives. These may include substance 1711 * abuse, bereavement, marital problems, weight issues, or general wellness 1712 * issues. The services are usually provided by a third-party, rather than the 1713 * company itself, and the company receives only summary statistical data from 1714 * the service provider. Employee's names and services received are kept 1715 * confidential. 1716 */ 1717 EAP, 1718 /** 1719 * Definition: A set of codes used to indicate a government program that is an 1720 * organized structure for administering and funding coverage of a benefit 1721 * package for covered parties meeting eligibility criteria, typically related 1722 * to employment, health and financial status. Government programs are 1723 * established or permitted by legislation with provisions for ongoing 1724 * government oversight. Regulation mandates the structure of the program, the 1725 * manner in which it is funded and administered, covered benefits, provider 1726 * types, eligibility criteria and financial participation. A government agency 1727 * is charged with implementing the program in accordance to the regulation 1728 * 1729 * 1730 * Example: Federal employee health benefit program in the U.S. 1731 */ 1732 GOVEMP, 1733 /** 1734 * Definition: A government program that provides health coverage to individuals 1735 * who are considered medically uninsurable or high risk, and who have been 1736 * denied health insurance due to a serious health condition. In certain cases, 1737 * it also applies to those who have been quoted very high premiums a" again, 1738 * due to a serious health condition. The pool charges premiums for coverage. 1739 * Because the pool covers high-risk people, it incurs a higher level of claims 1740 * than premiums can cover. The insurance industry pays into the pool to make up 1741 * the difference and help it remain viable. 1742 */ 1743 HIRISK, 1744 /** 1745 * Definition: Services provided directly and through contracted and operated 1746 * indigenous peoples health programs. 1747 * 1748 * 1749 * Example: Indian Health Service in the U.S. 1750 */ 1751 IND, 1752 /** 1753 * Definition: A government program that provides coverage for health services 1754 * to military personnel, retirees, and dependents. A covered party who is a 1755 * subscriber can choose from among Fee-for-Service (FFS) plans, and their 1756 * Preferred Provider Organizations (PPO), or Plans offering a Point of Service 1757 * (POS) Product, or Health Maintenance Organizations. 1758 * 1759 * 1760 * Example: In the U.S., TRICARE, CHAMPUS. 1761 */ 1762 MILITARY, 1763 /** 1764 * Definition: A government mandated program with specific eligibility 1765 * requirements based on premium contributions made during employment, length of 1766 * employment, age, and employment status, e.g., being retired, disabled, or a 1767 * dependent of a covered party under this program. Benefits typically include 1768 * ambulatory, inpatient, and long-term care, such as hospice care, home health 1769 * care and respite care. 1770 */ 1771 RETIRE, 1772 /** 1773 * Definition: A social service program funded by a public or governmental 1774 * entity. 1775 * 1776 * 1777 * Example: Programs providing habilitation, food, lodging, medicine, 1778 * transportation, equipment, devices, products, education, training, 1779 * counseling, alteration of living or work space, and other resources to 1780 * persons meeting eligibility criteria. 1781 */ 1782 SOCIAL, 1783 /** 1784 * Definition: Services provided directly and through contracted and operated 1785 * veteran health programs. 1786 */ 1787 VET, 1788 /** 1789 * Codes dealing with the management of Detected Issue observations 1790 */ 1791 _ACTDETECTEDISSUEMANAGEMENTCODE, 1792 /** 1793 * Codes dealing with the management of Detected Issue observations for the 1794 * administrative and patient administrative acts domains. 1795 */ 1796 _ACTADMINISTRATIVEDETECTEDISSUEMANAGEMENTCODE, 1797 /** 1798 * Authorization Issue Management Code 1799 */ 1800 _AUTHORIZATIONISSUEMANAGEMENTCODE, 1801 /** 1802 * Used to temporarily override normal authorization rules to gain access to 1803 * data in a case of emergency. Use of this override code will typically be 1804 * monitored, and a procedure to verify its proper use may be triggered when 1805 * used. 1806 */ 1807 EMAUTH, 1808 /** 1809 * Description: Indicates that the permissions have been externally verified and 1810 * the request should be processed. 1811 */ 1812 _21, 1813 /** 1814 * Confirmed drug therapy appropriate 1815 */ 1816 _1, 1817 /** 1818 * Consulted other supplier/pharmacy, therapy confirmed 1819 */ 1820 _19, 1821 /** 1822 * Assessed patient, therapy is appropriate 1823 */ 1824 _2, 1825 /** 1826 * Description: The patient has the appropriate indication or diagnosis for the 1827 * action to be taken. 1828 */ 1829 _22, 1830 /** 1831 * Description: It has been confirmed that the appropriate pre-requisite therapy 1832 * has been tried. 1833 */ 1834 _23, 1835 /** 1836 * Patient gave adequate explanation 1837 */ 1838 _3, 1839 /** 1840 * Consulted other supply source, therapy still appropriate 1841 */ 1842 _4, 1843 /** 1844 * Consulted prescriber, therapy confirmed 1845 */ 1846 _5, 1847 /** 1848 * Consulted prescriber and recommended change, prescriber declined 1849 */ 1850 _6, 1851 /** 1852 * Concurrent therapy triggering alert is no longer on-going or planned 1853 */ 1854 _7, 1855 /** 1856 * Confirmed supply action appropriate 1857 */ 1858 _14, 1859 /** 1860 * Patient's existing supply was lost/wasted 1861 */ 1862 _15, 1863 /** 1864 * Supply date is due to patient vacation 1865 */ 1866 _16, 1867 /** 1868 * Supply date is intended to carry patient over weekend 1869 */ 1870 _17, 1871 /** 1872 * Supply is intended for use during a leave of absence from an institution. 1873 */ 1874 _18, 1875 /** 1876 * Description: Supply is different than expected as an additional quantity has 1877 * been supplied in a separate dispense. 1878 */ 1879 _20, 1880 /** 1881 * Order is performed as issued, but other action taken to mitigate potential 1882 * adverse effects 1883 */ 1884 _8, 1885 /** 1886 * Provided education or training to the patient on appropriate therapy use 1887 */ 1888 _10, 1889 /** 1890 * Instituted an additional therapy to mitigate potential negative effects 1891 */ 1892 _11, 1893 /** 1894 * Suspended existing therapy that triggered interaction for the duration of 1895 * this therapy 1896 */ 1897 _12, 1898 /** 1899 * Aborted existing therapy that triggered interaction. 1900 */ 1901 _13, 1902 /** 1903 * Arranged to monitor patient for adverse effects 1904 */ 1905 _9, 1906 /** 1907 * Concepts that identify the type or nature of exposure interaction. Examples 1908 * include "household", "care giver", "intimate partner", "common space", 1909 * "common substance", etc. to further describe the nature of interaction. 1910 */ 1911 _ACTEXPOSURECODE, 1912 /** 1913 * Description: Exposure participants' interaction occurred in a child care 1914 * setting 1915 */ 1916 CHLDCARE, 1917 /** 1918 * Description: An interaction where the exposure participants traveled in/on 1919 * the same vehicle (not necessarily concurrently, e.g. both are passengers of 1920 * the same plane, but on different flights of that plane). 1921 */ 1922 CONVEYNC, 1923 /** 1924 * Description: Exposure participants' interaction occurred during the course of 1925 * health care delivery or in a health care delivery setting, but did not 1926 * involve the direct provision of care (e.g. a janitor cleaning a patient's 1927 * hospital room). 1928 */ 1929 HLTHCARE, 1930 /** 1931 * Description: Exposure interaction occurred in context of one providing care 1932 * for the other, i.e. a babysitter providing care for a child, a home-care aide 1933 * providing assistance to a paraplegic. 1934 */ 1935 HOMECARE, 1936 /** 1937 * Description: Exposure participants' interaction occurred when both were 1938 * patients being treated in the same (acute) health care delivery facility. 1939 */ 1940 HOSPPTNT, 1941 /** 1942 * Description: Exposure participants' interaction occurred when one visited the 1943 * other who was a patient being treated in a health care delivery facility. 1944 */ 1945 HOSPVSTR, 1946 /** 1947 * Description: Exposure interaction occurred in context of domestic 1948 * interaction, i.e. both participants reside in the same household. 1949 */ 1950 HOUSEHLD, 1951 /** 1952 * Description: Exposure participants' interaction occurred in the course of one 1953 * or both participants being incarcerated at a correctional facility 1954 */ 1955 INMATE, 1956 /** 1957 * Description: Exposure interaction was intimate, i.e. participants are 1958 * intimate companions (e.g. spouses, domestic partners). 1959 */ 1960 INTIMATE, 1961 /** 1962 * Description: Exposure participants' interaction occurred in the course of one 1963 * or both participants being resident at a long term care facility (second 1964 * participant may be a visitor, worker, resident or a physical place or object 1965 * within the facility). 1966 */ 1967 LTRMCARE, 1968 /** 1969 * Description: An interaction where the exposure participants were both present 1970 * in the same location/place/space. 1971 */ 1972 PLACE, 1973 /** 1974 * Description: Exposure participants' interaction occurred during the course of 1975 * health care delivery by a provider (e.g. a physician treating a patient in 1976 * her office). 1977 */ 1978 PTNTCARE, 1979 /** 1980 * Description: Exposure participants' interaction occurred in an academic 1981 * setting (e.g., participants are fellow students, or student and teacher). 1982 */ 1983 SCHOOL2, 1984 /** 1985 * Description: An interaction where the exposure participants are social 1986 * associates or members of the same extended family 1987 */ 1988 SOCIAL2, 1989 /** 1990 * Description: An interaction where the exposure participants shared or co-used 1991 * a common substance (e.g. drugs, needles, or common food item). 1992 */ 1993 SUBSTNCE, 1994 /** 1995 * Description: An interaction where the exposure participants traveled together 1996 * in/on the same vehicle/trip (e.g. concurrent co-passengers). 1997 */ 1998 TRAVINT, 1999 /** 2000 * Description: Exposure interaction occurred in a work setting, i.e. 2001 * participants are co-workers. 2002 */ 2003 WORK2, 2004 /** 2005 * ActFinancialTransactionCode 2006 */ 2007 _ACTFINANCIALTRANSACTIONCODE, 2008 /** 2009 * A type of transaction that represents a charge for a service or product. 2010 * Expressed in monetary terms. 2011 */ 2012 CHRG, 2013 /** 2014 * A type of transaction that represents a reversal of a previous charge for a 2015 * service or product. Expressed in monetary terms. It has the opposite effect 2016 * of a standard charge. 2017 */ 2018 REV, 2019 /** 2020 * Set of codes indicating the type of incident or accident. 2021 */ 2022 _ACTINCIDENTCODE, 2023 /** 2024 * Incident or accident as the result of a motor vehicle accident 2025 */ 2026 MVA, 2027 /** 2028 * Incident or accident is the result of a school place accident. 2029 */ 2030 SCHOOL, 2031 /** 2032 * Incident or accident is the result of a sporting accident. 2033 */ 2034 SPT, 2035 /** 2036 * Incident or accident is the result of a work place accident 2037 */ 2038 WPA, 2039 /** 2040 * Description: The type of health information to which the subject of the 2041 * information or the subject's delegate consents or dissents. 2042 */ 2043 _ACTINFORMATIONACCESSCODE, 2044 /** 2045 * Description: Provide consent to collect, use, disclose, or access adverse 2046 * drug reaction information for a patient. 2047 */ 2048 ACADR, 2049 /** 2050 * Description: Provide consent to collect, use, disclose, or access all 2051 * information for a patient. 2052 */ 2053 ACALL, 2054 /** 2055 * Description: Provide consent to collect, use, disclose, or access allergy 2056 * information for a patient. 2057 */ 2058 ACALLG, 2059 /** 2060 * Description: Provide consent to collect, use, disclose, or access 2061 * informational consent information for a patient. 2062 */ 2063 ACCONS, 2064 /** 2065 * Description: Provide consent to collect, use, disclose, or access 2066 * demographics information for a patient. 2067 */ 2068 ACDEMO, 2069 /** 2070 * Description: Provide consent to collect, use, disclose, or access diagnostic 2071 * imaging information for a patient. 2072 */ 2073 ACDI, 2074 /** 2075 * Description: Provide consent to collect, use, disclose, or access 2076 * immunization information for a patient. 2077 */ 2078 ACIMMUN, 2079 /** 2080 * Description: Provide consent to collect, use, disclose, or access lab test 2081 * result information for a patient. 2082 */ 2083 ACLAB, 2084 /** 2085 * Description: Provide consent to collect, use, disclose, or access medical 2086 * condition information for a patient. 2087 */ 2088 ACMED, 2089 /** 2090 * Definition: Provide consent to view or access medical condition information 2091 * for a patient. 2092 */ 2093 ACMEDC, 2094 /** 2095 * Description:Provide consent to collect, use, disclose, or access mental 2096 * health information for a patient. 2097 */ 2098 ACMEN, 2099 /** 2100 * Description: Provide consent to collect, use, disclose, or access common 2101 * observation information for a patient. 2102 */ 2103 ACOBS, 2104 /** 2105 * Description: Provide consent to collect, use, disclose, or access coverage 2106 * policy or program for a patient. 2107 */ 2108 ACPOLPRG, 2109 /** 2110 * Description: Provide consent to collect, use, disclose, or access provider 2111 * information for a patient. 2112 */ 2113 ACPROV, 2114 /** 2115 * Description: Provide consent to collect, use, disclose, or access 2116 * professional service information for a patient. 2117 */ 2118 ACPSERV, 2119 /** 2120 * Description:Provide consent to collect, use, disclose, or access substance 2121 * abuse information for a patient. 2122 */ 2123 ACSUBSTAB, 2124 /** 2125 * Concepts conveying the context in which authorization given under 2126 * jurisdictional law, by organizational policy, or by a patient consent 2127 * directive permits the collection, access, use or disclosure of specified 2128 * patient health information. 2129 */ 2130 _ACTINFORMATIONACCESSCONTEXTCODE, 2131 /** 2132 * Authorization to collect, access, use, or disclose specified patient health 2133 * information in accordance with jurisdictional law, organizational policy, or 2134 * a patient's consent directive, which may be implied, deemed, opt-in, opt-out, 2135 * or explicit. 2136 */ 2137 INFAUT, 2138 /** 2139 * Authorization to collect, access, use, or disclose specified patient health 2140 * information as explicitly consented to by the subject of the information or 2141 * the subject's representative. 2142 */ 2143 INFCON, 2144 /** 2145 * Authorization to collect, access, use, or disclose specified patient health 2146 * information in accordance with judicial system protocol, such as in the case 2147 * of a subpoena or court order. 2148 */ 2149 INFCRT, 2150 /** 2151 * Authorization to collect, access, use, or disclose specified patient health 2152 * information where deemed necessary to avert potential danger to other persons 2153 * in accordance with jurisdictional law, organizational policy, or standards of 2154 * practice. For example, disclosure about a person threatening violence. 2155 */ 2156 INFDNG, 2157 /** 2158 * Authorization to collect, access, use, or disclose specified patient health 2159 * information in accordance with emergency information transfer protocol 2160 * dictated by jurisdictional law, organization policy, or standards of 2161 * practice. For example, sharing of health information during disaster 2162 * response. 2163 */ 2164 INFEMER, 2165 /** 2166 * Authorization to collect, access, use, or disclose specified patient health 2167 * information necessary to avert potential public welfare risk in accordance 2168 * with jurisdictional law, organizational policy, or standards of practice. For 2169 * example, reporting that a person is a victim of abuse or demonstrating 2170 * suicidal tendencies. 2171 */ 2172 INFPWR, 2173 /** 2174 * Authorization to collect, access, use, or disclose specified patient health 2175 * information for public health, welfare, and safety purposes in accordance 2176 * with jurisdictional law, organizational policy, or standards of practice. For 2177 * example, public health reporting of notifiable conditions. 2178 */ 2179 INFREG, 2180 /** 2181 * Definition:Indicates the set of information types which may be manipulated or 2182 * referenced, such as for recommending access restrictions. 2183 */ 2184 _ACTINFORMATIONCATEGORYCODE, 2185 /** 2186 * Description: All patient information. 2187 */ 2188 ALLCAT, 2189 /** 2190 * Definition:All information pertaining to a patient's allergy and intolerance 2191 * records. 2192 */ 2193 ALLGCAT, 2194 /** 2195 * Description: All information pertaining to a patient's adverse drug 2196 * reactions. 2197 */ 2198 ARCAT, 2199 /** 2200 * Definition:All information pertaining to a patient's common observation 2201 * records (height, weight, blood pressure, temperature, etc.). 2202 */ 2203 COBSCAT, 2204 /** 2205 * Definition:All information pertaining to a patient's demographics (such as 2206 * name, date of birth, gender, address, etc). 2207 */ 2208 DEMOCAT, 2209 /** 2210 * Definition:All information pertaining to a patient's diagnostic image records 2211 * (orders & results). 2212 */ 2213 DICAT, 2214 /** 2215 * Definition:All information pertaining to a patient's vaccination records. 2216 */ 2217 IMMUCAT, 2218 /** 2219 * Description: All information pertaining to a patient's lab test records 2220 * (orders & results) 2221 */ 2222 LABCAT, 2223 /** 2224 * Definition:All information pertaining to a patient's medical condition 2225 * records. 2226 */ 2227 MEDCCAT, 2228 /** 2229 * Description: All information pertaining to a patient's mental health records. 2230 */ 2231 MENCAT, 2232 /** 2233 * Definition:All information pertaining to a patient's professional service 2234 * records (such as smoking cessation, counseling, medication review, mental 2235 * health). 2236 */ 2237 PSVCCAT, 2238 /** 2239 * Definition:All information pertaining to a patient's medication records 2240 * (orders, dispenses and other active medications). 2241 */ 2242 RXCAT, 2243 /** 2244 * Type of invoice element that is used to assist in describing an Invoice that 2245 * is either submitted for adjudication or for which is returned on adjudication 2246 * results. 2247 */ 2248 _ACTINVOICEELEMENTCODE, 2249 /** 2250 * Codes representing a grouping of invoice elements (totals, sub-totals), 2251 * reported through a Payment Advice or a Statement of Financial Activity 2252 * (SOFA). The code can represent summaries by day, location, payee and other 2253 * cost elements such as bonus, retroactive adjustment and transaction fees. 2254 */ 2255 _ACTINVOICEADJUDICATIONPAYMENTCODE, 2256 /** 2257 * Codes representing adjustments to a Payment Advice such as retroactive, 2258 * clawback, garnishee, etc. 2259 */ 2260 _ACTINVOICEADJUDICATIONPAYMENTGROUPCODE, 2261 /** 2262 * Payment initiated by the payor as the result of adjudicating a submitted 2263 * invoice that arrived to the payor from an electronic source that did not 2264 * provide a conformant set of HL7 messages (e.g. web claim submission). 2265 */ 2266 ALEC, 2267 /** 2268 * Bonus payments based on performance, volume, etc. as agreed to by the payor. 2269 */ 2270 BONUS, 2271 /** 2272 * An amount still owing to the payor but the payment is 0$ and this cannot be 2273 * settled until a future payment is made. 2274 */ 2275 CFWD, 2276 /** 2277 * Fees deducted on behalf of a payee for tuition and continuing education. 2278 */ 2279 EDU, 2280 /** 2281 * Fees deducted on behalf of a payee for charges based on a shorter payment 2282 * frequency (i.e. next day versus biweekly payments. 2283 */ 2284 EPYMT, 2285 /** 2286 * Fees deducted on behalf of a payee for charges based on a per-transaction or 2287 * time-period (e.g. monthly) fee. 2288 */ 2289 GARN, 2290 /** 2291 * Payment is based on a payment intent for a previously submitted Invoice, 2292 * based on formal adjudication results.. 2293 */ 2294 INVOICE, 2295 /** 2296 * Payment initiated by the payor as the result of adjudicating a paper 2297 * (original, may have been faxed) invoice. 2298 */ 2299 PINV, 2300 /** 2301 * An amount that was owed to the payor as indicated, by a carry forward 2302 * adjusment, in a previous payment advice 2303 */ 2304 PPRD, 2305 /** 2306 * Professional association fee that is collected by the payor from the 2307 * practitioner/provider on behalf of the association 2308 */ 2309 PROA, 2310 /** 2311 * Retroactive adjustment such as fee rate adjustment due to contract 2312 * negotiations. 2313 */ 2314 RECOV, 2315 /** 2316 * Bonus payments based on performance, volume, etc. as agreed to by the payor. 2317 */ 2318 RETRO, 2319 /** 2320 * Fees deducted on behalf of a payee for charges based on a per-transaction or 2321 * time-period (e.g. monthly) fee. 2322 */ 2323 TRAN, 2324 /** 2325 * Codes representing a grouping of invoice elements (totals, sub-totals), 2326 * reported through a Payment Advice or a Statement of Financial Activity 2327 * (SOFA). The code can represent summaries by day, location, payee, etc. 2328 */ 2329 _ACTINVOICEADJUDICATIONPAYMENTSUMMARYCODE, 2330 /** 2331 * Transaction counts and value totals by invoice type (e.g. RXDINV - Pharmacy 2332 * Dispense) 2333 */ 2334 INVTYPE, 2335 /** 2336 * Transaction counts and value totals by each instance of an invoice payee. 2337 */ 2338 PAYEE, 2339 /** 2340 * Transaction counts and value totals by each instance of an invoice payor. 2341 */ 2342 PAYOR, 2343 /** 2344 * Transaction counts and value totals by each instance of a messaging 2345 * application on a single processor. It is a registered identifier known to the 2346 * receivers. 2347 */ 2348 SENDAPP, 2349 /** 2350 * Codes representing a service or product that is being invoiced (billed). The 2351 * code can represent such concepts as "office visit", "drug X", "wheelchair" 2352 * and other billable items such as taxes, service charges and discounts. 2353 */ 2354 _ACTINVOICEDETAILCODE, 2355 /** 2356 * An identifying data string for healthcare products. 2357 */ 2358 _ACTINVOICEDETAILCLINICALPRODUCTCODE, 2359 /** 2360 * Description:United Nations Standard Products and Services Classification, 2361 * managed by Uniform Code Council (UCC): www.unspsc.org 2362 */ 2363 UNSPSC, 2364 /** 2365 * An identifying data string for A substance used as a medication or in the 2366 * preparation of medication. 2367 */ 2368 _ACTINVOICEDETAILDRUGPRODUCTCODE, 2369 /** 2370 * Description:Global Trade Item Number is an identifier for trade items 2371 * developed by GS1 (comprising the former EAN International and Uniform Code 2372 * Council). 2373 */ 2374 GTIN, 2375 /** 2376 * Description:Universal Product Code is one of a wide variety of bar code 2377 * languages widely used in the United States and Canada for items in stores. 2378 */ 2379 UPC, 2380 /** 2381 * The detail item codes to identify charges or changes to the total billing of 2382 * a claim due to insurance rules and payments. 2383 */ 2384 _ACTINVOICEDETAILGENERICCODE, 2385 /** 2386 * The billable item codes to identify adjudicator specified components to the 2387 * total billing of a claim. 2388 */ 2389 _ACTINVOICEDETAILGENERICADJUDICATORCODE, 2390 /** 2391 * That portion of the eligible charges which a covered party must pay for each 2392 * service and/or product. It is a percentage of the eligible amount for the 2393 * service/product that is typically charged after the covered party has met the 2394 * policy deductible. This amount represents the covered party's coinsurance 2395 * that is applied to a particular adjudication result. It is expressed as a 2396 * negative dollar amount in adjudication results. 2397 */ 2398 COIN, 2399 /** 2400 * That portion of the eligible charges which a covered party must pay for each 2401 * service and/or product. It is a defined amount per service/product of the 2402 * eligible amount for the service/product. This amount represents the covered 2403 * party's copayment that is applied to a particular adjudication result. It is 2404 * expressed as a negative dollar amount in adjudication results. 2405 */ 2406 COPAYMENT, 2407 /** 2408 * That portion of the eligible charges which a covered party must pay in a 2409 * particular period (e.g. annual) before the benefits are payable by the 2410 * adjudicator. This amount represents the covered party's deductible that is 2411 * applied to a particular adjudication result. It is expressed as a negative 2412 * dollar amount in adjudication results. 2413 */ 2414 DEDUCTIBLE, 2415 /** 2416 * The guarantor, who may be the patient, pays the entire charge for a service. 2417 * Reasons for such action may include: there is no insurance coverage for the 2418 * service (e.g. cosmetic surgery); the patient wishes to self-pay for the 2419 * service; or the insurer denies payment for the service due to contractual 2420 * provisions such as the need for prior authorization. 2421 */ 2422 PAY, 2423 /** 2424 * That total amount of the eligible charges which a covered party must 2425 * periodically pay for services and/or products prior to the Medicaid program 2426 * providing any coverage. This amount represents the covered party's spend down 2427 * that is applied to a particular adjudication result. It is expressed as a 2428 * negative dollar amount in adjudication results 2429 */ 2430 SPEND, 2431 /** 2432 * The covered party pays a percentage of the cost of covered services. 2433 */ 2434 COINS, 2435 /** 2436 * The billable item codes to identify modifications to a billable item charge. 2437 * As for example after hours increase in the office visit fee. 2438 */ 2439 _ACTINVOICEDETAILGENERICMODIFIERCODE, 2440 /** 2441 * Premium paid on service fees in compensation for practicing outside of normal 2442 * working hours. 2443 */ 2444 AFTHRS, 2445 /** 2446 * Premium paid on service fees in compensation for practicing in a remote 2447 * location. 2448 */ 2449 ISOL, 2450 /** 2451 * Premium paid on service fees in compensation for practicing at a location 2452 * other than normal working location. 2453 */ 2454 OOO, 2455 /** 2456 * The billable item codes to identify provider supplied charges or changes to 2457 * the total billing of a claim. 2458 */ 2459 _ACTINVOICEDETAILGENERICPROVIDERCODE, 2460 /** 2461 * A charge to compensate the provider when a patient cancels an appointment 2462 * with insufficient time for the provider to make another appointment with 2463 * another patient. 2464 */ 2465 CANCAPT, 2466 /** 2467 * A reduction in the amount charged as a percentage of the amount. For example 2468 * a 5% discount for volume purchase. 2469 */ 2470 DSC, 2471 /** 2472 * A premium on a service fee is requested because, due to extenuating 2473 * circumstances, the service took an extraordinary amount of time or supplies. 2474 */ 2475 ESA, 2476 /** 2477 * Under agreement between the parties (payor and provider), a guaranteed level 2478 * of income is established for the provider over a specific, pre-determined 2479 * period of time. The normal course of business for the provider is submission 2480 * of fee-for-service claims. Should the fee-for-service income during the 2481 * specified period of time be less than the agreed to amount, a top-up amount 2482 * is paid to the provider equal to the difference between the fee-for-service 2483 * total and the guaranteed income amount for that period of time. The details 2484 * of the agreement may specify (or not) a requirement for repayment to the 2485 * payor in the event that the fee-for-service income exceeds the guaranteed 2486 * amount. 2487 */ 2488 FFSTOP, 2489 /** 2490 * Anticipated or actual final fee associated with treating a patient. 2491 */ 2492 FNLFEE, 2493 /** 2494 * Anticipated or actual initial fee associated with treating a patient. 2495 */ 2496 FRSTFEE, 2497 /** 2498 * An increase in the amount charged as a percentage of the amount. For example, 2499 * 12% markup on product cost. 2500 */ 2501 MARKUP, 2502 /** 2503 * A charge to compensate the provider when a patient does not show for an 2504 * appointment. 2505 */ 2506 MISSAPT, 2507 /** 2508 * Anticipated or actual periodic fee associated with treating a patient. For 2509 * example, expected billing cycle such as monthly, quarterly. The actual period 2510 * (e.g. monthly, quarterly) is specified in the unit quantity of the Invoice 2511 * Element. 2512 */ 2513 PERFEE, 2514 /** 2515 * The amount for a performance bonus that is being requested from a payor for 2516 * the performance of certain services (childhood immunizations, influenza 2517 * immunizations, mammograms, pap smears) on a sliding scale. That is, for 90% 2518 * of childhood immunizations to a maximum of $2200/yr. An invoice is created at 2519 * the end of the service period (one year) and a code is submitted indicating 2520 * the percentage achieved and the dollar amount claimed. 2521 */ 2522 PERMBNS, 2523 /** 2524 * A charge is requested because the patient failed to pick up the item and it 2525 * took an amount of time to return it to stock for future use. 2526 */ 2527 RESTOCK, 2528 /** 2529 * A charge to cover the cost of travel time and/or cost in conjuction with 2530 * providing a service or product. It may be charged per kilometer or per hour 2531 * based on the effective agreement. 2532 */ 2533 TRAVEL, 2534 /** 2535 * Premium paid on service fees in compensation for providing an expedited 2536 * response to an urgent situation. 2537 */ 2538 URGENT, 2539 /** 2540 * The billable item codes to identify modifications to a billable item charge 2541 * by a tax factor applied to the amount. As for example 7% provincial sales 2542 * tax. 2543 */ 2544 _ACTINVOICEDETAILTAXCODE, 2545 /** 2546 * Federal tax on transactions such as the Goods and Services Tax (GST) 2547 */ 2548 FST, 2549 /** 2550 * Joint Federal/Provincial Sales Tax 2551 */ 2552 HST, 2553 /** 2554 * Tax levied by the provincial or state jurisdiction such as Provincial Sales 2555 * Tax 2556 */ 2557 PST, 2558 /** 2559 * An identifying data string for medical facility accommodations. 2560 */ 2561 _ACTINVOICEDETAILPREFERREDACCOMMODATIONCODE, 2562 /** 2563 * Accommodation type. In Intent mood, represents the accommodation type 2564 * requested. In Event mood, represents accommodation assigned/used. In 2565 * Definition mood, represents the available accommodation type. 2566 */ 2567 _ACTENCOUNTERACCOMMODATIONCODE, 2568 /** 2569 * Description:Accommodation type. In Intent mood, represents the accommodation 2570 * type requested. In Event mood, represents accommodation assigned/used. In 2571 * Definition mood, represents the available accommodation type. 2572 */ 2573 _HL7ACCOMMODATIONCODE, 2574 /** 2575 * Accommodations used in the care of diseases that are transmitted through 2576 * casual contact or respiratory transmission. 2577 */ 2578 I, 2579 /** 2580 * Accommodations in which there is only 1 bed. 2581 */ 2582 P, 2583 /** 2584 * Uniquely designed and elegantly decorated accommodations with many amenities 2585 * available for an additional charge. 2586 */ 2587 S, 2588 /** 2589 * Accommodations in which there are 2 beds. 2590 */ 2591 SP, 2592 /** 2593 * Accommodations in which there are 3 or more beds. 2594 */ 2595 W, 2596 /** 2597 * An identifying data string for healthcare procedures. 2598 */ 2599 _ACTINVOICEDETAILCLINICALSERVICECODE, 2600 /** 2601 * Type of invoice element that is used to assist in describing an Invoice that 2602 * is either submitted for adjudication or for which is returned on adjudication 2603 * results. 2604 * 2605 * Invoice elements of this type signify a grouping of one or more children 2606 * (detail) invoice elements. They do not have intrinsic costing associated with 2607 * them, but merely reflect the sum of all costing for it's immediate children 2608 * invoice elements. 2609 */ 2610 _ACTINVOICEGROUPCODE, 2611 /** 2612 * Type of invoice element that is used to assist in describing an Invoice that 2613 * is either submitted for adjudication or for which is returned on adjudication 2614 * results. 2615 * 2616 * Invoice elements of this type signify a grouping of one or more children 2617 * (detail) invoice elements. They do not have intrinsic costing associated with 2618 * them, but merely reflect the sum of all costing for it's immediate children 2619 * invoice elements. 2620 * 2621 * The domain is only specified for an intermediate invoice element group 2622 * (non-root or non-top level) for an Invoice. 2623 */ 2624 _ACTINVOICEINTERGROUPCODE, 2625 /** 2626 * A grouping of invoice element groups and details including the ones 2627 * specifying the compound ingredients being invoiced. It may also contain 2628 * generic detail items such as markup. 2629 */ 2630 CPNDDRGING, 2631 /** 2632 * A grouping of invoice element details including the one specifying an 2633 * ingredient drug being invoiced. It may also contain generic detail items such 2634 * as tax or markup. 2635 */ 2636 CPNDINDING, 2637 /** 2638 * A grouping of invoice element groups and details including the ones 2639 * specifying the compound supplies being invoiced. It may also contain generic 2640 * detail items such as markup. 2641 */ 2642 CPNDSUPING, 2643 /** 2644 * A grouping of invoice element details including the one specifying the drug 2645 * being invoiced. It may also contain generic detail items such as markup. 2646 */ 2647 DRUGING, 2648 /** 2649 * A grouping of invoice element details including the ones specifying the frame 2650 * fee and the frame dispensing cost that are being invoiced. 2651 */ 2652 FRAMEING, 2653 /** 2654 * A grouping of invoice element details including the ones specifying the lens 2655 * fee and the lens dispensing cost that are being invoiced. 2656 */ 2657 LENSING, 2658 /** 2659 * A grouping of invoice element details including the one specifying the 2660 * product (good or supply) being invoiced. It may also contain generic detail 2661 * items such as tax or discount. 2662 */ 2663 PRDING, 2664 /** 2665 * Type of invoice element that is used to assist in describing an Invoice that 2666 * is either submitted for adjudication or for which is returned on adjudication 2667 * results. 2668 * 2669 * Invoice elements of this type signify a grouping of one or more children 2670 * (detail) invoice elements. They do not have intrinsic costing associated with 2671 * them, but merely reflect the sum of all costing for it's immediate children 2672 * invoice elements. 2673 * 2674 * Codes from this domain reflect the type of Invoice such as Pharmacy Dispense, 2675 * Clinical Service and Clinical Product. The domain is only specified for the 2676 * root (top level) invoice element group for an Invoice. 2677 */ 2678 _ACTINVOICEROOTGROUPCODE, 2679 /** 2680 * Clinical product invoice where the Invoice Grouping contains one or more 2681 * billable item and is supported by clinical product(s). 2682 * 2683 * For example, a crutch or a wheelchair. 2684 */ 2685 CPINV, 2686 /** 2687 * Clinical Services Invoice which can be used to describe a single service, 2688 * multiple services or repeated services. 2689 * 2690 * [1] Single Clinical services invoice where the Invoice Grouping contains one 2691 * billable item and is supported by one clinical service. 2692 * 2693 * For example, a single service for an office visit or simple clinical 2694 * procedure (e.g. knee mobilization). 2695 * 2696 * [2] Multiple Clinical services invoice where the Invoice Grouping contains 2697 * more than one billable item, supported by one or more clinical services. The 2698 * services can be distinct and over multiple dates, but for the same patient. 2699 * This type of invoice includes a series of treatments which must be 2700 * adjudicated together. 2701 * 2702 * For example, an adjustment and ultrasound for a chiropractic session where 2703 * fees are associated for each of the services and adjudicated (invoiced) 2704 * together. 2705 * 2706 * [3] Repeated Clinical services invoice where the Invoice Grouping contains 2707 * one or more billable item, supported by the same clinical service repeated 2708 * over a period of time. 2709 * 2710 * For example, the same Chiropractic adjustment (service or treatment) 2711 * delivered on 3 separate occasions over a period of time at the discretion of 2712 * the provider (e.g. month). 2713 */ 2714 CSINV, 2715 /** 2716 * A clinical Invoice Grouping consisting of one or more services and one or 2717 * more product. Billing for these service(s) and product(s) are supported by 2718 * multiple clinical billable events (acts). 2719 * 2720 * All items in the Invoice Grouping must be adjudicated together to be 2721 * acceptable to the Adjudicator. 2722 * 2723 * For example , a brace (product) invoiced together with the fitting (service). 2724 */ 2725 CSPINV, 2726 /** 2727 * Invoice Grouping without clinical justification. These will not require 2728 * identification of participants and associations from a clinical context such 2729 * as patient and provider. 2730 * 2731 * Examples are interest charges and mileage. 2732 */ 2733 FININV, 2734 /** 2735 * A clinical Invoice Grouping consisting of one or more oral health services. 2736 * Billing for these service(s) are supported by multiple clinical billable 2737 * events (acts). 2738 * 2739 * All items in the Invoice Grouping must be adjudicated together to be 2740 * acceptable to the Adjudicator. 2741 */ 2742 OHSINV, 2743 /** 2744 * HealthCare facility preferred accommodation invoice. 2745 */ 2746 PAINV, 2747 /** 2748 * Pharmacy dispense invoice for a compound. 2749 */ 2750 RXCINV, 2751 /** 2752 * Pharmacy dispense invoice not involving a compound 2753 */ 2754 RXDINV, 2755 /** 2756 * Clinical services invoice where the Invoice Group contains one billable item 2757 * for multiple clinical services in one or more sessions. 2758 */ 2759 SBFINV, 2760 /** 2761 * Vision dispense invoice for up to 2 lens (left and right), frame and optional 2762 * discount. Eye exams are invoiced as a clinical service invoice. 2763 */ 2764 VRXINV, 2765 /** 2766 * Identifies the different types of summary information that can be reported by 2767 * queries dealing with Statement of Financial Activity (SOFA). The summary 2768 * information is generally used to help resolve balance discrepancies between 2769 * providers and payors. 2770 */ 2771 _ACTINVOICEELEMENTSUMMARYCODE, 2772 /** 2773 * Total counts and total net amounts adjudicated for all Invoice Groupings that 2774 * were adjudicated within a time period based on the adjudication date of the 2775 * Invoice Grouping. 2776 */ 2777 _INVOICEELEMENTADJUDICATED, 2778 /** 2779 * Identifies the total net amount of all Invoice Groupings that were 2780 * adjudicated as payable prior to the specified time period (based on 2781 * adjudication date), subsequently cancelled in the specified period and 2782 * submitted electronically. 2783 */ 2784 ADNFPPELAT, 2785 /** 2786 * Identifies the total number of all Invoice Groupings that were adjudicated as 2787 * payable prior to the specified time period (based on adjudication date), 2788 * subsequently cancelled in the specified period and submitted electronically. 2789 */ 2790 ADNFPPELCT, 2791 /** 2792 * Identifies the total net amount of all Invoice Groupings that were 2793 * adjudicated as payable prior to the specified time period (based on 2794 * adjudication date), subsequently cancelled in the specified period and 2795 * submitted manually. 2796 */ 2797 ADNFPPMNAT, 2798 /** 2799 * Identifies the total number of all Invoice Groupings that were adjudicated as 2800 * payable prior to the specified time period (based on adjudication date), 2801 * subsequently cancelled in the specified period and submitted manually. 2802 */ 2803 ADNFPPMNCT, 2804 /** 2805 * Identifies the total net amount of all Invoice Groupings that were 2806 * adjudicated as payable during the specified time period (based on 2807 * adjudication date), subsequently nullified in the specified period and 2808 * submitted electronically. 2809 */ 2810 ADNFSPELAT, 2811 /** 2812 * Identifies the total number of all Invoice Groupings that were adjudicated as 2813 * payable during the specified time period (based on adjudication date), 2814 * subsequently nullified in the specified period and submitted electronically. 2815 */ 2816 ADNFSPELCT, 2817 /** 2818 * Identifies the total net amount of all Invoice Groupings that were 2819 * adjudicated as payable during the specified time period (based on 2820 * adjudication date), subsequently cancelled in the specified period and 2821 * submitted manually. 2822 */ 2823 ADNFSPMNAT, 2824 /** 2825 * Identifies the total number of all Invoice Groupings that were adjudicated as 2826 * payable during the specified time period (based on adjudication date), 2827 * subsequently cancelled in the specified period and submitted manually. 2828 */ 2829 ADNFSPMNCT, 2830 /** 2831 * Identifies the total net amount of all Invoice Groupings that were 2832 * adjudicated as payable prior to the specified time period (based on 2833 * adjudication date) that do not match a specified payee (e.g. pay patient) and 2834 * submitted electronically. 2835 */ 2836 ADNPPPELAT, 2837 /** 2838 * Identifies the total number of all Invoice Groupings that were adjudicated as 2839 * payable prior to the specified time period (based on adjudication date) that 2840 * do not match a specified payee (e.g. pay patient) and submitted 2841 * electronically. 2842 */ 2843 ADNPPPELCT, 2844 /** 2845 * Identifies the total net amount of all Invoice Groupings that were 2846 * adjudicated as payable prior to the specified time period (based on 2847 * adjudication date) that do not match a specified payee (e.g. pay patient) and 2848 * submitted manually. 2849 */ 2850 ADNPPPMNAT, 2851 /** 2852 * Identifies the total number of all Invoice Groupings that were adjudicated as 2853 * payable prior to the specified time period (based on adjudication date) that 2854 * do not match a specified payee (e.g. pay patient) and submitted manually. 2855 */ 2856 ADNPPPMNCT, 2857 /** 2858 * Identifies the total net amount of all Invoice Groupings that were 2859 * adjudicated as payable during the specified time period (based on 2860 * adjudication date) that do not match a specified payee (e.g. pay patient) and 2861 * submitted electronically. 2862 */ 2863 ADNPSPELAT, 2864 /** 2865 * Identifies the total number of all Invoice Groupings that were adjudicated as 2866 * payable during the specified time period (based on adjudication date) that do 2867 * not match a specified payee (e.g. pay patient) and submitted electronically. 2868 */ 2869 ADNPSPELCT, 2870 /** 2871 * Identifies the total net amount of all Invoice Groupings that were 2872 * adjudicated as payable during the specified time period (based on 2873 * adjudication date) that do not match a specified payee (e.g. pay patient) and 2874 * submitted manually. 2875 */ 2876 ADNPSPMNAT, 2877 /** 2878 * Identifies the total number of all Invoice Groupings that were adjudicated as 2879 * payable during the specified time period (based on adjudication date) that do 2880 * not match a specified payee (e.g. pay patient) and submitted manually. 2881 */ 2882 ADNPSPMNCT, 2883 /** 2884 * Identifies the total net amount of all Invoice Groupings that were 2885 * adjudicated as payable prior to the specified time period (based on 2886 * adjudication date) that match a specified payee (e.g. pay provider) and 2887 * submitted electronically. 2888 */ 2889 ADPPPPELAT, 2890 /** 2891 * Identifies the total number of all Invoice Groupings that were adjudicated as 2892 * payable prior to the specified time period (based on adjudication date) that 2893 * match a specified payee (e.g. pay provider) and submitted electronically. 2894 */ 2895 ADPPPPELCT, 2896 /** 2897 * Identifies the total net amount of all Invoice Groupings that were 2898 * adjudicated as payable prior to the specified time period (based on 2899 * adjudication date) that match a specified payee (e.g. pay provider) and 2900 * submitted manually. 2901 */ 2902 ADPPPPMNAT, 2903 /** 2904 * Identifies the total number of all Invoice Groupings that were adjudicated as 2905 * payable prior to the specified time period (based on adjudication date) that 2906 * match a specified payee (e.g. pay provider) and submitted manually. 2907 */ 2908 ADPPPPMNCT, 2909 /** 2910 * Identifies the total net amount of all Invoice Groupings that were 2911 * adjudicated as payable during the specified time period (based on 2912 * adjudication date) that match a specified payee (e.g. pay provider) and 2913 * submitted electronically. 2914 */ 2915 ADPPSPELAT, 2916 /** 2917 * Identifies the total number of all Invoice Groupings that were adjudicated as 2918 * payable during the specified time period (based on adjudication date) that 2919 * match a specified payee (e.g. pay provider) and submitted electronically. 2920 */ 2921 ADPPSPELCT, 2922 /** 2923 * Identifies the total net amount of all Invoice Groupings that were 2924 * adjudicated as payable during the specified time period (based on 2925 * adjudication date) that match a specified payee (e.g. pay provider) and 2926 * submitted manually. 2927 */ 2928 ADPPSPMNAT, 2929 /** 2930 * Identifies the total number of all Invoice Groupings that were adjudicated as 2931 * payable during the specified time period (based on adjudication date) that 2932 * match a specified payee (e.g. pay provider) and submitted manually. 2933 */ 2934 ADPPSPMNCT, 2935 /** 2936 * Identifies the total net amount of all Invoice Groupings that were 2937 * adjudicated as refused prior to the specified time period (based on 2938 * adjudication date) and submitted electronically. 2939 */ 2940 ADRFPPELAT, 2941 /** 2942 * Identifies the total number of all Invoice Groupings that were adjudicated as 2943 * refused prior to the specified time period (based on adjudication date) and 2944 * submitted electronically. 2945 */ 2946 ADRFPPELCT, 2947 /** 2948 * Identifies the total net amount of all Invoice Groupings that were 2949 * adjudicated as refused prior to the specified time period (based on 2950 * adjudication date) and submitted manually. 2951 */ 2952 ADRFPPMNAT, 2953 /** 2954 * Identifies the total number of all Invoice Groupings that were adjudicated as 2955 * refused prior to the specified time period (based on adjudication date) and 2956 * submitted manually. 2957 */ 2958 ADRFPPMNCT, 2959 /** 2960 * Identifies the total net amount of all Invoice Groupings that were 2961 * adjudicated as refused during the specified time period (based on 2962 * adjudication date) and submitted electronically. 2963 */ 2964 ADRFSPELAT, 2965 /** 2966 * Identifies the total number of all Invoice Groupings that were adjudicated as 2967 * refused during the specified time period (based on adjudication date) and 2968 * submitted electronically. 2969 */ 2970 ADRFSPELCT, 2971 /** 2972 * Identifies the total net amount of all Invoice Groupings that were 2973 * adjudicated as refused during the specified time period (based on 2974 * adjudication date) and submitted manually. 2975 */ 2976 ADRFSPMNAT, 2977 /** 2978 * Identifies the total number of all Invoice Groupings that were adjudicated as 2979 * refused during the specified time period (based on adjudication date) and 2980 * submitted manually. 2981 */ 2982 ADRFSPMNCT, 2983 /** 2984 * Total counts and total net amounts paid for all Invoice Groupings that were 2985 * paid within a time period based on the payment date. 2986 */ 2987 _INVOICEELEMENTPAID, 2988 /** 2989 * Identifies the total net amount of all Invoice Groupings that were paid prior 2990 * to the specified time period (based on payment date), subsequently nullified 2991 * in the specified period and submitted electronically. 2992 */ 2993 PDNFPPELAT, 2994 /** 2995 * Identifies the total number of all Invoice Groupings that were paid prior to 2996 * the specified time period (based on payment date), subsequently nullified in 2997 * the specified period and submitted electronically. 2998 */ 2999 PDNFPPELCT, 3000 /** 3001 * Identifies the total net amount of all Invoice Groupings that were paid prior 3002 * to the specified time period (based on payment date), subsequently nullified 3003 * in the specified period and submitted manually. 3004 */ 3005 PDNFPPMNAT, 3006 /** 3007 * Identifies the total number of all Invoice Groupings that were paid prior to 3008 * the specified time period (based on payment date), subsequently nullified in 3009 * the specified period and submitted manually. 3010 */ 3011 PDNFPPMNCT, 3012 /** 3013 * Identifies the total net amount of all Invoice Groupings that were paid 3014 * during the specified time period (based on payment date), subsequently 3015 * nullified in the specified period and submitted electronically. 3016 */ 3017 PDNFSPELAT, 3018 /** 3019 * Identifies the total number of all Invoice Groupings that were paid during 3020 * the specified time period (based on payment date), subsequently cancelled in 3021 * the specified period and submitted electronically. 3022 */ 3023 PDNFSPELCT, 3024 /** 3025 * Identifies the total net amount of all Invoice Groupings that were paid 3026 * during the specified time period (based on payment date), subsequently 3027 * nullified in the specified period and submitted manually. 3028 */ 3029 PDNFSPMNAT, 3030 /** 3031 * Identifies the total number of all Invoice Groupings that were paid during 3032 * the specified time period (based on payment date), subsequently nullified in 3033 * the specified period and submitted manually. 3034 */ 3035 PDNFSPMNCT, 3036 /** 3037 * Identifies the total net amount of all Invoice Groupings that were paid prior 3038 * to the specified time period (based on payment date) that do not match a 3039 * specified payee (e.g. pay patient) and submitted electronically. 3040 */ 3041 PDNPPPELAT, 3042 /** 3043 * Identifies the total number of all Invoice Groupings that were paid prior to 3044 * the specified time period (based on payment date) that do not match a 3045 * specified payee (e.g. pay patient) and submitted electronically. 3046 */ 3047 PDNPPPELCT, 3048 /** 3049 * Identifies the total net amount of all Invoice Groupings that were paid prior 3050 * to the specified time period (based on payment date) that do not match a 3051 * specified payee (e.g. pay patient) and submitted manually. 3052 */ 3053 PDNPPPMNAT, 3054 /** 3055 * Identifies the total number of all Invoice Groupings that were paid prior to 3056 * the specified time period (based on payment date) that do not match a 3057 * specified payee (e.g. pay patient) and submitted manually. 3058 */ 3059 PDNPPPMNCT, 3060 /** 3061 * Identifies the total net amount of all Invoice Groupings that were paid 3062 * during the specified time period (based on payment date) that do not match a 3063 * specified payee (e.g. pay patient) and submitted electronically. 3064 */ 3065 PDNPSPELAT, 3066 /** 3067 * Identifies the total number of all Invoice Groupings that were paid during 3068 * the specified time period (based on payment date) that do not match a 3069 * specified payee (e.g. pay patient) and submitted electronically. 3070 */ 3071 PDNPSPELCT, 3072 /** 3073 * Identifies the total net amount of all Invoice Groupings that were paid 3074 * during the specified time period (based on payment date) that do not match a 3075 * specified payee (e.g. pay patient) and submitted manually. 3076 */ 3077 PDNPSPMNAT, 3078 /** 3079 * Identifies the total number of all Invoice Groupings that were paid during 3080 * the specified time period (based on payment date) that do not match a 3081 * specified payee (e.g. pay patient) and submitted manually. 3082 */ 3083 PDNPSPMNCT, 3084 /** 3085 * Identifies the total net amount of all Invoice Groupings that were paid prior 3086 * to the specified time period (based on payment date) that match a specified 3087 * payee (e.g. pay provider) and submitted electronically. 3088 */ 3089 PDPPPPELAT, 3090 /** 3091 * Identifies the total number of all Invoice Groupings that were paid prior to 3092 * the specified time period (based on payment date) that match a specified 3093 * payee (e.g. pay provider) and submitted electronically. 3094 */ 3095 PDPPPPELCT, 3096 /** 3097 * Identifies the total net amount of all Invoice Groupings that were paid prior 3098 * to the specified time period (based on payment date) that match a specified 3099 * payee (e.g. pay provider) and submitted manually. 3100 */ 3101 PDPPPPMNAT, 3102 /** 3103 * Identifies the total number of all Invoice Groupings that were paid prior to 3104 * the specified time period (based on payment date) that match a specified 3105 * payee (e.g. pay provider) and submitted manually. 3106 */ 3107 PDPPPPMNCT, 3108 /** 3109 * Identifies the total net amount of all Invoice Groupings that were paid 3110 * during the specified time period (based on payment date) that match a 3111 * specified payee (e.g. pay provider) and submitted electronically. 3112 */ 3113 PDPPSPELAT, 3114 /** 3115 * Identifies the total number of all Invoice Groupings that were paid during 3116 * the specified time period (based on payment date) that match a specified 3117 * payee (e.g. pay provider) and submitted electronically. 3118 */ 3119 PDPPSPELCT, 3120 /** 3121 * Identifies the total net amount of all Invoice Groupings that were paid 3122 * during the specified time period (based on payment date) that match a 3123 * specified payee (e.g. pay provider) and submitted manually. 3124 */ 3125 PDPPSPMNAT, 3126 /** 3127 * Identifies the total number of all Invoice Groupings that were paid during 3128 * the specified time period (based on payment date) that match a specified 3129 * payee (e.g. pay provider) and submitted manually. 3130 */ 3131 PDPPSPMNCT, 3132 /** 3133 * Total counts and total net amounts billed for all Invoice Groupings that were 3134 * submitted within a time period. Adjudicated invoice elements are included. 3135 */ 3136 _INVOICEELEMENTSUBMITTED, 3137 /** 3138 * Identifies the total net amount billed for all submitted Invoice Groupings 3139 * within a time period and submitted electronically. Adjudicated invoice 3140 * elements are included. 3141 */ 3142 SBBLELAT, 3143 /** 3144 * Identifies the total number of submitted Invoice Groupings within a time 3145 * period and submitted electronically. Adjudicated invoice elements are 3146 * included. 3147 */ 3148 SBBLELCT, 3149 /** 3150 * Identifies the total net amount billed for all submitted Invoice Groupings 3151 * that were nullified within a time period and submitted electronically. 3152 * Adjudicated invoice elements are included. 3153 */ 3154 SBNFELAT, 3155 /** 3156 * Identifies the total number of submitted Invoice Groupings that were 3157 * nullified within a time period and submitted electronically. Adjudicated 3158 * invoice elements are included. 3159 */ 3160 SBNFELCT, 3161 /** 3162 * Identifies the total net amount billed for all submitted Invoice Groupings 3163 * that are pended or held by the payor, within a time period and submitted 3164 * electronically. Adjudicated invoice elements are not included. 3165 */ 3166 SBPDELAT, 3167 /** 3168 * Identifies the total number of submitted Invoice Groupings that are pended or 3169 * held by the payor, within a time period and submitted electronically. 3170 * Adjudicated invoice elements are not included. 3171 */ 3172 SBPDELCT, 3173 /** 3174 * Includes coded responses that will occur as a result of the adjudication of 3175 * an electronic invoice at a summary level and provides guidance on 3176 * interpretation of the referenced adjudication results. 3177 */ 3178 _ACTINVOICEOVERRIDECODE, 3179 /** 3180 * Insurance coverage problems have been encountered. Additional explanation 3181 * information to be supplied. 3182 */ 3183 COVGE, 3184 /** 3185 * Electronic form with supporting or additional information to follow. 3186 */ 3187 EFORM, 3188 /** 3189 * Fax with supporting or additional information to follow. 3190 */ 3191 FAX, 3192 /** 3193 * The medical service was provided to a patient in good faith that they had 3194 * medical coverage, although no evidence of coverage was available before 3195 * service was rendered. 3196 */ 3197 GFTH, 3198 /** 3199 * Knowingly over the payor's published time limit for this invoice possibly due 3200 * to a previous payor's delays in processing. Additional reason information 3201 * will be supplied. 3202 */ 3203 LATE, 3204 /** 3205 * Manual review of the invoice is requested. Additional information to be 3206 * supplied. This may be used in the case of an appeal. 3207 */ 3208 MANUAL, 3209 /** 3210 * The medical service and/or product was provided to a patient that has 3211 * coverage in another jurisdiction. 3212 */ 3213 OOJ, 3214 /** 3215 * The service provided is required for orthodontic purposes. If the covered 3216 * party has orthodontic coverage, then the service may be paid. 3217 */ 3218 ORTHO, 3219 /** 3220 * Paper documentation (or other physical format) with supporting or additional 3221 * information to follow. 3222 */ 3223 PAPER, 3224 /** 3225 * Public Insurance has been exhausted. Invoice has not been sent to Public 3226 * Insuror and therefore no Explanation Of Benefits (EOB) is provided with this 3227 * Invoice submission. 3228 */ 3229 PIE, 3230 /** 3231 * Allows provider to explain lateness of invoice to a subsequent payor. 3232 */ 3233 PYRDELAY, 3234 /** 3235 * Rules of practice do not require a physician's referral for the provider to 3236 * perform a billable service. 3237 */ 3238 REFNR, 3239 /** 3240 * The same service was delivered within a time period that would usually 3241 * indicate a duplicate billing. However, the repeated service is a medical 3242 * necessity and therefore not a duplicate. 3243 */ 3244 REPSERV, 3245 /** 3246 * The service provided is not related to another billed service. For example, 2 3247 * unrelated services provided on the same day to the same patient which may 3248 * normally result in a refused payment for one of the items. 3249 */ 3250 UNRELAT, 3251 /** 3252 * The provider has received a verbal permission from an authoritative source to 3253 * perform the service or supply the item being invoiced. 3254 */ 3255 VERBAUTH, 3256 /** 3257 * Provides codes associated with ActClass value of LIST (working list) 3258 */ 3259 _ACTLISTCODE, 3260 /** 3261 * ActObservationList 3262 */ 3263 _ACTOBSERVATIONLIST, 3264 /** 3265 * List of acts representing a care plan. The acts can be in a varierty of moods 3266 * including event (EVN) to record acts that have been carried out as part of 3267 * the care plan. 3268 */ 3269 CARELIST, 3270 /** 3271 * List of condition observations. 3272 */ 3273 CONDLIST, 3274 /** 3275 * List of intolerance observations. 3276 */ 3277 INTOLIST, 3278 /** 3279 * List of problem observations. 3280 */ 3281 PROBLIST, 3282 /** 3283 * List of risk factor observations. 3284 */ 3285 RISKLIST, 3286 /** 3287 * List of observations in goal mood. 3288 */ 3289 GOALLIST, 3290 /** 3291 * Codes used to identify different types of 'duration-based' working lists. 3292 * Examples include "Continuous/Chronic", "Short-Term" and "As-Needed". 3293 */ 3294 _ACTTHERAPYDURATIONWORKINGLISTCODE, 3295 /** 3296 * Definition:A collection of concepts that identifies different types of 3297 * 'duration-based' mediation working lists. 3298 * 3299 * 3300 * Examples:"Continuous/Chronic" "Short-Term" and "As Needed" 3301 */ 3302 _ACTMEDICATIONTHERAPYDURATIONWORKINGLISTCODE, 3303 /** 3304 * Definition:A list of medications which the patient is only expected to 3305 * consume for the duration of the current order or limited set of orders and 3306 * which is not expected to be renewed. 3307 */ 3308 ACU, 3309 /** 3310 * Definition:A list of medications which are expected to be continued beyond 3311 * the present order and which the patient should be assumed to be taking unless 3312 * explicitly stopped. 3313 */ 3314 CHRON, 3315 /** 3316 * Definition:A list of medications which the patient is intended to be 3317 * administered only once. 3318 */ 3319 ONET, 3320 /** 3321 * Definition:A list of medications which the patient will consume 3322 * intermittently based on the behavior of the condition for which the 3323 * medication is indicated. 3324 */ 3325 PRN, 3326 /** 3327 * List of medications. 3328 */ 3329 MEDLIST, 3330 /** 3331 * List of current medications. 3332 */ 3333 CURMEDLIST, 3334 /** 3335 * List of discharge medications. 3336 */ 3337 DISCMEDLIST, 3338 /** 3339 * Historical list of medications. 3340 */ 3341 HISTMEDLIST, 3342 /** 3343 * Identifies types of monitoring programs 3344 */ 3345 _ACTMONITORINGPROTOCOLCODE, 3346 /** 3347 * A monitoring program that focuses on narcotics and/or commonly abused 3348 * substances that are subject to legal restriction. 3349 */ 3350 CTLSUB, 3351 /** 3352 * Definition:A monitoring program that focuses on a drug which is under 3353 * investigation and has not received regulatory approval for the condition 3354 * being investigated 3355 */ 3356 INV, 3357 /** 3358 * Description:A drug that can be prescribed (and reimbursed) only if it meets 3359 * certain criteria. 3360 */ 3361 LU, 3362 /** 3363 * Medicines designated in this way may be supplied for patient use without a 3364 * prescription. The exact form of categorisation will vary in different realms. 3365 */ 3366 OTC, 3367 /** 3368 * Some form of prescription is required before the related medicine can be 3369 * supplied for a patient. The exact form of regulation will vary in different 3370 * realms. 3371 */ 3372 RX, 3373 /** 3374 * Definition:A drug that requires prior approval (to be reimbursed) before 3375 * being dispensed 3376 */ 3377 SA, 3378 /** 3379 * Description:A drug that requires special access permission to be prescribed 3380 * and dispensed. 3381 */ 3382 SAC, 3383 /** 3384 * Description:Concepts representing indications (reasons for clinical action) 3385 * other than diagnosis and symptoms. 3386 */ 3387 _ACTNONOBSERVATIONINDICATIONCODE, 3388 /** 3389 * Description:Contrast agent required for imaging study. 3390 */ 3391 IND01, 3392 /** 3393 * Description:Provision of prescription or direction to consume a product for 3394 * purposes of bowel clearance in preparation for a colonoscopy. 3395 */ 3396 IND02, 3397 /** 3398 * Description:Provision of medication as a preventative measure during a 3399 * treatment or other period of increased risk. 3400 */ 3401 IND03, 3402 /** 3403 * Description:Provision of medication during pre-operative phase; e.g., 3404 * antibiotics before dental surgery or bowel prep before colon surgery. 3405 */ 3406 IND04, 3407 /** 3408 * Description:Provision of medication for pregnancy --e.g., vitamins, 3409 * antibiotic treatments for vaginal tract colonization, etc. 3410 */ 3411 IND05, 3412 /** 3413 * Identifies the type of verification investigation being undertaken with 3414 * respect to the subject of the verification activity. 3415 * 3416 * 3417 * Examples: 3418 * 3419 * 3420 * 3421 * 3422 * Verification of eligibility for coverage under a policy or program - aka 3423 * enrolled/covered by a policy or program 3424 * 3425 * 3426 * 3427 * Verification of record - e.g., person has record in an immunization registry 3428 * 3429 * 3430 * 3431 * Verification of enumeration - e.g. NPI 3432 * 3433 * 3434 * 3435 * Verification of Board Certification - provider specific 3436 * 3437 * 3438 * 3439 * Verification of Certification - e.g. JAHCO, NCQA, URAC 3440 * 3441 * 3442 * 3443 * Verification of Conformance - e.g. entity use with HIPAA, conformant to the 3444 * CCHIT EHR system criteria 3445 * 3446 * 3447 * 3448 * Verification of Provider Credentials 3449 * 3450 * 3451 * 3452 * Verification of no adverse findings - e.g. on National Provider Data Bank, 3453 * Health Integrity Protection Data Base (HIPDB) 3454 */ 3455 _ACTOBSERVATIONVERIFICATIONTYPE, 3456 /** 3457 * Definition:Indicates that the paper version of the record has, should be or 3458 * is being verified against the electronic version. 3459 */ 3460 VFPAPER, 3461 /** 3462 * Code identifying the method or the movement of payment instructions. 3463 * 3464 * Codes are drawn from X12 data element 591 (PaymentMethodCode) 3465 */ 3466 _ACTPAYMENTCODE, 3467 /** 3468 * Automated Clearing House (ACH). 3469 */ 3470 ACH, 3471 /** 3472 * A written order to a bank to pay the amount specified from funds on deposit. 3473 */ 3474 CHK, 3475 /** 3476 * Electronic Funds Transfer (EFT) deposit into the payee's bank account 3477 */ 3478 DDP, 3479 /** 3480 * Non-Payment Data. 3481 */ 3482 NON, 3483 /** 3484 * Identifies types of dispensing events 3485 */ 3486 _ACTPHARMACYSUPPLYTYPE, 3487 /** 3488 * A fill providing sufficient supply for one day 3489 */ 3490 DF, 3491 /** 3492 * A supply action where there is no 'valid' order for the supplied medication. 3493 * E.g. Emergency vacation supply, weekend supply (when prescriber is 3494 * unavailable to provide a renewal prescription) 3495 */ 3496 EM, 3497 /** 3498 * An emergency supply where the expectation is that a formal order authorizing 3499 * the supply will be provided at a later date. 3500 */ 3501 SO, 3502 /** 3503 * The initial fill against an order. (This includes initial fills against 3504 * refill orders.) 3505 */ 3506 FF, 3507 /** 3508 * A first fill where the quantity supplied is equal to one full repetition of 3509 * the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete 3510 * fill would be for the full 90 tablets). 3511 */ 3512 FFC, 3513 /** 3514 * A first fill where the quantity supplied is less than one full repetition of 3515 * the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial 3516 * fill might be for only 30 tablets.) 3517 */ 3518 FFP, 3519 /** 3520 * A first fill where the strength supplied is less than the ordered strength. 3521 * (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg 3522 * tablets). 3523 */ 3524 FFSS, 3525 /** 3526 * A fill where a small portion is provided to allow for determination of the 3527 * therapy effectiveness and patient tolerance. 3528 */ 3529 TF, 3530 /** 3531 * A supply action to restock a smaller more local dispensary. 3532 */ 3533 FS, 3534 /** 3535 * A supply of a manufacturer sample 3536 */ 3537 MS, 3538 /** 3539 * A fill against an order that has already been filled (or partially filled) at 3540 * least once. 3541 */ 3542 RF, 3543 /** 3544 * A supply action that provides sufficient material for a single dose. 3545 */ 3546 UD, 3547 /** 3548 * A refill where the quantity supplied is equal to one full repetition of the 3549 * ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill 3550 * would be for the full 90 tablets.) 3551 */ 3552 RFC, 3553 /** 3554 * A refill where the quantity supplied is equal to one full repetition of the 3555 * ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill 3556 * would be for the full 90 tablets.) and where the strength supplied is less 3557 * than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent 3558 * fill will dispense 40mg tablets). 3559 */ 3560 RFCS, 3561 /** 3562 * The first fill against an order that has already been filled at least once at 3563 * another facility. 3564 */ 3565 RFF, 3566 /** 3567 * The first fill against an order that has already been filled at least once at 3568 * another facility and where the strength supplied is less than the ordered 3569 * strength (e.g. 10mg for an order of 50mg where a subsequent fill will 3570 * dispense 40mg tablets). 3571 */ 3572 RFFS, 3573 /** 3574 * A refill where the quantity supplied is less than one full repetition of the 3575 * ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill 3576 * might be for only 30 tablets.) 3577 */ 3578 RFP, 3579 /** 3580 * A refill where the quantity supplied is less than one full repetition of the 3581 * ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill 3582 * might be for only 30 tablets.) and where the strength supplied is less than 3583 * the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill 3584 * will dispense 40mg tablets). 3585 */ 3586 RFPS, 3587 /** 3588 * A fill against an order that has already been filled (or partially filled) at 3589 * least once and where the strength supplied is less than the ordered strength 3590 * (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg 3591 * tablets). 3592 */ 3593 RFS, 3594 /** 3595 * A fill where the remainder of a 'complete' fill is provided after a trial 3596 * fill has been provided. 3597 */ 3598 TB, 3599 /** 3600 * A fill where the remainder of a 'complete' fill is provided after a trial 3601 * fill has been provided and where the strength supplied is less than the 3602 * ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will 3603 * dispense 40mg tablets). 3604 */ 3605 TBS, 3606 /** 3607 * A supply action that provides sufficient material for a single dose via 3608 * multiple products. E.g. 2 50mg tablets for a 100mg unit dose. 3609 */ 3610 UDE, 3611 /** 3612 * Description:Types of policies that further specify the ActClassPolicy value 3613 * set. 3614 */ 3615 _ACTPOLICYTYPE, 3616 /** 3617 * A policy deeming certain information to be private to an individual or 3618 * organization. 3619 * 3620 * 3621 * Definition: A mandate, obligation, requirement, rule, or expectation relating 3622 * to privacy. 3623 * 3624 * 3625 * Discussion: ActPrivacyPolicyType codes support the designation of the 1..* 3626 * policies that are applicable to an Act such as a Consent Directive, a Role 3627 * such as a VIP Patient, or an Entity such as a patient who is a minor. 1..* 3628 * ActPrivacyPolicyType values may be associated with an Act or Role to indicate 3629 * the policies that govern the assignment of an Act or Role 3630 * confidentialityCode. Use of multiple ActPrivacyPolicyType values enables fine 3631 * grain specification of applicable policies, but must be carefully assigned to 3632 * ensure cogency and avoid creation of conflicting policy mandates. 3633 * 3634 * 3635 * Usage Note: Statutory title may be named in the ActClassPolicy Act Act.title 3636 * to specify which privacy policy is being referenced. 3637 */ 3638 _ACTPRIVACYPOLICY, 3639 /** 3640 * Specifies the type of agreement between one or more grantor and grantee in 3641 * which rights and obligations related to one or more shared items of interest 3642 * are allocated. 3643 * 3644 * 3645 * Usage Note: Such agreements may be considered "consent directives" or 3646 * "contracts" depending on the context, and are considered closely related or 3647 * synonymous from a legal perspective. 3648 * 3649 * 3650 * Examples: 3651 * 3652 * 3653 * 3654 * Healthcare Privacy Consent Directive permitting or restricting in whole or 3655 * part the collection, access, use, and disclosure of health information, and 3656 * any associated handling caveats. Healthcare Medical Consent Directive to 3657 * receive medical procedures after being informed of risks and benefits, 3658 * thereby reducing the grantee's liability. Research Informed Consent for 3659 * participation in clinical trials and disclosure of health information after 3660 * being informed of risks and benefits, thereby reducing the grantee's 3661 * liability. Substitute decision maker delegation in which the grantee assumes 3662 * responsibility to act on behalf of the grantor. Contracts in which the 3663 * agreement requires assent/dissent by the grantor of terms offered by a 3664 * grantee, a consumer opts out of an "award" system for use of a retailer's 3665 * marketing or credit card vendor's point collection cards in exchange for 3666 * allowing purchase tracking and profiling. A mobile device or App privacy 3667 * policy and terms of service to which a user must agree in whole or in part in 3668 * order to utilize the service. Agreements between a client and an 3669 * authorization server or between an authorization server and a resource 3670 * operator and/or resource owner permitting or restricting e.g., collection, 3671 * access, use, and disclosure of information, and any associated handling 3672 * caveats. 3673 */ 3674 _ACTCONSENTDIRECTIVE, 3675 /** 3676 * This general consent directive specifically limits disclosure of health 3677 * information for purpose of emergency treatment. Additional parameters may 3678 * further limit the disclosure to specific users, roles, duration, types of 3679 * information, and impose uses obligations. 3680 * 3681 * 3682 * Definition: Opt-in to disclosure of health information for emergency only 3683 * consent directive. 3684 */ 3685 EMRGONLY, 3686 /** 3687 * A grantor's terms of agreement to which a grantee may assent or dissent, and 3688 * which may include an opportunity for a grantee to request restrictions or 3689 * extensions. 3690 * 3691 * 3692 * Comment: A grantor typically is able to stipulate preferred terms of 3693 * agreement when the grantor has control over the topic of the agreement, which 3694 * a grantee must accept in full or may be offered an opportunity to extend or 3695 * restrict certain terms. 3696 * 3697 * 3698 * Usage Note: If the grantor's term of agreement must be accepted in full, then 3699 * this is considered "basic consent". If a grantee is offered an opportunity to 3700 * extend or restrict certain terms, then the agreement is considered "granular 3701 * consent". 3702 * 3703 * 3704 * Examples: 3705 * 3706 * 3707 * 3708 * Healthcare: A PHR account holder [grantor] may require any PHR user [grantee] 3709 * to accept the terms of agreement in full, or may permit a PHR user to extend 3710 * or restrict terms selected by the account holder or requested by the PHR 3711 * user. Non-healthcare: The owner of a resource server [grantor] may require 3712 * any authorization server [grantee] to meet authorization requirements 3713 * stipulated in the grantor's terms of agreement. 3714 */ 3715 GRANTORCHOICE, 3716 /** 3717 * A grantor's presumed assent to the grantee's terms of agreement is based on 3718 * the grantor's behavior, which may result from not expressly assenting to the 3719 * consent directive offered, or from having no right to assent or dissent 3720 * offered by the grantee. 3721 * 3722 * 3723 * Comment: Implied or "implicit" consent occurs when the behavior of the 3724 * grantor is understood by a reasonable person to signal agreement to the 3725 * grantee's terms. 3726 * 3727 * 3728 * Usage Note: Implied consent with no opportunity to assent or dissent to 3729 * certain terms is considered "basic consent". 3730 * 3731 * 3732 * Examples: 3733 * 3734 * 3735 * 3736 * Healthcare: A patient schedules an appointment with a provider, and either 3737 * does not take the opportunity to expressly assent or dissent to the 3738 * provider's consent directive, does not have an opportunity to do so, as in 3739 * the case where emergency care is required, or simply behaves as though the 3740 * patient [grantor] agrees to the rights granted to the provider [grantee] in 3741 * an implicit consent directive. An injured and unconscious patient is deemed 3742 * to have assented to emergency treatment by those permitted to do so under 3743 * jurisdictional laws, e.g., Good Samaritan laws. Non-healthcare: Upon 3744 * receiving a driver's license, the driver is deemed to have assented without 3745 * explicitly consenting to undergoing field sobriety tests. A corporation that 3746 * does business in a foreign nation is deemed to have deemed to have assented 3747 * without explicitly consenting to abide by that nation's laws. 3748 */ 3749 IMPLIED, 3750 /** 3751 * A grantor's presumed assent to the grantee's terms of agreement, which is 3752 * based on the grantor's behavior, and includes a right to dissent to certain 3753 * terms. 3754 * 3755 * 3756 * Comment: A grantor assenting to the grantee's terms of agreement may or may 3757 * not exercise a right to dissent to grantor selected terms or to grantee's 3758 * selected terms to which a grantor may dissent. 3759 * 3760 * 3761 * Usage Note: Implied or "implicit" consent with an "opportunity to dissent" 3762 * occurs when the grantor's behavior is understood by a reasonable person to 3763 * signal assent to the grantee's terms of agreement whether the grantor 3764 * requests or the grantee approves further restrictions, is considered 3765 * "granular consent". 3766 * 3767 * 3768 * Examples: 3769 * 3770 * 3771 * 3772 * Healthcare Examples: A healthcare provider deems a patient's assent to 3773 * disclosure of health information to family members and friends, but offers an 3774 * opportunity or permits the patient to dissent to such disclosures. A health 3775 * information exchanges deems a patient to have assented to disclosure of 3776 * health information for treatment purposes, but offers the patient an 3777 * opportunity to dissents to disclosure to particular provider organizations. 3778 * Non-healthcare Examples: A bank deems a banking customer's assent to 3779 * specified collection, access, use, or disclosure of financial information as 3780 * a requirement of holding a bank account, but provides the user an opportunity 3781 * to limit third-party collection, access, use or disclosure of that 3782 * information for marketing purposes. 3783 */ 3784 IMPLIEDD, 3785 /** 3786 * No notification or opportunity is provided for a grantor to assent or dissent 3787 * to a grantee's terms of agreement. 3788 * 3789 * 3790 * Comment: A "No Consent" policy scheme provides no opportunity for 3791 * accommodation of an individual's preferences, and may not comply with Fair 3792 * Information Practice Principles [FIPP] by enabling the data subject to 3793 * object, access collected information, correct errors, or have accounting of 3794 * disclosures. 3795 * 3796 * 3797 * Usage Note: The grantee's terms of agreement, may be available to the grantor 3798 * by reviewing the grantee's privacy policies, but there is no notice by which 3799 * a grantor is apprised of the policy directly or able to acknowledge. 3800 * 3801 * 3802 * Examples: 3803 * 3804 * 3805 * 3806 * Healthcare: Without notification or an opportunity to assent or dissent, a 3807 * patient's health information is automatically included in and available 3808 * (often according to certain rules) through a health information exchange. 3809 * Note that this differs from implied consent, where the patient is assumed to 3810 * have consented. Without notification or an opportunity to assent or dissent, 3811 * a patient's health information is collected, accessed, used, or disclosed for 3812 * research, public health, security, fraud prevention, court order, or law 3813 * enforcement. Non-healthcare: Without notification or an opportunity to assent 3814 * or dissent, a consumer's healthcare or non-healthcare internet searches are 3815 * aggregated for secondary uses such as behavioral tracking and profiling. 3816 * Without notification or an opportunity to assent or dissent, a consumer's 3817 * location and activities in a shopping mall are tracked by RFID tags on 3818 * purchased items. 3819 */ 3820 NOCONSENT, 3821 /** 3822 * Acknowledgement of custodian notice of privacy practices. 3823 * 3824 * 3825 * Usage Notes: This type of consent directive acknowledges a custodian's notice 3826 * of privacy practices including its permitted collection, access, use and 3827 * disclosure of health information to users and for purposes of use specified. 3828 */ 3829 NOPP, 3830 /** 3831 * A grantor's assent to the terms of an agreement offered by a grantee without 3832 * an opportunity for to dissent to any terms. 3833 * 3834 * 3835 * Comment: Acceptance of a grantee's terms pertaining, for example, to 3836 * permissible activities, purposes of use, handling caveats, expiry date, and 3837 * revocation policies. 3838 * 3839 * 3840 * Usage Note: Opt-in with no opportunity for a grantor to restrict certain 3841 * permissions sought by the grantee is considered "basic consent". 3842 * 3843 * 3844 * Examples: 3845 * 3846 * 3847 * 3848 * Healthcare: A patient [grantor] signs a provider's [grantee's] consent 3849 * directive form, which lists permissible collection, access, use, or 3850 * disclosure activities, purposes of use, handling caveats, and revocation 3851 * policies. Non-healthcare: An employee [grantor] signs an employer's 3852 * [grantee's] non-disclosure and non-compete agreement. 3853 */ 3854 OPTIN, 3855 /** 3856 * A grantor's assent to the grantee's terms of an agreement with an opportunity 3857 * for to dissent to certain grantor or grantee selected terms. 3858 * 3859 * 3860 * Comment: A grantor dissenting to the grantee's terms of agreement may or may 3861 * not exercise a right to assent to grantor's pre-approved restrictions or to 3862 * grantee's selected terms to which a grantor may dissent. 3863 * 3864 * 3865 * Usage Note: Opt-in with restrictions is considered "granular consent" because 3866 * the grantor has an opportunity to narrow the permissions sought by the 3867 * grantee. 3868 * 3869 * 3870 * Examples: 3871 * 3872 * 3873 * 3874 * Healthcare: A patient assent to grantee's consent directive terms for 3875 * collection, access, use, or disclosure of health information, and dissents to 3876 * disclosure to certain recipients as allowed by the provider's pre-approved 3877 * restriction list. Non-Healthcare: A cell phone user assents to the cell 3878 * phone's privacy practices and terms of use, but dissents from location 3879 * tracking by turning off the cell phone's tracking capability. 3880 */ 3881 OPTINR, 3882 /** 3883 * A grantor's dissent to the terms of agreement offered by a grantee without an 3884 * opportunity for to assent to any terms. 3885 * 3886 * 3887 * Comment: Rejection of a grantee's terms of agreement pertaining, for example, 3888 * to permissible activities, purposes of use, handling caveats, expiry date, 3889 * and revocation policies. 3890 * 3891 * 3892 * Usage Note: Opt-out with no opportunity for a grantor to permit certain 3893 * permissions sought by the grantee is considered "basic consent". 3894 * 3895 * 3896 * Examples: 3897 * 3898 * 3899 * 3900 * Healthcare: A patient [grantor] declines to sign a provider's [grantee's] 3901 * consent directive form, which lists permissible collection, access, use, or 3902 * disclosure activities, purposes of use, handling caveats, revocation 3903 * policies, and consequences of not assenting. Non-healthcare: An employee 3904 * [grantor] refuses to sign an employer's [grantee's] agreement not to join 3905 * unions or participate in a strike where state law protects employee's 3906 * collective bargaining rights. A citizen [grantor] refuses to enroll in 3907 * mandatory government [grantee] health insurance based on religious beliefs, 3908 * which is an exemption. 3909 */ 3910 OPTOUT, 3911 /** 3912 * A grantor's dissent to the grantee's terms of agreement except for certain 3913 * grantor or grantee selected terms. 3914 * 3915 * 3916 * Comment: A rejection of a grantee's terms of agreement while assenting to 3917 * certain permissions sought by the grantee or requesting approval of 3918 * additional grantor terms. 3919 * 3920 * 3921 * Usage Note: Opt-out with exceptions is considered a "granular consent" 3922 * because the grantor has an opportunity to accept certain permissions sought 3923 * by the grantee or request additional grantor terms, while rejecting other 3924 * grantee terms. 3925 * 3926 * 3927 * Examples: 3928 * 3929 * 3930 * 3931 * Healthcare: A patient [grantor] dissents to a health information exchange 3932 * consent directive with the exception of disclosure based on a limited "time 3933 * to live" shared secret [e.g., a token or password], which the patient can 3934 * give to a provider when seeking care. Non-healthcare: A social media user 3935 * [grantor] dissents from public access to their account, but assents to access 3936 * to a circle of friends. 3937 */ 3938 OPTOUTE, 3939 /** 3940 * A jurisdictional mandate, regulation, obligation, requirement, rule, or 3941 * expectation deeming certain information to be private to an individual or 3942 * organization, which is imposed on: 3943 * 3944 * 3945 * The activity of a governed party The behavior of a governed party The manner 3946 * in which an act is executed by a governed party 3947 */ 3948 _ACTPRIVACYLAW, 3949 /** 3950 * Definition: A jurisdictional mandate in the U.S. relating to privacy. 3951 * 3952 * 3953 * Usage Note: ActPrivacyLaw codes may be associated with an Act or a Role to 3954 * indicate the legal provision to which the assignment of an 3955 * Act.confidentialityCode or Role.confidentialtyCode complies. May be used to 3956 * further specify rationale for assignment of other ActPrivacyPolicy codes in 3957 * the US realm, e.g., ETH and 42CFRPart2 can be differentiated from ETH and 3958 * Title38Part1. 3959 */ 3960 _ACTUSPRIVACYLAW, 3961 /** 3962 * 42 CFR Part 2 stipulates the right of an individual who has applied for or 3963 * been given diagnosis or treatment for alcohol or drug abuse at a federally 3964 * assisted program. 3965 * 3966 * 3967 * Definition: Non-disclosure of health information relating to health care paid 3968 * for by a federally assisted substance abuse program without patient consent. 3969 * 3970 * 3971 * Usage Note: May be associated with an Act or a Role to indicate the legal 3972 * provision to which the assignment of an Act.confidentialityCode or 3973 * Role.confidentialityCode complies. 3974 */ 3975 _42CFRPART2, 3976 /** 3977 * U.S. Federal regulations governing the protection of human subjects in 3978 * research (codified at Subpart A of 45 CFR part 46) that has been adopted by 3979 * 15 U.S. Federal departments and agencies in an effort to promote uniformity, 3980 * understanding, and compliance with human subject protections. Existing 3981 * regulations governing the protection of human subjects in Food and Drug 3982 * Administration (FDA)-regulated research (21 CFR parts 50, 56, 312, and 812) 3983 * are separate from the Common Rule but include similar requirements. 3984 * 3985 * 3986 * Definition: U.S. federal laws governing research-related privacy policies. 3987 * 3988 * 3989 * Usage Note: May be associated with an Act or a Role to indicate the legal 3990 * provision to which the assignment of an Act.confidentialityCode or 3991 * Role.confidentialtyCode complies. 3992 */ 3993 COMMONRULE, 3994 /** 3995 * The U.S. Public Law 104-191 Health Insurance Portability and Accountability 3996 * Act (HIPAA) Privacy Rule (45 CFR Part 164 Subpart E) permits access, use and 3997 * disclosure of certain personal health information (PHI as defined under the 3998 * law) for purposes of Treatment, Payment, and Operations, and requires that 3999 * the provider ask that patients acknowledge the Provider's Notice of Privacy 4000 * Practices as permitted conduct under the law. 4001 * 4002 * 4003 * Definition: Notification of HIPAA Privacy Practices. 4004 * 4005 * 4006 * Usage Note: May be associated with an Act or a Role to indicate the legal 4007 * provision to which the assignment of an Act.confidentialityCode or 4008 * Role.confidentialtyCode complies. 4009 */ 4010 HIPAANOPP, 4011 /** 4012 * The U.S. Public Law 104-191 Health Insurance Portability and Accountability 4013 * Act (HIPAA) Privacy Rule (45 CFR Part 164 Section 164.508) requires 4014 * authorization for certain uses and disclosure of psychotherapy notes. 4015 * 4016 * 4017 * Definition: Authorization that must be obtained for disclosure of 4018 * psychotherapy notes. 4019 * 4020 * 4021 * Usage Note: May be associated with an Act or a Role to indicate the legal 4022 * provision to which the assignment of an Act.confidentialityCode or 4023 * Role.confidentialityCode complies. 4024 */ 4025 HIPAAPSYNOTES, 4026 /** 4027 * Section 13405(a) of the Health Information Technology for Economic and 4028 * Clinical Health Act (HITECH) stipulates the right of an individual to have 4029 * disclosures regarding certain health care items or services for which the 4030 * individual pays out of pocket in full restricted from a health plan. 4031 * 4032 * 4033 * Definition: Non-disclosure of health information to a health plan relating to 4034 * health care items or services for which an individual pays out of pocket in 4035 * full. 4036 * 4037 * 4038 * Usage Note: May be associated with an Act or a Role to indicate the legal 4039 * provision to which the assignment of an Act.confidentialityCode or 4040 * Role.confidentialityCode complies. 4041 */ 4042 HIPAASELFPAY, 4043 /** 4044 * Title 38 Part 1-protected information may only be disclosed to a third party 4045 * with the special written consent of the patient except where expressly 4046 * authorized by 38 USC 7332. VA may disclose this information for specific 4047 * purposes to: VA employees on a need to know basis - more restrictive than 4048 * Privacy Act need to know; contractors who need the information in order to 4049 * perform or fulfil the duties of the contract; and researchers who provide 4050 * assurances that the information will not be identified in any report. This 4051 * information may also be disclosed without consent where patient lacks 4052 * decision-making capacity; in a medical emergency for the purpose of treating 4053 * a condition which poses an immediate threat to the health of any individual 4054 * and which requires immediate medical intervention; for eye, tissue, or organ 4055 * donation purposes; and disclosure of HIV information for public health 4056 * purposes. 4057 * 4058 * 4059 * Definition: Title 38 Part 1 - Section 1.462 Confidentiality restrictions. 4060 * 4061 * (a) General. The patient records to which Sections 1.460 through 1.499 of 4062 * this part apply may be disclosed or used only as permitted by these 4063 * regulations and may not otherwise be disclosed or used in any civil, 4064 * criminal, administrative, or legislative proceedings conducted by any 4065 * Federal, State, or local authority. Any disclosure made under these 4066 * regulations must be limited to that information which is necessary to carry 4067 * out the purpose of the disclosure. SUBCHAPTER III--PROTECTION OF PATIENT 4068 * RIGHTS Sec. 7332. Confidentiality of certain medical records (a)(1) Records 4069 * of the identity, diagnosis, prognosis, or treatment of any patient or subject 4070 * which are maintained in connection with the performance of any program or 4071 * activity (including education, training, treatment, rehabilitation, or 4072 * research) relating to drug abuse, alcoholism or alcohol abuse, infection with 4073 * the human immunodeficiency virus, or sickle cell anemia which is carried out 4074 * by or for the Department under this title shall, except as provided in 4075 * subsections (e) and (f), be confidential, and (section 5701 of this title to 4076 * the contrary notwithstanding) such records may be disclosed only for the 4077 * purposes and under the circumstances expressly authorized under subsection 4078 * (b). 4079 * 4080 * 4081 * Usage Note: May be associated with an Act or a Role to indicate the legal 4082 * provision to which the assignment of an Act.confidentialityCode or 4083 * Role.confidentialityCode complies. 4084 */ 4085 TITLE38SECTION7332, 4086 /** 4087 * A mandate, obligation, requirement, rule, or expectation characterizing the 4088 * value or importance of a resource and may include its vulnerability. (Based 4089 * on ISO7498-2:1989. Note: The vulnerability of personally identifiable 4090 * sensitive information may be based on concerns that the unauthorized 4091 * disclosure may result in social stigmatization or discrimination.) 4092 * Description: Types of Sensitivity policy that apply to Acts or Roles. A 4093 * sensitivity policy is adopted by an enterprise or group of enterprises (a 4094 * 'policy domain') through a formal data use agreement that stipulates the 4095 * value, importance, and vulnerability of information. A sensitivity code 4096 * representing a sensitivity policy may be associated with criteria such as 4097 * categories of information or sets of information identifiers (e.g., a value 4098 * set of clinical codes or branch in a code system hierarchy). These criteria 4099 * may in turn be used for the Policy Decision Point in a Security Engine. A 4100 * sensitivity code may be used to set the confidentiality code used on 4101 * information about Acts and Roles to trigger the security mechanisms required 4102 * to control how security principals (i.e., a person, a machine, a software 4103 * application) may act on the information (e.g., collection, access, use, or 4104 * disclosure). Sensitivity codes are never assigned to the transport or 4105 * business envelope containing patient specific information being exchanged 4106 * outside of a policy domain as this would disclose the information intended to 4107 * be protected by the policy. When sensitive information is exchanged with 4108 * others outside of a policy domain, the confidentiality code on the transport 4109 * or business envelope conveys the receiver's responsibilities and indicates 4110 * the how the information is to be safeguarded without unauthorized disclosure 4111 * of the sensitive information. This ensures that sensitive information is 4112 * treated by receivers as the sender intends, accomplishing interoperability 4113 * without point to point negotiations. 4114 * 4115 * 4116 * Usage Note: Sensitivity codes are not useful for interoperability outside of 4117 * a policy domain because sensitivity policies are typically localized and vary 4118 * drastically across policy domains even for the same information category 4119 * because of differing organizational business rules, security policies, and 4120 * jurisdictional requirements. For example, an employee's sensitivity code 4121 * would make little sense for use outside of a policy domain. 'Taboo' would 4122 * rarely be useful outside of a policy domain unless there are jurisdictional 4123 * requirements requiring that a provider disclose sensitive information to a 4124 * patient directly. Sensitivity codes may be more appropriate in a legacy 4125 * system's Master Files in order to notify those who access a patient's orders 4126 * and observations about the sensitivity policies that apply. Newer systems may 4127 * have a security engine that uses a sensitivity policy's criteria directly. 4128 * The specializable InformationSensitivityPolicy Act.code may be useful in some 4129 * scenarios if used in combination with a sensitivity identifier and/or 4130 * Act.title. 4131 */ 4132 _INFORMATIONSENSITIVITYPOLICY, 4133 /** 4134 * Types of sensitivity policies that apply to Acts. Act.confidentialityCode is 4135 * defined in the RIM as "constraints around appropriate disclosure of 4136 * information about this Act, regardless of mood." 4137 * 4138 * 4139 * Usage Note: ActSensitivity codes are used to bind information to an 4140 * Act.confidentialityCode according to local sensitivity policy so that those 4141 * confidentiality codes can then govern its handling across enterprises. 4142 * Internally to a policy domain, however, local policies guide the access 4143 * control system on how end users in that policy domain are able to use 4144 * information tagged with these sensitivity values. 4145 */ 4146 _ACTINFORMATIONSENSITIVITYPOLICY, 4147 /** 4148 * Policy for handling alcohol or drug-abuse information, which will be afforded 4149 * heightened confidentiality. Information handling protocols based on 4150 * organizational policies related to alcohol or drug-abuse information that is 4151 * deemed sensitive. 4152 * 4153 * 4154 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4155 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4156 * this more generic code. 4157 */ 4158 ETH, 4159 /** 4160 * Policy for handling genetic disease information, which will be afforded 4161 * heightened confidentiality. Information handling protocols based on 4162 * organizational policies related to genetic disease information that is deemed 4163 * sensitive. 4164 * 4165 * 4166 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4167 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4168 * this more generic code. 4169 */ 4170 GDIS, 4171 /** 4172 * Policy for handling HIV or AIDS information, which will be afforded 4173 * heightened confidentiality. Information handling protocols based on 4174 * organizational policies related to HIV or AIDS information that is deemed 4175 * sensitive. 4176 * 4177 * 4178 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4179 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4180 * this more generic code. 4181 */ 4182 HIV, 4183 /** 4184 * Policy for handling information related to sexual assault or repeated, 4185 * threatening sexual harassment that occurred while the patient was in the 4186 * military, which is afforded heightened confidentiality. 4187 * 4188 * Access control concerns for military sexual trauma is based on the patient 4189 * being subject to control by a higher ranking military perpetrator and/or 4190 * censure by others within the military unit. Due to the relatively unfettered 4191 * access to healthcare information by higher ranking military personnel and 4192 * those who have command over the patient, there is a need to sequester this 4193 * information outside of the typical controls on access to military health 4194 * records. 4195 * 4196 * 4197 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4198 * ActPrivacyLaw code system, and specify the law in addition to this more 4199 * generic code. 4200 */ 4201 MST, 4202 /** 4203 * Policy for handling sickle cell disease information, which is afforded 4204 * heightened confidentiality. Information handling protocols are based on 4205 * organizational policies related to sickle cell disease information, which is 4206 * deemed sensitive. 4207 * 4208 * 4209 * Usage Note: If there is a jurisdictional mandate, then the Act valued with 4210 * this ActCode should be associated with an Act valued with any applicable laws 4211 * from the ActPrivacyLaw code system. 4212 */ 4213 SCA, 4214 /** 4215 * Policy for handling sexual assault, abuse, or domestic violence information, 4216 * which will be afforded heightened confidentiality. Information handling 4217 * protocols based on organizational policies related to sexual assault, abuse, 4218 * or domestic violence information that is deemed sensitive. 4219 * 4220 * SDV code covers violence perpetrated by related and non-related persons. This 4221 * code should be specific to physical and mental trauma caused by a related 4222 * person only. The access control concerns are keeping the patient safe from 4223 * the perpetrator who may have an abusive psychological control over the 4224 * patient, may be stalking the patient, or may try to manipulate care givers 4225 * into allowing the perpetrator to make contact with the patient. The 4226 * definition needs to be clarified. 4227 * 4228 * 4229 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4230 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4231 * this more generic code. 4232 */ 4233 SDV, 4234 /** 4235 * Policy for handling sexuality and reproductive health information, which will 4236 * be afforded heightened confidentiality. Information handling protocols based 4237 * on organizational policies related to sexuality and reproductive health 4238 * information that is deemed sensitive. 4239 * 4240 * 4241 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4242 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4243 * this more generic code. 4244 */ 4245 SEX, 4246 /** 4247 * Policy for handling information deemed specially protected by law or policy 4248 * including substance abuse, substance use, psychiatric, mental health, 4249 * behavioral health, and cognitive disorders, which is afforded heightened 4250 * confidentiality. 4251 * 4252 * 4253 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4254 * ActPrivacyLaw code system, and specify the law in addition to this more 4255 * generic code. 4256 */ 4257 SPI, 4258 /** 4259 * Policy for handling information related to behavioral and emotional 4260 * disturbances affecting social adjustment and physical health, which is 4261 * afforded heightened confidentiality. 4262 * 4263 * 4264 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4265 * ActPrivacyLaw code system, and specify the law in addition to this more 4266 * generic code. 4267 */ 4268 BH, 4269 /** 4270 * Policy for handling information related to cognitive disability disorders and 4271 * conditions caused by these disorders, which are afforded heightened 4272 * confidentiality. 4273 * 4274 * 4275 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4276 * ActPrivacyLaw code system, and specify the law in addition to this more 4277 * generic code. 4278 * 4279 * Examples may include dementia, traumatic brain injury, attention deficit, 4280 * hearing and visual disability such as dyslexia and other disorders and 4281 * related conditions which impair learning and self-sufficiency. However, the 4282 * cognitive disabilities to which this term may apply versus other behavioral 4283 * health categories varies by jurisdiction and organizational policy in part 4284 * due to overlap with other behavioral health conditions. Implementers should 4285 * constrain to those diagnoses applicable in the domain in which this code is 4286 * used. 4287 */ 4288 COGN, 4289 /** 4290 * Policy for handling information related to developmental disability disorders 4291 * and conditions caused by these disorders, which is afforded heightened 4292 * confidentiality. 4293 * 4294 * 4295 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4296 * ActPrivacyLaw code system, and specify the law in addition to this more 4297 * generic code. 4298 * 4299 * A diverse group of chronic conditions that are due to mental or physical 4300 * impairments impacting activities of daily living, self-care, language acuity, 4301 * learning, mobility, independent living and economic self-sufficiency. 4302 * Examples may include Down syndrome and Autism spectrum. However, the 4303 * developmental disabilities to which this term applies versus other behavioral 4304 * health categories varies by jurisdiction and organizational policy in part 4305 * due to overlap with other behavioral health conditions. Implementers should 4306 * constrain to those diagnoses applicable in the domain in which this code is 4307 * used. 4308 */ 4309 DVD, 4310 /** 4311 * Policy for handling information related to emotional disturbance disorders 4312 * and conditions caused by these disorders, which is afforded heightened 4313 * confidentiality. 4314 * 4315 * 4316 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4317 * ActPrivacyLaw code system, and specify the law in addition to this more 4318 * generic code. 4319 * 4320 * Typical used to characterize behavioral and mental health issues of 4321 * adolescents where the disorder may be temporarily diagnosed in order to avoid 4322 * the potential and unnecessary stigmatizing diagnoses of disorder long term. 4323 */ 4324 EMOTDIS, 4325 /** 4326 * Policy for handling information related to psychological disorders, which is 4327 * afforded heightened confidentiality. Mental health information may be deemed 4328 * specifically sensitive and distinct from physical health, substance use 4329 * disorders, and behavioral disabilities and disorders in some jurisdictions. 4330 * 4331 * 4332 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4333 * ActPrivacyLaw code system, and specify the law in addition to this more 4334 * generic code. 4335 */ 4336 MH, 4337 /** 4338 * Policy for handling psychiatry psychiatric disorder information, which is 4339 * afforded heightened confidentiality. 4340 * 4341 * 4342 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4343 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4344 * this more generic code. 4345 */ 4346 PSY, 4347 /** 4348 * Policy for handling psychotherapy note information, which is afforded 4349 * heightened confidentiality. 4350 * 4351 * 4352 * Usage Note: In some jurisdiction, disclosure of psychotherapy notes requires 4353 * patient consent. 4354 * 4355 * If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw 4356 * code system, and specify the law rather than or in addition to this more 4357 * generic code. 4358 */ 4359 PSYTHPN, 4360 /** 4361 * Policy for handling information related to alcohol or drug use disorders and 4362 * conditions caused by these disorders, which is afforded heightened 4363 * confidentiality. 4364 * 4365 * 4366 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4367 * ActPrivacyLaw code system, and specify the law in addition to this more 4368 * generic code. 4369 */ 4370 SUD, 4371 /** 4372 * Policy for handling information related to alcohol use disorders and 4373 * conditions caused by these disorders, which is afforded heightened 4374 * confidentiality. 4375 * 4376 * 4377 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4378 * ActPrivacyLaw code system, and specify the law in addition to this more 4379 * generic code. 4380 */ 4381 ETHUD, 4382 /** 4383 * Policy for handling information related to opioid use disorders and 4384 * conditions caused by these disorders, which is afforded heightened 4385 * confidentiality. 4386 * 4387 * 4388 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4389 * ActPrivacyLaw code system, and specify the law in addition to this more 4390 * generic code. 4391 */ 4392 OPIOIDUD, 4393 /** 4394 * Policy for handling sexually transmitted disease information, which will be 4395 * afforded heightened confidentiality. Information handling protocols based on 4396 * organizational policies related to sexually transmitted disease information 4397 * that is deemed sensitive. 4398 * 4399 * 4400 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4401 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4402 * this more generic code. 4403 */ 4404 STD, 4405 /** 4406 * Policy for handling information not to be initially disclosed or discussed 4407 * with patient except by a physician assigned to patient in this case. 4408 * Information handling protocols based on organizational policies related to 4409 * sensitive patient information that must be initially discussed with the 4410 * patient by an attending physician before being disclosed to the patient. 4411 * 4412 * 4413 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4414 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4415 * this more generic code. 4416 * 4417 * 4418 * Open Issue: This definition conflates a rule and a characteristic, and there 4419 * may be a similar issue with ts sibling codes. 4420 */ 4421 TBOO, 4422 /** 4423 * Policy for handling information related to harm by violence, which is 4424 * afforded heightened confidentiality. Harm by violence is perpetrated by an 4425 * unrelated person. 4426 * 4427 * Access control concerns for information about mental or physical harm 4428 * resulting from violence caused by an unrelated person may include 4429 * manipulation of care givers or access to records that enable the perpetrator 4430 * contact or locate the patient, but the perpetrator will likely not have 4431 * established abusive psychological control over the patient. 4432 * 4433 * 4434 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4435 * ActPrivacyLaw code system, and specify the law in addition to this more 4436 * generic code. 4437 */ 4438 VIO, 4439 /** 4440 * Types of sensitivity policies that apply to Acts. Act.confidentialityCode is 4441 * defined in the RIM as "constraints around appropriate disclosure of 4442 * information about this Act, regardless of mood." 4443 * 4444 * 4445 * Usage Note: ActSensitivity codes are used to bind information to an 4446 * Act.confidentialityCode according to local sensitivity policy so that those 4447 * confidentiality codes can then govern its handling across enterprises. 4448 * Internally to a policy domain, however, local policies guide the access 4449 * control system on how end users in that policy domain are able to use 4450 * information tagged with these sensitivity values. 4451 */ 4452 SICKLE, 4453 /** 4454 * Types of sensitivity policies that may apply to a sensitive attribute on an 4455 * Entity. 4456 * 4457 * 4458 * Usage Note: EntitySensitivity codes are used to convey a policy that is 4459 * applicable to sensitive information conveyed by an entity attribute. May be 4460 * used to bind a Role.confidentialityCode associated with an Entity per 4461 * organizational policy. Role.confidentialityCode is defined in the RIM as "an 4462 * indication of the appropriate disclosure of information about this Role with 4463 * respect to the playing Entity." 4464 */ 4465 _ENTITYSENSITIVITYPOLICYTYPE, 4466 /** 4467 * Policy for handling all demographic information about an information subject, 4468 * which will be afforded heightened confidentiality. Policies may govern 4469 * sensitivity of information related to all demographic about an information 4470 * subject, the disclosure of which could impact the privacy, well-being, or 4471 * safety of that subject. 4472 * 4473 * 4474 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4475 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4476 * this more generic code. 4477 */ 4478 DEMO, 4479 /** 4480 * Policy for handling information related to an information subject's date of 4481 * birth, which will be afforded heightened confidentiality.Policies may govern 4482 * sensitivity of information related to an information subject's date of birth, 4483 * the disclosure of which could impact the privacy, well-being, or safety of 4484 * that subject. 4485 * 4486 * 4487 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4488 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4489 * this more generic code. 4490 */ 4491 DOB, 4492 /** 4493 * Policy for handling information related to an information subject's gender 4494 * and sexual orientation, which will be afforded heightened confidentiality. 4495 * Policies may govern sensitivity of information related to an information 4496 * subject's gender and sexual orientation, the disclosure of which could impact 4497 * the privacy, well-being, or safety of that subject. 4498 * 4499 * 4500 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4501 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4502 * this more generic code. 4503 */ 4504 GENDER, 4505 /** 4506 * Policy for handling information related to an information subject's living 4507 * arrangement, which will be afforded heightened confidentiality. Policies may 4508 * govern sensitivity of information related to an information subject's living 4509 * arrangement, the disclosure of which could impact the privacy, well-being, or 4510 * safety of that subject. 4511 * 4512 * 4513 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4514 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4515 * this more generic code. 4516 */ 4517 LIVARG, 4518 /** 4519 * Policy for handling information related to an information subject's marital 4520 * status, which will be afforded heightened confidentiality. Policies may 4521 * govern sensitivity of information related to an information subject's marital 4522 * status, the disclosure of which could impact the privacy, well-being, or 4523 * safety of that subject. 4524 * 4525 * 4526 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4527 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4528 * this more generic code. 4529 */ 4530 MARST, 4531 /** 4532 * Policy for handling information related to an information subject's race, 4533 * which will be afforded heightened confidentiality. Policies may govern 4534 * sensitivity of information related to an information subject's race, the 4535 * disclosure of which could impact the privacy, well-being, or safety of that 4536 * subject. 4537 * 4538 * 4539 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4540 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4541 * this more generic code. 4542 */ 4543 RACE, 4544 /** 4545 * Policy for handling information related to an information subject's religious 4546 * affiliation, which will be afforded heightened confidentiality. Policies may 4547 * govern sensitivity of information related to an information subject's 4548 * religion, the disclosure of which could impact the privacy, well-being, or 4549 * safety of that subject. 4550 * 4551 * 4552 * Usage Notes: If there is a jurisdictional mandate, then use the applicable 4553 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4554 * this more generic code. 4555 */ 4556 REL, 4557 /** 4558 * Types of sensitivity policies that apply to Roles. 4559 * 4560 * 4561 * Usage Notes: RoleSensitivity codes are used to bind information to a 4562 * Role.confidentialityCode per organizational policy. Role.confidentialityCode 4563 * is defined in the RIM as "an indication of the appropriate disclosure of 4564 * information about this Role with respect to the playing Entity." 4565 */ 4566 _ROLEINFORMATIONSENSITIVITYPOLICY, 4567 /** 4568 * Policy for handling trade secrets such as financial information or 4569 * intellectual property, which will be afforded heightened confidentiality. 4570 * Description: Since the service class can represent knowledge structures that 4571 * may be considered a trade or business secret, there is sometimes (though 4572 * rarely) the need to flag those items as of business level confidentiality. 4573 * 4574 * 4575 * Usage Notes: No patient related information may ever be of this 4576 * confidentiality level. If there is a jurisdictional mandate, then use the 4577 * applicable ActPrivacyLaw code system, and specify the law rather than or in 4578 * addition to this more generic code. 4579 */ 4580 B, 4581 /** 4582 * Policy for handling information related to an employer which is deemed 4583 * classified to protect an employee who is the information subject, and which 4584 * will be afforded heightened confidentiality. Description: Policies may govern 4585 * sensitivity of information related to an employer, such as law enforcement or 4586 * national security, the identity of which could impact the privacy, 4587 * well-being, or safety of an information subject who is an employee. 4588 * 4589 * 4590 * Usage Notes: If there is a jurisdictional mandate, then use the applicable 4591 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4592 * this more generic code. 4593 */ 4594 EMPL, 4595 /** 4596 * Policy for handling information related to the location of the information 4597 * subject, which will be afforded heightened confidentiality. Description: 4598 * Policies may govern sensitivity of information related to the location of the 4599 * information subject, the disclosure of which could impact the privacy, 4600 * well-being, or safety of that subject. 4601 * 4602 * 4603 * Usage Notes: If there is a jurisdictional mandate, then use the applicable 4604 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4605 * this more generic code. 4606 */ 4607 LOCIS, 4608 /** 4609 * Policy for handling information related to a provider of sensitive services, 4610 * which will be afforded heightened confidentiality. Description: Policies may 4611 * govern sensitivity of information related to providers who deliver sensitive 4612 * healthcare services in order to protect the privacy, well-being, and safety 4613 * of the provider and of patients receiving sensitive services. 4614 * 4615 * 4616 * Usage Notes: If there is a jurisdictional mandate, then use the applicable 4617 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4618 * this more generic code. 4619 */ 4620 SSP, 4621 /** 4622 * Policy for handling information related to an adolescent, which will be 4623 * afforded heightened confidentiality per applicable organizational or 4624 * jurisdictional policy. An enterprise may have a policy that requires that 4625 * adolescent patient information be provided heightened confidentiality. 4626 * Information deemed sensitive typically includes health information and 4627 * patient role information including patient status, demographics, next of kin, 4628 * and location. 4629 * 4630 * 4631 * Usage Note: For use within an enterprise in which an adolescent is the 4632 * information subject. If there is a jurisdictional mandate, then use the 4633 * applicable ActPrivacyLaw code system, and specify the law rather than or in 4634 * addition to this more generic code. 4635 */ 4636 ADOL, 4637 /** 4638 * Policy for handling information related to a celebrity (people of public 4639 * interest (VIP), which will be afforded heightened confidentiality. 4640 * Celebrities are people of public interest (VIP) about whose information an 4641 * enterprise may have a policy that requires heightened confidentiality. 4642 * Information deemed sensitive may include health information and patient role 4643 * information including patient status, demographics, next of kin, and 4644 * location. 4645 * 4646 * 4647 * Usage Note: For use within an enterprise in which the information subject is 4648 * deemed a celebrity or very important person. If there is a jurisdictional 4649 * mandate, then use the applicable ActPrivacyLaw code system, and specify the 4650 * law rather than or in addition to this more generic code. 4651 */ 4652 CEL, 4653 /** 4654 * Policy for handling information related to a diagnosis, health condition or 4655 * health problem, which will be afforded heightened confidentiality. 4656 * Diagnostic, health condition or health problem related information may be 4657 * deemed sensitive by organizational policy, and require heightened 4658 * confidentiality. 4659 * 4660 * 4661 * Usage Note: For use within an enterprise that provides heightened 4662 * confidentiality to diagnostic, health condition or health problem related 4663 * information deemed sensitive. If there is a jurisdictional mandate, then use 4664 * the applicable ActPrivacyLaw code system, and specify the law rather than or 4665 * in addition to this more generic code. 4666 */ 4667 DIA, 4668 /** 4669 * Policy for handling information related to a drug, which will be afforded 4670 * heightened confidentiality. Drug information may be deemed sensitive by 4671 * organizational policy, and require heightened confidentiality. 4672 * 4673 * 4674 * Usage Note: For use within an enterprise that provides heightened 4675 * confidentiality to drug information deemed sensitive. If there is a 4676 * jurisdictional mandate, then use the applicable ActPrivacyLaw code system, 4677 * and specify the law rather than or in addition to this more generic code. 4678 */ 4679 DRGIS, 4680 /** 4681 * Policy for handling information related to an employee, which will be 4682 * afforded heightened confidentiality. When a patient is an employee, an 4683 * enterprise may have a policy that requires heightened confidentiality. 4684 * Information deemed sensitive typically includes health information and 4685 * patient role information including patient status, demographics, next of kin, 4686 * and location. 4687 * 4688 * 4689 * Usage Note: Policy for handling information related to an employee, which 4690 * will be afforded heightened confidentiality. Description: When a patient is 4691 * an employee, an enterprise may have a policy that requires heightened 4692 * confidentiality. Information deemed sensitive typically includes health 4693 * information and patient role information including patient status, 4694 * demographics, next of kin, and location. 4695 */ 4696 EMP, 4697 /** 4698 * Policy for specially protecting information reported by or about a patient, 4699 * which is deemed sensitive within the enterprise (i.e., by default regardless 4700 * of whether the patient requested that the information be deemed sensitive for 4701 * another reason.) For example information reported by the patient about 4702 * another person, e.g., a family member, may be deemed sensitive by default. 4703 * Organizational policy may allow the sensitivity tag to be cleared on 4704 * patient's request. 4705 * 4706 * 4707 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4708 * ActPrivacyLaw code system, and specify the law in addition to this more 4709 * generic code. 4710 * 4711 * For example, VA deems employee information sensitive by default. Information 4712 * about a patient who is being stalked or a victim of abuse or violence may be 4713 * deemed sensitive by default per a provider organization's policies. 4714 */ 4715 PDS, 4716 /** 4717 * Policy for handling information about a patient, which a physician or other 4718 * licensed healthcare provider deems sensitive. Once tagged by the provider, 4719 * this may trigger alerts for follow up actions according to organizational 4720 * policy or jurisdictional law. 4721 * 4722 * 4723 * Usage Note: For use within an enterprise that provides heightened 4724 * confidentiality to certain types of information designated by a physician as 4725 * sensitive. If there is a jurisdictional mandate, then use the applicable 4726 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4727 * this more generic code. 4728 * 4729 * Use cases in which this code could be used are, e.g., in systems that lack 4730 * the ability to automatically detect sensitive information and must rely on 4731 * manual tagging; a system that lacks an applicable sensitivity tag, or for ad 4732 * hoc situations where criticality of the situation requires that the tagging 4733 * be done immediately by the provider before coding or transcription of consult 4734 * notes can be completed, e.g., upon detection of a patient with suicidal 4735 * tendencies or potential for violence. 4736 */ 4737 PHY, 4738 /** 4739 * Policy for specially protecting information reported by or about a patient, 4740 * which the patient deems sensitive, and the patient requests that collection, 4741 * access, use, or disclosure of that information be restricted. For example, a 4742 * minor patient may request that information about reproductive health not be 4743 * disclosed to the patient's family or to particular providers and payers. 4744 * 4745 * 4746 * Usage Note: If there is a jurisdictional mandate, then use the applicable 4747 * ActPrivacyLaw code system, and specify the law rather than or in addition to 4748 * this more generic code. 4749 */ 4750 PRS, 4751 /** 4752 * This is the healthcare analog to the US Intelligence Community's concept of a 4753 * Special Access Program. Compartment codes may be used in as a field value in 4754 * an initiator's clearance to indicate permission to access and use an IT 4755 * Resource with a security label having the same compartment value in security 4756 * category label field. 4757 * 4758 * Map: Aligns with ISO 2382-8 definition of Compartment - "A division of data 4759 * into isolated blocks with separate security controls for the purpose of 4760 * reducing risk." 4761 */ 4762 COMPT, 4763 /** 4764 * A group of health care entities, which may include health care providers, 4765 * care givers, hospitals, facilities, health plans, and other health care 4766 * constituents who coordinate care for reimbursement based on quality metrics 4767 * for improving outcomes and lowering costs, and may be authorized to access 4768 * the consumer's health information because of membership in that group. 4769 * 4770 * Security Compartment Labels assigned to a consumer's information use in 4771 * accountable care workflows should be met or exceeded by the Security 4772 * Compartment attribute claimed by a participant in a an accountable care 4773 * workflow who is requesting access to that information 4774 */ 4775 ACOCOMPT, 4776 /** 4777 * Care coordination across participants in a care plan requires sharing of a 4778 * healthcare consumer's information specific to that workflow. A care team 4779 * member should only have access to that information while participating in 4780 * that workflow or for other authorized uses. 4781 * 4782 * Security Compartment Labels assigned to a consumer's information use in care 4783 * coordination workflows should be met or exceeded by the Security Compartment 4784 * attribute claimed by a participant in a care team member workflow who is 4785 * requesting access to that information 4786 */ 4787 CTCOMPT, 4788 /** 4789 * Financial management department members who have access to healthcare 4790 * consumer information as part of a patient account, billing and claims 4791 * workflows. 4792 * 4793 * Security Compartment Labels assigned to consumer information used in these 4794 * workflows should be met or exceeded by the Security Compartment attribute 4795 * claimed by a participant in a financial management workflow who is requesting 4796 * access to that information. 4797 */ 4798 FMCOMPT, 4799 /** 4800 * A security category label field value, which indicates that access and use of 4801 * an IT resource is restricted to members of human resources department or 4802 * workflow. 4803 */ 4804 HRCOMPT, 4805 /** 4806 * Providers and care givers who have an established relationship per criteria 4807 * determined by policy are considered to have an established care provision 4808 * relations with a healthcare consumer, and may be authorized to access the 4809 * consumer's health information because of that relationship. Providers and 4810 * care givers should only have access to that information while participating 4811 * in legitimate relationship workflows or for other authorized uses. 4812 * 4813 * Security Compartment Labels assigned to a consumer's information use in 4814 * legitimate relationship workflows should be met or exceeded by the Security 4815 * Compartment attribute claimed by a participant in a legitimate relationship 4816 * workflow who is requesting access to that information. 4817 */ 4818 LRCOMPT, 4819 /** 4820 * Patient administration members who have access to healthcare consumer 4821 * information as part of a patient administration workflows. 4822 * 4823 * Security Compartment Labels assigned to consumer information used in these 4824 * workflows should be met or exceeded by the Security Compartment attribute 4825 * claimed by a participant in a patient administration workflow who is 4826 * requesting access to that information. 4827 */ 4828 PACOMPT, 4829 /** 4830 * A security category label field value, which indicates that access and use of 4831 * an IT resource is restricted to members of a research project. 4832 */ 4833 RESCOMPT, 4834 /** 4835 * A security category label field value, which indicates that access and use of 4836 * an IT resource is restricted to members of records management department or 4837 * workflow. 4838 */ 4839 RMGTCOMPT, 4840 /** 4841 * A mandate, obligation, requirement, rule, or expectation conveyed as security 4842 * metadata between senders and receivers required to establish the reliability, 4843 * authenticity, and trustworthiness of their transactions. 4844 * 4845 * Trust security metadata are observation made about aspects of trust 4846 * applicable to an IT resource (data, information object, service, or system 4847 * capability). 4848 * 4849 * Trust applicable to IT resources is established and maintained in and among 4850 * security domains, and may be comprised of observations about the domain's 4851 * trust authority, trust framework, trust policy, trust interaction rules, 4852 * means for assessing and monitoring adherence to trust policies, mechanisms 4853 * that enforce trust, and quality and reliability measures of assurance in 4854 * those mechanisms. [Based on ISO IEC 10181-1 and NIST SP 800-63-2] 4855 * 4856 * For example, identity proofing , level of assurance, and Trust Framework. 4857 */ 4858 ACTTRUSTPOLICYTYPE, 4859 /** 4860 * Type of security metadata about the formal declaration by an authority or 4861 * neutral third party that validates the technical, security, trust, and 4862 * business practice conformance of Trust Agents to facilitate security, 4863 * interoperability, and trust among participants within a security domain or 4864 * trust framework. 4865 */ 4866 TRSTACCRD, 4867 /** 4868 * Type of security metadata about privacy and security requirements with which 4869 * a security domain must comply. [ISO IEC 10181-1] 4870 */ 4871 TRSTAGRE, 4872 /** 4873 * Type of security metadata about the digital quality or reliability of a trust 4874 * assertion, activity, capability, information exchange, mechanism, process, or 4875 * protocol. 4876 */ 4877 TRSTASSUR, 4878 /** 4879 * Type of security metadata about a set of security-relevant data issued by a 4880 * security authority or trusted third party, together with security information 4881 * which is used to provide the integrity and data origin authentication 4882 * services for an IT resource (data, information object, service, or system 4883 * capability). [Based on ISO IEC 10181-1] 4884 */ 4885 TRSTCERT, 4886 /** 4887 * Type of security metadata about a complete set of contracts, regulations, or 4888 * commitments that enable participating actors to rely on certain assertions by 4889 * other actors to fulfill their information security requirements. [Kantara 4890 * Initiative] 4891 */ 4892 TRSTFWK, 4893 /** 4894 * Type of security metadata about a security architecture system component that 4895 * supports enforcement of security policies. 4896 */ 4897 TRSTMEC, 4898 /** 4899 * Description:A mandate, obligation, requirement, rule, or expectation 4900 * unilaterally imposed on benefit coverage under a policy or program by a 4901 * sponsor, underwriter or payor on: 4902 * 4903 * 4904 * 4905 * The activity of another party 4906 * 4907 * 4908 * 4909 * The behavior of another party 4910 * 4911 * 4912 * 4913 * The manner in which an act is executed 4914 * 4915 * 4916 * 4917 * 4918 * Examples:A clinical protocol imposed by a payer to which a provider must 4919 * adhere in order to be paid for providing the service. A formulary from which 4920 * a provider must select prescribed drugs in order for the patient to incur a 4921 * lower copay. 4922 */ 4923 COVPOL, 4924 /** 4925 * Types of security policies that further specify the ActClassPolicy value set. 4926 * 4927 * 4928 * Examples: 4929 * 4930 * 4931 * 4932 * obligation to encrypt refrain from redisclosure without consent 4933 */ 4934 SECURITYPOLICY, 4935 /** 4936 * Authorisation policies are essentially security policies related to 4937 * access-control and specify what activities a subject is permitted or 4938 * forbidden to do, to a set of target objects. They are designed to protect 4939 * target objects so are interpreted by access control agents or the run-time 4940 * systems at the target system. 4941 * 4942 * A positive authorisation policy defines the actions that a subject is 4943 * permitted to perform on a target. A negative authorisation policy specifies 4944 * the actions that a subject is forbidden to perform on a target. Positive 4945 * authorisation policies may also include filters to transform the parameters 4946 * associated with their actions. (Based on PONDERS) 4947 */ 4948 AUTHPOL, 4949 /** 4950 * An access control policy specific to the type of access control scheme, which 4951 * is used to enforce one or more authorization policies. 4952 * 4953 * 4954 * Usage Note: Access control schemes are the type of access control policy, 4955 * which is comprised of access control policy rules concerning the provision of 4956 * the access control service. 4957 * 4958 * There are two categories of access control policies, rule-based and 4959 * identity-based, which are identified in CCITT Rec. X.800 aka ISO 7498-2. 4960 * Rule-based access control policies are intended to apply to all access 4961 * requests by any initiator on any target in a security domain. Identity-based 4962 * access control policies are based on rules specific to an individual 4963 * initiator, a group of initiators, entities acting on behalf of initiators, or 4964 * originators acting in a specific role. Context can modify rule-based or 4965 * identity-based access control policies. Context rules may define the entire 4966 * policy in effect. Real systems will usually employ a combination of these 4967 * policy types; if a rule-based policy is used, then an identity-based policy 4968 * is usually in effect also. 4969 * 4970 * An access control scheme may be based on access control lists, capabilities, 4971 * labels, and context or a combination of these. An access control scheme is a 4972 * component of an access control mechanism or "service") along with the 4973 * supporting mechanisms required by that scheme to provide access control 4974 * decision information (ADI) supplied by the scheme to the access decision 4975 * facility (ADF also known as a PDP). (Based on ISO/IEC 10181-3:1996) 4976 * 4977 * 4978 * Examples: 4979 * 4980 * 4981 * 4982 * Attribute Based Access Control (ABAC) Discretionary Access Control (DAC) 4983 * History Based Access Control (HBAC) Identity Based Access Control (IBAC) 4984 * Mandatory Access Control (MAC) Organization Based Access Control (OrBAC) 4985 * Relationship Based Access Control (RelBac) Responsibility Based Access 4986 * Control (RespBAC) Risk Adaptable Access Control (RAdAC) > 4987 */ 4988 ACCESSCONSCHEME, 4989 /** 4990 * Delegation policies specify which actions subjects are allowed to delegate to 4991 * others. A delegation policy thus specifies an authorisation to delegate. 4992 * Subjects must already possess the access rights to be delegated. 4993 * 4994 * Delegation policies are aimed at subjects delegating rights to servers or 4995 * third parties to perform actions on their behalf and are not meant to be the 4996 * means by which security administrators would assign rights to subjects. A 4997 * negative delegation policy identifies what delegations are forbidden. 4998 * 4999 * A Delegation policy specifies the authorisation policy from which delegated 5000 * rights are derived, the grantors, which are the entities which can delegate 5001 * these access rights, and the grantees, which are the entities to which the 5002 * access rights can be delegated. There are two types of delegation policy, 5003 * positive and negative. (Based on PONDERS) 5004 */ 5005 DELEPOL, 5006 /** 5007 * Conveys the mandated workflow action that an information custodian, receiver, 5008 * or user must perform. 5009 * 5010 * 5011 * Usage Notes: Per ISO 22600-2, ObligationPolicy instances 'are event-triggered 5012 * and define actions to be performed by manager agent'. Per HL7 Composite 5013 * Security and Privacy Domain Analysis Model: This value set refers to the 5014 * action required to receive the permission specified in the privacy rule. Per 5015 * OASIS XACML, an obligation is an operation specified in a policy or policy 5016 * that is performed in conjunction with the enforcement of an access control 5017 * decision. 5018 */ 5019 OBLIGATIONPOLICY, 5020 /** 5021 * Custodian system must remove any information that could result in identifying 5022 * the information subject. 5023 */ 5024 ANONY, 5025 /** 5026 * Custodian system must make available to an information subject upon request 5027 * an accounting of certain disclosures of the individualâ??s protected health 5028 * information over a period of time. Policy may dictate that the accounting 5029 * include information about the information disclosed, the date of disclosure, 5030 * the identification of the receiver, the purpose of the disclosure, the time 5031 * in which the disclosing entity must provide a response and the time period 5032 * for which accountings of disclosure can be requested. 5033 */ 5034 AOD, 5035 /** 5036 * Custodian system must monitor systems to ensure that all users are authorized 5037 * to operate on information objects. 5038 */ 5039 AUDIT, 5040 /** 5041 * Custodian system must monitor and maintain retrievable log for each user and 5042 * operation on information. 5043 */ 5044 AUDTR, 5045 /** 5046 * Custodian security system must retrieve, evaluate, and comply with the 5047 * information handling directions of the Confidentiality Code associated with 5048 * an information target. 5049 */ 5050 CPLYCC, 5051 /** 5052 * Custodian security system must retrieve, evaluate, and comply with applicable 5053 * information subject consent directives. 5054 */ 5055 CPLYCD, 5056 /** 5057 * Custodian security system must retrieve, evaluate, and comply with applicable 5058 * jurisdictional privacy policies associated with the target information. 5059 */ 5060 CPLYJPP, 5061 /** 5062 * Custodian security system must retrieve, evaluate, and comply with applicable 5063 * organizational privacy policies associated with the target information. 5064 */ 5065 CPLYOPP, 5066 /** 5067 * Custodian security system must retrieve, evaluate, and comply with the 5068 * organizational security policies associated with the target information. 5069 */ 5070 CPLYOSP, 5071 /** 5072 * Custodian security system must retrieve, evaluate, and comply with applicable 5073 * policies associated with the target information. 5074 */ 5075 CPLYPOL, 5076 /** 5077 * Custodian security system must declassify information assigned security 5078 * labels by instantiating a new version of the classified information so as to 5079 * break the binding of the classifying security label when assigning a new 5080 * security label that marks the information as unclassified in accordance with 5081 * applicable jurisdictional privacy policies associated with the target 5082 * information. The system must retain an immutable record of the previous 5083 * assignment and binding. 5084 */ 5085 DECLASSIFYLABEL, 5086 /** 5087 * Custodian system must strip information of data that would allow the 5088 * identification of the source of the information or the information subject. 5089 */ 5090 DEID, 5091 /** 5092 * Custodian system must remove target information from access after use. 5093 */ 5094 DELAU, 5095 /** 5096 * Custodian security system must downgrade information assigned security labels 5097 * by instantiating a new version of the classified information so as to break 5098 * the binding of the classifying security label when assigning a new security 5099 * label that marks the information as classified at a less protected level in 5100 * accordance with applicable jurisdictional privacy policies associated with 5101 * the target information. The system must retain an immutable record of the 5102 * previous assignment and binding. 5103 */ 5104 DOWNGRDLABEL, 5105 /** 5106 * Custodian security system must assign and bind security labels derived from 5107 * compilations of information by aggregation or disaggregation in order to 5108 * classify information compiled in the information systems under its control 5109 * for collection, access, use and disclosure in accordance with applicable 5110 * jurisdictional privacy policies associated with the target information. The 5111 * system must retain an immutable record of the previous assignment and 5112 * binding. 5113 */ 5114 DRIVLABEL, 5115 /** 5116 * Custodian system must render information unreadable by algorithmically 5117 * transforming plaintext into ciphertext. 5118 * 5119 * 5120 * 5121 * 5122 * Usage Notes: A mathematical transposition of a file or data stream so that it 5123 * cannot be deciphered at the receiving end without the proper key. Encryption 5124 * is a security feature that assures that only the parties who are supposed to 5125 * be participating in a videoconference or data transfer are able to do so. It 5126 * can include a password, public and private keys, or a complex combination of 5127 * all. (Per Infoway.) 5128 */ 5129 ENCRYPT, 5130 /** 5131 * Custodian system must render information unreadable and unusable by 5132 * algorithmically transforming plaintext into ciphertext when "at rest" or in 5133 * storage. 5134 */ 5135 ENCRYPTR, 5136 /** 5137 * Custodian system must render information unreadable and unusable by 5138 * algorithmically transforming plaintext into ciphertext while "in transit" or 5139 * being transported by any means. 5140 */ 5141 ENCRYPTT, 5142 /** 5143 * Custodian system must render information unreadable and unusable by 5144 * algorithmically transforming plaintext into ciphertext while in use such that 5145 * operations permitted on the target information are limited by the license 5146 * granted to the end user. 5147 */ 5148 ENCRYPTU, 5149 /** 5150 * Custodian system must require human review and approval for permission 5151 * requested. 5152 */ 5153 HUAPRV, 5154 /** 5155 * Custodian security system must assign and bind security labels in order to 5156 * classify information created in the information systems under its control for 5157 * collection, access, use and disclosure in accordance with applicable 5158 * jurisdictional privacy policies associated with the target information. The 5159 * system must retain an immutable record of the assignment and binding. 5160 * 5161 * 5162 * Usage Note: In security systems, security policy label assignments do not 5163 * change, they may supersede prior assignments, and such reassignments are 5164 * always tracked for auditing and other purposes. 5165 */ 5166 LABEL, 5167 /** 5168 * Custodian system must render information unreadable and unusable by 5169 * algorithmically transforming plaintext into ciphertext. User may be provided 5170 * a key to decrypt per license or "shared secret". 5171 */ 5172 MASK, 5173 /** 5174 * Custodian must limit access and disclosure to the minimum information 5175 * required to support an authorized user's purpose of use. 5176 * 5177 * 5178 * Usage Note: Limiting the information available for access and disclosure to 5179 * that an authorized user or receiver "needs to know" in order to perform 5180 * permitted workflow or purpose of use. 5181 */ 5182 MINEC, 5183 /** 5184 * Custodian security system must persist the binding of security labels to 5185 * classify information received or imported by information systems under its 5186 * control for collection, access, use and disclosure in accordance with 5187 * applicable jurisdictional privacy policies associated with the target 5188 * information. The system must retain an immutable record of the assignment and 5189 * binding. 5190 */ 5191 PERSISTLABEL, 5192 /** 5193 * Custodian must create and/or maintain human readable security label tags as 5194 * required by policy. 5195 * 5196 * Map: Aligns with ISO 22600-3 Section A.3.4.3 description of privacy mark: "If 5197 * present, the privacy-mark is not used for access control. The content of the 5198 * privacy-mark may be defined by the security policy in force (identified by 5199 * the security-policy-identifier) which may define a list of values to be used. 5200 * Alternately, the value may be determined by the originator of the 5201 * security-label." 5202 */ 5203 PRIVMARK, 5204 /** 5205 * Custodian system must strip information of data that would allow the 5206 * identification of the source of the information or the information subject. 5207 * Custodian may retain a key to relink data necessary to reidentify the 5208 * information subject. 5209 */ 5210 PSEUD, 5211 /** 5212 * Custodian system must remove information, which is not authorized to be 5213 * access, used, or disclosed from records made available to otherwise 5214 * authorized users. 5215 */ 5216 REDACT, 5217 /** 5218 * Custodian security system must declassify information assigned security 5219 * labels by instantiating a new version of the classified information so as to 5220 * break the binding of the classifying security label when assigning a new 5221 * security label that marks the information as classified at a more protected 5222 * level in accordance with applicable jurisdictional privacy policies 5223 * associated with the target information. The system must retain an immutable 5224 * record of the previous assignment and binding. 5225 */ 5226 UPGRDLABEL, 5227 /** 5228 * Conveys prohibited actions which an information custodian, receiver, or user 5229 * is not permitted to perform unless otherwise authorized or permitted under 5230 * specified circumstances. 5231 * 5232 * 5233 * 5234 * 5235 * Usage Notes: ISO 22600-2 species that a Refrain Policy "defines actions the 5236 * subjects must refrain from performing". Per HL7 Composite Security and 5237 * Privacy Domain Analysis Model: May be used to indicate that a specific action 5238 * is prohibited based on specific access control attributes e.g., purpose of 5239 * use, information type, user role, etc. 5240 */ 5241 REFRAINPOLICY, 5242 /** 5243 * Prohibition on disclosure without information subject's authorization. 5244 */ 5245 NOAUTH, 5246 /** 5247 * Prohibition on collection or storage of the information. 5248 */ 5249 NOCOLLECT, 5250 /** 5251 * Prohibition on disclosure without organizational approved patient 5252 * restriction. 5253 */ 5254 NODSCLCD, 5255 /** 5256 * Prohibition on disclosure without a consent directive from the information 5257 * subject. 5258 */ 5259 NODSCLCDS, 5260 /** 5261 * Prohibition on Integration into other records. 5262 */ 5263 NOINTEGRATE, 5264 /** 5265 * Prohibition on disclosure except to entities on specific access list. 5266 */ 5267 NOLIST, 5268 /** 5269 * Prohibition on disclosure without an interagency service agreement or 5270 * memorandum of understanding (MOU). 5271 */ 5272 NOMOU, 5273 /** 5274 * Prohibition on disclosure without organizational authorization. 5275 */ 5276 NOORGPOL, 5277 /** 5278 * Prohibition on disclosing information to patient, family or caregivers 5279 * without attending provider's authorization. 5280 * 5281 * 5282 * Usage Note: The information may be labeled with the ActInformationSensitivity 5283 * TBOO code, triggering application of this RefrainPolicy code as a handling 5284 * caveat controlling access. 5285 * 5286 * Maps to FHIR NOPAT: Typically, this is used on an Alert resource, when the 5287 * alert records information on patient abuse or non-compliance. 5288 * 5289 * FHIR print name is "keep information from patient". Maps to the French realm 5290 * - code: INVISIBLE_PATIENT. 5291 * 5292 * 5293 * displayName: Document non visible par le patient codingScheme: 5294 * 1.2.250.1.213.1.1.4.13 5295 * 5296 * French use case: A label for documents that the author chose to hide from the 5297 * patient until the content can be disclose to the patient in a face to face 5298 * meeting between a healthcare professional and the patient (in French law some 5299 * results like cancer diagnosis or AIDS diagnosis must be announced to the 5300 * patient by a healthcare professional and should not be find out by the 5301 * patient alone). 5302 */ 5303 NOPAT, 5304 /** 5305 * Prohibition on collection of the information beyond time necessary to 5306 * accomplish authorized purpose of use is prohibited. 5307 */ 5308 NOPERSISTP, 5309 /** 5310 * Prohibition on redisclosure without patient consent directive. 5311 */ 5312 NORDSCLCD, 5313 /** 5314 * Prohibition on redisclosure without a consent directive from the information 5315 * subject. 5316 */ 5317 NORDSCLCDS, 5318 /** 5319 * Prohibition on disclosure without authorization under jurisdictional law. 5320 */ 5321 NORDSCLW, 5322 /** 5323 * Prohibition on associating de-identified or pseudonymized information with 5324 * other information in a manner that could or does result in disclosing 5325 * information intended to be masked. 5326 */ 5327 NORELINK, 5328 /** 5329 * Prohibition on use of the information beyond the purpose of use initially 5330 * authorized. 5331 */ 5332 NOREUSE, 5333 /** 5334 * Prohibition on disclosure except to principals with access permission to 5335 * specific VIP information. 5336 */ 5337 NOVIP, 5338 /** 5339 * Prohibition on disclosure except as permitted by the information originator. 5340 */ 5341 ORCON, 5342 /** 5343 * The method that a product is obtained for use by the subject of the supply 5344 * act (e.g. patient). Product examples are consumable or durable goods. 5345 */ 5346 _ACTPRODUCTACQUISITIONCODE, 5347 /** 5348 * Temporary supply of a product without transfer of ownership for the product. 5349 */ 5350 LOAN, 5351 /** 5352 * Temporary supply of a product with financial compensation, without transfer 5353 * of ownership for the product. 5354 */ 5355 RENT, 5356 /** 5357 * Transfer of ownership for a product. 5358 */ 5359 TRANSFER, 5360 /** 5361 * Transfer of ownership for a product for financial compensation. 5362 */ 5363 SALE, 5364 /** 5365 * Transportation of a specimen. 5366 */ 5367 _ACTSPECIMENTRANSPORTCODE, 5368 /** 5369 * Description:Specimen has been received by the participating 5370 * organization/department. 5371 */ 5372 SREC, 5373 /** 5374 * Description:Specimen has been placed into storage at a participating 5375 * location. 5376 */ 5377 SSTOR, 5378 /** 5379 * Description:Specimen has been put in transit to a participating receiver. 5380 */ 5381 STRAN, 5382 /** 5383 * Set of codes related to specimen treatments 5384 */ 5385 _ACTSPECIMENTREATMENTCODE, 5386 /** 5387 * The lowering of specimen pH through the addition of an acid 5388 */ 5389 ACID, 5390 /** 5391 * The act rendering alkaline by impregnating with an alkali; a conferring of 5392 * alkaline qualities. 5393 */ 5394 ALK, 5395 /** 5396 * The removal of fibrin from whole blood or plasma through physical or chemical 5397 * means 5398 */ 5399 DEFB, 5400 /** 5401 * The passage of a liquid through a filter, accomplished by gravity, pressure 5402 * or vacuum (suction). 5403 */ 5404 FILT, 5405 /** 5406 * LDL Precipitation 5407 */ 5408 LDLP, 5409 /** 5410 * The act or process by which an acid and a base are combined in such 5411 * proportions that the resulting compound is neutral. 5412 */ 5413 NEUT, 5414 /** 5415 * The addition of calcium back to a specimen after it was removed by chelating 5416 * agents 5417 */ 5418 RECA, 5419 /** 5420 * The filtration of a colloidal substance through a semipermeable medium that 5421 * allows only the passage of small molecules. 5422 */ 5423 UFIL, 5424 /** 5425 * Description: Describes the type of substance administration being performed. 5426 * This should not be used to carry codes for identification of products. Use an 5427 * associated role or entity to carry such information. 5428 */ 5429 _ACTSUBSTANCEADMINISTRATIONCODE, 5430 /** 5431 * The introduction of a drug into a subject with the intention of altering its 5432 * biologic state with the intent of improving its health status. 5433 */ 5434 DRUG, 5435 /** 5436 * Description: The introduction of material into a subject with the intent of 5437 * providing nutrition or other dietary supplements (e.g. minerals or vitamins). 5438 */ 5439 FD, 5440 /** 5441 * The introduction of an immunogen with the intent of stimulating an immune 5442 * response, aimed at preventing subsequent infections by more viable agents. 5443 */ 5444 IMMUNIZ, 5445 /** 5446 * An additional immunization administration within a series intended to bolster 5447 * or enhance immunity. 5448 */ 5449 BOOSTER, 5450 /** 5451 * The first immunization administration in a series intended to produce 5452 * immunity 5453 */ 5454 INITIMMUNIZ, 5455 /** 5456 * Description: A task or action that a user may perform in a clinical 5457 * information system (e.g., medication order entry, laboratory test results 5458 * review, problem list entry). 5459 */ 5460 _ACTTASKCODE, 5461 /** 5462 * A clinician creates a request for a service to be performed for a given 5463 * patient. 5464 */ 5465 OE, 5466 /** 5467 * A clinician creates a request for a laboratory test to be done for a given 5468 * patient. 5469 */ 5470 LABOE, 5471 /** 5472 * A clinician creates a request for the administration of one or more 5473 * medications to a given patient. 5474 */ 5475 MEDOE, 5476 /** 5477 * A person enters documentation about a given patient. 5478 */ 5479 PATDOC, 5480 /** 5481 * Description: A person reviews a list of known allergies of a given patient. 5482 */ 5483 ALLERLREV, 5484 /** 5485 * A clinician enters a clinical note about a given patient 5486 */ 5487 CLINNOTEE, 5488 /** 5489 * A clinician enters a diagnosis for a given patient. 5490 */ 5491 DIAGLISTE, 5492 /** 5493 * A person provides a discharge instruction to a patient. 5494 */ 5495 DISCHINSTE, 5496 /** 5497 * A clinician enters a discharge summary for a given patient. 5498 */ 5499 DISCHSUME, 5500 /** 5501 * A person provides a patient-specific education handout to a patient. 5502 */ 5503 PATEDUE, 5504 /** 5505 * A pathologist enters a report for a given patient. 5506 */ 5507 PATREPE, 5508 /** 5509 * A clinician enters a problem for a given patient. 5510 */ 5511 PROBLISTE, 5512 /** 5513 * A radiologist enters a report for a given patient. 5514 */ 5515 RADREPE, 5516 /** 5517 * Description: A person reviews a list of immunizations due or received for a 5518 * given patient. 5519 */ 5520 IMMLREV, 5521 /** 5522 * Description: A person reviews a list of health care reminders for a given 5523 * patient. 5524 */ 5525 REMLREV, 5526 /** 5527 * Description: A person reviews a list of wellness or preventive care reminders 5528 * for a given patient. 5529 */ 5530 WELLREMLREV, 5531 /** 5532 * A person (e.g., clinician, the patient herself) reviews patient information 5533 * in the electronic medical record. 5534 */ 5535 PATINFO, 5536 /** 5537 * Description: A person enters a known allergy for a given patient. 5538 */ 5539 ALLERLE, 5540 /** 5541 * A person reviews a recommendation/assessment provided automatically by a 5542 * clinical decision support application for a given patient. 5543 */ 5544 CDSREV, 5545 /** 5546 * A person reviews a clinical note of a given patient. 5547 */ 5548 CLINNOTEREV, 5549 /** 5550 * A person reviews a discharge summary of a given patient. 5551 */ 5552 DISCHSUMREV, 5553 /** 5554 * A person reviews a list of diagnoses of a given patient. 5555 */ 5556 DIAGLISTREV, 5557 /** 5558 * Description: A person enters an immunization due or received for a given 5559 * patient. 5560 */ 5561 IMMLE, 5562 /** 5563 * A person reviews a list of laboratory results of a given patient. 5564 */ 5565 LABRREV, 5566 /** 5567 * A person reviews a list of microbiology results of a given patient. 5568 */ 5569 MICRORREV, 5570 /** 5571 * A person reviews organisms of microbiology results of a given patient. 5572 */ 5573 MICROORGRREV, 5574 /** 5575 * A person reviews the sensitivity test of microbiology results of a given 5576 * patient. 5577 */ 5578 MICROSENSRREV, 5579 /** 5580 * A person reviews a list of medication orders submitted to a given patient 5581 */ 5582 MLREV, 5583 /** 5584 * A clinician reviews a work list of medications to be administered to a given 5585 * patient. 5586 */ 5587 MARWLREV, 5588 /** 5589 * A person reviews a list of orders submitted to a given patient. 5590 */ 5591 OREV, 5592 /** 5593 * A person reviews a pathology report of a given patient. 5594 */ 5595 PATREPREV, 5596 /** 5597 * A person reviews a list of problems of a given patient. 5598 */ 5599 PROBLISTREV, 5600 /** 5601 * A person reviews a radiology report of a given patient. 5602 */ 5603 RADREPREV, 5604 /** 5605 * Description: A person enters a health care reminder for a given patient. 5606 */ 5607 REMLE, 5608 /** 5609 * Description: A person enters a wellness or preventive care reminder for a 5610 * given patient. 5611 */ 5612 WELLREMLE, 5613 /** 5614 * A person reviews a Risk Assessment Instrument report of a given patient. 5615 */ 5616 RISKASSESS, 5617 /** 5618 * A person reviews a Falls Risk Assessment Instrument report of a given 5619 * patient. 5620 */ 5621 FALLRISK, 5622 /** 5623 * Characterizes how a transportation act was or will be carried out. 5624 * 5625 * 5626 * Examples: Via private transport, via public transit, via courier. 5627 */ 5628 _ACTTRANSPORTATIONMODECODE, 5629 /** 5630 * Definition: Characterizes how a patient was or will be transported to the 5631 * site of a patient encounter. 5632 * 5633 * 5634 * Examples: Via ambulance, via public transit, on foot. 5635 */ 5636 _ACTPATIENTTRANSPORTATIONMODECODE, 5637 /** 5638 * pedestrian transport 5639 */ 5640 AFOOT, 5641 /** 5642 * ambulance transport 5643 */ 5644 AMBT, 5645 /** 5646 * fixed-wing ambulance transport 5647 */ 5648 AMBAIR, 5649 /** 5650 * ground ambulance transport 5651 */ 5652 AMBGRND, 5653 /** 5654 * helicopter ambulance transport 5655 */ 5656 AMBHELO, 5657 /** 5658 * law enforcement transport 5659 */ 5660 LAWENF, 5661 /** 5662 * private transport 5663 */ 5664 PRVTRN, 5665 /** 5666 * public transport 5667 */ 5668 PUBTRN, 5669 /** 5670 * Identifies the kinds of observations that can be performed 5671 */ 5672 _OBSERVATIONTYPE, 5673 /** 5674 * Identifies the type of observation that is made about a specimen that may 5675 * affect its processing, analysis or further result interpretation 5676 */ 5677 _ACTSPECOBSCODE, 5678 /** 5679 * Describes the artificial blood identifier that is associated with the 5680 * specimen. 5681 */ 5682 ARTBLD, 5683 /** 5684 * An observation that reports the dilution of a sample. 5685 */ 5686 DILUTION, 5687 /** 5688 * The dilution of a sample performed by automated equipment. The value is 5689 * specified by the equipment 5690 */ 5691 AUTOHIGH, 5692 /** 5693 * The dilution of a sample performed by automated equipment. The value is 5694 * specified by the equipment 5695 */ 5696 AUTOLOW, 5697 /** 5698 * The dilution of the specimen made prior to being loaded onto analytical 5699 * equipment 5700 */ 5701 PRE, 5702 /** 5703 * The value of the dilution of a sample after it had been analyzed at a prior 5704 * dilution value 5705 */ 5706 RERUN, 5707 /** 5708 * Domain provides codes that qualify the ActLabObsEnvfctsCode domain. 5709 * (Environmental Factors) 5710 */ 5711 EVNFCTS, 5712 /** 5713 * An observation that relates to factors that may potentially cause 5714 * interference with the observation 5715 */ 5716 INTFR, 5717 /** 5718 * The Fibrin Index of the specimen. In the case of only differentiating between 5719 * Absent and Present, recommend using 0 and 1 5720 */ 5721 FIBRIN, 5722 /** 5723 * An observation of the hemolysis index of the specimen in g/L 5724 */ 5725 HEMOLYSIS, 5726 /** 5727 * An observation that describes the icterus index of the specimen. It is 5728 * recommended to use mMol/L of bilirubin 5729 */ 5730 ICTERUS, 5731 /** 5732 * An observation used to describe the Lipemia Index of the specimen. It is 5733 * recommended to use the optical turbidity at 600 nm (in absorbance units). 5734 */ 5735 LIPEMIA, 5736 /** 5737 * An observation that reports the volume of a sample. 5738 */ 5739 VOLUME, 5740 /** 5741 * The available quantity of specimen. This is the current quantity minus any 5742 * planned consumption (e.g., tests that are planned) 5743 */ 5744 AVAILABLE, 5745 /** 5746 * The quantity of specimen that is used each time the equipment uses this 5747 * substance 5748 */ 5749 CONSUMPTION, 5750 /** 5751 * The current quantity of the specimen, i.e., initial quantity minus what has 5752 * been actually used. 5753 */ 5754 CURRENT, 5755 /** 5756 * The initial quantity of the specimen in inventory 5757 */ 5758 INITIAL, 5759 /** 5760 * AnnotationType 5761 */ 5762 _ANNOTATIONTYPE, 5763 /** 5764 * Description:Provides a categorization for annotations recorded directly 5765 * against the patient . 5766 */ 5767 _ACTPATIENTANNOTATIONTYPE, 5768 /** 5769 * Description:A note that is specific to a patient's diagnostic images, either 5770 * historical, current or planned. 5771 */ 5772 ANNDI, 5773 /** 5774 * Description:A general or uncategorized note. 5775 */ 5776 ANNGEN, 5777 /** 5778 * A note that is specific to a patient's immunizations, either historical, 5779 * current or planned. 5780 */ 5781 ANNIMM, 5782 /** 5783 * Description:A note that is specific to a patient's laboratory results, either 5784 * historical, current or planned. 5785 */ 5786 ANNLAB, 5787 /** 5788 * Description:A note that is specific to a patient's medications, either 5789 * historical, current or planned. 5790 */ 5791 ANNMED, 5792 /** 5793 * Description: None provided 5794 */ 5795 _GENETICOBSERVATIONTYPE, 5796 /** 5797 * Description: A DNA segment that contributes to phenotype/function. In the 5798 * absence of demonstrated function a gene may be characterized by sequence, 5799 * transcription or homology 5800 */ 5801 GENE, 5802 /** 5803 * Description: Observation codes which describe characteristics of the 5804 * immunization material. 5805 */ 5806 _IMMUNIZATIONOBSERVATIONTYPE, 5807 /** 5808 * Description: Indicates the valid antigen count. 5809 */ 5810 OBSANTC, 5811 /** 5812 * Description: Indicates whether an antigen is valid or invalid. 5813 */ 5814 OBSANTV, 5815 /** 5816 * A code that is used to indicate the type of case safety report received from 5817 * sender. The current code example reference is from the International 5818 * Conference on Harmonisation (ICH) Expert Workgroup guideline on Clinical 5819 * Safety Data Management: Data Elements for Transmission of Individual Case 5820 * Safety Reports. The unknown/unavailable option allows the transmission of 5821 * information from a secondary sender where the initial sender did not specify 5822 * the type of report. 5823 * 5824 * Example concepts include: Spontaneous, Report from study, Other. 5825 */ 5826 _INDIVIDUALCASESAFETYREPORTTYPE, 5827 /** 5828 * Indicates that the ICSR is describing problems that a patient experienced 5829 * after receiving a vaccine product. 5830 */ 5831 PATADVEVNT, 5832 /** 5833 * Indicates that the ICSR is describing a problem with the actual vaccine 5834 * product such as physical defects (cloudy, particulate matter) or inability to 5835 * confer immunity. 5836 */ 5837 VACPROBLEM, 5838 /** 5839 * Definition:The set of LOINC codes for the act of determining the period of 5840 * time that has elapsed since an entity was born or created. 5841 */ 5842 _LOINCOBSERVATIONACTCONTEXTAGETYPE, 5843 /** 5844 * Definition:Estimated age. 5845 */ 5846 _216119, 5847 /** 5848 * Definition:Reported age. 5849 */ 5850 _216127, 5851 /** 5852 * Definition:Calculated age. 5853 */ 5854 _295535, 5855 /** 5856 * Definition:General specification of age with no implied method of 5857 * determination. 5858 */ 5859 _305250, 5860 /** 5861 * Definition:Age at onset of associated adverse event; no implied method of 5862 * determination. 5863 */ 5864 _309724, 5865 /** 5866 * MedicationObservationType 5867 */ 5868 _MEDICATIONOBSERVATIONTYPE, 5869 /** 5870 * Description:This observation represents an 'average' or 'expected' half-life 5871 * typical of the product. 5872 */ 5873 REPHALFLIFE, 5874 /** 5875 * Definition: A characteristic of an oral solid dosage form of a medicinal 5876 * product, indicating whether it has one or more coatings such as sugar 5877 * coating, film coating, or enteric coating. Only coatings to the external 5878 * surface or the dosage form should be considered (for example, coatings to 5879 * individual pellets or granules inside a capsule or tablet are excluded from 5880 * consideration). 5881 * 5882 * 5883 * Constraints: The Observation.value must be a Boolean (BL) with true for the 5884 * presence or false for the absence of one or more coatings on a solid dosage 5885 * form. 5886 */ 5887 SPLCOATING, 5888 /** 5889 * Definition: A characteristic of an oral solid dosage form of a medicinal 5890 * product, specifying the color or colors that most predominantly define the 5891 * appearance of the dose form. SPLCOLOR is not an FDA specification for the 5892 * actual color of solid dosage forms or the names of colors that can appear in 5893 * labeling. 5894 * 5895 * 5896 * Constraints: The Observation.value must be a single coded value or a list of 5897 * multiple coded values, specifying one or more distinct colors that 5898 * approximate of the color(s) of distinct areas of the solid dosage form, such 5899 * as the different sides of a tablet or one-part capsule, or the different 5900 * halves of a two-part capsule. Bands on banded capsules, regardless of the 5901 * color, are not considered when assigning an SPLCOLOR. Imprints on the dosage 5902 * form, regardless of their color are not considered when assigning an 5903 * SPLCOLOR. If more than one color exists on a particular side or half, then 5904 * the most predominant color on that side or half is recorded. If the gelatin 5905 * capsule shell is colorless and transparent, use the predominant color of the 5906 * contents that appears through the colorless and transparent capsule shell. 5907 * Colors can include: 5908 * Black;Gray;White;Red;Pink;Purple;Green;Yellow;Orange;Brown;Blue;Turquoise. 5909 */ 5910 SPLCOLOR, 5911 /** 5912 * Description: A characteristic representing a single file reference that 5913 * contains two or more views of the same dosage form of the product; in most 5914 * cases this should represent front and back views of the dosage form, but 5915 * occasionally additional views might be needed in order to capture all of the 5916 * important physical characteristics of the dosage form. Any imprint and/or 5917 * symbol should be clearly identifiable, and the viewer should not normally 5918 * need to rotate the image in order to read it. Images that are submitted with 5919 * SPL should be included in the same directory as the SPL file. 5920 */ 5921 SPLIMAGE, 5922 /** 5923 * Definition: A characteristic of an oral solid dosage form of a medicinal 5924 * product, specifying the alphanumeric text that appears on the solid dosage 5925 * form, including text that is embossed, debossed, engraved or printed with 5926 * ink. The presence of other non-textual distinguishing marks or symbols is 5927 * recorded by SPLSYMBOL. 5928 * 5929 * 5930 * Examples: Included in SPLIMPRINT are alphanumeric text that appears on the 5931 * bands of banded capsules and logos and other symbols that can be interpreted 5932 * as letters or numbers. 5933 * 5934 * 5935 * Constraints: The Observation.value must be of type Character String (ST). 5936 * Excluded from SPLIMPRINT are internal and external cut-outs in the form of 5937 * alphanumeric text and the letter 'R' with a circle around it (when referring 5938 * to a registered trademark) and the letters 'TM' (when referring to a 'trade 5939 * mark'). To record text, begin on either side or part of the dosage form. 5940 * Start at the top left and progress as one would normally read a book. Enter a 5941 * semicolon to show separation between words or line divisions. 5942 */ 5943 SPLIMPRINT, 5944 /** 5945 * Definition: A characteristic of an oral solid dosage form of a medicinal 5946 * product, specifying the number of equal pieces that the solid dosage form can 5947 * be divided into using score line(s). 5948 * 5949 * 5950 * Example: One score line creating two equal pieces is given a value of 2, two 5951 * parallel score lines creating three equal pieces is given a value of 3. 5952 * 5953 * 5954 * Constraints: Whether three parallel score lines create four equal pieces or 5955 * two intersecting score lines create two equal pieces using one score line and 5956 * four equal pieces using both score lines, both have the scoring value of 4. 5957 * Solid dosage forms that are not scored are given a value of 1. Solid dosage 5958 * forms that can only be divided into unequal pieces are given a null-value 5959 * with nullFlavor other (OTH). 5960 */ 5961 SPLSCORING, 5962 /** 5963 * Description: A characteristic of an oral solid dosage form of a medicinal 5964 * product, specifying the two dimensional representation of the solid dose 5965 * form, in terms of the outside perimeter of a solid dosage form when the 5966 * dosage form, resting on a flat surface, is viewed from directly above, 5967 * including slight rounding of corners. SPLSHAPE does not include embossing, 5968 * scoring, debossing, or internal cut-outs. SPLSHAPE is independent of the 5969 * orientation of the imprint and logo. Shapes can include: Triangle (3 sided); 5970 * Square; Round; Semicircle; Pentagon (5 sided); Diamond; Double circle; 5971 * Bullet; Hexagon (6 sided); Rectangle; Gear; Capsule; Heptagon (7 sided); 5972 * Trapezoid; Oval; Clover; Octagon (8 sided); Tear; Freeform. 5973 */ 5974 SPLSHAPE, 5975 /** 5976 * Definition: A characteristic of an oral solid dosage form of a medicinal 5977 * product, specifying the longest single dimension of the solid dosage form as 5978 * a physical quantity in the dimension of length (e.g., 3 mm). The length is 5979 * should be specified in millimeters and should be rounded to the nearest whole 5980 * millimeter. 5981 * 5982 * 5983 * Example: SPLSIZE for a rectangular shaped tablet is the length and SPLSIZE 5984 * for a round shaped tablet is the diameter. 5985 */ 5986 SPLSIZE, 5987 /** 5988 * Definition: A characteristic of an oral solid dosage form of a medicinal 5989 * product, to describe whether or not the medicinal product has a mark or 5990 * symbol appearing on it for easy and definite recognition. Score lines, 5991 * letters, numbers, and internal and external cut-outs are not considered marks 5992 * or symbols. See SPLSCORING and SPLIMPRINT for these characteristics. 5993 * 5994 * 5995 * Constraints: The Observation.value must be a Boolean (BL) with <u>true</u> 5996 * indicating the presence and <u>false</u> for the absence of marks or symbols. 5997 * 5998 * 5999 * Example: 6000 */ 6001 SPLSYMBOL, 6002 /** 6003 * Distinguishes the kinds of coded observations that could be the trigger for 6004 * clinical issue detection. These are observations that are not measurable, but 6005 * instead can be defined with codes. Coded observation types include: Allergy, 6006 * Intolerance, Medical Condition, Pregnancy status, etc. 6007 */ 6008 _OBSERVATIONISSUETRIGGERCODEDOBSERVATIONTYPE, 6009 /** 6010 * Code for the mechanism by which disease was acquired by the living subject 6011 * involved in the public health case. Includes sexually transmitted, airborne, 6012 * bloodborne, vectorborne, foodborne, zoonotic, nosocomial, mechanical, dermal, 6013 * congenital, environmental exposure, indeterminate. 6014 */ 6015 _CASETRANSMISSIONMODE, 6016 /** 6017 * Communication of an agent from a living subject or environmental source to a 6018 * living subject through indirect contact via oral or nasal inhalation. 6019 */ 6020 AIRTRNS, 6021 /** 6022 * Communication of an agent from one animal to another proximate animal. 6023 */ 6024 ANANTRNS, 6025 /** 6026 * Communication of an agent from an animal to a proximate person. 6027 */ 6028 ANHUMTRNS, 6029 /** 6030 * Communication of an agent from one living subject to another living subject 6031 * through direct contact with any body fluid. 6032 */ 6033 BDYFLDTRNS, 6034 /** 6035 * Communication of an agent to a living subject through direct contact with 6036 * blood or blood products whether the contact with blood is part of a 6037 * therapeutic procedure or not. 6038 */ 6039 BLDTRNS, 6040 /** 6041 * Communication of an agent from a living subject or environmental source to a 6042 * living subject via agent migration through intact skin. 6043 */ 6044 DERMTRNS, 6045 /** 6046 * Communication of an agent from an environmental surface or source to a living 6047 * subject by direct contact. 6048 */ 6049 ENVTRNS, 6050 /** 6051 * Communication of an agent from a living subject or environmental source to a 6052 * living subject through oral contact with material contaminated by person or 6053 * animal fecal material. 6054 */ 6055 FECTRNS, 6056 /** 6057 * Communication of an agent from an non-living material to a living subject 6058 * through direct contact. 6059 */ 6060 FOMTRNS, 6061 /** 6062 * Communication of an agent from a food source to a living subject via oral 6063 * consumption. 6064 */ 6065 FOODTRNS, 6066 /** 6067 * Communication of an agent from a person to a proximate person. 6068 */ 6069 HUMHUMTRNS, 6070 /** 6071 * Communication of an agent to a living subject via an undetermined route. 6072 */ 6073 INDTRNS, 6074 /** 6075 * Communication of an agent from one living subject to another living subject 6076 * through direct contact with mammalian milk or colostrum. 6077 */ 6078 LACTTRNS, 6079 /** 6080 * Communication of an agent from any entity to a living subject while the 6081 * living subject is in the patient role in a healthcare facility. 6082 */ 6083 NOSTRNS, 6084 /** 6085 * Communication of an agent from a living subject or environmental source to a 6086 * living subject where the acquisition of the agent is not via the alimentary 6087 * canal. 6088 */ 6089 PARTRNS, 6090 /** 6091 * Communication of an agent from a living subject to the progeny of that living 6092 * subject via agent migration across the maternal-fetal placental membranes 6093 * while in utero. 6094 */ 6095 PLACTRNS, 6096 /** 6097 * Communication of an agent from one living subject to another living subject 6098 * through direct contact with genital or oral tissues as part of a sexual act. 6099 */ 6100 SEXTRNS, 6101 /** 6102 * Communication of an agent from one living subject to another living subject 6103 * through direct contact with blood or blood products where the contact with 6104 * blood is part of a therapeutic procedure. 6105 */ 6106 TRNSFTRNS, 6107 /** 6108 * Communication of an agent from a living subject acting as a required 6109 * intermediary in the agent transmission process to a recipient living subject 6110 * via direct contact. 6111 */ 6112 VECTRNS, 6113 /** 6114 * Communication of an agent from a contaminated water source to a living 6115 * subject whether the water is ingested as a food or not. The route of entry of 6116 * the water may be through any bodily orifice. 6117 */ 6118 WATTRNS, 6119 /** 6120 * Codes used to define various metadata aspects of a health quality measure. 6121 */ 6122 _OBSERVATIONQUALITYMEASUREATTRIBUTE, 6123 /** 6124 * Indicates that the observation is carrying out an aggregation calculation, 6125 * contained in the value element. 6126 */ 6127 AGGREGATE, 6128 /** 6129 * Indicates what method is used in a quality measure to combine the component 6130 * measure results included in an composite measure. 6131 */ 6132 CMPMSRMTH, 6133 /** 6134 * An attribute of a quality measure describing the weight this component 6135 * measure score is to carry in determining the overall composite measure final 6136 * score. The value is real value greater than 0 and less than 1.0. Each 6137 * component measure score will be multiplied by its CMPMSRSCRWGHT and then 6138 * summed with the other component measures to determine the final overall 6139 * composite measure score. The sum across all CMPMSRSCRWGHT values within a 6140 * single composite measure SHALL be 1.0. The value assigned is scoped to the 6141 * composite measure referencing this component measure only. 6142 */ 6143 CMPMSRSCRWGHT, 6144 /** 6145 * Identifies the organization(s) who own the intellectual property represented 6146 * by the eMeasure. 6147 */ 6148 COPY, 6149 /** 6150 * Summary of relevant clinical guidelines or other clinical recommendations 6151 * supporting this eMeasure. 6152 */ 6153 CRS, 6154 /** 6155 * Description of individual terms, provided as needed. 6156 */ 6157 DEF, 6158 /** 6159 * Disclaimer information for the eMeasure. 6160 */ 6161 DISC, 6162 /** 6163 * The timestamp when the eMeasure was last packaged in the Measure Authoring 6164 * Tool. 6165 */ 6166 FINALDT, 6167 /** 6168 * Used to allow measure developers to provide additional guidance for 6169 * implementers to understand greater specificity than could be provided in the 6170 * logic for data criteria. 6171 */ 6172 GUIDE, 6173 /** 6174 * Information on whether an increase or decrease in score is the preferred 6175 * result (e.g., a higher score indicates better quality OR a lower score 6176 * indicates better quality OR quality is within a range). 6177 */ 6178 IDUR, 6179 /** 6180 * Describes the items counted by the measure (e.g., patients, encounters, 6181 * procedures, etc.) 6182 */ 6183 ITMCNT, 6184 /** 6185 * A significant word that aids in discoverability. 6186 */ 6187 KEY, 6188 /** 6189 * The end date of the measurement period. 6190 */ 6191 MEDT, 6192 /** 6193 * The start date of the measurement period. 6194 */ 6195 MSD, 6196 /** 6197 * The method of adjusting for clinical severity and conditions present at the 6198 * start of care that can influence patient outcomes for making valid 6199 * comparisons of outcome measures across providers. Indicates whether an 6200 * eMeasure is subject to the statistical process for reducing, removing, or 6201 * clarifying the influences of confounding factors to allow more useful 6202 * comparisons. 6203 */ 6204 MSRADJ, 6205 /** 6206 * Describes how to combine information calculated based on logic in each of 6207 * several populations into one summarized result. It can also be used to 6208 * describe how to risk adjust the data based on supplemental data elements 6209 * described in the eMeasure. (e.g., pneumonia hospital measures antibiotic 6210 * selection in the ICU versus non-ICU and then the roll-up of the two). 6211 * 6212 * 6213 * Open Issue: The description does NOT align well with the definition used in 6214 * the HQMF specfication; correct the MSGAGG definition, and the possible 6215 * distinction of MSRAGG as a child of AGGREGATE. 6216 */ 6217 MSRAGG, 6218 /** 6219 * Information on whether an increase or decrease in score is the preferred 6220 * result. This should reflect information on which way is better, an increase 6221 * or decrease in score. 6222 */ 6223 MSRIMPROV, 6224 /** 6225 * The list of jurisdiction(s) for which the measure applies. 6226 */ 6227 MSRJUR, 6228 /** 6229 * Type of person or organization that is expected to report the issue. 6230 */ 6231 MSRRPTR, 6232 /** 6233 * The maximum time that may elapse following completion of the measure until 6234 * the measure report must be sent to the receiver. 6235 */ 6236 MSRRPTTIME, 6237 /** 6238 * Indicates how the calculation is performed for the eMeasure (e.g., 6239 * proportion, continuous variable, ratio) 6240 */ 6241 MSRSCORE, 6242 /** 6243 * Location(s) in which care being measured is rendered 6244 * 6245 * Usage Note: MSRSET is used rather than RoleCode because the setting applies 6246 * to what is being measured, as opposed to participating directly in the health 6247 * quality measure documantion itself). 6248 */ 6249 MSRSET, 6250 /** 6251 * health quality measure topic type 6252 */ 6253 MSRTOPIC, 6254 /** 6255 * The time period for which the eMeasure applies. 6256 */ 6257 MSRTP, 6258 /** 6259 * Indicates whether the eMeasure is used to examine a process or an outcome 6260 * over time (e.g., Structure, Process, Outcome). 6261 */ 6262 MSRTYPE, 6263 /** 6264 * Succinct statement of the need for the measure. Usually includes statements 6265 * pertaining to Importance criterion: impact, gap in care and evidence. 6266 */ 6267 RAT, 6268 /** 6269 * Identifies bibliographic citations or references to clinical practice 6270 * guidelines, sources of evidence, or other relevant materials supporting the 6271 * intent and rationale of the eMeasure. 6272 */ 6273 REF, 6274 /** 6275 * Comparison of results across strata can be used to show where disparities 6276 * exist or where there is a need to expose differences in results. For example, 6277 * Centers for Medicare & Medicaid Services (CMS) in the U.S. defines four 6278 * required Supplemental Data Elements (payer, ethnicity, race, and gender), 6279 * which are variables used to aggregate data into various subgroups. Additional 6280 * supplemental data elements required for risk adjustment or other purposes of 6281 * data aggregation can be included in the Supplemental Data Element section. 6282 */ 6283 SDE, 6284 /** 6285 * Describes the strata for which the measure is to be evaluated. There are 6286 * three examples of reasons for stratification based on existing work. These 6287 * include: (1) evaluate the measure based on different age groupings within the 6288 * population described in the measure (e.g., evaluate the whole [age 14-25] and 6289 * each sub-stratum [14-19] and [20-25]); (2) evaluate the eMeasure based on 6290 * either a specific condition, a specific discharge location, or both; (3) 6291 * evaluate the eMeasure based on different locations within a facility (e.g., 6292 * evaluate the overall rate for all intensive care units and also some strata 6293 * include additional findings [specific birth weights for neonatal intensive 6294 * care units]). 6295 */ 6296 STRAT, 6297 /** 6298 * Can be a URL or hyperlinks that link to the transmission formats that are 6299 * specified for a particular reporting program. 6300 */ 6301 TRANF, 6302 /** 6303 * Usage notes. 6304 */ 6305 USE, 6306 /** 6307 * ObservationSequenceType 6308 */ 6309 _OBSERVATIONSEQUENCETYPE, 6310 /** 6311 * A sequence of values in the "absolute" time domain. This is the same time 6312 * domain that all HL7 timestamps use. It is time as measured by the Gregorian 6313 * calendar 6314 */ 6315 TIMEABSOLUTE, 6316 /** 6317 * A sequence of values in a "relative" time domain. The time is measured 6318 * relative to the earliest effective time in the Observation Series containing 6319 * this sequence. 6320 */ 6321 TIMERELATIVE, 6322 /** 6323 * ObservationSeriesType 6324 */ 6325 _OBSERVATIONSERIESTYPE, 6326 /** 6327 * ECGObservationSeriesType 6328 */ 6329 _ECGOBSERVATIONSERIESTYPE, 6330 /** 6331 * This Observation Series type contains waveforms of a "representative beat" 6332 * (a.k.a. "median beat" or "average beat"). The waveform samples are measured 6333 * in relative time, relative to the beginning of the beat as defined by the 6334 * Observation Series effective time. The waveforms are not directly acquired 6335 * from the subject, but rather algorithmically derived from the "rhythm" 6336 * waveforms. 6337 */ 6338 REPRESENTATIVEBEAT, 6339 /** 6340 * This Observation type contains ECG "rhythm" waveforms. The waveform samples 6341 * are measured in absolute time (a.k.a. "subject time" or "effective time"). 6342 * These waveforms are usually "raw" with some minimal amount of noise reduction 6343 * and baseline filtering applied. 6344 */ 6345 RHYTHM, 6346 /** 6347 * Description: Reporting codes that are related to an immunization event. 6348 */ 6349 _PATIENTIMMUNIZATIONRELATEDOBSERVATIONTYPE, 6350 /** 6351 * Description: The class room associated with the patient during the 6352 * immunization event. 6353 */ 6354 CLSSRM, 6355 /** 6356 * Description: The school grade or level the patient was in when immunized. 6357 */ 6358 GRADE, 6359 /** 6360 * Description: The school the patient attended when immunized. 6361 */ 6362 SCHL, 6363 /** 6364 * Description: The school division or district associated with the patient 6365 * during the immunization event. 6366 */ 6367 SCHLDIV, 6368 /** 6369 * Description: The patient's teacher when immunized. 6370 */ 6371 TEACHER, 6372 /** 6373 * Observation types for specifying criteria used to assert that a subject is 6374 * included in a particular population. 6375 */ 6376 _POPULATIONINCLUSIONOBSERVATIONTYPE, 6377 /** 6378 * Criteria which specify subjects who should be removed from the eMeasure 6379 * population and denominator before determining if numerator criteria are met. 6380 * Denominator exclusions are used in proportion and ratio measures to help 6381 * narrow the denominator. 6382 */ 6383 DENEX, 6384 /** 6385 * Criteria which specify the removal of a subject, procedure or unit of 6386 * measurement from the denominator, only if the numerator criteria are not met. 6387 * Denominator exceptions allow for adjustment of the calculated score for those 6388 * providers with higher risk populations. Denominator exceptions are used only 6389 * in proportion eMeasures. They are not appropriate for ratio or continuous 6390 * variable eMeasures. Denominator exceptions allow for the exercise of clinical 6391 * judgment and should be specifically defined where capturing the information 6392 * in a structured manner fits the clinical workflow. Generic denominator 6393 * exception reasons used in proportion eMeasures fall into three general 6394 * categories: 6395 * 6396 * 6397 * Medical reasons Patient (or subject) reasons System reasons 6398 */ 6399 DENEXCEP, 6400 /** 6401 * Criteria for specifying the entities to be evaluated by a specific quality 6402 * measure, based on a shared common set of characteristics (within a specific 6403 * measurement set to which a given measure belongs). The denominator can be the 6404 * same as the initial population, or it may be a subset of the initial 6405 * population to further constrain it for the purpose of the eMeasure. Different 6406 * measures within an eMeasure set may have different denominators. Continuous 6407 * Variable eMeasures do not have a denominator, but instead define a measure 6408 * population. 6409 */ 6410 DENOM, 6411 /** 6412 * Criteria for specifying the entities to be evaluated by a specific quality 6413 * measure, based on a shared common set of characteristics (within a specific 6414 * measurement set to which a given measure belongs). 6415 */ 6416 IPOP, 6417 /** 6418 * Criteria for specifying the patients to be evaluated by a specific quality 6419 * measure, based on a shared common set of characteristics (within a specific 6420 * measurement set to which a given measure belongs). Details often include 6421 * information based upon specific age groups, diagnoses, diagnostic and 6422 * procedure codes, and enrollment periods. 6423 */ 6424 IPPOP, 6425 /** 6426 * Defines the observation to be performed for each patient or event in the 6427 * measure population. Measure observations for each case in the population are 6428 * aggregated to determine the overall measure score for the population. 6429 * 6430 * 6431 * Examples: 6432 * 6433 * 6434 * 6435 * the median time from arrival in the Emergency Room to departure the median 6436 * time from decision to admit to a hospital to the actual admission for 6437 * Emergency Room patients 6438 */ 6439 MSROBS, 6440 /** 6441 * Criteria for specifying the measure population as a narrative description 6442 * (e.g., all patients seen in the Emergency Department during the measurement 6443 * period). This is used only in continuous variable eMeasures. 6444 */ 6445 MSRPOPL, 6446 /** 6447 * Criteria for specifying subjects who should be removed from the eMeasure's 6448 * Initial Population and Measure Population. Measure Population Exclusions are 6449 * used in Continuous Variable measures to help narrow the Measure Population 6450 * before determining the value(s) of the continuous variable(s). 6451 */ 6452 MSRPOPLEX, 6453 /** 6454 * Criteria for specifying the processes or outcomes expected for each patient, 6455 * procedure, or other unit of measurement defined in the denominator for 6456 * proportion measures, or related to (but not directly derived from) the 6457 * denominator for ratio measures (e.g., a numerator listing the number of 6458 * central line blood stream infections and a denominator indicating the days 6459 * per thousand of central line usage in a specific time period). 6460 */ 6461 NUMER, 6462 /** 6463 * Criteria for specifying instances that should not be included in the 6464 * numerator data. (e.g., if the number of central line blood stream infections 6465 * per 1000 catheter days were to exclude infections with a specific bacterium, 6466 * that bacterium would be listed as a numerator exclusion). Numerator 6467 * Exclusions are used only in ratio eMeasures. 6468 */ 6469 NUMEX, 6470 /** 6471 * Types of observations that can be made about Preferences. 6472 */ 6473 _PREFERENCEOBSERVATIONTYPE, 6474 /** 6475 * An observation about how important a preference is to the target of the 6476 * preference. 6477 */ 6478 PREFSTRENGTH, 6479 /** 6480 * Indicates that the observation is of an unexpected negative occurrence in the 6481 * subject suspected to result from the subject's exposure to one or more 6482 * agents. Observation values would be the symptom resulting from the reaction. 6483 */ 6484 ADVERSEREACTION, 6485 /** 6486 * Description:Refines classCode OBS to indicate an observation in which 6487 * observation.value contains a finding or other nominalized statement, where 6488 * the encoded information in Observation.value is not altered by 6489 * Observation.code. For instance, observation.code="ASSERTION" and 6490 * observation.value="fracture of femur present" is an assertion of a clinical 6491 * finding of femur fracture. 6492 */ 6493 ASSERTION, 6494 /** 6495 * Definition:An observation that provides a characterization of the level of 6496 * harm to an investigation subject as a result of a reaction or event. 6497 */ 6498 CASESER, 6499 /** 6500 * An observation that states whether the disease was likely acquired outside 6501 * the jurisdiction of observation, and if so, the nature of the 6502 * inter-jurisdictional relationship. 6503 * 6504 * 6505 * OpenIssue: This code could be moved to LOINC if it can be done before there 6506 * are significant implemenations using it. 6507 */ 6508 CDIO, 6509 /** 6510 * A clinical judgment as to the worst case result of a future exposure 6511 * (including substance administration). When the worst case result is assessed 6512 * to have a life-threatening or organ system threatening potential, it is 6513 * considered to be of high criticality. 6514 */ 6515 CRIT, 6516 /** 6517 * An observation that states the mechanism by which disease was acquired by the 6518 * living subject involved in the public health case. 6519 * 6520 * 6521 * OpenIssue: This code could be moved to LOINC if it can be done before there 6522 * are significant implemenations using it. 6523 */ 6524 CTMO, 6525 /** 6526 * Includes all codes defining types of indications such as diagnosis, symptom 6527 * and other indications such as contrast agents for lab tests. 6528 */ 6529 DX, 6530 /** 6531 * Admitting diagnosis are the diagnoses documented for administrative purposes 6532 * as the basis for a hospital admission. 6533 */ 6534 ADMDX, 6535 /** 6536 * Discharge diagnosis are the diagnoses documented for administrative purposes 6537 * as the time of hospital discharge. 6538 */ 6539 DISDX, 6540 /** 6541 * Intermediate diagnoses are those diagnoses documented for administrative 6542 * purposes during the course of a hospital stay. 6543 */ 6544 INTDX, 6545 /** 6546 * The type of injury that the injury coding specifies. 6547 */ 6548 NOI, 6549 /** 6550 * Description: Accuracy determined as per the GIS tier code system. 6551 */ 6552 GISTIER, 6553 /** 6554 * Indicates that the observation is of a person?s living situation in a 6555 * household including the household composition and circumstances. 6556 */ 6557 HHOBS, 6558 /** 6559 * There is a clinical issue for the therapy that makes continuation of the 6560 * therapy inappropriate. 6561 * 6562 * 6563 * Open Issue: The definition of this code does not correctly represent the 6564 * concept space of its specializations (children) 6565 */ 6566 ISSUE, 6567 /** 6568 * Identifies types of detectyed issues for Act class "ALRT" for the 6569 * administrative and patient administrative acts domains. 6570 */ 6571 _ACTADMINISTRATIVEDETECTEDISSUECODE, 6572 /** 6573 * ActAdministrativeAuthorizationDetectedIssueCode 6574 */ 6575 _ACTADMINISTRATIVEAUTHORIZATIONDETECTEDISSUECODE, 6576 /** 6577 * The requesting party has insufficient authorization to invoke the 6578 * interaction. 6579 */ 6580 NAT, 6581 /** 6582 * Description: One or more records in the query response have been suppressed 6583 * due to consent or privacy restrictions. 6584 */ 6585 SUPPRESSED, 6586 /** 6587 * Description:The specified element did not pass business-rule validation. 6588 */ 6589 VALIDAT, 6590 /** 6591 * The ID of the patient, order, etc., was not found. Used for transactions 6592 * other than additions, e.g. transfer of a non-existent patient. 6593 */ 6594 KEY204, 6595 /** 6596 * The ID of the patient, order, etc., already exists. Used in response to 6597 * addition transactions (Admit, New Order, etc.). 6598 */ 6599 KEY205, 6600 /** 6601 * There may be an issue with the patient complying with the intentions of the 6602 * proposed therapy 6603 */ 6604 COMPLY, 6605 /** 6606 * The proposed therapy appears to duplicate an existing therapy 6607 */ 6608 DUPTHPY, 6609 /** 6610 * Description:The proposed therapy appears to have the same intended 6611 * therapeutic benefit as an existing therapy, though the specific mechanisms of 6612 * action vary. 6613 */ 6614 DUPTHPCLS, 6615 /** 6616 * Description:The proposed therapy appears to have the same intended 6617 * therapeutic benefit as an existing therapy and uses the same mechanisms of 6618 * action as the existing therapy. 6619 */ 6620 DUPTHPGEN, 6621 /** 6622 * Description:The proposed therapy is frequently misused or abused and 6623 * therefore should be used with caution and/or monitoring. 6624 */ 6625 ABUSE, 6626 /** 6627 * Description:The request is suspected to have a fraudulent basis. 6628 */ 6629 FRAUD, 6630 /** 6631 * A similar or identical therapy was recently ordered by a different 6632 * practitioner. 6633 */ 6634 PLYDOC, 6635 /** 6636 * This patient was recently supplied a similar or identical therapy from a 6637 * different pharmacy or supplier. 6638 */ 6639 PLYPHRM, 6640 /** 6641 * Proposed dosage instructions for therapy differ from standard practice. 6642 */ 6643 DOSE, 6644 /** 6645 * Description:Proposed dosage is inappropriate due to patient's medical 6646 * condition. 6647 */ 6648 DOSECOND, 6649 /** 6650 * Proposed length of therapy differs from standard practice. 6651 */ 6652 DOSEDUR, 6653 /** 6654 * Proposed length of therapy is longer than standard practice 6655 */ 6656 DOSEDURH, 6657 /** 6658 * Proposed length of therapy is longer than standard practice for the 6659 * identified indication or diagnosis 6660 */ 6661 DOSEDURHIND, 6662 /** 6663 * Proposed length of therapy is shorter than that necessary for therapeutic 6664 * effect 6665 */ 6666 DOSEDURL, 6667 /** 6668 * Proposed length of therapy is shorter than standard practice for the 6669 * identified indication or diagnosis 6670 */ 6671 DOSEDURLIND, 6672 /** 6673 * Proposed dosage exceeds standard practice 6674 */ 6675 DOSEH, 6676 /** 6677 * Proposed dosage exceeds standard practice for the patient's age 6678 */ 6679 DOSEHINDA, 6680 /** 6681 * High Dose for Indication Alert 6682 */ 6683 DOSEHIND, 6684 /** 6685 * Proposed dosage exceeds standard practice for the patient's height or body 6686 * surface area 6687 */ 6688 DOSEHINDSA, 6689 /** 6690 * Proposed dosage exceeds standard practice for the patient's weight 6691 */ 6692 DOSEHINDW, 6693 /** 6694 * Proposed dosage interval/timing differs from standard practice 6695 */ 6696 DOSEIVL, 6697 /** 6698 * Proposed dosage interval/timing differs from standard practice for the 6699 * identified indication or diagnosis 6700 */ 6701 DOSEIVLIND, 6702 /** 6703 * Proposed dosage is below suggested therapeutic levels 6704 */ 6705 DOSEL, 6706 /** 6707 * Proposed dosage is below suggested therapeutic levels for the patient's age 6708 */ 6709 DOSELINDA, 6710 /** 6711 * Low Dose for Indication Alert 6712 */ 6713 DOSELIND, 6714 /** 6715 * Proposed dosage is below suggested therapeutic levels for the patient's 6716 * height or body surface area 6717 */ 6718 DOSELINDSA, 6719 /** 6720 * Proposed dosage is below suggested therapeutic levels for the patient's 6721 * weight 6722 */ 6723 DOSELINDW, 6724 /** 6725 * Description:The maximum quantity of this drug allowed to be administered 6726 * within a particular time-range (month, year, lifetime) has been reached or 6727 * exceeded. 6728 */ 6729 MDOSE, 6730 /** 6731 * Proposed therapy may be inappropriate or contraindicated due to conditions or 6732 * characteristics of the patient 6733 */ 6734 OBSA, 6735 /** 6736 * Proposed therapy may be inappropriate or contraindicated due to patient age 6737 */ 6738 AGE, 6739 /** 6740 * Proposed therapy is outside of the standard practice for an adult patient. 6741 */ 6742 ADALRT, 6743 /** 6744 * Proposed therapy is outside of standard practice for a geriatric patient. 6745 */ 6746 GEALRT, 6747 /** 6748 * Proposed therapy is outside of the standard practice for a pediatric patient. 6749 */ 6750 PEALRT, 6751 /** 6752 * Proposed therapy may be inappropriate or contraindicated due to an 6753 * existing/recent patient condition or diagnosis 6754 */ 6755 COND, 6756 /** 6757 * null 6758 */ 6759 HGHT, 6760 /** 6761 * Proposed therapy may be inappropriate or contraindicated when breast-feeding 6762 */ 6763 LACT, 6764 /** 6765 * Proposed therapy may be inappropriate or contraindicated during pregnancy 6766 */ 6767 PREG, 6768 /** 6769 * null 6770 */ 6771 WGHT, 6772 /** 6773 * Description:Proposed therapy may be inappropriate or contraindicated because 6774 * of a common but non-patient specific reaction to the product. 6775 * 6776 * 6777 * Example:There is no record of a specific sensitivity for the patient, but the 6778 * presence of the sensitivity is common and therefore caution is warranted. 6779 */ 6780 CREACT, 6781 /** 6782 * Proposed therapy may be inappropriate or contraindicated due to patient 6783 * genetic indicators. 6784 */ 6785 GEN, 6786 /** 6787 * Proposed therapy may be inappropriate or contraindicated due to patient 6788 * gender. 6789 */ 6790 GEND, 6791 /** 6792 * Proposed therapy may be inappropriate or contraindicated due to recent lab 6793 * test results 6794 */ 6795 LAB, 6796 /** 6797 * Proposed therapy may be inappropriate or contraindicated based on the 6798 * potential for a patient reaction to the proposed product 6799 */ 6800 REACT, 6801 /** 6802 * Proposed therapy may be inappropriate or contraindicated because of a 6803 * recorded patient allergy to the proposed product. (Allergies are immune based 6804 * reactions.) 6805 */ 6806 ALGY, 6807 /** 6808 * Proposed therapy may be inappropriate or contraindicated because of a 6809 * recorded patient intolerance to the proposed product. (Intolerances are 6810 * non-immune based sensitivities.) 6811 */ 6812 INT, 6813 /** 6814 * Proposed therapy may be inappropriate or contraindicated because of a 6815 * potential patient reaction to a cross-sensitivity related product. 6816 */ 6817 RREACT, 6818 /** 6819 * Proposed therapy may be inappropriate or contraindicated because of a 6820 * recorded patient allergy to a cross-sensitivity related product. (Allergies 6821 * are immune based reactions.) 6822 */ 6823 RALG, 6824 /** 6825 * Proposed therapy may be inappropriate or contraindicated because of a 6826 * recorded prior adverse reaction to a cross-sensitivity related product. 6827 */ 6828 RAR, 6829 /** 6830 * Proposed therapy may be inappropriate or contraindicated because of a 6831 * recorded patient intolerance to a cross-sensitivity related product. 6832 * (Intolerances are non-immune based sensitivities.) 6833 */ 6834 RINT, 6835 /** 6836 * Description:A local business rule relating multiple elements has been 6837 * violated. 6838 */ 6839 BUS, 6840 /** 6841 * Description:The specified code is not valid against the list of codes allowed 6842 * for the element. 6843 */ 6844 CODEINVAL, 6845 /** 6846 * Description:The specified code has been deprecated and should no longer be 6847 * used. Select another code from the code system. 6848 */ 6849 CODEDEPREC, 6850 /** 6851 * Description:The element does not follow the formatting or type rules defined 6852 * for the field. 6853 */ 6854 FORMAT, 6855 /** 6856 * Description:The request is missing elements or contains elements which cause 6857 * it to not meet the legal standards for actioning. 6858 */ 6859 ILLEGAL, 6860 /** 6861 * Description:The length of the data specified falls out of the range defined 6862 * for the element. 6863 */ 6864 LENRANGE, 6865 /** 6866 * Description:The length of the data specified is greater than the maximum 6867 * length defined for the element. 6868 */ 6869 LENLONG, 6870 /** 6871 * Description:The length of the data specified is less than the minimum length 6872 * defined for the element. 6873 */ 6874 LENSHORT, 6875 /** 6876 * Description:The specified element must be specified with a non-null value 6877 * under certain conditions. In this case, the conditions are true but the 6878 * element is still missing or null. 6879 */ 6880 MISSCOND, 6881 /** 6882 * Description:The specified element is mandatory and was not included in the 6883 * instance. 6884 */ 6885 MISSMAND, 6886 /** 6887 * Description:More than one element with the same value exists in the set. 6888 * Duplicates not permission in this set in a set. 6889 */ 6890 NODUPS, 6891 /** 6892 * Description: Element in submitted message will not persist in data storage 6893 * based on detected issue. 6894 */ 6895 NOPERSIST, 6896 /** 6897 * Description:The number of repeating elements falls outside the range of the 6898 * allowed number of repetitions. 6899 */ 6900 REPRANGE, 6901 /** 6902 * Description:The number of repeating elements is above the maximum number of 6903 * repetitions allowed. 6904 */ 6905 MAXOCCURS, 6906 /** 6907 * Description:The number of repeating elements is below the minimum number of 6908 * repetitions allowed. 6909 */ 6910 MINOCCURS, 6911 /** 6912 * ActAdministrativeRuleDetectedIssueCode 6913 */ 6914 _ACTADMINISTRATIVERULEDETECTEDISSUECODE, 6915 /** 6916 * Description: Metadata associated with the identification (e.g. name or 6917 * gender) does not match the identification being verified. 6918 */ 6919 KEY206, 6920 /** 6921 * Description: One or more records in the query response have a status of 6922 * 'obsolete'. 6923 */ 6924 OBSOLETE, 6925 /** 6926 * Identifies types of detected issues regarding the administration or supply of 6927 * an item to a patient. 6928 */ 6929 _ACTSUPPLIEDITEMDETECTEDISSUECODE, 6930 /** 6931 * Administration of the proposed therapy may be inappropriate or 6932 * contraindicated as proposed 6933 */ 6934 _ADMINISTRATIONDETECTEDISSUECODE, 6935 /** 6936 * AppropriatenessDetectedIssueCode 6937 */ 6938 _APPROPRIATENESSDETECTEDISSUECODE, 6939 /** 6940 * InteractionDetectedIssueCode 6941 */ 6942 _INTERACTIONDETECTEDISSUECODE, 6943 /** 6944 * Proposed therapy may interact with certain foods 6945 */ 6946 FOOD, 6947 /** 6948 * Proposed therapy may interact with an existing or recent therapeutic product 6949 */ 6950 TPROD, 6951 /** 6952 * Proposed therapy may interact with an existing or recent drug therapy 6953 */ 6954 DRG, 6955 /** 6956 * Proposed therapy may interact with existing or recent natural health product 6957 * therapy 6958 */ 6959 NHP, 6960 /** 6961 * Proposed therapy may interact with a non-prescription drug (e.g. alcohol, 6962 * tobacco, Aspirin) 6963 */ 6964 NONRX, 6965 /** 6966 * Definition:The same or similar treatment has previously been attempted with 6967 * the patient without achieving a positive effect. 6968 */ 6969 PREVINEF, 6970 /** 6971 * Description:Proposed therapy may be contraindicated or ineffective based on 6972 * an existing or recent drug therapy. 6973 */ 6974 DACT, 6975 /** 6976 * Description:Proposed therapy may be inappropriate or ineffective based on the 6977 * proposed start or end time. 6978 */ 6979 TIME, 6980 /** 6981 * Definition:Proposed therapy may be inappropriate or ineffective because the 6982 * end of administration is too close to another planned therapy. 6983 */ 6984 ALRTENDLATE, 6985 /** 6986 * Definition:Proposed therapy may be inappropriate or ineffective because the 6987 * start of administration is too late after the onset of the condition. 6988 */ 6989 ALRTSTRTLATE, 6990 /** 6991 * Proposed therapy may be inappropriate or ineffective based on the proposed 6992 * start or end time. 6993 */ 6994 _TIMINGDETECTEDISSUECODE, 6995 /** 6996 * Proposed therapy may be inappropriate or ineffective because the end of 6997 * administration is too close to another planned therapy 6998 */ 6999 ENDLATE, 7000 /** 7001 * Proposed therapy may be inappropriate or ineffective because the start of 7002 * administration is too late after the onset of the condition 7003 */ 7004 STRTLATE, 7005 /** 7006 * Supplying the product at this time may be inappropriate or indicate 7007 * compliance issues with the associated therapy 7008 */ 7009 _SUPPLYDETECTEDISSUECODE, 7010 /** 7011 * Definition:The requested action has already been performed and so this 7012 * request has no effect 7013 */ 7014 ALLDONE, 7015 /** 7016 * Definition:The therapy being performed is in some way out of alignment with 7017 * the requested therapy. 7018 */ 7019 FULFIL, 7020 /** 7021 * Definition:The status of the request being fulfilled has changed such that it 7022 * is no longer actionable. This may be because the request has expired, has 7023 * already been completely fulfilled or has been otherwise stopped or disabled. 7024 * (Not used for 'suspended' orders.) 7025 */ 7026 NOTACTN, 7027 /** 7028 * Definition:The therapy being performed is not sufficiently equivalent to the 7029 * therapy which was requested. 7030 */ 7031 NOTEQUIV, 7032 /** 7033 * Definition:The therapy being performed is not generically equivalent (having 7034 * the identical biological action) to the therapy which was requested. 7035 */ 7036 NOTEQUIVGEN, 7037 /** 7038 * Definition:The therapy being performed is not therapeutically equivalent 7039 * (having the same overall patient effect) to the therapy which was requested. 7040 */ 7041 NOTEQUIVTHER, 7042 /** 7043 * Definition:The therapy is being performed at a time which diverges from the 7044 * time the therapy was requested 7045 */ 7046 TIMING, 7047 /** 7048 * Definition:The therapy action is being performed outside the bounds of the 7049 * time period requested 7050 */ 7051 INTERVAL, 7052 /** 7053 * Definition:The therapy action is being performed too soon after the previous 7054 * occurrence based on the requested frequency 7055 */ 7056 MINFREQ, 7057 /** 7058 * Definition:There should be no actions taken in fulfillment of a request that 7059 * has been held or suspended. 7060 */ 7061 HELD, 7062 /** 7063 * The patient is receiving a subsequent fill significantly later than would be 7064 * expected based on the amount previously supplied and the therapy dosage 7065 * instructions 7066 */ 7067 TOOLATE, 7068 /** 7069 * The patient is receiving a subsequent fill significantly earlier than would 7070 * be expected based on the amount previously supplied and the therapy dosage 7071 * instructions 7072 */ 7073 TOOSOON, 7074 /** 7075 * Description: While the record was accepted in the repository, there is a more 7076 * recent version of a record of this type. 7077 */ 7078 HISTORIC, 7079 /** 7080 * Definition:The proposed therapy goes against preferences or consent 7081 * constraints recorded in the patient's record. 7082 */ 7083 PATPREF, 7084 /** 7085 * Definition:The proposed therapy goes against preferences or consent 7086 * constraints recorded in the patient's record. An alternate therapy meeting 7087 * those constraints is available. 7088 */ 7089 PATPREFALT, 7090 /** 7091 * Categorization of types of observation that capture the main clinical 7092 * knowledge subject which may be a medication, a laboratory test, a disease. 7093 */ 7094 KSUBJ, 7095 /** 7096 * Categorization of types of observation that capture a knowledge subtopic 7097 * which might be treatment, etiology, or prognosis. 7098 */ 7099 KSUBT, 7100 /** 7101 * Hypersensitivity resulting in an adverse reaction upon exposure to an agent. 7102 */ 7103 OINT, 7104 /** 7105 * Hypersensitivity to an agent caused by an immunologic response to an initial 7106 * exposure 7107 */ 7108 ALG, 7109 /** 7110 * An allergy to a pharmaceutical product. 7111 */ 7112 DALG, 7113 /** 7114 * An allergy to a substance other than a drug or a food. E.g. Latex, pollen, 7115 * etc. 7116 */ 7117 EALG, 7118 /** 7119 * An allergy to a substance generally consumed for nutritional purposes. 7120 */ 7121 FALG, 7122 /** 7123 * Hypersensitivity resulting in an adverse reaction upon exposure to a drug. 7124 */ 7125 DINT, 7126 /** 7127 * Hypersensitivity to an agent caused by a mechanism other than an immunologic 7128 * response to an initial exposure 7129 */ 7130 DNAINT, 7131 /** 7132 * Hypersensitivity resulting in an adverse reaction upon exposure to 7133 * environmental conditions. 7134 */ 7135 EINT, 7136 /** 7137 * Hypersensitivity to an agent caused by a mechanism other than an immunologic 7138 * response to an initial exposure 7139 */ 7140 ENAINT, 7141 /** 7142 * Hypersensitivity resulting in an adverse reaction upon exposure to food. 7143 */ 7144 FINT, 7145 /** 7146 * Hypersensitivity to an agent caused by a mechanism other than an immunologic 7147 * response to an initial exposure 7148 */ 7149 FNAINT, 7150 /** 7151 * Hypersensitivity to an agent caused by a mechanism other than an immunologic 7152 * response to an initial exposure 7153 */ 7154 NAINT, 7155 /** 7156 * A subjective evaluation of the seriousness or intensity associated with 7157 * another observation. 7158 */ 7159 SEV, 7160 /** 7161 * FDA label data 7162 */ 7163 _FDALABELDATA, 7164 /** 7165 * FDA label coating 7166 */ 7167 FDACOATING, 7168 /** 7169 * FDA label color 7170 */ 7171 FDACOLOR, 7172 /** 7173 * FDA label imprint code 7174 */ 7175 FDAIMPRINTCD, 7176 /** 7177 * FDA label logo 7178 */ 7179 FDALOGO, 7180 /** 7181 * FDA label scoring 7182 */ 7183 FDASCORING, 7184 /** 7185 * FDA label shape 7186 */ 7187 FDASHAPE, 7188 /** 7189 * FDA label size 7190 */ 7191 FDASIZE, 7192 /** 7193 * Shape of the region on the object being referenced 7194 */ 7195 _ROIOVERLAYSHAPE, 7196 /** 7197 * A circle defined by two (column,row) pairs. The first point is the center of 7198 * the circle and the second point is a point on the perimeter of the circle. 7199 */ 7200 CIRCLE, 7201 /** 7202 * An ellipse defined by four (column,row) pairs, the first two points 7203 * specifying the endpoints of the major axis and the second two points 7204 * specifying the endpoints of the minor axis. 7205 */ 7206 ELLIPSE, 7207 /** 7208 * A single point denoted by a single (column,row) pair, or multiple points each 7209 * denoted by a (column,row) pair. 7210 */ 7211 POINT, 7212 /** 7213 * A series of connected line segments with ordered vertices denoted by 7214 * (column,row) pairs; if the first and last vertices are the same, it is a 7215 * closed polygon. 7216 */ 7217 POLY, 7218 /** 7219 * Description:Indicates that result data has been corrected. 7220 */ 7221 C, 7222 /** 7223 * Code set to define specialized/allowed diets 7224 */ 7225 DIET, 7226 /** 7227 * A diet exclusively composed of oatmeal, semolina, or rice, to be extremely 7228 * easy to eat and digest. 7229 */ 7230 BR, 7231 /** 7232 * A diet that uses carbohydrates sparingly. Typically with a restriction in 7233 * daily energy content (e.g. 1600-2000 kcal). 7234 */ 7235 DM, 7236 /** 7237 * No enteral intake of foot or liquids whatsoever, no smoking. Typically 6 to 8 7238 * hours before anesthesia. 7239 */ 7240 FAST, 7241 /** 7242 * A diet consisting of a formula feeding, either for an infant or an adult, to 7243 * provide nutrition either orally or through the gastrointestinal tract via 7244 * tube, catheter or stoma. 7245 */ 7246 FORMULA, 7247 /** 7248 * Gluten free diet for celiac disease. 7249 */ 7250 GF, 7251 /** 7252 * A diet low in fat, particularly to patients with hepatic diseases. 7253 */ 7254 LF, 7255 /** 7256 * A low protein diet for patients with renal failure. 7257 */ 7258 LP, 7259 /** 7260 * A strictly liquid diet, that can be fully absorbed in the intestine, and 7261 * therefore may not contain fiber. Used before enteral surgeries. 7262 */ 7263 LQ, 7264 /** 7265 * A diet low in sodium for patients with congestive heart failure and/or renal 7266 * failure. 7267 */ 7268 LS, 7269 /** 7270 * A normal diet, i.e. no special preparations or restrictions for medical 7271 * reasons. This is notwithstanding any preferences the patient might have 7272 * regarding special foods, such as vegetarian, kosher, etc. 7273 */ 7274 N, 7275 /** 7276 * A no fat diet for acute hepatic diseases. 7277 */ 7278 NF, 7279 /** 7280 * Phenylketonuria diet. 7281 */ 7282 PAF, 7283 /** 7284 * Patient is supplied with parenteral nutrition, typically described in terms 7285 * of i.v. medications. 7286 */ 7287 PAR, 7288 /** 7289 * A diet that seeks to reduce body fat, typically low energy content (800-1600 7290 * kcal). 7291 */ 7292 RD, 7293 /** 7294 * A diet that avoids ingredients that might cause digestion problems, e.g., 7295 * avoid excessive fat, avoid too much fiber (cabbage, peas, beans). 7296 */ 7297 SCH, 7298 /** 7299 * A diet that is not intended to be complete but is added to other diets. 7300 */ 7301 SUPPLEMENT, 7302 /** 7303 * This is not really a diet, since it contains little nutritional value, but is 7304 * essentially just water. Used before coloscopy examinations. 7305 */ 7306 T, 7307 /** 7308 * Diet with low content of the amino-acids valin, leucin, and isoleucin, for 7309 * "maple syrup disease." 7310 */ 7311 VLI, 7312 /** 7313 * Definition: A public or government health program that administers and funds 7314 * coverage for prescription drugs to assist program eligible who meet financial 7315 * and health status criteria. 7316 */ 7317 DRUGPRG, 7318 /** 7319 * Description:Indicates that a result is complete. No further results are to 7320 * come. This maps to the 'complete' state in the observation result status 7321 * code. 7322 */ 7323 F, 7324 /** 7325 * Description:Indicates that a result is incomplete. There are further results 7326 * to come. This maps to the 'active' state in the observation result status 7327 * code. 7328 */ 7329 PRLMN, 7330 /** 7331 * An observation identifying security metadata about an IT resource (data, 7332 * information object, service, or system capability), which may be used to make 7333 * access control decisions. Security metadata are used to name security labels. 7334 * 7335 * 7336 * Rationale: According to ISO/TS 22600-3:2009(E) A.9.1.7 SECURITY LABEL 7337 * MATCHING, Security label matching compares the initiator's clearance to the 7338 * target's security label. All of the following must be true for authorization 7339 * to be granted: 7340 * 7341 * 7342 * The security policy identifiers shall be identical The classification level 7343 * of the initiator shall be greater than or equal to that of the target (that 7344 * is, there shall be at least one value in the classification list of the 7345 * clearance greater than or equal to the classification of the target), and For 7346 * each security category in the target label, there shall be a security 7347 * category of the same type in the initiator's clearance and the initiator's 7348 * classification level shall dominate that of the target. 7349 * 7350 * 7351 * Examples: SecurityObservationType security label fields include: 7352 * 7353 * 7354 * Confidentiality classification Compartment category Sensitivity category 7355 * Security mechanisms used to ensure data integrity or to perform authorized 7356 * data transformation Indicators of an IT resource completeness, veracity, 7357 * reliability, trustworthiness, or provenance. 7358 * 7359 * 7360 * Usage Note: SecurityObservationType codes designate security label field 7361 * types, which are valued with an applicable SecurityObservationValue code as 7362 * the "security label tag". 7363 */ 7364 SECOBS, 7365 /** 7366 * Type of security metadata observation made about the category of an IT 7367 * resource (data, information object, service, or system capability), which may 7368 * be used to make access control decisions. Security category metadata is 7369 * defined by ISO/IEC 2382-8:1998(E/F)/ T-REC-X.812-1995 as: "A nonhierarchical 7370 * grouping of sensitive information used to control access to data more finely 7371 * than with hierarchical security classification alone." 7372 * 7373 * 7374 * Rationale: A security category observation supports requirement to specify 7375 * the type of IT resource to facilitate application of appropriate levels of 7376 * information security according to a range of levels of impact or consequences 7377 * that might result from the unauthorized disclosure, modification, or use of 7378 * the information or information system. A resource is assigned to a specific 7379 * category of information (e.g., privacy, medical, proprietary, financial, 7380 * investigative, contractor sensitive, security management) defined by an 7381 * organization or in some instances, by a specific law, Executive Order, 7382 * directive, policy, or regulation. [FIPS 199] 7383 * 7384 * 7385 * Examples: Types of security categories include: 7386 * 7387 * 7388 * Compartment: A division of data into isolated blocks with separate security 7389 * controls for the purpose of reducing risk. (ISO 2382-8). A security label tag 7390 * that "segments" an IT resource by indicating that access and use is 7391 * restricted to members of a defined community or project. (HL7 Healthcare 7392 * Classification System) Sensitivity: The characteristic of an IT resource 7393 * which implies its value or importance and may include its vulnerability. (ISO 7394 * 7492-2) Privacy metadata for information perceived as undesirable to share. 7395 * (HL7 Healthcare Classification System) 7396 */ 7397 SECCATOBS, 7398 /** 7399 * Type of security metadata observation made about the classification of an IT 7400 * resource (data, information object, service, or system capability), which may 7401 * be used to make access control decisions. Security classification is defined 7402 * by ISO/IEC 2382-8:1998(E/F)/ T-REC-X.812-1995 as: "The determination of which 7403 * specific degree of protection against access the data or information 7404 * requires, together with a designation of that degree of protection." Security 7405 * classification metadata is based on an analysis of applicable policies and 7406 * the risk of financial, reputational, or other harm that could result from 7407 * unauthorized disclosure. 7408 * 7409 * 7410 * Rationale: A security classification observation may indicate that the 7411 * confidentiality level indicated by an Act or Role confidentiality attribute 7412 * has been overridden by the entity responsible for ascribing the 7413 * SecurityClassificationObservationValue. This supports the business 7414 * requirement for increasing or decreasing the level of confidentiality 7415 * (classification or declassification) based on parameters beyond the original 7416 * assignment of an Act or Role confidentiality. 7417 * 7418 * 7419 * Examples: Types of security classification include: HL7 Confidentiality Codes 7420 * such as very restricted, unrestricted, and normal. Intelligence community 7421 * examples include top secret, secret, and confidential. 7422 * 7423 * 7424 * Usage Note: Security classification observation type codes designate security 7425 * label field types, which are valued with an applicable 7426 * SecurityClassificationObservationValue code as the "security label tag". 7427 */ 7428 SECCLASSOBS, 7429 /** 7430 * Type of security metadata observation made about the control of an IT 7431 * resource (data, information object, service, or system capability), which may 7432 * be used to make access control decisions. Security control metadata convey 7433 * instructions to users and receivers for secure distribution, transmission, 7434 * and storage; dictate obligations or mandated actions; specify any action 7435 * prohibited by refrain policy such as dissemination controls; and stipulate 7436 * the permissible purpose of use of an IT resource. 7437 * 7438 * 7439 * Rationale: A security control observation supports requirement to specify 7440 * applicable management, operational, and technical controls (i.e., safeguards 7441 * or countermeasures) prescribed for an information system to protect the 7442 * confidentiality, integrity, and availability of the system and its 7443 * information. [FIPS 199] 7444 * 7445 * 7446 * Examples: Types of security control metadata include: 7447 * 7448 * 7449 * handling caveats dissemination controls obligations refrain policies purpose 7450 * of use constraints 7451 */ 7452 SECCONOBS, 7453 /** 7454 * Type of security metadata observation made about the integrity of an IT 7455 * resource (data, information object, service, or system capability), which may 7456 * be used to make access control decisions. 7457 * 7458 * 7459 * Rationale: A security integrity observation supports the requirement to guard 7460 * against improper information modification or destruction, and includes 7461 * ensuring information non-repudiation and authenticity. (44 U.S.C., SEC. 3542) 7462 * 7463 * 7464 * Examples: Types of security integrity metadata include: 7465 * 7466 * 7467 * Integrity status, which indicates the completeness or workflow status of an 7468 * IT resource (data, information object, service, or system capability) 7469 * Integrity confidence, which indicates the reliability and trustworthiness of 7470 * an IT resource Integrity control, which indicates pertinent handling caveats, 7471 * obligations, refrain policies, and purpose of use for the resource Data 7472 * integrity, which indicate the security mechanisms used to ensure that the 7473 * accuracy and consistency are preserved regardless of changes made (ISO/IEC 7474 * DIS 2382-8) Alteration integrity, which indicate the security mechanisms used 7475 * for authorized transformations of the resource Integrity provenance, which 7476 * indicates the entity responsible for a report or assertion relayed 7477 * "second-hand" about an IT resource 7478 */ 7479 SECINTOBS, 7480 /** 7481 * Type of security metadata observation made about the alteration integrity of 7482 * an IT resource (data, information object, service, or system capability), 7483 * which indicates the mechanism used for authorized transformations of the 7484 * resource. 7485 * 7486 * 7487 * Examples: Types of security alteration integrity observation metadata, which 7488 * may value the observation with a code used to indicate the mechanism used for 7489 * authorized transformation of an IT resource, including: 7490 * 7491 * 7492 * translation syntactic transformation semantic mapping redaction masking 7493 * pseudonymization anonymization 7494 */ 7495 SECALTINTOBS, 7496 /** 7497 * Type of security metadata observation made about the data integrity of an IT 7498 * resource (data, information object, service, or system capability), which 7499 * indicates the security mechanism used to preserve resource accuracy and 7500 * consistency. Data integrity is defined by ISO 22600-23.3.21 as: "The property 7501 * that data has not been altered or destroyed in an unauthorized manner", and 7502 * by ISO/IEC 2382-8: The property of data whose accuracy and consistency are 7503 * preserved regardless of changes made." 7504 * 7505 * 7506 * Examples: Types of security data integrity observation metadata, which may 7507 * value the observation, include cryptographic hash function and digital 7508 * signature. 7509 */ 7510 SECDATINTOBS, 7511 /** 7512 * Type of security metadata observation made about the integrity confidence of 7513 * an IT resource (data, information object, service, or system capability), 7514 * which may be used to make access control decisions. 7515 * 7516 * 7517 * Examples: Types of security integrity confidence observation metadata, which 7518 * may value the observation, include highly reliable, uncertain reliability, 7519 * and not reliable. 7520 * 7521 * 7522 * Usage Note: A security integrity confidence observation on an Act may 7523 * indicate that a valued Act.uncertaintycode attribute has been overridden by 7524 * the entity responsible for ascribing the 7525 * SecurityIntegrityConfidenceObservationValue. This supports the business 7526 * requirements for increasing or decreasing the assessment of the reliability 7527 * or trustworthiness of an IT resource based on parameters beyond the original 7528 * assignment of an Act statement level of uncertainty. 7529 */ 7530 SECINTCONOBS, 7531 /** 7532 * Type of security metadata observation made about the provenance integrity of 7533 * an IT resource (data, information object, service, or system capability), 7534 * which indicates the lifecycle completeness of an IT resource in terms of 7535 * workflow status such as its creation, modification, suspension, and deletion; 7536 * locations in which the resource has been collected or archived, from which it 7537 * may be retrieved, and the history of its distribution and disclosure. 7538 * Integrity provenance metadata about an IT resource may be used to assess its 7539 * veracity, reliability, and trustworthiness. 7540 * 7541 * 7542 * Examples: Types of security integrity provenance observation metadata, which 7543 * may value the observation about an IT resource, include: 7544 * 7545 * 7546 * completeness or workflow status, such as authentication the entity 7547 * responsible for original authoring or informing about an IT resource the 7548 * entity responsible for a report or assertion about an IT resource relayed 7549 * â??second-handâ?? the entity responsible for excerpting, transforming, or 7550 * compiling an IT resource 7551 */ 7552 SECINTPRVOBS, 7553 /** 7554 * Type of security metadata observation made about the integrity provenance of 7555 * an IT resource (data, information object, service, or system capability), 7556 * which indicates the entity that made assertions about the resource. The 7557 * asserting entity may not be the original informant about the resource. 7558 * 7559 * 7560 * Examples: Types of security integrity provenance asserted by observation 7561 * metadata, which may value the observation, including: 7562 * 7563 * 7564 * assertions about an IT resource by a patient assertions about an IT resource 7565 * by a clinician assertions about an IT resource by a device 7566 */ 7567 SECINTPRVABOBS, 7568 /** 7569 * Type of security metadata observation made about the integrity provenance of 7570 * an IT resource (data, information object, service, or system capability), 7571 * which indicates the entity that reported the existence of the resource. The 7572 * reporting entity may not be the original author of the resource. 7573 * 7574 * 7575 * Examples: Types of security integrity provenance reported by observation 7576 * metadata, which may value the observation, include: 7577 * 7578 * 7579 * reports about an IT resource by a patient reports about an IT resource by a 7580 * clinician reports about an IT resource by a device 7581 */ 7582 SECINTPRVRBOBS, 7583 /** 7584 * Type of security metadata observation made about the integrity status of an 7585 * IT resource (data, information object, service, or system capability), which 7586 * may be used to make access control decisions. Indicates the completeness of 7587 * an IT resource in terms of workflow status, which may impact users that are 7588 * authorized to access and use the resource. 7589 * 7590 * 7591 * Examples: Types of security integrity status observation metadata, which may 7592 * value the observation, include codes from the HL7 DocumentCompletion code 7593 * system such as legally authenticated, in progress, and incomplete. 7594 */ 7595 SECINTSTOBS, 7596 /** 7597 * An observation identifying trust metadata about an IT resource (data, 7598 * information object, service, or system capability), which may be used as a 7599 * trust attribute to populate a computable trust policy, trust credential, 7600 * trust assertion, or trust label field in a security label or trust policy, 7601 * which are principally used for authentication, authorization, and access 7602 * control decisions. 7603 */ 7604 SECTRSTOBS, 7605 /** 7606 * Type of security metadata observation made about the formal declaration by an 7607 * authority or neutral third party that validates the technical, security, 7608 * trust, and business practice conformance of Trust Agents to facilitate 7609 * security, interoperability, and trust among participants within a security 7610 * domain or trust framework. 7611 */ 7612 TRSTACCRDOBS, 7613 /** 7614 * Type of security metadata observation made about privacy and security 7615 * requirements with which a security domain must comply. [ISO IEC 10181-1] 7616 */ 7617 TRSTAGREOBS, 7618 /** 7619 * Type of security metadata observation made about a set of security-relevant 7620 * data issued by a security authority or trusted third party, together with 7621 * security information which is used to provide the integrity and data origin 7622 * authentication services for an IT resource (data, information object, 7623 * service, or system capability). [Based on ISO IEC 10181-1] 7624 * 7625 * 7626 * For example, 7627 * 7628 * 7629 * 7630 * A Certificate Policy (CP), which is a named set of rules that indicates the 7631 * applicability of a certificate to a particular community and/or class of 7632 * application with common security requirements. For example, a particular 7633 * Certificate Policy might indicate the applicability of a type of certificate 7634 * to the authentication of electronic data interchange transactions for the 7635 * trading of goods within a given price range. [Trust Service Principles and 7636 * Criteria for Certification Authorities Version 2.0 March 2011 Copyright 2011 7637 * by Canadian Institute of Chartered Accountants. A Certificate Practice 7638 * Statement (CSP), which is a statement of the practices which an Authority 7639 * employs in issuing and managing certificates. [Trust Service Principles and 7640 * Criteria for Certification Authorities Version 2.0 March 2011 Copyright 2011 7641 * by Canadian Institute of Chartered Accountants.] 7642 */ 7643 TRSTCERTOBS, 7644 /** 7645 * Type of security metadata observation made about a complete set of contracts, 7646 * regulations or commitments that enable participating actors to rely on 7647 * certain assertions by other actors to fulfill their information security 7648 * requirements. [Kantara Initiative] 7649 */ 7650 TRSTFWKOBS, 7651 /** 7652 * Type of security metadata observation made about the digital quality or 7653 * reliability of a trust assertion, activity, capability, information exchange, 7654 * mechanism, process, or protocol. 7655 */ 7656 TRSTLOAOBS, 7657 /** 7658 * Type of security metadata observation made about a security architecture 7659 * system component that supports enforcement of security policies. 7660 */ 7661 TRSTMECOBS, 7662 /** 7663 * Definition: A government health program that provides coverage on a fee for 7664 * service basis for health services to persons meeting eligibility criteria 7665 * such as income, location of residence, access to other coverages, health 7666 * condition, and age, the cost of which is to some extent subsidized by public 7667 * funds. 7668 * 7669 * 7670 * Discussion: The structure and business processes for underwriting and 7671 * administering a subsidized fee for service program is further specified by 7672 * the Underwriter and Payer Role.class and Role.code. 7673 */ 7674 SUBSIDFFS, 7675 /** 7676 * Definition: Government mandated program providing coverage, disability 7677 * income, and vocational rehabilitation for injuries sustained in the work 7678 * place or in the course of employment. Employers may either self-fund the 7679 * program, purchase commercial coverage, or pay a premium to a government 7680 * entity that administers the program. Employees may be required to pay 7681 * premiums toward the cost of coverage as well. 7682 */ 7683 WRKCOMP, 7684 /** 7685 * An identifying code for healthcare interventions/procedures. 7686 */ 7687 _ACTPROCEDURECODE, 7688 /** 7689 * Definition: An identifying code for billable services, as opposed to codes 7690 * for similar services used to identify them for functional purposes. 7691 */ 7692 _ACTBILLABLESERVICECODE, 7693 /** 7694 * Domain provides the root for HL7-defined detailed or rich codes for the Act 7695 * classes. 7696 */ 7697 _HL7DEFINEDACTCODES, 7698 /** 7699 * null 7700 */ 7701 COPAY, 7702 /** 7703 * null 7704 */ 7705 DEDUCT, 7706 /** 7707 * null 7708 */ 7709 DOSEIND, 7710 /** 7711 * null 7712 */ 7713 PRA, 7714 /** 7715 * The act of putting something away for safe keeping. The "something" may be 7716 * physical object such as a specimen, or information, such as observations 7717 * regarding a specimen. 7718 */ 7719 STORE, 7720 /** 7721 * added to help the parsers 7722 */ 7723 NULL; 7724 7725 public static V3ActCode fromCode(String codeString) throws FHIRException { 7726 if (codeString == null || "".equals(codeString)) 7727 return null; 7728 if ("_ActAccountCode".equals(codeString)) 7729 return _ACTACCOUNTCODE; 7730 if ("ACCTRECEIVABLE".equals(codeString)) 7731 return ACCTRECEIVABLE; 7732 if ("CASH".equals(codeString)) 7733 return CASH; 7734 if ("CC".equals(codeString)) 7735 return CC; 7736 if ("AE".equals(codeString)) 7737 return AE; 7738 if ("DN".equals(codeString)) 7739 return DN; 7740 if ("DV".equals(codeString)) 7741 return DV; 7742 if ("MC".equals(codeString)) 7743 return MC; 7744 if ("V".equals(codeString)) 7745 return V; 7746 if ("PBILLACCT".equals(codeString)) 7747 return PBILLACCT; 7748 if ("_ActAdjudicationCode".equals(codeString)) 7749 return _ACTADJUDICATIONCODE; 7750 if ("_ActAdjudicationGroupCode".equals(codeString)) 7751 return _ACTADJUDICATIONGROUPCODE; 7752 if ("CONT".equals(codeString)) 7753 return CONT; 7754 if ("DAY".equals(codeString)) 7755 return DAY; 7756 if ("LOC".equals(codeString)) 7757 return LOC; 7758 if ("MONTH".equals(codeString)) 7759 return MONTH; 7760 if ("PERIOD".equals(codeString)) 7761 return PERIOD; 7762 if ("PROV".equals(codeString)) 7763 return PROV; 7764 if ("WEEK".equals(codeString)) 7765 return WEEK; 7766 if ("YEAR".equals(codeString)) 7767 return YEAR; 7768 if ("AA".equals(codeString)) 7769 return AA; 7770 if ("ANF".equals(codeString)) 7771 return ANF; 7772 if ("AR".equals(codeString)) 7773 return AR; 7774 if ("AS".equals(codeString)) 7775 return AS; 7776 if ("_ActAdjudicationResultActionCode".equals(codeString)) 7777 return _ACTADJUDICATIONRESULTACTIONCODE; 7778 if ("DISPLAY".equals(codeString)) 7779 return DISPLAY; 7780 if ("FORM".equals(codeString)) 7781 return FORM; 7782 if ("_ActBillableModifierCode".equals(codeString)) 7783 return _ACTBILLABLEMODIFIERCODE; 7784 if ("CPTM".equals(codeString)) 7785 return CPTM; 7786 if ("HCPCSA".equals(codeString)) 7787 return HCPCSA; 7788 if ("_ActBillingArrangementCode".equals(codeString)) 7789 return _ACTBILLINGARRANGEMENTCODE; 7790 if ("BLK".equals(codeString)) 7791 return BLK; 7792 if ("CAP".equals(codeString)) 7793 return CAP; 7794 if ("CONTF".equals(codeString)) 7795 return CONTF; 7796 if ("FINBILL".equals(codeString)) 7797 return FINBILL; 7798 if ("ROST".equals(codeString)) 7799 return ROST; 7800 if ("SESS".equals(codeString)) 7801 return SESS; 7802 if ("FFS".equals(codeString)) 7803 return FFS; 7804 if ("FFPS".equals(codeString)) 7805 return FFPS; 7806 if ("FFCS".equals(codeString)) 7807 return FFCS; 7808 if ("TFS".equals(codeString)) 7809 return TFS; 7810 if ("_ActBoundedROICode".equals(codeString)) 7811 return _ACTBOUNDEDROICODE; 7812 if ("ROIFS".equals(codeString)) 7813 return ROIFS; 7814 if ("ROIPS".equals(codeString)) 7815 return ROIPS; 7816 if ("_ActCareProvisionCode".equals(codeString)) 7817 return _ACTCAREPROVISIONCODE; 7818 if ("_ActCredentialedCareCode".equals(codeString)) 7819 return _ACTCREDENTIALEDCARECODE; 7820 if ("_ActCredentialedCareProvisionPersonCode".equals(codeString)) 7821 return _ACTCREDENTIALEDCAREPROVISIONPERSONCODE; 7822 if ("CACC".equals(codeString)) 7823 return CACC; 7824 if ("CAIC".equals(codeString)) 7825 return CAIC; 7826 if ("CAMC".equals(codeString)) 7827 return CAMC; 7828 if ("CANC".equals(codeString)) 7829 return CANC; 7830 if ("CAPC".equals(codeString)) 7831 return CAPC; 7832 if ("CBGC".equals(codeString)) 7833 return CBGC; 7834 if ("CCCC".equals(codeString)) 7835 return CCCC; 7836 if ("CCGC".equals(codeString)) 7837 return CCGC; 7838 if ("CCPC".equals(codeString)) 7839 return CCPC; 7840 if ("CCSC".equals(codeString)) 7841 return CCSC; 7842 if ("CDEC".equals(codeString)) 7843 return CDEC; 7844 if ("CDRC".equals(codeString)) 7845 return CDRC; 7846 if ("CEMC".equals(codeString)) 7847 return CEMC; 7848 if ("CFPC".equals(codeString)) 7849 return CFPC; 7850 if ("CIMC".equals(codeString)) 7851 return CIMC; 7852 if ("CMGC".equals(codeString)) 7853 return CMGC; 7854 if ("CNEC".equals(codeString)) 7855 return CNEC; 7856 if ("CNMC".equals(codeString)) 7857 return CNMC; 7858 if ("CNQC".equals(codeString)) 7859 return CNQC; 7860 if ("CNSC".equals(codeString)) 7861 return CNSC; 7862 if ("COGC".equals(codeString)) 7863 return COGC; 7864 if ("COMC".equals(codeString)) 7865 return COMC; 7866 if ("COPC".equals(codeString)) 7867 return COPC; 7868 if ("COSC".equals(codeString)) 7869 return COSC; 7870 if ("COTC".equals(codeString)) 7871 return COTC; 7872 if ("CPEC".equals(codeString)) 7873 return CPEC; 7874 if ("CPGC".equals(codeString)) 7875 return CPGC; 7876 if ("CPHC".equals(codeString)) 7877 return CPHC; 7878 if ("CPRC".equals(codeString)) 7879 return CPRC; 7880 if ("CPSC".equals(codeString)) 7881 return CPSC; 7882 if ("CPYC".equals(codeString)) 7883 return CPYC; 7884 if ("CROC".equals(codeString)) 7885 return CROC; 7886 if ("CRPC".equals(codeString)) 7887 return CRPC; 7888 if ("CSUC".equals(codeString)) 7889 return CSUC; 7890 if ("CTSC".equals(codeString)) 7891 return CTSC; 7892 if ("CURC".equals(codeString)) 7893 return CURC; 7894 if ("CVSC".equals(codeString)) 7895 return CVSC; 7896 if ("LGPC".equals(codeString)) 7897 return LGPC; 7898 if ("_ActCredentialedCareProvisionProgramCode".equals(codeString)) 7899 return _ACTCREDENTIALEDCAREPROVISIONPROGRAMCODE; 7900 if ("AALC".equals(codeString)) 7901 return AALC; 7902 if ("AAMC".equals(codeString)) 7903 return AAMC; 7904 if ("ABHC".equals(codeString)) 7905 return ABHC; 7906 if ("ACAC".equals(codeString)) 7907 return ACAC; 7908 if ("ACHC".equals(codeString)) 7909 return ACHC; 7910 if ("AHOC".equals(codeString)) 7911 return AHOC; 7912 if ("ALTC".equals(codeString)) 7913 return ALTC; 7914 if ("AOSC".equals(codeString)) 7915 return AOSC; 7916 if ("CACS".equals(codeString)) 7917 return CACS; 7918 if ("CAMI".equals(codeString)) 7919 return CAMI; 7920 if ("CAST".equals(codeString)) 7921 return CAST; 7922 if ("CBAR".equals(codeString)) 7923 return CBAR; 7924 if ("CCAD".equals(codeString)) 7925 return CCAD; 7926 if ("CCAR".equals(codeString)) 7927 return CCAR; 7928 if ("CDEP".equals(codeString)) 7929 return CDEP; 7930 if ("CDGD".equals(codeString)) 7931 return CDGD; 7932 if ("CDIA".equals(codeString)) 7933 return CDIA; 7934 if ("CEPI".equals(codeString)) 7935 return CEPI; 7936 if ("CFEL".equals(codeString)) 7937 return CFEL; 7938 if ("CHFC".equals(codeString)) 7939 return CHFC; 7940 if ("CHRO".equals(codeString)) 7941 return CHRO; 7942 if ("CHYP".equals(codeString)) 7943 return CHYP; 7944 if ("CMIH".equals(codeString)) 7945 return CMIH; 7946 if ("CMSC".equals(codeString)) 7947 return CMSC; 7948 if ("COJR".equals(codeString)) 7949 return COJR; 7950 if ("CONC".equals(codeString)) 7951 return CONC; 7952 if ("COPD".equals(codeString)) 7953 return COPD; 7954 if ("CORT".equals(codeString)) 7955 return CORT; 7956 if ("CPAD".equals(codeString)) 7957 return CPAD; 7958 if ("CPND".equals(codeString)) 7959 return CPND; 7960 if ("CPST".equals(codeString)) 7961 return CPST; 7962 if ("CSDM".equals(codeString)) 7963 return CSDM; 7964 if ("CSIC".equals(codeString)) 7965 return CSIC; 7966 if ("CSLD".equals(codeString)) 7967 return CSLD; 7968 if ("CSPT".equals(codeString)) 7969 return CSPT; 7970 if ("CTBU".equals(codeString)) 7971 return CTBU; 7972 if ("CVDC".equals(codeString)) 7973 return CVDC; 7974 if ("CWMA".equals(codeString)) 7975 return CWMA; 7976 if ("CWOH".equals(codeString)) 7977 return CWOH; 7978 if ("_ActEncounterCode".equals(codeString)) 7979 return _ACTENCOUNTERCODE; 7980 if ("AMB".equals(codeString)) 7981 return AMB; 7982 if ("EMER".equals(codeString)) 7983 return EMER; 7984 if ("FLD".equals(codeString)) 7985 return FLD; 7986 if ("HH".equals(codeString)) 7987 return HH; 7988 if ("IMP".equals(codeString)) 7989 return IMP; 7990 if ("ACUTE".equals(codeString)) 7991 return ACUTE; 7992 if ("NONAC".equals(codeString)) 7993 return NONAC; 7994 if ("OBSENC".equals(codeString)) 7995 return OBSENC; 7996 if ("PRENC".equals(codeString)) 7997 return PRENC; 7998 if ("SS".equals(codeString)) 7999 return SS; 8000 if ("VR".equals(codeString)) 8001 return VR; 8002 if ("_ActMedicalServiceCode".equals(codeString)) 8003 return _ACTMEDICALSERVICECODE; 8004 if ("ALC".equals(codeString)) 8005 return ALC; 8006 if ("CARD".equals(codeString)) 8007 return CARD; 8008 if ("CHR".equals(codeString)) 8009 return CHR; 8010 if ("DNTL".equals(codeString)) 8011 return DNTL; 8012 if ("DRGRHB".equals(codeString)) 8013 return DRGRHB; 8014 if ("GENRL".equals(codeString)) 8015 return GENRL; 8016 if ("MED".equals(codeString)) 8017 return MED; 8018 if ("OBS".equals(codeString)) 8019 return OBS; 8020 if ("ONC".equals(codeString)) 8021 return ONC; 8022 if ("PALL".equals(codeString)) 8023 return PALL; 8024 if ("PED".equals(codeString)) 8025 return PED; 8026 if ("PHAR".equals(codeString)) 8027 return PHAR; 8028 if ("PHYRHB".equals(codeString)) 8029 return PHYRHB; 8030 if ("PSYCH".equals(codeString)) 8031 return PSYCH; 8032 if ("SURG".equals(codeString)) 8033 return SURG; 8034 if ("_ActClaimAttachmentCategoryCode".equals(codeString)) 8035 return _ACTCLAIMATTACHMENTCATEGORYCODE; 8036 if ("AUTOATTCH".equals(codeString)) 8037 return AUTOATTCH; 8038 if ("DOCUMENT".equals(codeString)) 8039 return DOCUMENT; 8040 if ("HEALTHREC".equals(codeString)) 8041 return HEALTHREC; 8042 if ("IMG".equals(codeString)) 8043 return IMG; 8044 if ("LABRESULTS".equals(codeString)) 8045 return LABRESULTS; 8046 if ("MODEL".equals(codeString)) 8047 return MODEL; 8048 if ("WIATTCH".equals(codeString)) 8049 return WIATTCH; 8050 if ("XRAY".equals(codeString)) 8051 return XRAY; 8052 if ("_ActConsentType".equals(codeString)) 8053 return _ACTCONSENTTYPE; 8054 if ("ICOL".equals(codeString)) 8055 return ICOL; 8056 if ("IDSCL".equals(codeString)) 8057 return IDSCL; 8058 if ("INFA".equals(codeString)) 8059 return INFA; 8060 if ("INFAO".equals(codeString)) 8061 return INFAO; 8062 if ("INFASO".equals(codeString)) 8063 return INFASO; 8064 if ("IRDSCL".equals(codeString)) 8065 return IRDSCL; 8066 if ("RESEARCH".equals(codeString)) 8067 return RESEARCH; 8068 if ("RSDID".equals(codeString)) 8069 return RSDID; 8070 if ("RSREID".equals(codeString)) 8071 return RSREID; 8072 if ("_ActContainerRegistrationCode".equals(codeString)) 8073 return _ACTCONTAINERREGISTRATIONCODE; 8074 if ("ID".equals(codeString)) 8075 return ID; 8076 if ("IP".equals(codeString)) 8077 return IP; 8078 if ("L".equals(codeString)) 8079 return L; 8080 if ("M".equals(codeString)) 8081 return M; 8082 if ("O".equals(codeString)) 8083 return O; 8084 if ("R".equals(codeString)) 8085 return R; 8086 if ("X".equals(codeString)) 8087 return X; 8088 if ("_ActControlVariable".equals(codeString)) 8089 return _ACTCONTROLVARIABLE; 8090 if ("AUTO".equals(codeString)) 8091 return AUTO; 8092 if ("ENDC".equals(codeString)) 8093 return ENDC; 8094 if ("REFLEX".equals(codeString)) 8095 return REFLEX; 8096 if ("_ActCoverageConfirmationCode".equals(codeString)) 8097 return _ACTCOVERAGECONFIRMATIONCODE; 8098 if ("_ActCoverageAuthorizationConfirmationCode".equals(codeString)) 8099 return _ACTCOVERAGEAUTHORIZATIONCONFIRMATIONCODE; 8100 if ("AUTH".equals(codeString)) 8101 return AUTH; 8102 if ("NAUTH".equals(codeString)) 8103 return NAUTH; 8104 if ("_ActCoverageEligibilityConfirmationCode".equals(codeString)) 8105 return _ACTCOVERAGEELIGIBILITYCONFIRMATIONCODE; 8106 if ("ELG".equals(codeString)) 8107 return ELG; 8108 if ("NELG".equals(codeString)) 8109 return NELG; 8110 if ("_ActCoverageLimitCode".equals(codeString)) 8111 return _ACTCOVERAGELIMITCODE; 8112 if ("_ActCoverageQuantityLimitCode".equals(codeString)) 8113 return _ACTCOVERAGEQUANTITYLIMITCODE; 8114 if ("COVPRD".equals(codeString)) 8115 return COVPRD; 8116 if ("LFEMX".equals(codeString)) 8117 return LFEMX; 8118 if ("NETAMT".equals(codeString)) 8119 return NETAMT; 8120 if ("PRDMX".equals(codeString)) 8121 return PRDMX; 8122 if ("UNITPRICE".equals(codeString)) 8123 return UNITPRICE; 8124 if ("UNITQTY".equals(codeString)) 8125 return UNITQTY; 8126 if ("COVMX".equals(codeString)) 8127 return COVMX; 8128 if ("_ActCoveredPartyLimitCode".equals(codeString)) 8129 return _ACTCOVEREDPARTYLIMITCODE; 8130 if ("_ActCoverageTypeCode".equals(codeString)) 8131 return _ACTCOVERAGETYPECODE; 8132 if ("_ActInsurancePolicyCode".equals(codeString)) 8133 return _ACTINSURANCEPOLICYCODE; 8134 if ("EHCPOL".equals(codeString)) 8135 return EHCPOL; 8136 if ("HSAPOL".equals(codeString)) 8137 return HSAPOL; 8138 if ("AUTOPOL".equals(codeString)) 8139 return AUTOPOL; 8140 if ("COL".equals(codeString)) 8141 return COL; 8142 if ("UNINSMOT".equals(codeString)) 8143 return UNINSMOT; 8144 if ("PUBLICPOL".equals(codeString)) 8145 return PUBLICPOL; 8146 if ("DENTPRG".equals(codeString)) 8147 return DENTPRG; 8148 if ("DISEASEPRG".equals(codeString)) 8149 return DISEASEPRG; 8150 if ("CANPRG".equals(codeString)) 8151 return CANPRG; 8152 if ("ENDRENAL".equals(codeString)) 8153 return ENDRENAL; 8154 if ("HIVAIDS".equals(codeString)) 8155 return HIVAIDS; 8156 if ("MANDPOL".equals(codeString)) 8157 return MANDPOL; 8158 if ("MENTPRG".equals(codeString)) 8159 return MENTPRG; 8160 if ("SAFNET".equals(codeString)) 8161 return SAFNET; 8162 if ("SUBPRG".equals(codeString)) 8163 return SUBPRG; 8164 if ("SUBSIDIZ".equals(codeString)) 8165 return SUBSIDIZ; 8166 if ("SUBSIDMC".equals(codeString)) 8167 return SUBSIDMC; 8168 if ("SUBSUPP".equals(codeString)) 8169 return SUBSUPP; 8170 if ("WCBPOL".equals(codeString)) 8171 return WCBPOL; 8172 if ("_ActInsuranceTypeCode".equals(codeString)) 8173 return _ACTINSURANCETYPECODE; 8174 if ("_ActHealthInsuranceTypeCode".equals(codeString)) 8175 return _ACTHEALTHINSURANCETYPECODE; 8176 if ("DENTAL".equals(codeString)) 8177 return DENTAL; 8178 if ("DISEASE".equals(codeString)) 8179 return DISEASE; 8180 if ("DRUGPOL".equals(codeString)) 8181 return DRUGPOL; 8182 if ("HIP".equals(codeString)) 8183 return HIP; 8184 if ("LTC".equals(codeString)) 8185 return LTC; 8186 if ("MCPOL".equals(codeString)) 8187 return MCPOL; 8188 if ("POS".equals(codeString)) 8189 return POS; 8190 if ("HMO".equals(codeString)) 8191 return HMO; 8192 if ("PPO".equals(codeString)) 8193 return PPO; 8194 if ("MENTPOL".equals(codeString)) 8195 return MENTPOL; 8196 if ("SUBPOL".equals(codeString)) 8197 return SUBPOL; 8198 if ("VISPOL".equals(codeString)) 8199 return VISPOL; 8200 if ("DIS".equals(codeString)) 8201 return DIS; 8202 if ("EWB".equals(codeString)) 8203 return EWB; 8204 if ("FLEXP".equals(codeString)) 8205 return FLEXP; 8206 if ("LIFE".equals(codeString)) 8207 return LIFE; 8208 if ("ANNU".equals(codeString)) 8209 return ANNU; 8210 if ("TLIFE".equals(codeString)) 8211 return TLIFE; 8212 if ("ULIFE".equals(codeString)) 8213 return ULIFE; 8214 if ("PNC".equals(codeString)) 8215 return PNC; 8216 if ("REI".equals(codeString)) 8217 return REI; 8218 if ("SURPL".equals(codeString)) 8219 return SURPL; 8220 if ("UMBRL".equals(codeString)) 8221 return UMBRL; 8222 if ("_ActProgramTypeCode".equals(codeString)) 8223 return _ACTPROGRAMTYPECODE; 8224 if ("CHAR".equals(codeString)) 8225 return CHAR; 8226 if ("CRIME".equals(codeString)) 8227 return CRIME; 8228 if ("EAP".equals(codeString)) 8229 return EAP; 8230 if ("GOVEMP".equals(codeString)) 8231 return GOVEMP; 8232 if ("HIRISK".equals(codeString)) 8233 return HIRISK; 8234 if ("IND".equals(codeString)) 8235 return IND; 8236 if ("MILITARY".equals(codeString)) 8237 return MILITARY; 8238 if ("RETIRE".equals(codeString)) 8239 return RETIRE; 8240 if ("SOCIAL".equals(codeString)) 8241 return SOCIAL; 8242 if ("VET".equals(codeString)) 8243 return VET; 8244 if ("_ActDetectedIssueManagementCode".equals(codeString)) 8245 return _ACTDETECTEDISSUEMANAGEMENTCODE; 8246 if ("_ActAdministrativeDetectedIssueManagementCode".equals(codeString)) 8247 return _ACTADMINISTRATIVEDETECTEDISSUEMANAGEMENTCODE; 8248 if ("_AuthorizationIssueManagementCode".equals(codeString)) 8249 return _AUTHORIZATIONISSUEMANAGEMENTCODE; 8250 if ("EMAUTH".equals(codeString)) 8251 return EMAUTH; 8252 if ("21".equals(codeString)) 8253 return _21; 8254 if ("1".equals(codeString)) 8255 return _1; 8256 if ("19".equals(codeString)) 8257 return _19; 8258 if ("2".equals(codeString)) 8259 return _2; 8260 if ("22".equals(codeString)) 8261 return _22; 8262 if ("23".equals(codeString)) 8263 return _23; 8264 if ("3".equals(codeString)) 8265 return _3; 8266 if ("4".equals(codeString)) 8267 return _4; 8268 if ("5".equals(codeString)) 8269 return _5; 8270 if ("6".equals(codeString)) 8271 return _6; 8272 if ("7".equals(codeString)) 8273 return _7; 8274 if ("14".equals(codeString)) 8275 return _14; 8276 if ("15".equals(codeString)) 8277 return _15; 8278 if ("16".equals(codeString)) 8279 return _16; 8280 if ("17".equals(codeString)) 8281 return _17; 8282 if ("18".equals(codeString)) 8283 return _18; 8284 if ("20".equals(codeString)) 8285 return _20; 8286 if ("8".equals(codeString)) 8287 return _8; 8288 if ("10".equals(codeString)) 8289 return _10; 8290 if ("11".equals(codeString)) 8291 return _11; 8292 if ("12".equals(codeString)) 8293 return _12; 8294 if ("13".equals(codeString)) 8295 return _13; 8296 if ("9".equals(codeString)) 8297 return _9; 8298 if ("_ActExposureCode".equals(codeString)) 8299 return _ACTEXPOSURECODE; 8300 if ("CHLDCARE".equals(codeString)) 8301 return CHLDCARE; 8302 if ("CONVEYNC".equals(codeString)) 8303 return CONVEYNC; 8304 if ("HLTHCARE".equals(codeString)) 8305 return HLTHCARE; 8306 if ("HOMECARE".equals(codeString)) 8307 return HOMECARE; 8308 if ("HOSPPTNT".equals(codeString)) 8309 return HOSPPTNT; 8310 if ("HOSPVSTR".equals(codeString)) 8311 return HOSPVSTR; 8312 if ("HOUSEHLD".equals(codeString)) 8313 return HOUSEHLD; 8314 if ("INMATE".equals(codeString)) 8315 return INMATE; 8316 if ("INTIMATE".equals(codeString)) 8317 return INTIMATE; 8318 if ("LTRMCARE".equals(codeString)) 8319 return LTRMCARE; 8320 if ("PLACE".equals(codeString)) 8321 return PLACE; 8322 if ("PTNTCARE".equals(codeString)) 8323 return PTNTCARE; 8324 if ("SCHOOL2".equals(codeString)) 8325 return SCHOOL2; 8326 if ("SOCIAL2".equals(codeString)) 8327 return SOCIAL2; 8328 if ("SUBSTNCE".equals(codeString)) 8329 return SUBSTNCE; 8330 if ("TRAVINT".equals(codeString)) 8331 return TRAVINT; 8332 if ("WORK2".equals(codeString)) 8333 return WORK2; 8334 if ("_ActFinancialTransactionCode".equals(codeString)) 8335 return _ACTFINANCIALTRANSACTIONCODE; 8336 if ("CHRG".equals(codeString)) 8337 return CHRG; 8338 if ("REV".equals(codeString)) 8339 return REV; 8340 if ("_ActIncidentCode".equals(codeString)) 8341 return _ACTINCIDENTCODE; 8342 if ("MVA".equals(codeString)) 8343 return MVA; 8344 if ("SCHOOL".equals(codeString)) 8345 return SCHOOL; 8346 if ("SPT".equals(codeString)) 8347 return SPT; 8348 if ("WPA".equals(codeString)) 8349 return WPA; 8350 if ("_ActInformationAccessCode".equals(codeString)) 8351 return _ACTINFORMATIONACCESSCODE; 8352 if ("ACADR".equals(codeString)) 8353 return ACADR; 8354 if ("ACALL".equals(codeString)) 8355 return ACALL; 8356 if ("ACALLG".equals(codeString)) 8357 return ACALLG; 8358 if ("ACCONS".equals(codeString)) 8359 return ACCONS; 8360 if ("ACDEMO".equals(codeString)) 8361 return ACDEMO; 8362 if ("ACDI".equals(codeString)) 8363 return ACDI; 8364 if ("ACIMMUN".equals(codeString)) 8365 return ACIMMUN; 8366 if ("ACLAB".equals(codeString)) 8367 return ACLAB; 8368 if ("ACMED".equals(codeString)) 8369 return ACMED; 8370 if ("ACMEDC".equals(codeString)) 8371 return ACMEDC; 8372 if ("ACMEN".equals(codeString)) 8373 return ACMEN; 8374 if ("ACOBS".equals(codeString)) 8375 return ACOBS; 8376 if ("ACPOLPRG".equals(codeString)) 8377 return ACPOLPRG; 8378 if ("ACPROV".equals(codeString)) 8379 return ACPROV; 8380 if ("ACPSERV".equals(codeString)) 8381 return ACPSERV; 8382 if ("ACSUBSTAB".equals(codeString)) 8383 return ACSUBSTAB; 8384 if ("_ActInformationAccessContextCode".equals(codeString)) 8385 return _ACTINFORMATIONACCESSCONTEXTCODE; 8386 if ("INFAUT".equals(codeString)) 8387 return INFAUT; 8388 if ("INFCON".equals(codeString)) 8389 return INFCON; 8390 if ("INFCRT".equals(codeString)) 8391 return INFCRT; 8392 if ("INFDNG".equals(codeString)) 8393 return INFDNG; 8394 if ("INFEMER".equals(codeString)) 8395 return INFEMER; 8396 if ("INFPWR".equals(codeString)) 8397 return INFPWR; 8398 if ("INFREG".equals(codeString)) 8399 return INFREG; 8400 if ("_ActInformationCategoryCode".equals(codeString)) 8401 return _ACTINFORMATIONCATEGORYCODE; 8402 if ("ALLCAT".equals(codeString)) 8403 return ALLCAT; 8404 if ("ALLGCAT".equals(codeString)) 8405 return ALLGCAT; 8406 if ("ARCAT".equals(codeString)) 8407 return ARCAT; 8408 if ("COBSCAT".equals(codeString)) 8409 return COBSCAT; 8410 if ("DEMOCAT".equals(codeString)) 8411 return DEMOCAT; 8412 if ("DICAT".equals(codeString)) 8413 return DICAT; 8414 if ("IMMUCAT".equals(codeString)) 8415 return IMMUCAT; 8416 if ("LABCAT".equals(codeString)) 8417 return LABCAT; 8418 if ("MEDCCAT".equals(codeString)) 8419 return MEDCCAT; 8420 if ("MENCAT".equals(codeString)) 8421 return MENCAT; 8422 if ("PSVCCAT".equals(codeString)) 8423 return PSVCCAT; 8424 if ("RXCAT".equals(codeString)) 8425 return RXCAT; 8426 if ("_ActInvoiceElementCode".equals(codeString)) 8427 return _ACTINVOICEELEMENTCODE; 8428 if ("_ActInvoiceAdjudicationPaymentCode".equals(codeString)) 8429 return _ACTINVOICEADJUDICATIONPAYMENTCODE; 8430 if ("_ActInvoiceAdjudicationPaymentGroupCode".equals(codeString)) 8431 return _ACTINVOICEADJUDICATIONPAYMENTGROUPCODE; 8432 if ("ALEC".equals(codeString)) 8433 return ALEC; 8434 if ("BONUS".equals(codeString)) 8435 return BONUS; 8436 if ("CFWD".equals(codeString)) 8437 return CFWD; 8438 if ("EDU".equals(codeString)) 8439 return EDU; 8440 if ("EPYMT".equals(codeString)) 8441 return EPYMT; 8442 if ("GARN".equals(codeString)) 8443 return GARN; 8444 if ("INVOICE".equals(codeString)) 8445 return INVOICE; 8446 if ("PINV".equals(codeString)) 8447 return PINV; 8448 if ("PPRD".equals(codeString)) 8449 return PPRD; 8450 if ("PROA".equals(codeString)) 8451 return PROA; 8452 if ("RECOV".equals(codeString)) 8453 return RECOV; 8454 if ("RETRO".equals(codeString)) 8455 return RETRO; 8456 if ("TRAN".equals(codeString)) 8457 return TRAN; 8458 if ("_ActInvoiceAdjudicationPaymentSummaryCode".equals(codeString)) 8459 return _ACTINVOICEADJUDICATIONPAYMENTSUMMARYCODE; 8460 if ("INVTYPE".equals(codeString)) 8461 return INVTYPE; 8462 if ("PAYEE".equals(codeString)) 8463 return PAYEE; 8464 if ("PAYOR".equals(codeString)) 8465 return PAYOR; 8466 if ("SENDAPP".equals(codeString)) 8467 return SENDAPP; 8468 if ("_ActInvoiceDetailCode".equals(codeString)) 8469 return _ACTINVOICEDETAILCODE; 8470 if ("_ActInvoiceDetailClinicalProductCode".equals(codeString)) 8471 return _ACTINVOICEDETAILCLINICALPRODUCTCODE; 8472 if ("UNSPSC".equals(codeString)) 8473 return UNSPSC; 8474 if ("_ActInvoiceDetailDrugProductCode".equals(codeString)) 8475 return _ACTINVOICEDETAILDRUGPRODUCTCODE; 8476 if ("GTIN".equals(codeString)) 8477 return GTIN; 8478 if ("UPC".equals(codeString)) 8479 return UPC; 8480 if ("_ActInvoiceDetailGenericCode".equals(codeString)) 8481 return _ACTINVOICEDETAILGENERICCODE; 8482 if ("_ActInvoiceDetailGenericAdjudicatorCode".equals(codeString)) 8483 return _ACTINVOICEDETAILGENERICADJUDICATORCODE; 8484 if ("COIN".equals(codeString)) 8485 return COIN; 8486 if ("COPAYMENT".equals(codeString)) 8487 return COPAYMENT; 8488 if ("DEDUCTIBLE".equals(codeString)) 8489 return DEDUCTIBLE; 8490 if ("PAY".equals(codeString)) 8491 return PAY; 8492 if ("SPEND".equals(codeString)) 8493 return SPEND; 8494 if ("COINS".equals(codeString)) 8495 return COINS; 8496 if ("_ActInvoiceDetailGenericModifierCode".equals(codeString)) 8497 return _ACTINVOICEDETAILGENERICMODIFIERCODE; 8498 if ("AFTHRS".equals(codeString)) 8499 return AFTHRS; 8500 if ("ISOL".equals(codeString)) 8501 return ISOL; 8502 if ("OOO".equals(codeString)) 8503 return OOO; 8504 if ("_ActInvoiceDetailGenericProviderCode".equals(codeString)) 8505 return _ACTINVOICEDETAILGENERICPROVIDERCODE; 8506 if ("CANCAPT".equals(codeString)) 8507 return CANCAPT; 8508 if ("DSC".equals(codeString)) 8509 return DSC; 8510 if ("ESA".equals(codeString)) 8511 return ESA; 8512 if ("FFSTOP".equals(codeString)) 8513 return FFSTOP; 8514 if ("FNLFEE".equals(codeString)) 8515 return FNLFEE; 8516 if ("FRSTFEE".equals(codeString)) 8517 return FRSTFEE; 8518 if ("MARKUP".equals(codeString)) 8519 return MARKUP; 8520 if ("MISSAPT".equals(codeString)) 8521 return MISSAPT; 8522 if ("PERFEE".equals(codeString)) 8523 return PERFEE; 8524 if ("PERMBNS".equals(codeString)) 8525 return PERMBNS; 8526 if ("RESTOCK".equals(codeString)) 8527 return RESTOCK; 8528 if ("TRAVEL".equals(codeString)) 8529 return TRAVEL; 8530 if ("URGENT".equals(codeString)) 8531 return URGENT; 8532 if ("_ActInvoiceDetailTaxCode".equals(codeString)) 8533 return _ACTINVOICEDETAILTAXCODE; 8534 if ("FST".equals(codeString)) 8535 return FST; 8536 if ("HST".equals(codeString)) 8537 return HST; 8538 if ("PST".equals(codeString)) 8539 return PST; 8540 if ("_ActInvoiceDetailPreferredAccommodationCode".equals(codeString)) 8541 return _ACTINVOICEDETAILPREFERREDACCOMMODATIONCODE; 8542 if ("_ActEncounterAccommodationCode".equals(codeString)) 8543 return _ACTENCOUNTERACCOMMODATIONCODE; 8544 if ("_HL7AccommodationCode".equals(codeString)) 8545 return _HL7ACCOMMODATIONCODE; 8546 if ("I".equals(codeString)) 8547 return I; 8548 if ("P".equals(codeString)) 8549 return P; 8550 if ("S".equals(codeString)) 8551 return S; 8552 if ("SP".equals(codeString)) 8553 return SP; 8554 if ("W".equals(codeString)) 8555 return W; 8556 if ("_ActInvoiceDetailClinicalServiceCode".equals(codeString)) 8557 return _ACTINVOICEDETAILCLINICALSERVICECODE; 8558 if ("_ActInvoiceGroupCode".equals(codeString)) 8559 return _ACTINVOICEGROUPCODE; 8560 if ("_ActInvoiceInterGroupCode".equals(codeString)) 8561 return _ACTINVOICEINTERGROUPCODE; 8562 if ("CPNDDRGING".equals(codeString)) 8563 return CPNDDRGING; 8564 if ("CPNDINDING".equals(codeString)) 8565 return CPNDINDING; 8566 if ("CPNDSUPING".equals(codeString)) 8567 return CPNDSUPING; 8568 if ("DRUGING".equals(codeString)) 8569 return DRUGING; 8570 if ("FRAMEING".equals(codeString)) 8571 return FRAMEING; 8572 if ("LENSING".equals(codeString)) 8573 return LENSING; 8574 if ("PRDING".equals(codeString)) 8575 return PRDING; 8576 if ("_ActInvoiceRootGroupCode".equals(codeString)) 8577 return _ACTINVOICEROOTGROUPCODE; 8578 if ("CPINV".equals(codeString)) 8579 return CPINV; 8580 if ("CSINV".equals(codeString)) 8581 return CSINV; 8582 if ("CSPINV".equals(codeString)) 8583 return CSPINV; 8584 if ("FININV".equals(codeString)) 8585 return FININV; 8586 if ("OHSINV".equals(codeString)) 8587 return OHSINV; 8588 if ("PAINV".equals(codeString)) 8589 return PAINV; 8590 if ("RXCINV".equals(codeString)) 8591 return RXCINV; 8592 if ("RXDINV".equals(codeString)) 8593 return RXDINV; 8594 if ("SBFINV".equals(codeString)) 8595 return SBFINV; 8596 if ("VRXINV".equals(codeString)) 8597 return VRXINV; 8598 if ("_ActInvoiceElementSummaryCode".equals(codeString)) 8599 return _ACTINVOICEELEMENTSUMMARYCODE; 8600 if ("_InvoiceElementAdjudicated".equals(codeString)) 8601 return _INVOICEELEMENTADJUDICATED; 8602 if ("ADNFPPELAT".equals(codeString)) 8603 return ADNFPPELAT; 8604 if ("ADNFPPELCT".equals(codeString)) 8605 return ADNFPPELCT; 8606 if ("ADNFPPMNAT".equals(codeString)) 8607 return ADNFPPMNAT; 8608 if ("ADNFPPMNCT".equals(codeString)) 8609 return ADNFPPMNCT; 8610 if ("ADNFSPELAT".equals(codeString)) 8611 return ADNFSPELAT; 8612 if ("ADNFSPELCT".equals(codeString)) 8613 return ADNFSPELCT; 8614 if ("ADNFSPMNAT".equals(codeString)) 8615 return ADNFSPMNAT; 8616 if ("ADNFSPMNCT".equals(codeString)) 8617 return ADNFSPMNCT; 8618 if ("ADNPPPELAT".equals(codeString)) 8619 return ADNPPPELAT; 8620 if ("ADNPPPELCT".equals(codeString)) 8621 return ADNPPPELCT; 8622 if ("ADNPPPMNAT".equals(codeString)) 8623 return ADNPPPMNAT; 8624 if ("ADNPPPMNCT".equals(codeString)) 8625 return ADNPPPMNCT; 8626 if ("ADNPSPELAT".equals(codeString)) 8627 return ADNPSPELAT; 8628 if ("ADNPSPELCT".equals(codeString)) 8629 return ADNPSPELCT; 8630 if ("ADNPSPMNAT".equals(codeString)) 8631 return ADNPSPMNAT; 8632 if ("ADNPSPMNCT".equals(codeString)) 8633 return ADNPSPMNCT; 8634 if ("ADPPPPELAT".equals(codeString)) 8635 return ADPPPPELAT; 8636 if ("ADPPPPELCT".equals(codeString)) 8637 return ADPPPPELCT; 8638 if ("ADPPPPMNAT".equals(codeString)) 8639 return ADPPPPMNAT; 8640 if ("ADPPPPMNCT".equals(codeString)) 8641 return ADPPPPMNCT; 8642 if ("ADPPSPELAT".equals(codeString)) 8643 return ADPPSPELAT; 8644 if ("ADPPSPELCT".equals(codeString)) 8645 return ADPPSPELCT; 8646 if ("ADPPSPMNAT".equals(codeString)) 8647 return ADPPSPMNAT; 8648 if ("ADPPSPMNCT".equals(codeString)) 8649 return ADPPSPMNCT; 8650 if ("ADRFPPELAT".equals(codeString)) 8651 return ADRFPPELAT; 8652 if ("ADRFPPELCT".equals(codeString)) 8653 return ADRFPPELCT; 8654 if ("ADRFPPMNAT".equals(codeString)) 8655 return ADRFPPMNAT; 8656 if ("ADRFPPMNCT".equals(codeString)) 8657 return ADRFPPMNCT; 8658 if ("ADRFSPELAT".equals(codeString)) 8659 return ADRFSPELAT; 8660 if ("ADRFSPELCT".equals(codeString)) 8661 return ADRFSPELCT; 8662 if ("ADRFSPMNAT".equals(codeString)) 8663 return ADRFSPMNAT; 8664 if ("ADRFSPMNCT".equals(codeString)) 8665 return ADRFSPMNCT; 8666 if ("_InvoiceElementPaid".equals(codeString)) 8667 return _INVOICEELEMENTPAID; 8668 if ("PDNFPPELAT".equals(codeString)) 8669 return PDNFPPELAT; 8670 if ("PDNFPPELCT".equals(codeString)) 8671 return PDNFPPELCT; 8672 if ("PDNFPPMNAT".equals(codeString)) 8673 return PDNFPPMNAT; 8674 if ("PDNFPPMNCT".equals(codeString)) 8675 return PDNFPPMNCT; 8676 if ("PDNFSPELAT".equals(codeString)) 8677 return PDNFSPELAT; 8678 if ("PDNFSPELCT".equals(codeString)) 8679 return PDNFSPELCT; 8680 if ("PDNFSPMNAT".equals(codeString)) 8681 return PDNFSPMNAT; 8682 if ("PDNFSPMNCT".equals(codeString)) 8683 return PDNFSPMNCT; 8684 if ("PDNPPPELAT".equals(codeString)) 8685 return PDNPPPELAT; 8686 if ("PDNPPPELCT".equals(codeString)) 8687 return PDNPPPELCT; 8688 if ("PDNPPPMNAT".equals(codeString)) 8689 return PDNPPPMNAT; 8690 if ("PDNPPPMNCT".equals(codeString)) 8691 return PDNPPPMNCT; 8692 if ("PDNPSPELAT".equals(codeString)) 8693 return PDNPSPELAT; 8694 if ("PDNPSPELCT".equals(codeString)) 8695 return PDNPSPELCT; 8696 if ("PDNPSPMNAT".equals(codeString)) 8697 return PDNPSPMNAT; 8698 if ("PDNPSPMNCT".equals(codeString)) 8699 return PDNPSPMNCT; 8700 if ("PDPPPPELAT".equals(codeString)) 8701 return PDPPPPELAT; 8702 if ("PDPPPPELCT".equals(codeString)) 8703 return PDPPPPELCT; 8704 if ("PDPPPPMNAT".equals(codeString)) 8705 return PDPPPPMNAT; 8706 if ("PDPPPPMNCT".equals(codeString)) 8707 return PDPPPPMNCT; 8708 if ("PDPPSPELAT".equals(codeString)) 8709 return PDPPSPELAT; 8710 if ("PDPPSPELCT".equals(codeString)) 8711 return PDPPSPELCT; 8712 if ("PDPPSPMNAT".equals(codeString)) 8713 return PDPPSPMNAT; 8714 if ("PDPPSPMNCT".equals(codeString)) 8715 return PDPPSPMNCT; 8716 if ("_InvoiceElementSubmitted".equals(codeString)) 8717 return _INVOICEELEMENTSUBMITTED; 8718 if ("SBBLELAT".equals(codeString)) 8719 return SBBLELAT; 8720 if ("SBBLELCT".equals(codeString)) 8721 return SBBLELCT; 8722 if ("SBNFELAT".equals(codeString)) 8723 return SBNFELAT; 8724 if ("SBNFELCT".equals(codeString)) 8725 return SBNFELCT; 8726 if ("SBPDELAT".equals(codeString)) 8727 return SBPDELAT; 8728 if ("SBPDELCT".equals(codeString)) 8729 return SBPDELCT; 8730 if ("_ActInvoiceOverrideCode".equals(codeString)) 8731 return _ACTINVOICEOVERRIDECODE; 8732 if ("COVGE".equals(codeString)) 8733 return COVGE; 8734 if ("EFORM".equals(codeString)) 8735 return EFORM; 8736 if ("FAX".equals(codeString)) 8737 return FAX; 8738 if ("GFTH".equals(codeString)) 8739 return GFTH; 8740 if ("LATE".equals(codeString)) 8741 return LATE; 8742 if ("MANUAL".equals(codeString)) 8743 return MANUAL; 8744 if ("OOJ".equals(codeString)) 8745 return OOJ; 8746 if ("ORTHO".equals(codeString)) 8747 return ORTHO; 8748 if ("PAPER".equals(codeString)) 8749 return PAPER; 8750 if ("PIE".equals(codeString)) 8751 return PIE; 8752 if ("PYRDELAY".equals(codeString)) 8753 return PYRDELAY; 8754 if ("REFNR".equals(codeString)) 8755 return REFNR; 8756 if ("REPSERV".equals(codeString)) 8757 return REPSERV; 8758 if ("UNRELAT".equals(codeString)) 8759 return UNRELAT; 8760 if ("VERBAUTH".equals(codeString)) 8761 return VERBAUTH; 8762 if ("_ActListCode".equals(codeString)) 8763 return _ACTLISTCODE; 8764 if ("_ActObservationList".equals(codeString)) 8765 return _ACTOBSERVATIONLIST; 8766 if ("CARELIST".equals(codeString)) 8767 return CARELIST; 8768 if ("CONDLIST".equals(codeString)) 8769 return CONDLIST; 8770 if ("INTOLIST".equals(codeString)) 8771 return INTOLIST; 8772 if ("PROBLIST".equals(codeString)) 8773 return PROBLIST; 8774 if ("RISKLIST".equals(codeString)) 8775 return RISKLIST; 8776 if ("GOALLIST".equals(codeString)) 8777 return GOALLIST; 8778 if ("_ActTherapyDurationWorkingListCode".equals(codeString)) 8779 return _ACTTHERAPYDURATIONWORKINGLISTCODE; 8780 if ("_ActMedicationTherapyDurationWorkingListCode".equals(codeString)) 8781 return _ACTMEDICATIONTHERAPYDURATIONWORKINGLISTCODE; 8782 if ("ACU".equals(codeString)) 8783 return ACU; 8784 if ("CHRON".equals(codeString)) 8785 return CHRON; 8786 if ("ONET".equals(codeString)) 8787 return ONET; 8788 if ("PRN".equals(codeString)) 8789 return PRN; 8790 if ("MEDLIST".equals(codeString)) 8791 return MEDLIST; 8792 if ("CURMEDLIST".equals(codeString)) 8793 return CURMEDLIST; 8794 if ("DISCMEDLIST".equals(codeString)) 8795 return DISCMEDLIST; 8796 if ("HISTMEDLIST".equals(codeString)) 8797 return HISTMEDLIST; 8798 if ("_ActMonitoringProtocolCode".equals(codeString)) 8799 return _ACTMONITORINGPROTOCOLCODE; 8800 if ("CTLSUB".equals(codeString)) 8801 return CTLSUB; 8802 if ("INV".equals(codeString)) 8803 return INV; 8804 if ("LU".equals(codeString)) 8805 return LU; 8806 if ("OTC".equals(codeString)) 8807 return OTC; 8808 if ("RX".equals(codeString)) 8809 return RX; 8810 if ("SA".equals(codeString)) 8811 return SA; 8812 if ("SAC".equals(codeString)) 8813 return SAC; 8814 if ("_ActNonObservationIndicationCode".equals(codeString)) 8815 return _ACTNONOBSERVATIONINDICATIONCODE; 8816 if ("IND01".equals(codeString)) 8817 return IND01; 8818 if ("IND02".equals(codeString)) 8819 return IND02; 8820 if ("IND03".equals(codeString)) 8821 return IND03; 8822 if ("IND04".equals(codeString)) 8823 return IND04; 8824 if ("IND05".equals(codeString)) 8825 return IND05; 8826 if ("_ActObservationVerificationType".equals(codeString)) 8827 return _ACTOBSERVATIONVERIFICATIONTYPE; 8828 if ("VFPAPER".equals(codeString)) 8829 return VFPAPER; 8830 if ("_ActPaymentCode".equals(codeString)) 8831 return _ACTPAYMENTCODE; 8832 if ("ACH".equals(codeString)) 8833 return ACH; 8834 if ("CHK".equals(codeString)) 8835 return CHK; 8836 if ("DDP".equals(codeString)) 8837 return DDP; 8838 if ("NON".equals(codeString)) 8839 return NON; 8840 if ("_ActPharmacySupplyType".equals(codeString)) 8841 return _ACTPHARMACYSUPPLYTYPE; 8842 if ("DF".equals(codeString)) 8843 return DF; 8844 if ("EM".equals(codeString)) 8845 return EM; 8846 if ("SO".equals(codeString)) 8847 return SO; 8848 if ("FF".equals(codeString)) 8849 return FF; 8850 if ("FFC".equals(codeString)) 8851 return FFC; 8852 if ("FFP".equals(codeString)) 8853 return FFP; 8854 if ("FFSS".equals(codeString)) 8855 return FFSS; 8856 if ("TF".equals(codeString)) 8857 return TF; 8858 if ("FS".equals(codeString)) 8859 return FS; 8860 if ("MS".equals(codeString)) 8861 return MS; 8862 if ("RF".equals(codeString)) 8863 return RF; 8864 if ("UD".equals(codeString)) 8865 return UD; 8866 if ("RFC".equals(codeString)) 8867 return RFC; 8868 if ("RFCS".equals(codeString)) 8869 return RFCS; 8870 if ("RFF".equals(codeString)) 8871 return RFF; 8872 if ("RFFS".equals(codeString)) 8873 return RFFS; 8874 if ("RFP".equals(codeString)) 8875 return RFP; 8876 if ("RFPS".equals(codeString)) 8877 return RFPS; 8878 if ("RFS".equals(codeString)) 8879 return RFS; 8880 if ("TB".equals(codeString)) 8881 return TB; 8882 if ("TBS".equals(codeString)) 8883 return TBS; 8884 if ("UDE".equals(codeString)) 8885 return UDE; 8886 if ("_ActPolicyType".equals(codeString)) 8887 return _ACTPOLICYTYPE; 8888 if ("_ActPrivacyPolicy".equals(codeString)) 8889 return _ACTPRIVACYPOLICY; 8890 if ("_ActConsentDirective".equals(codeString)) 8891 return _ACTCONSENTDIRECTIVE; 8892 if ("EMRGONLY".equals(codeString)) 8893 return EMRGONLY; 8894 if ("GRANTORCHOICE".equals(codeString)) 8895 return GRANTORCHOICE; 8896 if ("IMPLIED".equals(codeString)) 8897 return IMPLIED; 8898 if ("IMPLIEDD".equals(codeString)) 8899 return IMPLIEDD; 8900 if ("NOCONSENT".equals(codeString)) 8901 return NOCONSENT; 8902 if ("NOPP".equals(codeString)) 8903 return NOPP; 8904 if ("OPTIN".equals(codeString)) 8905 return OPTIN; 8906 if ("OPTINR".equals(codeString)) 8907 return OPTINR; 8908 if ("OPTOUT".equals(codeString)) 8909 return OPTOUT; 8910 if ("OPTOUTE".equals(codeString)) 8911 return OPTOUTE; 8912 if ("_ActPrivacyLaw".equals(codeString)) 8913 return _ACTPRIVACYLAW; 8914 if ("_ActUSPrivacyLaw".equals(codeString)) 8915 return _ACTUSPRIVACYLAW; 8916 if ("42CFRPart2".equals(codeString)) 8917 return _42CFRPART2; 8918 if ("CommonRule".equals(codeString)) 8919 return COMMONRULE; 8920 if ("HIPAANOPP".equals(codeString)) 8921 return HIPAANOPP; 8922 if ("HIPAAPsyNotes".equals(codeString)) 8923 return HIPAAPSYNOTES; 8924 if ("HIPAASelfPay".equals(codeString)) 8925 return HIPAASELFPAY; 8926 if ("Title38Section7332".equals(codeString)) 8927 return TITLE38SECTION7332; 8928 if ("_InformationSensitivityPolicy".equals(codeString)) 8929 return _INFORMATIONSENSITIVITYPOLICY; 8930 if ("_ActInformationSensitivityPolicy".equals(codeString)) 8931 return _ACTINFORMATIONSENSITIVITYPOLICY; 8932 if ("ETH".equals(codeString)) 8933 return ETH; 8934 if ("GDIS".equals(codeString)) 8935 return GDIS; 8936 if ("HIV".equals(codeString)) 8937 return HIV; 8938 if ("MST".equals(codeString)) 8939 return MST; 8940 if ("SCA".equals(codeString)) 8941 return SCA; 8942 if ("SDV".equals(codeString)) 8943 return SDV; 8944 if ("SEX".equals(codeString)) 8945 return SEX; 8946 if ("SPI".equals(codeString)) 8947 return SPI; 8948 if ("BH".equals(codeString)) 8949 return BH; 8950 if ("COGN".equals(codeString)) 8951 return COGN; 8952 if ("DVD".equals(codeString)) 8953 return DVD; 8954 if ("EMOTDIS".equals(codeString)) 8955 return EMOTDIS; 8956 if ("MH".equals(codeString)) 8957 return MH; 8958 if ("PSY".equals(codeString)) 8959 return PSY; 8960 if ("PSYTHPN".equals(codeString)) 8961 return PSYTHPN; 8962 if ("SUD".equals(codeString)) 8963 return SUD; 8964 if ("ETHUD".equals(codeString)) 8965 return ETHUD; 8966 if ("OPIOIDUD".equals(codeString)) 8967 return OPIOIDUD; 8968 if ("STD".equals(codeString)) 8969 return STD; 8970 if ("TBOO".equals(codeString)) 8971 return TBOO; 8972 if ("VIO".equals(codeString)) 8973 return VIO; 8974 if ("SICKLE".equals(codeString)) 8975 return SICKLE; 8976 if ("_EntitySensitivityPolicyType".equals(codeString)) 8977 return _ENTITYSENSITIVITYPOLICYTYPE; 8978 if ("DEMO".equals(codeString)) 8979 return DEMO; 8980 if ("DOB".equals(codeString)) 8981 return DOB; 8982 if ("GENDER".equals(codeString)) 8983 return GENDER; 8984 if ("LIVARG".equals(codeString)) 8985 return LIVARG; 8986 if ("MARST".equals(codeString)) 8987 return MARST; 8988 if ("RACE".equals(codeString)) 8989 return RACE; 8990 if ("REL".equals(codeString)) 8991 return REL; 8992 if ("_RoleInformationSensitivityPolicy".equals(codeString)) 8993 return _ROLEINFORMATIONSENSITIVITYPOLICY; 8994 if ("B".equals(codeString)) 8995 return B; 8996 if ("EMPL".equals(codeString)) 8997 return EMPL; 8998 if ("LOCIS".equals(codeString)) 8999 return LOCIS; 9000 if ("SSP".equals(codeString)) 9001 return SSP; 9002 if ("ADOL".equals(codeString)) 9003 return ADOL; 9004 if ("CEL".equals(codeString)) 9005 return CEL; 9006 if ("DIA".equals(codeString)) 9007 return DIA; 9008 if ("DRGIS".equals(codeString)) 9009 return DRGIS; 9010 if ("EMP".equals(codeString)) 9011 return EMP; 9012 if ("PDS".equals(codeString)) 9013 return PDS; 9014 if ("PHY".equals(codeString)) 9015 return PHY; 9016 if ("PRS".equals(codeString)) 9017 return PRS; 9018 if ("COMPT".equals(codeString)) 9019 return COMPT; 9020 if ("ACOCOMPT".equals(codeString)) 9021 return ACOCOMPT; 9022 if ("CTCOMPT".equals(codeString)) 9023 return CTCOMPT; 9024 if ("FMCOMPT".equals(codeString)) 9025 return FMCOMPT; 9026 if ("HRCOMPT".equals(codeString)) 9027 return HRCOMPT; 9028 if ("LRCOMPT".equals(codeString)) 9029 return LRCOMPT; 9030 if ("PACOMPT".equals(codeString)) 9031 return PACOMPT; 9032 if ("RESCOMPT".equals(codeString)) 9033 return RESCOMPT; 9034 if ("RMGTCOMPT".equals(codeString)) 9035 return RMGTCOMPT; 9036 if ("ActTrustPolicyType".equals(codeString)) 9037 return ACTTRUSTPOLICYTYPE; 9038 if ("TRSTACCRD".equals(codeString)) 9039 return TRSTACCRD; 9040 if ("TRSTAGRE".equals(codeString)) 9041 return TRSTAGRE; 9042 if ("TRSTASSUR".equals(codeString)) 9043 return TRSTASSUR; 9044 if ("TRSTCERT".equals(codeString)) 9045 return TRSTCERT; 9046 if ("TRSTFWK".equals(codeString)) 9047 return TRSTFWK; 9048 if ("TRSTMEC".equals(codeString)) 9049 return TRSTMEC; 9050 if ("COVPOL".equals(codeString)) 9051 return COVPOL; 9052 if ("SecurityPolicy".equals(codeString)) 9053 return SECURITYPOLICY; 9054 if ("AUTHPOL".equals(codeString)) 9055 return AUTHPOL; 9056 if ("ACCESSCONSCHEME".equals(codeString)) 9057 return ACCESSCONSCHEME; 9058 if ("DELEPOL".equals(codeString)) 9059 return DELEPOL; 9060 if ("ObligationPolicy".equals(codeString)) 9061 return OBLIGATIONPOLICY; 9062 if ("ANONY".equals(codeString)) 9063 return ANONY; 9064 if ("AOD".equals(codeString)) 9065 return AOD; 9066 if ("AUDIT".equals(codeString)) 9067 return AUDIT; 9068 if ("AUDTR".equals(codeString)) 9069 return AUDTR; 9070 if ("CPLYCC".equals(codeString)) 9071 return CPLYCC; 9072 if ("CPLYCD".equals(codeString)) 9073 return CPLYCD; 9074 if ("CPLYJPP".equals(codeString)) 9075 return CPLYJPP; 9076 if ("CPLYOPP".equals(codeString)) 9077 return CPLYOPP; 9078 if ("CPLYOSP".equals(codeString)) 9079 return CPLYOSP; 9080 if ("CPLYPOL".equals(codeString)) 9081 return CPLYPOL; 9082 if ("DECLASSIFYLABEL".equals(codeString)) 9083 return DECLASSIFYLABEL; 9084 if ("DEID".equals(codeString)) 9085 return DEID; 9086 if ("DELAU".equals(codeString)) 9087 return DELAU; 9088 if ("DOWNGRDLABEL".equals(codeString)) 9089 return DOWNGRDLABEL; 9090 if ("DRIVLABEL".equals(codeString)) 9091 return DRIVLABEL; 9092 if ("ENCRYPT".equals(codeString)) 9093 return ENCRYPT; 9094 if ("ENCRYPTR".equals(codeString)) 9095 return ENCRYPTR; 9096 if ("ENCRYPTT".equals(codeString)) 9097 return ENCRYPTT; 9098 if ("ENCRYPTU".equals(codeString)) 9099 return ENCRYPTU; 9100 if ("HUAPRV".equals(codeString)) 9101 return HUAPRV; 9102 if ("LABEL".equals(codeString)) 9103 return LABEL; 9104 if ("MASK".equals(codeString)) 9105 return MASK; 9106 if ("MINEC".equals(codeString)) 9107 return MINEC; 9108 if ("PERSISTLABEL".equals(codeString)) 9109 return PERSISTLABEL; 9110 if ("PRIVMARK".equals(codeString)) 9111 return PRIVMARK; 9112 if ("PSEUD".equals(codeString)) 9113 return PSEUD; 9114 if ("REDACT".equals(codeString)) 9115 return REDACT; 9116 if ("UPGRDLABEL".equals(codeString)) 9117 return UPGRDLABEL; 9118 if ("RefrainPolicy".equals(codeString)) 9119 return REFRAINPOLICY; 9120 if ("NOAUTH".equals(codeString)) 9121 return NOAUTH; 9122 if ("NOCOLLECT".equals(codeString)) 9123 return NOCOLLECT; 9124 if ("NODSCLCD".equals(codeString)) 9125 return NODSCLCD; 9126 if ("NODSCLCDS".equals(codeString)) 9127 return NODSCLCDS; 9128 if ("NOINTEGRATE".equals(codeString)) 9129 return NOINTEGRATE; 9130 if ("NOLIST".equals(codeString)) 9131 return NOLIST; 9132 if ("NOMOU".equals(codeString)) 9133 return NOMOU; 9134 if ("NOORGPOL".equals(codeString)) 9135 return NOORGPOL; 9136 if ("NOPAT".equals(codeString)) 9137 return NOPAT; 9138 if ("NOPERSISTP".equals(codeString)) 9139 return NOPERSISTP; 9140 if ("NORDSCLCD".equals(codeString)) 9141 return NORDSCLCD; 9142 if ("NORDSCLCDS".equals(codeString)) 9143 return NORDSCLCDS; 9144 if ("NORDSCLW".equals(codeString)) 9145 return NORDSCLW; 9146 if ("NORELINK".equals(codeString)) 9147 return NORELINK; 9148 if ("NOREUSE".equals(codeString)) 9149 return NOREUSE; 9150 if ("NOVIP".equals(codeString)) 9151 return NOVIP; 9152 if ("ORCON".equals(codeString)) 9153 return ORCON; 9154 if ("_ActProductAcquisitionCode".equals(codeString)) 9155 return _ACTPRODUCTACQUISITIONCODE; 9156 if ("LOAN".equals(codeString)) 9157 return LOAN; 9158 if ("RENT".equals(codeString)) 9159 return RENT; 9160 if ("TRANSFER".equals(codeString)) 9161 return TRANSFER; 9162 if ("SALE".equals(codeString)) 9163 return SALE; 9164 if ("_ActSpecimenTransportCode".equals(codeString)) 9165 return _ACTSPECIMENTRANSPORTCODE; 9166 if ("SREC".equals(codeString)) 9167 return SREC; 9168 if ("SSTOR".equals(codeString)) 9169 return SSTOR; 9170 if ("STRAN".equals(codeString)) 9171 return STRAN; 9172 if ("_ActSpecimenTreatmentCode".equals(codeString)) 9173 return _ACTSPECIMENTREATMENTCODE; 9174 if ("ACID".equals(codeString)) 9175 return ACID; 9176 if ("ALK".equals(codeString)) 9177 return ALK; 9178 if ("DEFB".equals(codeString)) 9179 return DEFB; 9180 if ("FILT".equals(codeString)) 9181 return FILT; 9182 if ("LDLP".equals(codeString)) 9183 return LDLP; 9184 if ("NEUT".equals(codeString)) 9185 return NEUT; 9186 if ("RECA".equals(codeString)) 9187 return RECA; 9188 if ("UFIL".equals(codeString)) 9189 return UFIL; 9190 if ("_ActSubstanceAdministrationCode".equals(codeString)) 9191 return _ACTSUBSTANCEADMINISTRATIONCODE; 9192 if ("DRUG".equals(codeString)) 9193 return DRUG; 9194 if ("FD".equals(codeString)) 9195 return FD; 9196 if ("IMMUNIZ".equals(codeString)) 9197 return IMMUNIZ; 9198 if ("BOOSTER".equals(codeString)) 9199 return BOOSTER; 9200 if ("INITIMMUNIZ".equals(codeString)) 9201 return INITIMMUNIZ; 9202 if ("_ActTaskCode".equals(codeString)) 9203 return _ACTTASKCODE; 9204 if ("OE".equals(codeString)) 9205 return OE; 9206 if ("LABOE".equals(codeString)) 9207 return LABOE; 9208 if ("MEDOE".equals(codeString)) 9209 return MEDOE; 9210 if ("PATDOC".equals(codeString)) 9211 return PATDOC; 9212 if ("ALLERLREV".equals(codeString)) 9213 return ALLERLREV; 9214 if ("CLINNOTEE".equals(codeString)) 9215 return CLINNOTEE; 9216 if ("DIAGLISTE".equals(codeString)) 9217 return DIAGLISTE; 9218 if ("DISCHINSTE".equals(codeString)) 9219 return DISCHINSTE; 9220 if ("DISCHSUME".equals(codeString)) 9221 return DISCHSUME; 9222 if ("PATEDUE".equals(codeString)) 9223 return PATEDUE; 9224 if ("PATREPE".equals(codeString)) 9225 return PATREPE; 9226 if ("PROBLISTE".equals(codeString)) 9227 return PROBLISTE; 9228 if ("RADREPE".equals(codeString)) 9229 return RADREPE; 9230 if ("IMMLREV".equals(codeString)) 9231 return IMMLREV; 9232 if ("REMLREV".equals(codeString)) 9233 return REMLREV; 9234 if ("WELLREMLREV".equals(codeString)) 9235 return WELLREMLREV; 9236 if ("PATINFO".equals(codeString)) 9237 return PATINFO; 9238 if ("ALLERLE".equals(codeString)) 9239 return ALLERLE; 9240 if ("CDSREV".equals(codeString)) 9241 return CDSREV; 9242 if ("CLINNOTEREV".equals(codeString)) 9243 return CLINNOTEREV; 9244 if ("DISCHSUMREV".equals(codeString)) 9245 return DISCHSUMREV; 9246 if ("DIAGLISTREV".equals(codeString)) 9247 return DIAGLISTREV; 9248 if ("IMMLE".equals(codeString)) 9249 return IMMLE; 9250 if ("LABRREV".equals(codeString)) 9251 return LABRREV; 9252 if ("MICRORREV".equals(codeString)) 9253 return MICRORREV; 9254 if ("MICROORGRREV".equals(codeString)) 9255 return MICROORGRREV; 9256 if ("MICROSENSRREV".equals(codeString)) 9257 return MICROSENSRREV; 9258 if ("MLREV".equals(codeString)) 9259 return MLREV; 9260 if ("MARWLREV".equals(codeString)) 9261 return MARWLREV; 9262 if ("OREV".equals(codeString)) 9263 return OREV; 9264 if ("PATREPREV".equals(codeString)) 9265 return PATREPREV; 9266 if ("PROBLISTREV".equals(codeString)) 9267 return PROBLISTREV; 9268 if ("RADREPREV".equals(codeString)) 9269 return RADREPREV; 9270 if ("REMLE".equals(codeString)) 9271 return REMLE; 9272 if ("WELLREMLE".equals(codeString)) 9273 return WELLREMLE; 9274 if ("RISKASSESS".equals(codeString)) 9275 return RISKASSESS; 9276 if ("FALLRISK".equals(codeString)) 9277 return FALLRISK; 9278 if ("_ActTransportationModeCode".equals(codeString)) 9279 return _ACTTRANSPORTATIONMODECODE; 9280 if ("_ActPatientTransportationModeCode".equals(codeString)) 9281 return _ACTPATIENTTRANSPORTATIONMODECODE; 9282 if ("AFOOT".equals(codeString)) 9283 return AFOOT; 9284 if ("AMBT".equals(codeString)) 9285 return AMBT; 9286 if ("AMBAIR".equals(codeString)) 9287 return AMBAIR; 9288 if ("AMBGRND".equals(codeString)) 9289 return AMBGRND; 9290 if ("AMBHELO".equals(codeString)) 9291 return AMBHELO; 9292 if ("LAWENF".equals(codeString)) 9293 return LAWENF; 9294 if ("PRVTRN".equals(codeString)) 9295 return PRVTRN; 9296 if ("PUBTRN".equals(codeString)) 9297 return PUBTRN; 9298 if ("_ObservationType".equals(codeString)) 9299 return _OBSERVATIONTYPE; 9300 if ("_ActSpecObsCode".equals(codeString)) 9301 return _ACTSPECOBSCODE; 9302 if ("ARTBLD".equals(codeString)) 9303 return ARTBLD; 9304 if ("DILUTION".equals(codeString)) 9305 return DILUTION; 9306 if ("AUTO-HIGH".equals(codeString)) 9307 return AUTOHIGH; 9308 if ("AUTO-LOW".equals(codeString)) 9309 return AUTOLOW; 9310 if ("PRE".equals(codeString)) 9311 return PRE; 9312 if ("RERUN".equals(codeString)) 9313 return RERUN; 9314 if ("EVNFCTS".equals(codeString)) 9315 return EVNFCTS; 9316 if ("INTFR".equals(codeString)) 9317 return INTFR; 9318 if ("FIBRIN".equals(codeString)) 9319 return FIBRIN; 9320 if ("HEMOLYSIS".equals(codeString)) 9321 return HEMOLYSIS; 9322 if ("ICTERUS".equals(codeString)) 9323 return ICTERUS; 9324 if ("LIPEMIA".equals(codeString)) 9325 return LIPEMIA; 9326 if ("VOLUME".equals(codeString)) 9327 return VOLUME; 9328 if ("AVAILABLE".equals(codeString)) 9329 return AVAILABLE; 9330 if ("CONSUMPTION".equals(codeString)) 9331 return CONSUMPTION; 9332 if ("CURRENT".equals(codeString)) 9333 return CURRENT; 9334 if ("INITIAL".equals(codeString)) 9335 return INITIAL; 9336 if ("_AnnotationType".equals(codeString)) 9337 return _ANNOTATIONTYPE; 9338 if ("_ActPatientAnnotationType".equals(codeString)) 9339 return _ACTPATIENTANNOTATIONTYPE; 9340 if ("ANNDI".equals(codeString)) 9341 return ANNDI; 9342 if ("ANNGEN".equals(codeString)) 9343 return ANNGEN; 9344 if ("ANNIMM".equals(codeString)) 9345 return ANNIMM; 9346 if ("ANNLAB".equals(codeString)) 9347 return ANNLAB; 9348 if ("ANNMED".equals(codeString)) 9349 return ANNMED; 9350 if ("_GeneticObservationType".equals(codeString)) 9351 return _GENETICOBSERVATIONTYPE; 9352 if ("GENE".equals(codeString)) 9353 return GENE; 9354 if ("_ImmunizationObservationType".equals(codeString)) 9355 return _IMMUNIZATIONOBSERVATIONTYPE; 9356 if ("OBSANTC".equals(codeString)) 9357 return OBSANTC; 9358 if ("OBSANTV".equals(codeString)) 9359 return OBSANTV; 9360 if ("_IndividualCaseSafetyReportType".equals(codeString)) 9361 return _INDIVIDUALCASESAFETYREPORTTYPE; 9362 if ("PAT_ADV_EVNT".equals(codeString)) 9363 return PATADVEVNT; 9364 if ("VAC_PROBLEM".equals(codeString)) 9365 return VACPROBLEM; 9366 if ("_LOINCObservationActContextAgeType".equals(codeString)) 9367 return _LOINCOBSERVATIONACTCONTEXTAGETYPE; 9368 if ("21611-9".equals(codeString)) 9369 return _216119; 9370 if ("21612-7".equals(codeString)) 9371 return _216127; 9372 if ("29553-5".equals(codeString)) 9373 return _295535; 9374 if ("30525-0".equals(codeString)) 9375 return _305250; 9376 if ("30972-4".equals(codeString)) 9377 return _309724; 9378 if ("_MedicationObservationType".equals(codeString)) 9379 return _MEDICATIONOBSERVATIONTYPE; 9380 if ("REP_HALF_LIFE".equals(codeString)) 9381 return REPHALFLIFE; 9382 if ("SPLCOATING".equals(codeString)) 9383 return SPLCOATING; 9384 if ("SPLCOLOR".equals(codeString)) 9385 return SPLCOLOR; 9386 if ("SPLIMAGE".equals(codeString)) 9387 return SPLIMAGE; 9388 if ("SPLIMPRINT".equals(codeString)) 9389 return SPLIMPRINT; 9390 if ("SPLSCORING".equals(codeString)) 9391 return SPLSCORING; 9392 if ("SPLSHAPE".equals(codeString)) 9393 return SPLSHAPE; 9394 if ("SPLSIZE".equals(codeString)) 9395 return SPLSIZE; 9396 if ("SPLSYMBOL".equals(codeString)) 9397 return SPLSYMBOL; 9398 if ("_ObservationIssueTriggerCodedObservationType".equals(codeString)) 9399 return _OBSERVATIONISSUETRIGGERCODEDOBSERVATIONTYPE; 9400 if ("_CaseTransmissionMode".equals(codeString)) 9401 return _CASETRANSMISSIONMODE; 9402 if ("AIRTRNS".equals(codeString)) 9403 return AIRTRNS; 9404 if ("ANANTRNS".equals(codeString)) 9405 return ANANTRNS; 9406 if ("ANHUMTRNS".equals(codeString)) 9407 return ANHUMTRNS; 9408 if ("BDYFLDTRNS".equals(codeString)) 9409 return BDYFLDTRNS; 9410 if ("BLDTRNS".equals(codeString)) 9411 return BLDTRNS; 9412 if ("DERMTRNS".equals(codeString)) 9413 return DERMTRNS; 9414 if ("ENVTRNS".equals(codeString)) 9415 return ENVTRNS; 9416 if ("FECTRNS".equals(codeString)) 9417 return FECTRNS; 9418 if ("FOMTRNS".equals(codeString)) 9419 return FOMTRNS; 9420 if ("FOODTRNS".equals(codeString)) 9421 return FOODTRNS; 9422 if ("HUMHUMTRNS".equals(codeString)) 9423 return HUMHUMTRNS; 9424 if ("INDTRNS".equals(codeString)) 9425 return INDTRNS; 9426 if ("LACTTRNS".equals(codeString)) 9427 return LACTTRNS; 9428 if ("NOSTRNS".equals(codeString)) 9429 return NOSTRNS; 9430 if ("PARTRNS".equals(codeString)) 9431 return PARTRNS; 9432 if ("PLACTRNS".equals(codeString)) 9433 return PLACTRNS; 9434 if ("SEXTRNS".equals(codeString)) 9435 return SEXTRNS; 9436 if ("TRNSFTRNS".equals(codeString)) 9437 return TRNSFTRNS; 9438 if ("VECTRNS".equals(codeString)) 9439 return VECTRNS; 9440 if ("WATTRNS".equals(codeString)) 9441 return WATTRNS; 9442 if ("_ObservationQualityMeasureAttribute".equals(codeString)) 9443 return _OBSERVATIONQUALITYMEASUREATTRIBUTE; 9444 if ("AGGREGATE".equals(codeString)) 9445 return AGGREGATE; 9446 if ("CMPMSRMTH".equals(codeString)) 9447 return CMPMSRMTH; 9448 if ("CMPMSRSCRWGHT".equals(codeString)) 9449 return CMPMSRSCRWGHT; 9450 if ("COPY".equals(codeString)) 9451 return COPY; 9452 if ("CRS".equals(codeString)) 9453 return CRS; 9454 if ("DEF".equals(codeString)) 9455 return DEF; 9456 if ("DISC".equals(codeString)) 9457 return DISC; 9458 if ("FINALDT".equals(codeString)) 9459 return FINALDT; 9460 if ("GUIDE".equals(codeString)) 9461 return GUIDE; 9462 if ("IDUR".equals(codeString)) 9463 return IDUR; 9464 if ("ITMCNT".equals(codeString)) 9465 return ITMCNT; 9466 if ("KEY".equals(codeString)) 9467 return KEY; 9468 if ("MEDT".equals(codeString)) 9469 return MEDT; 9470 if ("MSD".equals(codeString)) 9471 return MSD; 9472 if ("MSRADJ".equals(codeString)) 9473 return MSRADJ; 9474 if ("MSRAGG".equals(codeString)) 9475 return MSRAGG; 9476 if ("MSRIMPROV".equals(codeString)) 9477 return MSRIMPROV; 9478 if ("MSRJUR".equals(codeString)) 9479 return MSRJUR; 9480 if ("MSRRPTR".equals(codeString)) 9481 return MSRRPTR; 9482 if ("MSRRPTTIME".equals(codeString)) 9483 return MSRRPTTIME; 9484 if ("MSRSCORE".equals(codeString)) 9485 return MSRSCORE; 9486 if ("MSRSET".equals(codeString)) 9487 return MSRSET; 9488 if ("MSRTOPIC".equals(codeString)) 9489 return MSRTOPIC; 9490 if ("MSRTP".equals(codeString)) 9491 return MSRTP; 9492 if ("MSRTYPE".equals(codeString)) 9493 return MSRTYPE; 9494 if ("RAT".equals(codeString)) 9495 return RAT; 9496 if ("REF".equals(codeString)) 9497 return REF; 9498 if ("SDE".equals(codeString)) 9499 return SDE; 9500 if ("STRAT".equals(codeString)) 9501 return STRAT; 9502 if ("TRANF".equals(codeString)) 9503 return TRANF; 9504 if ("USE".equals(codeString)) 9505 return USE; 9506 if ("_ObservationSequenceType".equals(codeString)) 9507 return _OBSERVATIONSEQUENCETYPE; 9508 if ("TIME_ABSOLUTE".equals(codeString)) 9509 return TIMEABSOLUTE; 9510 if ("TIME_RELATIVE".equals(codeString)) 9511 return TIMERELATIVE; 9512 if ("_ObservationSeriesType".equals(codeString)) 9513 return _OBSERVATIONSERIESTYPE; 9514 if ("_ECGObservationSeriesType".equals(codeString)) 9515 return _ECGOBSERVATIONSERIESTYPE; 9516 if ("REPRESENTATIVE_BEAT".equals(codeString)) 9517 return REPRESENTATIVEBEAT; 9518 if ("RHYTHM".equals(codeString)) 9519 return RHYTHM; 9520 if ("_PatientImmunizationRelatedObservationType".equals(codeString)) 9521 return _PATIENTIMMUNIZATIONRELATEDOBSERVATIONTYPE; 9522 if ("CLSSRM".equals(codeString)) 9523 return CLSSRM; 9524 if ("GRADE".equals(codeString)) 9525 return GRADE; 9526 if ("SCHL".equals(codeString)) 9527 return SCHL; 9528 if ("SCHLDIV".equals(codeString)) 9529 return SCHLDIV; 9530 if ("TEACHER".equals(codeString)) 9531 return TEACHER; 9532 if ("_PopulationInclusionObservationType".equals(codeString)) 9533 return _POPULATIONINCLUSIONOBSERVATIONTYPE; 9534 if ("DENEX".equals(codeString)) 9535 return DENEX; 9536 if ("DENEXCEP".equals(codeString)) 9537 return DENEXCEP; 9538 if ("DENOM".equals(codeString)) 9539 return DENOM; 9540 if ("IPOP".equals(codeString)) 9541 return IPOP; 9542 if ("IPPOP".equals(codeString)) 9543 return IPPOP; 9544 if ("MSROBS".equals(codeString)) 9545 return MSROBS; 9546 if ("MSRPOPL".equals(codeString)) 9547 return MSRPOPL; 9548 if ("MSRPOPLEX".equals(codeString)) 9549 return MSRPOPLEX; 9550 if ("NUMER".equals(codeString)) 9551 return NUMER; 9552 if ("NUMEX".equals(codeString)) 9553 return NUMEX; 9554 if ("_PreferenceObservationType".equals(codeString)) 9555 return _PREFERENCEOBSERVATIONTYPE; 9556 if ("PREFSTRENGTH".equals(codeString)) 9557 return PREFSTRENGTH; 9558 if ("ADVERSE_REACTION".equals(codeString)) 9559 return ADVERSEREACTION; 9560 if ("ASSERTION".equals(codeString)) 9561 return ASSERTION; 9562 if ("CASESER".equals(codeString)) 9563 return CASESER; 9564 if ("CDIO".equals(codeString)) 9565 return CDIO; 9566 if ("CRIT".equals(codeString)) 9567 return CRIT; 9568 if ("CTMO".equals(codeString)) 9569 return CTMO; 9570 if ("DX".equals(codeString)) 9571 return DX; 9572 if ("ADMDX".equals(codeString)) 9573 return ADMDX; 9574 if ("DISDX".equals(codeString)) 9575 return DISDX; 9576 if ("INTDX".equals(codeString)) 9577 return INTDX; 9578 if ("NOI".equals(codeString)) 9579 return NOI; 9580 if ("GISTIER".equals(codeString)) 9581 return GISTIER; 9582 if ("HHOBS".equals(codeString)) 9583 return HHOBS; 9584 if ("ISSUE".equals(codeString)) 9585 return ISSUE; 9586 if ("_ActAdministrativeDetectedIssueCode".equals(codeString)) 9587 return _ACTADMINISTRATIVEDETECTEDISSUECODE; 9588 if ("_ActAdministrativeAuthorizationDetectedIssueCode".equals(codeString)) 9589 return _ACTADMINISTRATIVEAUTHORIZATIONDETECTEDISSUECODE; 9590 if ("NAT".equals(codeString)) 9591 return NAT; 9592 if ("SUPPRESSED".equals(codeString)) 9593 return SUPPRESSED; 9594 if ("VALIDAT".equals(codeString)) 9595 return VALIDAT; 9596 if ("KEY204".equals(codeString)) 9597 return KEY204; 9598 if ("KEY205".equals(codeString)) 9599 return KEY205; 9600 if ("COMPLY".equals(codeString)) 9601 return COMPLY; 9602 if ("DUPTHPY".equals(codeString)) 9603 return DUPTHPY; 9604 if ("DUPTHPCLS".equals(codeString)) 9605 return DUPTHPCLS; 9606 if ("DUPTHPGEN".equals(codeString)) 9607 return DUPTHPGEN; 9608 if ("ABUSE".equals(codeString)) 9609 return ABUSE; 9610 if ("FRAUD".equals(codeString)) 9611 return FRAUD; 9612 if ("PLYDOC".equals(codeString)) 9613 return PLYDOC; 9614 if ("PLYPHRM".equals(codeString)) 9615 return PLYPHRM; 9616 if ("DOSE".equals(codeString)) 9617 return DOSE; 9618 if ("DOSECOND".equals(codeString)) 9619 return DOSECOND; 9620 if ("DOSEDUR".equals(codeString)) 9621 return DOSEDUR; 9622 if ("DOSEDURH".equals(codeString)) 9623 return DOSEDURH; 9624 if ("DOSEDURHIND".equals(codeString)) 9625 return DOSEDURHIND; 9626 if ("DOSEDURL".equals(codeString)) 9627 return DOSEDURL; 9628 if ("DOSEDURLIND".equals(codeString)) 9629 return DOSEDURLIND; 9630 if ("DOSEH".equals(codeString)) 9631 return DOSEH; 9632 if ("DOSEHINDA".equals(codeString)) 9633 return DOSEHINDA; 9634 if ("DOSEHIND".equals(codeString)) 9635 return DOSEHIND; 9636 if ("DOSEHINDSA".equals(codeString)) 9637 return DOSEHINDSA; 9638 if ("DOSEHINDW".equals(codeString)) 9639 return DOSEHINDW; 9640 if ("DOSEIVL".equals(codeString)) 9641 return DOSEIVL; 9642 if ("DOSEIVLIND".equals(codeString)) 9643 return DOSEIVLIND; 9644 if ("DOSEL".equals(codeString)) 9645 return DOSEL; 9646 if ("DOSELINDA".equals(codeString)) 9647 return DOSELINDA; 9648 if ("DOSELIND".equals(codeString)) 9649 return DOSELIND; 9650 if ("DOSELINDSA".equals(codeString)) 9651 return DOSELINDSA; 9652 if ("DOSELINDW".equals(codeString)) 9653 return DOSELINDW; 9654 if ("MDOSE".equals(codeString)) 9655 return MDOSE; 9656 if ("OBSA".equals(codeString)) 9657 return OBSA; 9658 if ("AGE".equals(codeString)) 9659 return AGE; 9660 if ("ADALRT".equals(codeString)) 9661 return ADALRT; 9662 if ("GEALRT".equals(codeString)) 9663 return GEALRT; 9664 if ("PEALRT".equals(codeString)) 9665 return PEALRT; 9666 if ("COND".equals(codeString)) 9667 return COND; 9668 if ("HGHT".equals(codeString)) 9669 return HGHT; 9670 if ("LACT".equals(codeString)) 9671 return LACT; 9672 if ("PREG".equals(codeString)) 9673 return PREG; 9674 if ("WGHT".equals(codeString)) 9675 return WGHT; 9676 if ("CREACT".equals(codeString)) 9677 return CREACT; 9678 if ("GEN".equals(codeString)) 9679 return GEN; 9680 if ("GEND".equals(codeString)) 9681 return GEND; 9682 if ("LAB".equals(codeString)) 9683 return LAB; 9684 if ("REACT".equals(codeString)) 9685 return REACT; 9686 if ("ALGY".equals(codeString)) 9687 return ALGY; 9688 if ("INT".equals(codeString)) 9689 return INT; 9690 if ("RREACT".equals(codeString)) 9691 return RREACT; 9692 if ("RALG".equals(codeString)) 9693 return RALG; 9694 if ("RAR".equals(codeString)) 9695 return RAR; 9696 if ("RINT".equals(codeString)) 9697 return RINT; 9698 if ("BUS".equals(codeString)) 9699 return BUS; 9700 if ("CODE_INVAL".equals(codeString)) 9701 return CODEINVAL; 9702 if ("CODE_DEPREC".equals(codeString)) 9703 return CODEDEPREC; 9704 if ("FORMAT".equals(codeString)) 9705 return FORMAT; 9706 if ("ILLEGAL".equals(codeString)) 9707 return ILLEGAL; 9708 if ("LEN_RANGE".equals(codeString)) 9709 return LENRANGE; 9710 if ("LEN_LONG".equals(codeString)) 9711 return LENLONG; 9712 if ("LEN_SHORT".equals(codeString)) 9713 return LENSHORT; 9714 if ("MISSCOND".equals(codeString)) 9715 return MISSCOND; 9716 if ("MISSMAND".equals(codeString)) 9717 return MISSMAND; 9718 if ("NODUPS".equals(codeString)) 9719 return NODUPS; 9720 if ("NOPERSIST".equals(codeString)) 9721 return NOPERSIST; 9722 if ("REP_RANGE".equals(codeString)) 9723 return REPRANGE; 9724 if ("MAXOCCURS".equals(codeString)) 9725 return MAXOCCURS; 9726 if ("MINOCCURS".equals(codeString)) 9727 return MINOCCURS; 9728 if ("_ActAdministrativeRuleDetectedIssueCode".equals(codeString)) 9729 return _ACTADMINISTRATIVERULEDETECTEDISSUECODE; 9730 if ("KEY206".equals(codeString)) 9731 return KEY206; 9732 if ("OBSOLETE".equals(codeString)) 9733 return OBSOLETE; 9734 if ("_ActSuppliedItemDetectedIssueCode".equals(codeString)) 9735 return _ACTSUPPLIEDITEMDETECTEDISSUECODE; 9736 if ("_AdministrationDetectedIssueCode".equals(codeString)) 9737 return _ADMINISTRATIONDETECTEDISSUECODE; 9738 if ("_AppropriatenessDetectedIssueCode".equals(codeString)) 9739 return _APPROPRIATENESSDETECTEDISSUECODE; 9740 if ("_InteractionDetectedIssueCode".equals(codeString)) 9741 return _INTERACTIONDETECTEDISSUECODE; 9742 if ("FOOD".equals(codeString)) 9743 return FOOD; 9744 if ("TPROD".equals(codeString)) 9745 return TPROD; 9746 if ("DRG".equals(codeString)) 9747 return DRG; 9748 if ("NHP".equals(codeString)) 9749 return NHP; 9750 if ("NONRX".equals(codeString)) 9751 return NONRX; 9752 if ("PREVINEF".equals(codeString)) 9753 return PREVINEF; 9754 if ("DACT".equals(codeString)) 9755 return DACT; 9756 if ("TIME".equals(codeString)) 9757 return TIME; 9758 if ("ALRTENDLATE".equals(codeString)) 9759 return ALRTENDLATE; 9760 if ("ALRTSTRTLATE".equals(codeString)) 9761 return ALRTSTRTLATE; 9762 if ("_TimingDetectedIssueCode".equals(codeString)) 9763 return _TIMINGDETECTEDISSUECODE; 9764 if ("ENDLATE".equals(codeString)) 9765 return ENDLATE; 9766 if ("STRTLATE".equals(codeString)) 9767 return STRTLATE; 9768 if ("_SupplyDetectedIssueCode".equals(codeString)) 9769 return _SUPPLYDETECTEDISSUECODE; 9770 if ("ALLDONE".equals(codeString)) 9771 return ALLDONE; 9772 if ("FULFIL".equals(codeString)) 9773 return FULFIL; 9774 if ("NOTACTN".equals(codeString)) 9775 return NOTACTN; 9776 if ("NOTEQUIV".equals(codeString)) 9777 return NOTEQUIV; 9778 if ("NOTEQUIVGEN".equals(codeString)) 9779 return NOTEQUIVGEN; 9780 if ("NOTEQUIVTHER".equals(codeString)) 9781 return NOTEQUIVTHER; 9782 if ("TIMING".equals(codeString)) 9783 return TIMING; 9784 if ("INTERVAL".equals(codeString)) 9785 return INTERVAL; 9786 if ("MINFREQ".equals(codeString)) 9787 return MINFREQ; 9788 if ("HELD".equals(codeString)) 9789 return HELD; 9790 if ("TOOLATE".equals(codeString)) 9791 return TOOLATE; 9792 if ("TOOSOON".equals(codeString)) 9793 return TOOSOON; 9794 if ("HISTORIC".equals(codeString)) 9795 return HISTORIC; 9796 if ("PATPREF".equals(codeString)) 9797 return PATPREF; 9798 if ("PATPREFALT".equals(codeString)) 9799 return PATPREFALT; 9800 if ("KSUBJ".equals(codeString)) 9801 return KSUBJ; 9802 if ("KSUBT".equals(codeString)) 9803 return KSUBT; 9804 if ("OINT".equals(codeString)) 9805 return OINT; 9806 if ("ALG".equals(codeString)) 9807 return ALG; 9808 if ("DALG".equals(codeString)) 9809 return DALG; 9810 if ("EALG".equals(codeString)) 9811 return EALG; 9812 if ("FALG".equals(codeString)) 9813 return FALG; 9814 if ("DINT".equals(codeString)) 9815 return DINT; 9816 if ("DNAINT".equals(codeString)) 9817 return DNAINT; 9818 if ("EINT".equals(codeString)) 9819 return EINT; 9820 if ("ENAINT".equals(codeString)) 9821 return ENAINT; 9822 if ("FINT".equals(codeString)) 9823 return FINT; 9824 if ("FNAINT".equals(codeString)) 9825 return FNAINT; 9826 if ("NAINT".equals(codeString)) 9827 return NAINT; 9828 if ("SEV".equals(codeString)) 9829 return SEV; 9830 if ("_FDALabelData".equals(codeString)) 9831 return _FDALABELDATA; 9832 if ("FDACOATING".equals(codeString)) 9833 return FDACOATING; 9834 if ("FDACOLOR".equals(codeString)) 9835 return FDACOLOR; 9836 if ("FDAIMPRINTCD".equals(codeString)) 9837 return FDAIMPRINTCD; 9838 if ("FDALOGO".equals(codeString)) 9839 return FDALOGO; 9840 if ("FDASCORING".equals(codeString)) 9841 return FDASCORING; 9842 if ("FDASHAPE".equals(codeString)) 9843 return FDASHAPE; 9844 if ("FDASIZE".equals(codeString)) 9845 return FDASIZE; 9846 if ("_ROIOverlayShape".equals(codeString)) 9847 return _ROIOVERLAYSHAPE; 9848 if ("CIRCLE".equals(codeString)) 9849 return CIRCLE; 9850 if ("ELLIPSE".equals(codeString)) 9851 return ELLIPSE; 9852 if ("POINT".equals(codeString)) 9853 return POINT; 9854 if ("POLY".equals(codeString)) 9855 return POLY; 9856 if ("C".equals(codeString)) 9857 return C; 9858 if ("DIET".equals(codeString)) 9859 return DIET; 9860 if ("BR".equals(codeString)) 9861 return BR; 9862 if ("DM".equals(codeString)) 9863 return DM; 9864 if ("FAST".equals(codeString)) 9865 return FAST; 9866 if ("FORMULA".equals(codeString)) 9867 return FORMULA; 9868 if ("GF".equals(codeString)) 9869 return GF; 9870 if ("LF".equals(codeString)) 9871 return LF; 9872 if ("LP".equals(codeString)) 9873 return LP; 9874 if ("LQ".equals(codeString)) 9875 return LQ; 9876 if ("LS".equals(codeString)) 9877 return LS; 9878 if ("N".equals(codeString)) 9879 return N; 9880 if ("NF".equals(codeString)) 9881 return NF; 9882 if ("PAF".equals(codeString)) 9883 return PAF; 9884 if ("PAR".equals(codeString)) 9885 return PAR; 9886 if ("RD".equals(codeString)) 9887 return RD; 9888 if ("SCH".equals(codeString)) 9889 return SCH; 9890 if ("SUPPLEMENT".equals(codeString)) 9891 return SUPPLEMENT; 9892 if ("T".equals(codeString)) 9893 return T; 9894 if ("VLI".equals(codeString)) 9895 return VLI; 9896 if ("DRUGPRG".equals(codeString)) 9897 return DRUGPRG; 9898 if ("F".equals(codeString)) 9899 return F; 9900 if ("PRLMN".equals(codeString)) 9901 return PRLMN; 9902 if ("SECOBS".equals(codeString)) 9903 return SECOBS; 9904 if ("SECCATOBS".equals(codeString)) 9905 return SECCATOBS; 9906 if ("SECCLASSOBS".equals(codeString)) 9907 return SECCLASSOBS; 9908 if ("SECCONOBS".equals(codeString)) 9909 return SECCONOBS; 9910 if ("SECINTOBS".equals(codeString)) 9911 return SECINTOBS; 9912 if ("SECALTINTOBS".equals(codeString)) 9913 return SECALTINTOBS; 9914 if ("SECDATINTOBS".equals(codeString)) 9915 return SECDATINTOBS; 9916 if ("SECINTCONOBS".equals(codeString)) 9917 return SECINTCONOBS; 9918 if ("SECINTPRVOBS".equals(codeString)) 9919 return SECINTPRVOBS; 9920 if ("SECINTPRVABOBS".equals(codeString)) 9921 return SECINTPRVABOBS; 9922 if ("SECINTPRVRBOBS".equals(codeString)) 9923 return SECINTPRVRBOBS; 9924 if ("SECINTSTOBS".equals(codeString)) 9925 return SECINTSTOBS; 9926 if ("SECTRSTOBS".equals(codeString)) 9927 return SECTRSTOBS; 9928 if ("TRSTACCRDOBS".equals(codeString)) 9929 return TRSTACCRDOBS; 9930 if ("TRSTAGREOBS".equals(codeString)) 9931 return TRSTAGREOBS; 9932 if ("TRSTCERTOBS".equals(codeString)) 9933 return TRSTCERTOBS; 9934 if ("TRSTFWKOBS".equals(codeString)) 9935 return TRSTFWKOBS; 9936 if ("TRSTLOAOBS".equals(codeString)) 9937 return TRSTLOAOBS; 9938 if ("TRSTMECOBS".equals(codeString)) 9939 return TRSTMECOBS; 9940 if ("SUBSIDFFS".equals(codeString)) 9941 return SUBSIDFFS; 9942 if ("WRKCOMP".equals(codeString)) 9943 return WRKCOMP; 9944 if ("_ActProcedureCode".equals(codeString)) 9945 return _ACTPROCEDURECODE; 9946 if ("_ActBillableServiceCode".equals(codeString)) 9947 return _ACTBILLABLESERVICECODE; 9948 if ("_HL7DefinedActCodes".equals(codeString)) 9949 return _HL7DEFINEDACTCODES; 9950 if ("COPAY".equals(codeString)) 9951 return COPAY; 9952 if ("DEDUCT".equals(codeString)) 9953 return DEDUCT; 9954 if ("DOSEIND".equals(codeString)) 9955 return DOSEIND; 9956 if ("PRA".equals(codeString)) 9957 return PRA; 9958 if ("STORE".equals(codeString)) 9959 return STORE; 9960 throw new FHIRException("Unknown V3ActCode code '" + codeString + "'"); 9961 } 9962 9963 public String toCode() { 9964 switch (this) { 9965 case _ACTACCOUNTCODE: 9966 return "_ActAccountCode"; 9967 case ACCTRECEIVABLE: 9968 return "ACCTRECEIVABLE"; 9969 case CASH: 9970 return "CASH"; 9971 case CC: 9972 return "CC"; 9973 case AE: 9974 return "AE"; 9975 case DN: 9976 return "DN"; 9977 case DV: 9978 return "DV"; 9979 case MC: 9980 return "MC"; 9981 case V: 9982 return "V"; 9983 case PBILLACCT: 9984 return "PBILLACCT"; 9985 case _ACTADJUDICATIONCODE: 9986 return "_ActAdjudicationCode"; 9987 case _ACTADJUDICATIONGROUPCODE: 9988 return "_ActAdjudicationGroupCode"; 9989 case CONT: 9990 return "CONT"; 9991 case DAY: 9992 return "DAY"; 9993 case LOC: 9994 return "LOC"; 9995 case MONTH: 9996 return "MONTH"; 9997 case PERIOD: 9998 return "PERIOD"; 9999 case PROV: 10000 return "PROV"; 10001 case WEEK: 10002 return "WEEK"; 10003 case YEAR: 10004 return "YEAR"; 10005 case AA: 10006 return "AA"; 10007 case ANF: 10008 return "ANF"; 10009 case AR: 10010 return "AR"; 10011 case AS: 10012 return "AS"; 10013 case _ACTADJUDICATIONRESULTACTIONCODE: 10014 return "_ActAdjudicationResultActionCode"; 10015 case DISPLAY: 10016 return "DISPLAY"; 10017 case FORM: 10018 return "FORM"; 10019 case _ACTBILLABLEMODIFIERCODE: 10020 return "_ActBillableModifierCode"; 10021 case CPTM: 10022 return "CPTM"; 10023 case HCPCSA: 10024 return "HCPCSA"; 10025 case _ACTBILLINGARRANGEMENTCODE: 10026 return "_ActBillingArrangementCode"; 10027 case BLK: 10028 return "BLK"; 10029 case CAP: 10030 return "CAP"; 10031 case CONTF: 10032 return "CONTF"; 10033 case FINBILL: 10034 return "FINBILL"; 10035 case ROST: 10036 return "ROST"; 10037 case SESS: 10038 return "SESS"; 10039 case FFS: 10040 return "FFS"; 10041 case FFPS: 10042 return "FFPS"; 10043 case FFCS: 10044 return "FFCS"; 10045 case TFS: 10046 return "TFS"; 10047 case _ACTBOUNDEDROICODE: 10048 return "_ActBoundedROICode"; 10049 case ROIFS: 10050 return "ROIFS"; 10051 case ROIPS: 10052 return "ROIPS"; 10053 case _ACTCAREPROVISIONCODE: 10054 return "_ActCareProvisionCode"; 10055 case _ACTCREDENTIALEDCARECODE: 10056 return "_ActCredentialedCareCode"; 10057 case _ACTCREDENTIALEDCAREPROVISIONPERSONCODE: 10058 return "_ActCredentialedCareProvisionPersonCode"; 10059 case CACC: 10060 return "CACC"; 10061 case CAIC: 10062 return "CAIC"; 10063 case CAMC: 10064 return "CAMC"; 10065 case CANC: 10066 return "CANC"; 10067 case CAPC: 10068 return "CAPC"; 10069 case CBGC: 10070 return "CBGC"; 10071 case CCCC: 10072 return "CCCC"; 10073 case CCGC: 10074 return "CCGC"; 10075 case CCPC: 10076 return "CCPC"; 10077 case CCSC: 10078 return "CCSC"; 10079 case CDEC: 10080 return "CDEC"; 10081 case CDRC: 10082 return "CDRC"; 10083 case CEMC: 10084 return "CEMC"; 10085 case CFPC: 10086 return "CFPC"; 10087 case CIMC: 10088 return "CIMC"; 10089 case CMGC: 10090 return "CMGC"; 10091 case CNEC: 10092 return "CNEC"; 10093 case CNMC: 10094 return "CNMC"; 10095 case CNQC: 10096 return "CNQC"; 10097 case CNSC: 10098 return "CNSC"; 10099 case COGC: 10100 return "COGC"; 10101 case COMC: 10102 return "COMC"; 10103 case COPC: 10104 return "COPC"; 10105 case COSC: 10106 return "COSC"; 10107 case COTC: 10108 return "COTC"; 10109 case CPEC: 10110 return "CPEC"; 10111 case CPGC: 10112 return "CPGC"; 10113 case CPHC: 10114 return "CPHC"; 10115 case CPRC: 10116 return "CPRC"; 10117 case CPSC: 10118 return "CPSC"; 10119 case CPYC: 10120 return "CPYC"; 10121 case CROC: 10122 return "CROC"; 10123 case CRPC: 10124 return "CRPC"; 10125 case CSUC: 10126 return "CSUC"; 10127 case CTSC: 10128 return "CTSC"; 10129 case CURC: 10130 return "CURC"; 10131 case CVSC: 10132 return "CVSC"; 10133 case LGPC: 10134 return "LGPC"; 10135 case _ACTCREDENTIALEDCAREPROVISIONPROGRAMCODE: 10136 return "_ActCredentialedCareProvisionProgramCode"; 10137 case AALC: 10138 return "AALC"; 10139 case AAMC: 10140 return "AAMC"; 10141 case ABHC: 10142 return "ABHC"; 10143 case ACAC: 10144 return "ACAC"; 10145 case ACHC: 10146 return "ACHC"; 10147 case AHOC: 10148 return "AHOC"; 10149 case ALTC: 10150 return "ALTC"; 10151 case AOSC: 10152 return "AOSC"; 10153 case CACS: 10154 return "CACS"; 10155 case CAMI: 10156 return "CAMI"; 10157 case CAST: 10158 return "CAST"; 10159 case CBAR: 10160 return "CBAR"; 10161 case CCAD: 10162 return "CCAD"; 10163 case CCAR: 10164 return "CCAR"; 10165 case CDEP: 10166 return "CDEP"; 10167 case CDGD: 10168 return "CDGD"; 10169 case CDIA: 10170 return "CDIA"; 10171 case CEPI: 10172 return "CEPI"; 10173 case CFEL: 10174 return "CFEL"; 10175 case CHFC: 10176 return "CHFC"; 10177 case CHRO: 10178 return "CHRO"; 10179 case CHYP: 10180 return "CHYP"; 10181 case CMIH: 10182 return "CMIH"; 10183 case CMSC: 10184 return "CMSC"; 10185 case COJR: 10186 return "COJR"; 10187 case CONC: 10188 return "CONC"; 10189 case COPD: 10190 return "COPD"; 10191 case CORT: 10192 return "CORT"; 10193 case CPAD: 10194 return "CPAD"; 10195 case CPND: 10196 return "CPND"; 10197 case CPST: 10198 return "CPST"; 10199 case CSDM: 10200 return "CSDM"; 10201 case CSIC: 10202 return "CSIC"; 10203 case CSLD: 10204 return "CSLD"; 10205 case CSPT: 10206 return "CSPT"; 10207 case CTBU: 10208 return "CTBU"; 10209 case CVDC: 10210 return "CVDC"; 10211 case CWMA: 10212 return "CWMA"; 10213 case CWOH: 10214 return "CWOH"; 10215 case _ACTENCOUNTERCODE: 10216 return "_ActEncounterCode"; 10217 case AMB: 10218 return "AMB"; 10219 case EMER: 10220 return "EMER"; 10221 case FLD: 10222 return "FLD"; 10223 case HH: 10224 return "HH"; 10225 case IMP: 10226 return "IMP"; 10227 case ACUTE: 10228 return "ACUTE"; 10229 case NONAC: 10230 return "NONAC"; 10231 case OBSENC: 10232 return "OBSENC"; 10233 case PRENC: 10234 return "PRENC"; 10235 case SS: 10236 return "SS"; 10237 case VR: 10238 return "VR"; 10239 case _ACTMEDICALSERVICECODE: 10240 return "_ActMedicalServiceCode"; 10241 case ALC: 10242 return "ALC"; 10243 case CARD: 10244 return "CARD"; 10245 case CHR: 10246 return "CHR"; 10247 case DNTL: 10248 return "DNTL"; 10249 case DRGRHB: 10250 return "DRGRHB"; 10251 case GENRL: 10252 return "GENRL"; 10253 case MED: 10254 return "MED"; 10255 case OBS: 10256 return "OBS"; 10257 case ONC: 10258 return "ONC"; 10259 case PALL: 10260 return "PALL"; 10261 case PED: 10262 return "PED"; 10263 case PHAR: 10264 return "PHAR"; 10265 case PHYRHB: 10266 return "PHYRHB"; 10267 case PSYCH: 10268 return "PSYCH"; 10269 case SURG: 10270 return "SURG"; 10271 case _ACTCLAIMATTACHMENTCATEGORYCODE: 10272 return "_ActClaimAttachmentCategoryCode"; 10273 case AUTOATTCH: 10274 return "AUTOATTCH"; 10275 case DOCUMENT: 10276 return "DOCUMENT"; 10277 case HEALTHREC: 10278 return "HEALTHREC"; 10279 case IMG: 10280 return "IMG"; 10281 case LABRESULTS: 10282 return "LABRESULTS"; 10283 case MODEL: 10284 return "MODEL"; 10285 case WIATTCH: 10286 return "WIATTCH"; 10287 case XRAY: 10288 return "XRAY"; 10289 case _ACTCONSENTTYPE: 10290 return "_ActConsentType"; 10291 case ICOL: 10292 return "ICOL"; 10293 case IDSCL: 10294 return "IDSCL"; 10295 case INFA: 10296 return "INFA"; 10297 case INFAO: 10298 return "INFAO"; 10299 case INFASO: 10300 return "INFASO"; 10301 case IRDSCL: 10302 return "IRDSCL"; 10303 case RESEARCH: 10304 return "RESEARCH"; 10305 case RSDID: 10306 return "RSDID"; 10307 case RSREID: 10308 return "RSREID"; 10309 case _ACTCONTAINERREGISTRATIONCODE: 10310 return "_ActContainerRegistrationCode"; 10311 case ID: 10312 return "ID"; 10313 case IP: 10314 return "IP"; 10315 case L: 10316 return "L"; 10317 case M: 10318 return "M"; 10319 case O: 10320 return "O"; 10321 case R: 10322 return "R"; 10323 case X: 10324 return "X"; 10325 case _ACTCONTROLVARIABLE: 10326 return "_ActControlVariable"; 10327 case AUTO: 10328 return "AUTO"; 10329 case ENDC: 10330 return "ENDC"; 10331 case REFLEX: 10332 return "REFLEX"; 10333 case _ACTCOVERAGECONFIRMATIONCODE: 10334 return "_ActCoverageConfirmationCode"; 10335 case _ACTCOVERAGEAUTHORIZATIONCONFIRMATIONCODE: 10336 return "_ActCoverageAuthorizationConfirmationCode"; 10337 case AUTH: 10338 return "AUTH"; 10339 case NAUTH: 10340 return "NAUTH"; 10341 case _ACTCOVERAGEELIGIBILITYCONFIRMATIONCODE: 10342 return "_ActCoverageEligibilityConfirmationCode"; 10343 case ELG: 10344 return "ELG"; 10345 case NELG: 10346 return "NELG"; 10347 case _ACTCOVERAGELIMITCODE: 10348 return "_ActCoverageLimitCode"; 10349 case _ACTCOVERAGEQUANTITYLIMITCODE: 10350 return "_ActCoverageQuantityLimitCode"; 10351 case COVPRD: 10352 return "COVPRD"; 10353 case LFEMX: 10354 return "LFEMX"; 10355 case NETAMT: 10356 return "NETAMT"; 10357 case PRDMX: 10358 return "PRDMX"; 10359 case UNITPRICE: 10360 return "UNITPRICE"; 10361 case UNITQTY: 10362 return "UNITQTY"; 10363 case COVMX: 10364 return "COVMX"; 10365 case _ACTCOVEREDPARTYLIMITCODE: 10366 return "_ActCoveredPartyLimitCode"; 10367 case _ACTCOVERAGETYPECODE: 10368 return "_ActCoverageTypeCode"; 10369 case _ACTINSURANCEPOLICYCODE: 10370 return "_ActInsurancePolicyCode"; 10371 case EHCPOL: 10372 return "EHCPOL"; 10373 case HSAPOL: 10374 return "HSAPOL"; 10375 case AUTOPOL: 10376 return "AUTOPOL"; 10377 case COL: 10378 return "COL"; 10379 case UNINSMOT: 10380 return "UNINSMOT"; 10381 case PUBLICPOL: 10382 return "PUBLICPOL"; 10383 case DENTPRG: 10384 return "DENTPRG"; 10385 case DISEASEPRG: 10386 return "DISEASEPRG"; 10387 case CANPRG: 10388 return "CANPRG"; 10389 case ENDRENAL: 10390 return "ENDRENAL"; 10391 case HIVAIDS: 10392 return "HIVAIDS"; 10393 case MANDPOL: 10394 return "MANDPOL"; 10395 case MENTPRG: 10396 return "MENTPRG"; 10397 case SAFNET: 10398 return "SAFNET"; 10399 case SUBPRG: 10400 return "SUBPRG"; 10401 case SUBSIDIZ: 10402 return "SUBSIDIZ"; 10403 case SUBSIDMC: 10404 return "SUBSIDMC"; 10405 case SUBSUPP: 10406 return "SUBSUPP"; 10407 case WCBPOL: 10408 return "WCBPOL"; 10409 case _ACTINSURANCETYPECODE: 10410 return "_ActInsuranceTypeCode"; 10411 case _ACTHEALTHINSURANCETYPECODE: 10412 return "_ActHealthInsuranceTypeCode"; 10413 case DENTAL: 10414 return "DENTAL"; 10415 case DISEASE: 10416 return "DISEASE"; 10417 case DRUGPOL: 10418 return "DRUGPOL"; 10419 case HIP: 10420 return "HIP"; 10421 case LTC: 10422 return "LTC"; 10423 case MCPOL: 10424 return "MCPOL"; 10425 case POS: 10426 return "POS"; 10427 case HMO: 10428 return "HMO"; 10429 case PPO: 10430 return "PPO"; 10431 case MENTPOL: 10432 return "MENTPOL"; 10433 case SUBPOL: 10434 return "SUBPOL"; 10435 case VISPOL: 10436 return "VISPOL"; 10437 case DIS: 10438 return "DIS"; 10439 case EWB: 10440 return "EWB"; 10441 case FLEXP: 10442 return "FLEXP"; 10443 case LIFE: 10444 return "LIFE"; 10445 case ANNU: 10446 return "ANNU"; 10447 case TLIFE: 10448 return "TLIFE"; 10449 case ULIFE: 10450 return "ULIFE"; 10451 case PNC: 10452 return "PNC"; 10453 case REI: 10454 return "REI"; 10455 case SURPL: 10456 return "SURPL"; 10457 case UMBRL: 10458 return "UMBRL"; 10459 case _ACTPROGRAMTYPECODE: 10460 return "_ActProgramTypeCode"; 10461 case CHAR: 10462 return "CHAR"; 10463 case CRIME: 10464 return "CRIME"; 10465 case EAP: 10466 return "EAP"; 10467 case GOVEMP: 10468 return "GOVEMP"; 10469 case HIRISK: 10470 return "HIRISK"; 10471 case IND: 10472 return "IND"; 10473 case MILITARY: 10474 return "MILITARY"; 10475 case RETIRE: 10476 return "RETIRE"; 10477 case SOCIAL: 10478 return "SOCIAL"; 10479 case VET: 10480 return "VET"; 10481 case _ACTDETECTEDISSUEMANAGEMENTCODE: 10482 return "_ActDetectedIssueManagementCode"; 10483 case _ACTADMINISTRATIVEDETECTEDISSUEMANAGEMENTCODE: 10484 return "_ActAdministrativeDetectedIssueManagementCode"; 10485 case _AUTHORIZATIONISSUEMANAGEMENTCODE: 10486 return "_AuthorizationIssueManagementCode"; 10487 case EMAUTH: 10488 return "EMAUTH"; 10489 case _21: 10490 return "21"; 10491 case _1: 10492 return "1"; 10493 case _19: 10494 return "19"; 10495 case _2: 10496 return "2"; 10497 case _22: 10498 return "22"; 10499 case _23: 10500 return "23"; 10501 case _3: 10502 return "3"; 10503 case _4: 10504 return "4"; 10505 case _5: 10506 return "5"; 10507 case _6: 10508 return "6"; 10509 case _7: 10510 return "7"; 10511 case _14: 10512 return "14"; 10513 case _15: 10514 return "15"; 10515 case _16: 10516 return "16"; 10517 case _17: 10518 return "17"; 10519 case _18: 10520 return "18"; 10521 case _20: 10522 return "20"; 10523 case _8: 10524 return "8"; 10525 case _10: 10526 return "10"; 10527 case _11: 10528 return "11"; 10529 case _12: 10530 return "12"; 10531 case _13: 10532 return "13"; 10533 case _9: 10534 return "9"; 10535 case _ACTEXPOSURECODE: 10536 return "_ActExposureCode"; 10537 case CHLDCARE: 10538 return "CHLDCARE"; 10539 case CONVEYNC: 10540 return "CONVEYNC"; 10541 case HLTHCARE: 10542 return "HLTHCARE"; 10543 case HOMECARE: 10544 return "HOMECARE"; 10545 case HOSPPTNT: 10546 return "HOSPPTNT"; 10547 case HOSPVSTR: 10548 return "HOSPVSTR"; 10549 case HOUSEHLD: 10550 return "HOUSEHLD"; 10551 case INMATE: 10552 return "INMATE"; 10553 case INTIMATE: 10554 return "INTIMATE"; 10555 case LTRMCARE: 10556 return "LTRMCARE"; 10557 case PLACE: 10558 return "PLACE"; 10559 case PTNTCARE: 10560 return "PTNTCARE"; 10561 case SCHOOL2: 10562 return "SCHOOL2"; 10563 case SOCIAL2: 10564 return "SOCIAL2"; 10565 case SUBSTNCE: 10566 return "SUBSTNCE"; 10567 case TRAVINT: 10568 return "TRAVINT"; 10569 case WORK2: 10570 return "WORK2"; 10571 case _ACTFINANCIALTRANSACTIONCODE: 10572 return "_ActFinancialTransactionCode"; 10573 case CHRG: 10574 return "CHRG"; 10575 case REV: 10576 return "REV"; 10577 case _ACTINCIDENTCODE: 10578 return "_ActIncidentCode"; 10579 case MVA: 10580 return "MVA"; 10581 case SCHOOL: 10582 return "SCHOOL"; 10583 case SPT: 10584 return "SPT"; 10585 case WPA: 10586 return "WPA"; 10587 case _ACTINFORMATIONACCESSCODE: 10588 return "_ActInformationAccessCode"; 10589 case ACADR: 10590 return "ACADR"; 10591 case ACALL: 10592 return "ACALL"; 10593 case ACALLG: 10594 return "ACALLG"; 10595 case ACCONS: 10596 return "ACCONS"; 10597 case ACDEMO: 10598 return "ACDEMO"; 10599 case ACDI: 10600 return "ACDI"; 10601 case ACIMMUN: 10602 return "ACIMMUN"; 10603 case ACLAB: 10604 return "ACLAB"; 10605 case ACMED: 10606 return "ACMED"; 10607 case ACMEDC: 10608 return "ACMEDC"; 10609 case ACMEN: 10610 return "ACMEN"; 10611 case ACOBS: 10612 return "ACOBS"; 10613 case ACPOLPRG: 10614 return "ACPOLPRG"; 10615 case ACPROV: 10616 return "ACPROV"; 10617 case ACPSERV: 10618 return "ACPSERV"; 10619 case ACSUBSTAB: 10620 return "ACSUBSTAB"; 10621 case _ACTINFORMATIONACCESSCONTEXTCODE: 10622 return "_ActInformationAccessContextCode"; 10623 case INFAUT: 10624 return "INFAUT"; 10625 case INFCON: 10626 return "INFCON"; 10627 case INFCRT: 10628 return "INFCRT"; 10629 case INFDNG: 10630 return "INFDNG"; 10631 case INFEMER: 10632 return "INFEMER"; 10633 case INFPWR: 10634 return "INFPWR"; 10635 case INFREG: 10636 return "INFREG"; 10637 case _ACTINFORMATIONCATEGORYCODE: 10638 return "_ActInformationCategoryCode"; 10639 case ALLCAT: 10640 return "ALLCAT"; 10641 case ALLGCAT: 10642 return "ALLGCAT"; 10643 case ARCAT: 10644 return "ARCAT"; 10645 case COBSCAT: 10646 return "COBSCAT"; 10647 case DEMOCAT: 10648 return "DEMOCAT"; 10649 case DICAT: 10650 return "DICAT"; 10651 case IMMUCAT: 10652 return "IMMUCAT"; 10653 case LABCAT: 10654 return "LABCAT"; 10655 case MEDCCAT: 10656 return "MEDCCAT"; 10657 case MENCAT: 10658 return "MENCAT"; 10659 case PSVCCAT: 10660 return "PSVCCAT"; 10661 case RXCAT: 10662 return "RXCAT"; 10663 case _ACTINVOICEELEMENTCODE: 10664 return "_ActInvoiceElementCode"; 10665 case _ACTINVOICEADJUDICATIONPAYMENTCODE: 10666 return "_ActInvoiceAdjudicationPaymentCode"; 10667 case _ACTINVOICEADJUDICATIONPAYMENTGROUPCODE: 10668 return "_ActInvoiceAdjudicationPaymentGroupCode"; 10669 case ALEC: 10670 return "ALEC"; 10671 case BONUS: 10672 return "BONUS"; 10673 case CFWD: 10674 return "CFWD"; 10675 case EDU: 10676 return "EDU"; 10677 case EPYMT: 10678 return "EPYMT"; 10679 case GARN: 10680 return "GARN"; 10681 case INVOICE: 10682 return "INVOICE"; 10683 case PINV: 10684 return "PINV"; 10685 case PPRD: 10686 return "PPRD"; 10687 case PROA: 10688 return "PROA"; 10689 case RECOV: 10690 return "RECOV"; 10691 case RETRO: 10692 return "RETRO"; 10693 case TRAN: 10694 return "TRAN"; 10695 case _ACTINVOICEADJUDICATIONPAYMENTSUMMARYCODE: 10696 return "_ActInvoiceAdjudicationPaymentSummaryCode"; 10697 case INVTYPE: 10698 return "INVTYPE"; 10699 case PAYEE: 10700 return "PAYEE"; 10701 case PAYOR: 10702 return "PAYOR"; 10703 case SENDAPP: 10704 return "SENDAPP"; 10705 case _ACTINVOICEDETAILCODE: 10706 return "_ActInvoiceDetailCode"; 10707 case _ACTINVOICEDETAILCLINICALPRODUCTCODE: 10708 return "_ActInvoiceDetailClinicalProductCode"; 10709 case UNSPSC: 10710 return "UNSPSC"; 10711 case _ACTINVOICEDETAILDRUGPRODUCTCODE: 10712 return "_ActInvoiceDetailDrugProductCode"; 10713 case GTIN: 10714 return "GTIN"; 10715 case UPC: 10716 return "UPC"; 10717 case _ACTINVOICEDETAILGENERICCODE: 10718 return "_ActInvoiceDetailGenericCode"; 10719 case _ACTINVOICEDETAILGENERICADJUDICATORCODE: 10720 return "_ActInvoiceDetailGenericAdjudicatorCode"; 10721 case COIN: 10722 return "COIN"; 10723 case COPAYMENT: 10724 return "COPAYMENT"; 10725 case DEDUCTIBLE: 10726 return "DEDUCTIBLE"; 10727 case PAY: 10728 return "PAY"; 10729 case SPEND: 10730 return "SPEND"; 10731 case COINS: 10732 return "COINS"; 10733 case _ACTINVOICEDETAILGENERICMODIFIERCODE: 10734 return "_ActInvoiceDetailGenericModifierCode"; 10735 case AFTHRS: 10736 return "AFTHRS"; 10737 case ISOL: 10738 return "ISOL"; 10739 case OOO: 10740 return "OOO"; 10741 case _ACTINVOICEDETAILGENERICPROVIDERCODE: 10742 return "_ActInvoiceDetailGenericProviderCode"; 10743 case CANCAPT: 10744 return "CANCAPT"; 10745 case DSC: 10746 return "DSC"; 10747 case ESA: 10748 return "ESA"; 10749 case FFSTOP: 10750 return "FFSTOP"; 10751 case FNLFEE: 10752 return "FNLFEE"; 10753 case FRSTFEE: 10754 return "FRSTFEE"; 10755 case MARKUP: 10756 return "MARKUP"; 10757 case MISSAPT: 10758 return "MISSAPT"; 10759 case PERFEE: 10760 return "PERFEE"; 10761 case PERMBNS: 10762 return "PERMBNS"; 10763 case RESTOCK: 10764 return "RESTOCK"; 10765 case TRAVEL: 10766 return "TRAVEL"; 10767 case URGENT: 10768 return "URGENT"; 10769 case _ACTINVOICEDETAILTAXCODE: 10770 return "_ActInvoiceDetailTaxCode"; 10771 case FST: 10772 return "FST"; 10773 case HST: 10774 return "HST"; 10775 case PST: 10776 return "PST"; 10777 case _ACTINVOICEDETAILPREFERREDACCOMMODATIONCODE: 10778 return "_ActInvoiceDetailPreferredAccommodationCode"; 10779 case _ACTENCOUNTERACCOMMODATIONCODE: 10780 return "_ActEncounterAccommodationCode"; 10781 case _HL7ACCOMMODATIONCODE: 10782 return "_HL7AccommodationCode"; 10783 case I: 10784 return "I"; 10785 case P: 10786 return "P"; 10787 case S: 10788 return "S"; 10789 case SP: 10790 return "SP"; 10791 case W: 10792 return "W"; 10793 case _ACTINVOICEDETAILCLINICALSERVICECODE: 10794 return "_ActInvoiceDetailClinicalServiceCode"; 10795 case _ACTINVOICEGROUPCODE: 10796 return "_ActInvoiceGroupCode"; 10797 case _ACTINVOICEINTERGROUPCODE: 10798 return "_ActInvoiceInterGroupCode"; 10799 case CPNDDRGING: 10800 return "CPNDDRGING"; 10801 case CPNDINDING: 10802 return "CPNDINDING"; 10803 case CPNDSUPING: 10804 return "CPNDSUPING"; 10805 case DRUGING: 10806 return "DRUGING"; 10807 case FRAMEING: 10808 return "FRAMEING"; 10809 case LENSING: 10810 return "LENSING"; 10811 case PRDING: 10812 return "PRDING"; 10813 case _ACTINVOICEROOTGROUPCODE: 10814 return "_ActInvoiceRootGroupCode"; 10815 case CPINV: 10816 return "CPINV"; 10817 case CSINV: 10818 return "CSINV"; 10819 case CSPINV: 10820 return "CSPINV"; 10821 case FININV: 10822 return "FININV"; 10823 case OHSINV: 10824 return "OHSINV"; 10825 case PAINV: 10826 return "PAINV"; 10827 case RXCINV: 10828 return "RXCINV"; 10829 case RXDINV: 10830 return "RXDINV"; 10831 case SBFINV: 10832 return "SBFINV"; 10833 case VRXINV: 10834 return "VRXINV"; 10835 case _ACTINVOICEELEMENTSUMMARYCODE: 10836 return "_ActInvoiceElementSummaryCode"; 10837 case _INVOICEELEMENTADJUDICATED: 10838 return "_InvoiceElementAdjudicated"; 10839 case ADNFPPELAT: 10840 return "ADNFPPELAT"; 10841 case ADNFPPELCT: 10842 return "ADNFPPELCT"; 10843 case ADNFPPMNAT: 10844 return "ADNFPPMNAT"; 10845 case ADNFPPMNCT: 10846 return "ADNFPPMNCT"; 10847 case ADNFSPELAT: 10848 return "ADNFSPELAT"; 10849 case ADNFSPELCT: 10850 return "ADNFSPELCT"; 10851 case ADNFSPMNAT: 10852 return "ADNFSPMNAT"; 10853 case ADNFSPMNCT: 10854 return "ADNFSPMNCT"; 10855 case ADNPPPELAT: 10856 return "ADNPPPELAT"; 10857 case ADNPPPELCT: 10858 return "ADNPPPELCT"; 10859 case ADNPPPMNAT: 10860 return "ADNPPPMNAT"; 10861 case ADNPPPMNCT: 10862 return "ADNPPPMNCT"; 10863 case ADNPSPELAT: 10864 return "ADNPSPELAT"; 10865 case ADNPSPELCT: 10866 return "ADNPSPELCT"; 10867 case ADNPSPMNAT: 10868 return "ADNPSPMNAT"; 10869 case ADNPSPMNCT: 10870 return "ADNPSPMNCT"; 10871 case ADPPPPELAT: 10872 return "ADPPPPELAT"; 10873 case ADPPPPELCT: 10874 return "ADPPPPELCT"; 10875 case ADPPPPMNAT: 10876 return "ADPPPPMNAT"; 10877 case ADPPPPMNCT: 10878 return "ADPPPPMNCT"; 10879 case ADPPSPELAT: 10880 return "ADPPSPELAT"; 10881 case ADPPSPELCT: 10882 return "ADPPSPELCT"; 10883 case ADPPSPMNAT: 10884 return "ADPPSPMNAT"; 10885 case ADPPSPMNCT: 10886 return "ADPPSPMNCT"; 10887 case ADRFPPELAT: 10888 return "ADRFPPELAT"; 10889 case ADRFPPELCT: 10890 return "ADRFPPELCT"; 10891 case ADRFPPMNAT: 10892 return "ADRFPPMNAT"; 10893 case ADRFPPMNCT: 10894 return "ADRFPPMNCT"; 10895 case ADRFSPELAT: 10896 return "ADRFSPELAT"; 10897 case ADRFSPELCT: 10898 return "ADRFSPELCT"; 10899 case ADRFSPMNAT: 10900 return "ADRFSPMNAT"; 10901 case ADRFSPMNCT: 10902 return "ADRFSPMNCT"; 10903 case _INVOICEELEMENTPAID: 10904 return "_InvoiceElementPaid"; 10905 case PDNFPPELAT: 10906 return "PDNFPPELAT"; 10907 case PDNFPPELCT: 10908 return "PDNFPPELCT"; 10909 case PDNFPPMNAT: 10910 return "PDNFPPMNAT"; 10911 case PDNFPPMNCT: 10912 return "PDNFPPMNCT"; 10913 case PDNFSPELAT: 10914 return "PDNFSPELAT"; 10915 case PDNFSPELCT: 10916 return "PDNFSPELCT"; 10917 case PDNFSPMNAT: 10918 return "PDNFSPMNAT"; 10919 case PDNFSPMNCT: 10920 return "PDNFSPMNCT"; 10921 case PDNPPPELAT: 10922 return "PDNPPPELAT"; 10923 case PDNPPPELCT: 10924 return "PDNPPPELCT"; 10925 case PDNPPPMNAT: 10926 return "PDNPPPMNAT"; 10927 case PDNPPPMNCT: 10928 return "PDNPPPMNCT"; 10929 case PDNPSPELAT: 10930 return "PDNPSPELAT"; 10931 case PDNPSPELCT: 10932 return "PDNPSPELCT"; 10933 case PDNPSPMNAT: 10934 return "PDNPSPMNAT"; 10935 case PDNPSPMNCT: 10936 return "PDNPSPMNCT"; 10937 case PDPPPPELAT: 10938 return "PDPPPPELAT"; 10939 case PDPPPPELCT: 10940 return "PDPPPPELCT"; 10941 case PDPPPPMNAT: 10942 return "PDPPPPMNAT"; 10943 case PDPPPPMNCT: 10944 return "PDPPPPMNCT"; 10945 case PDPPSPELAT: 10946 return "PDPPSPELAT"; 10947 case PDPPSPELCT: 10948 return "PDPPSPELCT"; 10949 case PDPPSPMNAT: 10950 return "PDPPSPMNAT"; 10951 case PDPPSPMNCT: 10952 return "PDPPSPMNCT"; 10953 case _INVOICEELEMENTSUBMITTED: 10954 return "_InvoiceElementSubmitted"; 10955 case SBBLELAT: 10956 return "SBBLELAT"; 10957 case SBBLELCT: 10958 return "SBBLELCT"; 10959 case SBNFELAT: 10960 return "SBNFELAT"; 10961 case SBNFELCT: 10962 return "SBNFELCT"; 10963 case SBPDELAT: 10964 return "SBPDELAT"; 10965 case SBPDELCT: 10966 return "SBPDELCT"; 10967 case _ACTINVOICEOVERRIDECODE: 10968 return "_ActInvoiceOverrideCode"; 10969 case COVGE: 10970 return "COVGE"; 10971 case EFORM: 10972 return "EFORM"; 10973 case FAX: 10974 return "FAX"; 10975 case GFTH: 10976 return "GFTH"; 10977 case LATE: 10978 return "LATE"; 10979 case MANUAL: 10980 return "MANUAL"; 10981 case OOJ: 10982 return "OOJ"; 10983 case ORTHO: 10984 return "ORTHO"; 10985 case PAPER: 10986 return "PAPER"; 10987 case PIE: 10988 return "PIE"; 10989 case PYRDELAY: 10990 return "PYRDELAY"; 10991 case REFNR: 10992 return "REFNR"; 10993 case REPSERV: 10994 return "REPSERV"; 10995 case UNRELAT: 10996 return "UNRELAT"; 10997 case VERBAUTH: 10998 return "VERBAUTH"; 10999 case _ACTLISTCODE: 11000 return "_ActListCode"; 11001 case _ACTOBSERVATIONLIST: 11002 return "_ActObservationList"; 11003 case CARELIST: 11004 return "CARELIST"; 11005 case CONDLIST: 11006 return "CONDLIST"; 11007 case INTOLIST: 11008 return "INTOLIST"; 11009 case PROBLIST: 11010 return "PROBLIST"; 11011 case RISKLIST: 11012 return "RISKLIST"; 11013 case GOALLIST: 11014 return "GOALLIST"; 11015 case _ACTTHERAPYDURATIONWORKINGLISTCODE: 11016 return "_ActTherapyDurationWorkingListCode"; 11017 case _ACTMEDICATIONTHERAPYDURATIONWORKINGLISTCODE: 11018 return "_ActMedicationTherapyDurationWorkingListCode"; 11019 case ACU: 11020 return "ACU"; 11021 case CHRON: 11022 return "CHRON"; 11023 case ONET: 11024 return "ONET"; 11025 case PRN: 11026 return "PRN"; 11027 case MEDLIST: 11028 return "MEDLIST"; 11029 case CURMEDLIST: 11030 return "CURMEDLIST"; 11031 case DISCMEDLIST: 11032 return "DISCMEDLIST"; 11033 case HISTMEDLIST: 11034 return "HISTMEDLIST"; 11035 case _ACTMONITORINGPROTOCOLCODE: 11036 return "_ActMonitoringProtocolCode"; 11037 case CTLSUB: 11038 return "CTLSUB"; 11039 case INV: 11040 return "INV"; 11041 case LU: 11042 return "LU"; 11043 case OTC: 11044 return "OTC"; 11045 case RX: 11046 return "RX"; 11047 case SA: 11048 return "SA"; 11049 case SAC: 11050 return "SAC"; 11051 case _ACTNONOBSERVATIONINDICATIONCODE: 11052 return "_ActNonObservationIndicationCode"; 11053 case IND01: 11054 return "IND01"; 11055 case IND02: 11056 return "IND02"; 11057 case IND03: 11058 return "IND03"; 11059 case IND04: 11060 return "IND04"; 11061 case IND05: 11062 return "IND05"; 11063 case _ACTOBSERVATIONVERIFICATIONTYPE: 11064 return "_ActObservationVerificationType"; 11065 case VFPAPER: 11066 return "VFPAPER"; 11067 case _ACTPAYMENTCODE: 11068 return "_ActPaymentCode"; 11069 case ACH: 11070 return "ACH"; 11071 case CHK: 11072 return "CHK"; 11073 case DDP: 11074 return "DDP"; 11075 case NON: 11076 return "NON"; 11077 case _ACTPHARMACYSUPPLYTYPE: 11078 return "_ActPharmacySupplyType"; 11079 case DF: 11080 return "DF"; 11081 case EM: 11082 return "EM"; 11083 case SO: 11084 return "SO"; 11085 case FF: 11086 return "FF"; 11087 case FFC: 11088 return "FFC"; 11089 case FFP: 11090 return "FFP"; 11091 case FFSS: 11092 return "FFSS"; 11093 case TF: 11094 return "TF"; 11095 case FS: 11096 return "FS"; 11097 case MS: 11098 return "MS"; 11099 case RF: 11100 return "RF"; 11101 case UD: 11102 return "UD"; 11103 case RFC: 11104 return "RFC"; 11105 case RFCS: 11106 return "RFCS"; 11107 case RFF: 11108 return "RFF"; 11109 case RFFS: 11110 return "RFFS"; 11111 case RFP: 11112 return "RFP"; 11113 case RFPS: 11114 return "RFPS"; 11115 case RFS: 11116 return "RFS"; 11117 case TB: 11118 return "TB"; 11119 case TBS: 11120 return "TBS"; 11121 case UDE: 11122 return "UDE"; 11123 case _ACTPOLICYTYPE: 11124 return "_ActPolicyType"; 11125 case _ACTPRIVACYPOLICY: 11126 return "_ActPrivacyPolicy"; 11127 case _ACTCONSENTDIRECTIVE: 11128 return "_ActConsentDirective"; 11129 case EMRGONLY: 11130 return "EMRGONLY"; 11131 case GRANTORCHOICE: 11132 return "GRANTORCHOICE"; 11133 case IMPLIED: 11134 return "IMPLIED"; 11135 case IMPLIEDD: 11136 return "IMPLIEDD"; 11137 case NOCONSENT: 11138 return "NOCONSENT"; 11139 case NOPP: 11140 return "NOPP"; 11141 case OPTIN: 11142 return "OPTIN"; 11143 case OPTINR: 11144 return "OPTINR"; 11145 case OPTOUT: 11146 return "OPTOUT"; 11147 case OPTOUTE: 11148 return "OPTOUTE"; 11149 case _ACTPRIVACYLAW: 11150 return "_ActPrivacyLaw"; 11151 case _ACTUSPRIVACYLAW: 11152 return "_ActUSPrivacyLaw"; 11153 case _42CFRPART2: 11154 return "42CFRPart2"; 11155 case COMMONRULE: 11156 return "CommonRule"; 11157 case HIPAANOPP: 11158 return "HIPAANOPP"; 11159 case HIPAAPSYNOTES: 11160 return "HIPAAPsyNotes"; 11161 case HIPAASELFPAY: 11162 return "HIPAASelfPay"; 11163 case TITLE38SECTION7332: 11164 return "Title38Section7332"; 11165 case _INFORMATIONSENSITIVITYPOLICY: 11166 return "_InformationSensitivityPolicy"; 11167 case _ACTINFORMATIONSENSITIVITYPOLICY: 11168 return "_ActInformationSensitivityPolicy"; 11169 case ETH: 11170 return "ETH"; 11171 case GDIS: 11172 return "GDIS"; 11173 case HIV: 11174 return "HIV"; 11175 case MST: 11176 return "MST"; 11177 case SCA: 11178 return "SCA"; 11179 case SDV: 11180 return "SDV"; 11181 case SEX: 11182 return "SEX"; 11183 case SPI: 11184 return "SPI"; 11185 case BH: 11186 return "BH"; 11187 case COGN: 11188 return "COGN"; 11189 case DVD: 11190 return "DVD"; 11191 case EMOTDIS: 11192 return "EMOTDIS"; 11193 case MH: 11194 return "MH"; 11195 case PSY: 11196 return "PSY"; 11197 case PSYTHPN: 11198 return "PSYTHPN"; 11199 case SUD: 11200 return "SUD"; 11201 case ETHUD: 11202 return "ETHUD"; 11203 case OPIOIDUD: 11204 return "OPIOIDUD"; 11205 case STD: 11206 return "STD"; 11207 case TBOO: 11208 return "TBOO"; 11209 case VIO: 11210 return "VIO"; 11211 case SICKLE: 11212 return "SICKLE"; 11213 case _ENTITYSENSITIVITYPOLICYTYPE: 11214 return "_EntitySensitivityPolicyType"; 11215 case DEMO: 11216 return "DEMO"; 11217 case DOB: 11218 return "DOB"; 11219 case GENDER: 11220 return "GENDER"; 11221 case LIVARG: 11222 return "LIVARG"; 11223 case MARST: 11224 return "MARST"; 11225 case RACE: 11226 return "RACE"; 11227 case REL: 11228 return "REL"; 11229 case _ROLEINFORMATIONSENSITIVITYPOLICY: 11230 return "_RoleInformationSensitivityPolicy"; 11231 case B: 11232 return "B"; 11233 case EMPL: 11234 return "EMPL"; 11235 case LOCIS: 11236 return "LOCIS"; 11237 case SSP: 11238 return "SSP"; 11239 case ADOL: 11240 return "ADOL"; 11241 case CEL: 11242 return "CEL"; 11243 case DIA: 11244 return "DIA"; 11245 case DRGIS: 11246 return "DRGIS"; 11247 case EMP: 11248 return "EMP"; 11249 case PDS: 11250 return "PDS"; 11251 case PHY: 11252 return "PHY"; 11253 case PRS: 11254 return "PRS"; 11255 case COMPT: 11256 return "COMPT"; 11257 case ACOCOMPT: 11258 return "ACOCOMPT"; 11259 case CTCOMPT: 11260 return "CTCOMPT"; 11261 case FMCOMPT: 11262 return "FMCOMPT"; 11263 case HRCOMPT: 11264 return "HRCOMPT"; 11265 case LRCOMPT: 11266 return "LRCOMPT"; 11267 case PACOMPT: 11268 return "PACOMPT"; 11269 case RESCOMPT: 11270 return "RESCOMPT"; 11271 case RMGTCOMPT: 11272 return "RMGTCOMPT"; 11273 case ACTTRUSTPOLICYTYPE: 11274 return "ActTrustPolicyType"; 11275 case TRSTACCRD: 11276 return "TRSTACCRD"; 11277 case TRSTAGRE: 11278 return "TRSTAGRE"; 11279 case TRSTASSUR: 11280 return "TRSTASSUR"; 11281 case TRSTCERT: 11282 return "TRSTCERT"; 11283 case TRSTFWK: 11284 return "TRSTFWK"; 11285 case TRSTMEC: 11286 return "TRSTMEC"; 11287 case COVPOL: 11288 return "COVPOL"; 11289 case SECURITYPOLICY: 11290 return "SecurityPolicy"; 11291 case AUTHPOL: 11292 return "AUTHPOL"; 11293 case ACCESSCONSCHEME: 11294 return "ACCESSCONSCHEME"; 11295 case DELEPOL: 11296 return "DELEPOL"; 11297 case OBLIGATIONPOLICY: 11298 return "ObligationPolicy"; 11299 case ANONY: 11300 return "ANONY"; 11301 case AOD: 11302 return "AOD"; 11303 case AUDIT: 11304 return "AUDIT"; 11305 case AUDTR: 11306 return "AUDTR"; 11307 case CPLYCC: 11308 return "CPLYCC"; 11309 case CPLYCD: 11310 return "CPLYCD"; 11311 case CPLYJPP: 11312 return "CPLYJPP"; 11313 case CPLYOPP: 11314 return "CPLYOPP"; 11315 case CPLYOSP: 11316 return "CPLYOSP"; 11317 case CPLYPOL: 11318 return "CPLYPOL"; 11319 case DECLASSIFYLABEL: 11320 return "DECLASSIFYLABEL"; 11321 case DEID: 11322 return "DEID"; 11323 case DELAU: 11324 return "DELAU"; 11325 case DOWNGRDLABEL: 11326 return "DOWNGRDLABEL"; 11327 case DRIVLABEL: 11328 return "DRIVLABEL"; 11329 case ENCRYPT: 11330 return "ENCRYPT"; 11331 case ENCRYPTR: 11332 return "ENCRYPTR"; 11333 case ENCRYPTT: 11334 return "ENCRYPTT"; 11335 case ENCRYPTU: 11336 return "ENCRYPTU"; 11337 case HUAPRV: 11338 return "HUAPRV"; 11339 case LABEL: 11340 return "LABEL"; 11341 case MASK: 11342 return "MASK"; 11343 case MINEC: 11344 return "MINEC"; 11345 case PERSISTLABEL: 11346 return "PERSISTLABEL"; 11347 case PRIVMARK: 11348 return "PRIVMARK"; 11349 case PSEUD: 11350 return "PSEUD"; 11351 case REDACT: 11352 return "REDACT"; 11353 case UPGRDLABEL: 11354 return "UPGRDLABEL"; 11355 case REFRAINPOLICY: 11356 return "RefrainPolicy"; 11357 case NOAUTH: 11358 return "NOAUTH"; 11359 case NOCOLLECT: 11360 return "NOCOLLECT"; 11361 case NODSCLCD: 11362 return "NODSCLCD"; 11363 case NODSCLCDS: 11364 return "NODSCLCDS"; 11365 case NOINTEGRATE: 11366 return "NOINTEGRATE"; 11367 case NOLIST: 11368 return "NOLIST"; 11369 case NOMOU: 11370 return "NOMOU"; 11371 case NOORGPOL: 11372 return "NOORGPOL"; 11373 case NOPAT: 11374 return "NOPAT"; 11375 case NOPERSISTP: 11376 return "NOPERSISTP"; 11377 case NORDSCLCD: 11378 return "NORDSCLCD"; 11379 case NORDSCLCDS: 11380 return "NORDSCLCDS"; 11381 case NORDSCLW: 11382 return "NORDSCLW"; 11383 case NORELINK: 11384 return "NORELINK"; 11385 case NOREUSE: 11386 return "NOREUSE"; 11387 case NOVIP: 11388 return "NOVIP"; 11389 case ORCON: 11390 return "ORCON"; 11391 case _ACTPRODUCTACQUISITIONCODE: 11392 return "_ActProductAcquisitionCode"; 11393 case LOAN: 11394 return "LOAN"; 11395 case RENT: 11396 return "RENT"; 11397 case TRANSFER: 11398 return "TRANSFER"; 11399 case SALE: 11400 return "SALE"; 11401 case _ACTSPECIMENTRANSPORTCODE: 11402 return "_ActSpecimenTransportCode"; 11403 case SREC: 11404 return "SREC"; 11405 case SSTOR: 11406 return "SSTOR"; 11407 case STRAN: 11408 return "STRAN"; 11409 case _ACTSPECIMENTREATMENTCODE: 11410 return "_ActSpecimenTreatmentCode"; 11411 case ACID: 11412 return "ACID"; 11413 case ALK: 11414 return "ALK"; 11415 case DEFB: 11416 return "DEFB"; 11417 case FILT: 11418 return "FILT"; 11419 case LDLP: 11420 return "LDLP"; 11421 case NEUT: 11422 return "NEUT"; 11423 case RECA: 11424 return "RECA"; 11425 case UFIL: 11426 return "UFIL"; 11427 case _ACTSUBSTANCEADMINISTRATIONCODE: 11428 return "_ActSubstanceAdministrationCode"; 11429 case DRUG: 11430 return "DRUG"; 11431 case FD: 11432 return "FD"; 11433 case IMMUNIZ: 11434 return "IMMUNIZ"; 11435 case BOOSTER: 11436 return "BOOSTER"; 11437 case INITIMMUNIZ: 11438 return "INITIMMUNIZ"; 11439 case _ACTTASKCODE: 11440 return "_ActTaskCode"; 11441 case OE: 11442 return "OE"; 11443 case LABOE: 11444 return "LABOE"; 11445 case MEDOE: 11446 return "MEDOE"; 11447 case PATDOC: 11448 return "PATDOC"; 11449 case ALLERLREV: 11450 return "ALLERLREV"; 11451 case CLINNOTEE: 11452 return "CLINNOTEE"; 11453 case DIAGLISTE: 11454 return "DIAGLISTE"; 11455 case DISCHINSTE: 11456 return "DISCHINSTE"; 11457 case DISCHSUME: 11458 return "DISCHSUME"; 11459 case PATEDUE: 11460 return "PATEDUE"; 11461 case PATREPE: 11462 return "PATREPE"; 11463 case PROBLISTE: 11464 return "PROBLISTE"; 11465 case RADREPE: 11466 return "RADREPE"; 11467 case IMMLREV: 11468 return "IMMLREV"; 11469 case REMLREV: 11470 return "REMLREV"; 11471 case WELLREMLREV: 11472 return "WELLREMLREV"; 11473 case PATINFO: 11474 return "PATINFO"; 11475 case ALLERLE: 11476 return "ALLERLE"; 11477 case CDSREV: 11478 return "CDSREV"; 11479 case CLINNOTEREV: 11480 return "CLINNOTEREV"; 11481 case DISCHSUMREV: 11482 return "DISCHSUMREV"; 11483 case DIAGLISTREV: 11484 return "DIAGLISTREV"; 11485 case IMMLE: 11486 return "IMMLE"; 11487 case LABRREV: 11488 return "LABRREV"; 11489 case MICRORREV: 11490 return "MICRORREV"; 11491 case MICROORGRREV: 11492 return "MICROORGRREV"; 11493 case MICROSENSRREV: 11494 return "MICROSENSRREV"; 11495 case MLREV: 11496 return "MLREV"; 11497 case MARWLREV: 11498 return "MARWLREV"; 11499 case OREV: 11500 return "OREV"; 11501 case PATREPREV: 11502 return "PATREPREV"; 11503 case PROBLISTREV: 11504 return "PROBLISTREV"; 11505 case RADREPREV: 11506 return "RADREPREV"; 11507 case REMLE: 11508 return "REMLE"; 11509 case WELLREMLE: 11510 return "WELLREMLE"; 11511 case RISKASSESS: 11512 return "RISKASSESS"; 11513 case FALLRISK: 11514 return "FALLRISK"; 11515 case _ACTTRANSPORTATIONMODECODE: 11516 return "_ActTransportationModeCode"; 11517 case _ACTPATIENTTRANSPORTATIONMODECODE: 11518 return "_ActPatientTransportationModeCode"; 11519 case AFOOT: 11520 return "AFOOT"; 11521 case AMBT: 11522 return "AMBT"; 11523 case AMBAIR: 11524 return "AMBAIR"; 11525 case AMBGRND: 11526 return "AMBGRND"; 11527 case AMBHELO: 11528 return "AMBHELO"; 11529 case LAWENF: 11530 return "LAWENF"; 11531 case PRVTRN: 11532 return "PRVTRN"; 11533 case PUBTRN: 11534 return "PUBTRN"; 11535 case _OBSERVATIONTYPE: 11536 return "_ObservationType"; 11537 case _ACTSPECOBSCODE: 11538 return "_ActSpecObsCode"; 11539 case ARTBLD: 11540 return "ARTBLD"; 11541 case DILUTION: 11542 return "DILUTION"; 11543 case AUTOHIGH: 11544 return "AUTO-HIGH"; 11545 case AUTOLOW: 11546 return "AUTO-LOW"; 11547 case PRE: 11548 return "PRE"; 11549 case RERUN: 11550 return "RERUN"; 11551 case EVNFCTS: 11552 return "EVNFCTS"; 11553 case INTFR: 11554 return "INTFR"; 11555 case FIBRIN: 11556 return "FIBRIN"; 11557 case HEMOLYSIS: 11558 return "HEMOLYSIS"; 11559 case ICTERUS: 11560 return "ICTERUS"; 11561 case LIPEMIA: 11562 return "LIPEMIA"; 11563 case VOLUME: 11564 return "VOLUME"; 11565 case AVAILABLE: 11566 return "AVAILABLE"; 11567 case CONSUMPTION: 11568 return "CONSUMPTION"; 11569 case CURRENT: 11570 return "CURRENT"; 11571 case INITIAL: 11572 return "INITIAL"; 11573 case _ANNOTATIONTYPE: 11574 return "_AnnotationType"; 11575 case _ACTPATIENTANNOTATIONTYPE: 11576 return "_ActPatientAnnotationType"; 11577 case ANNDI: 11578 return "ANNDI"; 11579 case ANNGEN: 11580 return "ANNGEN"; 11581 case ANNIMM: 11582 return "ANNIMM"; 11583 case ANNLAB: 11584 return "ANNLAB"; 11585 case ANNMED: 11586 return "ANNMED"; 11587 case _GENETICOBSERVATIONTYPE: 11588 return "_GeneticObservationType"; 11589 case GENE: 11590 return "GENE"; 11591 case _IMMUNIZATIONOBSERVATIONTYPE: 11592 return "_ImmunizationObservationType"; 11593 case OBSANTC: 11594 return "OBSANTC"; 11595 case OBSANTV: 11596 return "OBSANTV"; 11597 case _INDIVIDUALCASESAFETYREPORTTYPE: 11598 return "_IndividualCaseSafetyReportType"; 11599 case PATADVEVNT: 11600 return "PAT_ADV_EVNT"; 11601 case VACPROBLEM: 11602 return "VAC_PROBLEM"; 11603 case _LOINCOBSERVATIONACTCONTEXTAGETYPE: 11604 return "_LOINCObservationActContextAgeType"; 11605 case _216119: 11606 return "21611-9"; 11607 case _216127: 11608 return "21612-7"; 11609 case _295535: 11610 return "29553-5"; 11611 case _305250: 11612 return "30525-0"; 11613 case _309724: 11614 return "30972-4"; 11615 case _MEDICATIONOBSERVATIONTYPE: 11616 return "_MedicationObservationType"; 11617 case REPHALFLIFE: 11618 return "REP_HALF_LIFE"; 11619 case SPLCOATING: 11620 return "SPLCOATING"; 11621 case SPLCOLOR: 11622 return "SPLCOLOR"; 11623 case SPLIMAGE: 11624 return "SPLIMAGE"; 11625 case SPLIMPRINT: 11626 return "SPLIMPRINT"; 11627 case SPLSCORING: 11628 return "SPLSCORING"; 11629 case SPLSHAPE: 11630 return "SPLSHAPE"; 11631 case SPLSIZE: 11632 return "SPLSIZE"; 11633 case SPLSYMBOL: 11634 return "SPLSYMBOL"; 11635 case _OBSERVATIONISSUETRIGGERCODEDOBSERVATIONTYPE: 11636 return "_ObservationIssueTriggerCodedObservationType"; 11637 case _CASETRANSMISSIONMODE: 11638 return "_CaseTransmissionMode"; 11639 case AIRTRNS: 11640 return "AIRTRNS"; 11641 case ANANTRNS: 11642 return "ANANTRNS"; 11643 case ANHUMTRNS: 11644 return "ANHUMTRNS"; 11645 case BDYFLDTRNS: 11646 return "BDYFLDTRNS"; 11647 case BLDTRNS: 11648 return "BLDTRNS"; 11649 case DERMTRNS: 11650 return "DERMTRNS"; 11651 case ENVTRNS: 11652 return "ENVTRNS"; 11653 case FECTRNS: 11654 return "FECTRNS"; 11655 case FOMTRNS: 11656 return "FOMTRNS"; 11657 case FOODTRNS: 11658 return "FOODTRNS"; 11659 case HUMHUMTRNS: 11660 return "HUMHUMTRNS"; 11661 case INDTRNS: 11662 return "INDTRNS"; 11663 case LACTTRNS: 11664 return "LACTTRNS"; 11665 case NOSTRNS: 11666 return "NOSTRNS"; 11667 case PARTRNS: 11668 return "PARTRNS"; 11669 case PLACTRNS: 11670 return "PLACTRNS"; 11671 case SEXTRNS: 11672 return "SEXTRNS"; 11673 case TRNSFTRNS: 11674 return "TRNSFTRNS"; 11675 case VECTRNS: 11676 return "VECTRNS"; 11677 case WATTRNS: 11678 return "WATTRNS"; 11679 case _OBSERVATIONQUALITYMEASUREATTRIBUTE: 11680 return "_ObservationQualityMeasureAttribute"; 11681 case AGGREGATE: 11682 return "AGGREGATE"; 11683 case CMPMSRMTH: 11684 return "CMPMSRMTH"; 11685 case CMPMSRSCRWGHT: 11686 return "CMPMSRSCRWGHT"; 11687 case COPY: 11688 return "COPY"; 11689 case CRS: 11690 return "CRS"; 11691 case DEF: 11692 return "DEF"; 11693 case DISC: 11694 return "DISC"; 11695 case FINALDT: 11696 return "FINALDT"; 11697 case GUIDE: 11698 return "GUIDE"; 11699 case IDUR: 11700 return "IDUR"; 11701 case ITMCNT: 11702 return "ITMCNT"; 11703 case KEY: 11704 return "KEY"; 11705 case MEDT: 11706 return "MEDT"; 11707 case MSD: 11708 return "MSD"; 11709 case MSRADJ: 11710 return "MSRADJ"; 11711 case MSRAGG: 11712 return "MSRAGG"; 11713 case MSRIMPROV: 11714 return "MSRIMPROV"; 11715 case MSRJUR: 11716 return "MSRJUR"; 11717 case MSRRPTR: 11718 return "MSRRPTR"; 11719 case MSRRPTTIME: 11720 return "MSRRPTTIME"; 11721 case MSRSCORE: 11722 return "MSRSCORE"; 11723 case MSRSET: 11724 return "MSRSET"; 11725 case MSRTOPIC: 11726 return "MSRTOPIC"; 11727 case MSRTP: 11728 return "MSRTP"; 11729 case MSRTYPE: 11730 return "MSRTYPE"; 11731 case RAT: 11732 return "RAT"; 11733 case REF: 11734 return "REF"; 11735 case SDE: 11736 return "SDE"; 11737 case STRAT: 11738 return "STRAT"; 11739 case TRANF: 11740 return "TRANF"; 11741 case USE: 11742 return "USE"; 11743 case _OBSERVATIONSEQUENCETYPE: 11744 return "_ObservationSequenceType"; 11745 case TIMEABSOLUTE: 11746 return "TIME_ABSOLUTE"; 11747 case TIMERELATIVE: 11748 return "TIME_RELATIVE"; 11749 case _OBSERVATIONSERIESTYPE: 11750 return "_ObservationSeriesType"; 11751 case _ECGOBSERVATIONSERIESTYPE: 11752 return "_ECGObservationSeriesType"; 11753 case REPRESENTATIVEBEAT: 11754 return "REPRESENTATIVE_BEAT"; 11755 case RHYTHM: 11756 return "RHYTHM"; 11757 case _PATIENTIMMUNIZATIONRELATEDOBSERVATIONTYPE: 11758 return "_PatientImmunizationRelatedObservationType"; 11759 case CLSSRM: 11760 return "CLSSRM"; 11761 case GRADE: 11762 return "GRADE"; 11763 case SCHL: 11764 return "SCHL"; 11765 case SCHLDIV: 11766 return "SCHLDIV"; 11767 case TEACHER: 11768 return "TEACHER"; 11769 case _POPULATIONINCLUSIONOBSERVATIONTYPE: 11770 return "_PopulationInclusionObservationType"; 11771 case DENEX: 11772 return "DENEX"; 11773 case DENEXCEP: 11774 return "DENEXCEP"; 11775 case DENOM: 11776 return "DENOM"; 11777 case IPOP: 11778 return "IPOP"; 11779 case IPPOP: 11780 return "IPPOP"; 11781 case MSROBS: 11782 return "MSROBS"; 11783 case MSRPOPL: 11784 return "MSRPOPL"; 11785 case MSRPOPLEX: 11786 return "MSRPOPLEX"; 11787 case NUMER: 11788 return "NUMER"; 11789 case NUMEX: 11790 return "NUMEX"; 11791 case _PREFERENCEOBSERVATIONTYPE: 11792 return "_PreferenceObservationType"; 11793 case PREFSTRENGTH: 11794 return "PREFSTRENGTH"; 11795 case ADVERSEREACTION: 11796 return "ADVERSE_REACTION"; 11797 case ASSERTION: 11798 return "ASSERTION"; 11799 case CASESER: 11800 return "CASESER"; 11801 case CDIO: 11802 return "CDIO"; 11803 case CRIT: 11804 return "CRIT"; 11805 case CTMO: 11806 return "CTMO"; 11807 case DX: 11808 return "DX"; 11809 case ADMDX: 11810 return "ADMDX"; 11811 case DISDX: 11812 return "DISDX"; 11813 case INTDX: 11814 return "INTDX"; 11815 case NOI: 11816 return "NOI"; 11817 case GISTIER: 11818 return "GISTIER"; 11819 case HHOBS: 11820 return "HHOBS"; 11821 case ISSUE: 11822 return "ISSUE"; 11823 case _ACTADMINISTRATIVEDETECTEDISSUECODE: 11824 return "_ActAdministrativeDetectedIssueCode"; 11825 case _ACTADMINISTRATIVEAUTHORIZATIONDETECTEDISSUECODE: 11826 return "_ActAdministrativeAuthorizationDetectedIssueCode"; 11827 case NAT: 11828 return "NAT"; 11829 case SUPPRESSED: 11830 return "SUPPRESSED"; 11831 case VALIDAT: 11832 return "VALIDAT"; 11833 case KEY204: 11834 return "KEY204"; 11835 case KEY205: 11836 return "KEY205"; 11837 case COMPLY: 11838 return "COMPLY"; 11839 case DUPTHPY: 11840 return "DUPTHPY"; 11841 case DUPTHPCLS: 11842 return "DUPTHPCLS"; 11843 case DUPTHPGEN: 11844 return "DUPTHPGEN"; 11845 case ABUSE: 11846 return "ABUSE"; 11847 case FRAUD: 11848 return "FRAUD"; 11849 case PLYDOC: 11850 return "PLYDOC"; 11851 case PLYPHRM: 11852 return "PLYPHRM"; 11853 case DOSE: 11854 return "DOSE"; 11855 case DOSECOND: 11856 return "DOSECOND"; 11857 case DOSEDUR: 11858 return "DOSEDUR"; 11859 case DOSEDURH: 11860 return "DOSEDURH"; 11861 case DOSEDURHIND: 11862 return "DOSEDURHIND"; 11863 case DOSEDURL: 11864 return "DOSEDURL"; 11865 case DOSEDURLIND: 11866 return "DOSEDURLIND"; 11867 case DOSEH: 11868 return "DOSEH"; 11869 case DOSEHINDA: 11870 return "DOSEHINDA"; 11871 case DOSEHIND: 11872 return "DOSEHIND"; 11873 case DOSEHINDSA: 11874 return "DOSEHINDSA"; 11875 case DOSEHINDW: 11876 return "DOSEHINDW"; 11877 case DOSEIVL: 11878 return "DOSEIVL"; 11879 case DOSEIVLIND: 11880 return "DOSEIVLIND"; 11881 case DOSEL: 11882 return "DOSEL"; 11883 case DOSELINDA: 11884 return "DOSELINDA"; 11885 case DOSELIND: 11886 return "DOSELIND"; 11887 case DOSELINDSA: 11888 return "DOSELINDSA"; 11889 case DOSELINDW: 11890 return "DOSELINDW"; 11891 case MDOSE: 11892 return "MDOSE"; 11893 case OBSA: 11894 return "OBSA"; 11895 case AGE: 11896 return "AGE"; 11897 case ADALRT: 11898 return "ADALRT"; 11899 case GEALRT: 11900 return "GEALRT"; 11901 case PEALRT: 11902 return "PEALRT"; 11903 case COND: 11904 return "COND"; 11905 case HGHT: 11906 return "HGHT"; 11907 case LACT: 11908 return "LACT"; 11909 case PREG: 11910 return "PREG"; 11911 case WGHT: 11912 return "WGHT"; 11913 case CREACT: 11914 return "CREACT"; 11915 case GEN: 11916 return "GEN"; 11917 case GEND: 11918 return "GEND"; 11919 case LAB: 11920 return "LAB"; 11921 case REACT: 11922 return "REACT"; 11923 case ALGY: 11924 return "ALGY"; 11925 case INT: 11926 return "INT"; 11927 case RREACT: 11928 return "RREACT"; 11929 case RALG: 11930 return "RALG"; 11931 case RAR: 11932 return "RAR"; 11933 case RINT: 11934 return "RINT"; 11935 case BUS: 11936 return "BUS"; 11937 case CODEINVAL: 11938 return "CODE_INVAL"; 11939 case CODEDEPREC: 11940 return "CODE_DEPREC"; 11941 case FORMAT: 11942 return "FORMAT"; 11943 case ILLEGAL: 11944 return "ILLEGAL"; 11945 case LENRANGE: 11946 return "LEN_RANGE"; 11947 case LENLONG: 11948 return "LEN_LONG"; 11949 case LENSHORT: 11950 return "LEN_SHORT"; 11951 case MISSCOND: 11952 return "MISSCOND"; 11953 case MISSMAND: 11954 return "MISSMAND"; 11955 case NODUPS: 11956 return "NODUPS"; 11957 case NOPERSIST: 11958 return "NOPERSIST"; 11959 case REPRANGE: 11960 return "REP_RANGE"; 11961 case MAXOCCURS: 11962 return "MAXOCCURS"; 11963 case MINOCCURS: 11964 return "MINOCCURS"; 11965 case _ACTADMINISTRATIVERULEDETECTEDISSUECODE: 11966 return "_ActAdministrativeRuleDetectedIssueCode"; 11967 case KEY206: 11968 return "KEY206"; 11969 case OBSOLETE: 11970 return "OBSOLETE"; 11971 case _ACTSUPPLIEDITEMDETECTEDISSUECODE: 11972 return "_ActSuppliedItemDetectedIssueCode"; 11973 case _ADMINISTRATIONDETECTEDISSUECODE: 11974 return "_AdministrationDetectedIssueCode"; 11975 case _APPROPRIATENESSDETECTEDISSUECODE: 11976 return "_AppropriatenessDetectedIssueCode"; 11977 case _INTERACTIONDETECTEDISSUECODE: 11978 return "_InteractionDetectedIssueCode"; 11979 case FOOD: 11980 return "FOOD"; 11981 case TPROD: 11982 return "TPROD"; 11983 case DRG: 11984 return "DRG"; 11985 case NHP: 11986 return "NHP"; 11987 case NONRX: 11988 return "NONRX"; 11989 case PREVINEF: 11990 return "PREVINEF"; 11991 case DACT: 11992 return "DACT"; 11993 case TIME: 11994 return "TIME"; 11995 case ALRTENDLATE: 11996 return "ALRTENDLATE"; 11997 case ALRTSTRTLATE: 11998 return "ALRTSTRTLATE"; 11999 case _TIMINGDETECTEDISSUECODE: 12000 return "_TimingDetectedIssueCode"; 12001 case ENDLATE: 12002 return "ENDLATE"; 12003 case STRTLATE: 12004 return "STRTLATE"; 12005 case _SUPPLYDETECTEDISSUECODE: 12006 return "_SupplyDetectedIssueCode"; 12007 case ALLDONE: 12008 return "ALLDONE"; 12009 case FULFIL: 12010 return "FULFIL"; 12011 case NOTACTN: 12012 return "NOTACTN"; 12013 case NOTEQUIV: 12014 return "NOTEQUIV"; 12015 case NOTEQUIVGEN: 12016 return "NOTEQUIVGEN"; 12017 case NOTEQUIVTHER: 12018 return "NOTEQUIVTHER"; 12019 case TIMING: 12020 return "TIMING"; 12021 case INTERVAL: 12022 return "INTERVAL"; 12023 case MINFREQ: 12024 return "MINFREQ"; 12025 case HELD: 12026 return "HELD"; 12027 case TOOLATE: 12028 return "TOOLATE"; 12029 case TOOSOON: 12030 return "TOOSOON"; 12031 case HISTORIC: 12032 return "HISTORIC"; 12033 case PATPREF: 12034 return "PATPREF"; 12035 case PATPREFALT: 12036 return "PATPREFALT"; 12037 case KSUBJ: 12038 return "KSUBJ"; 12039 case KSUBT: 12040 return "KSUBT"; 12041 case OINT: 12042 return "OINT"; 12043 case ALG: 12044 return "ALG"; 12045 case DALG: 12046 return "DALG"; 12047 case EALG: 12048 return "EALG"; 12049 case FALG: 12050 return "FALG"; 12051 case DINT: 12052 return "DINT"; 12053 case DNAINT: 12054 return "DNAINT"; 12055 case EINT: 12056 return "EINT"; 12057 case ENAINT: 12058 return "ENAINT"; 12059 case FINT: 12060 return "FINT"; 12061 case FNAINT: 12062 return "FNAINT"; 12063 case NAINT: 12064 return "NAINT"; 12065 case SEV: 12066 return "SEV"; 12067 case _FDALABELDATA: 12068 return "_FDALabelData"; 12069 case FDACOATING: 12070 return "FDACOATING"; 12071 case FDACOLOR: 12072 return "FDACOLOR"; 12073 case FDAIMPRINTCD: 12074 return "FDAIMPRINTCD"; 12075 case FDALOGO: 12076 return "FDALOGO"; 12077 case FDASCORING: 12078 return "FDASCORING"; 12079 case FDASHAPE: 12080 return "FDASHAPE"; 12081 case FDASIZE: 12082 return "FDASIZE"; 12083 case _ROIOVERLAYSHAPE: 12084 return "_ROIOverlayShape"; 12085 case CIRCLE: 12086 return "CIRCLE"; 12087 case ELLIPSE: 12088 return "ELLIPSE"; 12089 case POINT: 12090 return "POINT"; 12091 case POLY: 12092 return "POLY"; 12093 case C: 12094 return "C"; 12095 case DIET: 12096 return "DIET"; 12097 case BR: 12098 return "BR"; 12099 case DM: 12100 return "DM"; 12101 case FAST: 12102 return "FAST"; 12103 case FORMULA: 12104 return "FORMULA"; 12105 case GF: 12106 return "GF"; 12107 case LF: 12108 return "LF"; 12109 case LP: 12110 return "LP"; 12111 case LQ: 12112 return "LQ"; 12113 case LS: 12114 return "LS"; 12115 case N: 12116 return "N"; 12117 case NF: 12118 return "NF"; 12119 case PAF: 12120 return "PAF"; 12121 case PAR: 12122 return "PAR"; 12123 case RD: 12124 return "RD"; 12125 case SCH: 12126 return "SCH"; 12127 case SUPPLEMENT: 12128 return "SUPPLEMENT"; 12129 case T: 12130 return "T"; 12131 case VLI: 12132 return "VLI"; 12133 case DRUGPRG: 12134 return "DRUGPRG"; 12135 case F: 12136 return "F"; 12137 case PRLMN: 12138 return "PRLMN"; 12139 case SECOBS: 12140 return "SECOBS"; 12141 case SECCATOBS: 12142 return "SECCATOBS"; 12143 case SECCLASSOBS: 12144 return "SECCLASSOBS"; 12145 case SECCONOBS: 12146 return "SECCONOBS"; 12147 case SECINTOBS: 12148 return "SECINTOBS"; 12149 case SECALTINTOBS: 12150 return "SECALTINTOBS"; 12151 case SECDATINTOBS: 12152 return "SECDATINTOBS"; 12153 case SECINTCONOBS: 12154 return "SECINTCONOBS"; 12155 case SECINTPRVOBS: 12156 return "SECINTPRVOBS"; 12157 case SECINTPRVABOBS: 12158 return "SECINTPRVABOBS"; 12159 case SECINTPRVRBOBS: 12160 return "SECINTPRVRBOBS"; 12161 case SECINTSTOBS: 12162 return "SECINTSTOBS"; 12163 case SECTRSTOBS: 12164 return "SECTRSTOBS"; 12165 case TRSTACCRDOBS: 12166 return "TRSTACCRDOBS"; 12167 case TRSTAGREOBS: 12168 return "TRSTAGREOBS"; 12169 case TRSTCERTOBS: 12170 return "TRSTCERTOBS"; 12171 case TRSTFWKOBS: 12172 return "TRSTFWKOBS"; 12173 case TRSTLOAOBS: 12174 return "TRSTLOAOBS"; 12175 case TRSTMECOBS: 12176 return "TRSTMECOBS"; 12177 case SUBSIDFFS: 12178 return "SUBSIDFFS"; 12179 case WRKCOMP: 12180 return "WRKCOMP"; 12181 case _ACTPROCEDURECODE: 12182 return "_ActProcedureCode"; 12183 case _ACTBILLABLESERVICECODE: 12184 return "_ActBillableServiceCode"; 12185 case _HL7DEFINEDACTCODES: 12186 return "_HL7DefinedActCodes"; 12187 case COPAY: 12188 return "COPAY"; 12189 case DEDUCT: 12190 return "DEDUCT"; 12191 case DOSEIND: 12192 return "DOSEIND"; 12193 case PRA: 12194 return "PRA"; 12195 case STORE: 12196 return "STORE"; 12197 case NULL: 12198 return null; 12199 default: 12200 return "?"; 12201 } 12202 } 12203 12204 public String getSystem() { 12205 return "http://terminology.hl7.org/CodeSystem/v3-ActCode"; 12206 } 12207 12208 public String getDefinition() { 12209 switch (this) { 12210 case _ACTACCOUNTCODE: 12211 return "An account represents a grouping of financial transactions that are tracked and reported together with a single balance. Examples of account codes (types) are Patient billing accounts (collection of charges), Cost centers; Cash."; 12212 case ACCTRECEIVABLE: 12213 return "An account for collecting charges, reversals, adjustments and payments, including deductibles, copayments, coinsurance (financial transactions) credited or debited to the account receivable account for a patient's encounter."; 12214 case CASH: 12215 return "Cash"; 12216 case CC: 12217 return "Description: Types of advance payment to be made on a plastic card usually issued by a financial institution used of purchasing services and/or products."; 12218 case AE: 12219 return "American Express"; 12220 case DN: 12221 return "Diner's Club"; 12222 case DV: 12223 return "Discover Card"; 12224 case MC: 12225 return "Master Card"; 12226 case V: 12227 return "Visa"; 12228 case PBILLACCT: 12229 return "An account representing charges and credits (financial transactions) for a patient's encounter."; 12230 case _ACTADJUDICATIONCODE: 12231 return "Includes coded responses that will occur as a result of the adjudication of an electronic invoice at a summary level and provides guidance on interpretation of the referenced adjudication results."; 12232 case _ACTADJUDICATIONGROUPCODE: 12233 return "Catagorization of grouping criteria for the associated transactions and/or summary (totals, subtotals)."; 12234 case CONT: 12235 return "Transaction counts and value totals by Contract Identifier."; 12236 case DAY: 12237 return "Transaction counts and value totals for each calendar day within the date range specified."; 12238 case LOC: 12239 return "Transaction counts and value totals by service location (e.g clinic)."; 12240 case MONTH: 12241 return "Transaction counts and value totals for each calendar month within the date range specified."; 12242 case PERIOD: 12243 return "Transaction counts and value totals for the date range specified."; 12244 case PROV: 12245 return "Transaction counts and value totals by Provider Identifier."; 12246 case WEEK: 12247 return "Transaction counts and value totals for each calendar week within the date range specified."; 12248 case YEAR: 12249 return "Transaction counts and value totals for each calendar year within the date range specified."; 12250 case AA: 12251 return "The invoice element has been accepted for payment but one or more adjustment(s) have been made to one or more invoice element line items (component charges). \r\n\n Also includes the concept 'Adjudicate as zero' and items not covered under a particular Policy. \r\n\n Invoice element can be reversed (nullified). \r\n\n Recommend that the invoice element is saved for DUR (Drug Utilization Reporting)."; 12252 case ANF: 12253 return "The invoice element has been accepted for payment but one or more adjustment(s) have been made to one or more invoice element line items (component charges) without changing the amount. \r\n\n Invoice element can be reversed (nullified). \r\n\n Recommend that the invoice element is saved for DUR (Drug Utilization Reporting)."; 12254 case AR: 12255 return "The invoice element has passed through the adjudication process but payment is refused due to one or more reasons.\r\n\n Includes items such as patient not covered, or invoice element is not constructed according to payer rules (e.g. 'invoice submitted too late').\r\n\n If one invoice element line item in the invoice element structure is rejected, the remaining line items may not be adjudicated and the complete group is treated as rejected.\r\n\n A refused invoice element can be forwarded to the next payer (for Coordination of Benefits) or modified and resubmitted to refusing payer.\r\n\n Invoice element cannot be reversed (nullified) as there is nothing to reverse. \r\n\n Recommend that the invoice element is not saved for DUR (Drug Utilization Reporting)."; 12256 case AS: 12257 return "The invoice element was/will be paid exactly as submitted, without financial adjustment(s).\r\n\n If the dollar amount stays the same, but the billing codes have been amended or financial adjustments have been applied through the adjudication process, the invoice element is treated as \"Adjudicated with Adjustment\".\r\n\n If information items are included in the adjudication results that do not affect the monetary amounts paid, then this is still Adjudicated as Submitted (e.g. 'reached Plan Maximum on this Claim'). \r\n\n Invoice element can be reversed (nullified). \r\n\n Recommend that the invoice element is saved for DUR (Drug Utilization Reporting)."; 12258 case _ACTADJUDICATIONRESULTACTIONCODE: 12259 return "Actions to be carried out by the recipient of the Adjudication Result information."; 12260 case DISPLAY: 12261 return "The adjudication result associated is to be displayed to the receiver of the adjudication result."; 12262 case FORM: 12263 return "The adjudication result associated is to be printed on the specified form, which is then provided to the covered party."; 12264 case _ACTBILLABLEMODIFIERCODE: 12265 return "Definition:An identifying modifier code for healthcare interventions or procedures."; 12266 case CPTM: 12267 return "Description:CPT modifier codes are found in Appendix A of CPT 2000 Standard Edition."; 12268 case HCPCSA: 12269 return "Description:HCPCS Level II (HCFA-assigned) and Carrier-assigned (Level III) modifiers are reported in Appendix A of CPT 2000 Standard Edition and in the Medicare Bulletin."; 12270 case _ACTBILLINGARRANGEMENTCODE: 12271 return "The type of provision(s) made for reimbursing for the deliver of healthcare services and/or goods provided by a Provider, over a specified period."; 12272 case BLK: 12273 return "A billing arrangement where a Provider charges a lump sum to provide a prescribed group (volume) of services to a single patient which occur over a period of time. Services included in the block may vary. \r\n\n This billing arrangement is also known as Program of Care for some specific Payors and Program Fees for other Payors."; 12274 case CAP: 12275 return "A billing arrangement where the payment made to a Provider is determined by analyzing one or more demographic attributes about the persons/patients who are enrolled with the Provider (in their practice)."; 12276 case CONTF: 12277 return "A billing arrangement where a Provider charges a lump sum to provide a particular volume of one or more interventions/procedures or groups of interventions/procedures."; 12278 case FINBILL: 12279 return "A billing arrangement where a Provider charges for non-clinical items. This includes interest in arrears, mileage, etc. Clinical content is not included in Invoices submitted with this type of billing arrangement."; 12280 case ROST: 12281 return "A billing arrangement where funding is based on a list of individuals registered as patients of the Provider."; 12282 case SESS: 12283 return "A billing arrangement where a Provider charges a sum to provide a group (volume) of interventions/procedures to one or more patients within a defined period of time, typically on the same date. Interventions/procedures included in the session may vary."; 12284 case FFS: 12285 return "A billing arrangement where a Provider charges a separate fee for each intervention/procedure/event or product.\r\n\n Fee for Service is used when an individual intervention/procedure/event is used for billing purposes. In other words, fees are associated with the intervention/procedure/event. For example, a specific CCI (Canadian Classification of Interventions) code has an associated fee and is used for billing purposes."; 12286 case FFPS: 12287 return "A first fill where the quantity supplied is less than one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill might be for only 30 tablets.) and also where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)"; 12288 case FFCS: 12289 return "A first fill where the quantity supplied is equal to one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill would be for the full 90 tablets) and also where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 12290 case TFS: 12291 return "A fill where a small portion is provided to allow for determination of the therapy effectiveness and patient tolerance and also where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 12292 case _ACTBOUNDEDROICODE: 12293 return "Type of bounded ROI."; 12294 case ROIFS: 12295 return "A fully specified bounded Region of Interest (ROI) delineates a ROI in which only those dimensions participate that are specified by boundary criteria, whereas all other dimensions are excluded. For example a ROI to mark an episode of \"ST elevation\" in a subset of the EKG leads V2, V3, and V4 would include 4 boundaries, one each for time, V2, V3, and V4."; 12296 case ROIPS: 12297 return "A partially specified bounded Region of Interest (ROI) specifies a ROI in which at least all values in the dimensions specified by the boundary criteria participate. For example, if an episode of ventricular fibrillations (VFib) is observed, it usually doesn't make sense to exclude any EKG leads from the observation and the partially specified ROI would contain only one boundary for time indicating the time interval where VFib was observed."; 12298 case _ACTCAREPROVISIONCODE: 12299 return "Description:The type and scope of responsibility taken-on by the performer of the Act for a specific subject of care."; 12300 case _ACTCREDENTIALEDCARECODE: 12301 return "Description:The type and scope of legal and/or professional responsibility taken-on by the performer of the Act for a specific subject of care as described by a credentialing agency, i.e. government or non-government agency. Failure in executing this Act may result in loss of credential to the person or organization who participates as performer of the Act. Excludes employment agreements.\r\n\n \n Example:Hospital license; physician license; clinic accreditation."; 12302 case _ACTCREDENTIALEDCAREPROVISIONPERSONCODE: 12303 return "Description:The type and scope of legal and/or professional responsibility taken-on by the performer of the Act for a specific subject of care as described by an agency for credentialing individuals."; 12304 case CACC: 12305 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12306 case CAIC: 12307 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12308 case CAMC: 12309 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12310 case CANC: 12311 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12312 case CAPC: 12313 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12314 case CBGC: 12315 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12316 case CCCC: 12317 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12318 case CCGC: 12319 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12320 case CCPC: 12321 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12322 case CCSC: 12323 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12324 case CDEC: 12325 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12326 case CDRC: 12327 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12328 case CEMC: 12329 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12330 case CFPC: 12331 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12332 case CIMC: 12333 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12334 case CMGC: 12335 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12336 case CNEC: 12337 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board"; 12338 case CNMC: 12339 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12340 case CNQC: 12341 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12342 case CNSC: 12343 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12344 case COGC: 12345 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12346 case COMC: 12347 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12348 case COPC: 12349 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12350 case COSC: 12351 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12352 case COTC: 12353 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12354 case CPEC: 12355 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12356 case CPGC: 12357 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12358 case CPHC: 12359 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12360 case CPRC: 12361 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12362 case CPSC: 12363 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12364 case CPYC: 12365 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12366 case CROC: 12367 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12368 case CRPC: 12369 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12370 case CSUC: 12371 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12372 case CTSC: 12373 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12374 case CURC: 12375 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12376 case CVSC: 12377 return "Description:Scope of responsibility taken on for specialty care as defined by the respective Specialty Board."; 12378 case LGPC: 12379 return "Description:Scope of responsibility taken-on for physician care of a patient as defined by a governmental licensing agency."; 12380 case _ACTCREDENTIALEDCAREPROVISIONPROGRAMCODE: 12381 return "Description:The type and scope of legal and/or professional responsibility taken-on by the performer of the Act for a specific subject of care as described by an agency for credentialing programs within organizations."; 12382 case AALC: 12383 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12384 case AAMC: 12385 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12386 case ABHC: 12387 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12388 case ACAC: 12389 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12390 case ACHC: 12391 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12392 case AHOC: 12393 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12394 case ALTC: 12395 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12396 case AOSC: 12397 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the respective accreditation agency."; 12398 case CACS: 12399 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12400 case CAMI: 12401 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12402 case CAST: 12403 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12404 case CBAR: 12405 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12406 case CCAD: 12407 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12408 case CCAR: 12409 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12410 case CDEP: 12411 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12412 case CDGD: 12413 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12414 case CDIA: 12415 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12416 case CEPI: 12417 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12418 case CFEL: 12419 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12420 case CHFC: 12421 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12422 case CHRO: 12423 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12424 case CHYP: 12425 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12426 case CMIH: 12427 return "Description:."; 12428 case CMSC: 12429 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12430 case COJR: 12431 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12432 case CONC: 12433 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12434 case COPD: 12435 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12436 case CORT: 12437 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12438 case CPAD: 12439 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12440 case CPND: 12441 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12442 case CPST: 12443 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12444 case CSDM: 12445 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12446 case CSIC: 12447 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12448 case CSLD: 12449 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12450 case CSPT: 12451 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12452 case CTBU: 12453 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12454 case CVDC: 12455 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12456 case CWMA: 12457 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12458 case CWOH: 12459 return "Description:Scope of responsibility taken on by an organization for care of a patient as defined by the disease management certification agency."; 12460 case _ACTENCOUNTERCODE: 12461 return "Domain provides codes that qualify the ActEncounterClass (ENC)"; 12462 case AMB: 12463 return "A comprehensive term for health care provided in a healthcare facility (e.g. a practitioneraTMs office, clinic setting, or hospital) on a nonresident basis. The term ambulatory usually implies that the patient has come to the location and is not assigned to a bed. Sometimes referred to as an outpatient encounter."; 12464 case EMER: 12465 return "A patient encounter that takes place at a dedicated healthcare service delivery location where the patient receives immediate evaluation and treatment, provided until the patient can be discharged or responsibility for the patient's care is transferred elsewhere (for example, the patient could be admitted as an inpatient or transferred to another facility.)"; 12466 case FLD: 12467 return "A patient encounter that takes place both outside a dedicated service delivery location and outside a patient's residence. Example locations might include an accident site and at a supermarket."; 12468 case HH: 12469 return "Healthcare encounter that takes place in the residence of the patient or a designee"; 12470 case IMP: 12471 return "A patient encounter where a patient is admitted by a hospital or equivalent facility, assigned to a location where patients generally stay at least overnight and provided with room, board, and continuous nursing service."; 12472 case ACUTE: 12473 return "An acute inpatient encounter."; 12474 case NONAC: 12475 return "Any category of inpatient encounter except 'acute'"; 12476 case OBSENC: 12477 return "An encounter where the patient usually will start in different encounter, such as one in the emergency department (EMER) but then transition to this type of encounter because they require a significant period of treatment and monitoring to determine whether or not their condition warrants an inpatient admission or discharge. In the majority of cases the decision about admission or discharge will occur within a time period determined by local, regional or national regulation, often between 24 and 48 hours."; 12478 case PRENC: 12479 return "A patient encounter where patient is scheduled or planned to receive service delivery in the future, and the patient is given a pre-admission account number. When the patient comes back for subsequent service, the pre-admission encounter is selected and is encapsulated into the service registration, and a new account number is generated.\r\n\n \n Usage Note: This is intended to be used in advance of encounter types such as ambulatory, inpatient encounter, virtual, etc."; 12480 case SS: 12481 return "An encounter where the patient is admitted to a health care facility for a predetermined length of time, usually less than 24 hours."; 12482 case VR: 12483 return "A patient encounter where the patient and the practitioner(s) are not in the same physical location. Examples include telephone conference, email exchange, robotic surgery, and televideo conference."; 12484 case _ACTMEDICALSERVICECODE: 12485 return "General category of medical service provided to the patient during their encounter."; 12486 case ALC: 12487 return "Provision of Alternate Level of Care to a patient in an acute bed. Patient is waiting for placement in a long-term care facility and is unable to return home."; 12488 case CARD: 12489 return "Provision of diagnosis and treatment of diseases and disorders affecting the heart"; 12490 case CHR: 12491 return "Provision of recurring care for chronic illness."; 12492 case DNTL: 12493 return "Provision of treatment for oral health and/or dental surgery."; 12494 case DRGRHB: 12495 return "Provision of treatment for drug abuse."; 12496 case GENRL: 12497 return "General care performed by a general practitioner or family doctor as a responsible provider for a patient."; 12498 case MED: 12499 return "Provision of diagnostic and/or therapeutic treatment."; 12500 case OBS: 12501 return "Provision of care of women during pregnancy, childbirth and immediate postpartum period. Also known as Maternity."; 12502 case ONC: 12503 return "Provision of treatment and/or diagnosis related to tumors and/or cancer."; 12504 case PALL: 12505 return "Provision of care for patients who are living or dying from an advanced illness."; 12506 case PED: 12507 return "Provision of diagnosis and treatment of diseases and disorders affecting children."; 12508 case PHAR: 12509 return "Pharmaceutical care performed by a pharmacist."; 12510 case PHYRHB: 12511 return "Provision of treatment for physical injury."; 12512 case PSYCH: 12513 return "Provision of treatment of psychiatric disorder relating to mental illness."; 12514 case SURG: 12515 return "Provision of surgical treatment."; 12516 case _ACTCLAIMATTACHMENTCATEGORYCODE: 12517 return "Description: Coded types of attachments included to support a healthcare claim."; 12518 case AUTOATTCH: 12519 return "Description: Automobile Information Attachment"; 12520 case DOCUMENT: 12521 return "Description: Document Attachment"; 12522 case HEALTHREC: 12523 return "Description: Health Record Attachment"; 12524 case IMG: 12525 return "Description: Image Attachment"; 12526 case LABRESULTS: 12527 return "Description: Lab Results Attachment"; 12528 case MODEL: 12529 return "Description: Digital Model Attachment"; 12530 case WIATTCH: 12531 return "Description: Work Injury related additional Information Attachment"; 12532 case XRAY: 12533 return "Description: Digital X-Ray Attachment"; 12534 case _ACTCONSENTTYPE: 12535 return "Definition: The type of consent directive, e.g., to consent or dissent to collect, access, or use in specific ways within an EHRS or for health information exchange; or to disclose health information for purposes such as research."; 12536 case ICOL: 12537 return "Definition: Consent to have healthcare information collected in an electronic health record. This entails that the information may be used in analysis, modified, updated."; 12538 case IDSCL: 12539 return "Definition: Consent to have collected healthcare information disclosed."; 12540 case INFA: 12541 return "Definition: Consent to access healthcare information."; 12542 case INFAO: 12543 return "Definition: Consent to access or \"read\" only, which entails that the information is not to be copied, screen printed, saved, emailed, stored, re-disclosed or altered in any way. This level ensures that data which is masked or to which access is restricted will not be.\r\n\n \n Example: Opened and then emailed or screen printed for use outside of the consent directive purpose."; 12544 case INFASO: 12545 return "Definition: Consent to access and save only, which entails that access to the saved copy will remain locked."; 12546 case IRDSCL: 12547 return "Definition: Information re-disclosed without the patient's consent."; 12548 case RESEARCH: 12549 return "Definition: Consent to have healthcare information in an electronic health record accessed for research purposes."; 12550 case RSDID: 12551 return "Definition: Consent to have de-identified healthcare information in an electronic health record that is accessed for research purposes, but without consent to re-identify the information under any circumstance."; 12552 case RSREID: 12553 return "Definition: Consent to have de-identified healthcare information in an electronic health record that is accessed for research purposes re-identified under specific circumstances outlined in the consent.\r\n\n \n Example:: Where there is a need to inform the subject of potential health issues."; 12554 case _ACTCONTAINERREGISTRATIONCODE: 12555 return "Constrains the ActCode to the domain of Container Registration"; 12556 case ID: 12557 return "Used by one system to inform another that it has received a container."; 12558 case IP: 12559 return "Used by one system to inform another that the container is in position for specimen transfer (e.g., container removal from track, pipetting, etc.)."; 12560 case L: 12561 return "Used by one system to inform another that the container has been released from that system."; 12562 case M: 12563 return "Used by one system to inform another that the container did not arrive at its next expected location."; 12564 case O: 12565 return "Used by one system to inform another that the specific container is being processed by the equipment. It is useful as a response to a query about Container Status, when the specific step of the process is not relevant."; 12566 case R: 12567 return "Status is used by one system to inform another that the processing has been completed, but the container has not been released from that system."; 12568 case X: 12569 return "Used by one system to inform another that the container is no longer available within the scope of the system (e.g., tube broken or discarded)."; 12570 case _ACTCONTROLVARIABLE: 12571 return "An observation form that determines parameters or attributes of an Act. Examples are the settings of a ventilator machine as parameters of a ventilator treatment act; the controls on dillution factors of a chemical analyzer as a parameter of a laboratory observation act; the settings of a physiologic measurement assembly (e.g., time skew) or the position of the body while measuring blood pressure.\r\n\n Control variables are forms of observations because just as with clinical observations, the Observation.code determines the parameter and the Observation.value assigns the value. While control variables sometimes can be observed (by noting the control settings or an actually measured feedback loop) they are not primary observations, in the sense that a control variable without a primary act is of no use (e.g., it makes no sense to record a blood pressure position without recording a blood pressure, whereas it does make sense to record a systolic blood pressure without a diastolic blood pressure)."; 12572 case AUTO: 12573 return "Specifies whether or not automatic repeat testing is to be initiated on specimens."; 12574 case ENDC: 12575 return "A baseline value for the measured test that is inherently contained in the diluent. In the calculation of the actual result for the measured test, this baseline value is normally considered."; 12576 case REFLEX: 12577 return "Specifies whether or not further testing may be automatically or manually initiated on specimens."; 12578 case _ACTCOVERAGECONFIRMATIONCODE: 12579 return "Response to an insurance coverage eligibility query or authorization request."; 12580 case _ACTCOVERAGEAUTHORIZATIONCONFIRMATIONCODE: 12581 return "Indication of authorization for healthcare service(s) and/or product(s). If authorization is approved, funds are set aside."; 12582 case AUTH: 12583 return "Authorization approved and funds have been set aside to pay for specified healthcare service(s) and/or product(s) within defined criteria for the authorization."; 12584 case NAUTH: 12585 return "Authorization for specified healthcare service(s) and/or product(s) denied."; 12586 case _ACTCOVERAGEELIGIBILITYCONFIRMATIONCODE: 12587 return "Indication of eligibility coverage for healthcare service(s) and/or product(s)."; 12588 case ELG: 12589 return "Insurance coverage is in effect for healthcare service(s) and/or product(s)."; 12590 case NELG: 12591 return "Insurance coverage is not in effect for healthcare service(s) and/or product(s). May optionally include reasons for the ineligibility."; 12592 case _ACTCOVERAGELIMITCODE: 12593 return "Criteria that are applicable to the authorized coverage."; 12594 case _ACTCOVERAGEQUANTITYLIMITCODE: 12595 return "Maximum amount paid or maximum number of services/products covered; or maximum amount or number covered during a specified time period under the policy or program."; 12596 case COVPRD: 12597 return "Codes representing the time period during which coverage is available; or financial participation requirements are in effect."; 12598 case LFEMX: 12599 return "Definition: Maximum amount paid by payer or covered party; or maximum number of services or products covered under the policy or program during a covered party's lifetime."; 12600 case NETAMT: 12601 return "Maximum net amount that will be covered for the product or service specified."; 12602 case PRDMX: 12603 return "Definition: Maximum amount paid by payer or covered party; or maximum number of services/products covered under the policy or program by time period specified by the effective time on the act."; 12604 case UNITPRICE: 12605 return "Maximum unit price that will be covered for the authorized product or service."; 12606 case UNITQTY: 12607 return "Maximum number of items that will be covered of the product or service specified."; 12608 case COVMX: 12609 return "Definition: Codes representing the maximum coverate or financial participation requirements."; 12610 case _ACTCOVEREDPARTYLIMITCODE: 12611 return "Codes representing the types of covered parties that may receive covered benefits under a policy or program."; 12612 case _ACTCOVERAGETYPECODE: 12613 return "Definition: Set of codes indicating the type of insurance policy or program that pays for the cost of benefits provided to covered parties."; 12614 case _ACTINSURANCEPOLICYCODE: 12615 return "Set of codes indicating the type of insurance policy or other source of funds to cover healthcare costs."; 12616 case EHCPOL: 12617 return "Private insurance policy that provides coverage in addition to other policies (e.g. in addition to a Public Healthcare insurance policy)."; 12618 case HSAPOL: 12619 return "Insurance policy that provides for an allotment of funds replenished on a periodic (e.g. annual) basis. The use of the funds under this policy is at the discretion of the covered party."; 12620 case AUTOPOL: 12621 return "Insurance policy for injuries sustained in an automobile accident. Will also typically covered non-named parties to the policy, such as pedestrians and passengers."; 12622 case COL: 12623 return "Definition: An automobile insurance policy under which the insurance company will cover the cost of damages to an automobile owned by the named insured that are caused by accident or intentionally by another party."; 12624 case UNINSMOT: 12625 return "Definition: An automobile insurance policy under which the insurance company will indemnify a loss for which another motorist is liable if that motorist is unable to pay because he or she is uninsured. Coverage under the policy applies to bodily injury damages only. Injuries to the covered party caused by a hit-and-run driver are also covered."; 12626 case PUBLICPOL: 12627 return "Insurance policy funded by a public health system such as a provincial or national health plan. Examples include BC MSP (British Columbia Medical Services Plan) OHIP (Ontario Health Insurance Plan), NHS (National Health Service)."; 12628 case DENTPRG: 12629 return "Definition: A public or government health program that administers and funds coverage for dental care to assist program eligible who meet financial and health status criteria."; 12630 case DISEASEPRG: 12631 return "Definition: A public or government health program that administers and funds coverage for health and social services to assist program eligible who meet financial and health status criteria related to a particular disease.\r\n\n \n Example: Reproductive health, sexually transmitted disease, and end renal disease programs."; 12632 case CANPRG: 12633 return "Definition: A program that provides low-income, uninsured, and underserved women access to timely, high-quality screening and diagnostic services, to detect breast and cervical cancer at the earliest stages.\r\n\n \n Example: To improve women's access to screening for breast and cervical cancers, Congress passed the Breast and Cervical Cancer Mortality Prevention Act of 1990, which guided CDC in creating the National Breast and Cervical Cancer Early Detection Program (NBCCEDP), which provides access to critical breast and cervical cancer screening services for underserved women in the United States. An estimated 7 to 10% of U.S. women of screening age are eligible to receive NBCCEDP services. Federal guidelines establish an eligibility baseline to direct services to uninsured and underinsured women at or below 250% of federal poverty level; ages 18 to 64 for cervical screening; ages 40 to 64 for breast screening."; 12634 case ENDRENAL: 12635 return "Definition: A public or government program that administers publicly funded coverage of kidney dialysis and kidney transplant services.\r\n\n Example: In the U.S., the Medicare End-stage Renal Disease program (ESRD), the National Kidney Foundation (NKF) American Kidney Fund (AKF) The Organ Transplant Fund."; 12636 case HIVAIDS: 12637 return "Definition: Government administered and funded HIV-AIDS program for beneficiaries meeting financial and health status criteria. Administration, funding levels, eligibility criteria, covered benefits, provider types, and financial participation are typically set by a regulatory process. Payer responsibilities for administering the program may be delegated to contractors.\r\n\n \n Example: In the U.S., the Ryan White program, which is administered by the Health Resources and Services Administration."; 12638 case MANDPOL: 12639 return "mandatory health program"; 12640 case MENTPRG: 12641 return "Definition: Government administered and funded mental health program for beneficiaries meeting financial and mental health status criteria. Administration, funding levels, eligibility criteria, covered benefits, provider types, and financial participation are typically set by a regulatory process. Payer responsibilities for administering the program may be delegated to contractors.\r\n\n \n Example: In the U.S., states receive funding for substance use programs from the Substance Abuse Mental Health Administration (SAMHSA)."; 12642 case SAFNET: 12643 return "Definition: Government administered and funded program to support provision of care to underserved populations through safety net clinics.\r\n\n \n Example: In the U.S., safety net providers such as federally qualified health centers (FQHC) receive funding under PHSA Section 330 grants administered by the Health Resources and Services Administration."; 12644 case SUBPRG: 12645 return "Definition: Government administered and funded substance use program for beneficiaries meeting financial, substance use behavior, and health status criteria. Beneficiaries may be required to enroll as a result of legal proceedings. Administration, funding levels, eligibility criteria, covered benefits, provider types, and financial participation are typically set by a regulatory process. Payer responsibilities for administering the program may be delegated to contractors.\r\n\n \n Example: In the U.S., states receive funding for substance use programs from the Substance Abuse Mental Health Administration (SAMHSA)."; 12646 case SUBSIDIZ: 12647 return "Definition: A government health program that provides coverage for health services to persons meeting eligibility criteria such as income, location of residence, access to other coverages, health condition, and age, the cost of which is to some extent subsidized by public funds."; 12648 case SUBSIDMC: 12649 return "Definition: A government health program that provides coverage through managed care contracts for health services to persons meeting eligibility criteria such as income, location of residence, access to other coverages, health condition, and age, the cost of which is to some extent subsidized by public funds. \r\n\n \n Discussion: The structure and business processes for underwriting and administering a subsidized managed care program is further specified by the Underwriter and Payer Role.class and Role.code."; 12650 case SUBSUPP: 12651 return "Definition: A government health program that provides coverage for health services to persons meeting eligibility criteria for a supplemental health policy or program such as income, location of residence, access to other coverages, health condition, and age, the cost of which is to some extent subsidized by public funds.\r\n\n \n Example: Supplemental health coverage program may cover the cost of a health program or policy financial participations, such as the copays and the premiums, and may provide coverage for services in addition to those covered under the supplemented health program or policy. In the U.S., Medicaid programs may pay the premium for a covered party who is also covered under the Medicare program or a private health policy.\r\n\n \n Discussion: The structure and business processes for underwriting and administering a subsidized supplemental retiree health program is further specified by the Underwriter and Payer Role.class and Role.code."; 12652 case WCBPOL: 12653 return "Insurance policy for injuries sustained in the work place or in the course of employment."; 12654 case _ACTINSURANCETYPECODE: 12655 return "Definition: Set of codes indicating the type of insurance policy. Insurance, in law and economics, is a form of risk management primarily used to hedge against the risk of potential financial loss. Insurance is defined as the equitable transfer of the risk of a potential loss, from one entity to another, in exchange for a premium and duty of care. A policy holder is an individual or an organization enters into a contract with an underwriter which stipulates that, in exchange for payment of a sum of money (a premium), one or more covered parties (insureds) is guaranteed compensation for losses resulting from certain perils under specified conditions. The underwriter analyzes the risk of loss, makes a decision as to whether the risk is insurable, and prices the premium accordingly. A policy provides benefits that indemnify or cover the cost of a loss incurred by a covered party, and may include coverage for services required to remediate a loss. An insurance policy contains pertinent facts about the policy holder, the insurance coverage, the covered parties, and the insurer. A policy may include exemptions and provisions specifying the extent to which the indemnification clause cannot be enforced for intentional tortious conduct of a covered party, e.g., whether the covered parties are jointly or severably insured.\r\n\n \n Discussion: In contrast to programs, an insurance policy has one or more policy holders, who own the policy. The policy holder may be the covered party, a relative of the covered party, a partnership, or a corporation, e.g., an employer. A subscriber of a self-insured health insurance policy is a policy holder. A subscriber of an employer sponsored health insurance policy is holds a certificate of coverage, but is not a policy holder; the policy holder is the employer. See CoveredRoleType."; 12656 case _ACTHEALTHINSURANCETYPECODE: 12657 return "Definition: Set of codes indicating the type of health insurance policy that covers health services provided to covered parties. A health insurance policy is a written contract for insurance between the insurance company and the policyholder, and contains pertinent facts about the policy owner (the policy holder), the health insurance coverage, the insured subscribers and dependents, and the insurer. Health insurance is typically administered in accordance with a plan, which specifies (1) the type of health services and health conditions that will be covered under what circumstances (e.g., exclusion of a pre-existing condition, service must be deemed medically necessary; service must not be experimental; service must provided in accordance with a protocol; drug must be on a formulary; service must be prior authorized; or be a referral from a primary care provider); (2) the type and affiliation of providers (e.g., only allopathic physicians, only in network, only providers employed by an HMO); (3) financial participations required of covered parties (e.g., co-pays, coinsurance, deductibles, out-of-pocket); and (4) the manner in which services will be paid (e.g., under indemnity or fee-for-service health plans, the covered party typically pays out-of-pocket and then file a claim for reimbursement, while health plans that have contractual relationships with providers, i.e., network providers, typically do not allow the providers to bill the covered party for the cost of the service until after filing a claim with the payer and receiving reimbursement)."; 12658 case DENTAL: 12659 return "Definition: A health insurance policy that that covers benefits for dental services."; 12660 case DISEASE: 12661 return "Definition: A health insurance policy that covers benefits for healthcare services provided for named conditions under the policy, e.g., cancer, diabetes, or HIV-AIDS."; 12662 case DRUGPOL: 12663 return "Definition: A health insurance policy that covers benefits for prescription drugs, pharmaceuticals, and supplies."; 12664 case HIP: 12665 return "Definition: A health insurance policy that covers healthcare benefits by protecting covered parties from medical expenses arising from health conditions, sickness, or accidental injury as well as preventive care. Health insurance policies explicitly exclude coverage for losses insured under a disability policy, workers' compensation program, liability insurance (including automobile insurance); or for medical expenses, coverage for on-site medical clinics or for limited dental or vision benefits when these are provided under a separate policy.\r\n\n \n Discussion: Health insurance policies are offered by health insurance plans that typically reimburse providers for covered services on a fee-for-service basis, that is, a fee that is the allowable amount that a provider may charge. This is in contrast to managed care plans, which typically prepay providers a per-member/per-month amount or capitation as reimbursement for all covered services rendered. Health insurance plans include indemnity and healthcare services plans."; 12666 case LTC: 12667 return "Definition: An insurance policy that covers benefits for long-term care services people need when they no longer can care for themselves. This may be due to an accident, disability, prolonged illness or the simple process of aging. Long-term care services assist with activities of daily living including:\r\n\n \n \n Help at home with day-to-day activities, such as cooking, cleaning, bathing and dressing\r\n\n \n \n Care in the community, such as in an adult day care facility\r\n\n \n \n Supervised care provided in an assisted living facility\r\n\n \n \n Skilled care provided in a nursing home"; 12668 case MCPOL: 12669 return "Definition: Government mandated program providing coverage, disability income, and vocational rehabilitation for injuries sustained in the work place or in the course of employment. Employers may either self-fund the program, purchase commercial coverage, or pay a premium to a government entity that administers the program. Employees may be required to pay premiums toward the cost of coverage as well.\r\n\n Managed care policies specifically exclude coverage for losses insured under a disability policy, workers' compensation program, liability insurance (including automobile insurance); or for medical expenses, coverage for on-site medical clinics or for limited dental or vision benefits when these are provided under a separate policy.\r\n\n \n Discussion: Managed care policies are offered by managed care plans that contract with selected providers or health care organizations to provide comprehensive health care at a discount to covered parties and coordinate the financing and delivery of health care. Managed care uses medical protocols and procedures agreed on by the medical profession to be cost effective, also known as medical practice guidelines. Providers are typically reimbursed for covered services by a capitated amount on a per member per month basis that may reflect difference in the health status and level of services anticipated to be needed by the member."; 12670 case POS: 12671 return "Definition: A policy for a health plan that has features of both an HMO and a FFS plan. Like an HMO, a POS plan encourages the use its HMO network to maintain discounted fees with participating providers, but recognizes that sometimes covered parties want to choose their own provider. The POS plan allows a covered party to use providers who are not part of the HMO network (non-participating providers). However, there is a greater cost associated with choosing these non-network providers. A covered party will usually pay deductibles and coinsurances that are substantially higher than the payments when he or she uses a plan provider. Use of non-participating providers often requires the covered party to pay the provider directly and then to file a claim for reimbursement, like in an FFS plan."; 12672 case HMO: 12673 return "Definition: A policy for a health plan that provides coverage for health care only through contracted or employed physicians and hospitals located in particular geographic or service areas. HMOs emphasize prevention and early detection of illness. Eligibility to enroll in an HMO is determined by where a covered party lives or works."; 12674 case PPO: 12675 return "Definition: A network-based, managed care plan that allows a covered party to choose any health care provider. However, if care is received from a \"preferred\" (participating in-network) provider, there are generally higher benefit coverage and lower deductibles."; 12676 case MENTPOL: 12677 return "Definition: A health insurance policy that covers benefits for mental health services and prescriptions."; 12678 case SUBPOL: 12679 return "Definition: A health insurance policy that covers benefits for substance use services."; 12680 case VISPOL: 12681 return "Definition: Set of codes for a policy that provides coverage for health care expenses arising from vision services.\r\n\n A health insurance policy that covers benefits for vision care services, prescriptions, and products."; 12682 case DIS: 12683 return "Definition: An insurance policy that provides a regular payment to compensate for income lost due to the covered party's inability to work because of illness or injury."; 12684 case EWB: 12685 return "Definition: An insurance policy under a benefit plan run by an employer or employee organization for the purpose of providing benefits other than pension-related to employees and their families. Typically provides health-related benefits, benefits for disability, disease or unemployment, or day care and scholarship benefits, among others. An employer sponsored health policy includes coverage of health care expenses arising from sickness or accidental injury, coverage for on-site medical clinics or for dental or vision benefits, which are typically provided under a separate policy. Coverage excludes health care expenses covered by accident or disability, workers' compensation, liability or automobile insurance."; 12686 case FLEXP: 12687 return "Definition: An insurance policy that covers qualified benefits under a Flexible Benefit plan such as group medical insurance, long and short term disability income insurance, group term life insurance for employees only up to $50,000 face amount, specified disease coverage such as a cancer policy, dental and/or vision insurance, hospital indemnity insurance, accidental death and dismemberment insurance, a medical expense reimbursement plan and a dependent care reimbursement plan.\r\n\n \n Discussion: See UnderwriterRoleTypeCode flexible benefit plan which is defined as a benefit plan that allows employees to choose from several life, health, disability, dental, and other insurance plans according to their individual needs. Also known as cafeteria plans. Authorized under Section 125 of the Revenue Act of 1978."; 12688 case LIFE: 12689 return "Definition: A policy under which the insurer agrees to pay a sum of money upon the occurrence of the covered partys death. In return, the policyholder agrees to pay a stipulated amount called a premium at regular intervals. Life insurance indemnifies the beneficiary for the loss of the insurable interest that a beneficiary has in the life of a covered party. For persons related by blood, a substantial interest established through love and affection, and for all other persons, a lawful and substantial economic interest in having the life of the insured continue. An insurable interest is required when purchasing life insurance on another person. Specific exclusions are often written into the contract to limit the liability of the insurer; for example claims resulting from suicide or relating to war, riot and civil commotion.\r\n\n \n Discussion:A life insurance policy may be used by the covered party as a source of health care coverage in the case of a viatical settlement, which is the sale of a life insurance policy by the policy owner, before the policy matures. Such a sale, at a price discounted from the face amount of the policy but usually in excess of the premiums paid or current cash surrender value, provides the seller an immediate cash settlement. Generally, viatical settlements involve insured individuals with a life expectancy of less than two years. In countries without state-subsidized healthcare and high healthcare costs (e.g. United States), this is a practical way to pay extremely high health insurance premiums that severely ill people face. Some people are also familiar with life settlements, which are similar transactions but involve insureds with longer life expectancies (two to fifteen years)."; 12690 case ANNU: 12691 return "Definition: A policy that, after an initial premium or premiums, pays out a sum at pre-determined intervals.\r\n\n For example, a policy holder may pay $10,000, and in return receive $150 each month until he dies; or $1,000 for each of 14 years or death benefits if he dies before the full term of the annuity has elapsed."; 12692 case TLIFE: 12693 return "Definition: Life insurance under which the benefit is payable only if the insured dies during a specified period. If an insured dies during that period, the beneficiary receives the death payments. If the insured survives, the policy ends and the beneficiary receives nothing."; 12694 case ULIFE: 12695 return "Definition: Life insurance under which the benefit is payable upon the insuredaTMs death or diagnosis of a terminal illness. If an insured dies during that period, the beneficiary receives the death payments. If the insured survives, the policy ends and the beneficiary receives nothing"; 12696 case PNC: 12697 return "Definition: A type of insurance that covers damage to or loss of the policyholderaTMs property by providing payments for damages to property damage or the injury or death of living subjects. The terms \"casualty\" and \"liability\" insurance are often used interchangeably. Both cover the policyholder's legal liability for damages caused to other persons and/or their property."; 12698 case REI: 12699 return "Definition: An agreement between two or more insurance companies by which the risk of loss is proportioned. Thus the risk of loss is spread and a disproportionately large loss under a single policy does not fall on one insurance company. Acceptance by an insurer, called a reinsurer, of all or part of the risk of loss of another insurance company.\r\n\n \n Discussion: Reinsurance is a means by which an insurance company can protect itself against the risk of losses with other insurance companies. Individuals and corporations obtain insurance policies to provide protection for various risks (hurricanes, earthquakes, lawsuits, collisions, sickness and death, etc.). Reinsurers, in turn, provide insurance to insurance companies.\r\n\n For example, an HMO may purchase a reinsurance policy to protect itself from losing too much money from one insured's particularly expensive health care costs. An insurance company issuing an automobile liability policy, with a limit of $100,000 per accident may reinsure its liability in excess of $10,000. A fire insurance company which issues a large policy generally reinsures a portion of the risk with one or several other companies. Also called risk control insurance or stop-loss insurance."; 12700 case SURPL: 12701 return "Definition: \n \r\n\n \n \n A risk or part of a risk for which there is no normal insurance market available.\r\n\n \n \n Insurance written by unauthorized insurance companies. Surplus lines insurance is insurance placed with unauthorized insurance companies through licensed surplus lines agents or brokers."; 12702 case UMBRL: 12703 return "Definition: A form of insurance protection that provides additional liability coverage after the limits of your underlying policy are reached. An umbrella liability policy also protects you (the insured) in many situations not covered by the usual liability policies."; 12704 case _ACTPROGRAMTYPECODE: 12705 return "Definition: A set of codes used to indicate coverage under a program. A program is an organized structure for administering and funding coverage of a benefit package for covered parties meeting eligibility criteria, typically related to employment, health, financial, and demographic status. Programs are typically established or permitted by legislation with provisions for ongoing government oversight. Regulations may mandate the structure of the program, the manner in which it is funded and administered, covered benefits, provider types, eligibility criteria and financial participation. A government agency may be charged with implementing the program in accordance to the regulation. Risk of loss under a program in most cases would not meet what an underwriter would consider an insurable risk, i.e., the risk is not random in nature, not financially measurable, and likely requires subsidization with government funds.\r\n\n \n Discussion: Programs do not have policy holders or subscribers. Program eligibles are enrolled based on health status, statutory eligibility, financial status, or age. Program eligibles who are covered parties under the program may be referred to as members, beneficiaries, eligibles, or recipients. Programs risk are underwritten by not for profit organizations such as governmental entities, and the beneficiaries typically do not pay for any or some portion of the cost of coverage. See CoveredPartyRoleType."; 12706 case CHAR: 12707 return "Definition: A program that covers the cost of services provided directly to a beneficiary who typically has no other source of coverage without charge."; 12708 case CRIME: 12709 return "Definition: A program that covers the cost of services provided to crime victims for injuries or losses related to the occurrence of a crime."; 12710 case EAP: 12711 return "Definition: An employee assistance program is run by an employer or employee organization for the purpose of providing benefits and covering all or part of the cost for employees to receive counseling, referrals, and advice in dealing with stressful issues in their lives. These may include substance abuse, bereavement, marital problems, weight issues, or general wellness issues. The services are usually provided by a third-party, rather than the company itself, and the company receives only summary statistical data from the service provider. Employee's names and services received are kept confidential."; 12712 case GOVEMP: 12713 return "Definition: A set of codes used to indicate a government program that is an organized structure for administering and funding coverage of a benefit package for covered parties meeting eligibility criteria, typically related to employment, health and financial status. Government programs are established or permitted by legislation with provisions for ongoing government oversight. Regulation mandates the structure of the program, the manner in which it is funded and administered, covered benefits, provider types, eligibility criteria and financial participation. A government agency is charged with implementing the program in accordance to the regulation\r\n\n \n Example: Federal employee health benefit program in the U.S."; 12714 case HIRISK: 12715 return "Definition: A government program that provides health coverage to individuals who are considered medically uninsurable or high risk, and who have been denied health insurance due to a serious health condition. In certain cases, it also applies to those who have been quoted very high premiums a\" again, due to a serious health condition. The pool charges premiums for coverage. Because the pool covers high-risk people, it incurs a higher level of claims than premiums can cover. The insurance industry pays into the pool to make up the difference and help it remain viable."; 12716 case IND: 12717 return "Definition: Services provided directly and through contracted and operated indigenous peoples health programs.\r\n\n \n Example: Indian Health Service in the U.S."; 12718 case MILITARY: 12719 return "Definition: A government program that provides coverage for health services to military personnel, retirees, and dependents. A covered party who is a subscriber can choose from among Fee-for-Service (FFS) plans, and their Preferred Provider Organizations (PPO), or Plans offering a Point of Service (POS) Product, or Health Maintenance Organizations.\r\n\n \n Example: In the U.S., TRICARE, CHAMPUS."; 12720 case RETIRE: 12721 return "Definition: A government mandated program with specific eligibility requirements based on premium contributions made during employment, length of employment, age, and employment status, e.g., being retired, disabled, or a dependent of a covered party under this program. Benefits typically include ambulatory, inpatient, and long-term care, such as hospice care, home health care and respite care."; 12722 case SOCIAL: 12723 return "Definition: A social service program funded by a public or governmental entity.\r\n\n \n Example: Programs providing habilitation, food, lodging, medicine, transportation, equipment, devices, products, education, training, counseling, alteration of living or work space, and other resources to persons meeting eligibility criteria."; 12724 case VET: 12725 return "Definition: Services provided directly and through contracted and operated veteran health programs."; 12726 case _ACTDETECTEDISSUEMANAGEMENTCODE: 12727 return "Codes dealing with the management of Detected Issue observations"; 12728 case _ACTADMINISTRATIVEDETECTEDISSUEMANAGEMENTCODE: 12729 return "Codes dealing with the management of Detected Issue observations for the administrative and patient administrative acts domains."; 12730 case _AUTHORIZATIONISSUEMANAGEMENTCODE: 12731 return "Authorization Issue Management Code"; 12732 case EMAUTH: 12733 return "Used to temporarily override normal authorization rules to gain access to data in a case of emergency. Use of this override code will typically be monitored, and a procedure to verify its proper use may be triggered when used."; 12734 case _21: 12735 return "Description: Indicates that the permissions have been externally verified and the request should be processed."; 12736 case _1: 12737 return "Confirmed drug therapy appropriate"; 12738 case _19: 12739 return "Consulted other supplier/pharmacy, therapy confirmed"; 12740 case _2: 12741 return "Assessed patient, therapy is appropriate"; 12742 case _22: 12743 return "Description: The patient has the appropriate indication or diagnosis for the action to be taken."; 12744 case _23: 12745 return "Description: It has been confirmed that the appropriate pre-requisite therapy has been tried."; 12746 case _3: 12747 return "Patient gave adequate explanation"; 12748 case _4: 12749 return "Consulted other supply source, therapy still appropriate"; 12750 case _5: 12751 return "Consulted prescriber, therapy confirmed"; 12752 case _6: 12753 return "Consulted prescriber and recommended change, prescriber declined"; 12754 case _7: 12755 return "Concurrent therapy triggering alert is no longer on-going or planned"; 12756 case _14: 12757 return "Confirmed supply action appropriate"; 12758 case _15: 12759 return "Patient's existing supply was lost/wasted"; 12760 case _16: 12761 return "Supply date is due to patient vacation"; 12762 case _17: 12763 return "Supply date is intended to carry patient over weekend"; 12764 case _18: 12765 return "Supply is intended for use during a leave of absence from an institution."; 12766 case _20: 12767 return "Description: Supply is different than expected as an additional quantity has been supplied in a separate dispense."; 12768 case _8: 12769 return "Order is performed as issued, but other action taken to mitigate potential adverse effects"; 12770 case _10: 12771 return "Provided education or training to the patient on appropriate therapy use"; 12772 case _11: 12773 return "Instituted an additional therapy to mitigate potential negative effects"; 12774 case _12: 12775 return "Suspended existing therapy that triggered interaction for the duration of this therapy"; 12776 case _13: 12777 return "Aborted existing therapy that triggered interaction."; 12778 case _9: 12779 return "Arranged to monitor patient for adverse effects"; 12780 case _ACTEXPOSURECODE: 12781 return "Concepts that identify the type or nature of exposure interaction. Examples include \"household\", \"care giver\", \"intimate partner\", \"common space\", \"common substance\", etc. to further describe the nature of interaction."; 12782 case CHLDCARE: 12783 return "Description: Exposure participants' interaction occurred in a child care setting"; 12784 case CONVEYNC: 12785 return "Description: An interaction where the exposure participants traveled in/on the same vehicle (not necessarily concurrently, e.g. both are passengers of the same plane, but on different flights of that plane)."; 12786 case HLTHCARE: 12787 return "Description: Exposure participants' interaction occurred during the course of health care delivery or in a health care delivery setting, but did not involve the direct provision of care (e.g. a janitor cleaning a patient's hospital room)."; 12788 case HOMECARE: 12789 return "Description: Exposure interaction occurred in context of one providing care for the other, i.e. a babysitter providing care for a child, a home-care aide providing assistance to a paraplegic."; 12790 case HOSPPTNT: 12791 return "Description: Exposure participants' interaction occurred when both were patients being treated in the same (acute) health care delivery facility."; 12792 case HOSPVSTR: 12793 return "Description: Exposure participants' interaction occurred when one visited the other who was a patient being treated in a health care delivery facility."; 12794 case HOUSEHLD: 12795 return "Description: Exposure interaction occurred in context of domestic interaction, i.e. both participants reside in the same household."; 12796 case INMATE: 12797 return "Description: Exposure participants' interaction occurred in the course of one or both participants being incarcerated at a correctional facility"; 12798 case INTIMATE: 12799 return "Description: Exposure interaction was intimate, i.e. participants are intimate companions (e.g. spouses, domestic partners)."; 12800 case LTRMCARE: 12801 return "Description: Exposure participants' interaction occurred in the course of one or both participants being resident at a long term care facility (second participant may be a visitor, worker, resident or a physical place or object within the facility)."; 12802 case PLACE: 12803 return "Description: An interaction where the exposure participants were both present in the same location/place/space."; 12804 case PTNTCARE: 12805 return "Description: Exposure participants' interaction occurred during the course of health care delivery by a provider (e.g. a physician treating a patient in her office)."; 12806 case SCHOOL2: 12807 return "Description: Exposure participants' interaction occurred in an academic setting (e.g., participants are fellow students, or student and teacher)."; 12808 case SOCIAL2: 12809 return "Description: An interaction where the exposure participants are social associates or members of the same extended family"; 12810 case SUBSTNCE: 12811 return "Description: An interaction where the exposure participants shared or co-used a common substance (e.g. drugs, needles, or common food item)."; 12812 case TRAVINT: 12813 return "Description: An interaction where the exposure participants traveled together in/on the same vehicle/trip (e.g. concurrent co-passengers)."; 12814 case WORK2: 12815 return "Description: Exposure interaction occurred in a work setting, i.e. participants are co-workers."; 12816 case _ACTFINANCIALTRANSACTIONCODE: 12817 return "ActFinancialTransactionCode"; 12818 case CHRG: 12819 return "A type of transaction that represents a charge for a service or product. Expressed in monetary terms."; 12820 case REV: 12821 return "A type of transaction that represents a reversal of a previous charge for a service or product. Expressed in monetary terms. It has the opposite effect of a standard charge."; 12822 case _ACTINCIDENTCODE: 12823 return "Set of codes indicating the type of incident or accident."; 12824 case MVA: 12825 return "Incident or accident as the result of a motor vehicle accident"; 12826 case SCHOOL: 12827 return "Incident or accident is the result of a school place accident."; 12828 case SPT: 12829 return "Incident or accident is the result of a sporting accident."; 12830 case WPA: 12831 return "Incident or accident is the result of a work place accident"; 12832 case _ACTINFORMATIONACCESSCODE: 12833 return "Description: The type of health information to which the subject of the information or the subject's delegate consents or dissents."; 12834 case ACADR: 12835 return "Description: Provide consent to collect, use, disclose, or access adverse drug reaction information for a patient."; 12836 case ACALL: 12837 return "Description: Provide consent to collect, use, disclose, or access all information for a patient."; 12838 case ACALLG: 12839 return "Description: Provide consent to collect, use, disclose, or access allergy information for a patient."; 12840 case ACCONS: 12841 return "Description: Provide consent to collect, use, disclose, or access informational consent information for a patient."; 12842 case ACDEMO: 12843 return "Description: Provide consent to collect, use, disclose, or access demographics information for a patient."; 12844 case ACDI: 12845 return "Description: Provide consent to collect, use, disclose, or access diagnostic imaging information for a patient."; 12846 case ACIMMUN: 12847 return "Description: Provide consent to collect, use, disclose, or access immunization information for a patient."; 12848 case ACLAB: 12849 return "Description: Provide consent to collect, use, disclose, or access lab test result information for a patient."; 12850 case ACMED: 12851 return "Description: Provide consent to collect, use, disclose, or access medical condition information for a patient."; 12852 case ACMEDC: 12853 return "Definition: Provide consent to view or access medical condition information for a patient."; 12854 case ACMEN: 12855 return "Description:Provide consent to collect, use, disclose, or access mental health information for a patient."; 12856 case ACOBS: 12857 return "Description: Provide consent to collect, use, disclose, or access common observation information for a patient."; 12858 case ACPOLPRG: 12859 return "Description: Provide consent to collect, use, disclose, or access coverage policy or program for a patient."; 12860 case ACPROV: 12861 return "Description: Provide consent to collect, use, disclose, or access provider information for a patient."; 12862 case ACPSERV: 12863 return "Description: Provide consent to collect, use, disclose, or access professional service information for a patient."; 12864 case ACSUBSTAB: 12865 return "Description:Provide consent to collect, use, disclose, or access substance abuse information for a patient."; 12866 case _ACTINFORMATIONACCESSCONTEXTCODE: 12867 return "Concepts conveying the context in which authorization given under jurisdictional law, by organizational policy, or by a patient consent directive permits the collection, access, use or disclosure of specified patient health information."; 12868 case INFAUT: 12869 return "Authorization to collect, access, use, or disclose specified patient health information in accordance with jurisdictional law, organizational policy, or a patient's consent directive, which may be implied, deemed, opt-in, opt-out, or explicit."; 12870 case INFCON: 12871 return "Authorization to collect, access, use, or disclose specified patient health information as explicitly consented to by the subject of the information or the subject's representative."; 12872 case INFCRT: 12873 return "Authorization to collect, access, use, or disclose specified patient health information in accordance with judicial system protocol, such as in the case of a subpoena or court order."; 12874 case INFDNG: 12875 return "Authorization to collect, access, use, or disclose specified patient health information where deemed necessary to avert potential danger to other persons in accordance with jurisdictional law, organizational policy, or standards of practice. For example, disclosure about a person threatening violence."; 12876 case INFEMER: 12877 return "Authorization to collect, access, use, or disclose specified patient health information in accordance with emergency information transfer protocol dictated by jurisdictional law, organization policy, or standards of practice. For example, sharing of health information during disaster response."; 12878 case INFPWR: 12879 return "Authorization to collect, access, use, or disclose specified patient health information necessary to avert potential public welfare risk in accordance with jurisdictional law, organizational policy, or standards of practice. For example, reporting that a person is a victim of abuse or demonstrating suicidal tendencies."; 12880 case INFREG: 12881 return "Authorization to collect, access, use, or disclose specified patient health information for public health, welfare, and safety purposes in accordance with jurisdictional law, organizational policy, or standards of practice. For example, public health reporting of notifiable conditions."; 12882 case _ACTINFORMATIONCATEGORYCODE: 12883 return "Definition:Indicates the set of information types which may be manipulated or referenced, such as for recommending access restrictions."; 12884 case ALLCAT: 12885 return "Description: All patient information."; 12886 case ALLGCAT: 12887 return "Definition:All information pertaining to a patient's allergy and intolerance records."; 12888 case ARCAT: 12889 return "Description: All information pertaining to a patient's adverse drug reactions."; 12890 case COBSCAT: 12891 return "Definition:All information pertaining to a patient's common observation records (height, weight, blood pressure, temperature, etc.)."; 12892 case DEMOCAT: 12893 return "Definition:All information pertaining to a patient's demographics (such as name, date of birth, gender, address, etc)."; 12894 case DICAT: 12895 return "Definition:All information pertaining to a patient's diagnostic image records (orders & results)."; 12896 case IMMUCAT: 12897 return "Definition:All information pertaining to a patient's vaccination records."; 12898 case LABCAT: 12899 return "Description: All information pertaining to a patient's lab test records (orders & results)"; 12900 case MEDCCAT: 12901 return "Definition:All information pertaining to a patient's medical condition records."; 12902 case MENCAT: 12903 return "Description: All information pertaining to a patient's mental health records."; 12904 case PSVCCAT: 12905 return "Definition:All information pertaining to a patient's professional service records (such as smoking cessation, counseling, medication review, mental health)."; 12906 case RXCAT: 12907 return "Definition:All information pertaining to a patient's medication records (orders, dispenses and other active medications)."; 12908 case _ACTINVOICEELEMENTCODE: 12909 return "Type of invoice element that is used to assist in describing an Invoice that is either submitted for adjudication or for which is returned on adjudication results."; 12910 case _ACTINVOICEADJUDICATIONPAYMENTCODE: 12911 return "Codes representing a grouping of invoice elements (totals, sub-totals), reported through a Payment Advice or a Statement of Financial Activity (SOFA). The code can represent summaries by day, location, payee and other cost elements such as bonus, retroactive adjustment and transaction fees."; 12912 case _ACTINVOICEADJUDICATIONPAYMENTGROUPCODE: 12913 return "Codes representing adjustments to a Payment Advice such as retroactive, clawback, garnishee, etc."; 12914 case ALEC: 12915 return "Payment initiated by the payor as the result of adjudicating a submitted invoice that arrived to the payor from an electronic source that did not provide a conformant set of HL7 messages (e.g. web claim submission)."; 12916 case BONUS: 12917 return "Bonus payments based on performance, volume, etc. as agreed to by the payor."; 12918 case CFWD: 12919 return "An amount still owing to the payor but the payment is 0$ and this cannot be settled until a future payment is made."; 12920 case EDU: 12921 return "Fees deducted on behalf of a payee for tuition and continuing education."; 12922 case EPYMT: 12923 return "Fees deducted on behalf of a payee for charges based on a shorter payment frequency (i.e. next day versus biweekly payments."; 12924 case GARN: 12925 return "Fees deducted on behalf of a payee for charges based on a per-transaction or time-period (e.g. monthly) fee."; 12926 case INVOICE: 12927 return "Payment is based on a payment intent for a previously submitted Invoice, based on formal adjudication results.."; 12928 case PINV: 12929 return "Payment initiated by the payor as the result of adjudicating a paper (original, may have been faxed) invoice."; 12930 case PPRD: 12931 return "An amount that was owed to the payor as indicated, by a carry forward adjusment, in a previous payment advice"; 12932 case PROA: 12933 return "Professional association fee that is collected by the payor from the practitioner/provider on behalf of the association"; 12934 case RECOV: 12935 return "Retroactive adjustment such as fee rate adjustment due to contract negotiations."; 12936 case RETRO: 12937 return "Bonus payments based on performance, volume, etc. as agreed to by the payor."; 12938 case TRAN: 12939 return "Fees deducted on behalf of a payee for charges based on a per-transaction or time-period (e.g. monthly) fee."; 12940 case _ACTINVOICEADJUDICATIONPAYMENTSUMMARYCODE: 12941 return "Codes representing a grouping of invoice elements (totals, sub-totals), reported through a Payment Advice or a Statement of Financial Activity (SOFA). The code can represent summaries by day, location, payee, etc."; 12942 case INVTYPE: 12943 return "Transaction counts and value totals by invoice type (e.g. RXDINV - Pharmacy Dispense)"; 12944 case PAYEE: 12945 return "Transaction counts and value totals by each instance of an invoice payee."; 12946 case PAYOR: 12947 return "Transaction counts and value totals by each instance of an invoice payor."; 12948 case SENDAPP: 12949 return "Transaction counts and value totals by each instance of a messaging application on a single processor. It is a registered identifier known to the receivers."; 12950 case _ACTINVOICEDETAILCODE: 12951 return "Codes representing a service or product that is being invoiced (billed). The code can represent such concepts as \"office visit\", \"drug X\", \"wheelchair\" and other billable items such as taxes, service charges and discounts."; 12952 case _ACTINVOICEDETAILCLINICALPRODUCTCODE: 12953 return "An identifying data string for healthcare products."; 12954 case UNSPSC: 12955 return "Description:United Nations Standard Products and Services Classification, managed by Uniform Code Council (UCC): www.unspsc.org"; 12956 case _ACTINVOICEDETAILDRUGPRODUCTCODE: 12957 return "An identifying data string for A substance used as a medication or in the preparation of medication."; 12958 case GTIN: 12959 return "Description:Global Trade Item Number is an identifier for trade items developed by GS1 (comprising the former EAN International and Uniform Code Council)."; 12960 case UPC: 12961 return "Description:Universal Product Code is one of a wide variety of bar code languages widely used in the United States and Canada for items in stores."; 12962 case _ACTINVOICEDETAILGENERICCODE: 12963 return "The detail item codes to identify charges or changes to the total billing of a claim due to insurance rules and payments."; 12964 case _ACTINVOICEDETAILGENERICADJUDICATORCODE: 12965 return "The billable item codes to identify adjudicator specified components to the total billing of a claim."; 12966 case COIN: 12967 return "That portion of the eligible charges which a covered party must pay for each service and/or product. It is a percentage of the eligible amount for the service/product that is typically charged after the covered party has met the policy deductible. This amount represents the covered party's coinsurance that is applied to a particular adjudication result. It is expressed as a negative dollar amount in adjudication results."; 12968 case COPAYMENT: 12969 return "That portion of the eligible charges which a covered party must pay for each service and/or product. It is a defined amount per service/product of the eligible amount for the service/product. This amount represents the covered party's copayment that is applied to a particular adjudication result. It is expressed as a negative dollar amount in adjudication results."; 12970 case DEDUCTIBLE: 12971 return "That portion of the eligible charges which a covered party must pay in a particular period (e.g. annual) before the benefits are payable by the adjudicator. This amount represents the covered party's deductible that is applied to a particular adjudication result. It is expressed as a negative dollar amount in adjudication results."; 12972 case PAY: 12973 return "The guarantor, who may be the patient, pays the entire charge for a service. Reasons for such action may include: there is no insurance coverage for the service (e.g. cosmetic surgery); the patient wishes to self-pay for the service; or the insurer denies payment for the service due to contractual provisions such as the need for prior authorization."; 12974 case SPEND: 12975 return "That total amount of the eligible charges which a covered party must periodically pay for services and/or products prior to the Medicaid program providing any coverage. This amount represents the covered party's spend down that is applied to a particular adjudication result. It is expressed as a negative dollar amount in adjudication results"; 12976 case COINS: 12977 return "The covered party pays a percentage of the cost of covered services."; 12978 case _ACTINVOICEDETAILGENERICMODIFIERCODE: 12979 return "The billable item codes to identify modifications to a billable item charge. As for example after hours increase in the office visit fee."; 12980 case AFTHRS: 12981 return "Premium paid on service fees in compensation for practicing outside of normal working hours."; 12982 case ISOL: 12983 return "Premium paid on service fees in compensation for practicing in a remote location."; 12984 case OOO: 12985 return "Premium paid on service fees in compensation for practicing at a location other than normal working location."; 12986 case _ACTINVOICEDETAILGENERICPROVIDERCODE: 12987 return "The billable item codes to identify provider supplied charges or changes to the total billing of a claim."; 12988 case CANCAPT: 12989 return "A charge to compensate the provider when a patient cancels an appointment with insufficient time for the provider to make another appointment with another patient."; 12990 case DSC: 12991 return "A reduction in the amount charged as a percentage of the amount. For example a 5% discount for volume purchase."; 12992 case ESA: 12993 return "A premium on a service fee is requested because, due to extenuating circumstances, the service took an extraordinary amount of time or supplies."; 12994 case FFSTOP: 12995 return "Under agreement between the parties (payor and provider), a guaranteed level of income is established for the provider over a specific, pre-determined period of time. The normal course of business for the provider is submission of fee-for-service claims. Should the fee-for-service income during the specified period of time be less than the agreed to amount, a top-up amount is paid to the provider equal to the difference between the fee-for-service total and the guaranteed income amount for that period of time. The details of the agreement may specify (or not) a requirement for repayment to the payor in the event that the fee-for-service income exceeds the guaranteed amount."; 12996 case FNLFEE: 12997 return "Anticipated or actual final fee associated with treating a patient."; 12998 case FRSTFEE: 12999 return "Anticipated or actual initial fee associated with treating a patient."; 13000 case MARKUP: 13001 return "An increase in the amount charged as a percentage of the amount. For example, 12% markup on product cost."; 13002 case MISSAPT: 13003 return "A charge to compensate the provider when a patient does not show for an appointment."; 13004 case PERFEE: 13005 return "Anticipated or actual periodic fee associated with treating a patient. For example, expected billing cycle such as monthly, quarterly. The actual period (e.g. monthly, quarterly) is specified in the unit quantity of the Invoice Element."; 13006 case PERMBNS: 13007 return "The amount for a performance bonus that is being requested from a payor for the performance of certain services (childhood immunizations, influenza immunizations, mammograms, pap smears) on a sliding scale. That is, for 90% of childhood immunizations to a maximum of $2200/yr. An invoice is created at the end of the service period (one year) and a code is submitted indicating the percentage achieved and the dollar amount claimed."; 13008 case RESTOCK: 13009 return "A charge is requested because the patient failed to pick up the item and it took an amount of time to return it to stock for future use."; 13010 case TRAVEL: 13011 return "A charge to cover the cost of travel time and/or cost in conjuction with providing a service or product. It may be charged per kilometer or per hour based on the effective agreement."; 13012 case URGENT: 13013 return "Premium paid on service fees in compensation for providing an expedited response to an urgent situation."; 13014 case _ACTINVOICEDETAILTAXCODE: 13015 return "The billable item codes to identify modifications to a billable item charge by a tax factor applied to the amount. As for example 7% provincial sales tax."; 13016 case FST: 13017 return "Federal tax on transactions such as the Goods and Services Tax (GST)"; 13018 case HST: 13019 return "Joint Federal/Provincial Sales Tax"; 13020 case PST: 13021 return "Tax levied by the provincial or state jurisdiction such as Provincial Sales Tax"; 13022 case _ACTINVOICEDETAILPREFERREDACCOMMODATIONCODE: 13023 return "An identifying data string for medical facility accommodations."; 13024 case _ACTENCOUNTERACCOMMODATIONCODE: 13025 return "Accommodation type. In Intent mood, represents the accommodation type requested. In Event mood, represents accommodation assigned/used. In Definition mood, represents the available accommodation type."; 13026 case _HL7ACCOMMODATIONCODE: 13027 return "Description:Accommodation type. In Intent mood, represents the accommodation type requested. In Event mood, represents accommodation assigned/used. In Definition mood, represents the available accommodation type."; 13028 case I: 13029 return "Accommodations used in the care of diseases that are transmitted through casual contact or respiratory transmission."; 13030 case P: 13031 return "Accommodations in which there is only 1 bed."; 13032 case S: 13033 return "Uniquely designed and elegantly decorated accommodations with many amenities available for an additional charge."; 13034 case SP: 13035 return "Accommodations in which there are 2 beds."; 13036 case W: 13037 return "Accommodations in which there are 3 or more beds."; 13038 case _ACTINVOICEDETAILCLINICALSERVICECODE: 13039 return "An identifying data string for healthcare procedures."; 13040 case _ACTINVOICEGROUPCODE: 13041 return "Type of invoice element that is used to assist in describing an Invoice that is either submitted for adjudication or for which is returned on adjudication results.\r\n\n Invoice elements of this type signify a grouping of one or more children (detail) invoice elements. They do not have intrinsic costing associated with them, but merely reflect the sum of all costing for it's immediate children invoice elements."; 13042 case _ACTINVOICEINTERGROUPCODE: 13043 return "Type of invoice element that is used to assist in describing an Invoice that is either submitted for adjudication or for which is returned on adjudication results.\r\n\n Invoice elements of this type signify a grouping of one or more children (detail) invoice elements. They do not have intrinsic costing associated with them, but merely reflect the sum of all costing for it's immediate children invoice elements.\r\n\n The domain is only specified for an intermediate invoice element group (non-root or non-top level) for an Invoice."; 13044 case CPNDDRGING: 13045 return "A grouping of invoice element groups and details including the ones specifying the compound ingredients being invoiced. It may also contain generic detail items such as markup."; 13046 case CPNDINDING: 13047 return "A grouping of invoice element details including the one specifying an ingredient drug being invoiced. It may also contain generic detail items such as tax or markup."; 13048 case CPNDSUPING: 13049 return "A grouping of invoice element groups and details including the ones specifying the compound supplies being invoiced. It may also contain generic detail items such as markup."; 13050 case DRUGING: 13051 return "A grouping of invoice element details including the one specifying the drug being invoiced. It may also contain generic detail items such as markup."; 13052 case FRAMEING: 13053 return "A grouping of invoice element details including the ones specifying the frame fee and the frame dispensing cost that are being invoiced."; 13054 case LENSING: 13055 return "A grouping of invoice element details including the ones specifying the lens fee and the lens dispensing cost that are being invoiced."; 13056 case PRDING: 13057 return "A grouping of invoice element details including the one specifying the product (good or supply) being invoiced. It may also contain generic detail items such as tax or discount."; 13058 case _ACTINVOICEROOTGROUPCODE: 13059 return "Type of invoice element that is used to assist in describing an Invoice that is either submitted for adjudication or for which is returned on adjudication results.\r\n\n Invoice elements of this type signify a grouping of one or more children (detail) invoice elements. They do not have intrinsic costing associated with them, but merely reflect the sum of all costing for it's immediate children invoice elements.\r\n\n Codes from this domain reflect the type of Invoice such as Pharmacy Dispense, Clinical Service and Clinical Product. The domain is only specified for the root (top level) invoice element group for an Invoice."; 13060 case CPINV: 13061 return "Clinical product invoice where the Invoice Grouping contains one or more billable item and is supported by clinical product(s).\r\n\n For example, a crutch or a wheelchair."; 13062 case CSINV: 13063 return "Clinical Services Invoice which can be used to describe a single service, multiple services or repeated services.\r\n\n [1] Single Clinical services invoice where the Invoice Grouping contains one billable item and is supported by one clinical service.\r\n\n For example, a single service for an office visit or simple clinical procedure (e.g. knee mobilization).\r\n\n [2] Multiple Clinical services invoice where the Invoice Grouping contains more than one billable item, supported by one or more clinical services. The services can be distinct and over multiple dates, but for the same patient. This type of invoice includes a series of treatments which must be adjudicated together.\r\n\n For example, an adjustment and ultrasound for a chiropractic session where fees are associated for each of the services and adjudicated (invoiced) together.\r\n\n [3] Repeated Clinical services invoice where the Invoice Grouping contains one or more billable item, supported by the same clinical service repeated over a period of time.\r\n\n For example, the same Chiropractic adjustment (service or treatment) delivered on 3 separate occasions over a period of time at the discretion of the provider (e.g. month)."; 13064 case CSPINV: 13065 return "A clinical Invoice Grouping consisting of one or more services and one or more product. Billing for these service(s) and product(s) are supported by multiple clinical billable events (acts).\r\n\n All items in the Invoice Grouping must be adjudicated together to be acceptable to the Adjudicator.\r\n\n For example , a brace (product) invoiced together with the fitting (service)."; 13066 case FININV: 13067 return "Invoice Grouping without clinical justification. These will not require identification of participants and associations from a clinical context such as patient and provider.\r\n\n Examples are interest charges and mileage."; 13068 case OHSINV: 13069 return "A clinical Invoice Grouping consisting of one or more oral health services. Billing for these service(s) are supported by multiple clinical billable events (acts).\r\n\n All items in the Invoice Grouping must be adjudicated together to be acceptable to the Adjudicator."; 13070 case PAINV: 13071 return "HealthCare facility preferred accommodation invoice."; 13072 case RXCINV: 13073 return "Pharmacy dispense invoice for a compound."; 13074 case RXDINV: 13075 return "Pharmacy dispense invoice not involving a compound"; 13076 case SBFINV: 13077 return "Clinical services invoice where the Invoice Group contains one billable item for multiple clinical services in one or more sessions."; 13078 case VRXINV: 13079 return "Vision dispense invoice for up to 2 lens (left and right), frame and optional discount. Eye exams are invoiced as a clinical service invoice."; 13080 case _ACTINVOICEELEMENTSUMMARYCODE: 13081 return "Identifies the different types of summary information that can be reported by queries dealing with Statement of Financial Activity (SOFA). The summary information is generally used to help resolve balance discrepancies between providers and payors."; 13082 case _INVOICEELEMENTADJUDICATED: 13083 return "Total counts and total net amounts adjudicated for all Invoice Groupings that were adjudicated within a time period based on the adjudication date of the Invoice Grouping."; 13084 case ADNFPPELAT: 13085 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted electronically."; 13086 case ADNFPPELCT: 13087 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted electronically."; 13088 case ADNFPPMNAT: 13089 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted manually."; 13090 case ADNFPPMNCT: 13091 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted manually."; 13092 case ADNFSPELAT: 13093 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date), subsequently nullified in the specified period and submitted electronically."; 13094 case ADNFSPELCT: 13095 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date), subsequently nullified in the specified period and submitted electronically."; 13096 case ADNFSPMNAT: 13097 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted manually."; 13098 case ADNFSPMNCT: 13099 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date), subsequently cancelled in the specified period and submitted manually."; 13100 case ADNPPPELAT: 13101 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13102 case ADNPPPELCT: 13103 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13104 case ADNPPPMNAT: 13105 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13106 case ADNPPPMNCT: 13107 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13108 case ADNPSPELAT: 13109 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13110 case ADNPSPELCT: 13111 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13112 case ADNPSPMNAT: 13113 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13114 case ADNPSPMNCT: 13115 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13116 case ADPPPPELAT: 13117 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13118 case ADPPPPELCT: 13119 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13120 case ADPPPPMNAT: 13121 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted manually."; 13122 case ADPPPPMNCT: 13123 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable prior to the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted manually."; 13124 case ADPPSPELAT: 13125 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13126 case ADPPSPELCT: 13127 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13128 case ADPPSPMNAT: 13129 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted manually."; 13130 case ADPPSPMNCT: 13131 return "Identifies the total number of all Invoice Groupings that were adjudicated as payable during the specified time period (based on adjudication date) that match a specified payee (e.g. pay provider) and submitted manually."; 13132 case ADRFPPELAT: 13133 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as refused prior to the specified time period (based on adjudication date) and submitted electronically."; 13134 case ADRFPPELCT: 13135 return "Identifies the total number of all Invoice Groupings that were adjudicated as refused prior to the specified time period (based on adjudication date) and submitted electronically."; 13136 case ADRFPPMNAT: 13137 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as refused prior to the specified time period (based on adjudication date) and submitted manually."; 13138 case ADRFPPMNCT: 13139 return "Identifies the total number of all Invoice Groupings that were adjudicated as refused prior to the specified time period (based on adjudication date) and submitted manually."; 13140 case ADRFSPELAT: 13141 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as refused during the specified time period (based on adjudication date) and submitted electronically."; 13142 case ADRFSPELCT: 13143 return "Identifies the total number of all Invoice Groupings that were adjudicated as refused during the specified time period (based on adjudication date) and submitted electronically."; 13144 case ADRFSPMNAT: 13145 return "Identifies the total net amount of all Invoice Groupings that were adjudicated as refused during the specified time period (based on adjudication date) and submitted manually."; 13146 case ADRFSPMNCT: 13147 return "Identifies the total number of all Invoice Groupings that were adjudicated as refused during the specified time period (based on adjudication date) and submitted manually."; 13148 case _INVOICEELEMENTPAID: 13149 return "Total counts and total net amounts paid for all Invoice Groupings that were paid within a time period based on the payment date."; 13150 case PDNFPPELAT: 13151 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date), subsequently nullified in the specified period and submitted electronically."; 13152 case PDNFPPELCT: 13153 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date), subsequently nullified in the specified period and submitted electronically."; 13154 case PDNFPPMNAT: 13155 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date), subsequently nullified in the specified period and submitted manually."; 13156 case PDNFPPMNCT: 13157 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date), subsequently nullified in the specified period and submitted manually."; 13158 case PDNFSPELAT: 13159 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date), subsequently nullified in the specified period and submitted electronically."; 13160 case PDNFSPELCT: 13161 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date), subsequently cancelled in the specified period and submitted electronically."; 13162 case PDNFSPMNAT: 13163 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date), subsequently nullified in the specified period and submitted manually."; 13164 case PDNFSPMNCT: 13165 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date), subsequently nullified in the specified period and submitted manually."; 13166 case PDNPPPELAT: 13167 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13168 case PDNPPPELCT: 13169 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13170 case PDNPPPMNAT: 13171 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13172 case PDNPPPMNCT: 13173 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13174 case PDNPSPELAT: 13175 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13176 case PDNPSPELCT: 13177 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted electronically."; 13178 case PDNPSPMNAT: 13179 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13180 case PDNPSPMNCT: 13181 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date) that do not match a specified payee (e.g. pay patient) and submitted manually."; 13182 case PDPPPPELAT: 13183 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13184 case PDPPPPELCT: 13185 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13186 case PDPPPPMNAT: 13187 return "Identifies the total net amount of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted manually."; 13188 case PDPPPPMNCT: 13189 return "Identifies the total number of all Invoice Groupings that were paid prior to the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted manually."; 13190 case PDPPSPELAT: 13191 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13192 case PDPPSPELCT: 13193 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted electronically."; 13194 case PDPPSPMNAT: 13195 return "Identifies the total net amount of all Invoice Groupings that were paid during the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted manually."; 13196 case PDPPSPMNCT: 13197 return "Identifies the total number of all Invoice Groupings that were paid during the specified time period (based on payment date) that match a specified payee (e.g. pay provider) and submitted manually."; 13198 case _INVOICEELEMENTSUBMITTED: 13199 return "Total counts and total net amounts billed for all Invoice Groupings that were submitted within a time period. Adjudicated invoice elements are included."; 13200 case SBBLELAT: 13201 return "Identifies the total net amount billed for all submitted Invoice Groupings within a time period and submitted electronically. Adjudicated invoice elements are included."; 13202 case SBBLELCT: 13203 return "Identifies the total number of submitted Invoice Groupings within a time period and submitted electronically. Adjudicated invoice elements are included."; 13204 case SBNFELAT: 13205 return "Identifies the total net amount billed for all submitted Invoice Groupings that were nullified within a time period and submitted electronically. Adjudicated invoice elements are included."; 13206 case SBNFELCT: 13207 return "Identifies the total number of submitted Invoice Groupings that were nullified within a time period and submitted electronically. Adjudicated invoice elements are included."; 13208 case SBPDELAT: 13209 return "Identifies the total net amount billed for all submitted Invoice Groupings that are pended or held by the payor, within a time period and submitted electronically. Adjudicated invoice elements are not included."; 13210 case SBPDELCT: 13211 return "Identifies the total number of submitted Invoice Groupings that are pended or held by the payor, within a time period and submitted electronically. Adjudicated invoice elements are not included."; 13212 case _ACTINVOICEOVERRIDECODE: 13213 return "Includes coded responses that will occur as a result of the adjudication of an electronic invoice at a summary level and provides guidance on interpretation of the referenced adjudication results."; 13214 case COVGE: 13215 return "Insurance coverage problems have been encountered. Additional explanation information to be supplied."; 13216 case EFORM: 13217 return "Electronic form with supporting or additional information to follow."; 13218 case FAX: 13219 return "Fax with supporting or additional information to follow."; 13220 case GFTH: 13221 return "The medical service was provided to a patient in good faith that they had medical coverage, although no evidence of coverage was available before service was rendered."; 13222 case LATE: 13223 return "Knowingly over the payor's published time limit for this invoice possibly due to a previous payor's delays in processing. Additional reason information will be supplied."; 13224 case MANUAL: 13225 return "Manual review of the invoice is requested. Additional information to be supplied. This may be used in the case of an appeal."; 13226 case OOJ: 13227 return "The medical service and/or product was provided to a patient that has coverage in another jurisdiction."; 13228 case ORTHO: 13229 return "The service provided is required for orthodontic purposes. If the covered party has orthodontic coverage, then the service may be paid."; 13230 case PAPER: 13231 return "Paper documentation (or other physical format) with supporting or additional information to follow."; 13232 case PIE: 13233 return "Public Insurance has been exhausted. Invoice has not been sent to Public Insuror and therefore no Explanation Of Benefits (EOB) is provided with this Invoice submission."; 13234 case PYRDELAY: 13235 return "Allows provider to explain lateness of invoice to a subsequent payor."; 13236 case REFNR: 13237 return "Rules of practice do not require a physician's referral for the provider to perform a billable service."; 13238 case REPSERV: 13239 return "The same service was delivered within a time period that would usually indicate a duplicate billing. However, the repeated service is a medical necessity and therefore not a duplicate."; 13240 case UNRELAT: 13241 return "The service provided is not related to another billed service. For example, 2 unrelated services provided on the same day to the same patient which may normally result in a refused payment for one of the items."; 13242 case VERBAUTH: 13243 return "The provider has received a verbal permission from an authoritative source to perform the service or supply the item being invoiced."; 13244 case _ACTLISTCODE: 13245 return "Provides codes associated with ActClass value of LIST (working list)"; 13246 case _ACTOBSERVATIONLIST: 13247 return "ActObservationList"; 13248 case CARELIST: 13249 return "List of acts representing a care plan. The acts can be in a varierty of moods including event (EVN) to record acts that have been carried out as part of the care plan."; 13250 case CONDLIST: 13251 return "List of condition observations."; 13252 case INTOLIST: 13253 return "List of intolerance observations."; 13254 case PROBLIST: 13255 return "List of problem observations."; 13256 case RISKLIST: 13257 return "List of risk factor observations."; 13258 case GOALLIST: 13259 return "List of observations in goal mood."; 13260 case _ACTTHERAPYDURATIONWORKINGLISTCODE: 13261 return "Codes used to identify different types of 'duration-based' working lists. Examples include \"Continuous/Chronic\", \"Short-Term\" and \"As-Needed\"."; 13262 case _ACTMEDICATIONTHERAPYDURATIONWORKINGLISTCODE: 13263 return "Definition:A collection of concepts that identifies different types of 'duration-based' mediation working lists.\r\n\n \n Examples:\"Continuous/Chronic\" \"Short-Term\" and \"As Needed\""; 13264 case ACU: 13265 return "Definition:A list of medications which the patient is only expected to consume for the duration of the current order or limited set of orders and which is not expected to be renewed."; 13266 case CHRON: 13267 return "Definition:A list of medications which are expected to be continued beyond the present order and which the patient should be assumed to be taking unless explicitly stopped."; 13268 case ONET: 13269 return "Definition:A list of medications which the patient is intended to be administered only once."; 13270 case PRN: 13271 return "Definition:A list of medications which the patient will consume intermittently based on the behavior of the condition for which the medication is indicated."; 13272 case MEDLIST: 13273 return "List of medications."; 13274 case CURMEDLIST: 13275 return "List of current medications."; 13276 case DISCMEDLIST: 13277 return "List of discharge medications."; 13278 case HISTMEDLIST: 13279 return "Historical list of medications."; 13280 case _ACTMONITORINGPROTOCOLCODE: 13281 return "Identifies types of monitoring programs"; 13282 case CTLSUB: 13283 return "A monitoring program that focuses on narcotics and/or commonly abused substances that are subject to legal restriction."; 13284 case INV: 13285 return "Definition:A monitoring program that focuses on a drug which is under investigation and has not received regulatory approval for the condition being investigated"; 13286 case LU: 13287 return "Description:A drug that can be prescribed (and reimbursed) only if it meets certain criteria."; 13288 case OTC: 13289 return "Medicines designated in this way may be supplied for patient use without a prescription. The exact form of categorisation will vary in different realms."; 13290 case RX: 13291 return "Some form of prescription is required before the related medicine can be supplied for a patient. The exact form of regulation will vary in different realms."; 13292 case SA: 13293 return "Definition:A drug that requires prior approval (to be reimbursed) before being dispensed"; 13294 case SAC: 13295 return "Description:A drug that requires special access permission to be prescribed and dispensed."; 13296 case _ACTNONOBSERVATIONINDICATIONCODE: 13297 return "Description:Concepts representing indications (reasons for clinical action) other than diagnosis and symptoms."; 13298 case IND01: 13299 return "Description:Contrast agent required for imaging study."; 13300 case IND02: 13301 return "Description:Provision of prescription or direction to consume a product for purposes of bowel clearance in preparation for a colonoscopy."; 13302 case IND03: 13303 return "Description:Provision of medication as a preventative measure during a treatment or other period of increased risk."; 13304 case IND04: 13305 return "Description:Provision of medication during pre-operative phase; e.g., antibiotics before dental surgery or bowel prep before colon surgery."; 13306 case IND05: 13307 return "Description:Provision of medication for pregnancy --e.g., vitamins, antibiotic treatments for vaginal tract colonization, etc."; 13308 case _ACTOBSERVATIONVERIFICATIONTYPE: 13309 return "Identifies the type of verification investigation being undertaken with respect to the subject of the verification activity.\r\n\n \n Examples:\n \r\n\n \n \n Verification of eligibility for coverage under a policy or program - aka enrolled/covered by a policy or program\r\n\n \n \n Verification of record - e.g., person has record in an immunization registry\r\n\n \n \n Verification of enumeration - e.g. NPI\r\n\n \n \n Verification of Board Certification - provider specific\r\n\n \n \n Verification of Certification - e.g. JAHCO, NCQA, URAC\r\n\n \n \n Verification of Conformance - e.g. entity use with HIPAA, conformant to the CCHIT EHR system criteria\r\n\n \n \n Verification of Provider Credentials\r\n\n \n \n Verification of no adverse findings - e.g. on National Provider Data Bank, Health Integrity Protection Data Base (HIPDB)"; 13310 case VFPAPER: 13311 return "Definition:Indicates that the paper version of the record has, should be or is being verified against the electronic version."; 13312 case _ACTPAYMENTCODE: 13313 return "Code identifying the method or the movement of payment instructions.\r\n\n Codes are drawn from X12 data element 591 (PaymentMethodCode)"; 13314 case ACH: 13315 return "Automated Clearing House (ACH)."; 13316 case CHK: 13317 return "A written order to a bank to pay the amount specified from funds on deposit."; 13318 case DDP: 13319 return "Electronic Funds Transfer (EFT) deposit into the payee's bank account"; 13320 case NON: 13321 return "Non-Payment Data."; 13322 case _ACTPHARMACYSUPPLYTYPE: 13323 return "Identifies types of dispensing events"; 13324 case DF: 13325 return "A fill providing sufficient supply for one day"; 13326 case EM: 13327 return "A supply action where there is no 'valid' order for the supplied medication. E.g. Emergency vacation supply, weekend supply (when prescriber is unavailable to provide a renewal prescription)"; 13328 case SO: 13329 return "An emergency supply where the expectation is that a formal order authorizing the supply will be provided at a later date."; 13330 case FF: 13331 return "The initial fill against an order. (This includes initial fills against refill orders.)"; 13332 case FFC: 13333 return "A first fill where the quantity supplied is equal to one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill would be for the full 90 tablets)."; 13334 case FFP: 13335 return "A first fill where the quantity supplied is less than one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill might be for only 30 tablets.)"; 13336 case FFSS: 13337 return "A first fill where the strength supplied is less than the ordered strength. (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13338 case TF: 13339 return "A fill where a small portion is provided to allow for determination of the therapy effectiveness and patient tolerance."; 13340 case FS: 13341 return "A supply action to restock a smaller more local dispensary."; 13342 case MS: 13343 return "A supply of a manufacturer sample"; 13344 case RF: 13345 return "A fill against an order that has already been filled (or partially filled) at least once."; 13346 case UD: 13347 return "A supply action that provides sufficient material for a single dose."; 13348 case RFC: 13349 return "A refill where the quantity supplied is equal to one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill would be for the full 90 tablets.)"; 13350 case RFCS: 13351 return "A refill where the quantity supplied is equal to one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a complete fill would be for the full 90 tablets.) and where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13352 case RFF: 13353 return "The first fill against an order that has already been filled at least once at another facility."; 13354 case RFFS: 13355 return "The first fill against an order that has already been filled at least once at another facility and where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13356 case RFP: 13357 return "A refill where the quantity supplied is less than one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill might be for only 30 tablets.)"; 13358 case RFPS: 13359 return "A refill where the quantity supplied is less than one full repetition of the ordered amount. (e.g. If the order was 90 tablets, 3 refills, a partial fill might be for only 30 tablets.) and where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13360 case RFS: 13361 return "A fill against an order that has already been filled (or partially filled) at least once and where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13362 case TB: 13363 return "A fill where the remainder of a 'complete' fill is provided after a trial fill has been provided."; 13364 case TBS: 13365 return "A fill where the remainder of a 'complete' fill is provided after a trial fill has been provided and where the strength supplied is less than the ordered strength (e.g. 10mg for an order of 50mg where a subsequent fill will dispense 40mg tablets)."; 13366 case UDE: 13367 return "A supply action that provides sufficient material for a single dose via multiple products. E.g. 2 50mg tablets for a 100mg unit dose."; 13368 case _ACTPOLICYTYPE: 13369 return "Description:Types of policies that further specify the ActClassPolicy value set."; 13370 case _ACTPRIVACYPOLICY: 13371 return "A policy deeming certain information to be private to an individual or organization.\r\n\n \n Definition: A mandate, obligation, requirement, rule, or expectation relating to privacy.\r\n\n \n Discussion: ActPrivacyPolicyType codes support the designation of the 1..* policies that are applicable to an Act such as a Consent Directive, a Role such as a VIP Patient, or an Entity such as a patient who is a minor. 1..* ActPrivacyPolicyType values may be associated with an Act or Role to indicate the policies that govern the assignment of an Act or Role confidentialityCode. Use of multiple ActPrivacyPolicyType values enables fine grain specification of applicable policies, but must be carefully assigned to ensure cogency and avoid creation of conflicting policy mandates.\r\n\n \n Usage Note: Statutory title may be named in the ActClassPolicy Act Act.title to specify which privacy policy is being referenced."; 13372 case _ACTCONSENTDIRECTIVE: 13373 return "Specifies the type of agreement between one or more grantor and grantee in which rights and obligations related to one or more shared items of interest are allocated.\r\n\n \n Usage Note: Such agreements may be considered \"consent directives\" or \"contracts\" depending on the context, and are considered closely related or synonymous from a legal perspective.\r\n\n \n Examples: \n \r\n\n \n Healthcare Privacy Consent Directive permitting or restricting in whole or part the collection, access, use, and disclosure of health information, and any associated handling caveats.\n Healthcare Medical Consent Directive to receive medical procedures after being informed of risks and benefits, thereby reducing the grantee's liability.\n Research Informed Consent for participation in clinical trials and disclosure of health information after being informed of risks and benefits, thereby reducing the grantee's liability.\n Substitute decision maker delegation in which the grantee assumes responsibility to act on behalf of the grantor.\n Contracts in which the agreement requires assent/dissent by the grantor of terms offered by a grantee, a consumer opts out of an \"award\" system for use of a retailer's marketing or credit card vendor's point collection cards in exchange for allowing purchase tracking and profiling.\n A mobile device or App privacy policy and terms of service to which a user must agree in whole or in part in order to utilize the service.\n Agreements between a client and an authorization server or between an authorization server and a resource operator and/or resource owner permitting or restricting e.g., collection, access, use, and disclosure of information, and any associated handling caveats."; 13374 case EMRGONLY: 13375 return "This general consent directive specifically limits disclosure of health information for purpose of emergency treatment. Additional parameters may further limit the disclosure to specific users, roles, duration, types of information, and impose uses obligations.\r\n\n \n Definition: Opt-in to disclosure of health information for emergency only consent directive."; 13376 case GRANTORCHOICE: 13377 return "A grantor's terms of agreement to which a grantee may assent or dissent, and which may include an opportunity for a grantee to request restrictions or extensions.\r\n\n \n Comment: A grantor typically is able to stipulate preferred terms of agreement when the grantor has control over the topic of the agreement, which a grantee must accept in full or may be offered an opportunity to extend or restrict certain terms.\r\n\n \n Usage Note: If the grantor's term of agreement must be accepted in full, then this is considered \"basic consent\". If a grantee is offered an opportunity to extend or restrict certain terms, then the agreement is considered \"granular consent\".\r\n\n \n Examples: \n \r\n\n \n Healthcare: A PHR account holder [grantor] may require any PHR user [grantee] to accept the terms of agreement in full, or may permit a PHR user to extend or restrict terms selected by the account holder or requested by the PHR user.\n Non-healthcare: The owner of a resource server [grantor] may require any authorization server [grantee] to meet authorization requirements stipulated in the grantor's terms of agreement."; 13378 case IMPLIED: 13379 return "A grantor's presumed assent to the grantee's terms of agreement is based on the grantor's behavior, which may result from not expressly assenting to the consent directive offered, or from having no right to assent or dissent offered by the grantee.\r\n\n \n Comment: Implied or \"implicit\" consent occurs when the behavior of the grantor is understood by a reasonable person to signal agreement to the grantee's terms.\r\n\n \n Usage Note: Implied consent with no opportunity to assent or dissent to certain terms is considered \"basic consent\".\r\n\n \n Examples: \n \r\n\n \n Healthcare: A patient schedules an appointment with a provider, and either does not take the opportunity to expressly assent or dissent to the provider's consent directive, does not have an opportunity to do so, as in the case where emergency care is required, or simply behaves as though the patient [grantor] agrees to the rights granted to the provider [grantee] in an implicit consent directive.\n An injured and unconscious patient is deemed to have assented to emergency treatment by those permitted to do so under jurisdictional laws, e.g., Good Samaritan laws.\n Non-healthcare: Upon receiving a driver's license, the driver is deemed to have assented without explicitly consenting to undergoing field sobriety tests.\n A corporation that does business in a foreign nation is deemed to have deemed to have assented without explicitly consenting to abide by that nation's laws."; 13380 case IMPLIEDD: 13381 return "A grantor's presumed assent to the grantee's terms of agreement, which is based on the grantor's behavior, and includes a right to dissent to certain terms. \r\n\n \n Comment: A grantor assenting to the grantee's terms of agreement may or may not exercise a right to dissent to grantor selected terms or to grantee's selected terms to which a grantor may dissent.\r\n\n \n Usage Note: Implied or \"implicit\" consent with an \"opportunity to dissent\" occurs when the grantor's behavior is understood by a reasonable person to signal assent to the grantee's terms of agreement whether the grantor requests or the grantee approves further restrictions, is considered \"granular consent\".\r\n\n \n Examples: \n \r\n\n \n Healthcare Examples: A healthcare provider deems a patient's assent to disclosure of health information to family members and friends, but offers an opportunity or permits the patient to dissent to such disclosures.\n A health information exchanges deems a patient to have assented to disclosure of health information for treatment purposes, but offers the patient an opportunity to dissents to disclosure to particular provider organizations.\n Non-healthcare Examples: A bank deems a banking customer's assent to specified collection, access, use, or disclosure of financial information as a requirement of holding a bank account, but provides the user an opportunity to limit third-party collection, access, use or disclosure of that information for marketing purposes."; 13382 case NOCONSENT: 13383 return "No notification or opportunity is provided for a grantor to assent or dissent to a grantee's terms of agreement.\r\n\n \n Comment: A \"No Consent\" policy scheme provides no opportunity for accommodation of an individual's preferences, and may not comply with Fair Information Practice Principles [FIPP] by enabling the data subject to object, access collected information, correct errors, or have accounting of disclosures.\r\n\n \n Usage Note: The grantee's terms of agreement, may be available to the grantor by reviewing the grantee's privacy policies, but there is no notice by which a grantor is apprised of the policy directly or able to acknowledge.\r\n\n \n Examples: \n \r\n\n \n Healthcare: Without notification or an opportunity to assent or dissent, a patient's health information is automatically included in and available (often according to certain rules) through a health information exchange. Note that this differs from implied consent, where the patient is assumed to have consented.\n Without notification or an opportunity to assent or dissent, a patient's health information is collected, accessed, used, or disclosed for research, public health, security, fraud prevention, court order, or law enforcement.\n Non-healthcare: Without notification or an opportunity to assent or dissent, a consumer's healthcare or non-healthcare internet searches are aggregated for secondary uses such as behavioral tracking and profiling.\n Without notification or an opportunity to assent or dissent, a consumer's location and activities in a shopping mall are tracked by RFID tags on purchased items."; 13384 case NOPP: 13385 return "Acknowledgement of custodian notice of privacy practices.\r\n\n \n Usage Notes: This type of consent directive acknowledges a custodian's notice of privacy practices including its permitted collection, access, use and disclosure of health information to users and for purposes of use specified."; 13386 case OPTIN: 13387 return "A grantor's assent to the terms of an agreement offered by a grantee without an opportunity for to dissent to any terms.\r\n\n \n Comment: Acceptance of a grantee's terms pertaining, for example, to permissible activities, purposes of use, handling caveats, expiry date, and revocation policies.\r\n\n \n Usage Note: Opt-in with no opportunity for a grantor to restrict certain permissions sought by the grantee is considered \"basic consent\".\r\n\n \n Examples: \n \r\n\n \n Healthcare: A patient [grantor] signs a provider's [grantee's] consent directive form, which lists permissible collection, access, use, or disclosure activities, purposes of use, handling caveats, and revocation policies.\n Non-healthcare: An employee [grantor] signs an employer's [grantee's] non-disclosure and non-compete agreement."; 13388 case OPTINR: 13389 return "A grantor's assent to the grantee's terms of an agreement with an opportunity for to dissent to certain grantor or grantee selected terms.\r\n\n \n Comment: A grantor dissenting to the grantee's terms of agreement may or may not exercise a right to assent to grantor's pre-approved restrictions or to grantee's selected terms to which a grantor may dissent.\r\n\n \n Usage Note: Opt-in with restrictions is considered \"granular consent\" because the grantor has an opportunity to narrow the permissions sought by the grantee.\r\n\n \n Examples: \n \r\n\n \n Healthcare: A patient assent to grantee's consent directive terms for collection, access, use, or disclosure of health information, and dissents to disclosure to certain recipients as allowed by the provider's pre-approved restriction list.\n Non-Healthcare: A cell phone user assents to the cell phone's privacy practices and terms of use, but dissents from location tracking by turning off the cell phone's tracking capability."; 13390 case OPTOUT: 13391 return "A grantor's dissent to the terms of agreement offered by a grantee without an opportunity for to assent to any terms.\r\n\n \n Comment: Rejection of a grantee's terms of agreement pertaining, for example, to permissible activities, purposes of use, handling caveats, expiry date, and revocation policies.\r\n\n \n Usage Note: Opt-out with no opportunity for a grantor to permit certain permissions sought by the grantee is considered \"basic consent\".\r\n\n \n Examples: \n \r\n\n \n Healthcare: A patient [grantor] declines to sign a provider's [grantee's] consent directive form, which lists permissible collection, access, use, or disclosure activities, purposes of use, handling caveats, revocation policies, and consequences of not assenting.\n Non-healthcare: An employee [grantor] refuses to sign an employer's [grantee's] agreement not to join unions or participate in a strike where state law protects employee's collective bargaining rights.\n A citizen [grantor] refuses to enroll in mandatory government [grantee] health insurance based on religious beliefs, which is an exemption."; 13392 case OPTOUTE: 13393 return "A grantor's dissent to the grantee's terms of agreement except for certain grantor or grantee selected terms.\r\n\n \n Comment: A rejection of a grantee's terms of agreement while assenting to certain permissions sought by the grantee or requesting approval of additional grantor terms.\r\n\n \n Usage Note: Opt-out with exceptions is considered a \"granular consent\" because the grantor has an opportunity to accept certain permissions sought by the grantee or request additional grantor terms, while rejecting other grantee terms.\r\n\n \n Examples: \n \r\n\n \n Healthcare: A patient [grantor] dissents to a health information exchange consent directive with the exception of disclosure based on a limited \"time to live\" shared secret [e.g., a token or password], which the patient can give to a provider when seeking care.\n Non-healthcare: A social media user [grantor] dissents from public access to their account, but assents to access to a circle of friends."; 13394 case _ACTPRIVACYLAW: 13395 return "A jurisdictional mandate, regulation, obligation, requirement, rule, or expectation deeming certain information to be private to an individual or organization, which is imposed on:\r\n\n \n The activity of a governed party\n The behavior of a governed party\n The manner in which an act is executed by a governed party"; 13396 case _ACTUSPRIVACYLAW: 13397 return "Definition: A jurisdictional mandate in the U.S. relating to privacy.\r\n\n \n Usage Note: ActPrivacyLaw codes may be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialtyCode complies. May be used to further specify rationale for assignment of other ActPrivacyPolicy codes in the US realm, e.g., ETH and 42CFRPart2 can be differentiated from ETH and Title38Part1."; 13398 case _42CFRPART2: 13399 return "42 CFR Part 2 stipulates the right of an individual who has applied for or been given diagnosis or treatment for alcohol or drug abuse at a federally assisted program.\r\n\n \n Definition: Non-disclosure of health information relating to health care paid for by a federally assisted substance abuse program without patient consent.\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialityCode complies."; 13400 case COMMONRULE: 13401 return "U.S. Federal regulations governing the protection of human subjects in research (codified at Subpart A of 45 CFR part 46) that has been adopted by 15 U.S. Federal departments and agencies in an effort to promote uniformity, understanding, and compliance with human subject protections. Existing regulations governing the protection of human subjects in Food and Drug Administration (FDA)-regulated research (21 CFR parts 50, 56, 312, and 812) are separate from the Common Rule but include similar requirements.\r\n\n \n Definition: U.S. federal laws governing research-related privacy policies.\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialtyCode complies."; 13402 case HIPAANOPP: 13403 return "The U.S. Public Law 104-191 Health Insurance Portability and Accountability Act (HIPAA) Privacy Rule (45 CFR Part 164 Subpart E) permits access, use and disclosure of certain personal health information (PHI as defined under the law) for purposes of Treatment, Payment, and Operations, and requires that the provider ask that patients acknowledge the Provider's Notice of Privacy Practices as permitted conduct under the law.\r\n\n \n Definition: Notification of HIPAA Privacy Practices.\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialtyCode complies."; 13404 case HIPAAPSYNOTES: 13405 return "The U.S. Public Law 104-191 Health Insurance Portability and Accountability Act (HIPAA) Privacy Rule (45 CFR Part 164 Section 164.508) requires authorization for certain uses and disclosure of psychotherapy notes.\r\n\n \n Definition: Authorization that must be obtained for disclosure of psychotherapy notes.\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialityCode complies."; 13406 case HIPAASELFPAY: 13407 return "Section 13405(a) of the Health Information Technology for Economic and Clinical Health Act (HITECH) stipulates the right of an individual to have disclosures regarding certain health care items or services for which the individual pays out of pocket in full restricted from a health plan.\r\n\n \n Definition: Non-disclosure of health information to a health plan relating to health care items or services for which an individual pays out of pocket in full.\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialityCode complies."; 13408 case TITLE38SECTION7332: 13409 return "Title 38 Part 1-protected information may only be disclosed to a third party with the special written consent of the patient except where expressly authorized by 38 USC 7332. VA may disclose this information for specific purposes to: VA employees on a need to know basis - more restrictive than Privacy Act need to know; contractors who need the information in order to perform or fulfil the duties of the contract; and researchers who provide assurances that the information will not be identified in any report. This information may also be disclosed without consent where patient lacks decision-making capacity; in a medical emergency for the purpose of treating a condition which poses an immediate threat to the health of any individual and which requires immediate medical intervention; for eye, tissue, or organ donation purposes; and disclosure of HIV information for public health purposes.\r\n\n \n Definition: Title 38 Part 1 - Section 1.462 Confidentiality restrictions.\r\n\n (a) General. The patient records to which Sections 1.460 through 1.499 of this part apply may be disclosed or used only as permitted by these regulations and may not otherwise be disclosed or used in any civil, criminal, administrative, or legislative proceedings conducted by any Federal, State, or local authority. Any disclosure made under these regulations must be limited to that information which is necessary to carry out the purpose of the disclosure. SUBCHAPTER III--PROTECTION OF PATIENT RIGHTS Sec. 7332. Confidentiality of certain medical records (a)(1) Records of the identity, diagnosis, prognosis, or treatment of any patient or subject which are maintained in connection with the performance of any program or activity (including education, training, treatment, rehabilitation, or research) relating to drug abuse, alcoholism or alcohol abuse, infection with the human immunodeficiency virus, or sickle cell anemia which is carried out by or for the Department under this title shall, except as provided in subsections (e) and (f), be confidential, and (section 5701 of this title to the contrary notwithstanding) such records may be disclosed only for the purposes and under the circumstances expressly authorized under subsection (b).\r\n\n \n Usage Note: May be associated with an Act or a Role to indicate the legal provision to which the assignment of an Act.confidentialityCode or Role.confidentialityCode complies."; 13410 case _INFORMATIONSENSITIVITYPOLICY: 13411 return "A mandate, obligation, requirement, rule, or expectation characterizing the value or importance of a resource and may include its vulnerability. (Based on ISO7498-2:1989. Note: The vulnerability of personally identifiable sensitive information may be based on concerns that the unauthorized disclosure may result in social stigmatization or discrimination.) Description: Types of Sensitivity policy that apply to Acts or Roles. A sensitivity policy is adopted by an enterprise or group of enterprises (a 'policy domain') through a formal data use agreement that stipulates the value, importance, and vulnerability of information. A sensitivity code representing a sensitivity policy may be associated with criteria such as categories of information or sets of information identifiers (e.g., a value set of clinical codes or branch in a code system hierarchy). These criteria may in turn be used for the Policy Decision Point in a Security Engine. A sensitivity code may be used to set the confidentiality code used on information about Acts and Roles to trigger the security mechanisms required to control how security principals (i.e., a person, a machine, a software application) may act on the information (e.g., collection, access, use, or disclosure). Sensitivity codes are never assigned to the transport or business envelope containing patient specific information being exchanged outside of a policy domain as this would disclose the information intended to be protected by the policy. When sensitive information is exchanged with others outside of a policy domain, the confidentiality code on the transport or business envelope conveys the receiver's responsibilities and indicates the how the information is to be safeguarded without unauthorized disclosure of the sensitive information. This ensures that sensitive information is treated by receivers as the sender intends, accomplishing interoperability without point to point negotiations.\r\n\n \n Usage Note: Sensitivity codes are not useful for interoperability outside of a policy domain because sensitivity policies are typically localized and vary drastically across policy domains even for the same information category because of differing organizational business rules, security policies, and jurisdictional requirements. For example, an employee's sensitivity code would make little sense for use outside of a policy domain. 'Taboo' would rarely be useful outside of a policy domain unless there are jurisdictional requirements requiring that a provider disclose sensitive information to a patient directly. Sensitivity codes may be more appropriate in a legacy system's Master Files in order to notify those who access a patient's orders and observations about the sensitivity policies that apply. Newer systems may have a security engine that uses a sensitivity policy's criteria directly. The specializable InformationSensitivityPolicy Act.code may be useful in some scenarios if used in combination with a sensitivity identifier and/or Act.title."; 13412 case _ACTINFORMATIONSENSITIVITYPOLICY: 13413 return "Types of sensitivity policies that apply to Acts. Act.confidentialityCode is defined in the RIM as \"constraints around appropriate disclosure of information about this Act, regardless of mood.\"\r\n\n \n Usage Note: ActSensitivity codes are used to bind information to an Act.confidentialityCode according to local sensitivity policy so that those confidentiality codes can then govern its handling across enterprises. Internally to a policy domain, however, local policies guide the access control system on how end users in that policy domain are able to use information tagged with these sensitivity values."; 13414 case ETH: 13415 return "Policy for handling alcohol or drug-abuse information, which will be afforded heightened confidentiality. Information handling protocols based on organizational policies related to alcohol or drug-abuse information that is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13416 case GDIS: 13417 return "Policy for handling genetic disease information, which will be afforded heightened confidentiality. Information handling protocols based on organizational policies related to genetic disease information that is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13418 case HIV: 13419 return "Policy for handling HIV or AIDS information, which will be afforded heightened confidentiality. Information handling protocols based on organizational policies related to HIV or AIDS information that is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13420 case MST: 13421 return "Policy for handling information related to sexual assault or repeated, threatening sexual harassment that occurred while the patient was in the military, which is afforded heightened confidentiality. \r\n\n Access control concerns for military sexual trauma is based on the patient being subject to control by a higher ranking military perpetrator and/or censure by others within the military unit. Due to the relatively unfettered access to healthcare information by higher ranking military personnel and those who have command over the patient, there is a need to sequester this information outside of the typical controls on access to military health records.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13422 case SCA: 13423 return "Policy for handling sickle cell disease information, which is afforded heightened confidentiality. Information handling protocols are based on organizational policies related to sickle cell disease information, which is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then the Act valued with this ActCode should be associated with an Act valued with any applicable laws from the ActPrivacyLaw code system."; 13424 case SDV: 13425 return "Policy for handling sexual assault, abuse, or domestic violence information, which will be afforded heightened confidentiality. Information handling protocols based on organizational policies related to sexual assault, abuse, or domestic violence information that is deemed sensitive.\r\n\n SDV code covers violence perpetrated by related and non-related persons. This code should be specific to physical and mental trauma caused by a related person only. The access control concerns are keeping the patient safe from the perpetrator who may have an abusive psychological control over the patient, may be stalking the patient, or may try to manipulate care givers into allowing the perpetrator to make contact with the patient. The definition needs to be clarified.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13426 case SEX: 13427 return "Policy for handling sexuality and reproductive health information, which will be afforded heightened confidentiality. Information handling protocols based on organizational policies related to sexuality and reproductive health information that is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13428 case SPI: 13429 return "Policy for handling information deemed specially protected by law or policy including substance abuse, substance use, psychiatric, mental health, behavioral health, and cognitive disorders, which is afforded heightened confidentiality.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13430 case BH: 13431 return "Policy for handling information related to behavioral and emotional disturbances affecting social adjustment and physical health, which is afforded heightened confidentiality.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13432 case COGN: 13433 return "Policy for handling information related to cognitive disability disorders and conditions caused by these disorders, which are afforded heightened confidentiality.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code.\r\n\n Examples may include dementia, traumatic brain injury, attention deficit, hearing and visual disability such as dyslexia and other disorders and related conditions which impair learning and self-sufficiency. However, the cognitive disabilities to which this term may apply versus other behavioral health categories varies by jurisdiction and organizational policy in part due to overlap with other behavioral health conditions. Implementers should constrain to those diagnoses applicable in the domain in which this code is used."; 13434 case DVD: 13435 return "Policy for handling information related to developmental disability disorders and conditions caused by these disorders, which is afforded heightened confidentiality.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code.\r\n\n A diverse group of chronic conditions that are due to mental or physical impairments impacting activities of daily living, self-care, language acuity, learning, mobility, independent living and economic self-sufficiency. Examples may include Down syndrome and Autism spectrum. However, the developmental disabilities to which this term applies versus other behavioral health categories varies by jurisdiction and organizational policy in part due to overlap with other behavioral health conditions. Implementers should constrain to those diagnoses applicable in the domain in which this code is used."; 13436 case EMOTDIS: 13437 return "Policy for handling information related to emotional disturbance disorders and conditions caused by these disorders, which is afforded heightened confidentiality.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code.\r\n\n Typical used to characterize behavioral and mental health issues of adolescents where the disorder may be temporarily diagnosed in order to avoid the potential and unnecessary stigmatizing diagnoses of disorder long term."; 13438 case MH: 13439 return "Policy for handling information related to psychological disorders, which is afforded heightened confidentiality. Mental health information may be deemed specifically sensitive and distinct from physical health, substance use disorders, and behavioral disabilities and disorders in some jurisdictions.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13440 case PSY: 13441 return "Policy for handling psychiatry psychiatric disorder information, which is afforded heightened confidentiality. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13442 case PSYTHPN: 13443 return "Policy for handling psychotherapy note information, which is afforded heightened confidentiality. \r\n\n \n Usage Note: In some jurisdiction, disclosure of psychotherapy notes requires patient consent.\r\n\n If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13444 case SUD: 13445 return "Policy for handling information related to alcohol or drug use disorders and conditions caused by these disorders, which is afforded heightened confidentiality. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13446 case ETHUD: 13447 return "Policy for handling information related to alcohol use disorders and conditions caused by these disorders, which is afforded heightened confidentiality. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13448 case OPIOIDUD: 13449 return "Policy for handling information related to opioid use disorders and conditions caused by these disorders, which is afforded heightened confidentiality. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13450 case STD: 13451 return "Policy for handling sexually transmitted disease information, which will be afforded heightened confidentiality.\n Information handling protocols based on organizational policies related to sexually transmitted disease information that is deemed sensitive.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13452 case TBOO: 13453 return "Policy for handling information not to be initially disclosed or discussed with patient except by a physician assigned to patient in this case. Information handling protocols based on organizational policies related to sensitive patient information that must be initially discussed with the patient by an attending physician before being disclosed to the patient.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code.\r\n\n \n Open Issue: This definition conflates a rule and a characteristic, and there may be a similar issue with ts sibling codes."; 13454 case VIO: 13455 return "Policy for handling information related to harm by violence, which is afforded heightened confidentiality. Harm by violence is perpetrated by an unrelated person.\r\n\n Access control concerns for information about mental or physical harm resulting from violence caused by an unrelated person may include manipulation of care givers or access to records that enable the perpetrator contact or locate the patient, but the perpetrator will likely not have established abusive psychological control over the patient. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code."; 13456 case SICKLE: 13457 return "Types of sensitivity policies that apply to Acts. Act.confidentialityCode is defined in the RIM as \"constraints around appropriate disclosure of information about this Act, regardless of mood.\"\r\n\n \n Usage Note: ActSensitivity codes are used to bind information to an Act.confidentialityCode according to local sensitivity policy so that those confidentiality codes can then govern its handling across enterprises. Internally to a policy domain, however, local policies guide the access control system on how end users in that policy domain are able to use information tagged with these sensitivity values."; 13458 case _ENTITYSENSITIVITYPOLICYTYPE: 13459 return "Types of sensitivity policies that may apply to a sensitive attribute on an Entity.\r\n\n \n Usage Note: EntitySensitivity codes are used to convey a policy that is applicable to sensitive information conveyed by an entity attribute. May be used to bind a Role.confidentialityCode associated with an Entity per organizational policy. Role.confidentialityCode is defined in the RIM as \"an indication of the appropriate disclosure of information about this Role with respect to the playing Entity.\""; 13460 case DEMO: 13461 return "Policy for handling all demographic information about an information subject, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to all demographic about an information subject, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13462 case DOB: 13463 return "Policy for handling information related to an information subject's date of birth, which will be afforded heightened confidentiality.Policies may govern sensitivity of information related to an information subject's date of birth, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13464 case GENDER: 13465 return "Policy for handling information related to an information subject's gender and sexual orientation, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to an information subject's gender and sexual orientation, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13466 case LIVARG: 13467 return "Policy for handling information related to an information subject's living arrangement, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to an information subject's living arrangement, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13468 case MARST: 13469 return "Policy for handling information related to an information subject's marital status, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to an information subject's marital status, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13470 case RACE: 13471 return "Policy for handling information related to an information subject's race, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to an information subject's race, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13472 case REL: 13473 return "Policy for handling information related to an information subject's religious affiliation, which will be afforded heightened confidentiality. Policies may govern sensitivity of information related to an information subject's religion, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Notes: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13474 case _ROLEINFORMATIONSENSITIVITYPOLICY: 13475 return "Types of sensitivity policies that apply to Roles.\r\n\n \n Usage Notes: RoleSensitivity codes are used to bind information to a Role.confidentialityCode per organizational policy. Role.confidentialityCode is defined in the RIM as \"an indication of the appropriate disclosure of information about this Role with respect to the playing Entity.\""; 13476 case B: 13477 return "Policy for handling trade secrets such as financial information or intellectual property, which will be afforded heightened confidentiality. Description: Since the service class can represent knowledge structures that may be considered a trade or business secret, there is sometimes (though rarely) the need to flag those items as of business level confidentiality.\r\n\n \n Usage Notes: No patient related information may ever be of this confidentiality level. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13478 case EMPL: 13479 return "Policy for handling information related to an employer which is deemed classified to protect an employee who is the information subject, and which will be afforded heightened confidentiality. Description: Policies may govern sensitivity of information related to an employer, such as law enforcement or national security, the identity of which could impact the privacy, well-being, or safety of an information subject who is an employee.\r\n\n \n Usage Notes: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13480 case LOCIS: 13481 return "Policy for handling information related to the location of the information subject, which will be afforded heightened confidentiality. Description: Policies may govern sensitivity of information related to the location of the information subject, the disclosure of which could impact the privacy, well-being, or safety of that subject.\r\n\n \n Usage Notes: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13482 case SSP: 13483 return "Policy for handling information related to a provider of sensitive services, which will be afforded heightened confidentiality. Description: Policies may govern sensitivity of information related to providers who deliver sensitive healthcare services in order to protect the privacy, well-being, and safety of the provider and of patients receiving sensitive services.\r\n\n \n Usage Notes: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13484 case ADOL: 13485 return "Policy for handling information related to an adolescent, which will be afforded heightened confidentiality per applicable organizational or jurisdictional policy. An enterprise may have a policy that requires that adolescent patient information be provided heightened confidentiality. Information deemed sensitive typically includes health information and patient role information including patient status, demographics, next of kin, and location.\r\n\n \n Usage Note: For use within an enterprise in which an adolescent is the information subject. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13486 case CEL: 13487 return "Policy for handling information related to a celebrity (people of public interest (VIP), which will be afforded heightened confidentiality. Celebrities are people of public interest (VIP) about whose information an enterprise may have a policy that requires heightened confidentiality. Information deemed sensitive may include health information and patient role information including patient status, demographics, next of kin, and location.\r\n\n \n Usage Note: For use within an enterprise in which the information subject is deemed a celebrity or very important person. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13488 case DIA: 13489 return "Policy for handling information related to a diagnosis, health condition or health problem, which will be afforded heightened confidentiality. Diagnostic, health condition or health problem related information may be deemed sensitive by organizational policy, and require heightened confidentiality.\r\n\n \n Usage Note: For use within an enterprise that provides heightened confidentiality to diagnostic, health condition or health problem related information deemed sensitive. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13490 case DRGIS: 13491 return "Policy for handling information related to a drug, which will be afforded heightened confidentiality. Drug information may be deemed sensitive by organizational policy, and require heightened confidentiality.\r\n\n \n Usage Note: For use within an enterprise that provides heightened confidentiality to drug information deemed sensitive. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13492 case EMP: 13493 return "Policy for handling information related to an employee, which will be afforded heightened confidentiality. When a patient is an employee, an enterprise may have a policy that requires heightened confidentiality. Information deemed sensitive typically includes health information and patient role information including patient status, demographics, next of kin, and location.\r\n\n \n Usage Note: Policy for handling information related to an employee, which will be afforded heightened confidentiality. Description: When a patient is an employee, an enterprise may have a policy that requires heightened confidentiality. Information deemed sensitive typically includes health information and patient role information including patient status, demographics, next of kin, and location."; 13494 case PDS: 13495 return "Policy for specially protecting information reported by or about a patient, which is deemed sensitive within the enterprise (i.e., by default regardless of whether the patient requested that the information be deemed sensitive for another reason.) For example information reported by the patient about another person, e.g., a family member, may be deemed sensitive by default. Organizational policy may allow the sensitivity tag to be cleared on patient's request. \r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law in addition to this more generic code.\r\n\n For example, VA deems employee information sensitive by default. Information about a patient who is being stalked or a victim of abuse or violence may be deemed sensitive by default per a provider organization's policies."; 13496 case PHY: 13497 return "Policy for handling information about a patient, which a physician or other licensed healthcare provider deems sensitive. Once tagged by the provider, this may trigger alerts for follow up actions according to organizational policy or jurisdictional law.\r\n\n \n Usage Note: For use within an enterprise that provides heightened confidentiality to certain types of information designated by a physician as sensitive. If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code.\r\n\n Use cases in which this code could be used are, e.g., in systems that lack the ability to automatically detect sensitive information and must rely on manual tagging; a system that lacks an applicable sensitivity tag, or for ad hoc situations where criticality of the situation requires that the tagging be done immediately by the provider before coding or transcription of consult notes can be completed, e.g., upon detection of a patient with suicidal tendencies or potential for violence."; 13498 case PRS: 13499 return "Policy for specially protecting information reported by or about a patient, which the patient deems sensitive, and the patient requests that collection, access, use, or disclosure of that information be restricted. For example, a minor patient may request that information about reproductive health not be disclosed to the patient's family or to particular providers and payers.\r\n\n \n Usage Note: If there is a jurisdictional mandate, then use the applicable ActPrivacyLaw code system, and specify the law rather than or in addition to this more generic code."; 13500 case COMPT: 13501 return "This is the healthcare analog to the US Intelligence Community's concept of a Special Access Program. Compartment codes may be used in as a field value in an initiator's clearance to indicate permission to access and use an IT Resource with a security label having the same compartment value in security category label field.\r\n\n Map: Aligns with ISO 2382-8 definition of Compartment - \"A division of data into isolated blocks with separate security controls for the purpose of reducing risk.\""; 13502 case ACOCOMPT: 13503 return "A group of health care entities, which may include health care providers, care givers, hospitals, facilities, health plans, and other health care constituents who coordinate care for reimbursement based on quality metrics for improving outcomes and lowering costs, and may be authorized to access the consumer's health information because of membership in that group.\r\n\n Security Compartment Labels assigned to a consumer's information use in accountable care workflows should be met or exceeded by the Security Compartment attribute claimed by a participant in a an accountable care workflow who is requesting access to that information"; 13504 case CTCOMPT: 13505 return "Care coordination across participants in a care plan requires sharing of a healthcare consumer's information specific to that workflow. A care team member should only have access to that information while participating in that workflow or for other authorized uses.\r\n\n Security Compartment Labels assigned to a consumer's information use in care coordination workflows should be met or exceeded by the Security Compartment attribute claimed by a participant in a care team member workflow who is requesting access to that information"; 13506 case FMCOMPT: 13507 return "Financial management department members who have access to healthcare consumer information as part of a patient account, billing and claims workflows.\r\n\n Security Compartment Labels assigned to consumer information used in these workflows should be met or exceeded by the Security Compartment attribute claimed by a participant in a financial management workflow who is requesting access to that information."; 13508 case HRCOMPT: 13509 return "A security category label field value, which indicates that access and use of an IT resource is restricted to members of human resources department or workflow."; 13510 case LRCOMPT: 13511 return "Providers and care givers who have an established relationship per criteria determined by policy are considered to have an established care provision relations with a healthcare consumer, and may be authorized to access the consumer's health information because of that relationship. Providers and care givers should only have access to that information while participating in legitimate relationship workflows or for other authorized uses.\r\n\n Security Compartment Labels assigned to a consumer's information use in legitimate relationship workflows should be met or exceeded by the Security Compartment attribute claimed by a participant in a legitimate relationship workflow who is requesting access to that information."; 13512 case PACOMPT: 13513 return "Patient administration members who have access to healthcare consumer information as part of a patient administration workflows.\r\n\n Security Compartment Labels assigned to consumer information used in these workflows should be met or exceeded by the Security Compartment attribute claimed by a participant in a patient administration workflow who is requesting access to that information."; 13514 case RESCOMPT: 13515 return "A security category label field value, which indicates that access and use of an IT resource is restricted to members of a research project."; 13516 case RMGTCOMPT: 13517 return "A security category label field value, which indicates that access and use of an IT resource is restricted to members of records management department or workflow."; 13518 case ACTTRUSTPOLICYTYPE: 13519 return "A mandate, obligation, requirement, rule, or expectation conveyed as security metadata between senders and receivers required to establish the reliability, authenticity, and trustworthiness of their transactions.\r\n\n Trust security metadata are observation made about aspects of trust applicable to an IT resource (data, information object, service, or system capability).\r\n\n Trust applicable to IT resources is established and maintained in and among security domains, and may be comprised of observations about the domain's trust authority, trust framework, trust policy, trust interaction rules, means for assessing and monitoring adherence to trust policies, mechanisms that enforce trust, and quality and reliability measures of assurance in those mechanisms. [Based on ISO IEC 10181-1 and NIST SP 800-63-2]\r\n\n For example, identity proofing , level of assurance, and Trust Framework."; 13520 case TRSTACCRD: 13521 return "Type of security metadata about the formal declaration by an authority or neutral third party that validates the technical, security, trust, and business practice conformance of Trust Agents to facilitate security, interoperability, and trust among participants within a security domain or trust framework."; 13522 case TRSTAGRE: 13523 return "Type of security metadata about privacy and security requirements with which a security domain must comply. [ISO IEC 10181-1]"; 13524 case TRSTASSUR: 13525 return "Type of security metadata about the digital quality or reliability of a trust assertion, activity, capability, information exchange, mechanism, process, or protocol."; 13526 case TRSTCERT: 13527 return "Type of security metadata about a set of security-relevant data issued by a security authority or trusted third party, together with security information which is used to provide the integrity and data origin authentication services for an IT resource (data, information object, service, or system capability). [Based on ISO IEC 10181-1]"; 13528 case TRSTFWK: 13529 return "Type of security metadata about a complete set of contracts, regulations, or commitments that enable participating actors to rely on certain assertions by other actors to fulfill their information security requirements. [Kantara Initiative]"; 13530 case TRSTMEC: 13531 return "Type of security metadata about a security architecture system component that supports enforcement of security policies."; 13532 case COVPOL: 13533 return "Description:A mandate, obligation, requirement, rule, or expectation unilaterally imposed on benefit coverage under a policy or program by a sponsor, underwriter or payor on:\r\n\n \n \n The activity of another party\r\n\n \n \n The behavior of another party\r\n\n \n \n The manner in which an act is executed\r\n\n \n \n \n Examples:A clinical protocol imposed by a payer to which a provider must adhere in order to be paid for providing the service. A formulary from which a provider must select prescribed drugs in order for the patient to incur a lower copay."; 13534 case SECURITYPOLICY: 13535 return "Types of security policies that further specify the ActClassPolicy value set.\r\n\n \n Examples:\n \r\n\n \n obligation to encrypt\n refrain from redisclosure without consent"; 13536 case AUTHPOL: 13537 return "Authorisation policies are essentially security policies related to access-control and specify what activities a subject is permitted or forbidden to do, to a set of target objects. They are designed to protect target objects so are interpreted by access control agents or the run-time systems at the target system.\r\n\n A positive authorisation policy defines the actions that a subject is permitted to perform on a target. A negative authorisation policy specifies the actions that a subject is forbidden to perform on a target. Positive authorisation policies may also include filters to transform the parameters associated with their actions. (Based on PONDERS)"; 13538 case ACCESSCONSCHEME: 13539 return "An access control policy specific to the type of access control scheme, which is used to enforce one or more authorization policies. \r\n\n \n Usage Note: Access control schemes are the type of access control policy, which is comprised of access control policy rules concerning the provision of the access control service.\r\n\n There are two categories of access control policies, rule-based and identity-based, which are identified in CCITT Rec. X.800 aka ISO 7498-2. Rule-based access control policies are intended to apply to all access requests by any initiator on any target in a security domain. Identity-based access control policies are based on rules specific to an individual initiator, a group of initiators, entities acting on behalf of initiators, or originators acting in a specific role. Context can modify rule-based or identity-based access control policies. Context rules may define the entire policy in effect. Real systems will usually employ a combination of these policy types; if a rule-based policy is used, then an identity-based policy is usually in effect also.\r\n\n An access control scheme may be based on access control lists, capabilities, labels, and context or a combination of these. An access control scheme is a component of an access control mechanism or \"service\") along with the supporting mechanisms required by that scheme to provide access control decision information (ADI) supplied by the scheme to the access decision facility (ADF also known as a PDP). (Based on ISO/IEC 10181-3:1996)\r\n\n \n Examples: \n \r\n\n \n Attribute Based Access Control (ABAC)\n Discretionary Access Control (DAC)\n History Based Access Control (HBAC)\n Identity Based Access Control (IBAC)\n Mandatory Access Control (MAC)\n Organization Based Access Control (OrBAC)\n Relationship Based Access Control (RelBac)\n Responsibility Based Access Control (RespBAC)\n Risk Adaptable Access Control (RAdAC)\n >"; 13540 case DELEPOL: 13541 return "Delegation policies specify which actions subjects are allowed to delegate to others. A delegation policy thus specifies an authorisation to delegate. Subjects must already possess the access rights to be delegated.\r\n\n Delegation policies are aimed at subjects delegating rights to servers or third parties to perform actions on their behalf and are not meant to be the means by which security administrators would assign rights to subjects. A negative delegation policy identifies what delegations are forbidden.\r\n\n A Delegation policy specifies the authorisation policy from which delegated rights are derived, the grantors, which are the entities which can delegate these access rights, and the grantees, which are the entities to which the access rights can be delegated. There are two types of delegation policy, positive and negative. (Based on PONDERS)"; 13542 case OBLIGATIONPOLICY: 13543 return "Conveys the mandated workflow action that an information custodian, receiver, or user must perform. \r\n\n \n Usage Notes: Per ISO 22600-2, ObligationPolicy instances 'are event-triggered and define actions to be performed by manager agent'. Per HL7 Composite Security and Privacy Domain Analysis Model: This value set refers to the action required to receive the permission specified in the privacy rule. Per OASIS XACML, an obligation is an operation specified in a policy or policy that is performed in conjunction with the enforcement of an access control decision."; 13544 case ANONY: 13545 return "Custodian system must remove any information that could result in identifying the information subject."; 13546 case AOD: 13547 return "Custodian system must make available to an information subject upon request an accounting of certain disclosures of the individualâ??s protected health information over a period of time. Policy may dictate that the accounting include information about the information disclosed, the date of disclosure, the identification of the receiver, the purpose of the disclosure, the time in which the disclosing entity must provide a response and the time period for which accountings of disclosure can be requested."; 13548 case AUDIT: 13549 return "Custodian system must monitor systems to ensure that all users are authorized to operate on information objects."; 13550 case AUDTR: 13551 return "Custodian system must monitor and maintain retrievable log for each user and operation on information."; 13552 case CPLYCC: 13553 return "Custodian security system must retrieve, evaluate, and comply with the information handling directions of the Confidentiality Code associated with an information target."; 13554 case CPLYCD: 13555 return "Custodian security system must retrieve, evaluate, and comply with applicable information subject consent directives."; 13556 case CPLYJPP: 13557 return "Custodian security system must retrieve, evaluate, and comply with applicable jurisdictional privacy policies associated with the target information."; 13558 case CPLYOPP: 13559 return "Custodian security system must retrieve, evaluate, and comply with applicable organizational privacy policies associated with the target information."; 13560 case CPLYOSP: 13561 return "Custodian security system must retrieve, evaluate, and comply with the organizational security policies associated with the target information."; 13562 case CPLYPOL: 13563 return "Custodian security system must retrieve, evaluate, and comply with applicable policies associated with the target information."; 13564 case DECLASSIFYLABEL: 13565 return "Custodian security system must declassify information assigned security labels by instantiating a new version of the classified information so as to break the binding of the classifying security label when assigning a new security label that marks the information as unclassified in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the previous assignment and binding."; 13566 case DEID: 13567 return "Custodian system must strip information of data that would allow the identification of the source of the information or the information subject."; 13568 case DELAU: 13569 return "Custodian system must remove target information from access after use."; 13570 case DOWNGRDLABEL: 13571 return "Custodian security system must downgrade information assigned security labels by instantiating a new version of the classified information so as to break the binding of the classifying security label when assigning a new security label that marks the information as classified at a less protected level in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the previous assignment and binding."; 13572 case DRIVLABEL: 13573 return "Custodian security system must assign and bind security labels derived from compilations of information by aggregation or disaggregation in order to classify information compiled in the information systems under its control for collection, access, use and disclosure in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the previous assignment and binding."; 13574 case ENCRYPT: 13575 return "Custodian system must render information unreadable by algorithmically transforming plaintext into ciphertext. \r\n\n \r\n\n \n Usage Notes: A mathematical transposition of a file or data stream so that it cannot be deciphered at the receiving end without the proper key. Encryption is a security feature that assures that only the parties who are supposed to be participating in a videoconference or data transfer are able to do so. It can include a password, public and private keys, or a complex combination of all. (Per Infoway.)"; 13576 case ENCRYPTR: 13577 return "Custodian system must render information unreadable and unusable by algorithmically transforming plaintext into ciphertext when \"at rest\" or in storage."; 13578 case ENCRYPTT: 13579 return "Custodian system must render information unreadable and unusable by algorithmically transforming plaintext into ciphertext while \"in transit\" or being transported by any means."; 13580 case ENCRYPTU: 13581 return "Custodian system must render information unreadable and unusable by algorithmically transforming plaintext into ciphertext while in use such that operations permitted on the target information are limited by the license granted to the end user."; 13582 case HUAPRV: 13583 return "Custodian system must require human review and approval for permission requested."; 13584 case LABEL: 13585 return "Custodian security system must assign and bind security labels in order to classify information created in the information systems under its control for collection, access, use and disclosure in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the assignment and binding.\r\n\n \n Usage Note: In security systems, security policy label assignments do not change, they may supersede prior assignments, and such reassignments are always tracked for auditing and other purposes."; 13586 case MASK: 13587 return "Custodian system must render information unreadable and unusable by algorithmically transforming plaintext into ciphertext. User may be provided a key to decrypt per license or \"shared secret\"."; 13588 case MINEC: 13589 return "Custodian must limit access and disclosure to the minimum information required to support an authorized user's purpose of use. \r\n\n \n Usage Note: Limiting the information available for access and disclosure to that an authorized user or receiver \"needs to know\" in order to perform permitted workflow or purpose of use."; 13590 case PERSISTLABEL: 13591 return "Custodian security system must persist the binding of security labels to classify information received or imported by information systems under its control for collection, access, use and disclosure in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the assignment and binding."; 13592 case PRIVMARK: 13593 return "Custodian must create and/or maintain human readable security label tags as required by policy.\r\n\n Map: Aligns with ISO 22600-3 Section A.3.4.3 description of privacy mark: \"If present, the privacy-mark is not used for access control. The content of the privacy-mark may be defined by the security policy in force (identified by the security-policy-identifier) which may define a list of values to be used. Alternately, the value may be determined by the originator of the security-label.\""; 13594 case PSEUD: 13595 return "Custodian system must strip information of data that would allow the identification of the source of the information or the information subject. Custodian may retain a key to relink data necessary to reidentify the information subject."; 13596 case REDACT: 13597 return "Custodian system must remove information, which is not authorized to be access, used, or disclosed from records made available to otherwise authorized users."; 13598 case UPGRDLABEL: 13599 return "Custodian security system must declassify information assigned security labels by instantiating a new version of the classified information so as to break the binding of the classifying security label when assigning a new security label that marks the information as classified at a more protected level in accordance with applicable jurisdictional privacy policies associated with the target information. The system must retain an immutable record of the previous assignment and binding."; 13600 case REFRAINPOLICY: 13601 return "Conveys prohibited actions which an information custodian, receiver, or user is not permitted to perform unless otherwise authorized or permitted under specified circumstances.\r\n\n \r\n\n \n Usage Notes: ISO 22600-2 species that a Refrain Policy \"defines actions the subjects must refrain from performing\". Per HL7 Composite Security and Privacy Domain Analysis Model: May be used to indicate that a specific action is prohibited based on specific access control attributes e.g., purpose of use, information type, user role, etc."; 13602 case NOAUTH: 13603 return "Prohibition on disclosure without information subject's authorization."; 13604 case NOCOLLECT: 13605 return "Prohibition on collection or storage of the information."; 13606 case NODSCLCD: 13607 return "Prohibition on disclosure without organizational approved patient restriction."; 13608 case NODSCLCDS: 13609 return "Prohibition on disclosure without a consent directive from the information subject."; 13610 case NOINTEGRATE: 13611 return "Prohibition on Integration into other records."; 13612 case NOLIST: 13613 return "Prohibition on disclosure except to entities on specific access list."; 13614 case NOMOU: 13615 return "Prohibition on disclosure without an interagency service agreement or memorandum of understanding (MOU)."; 13616 case NOORGPOL: 13617 return "Prohibition on disclosure without organizational authorization."; 13618 case NOPAT: 13619 return "Prohibition on disclosing information to patient, family or caregivers without attending provider's authorization.\r\n\n \n Usage Note: The information may be labeled with the ActInformationSensitivity TBOO code, triggering application of this RefrainPolicy code as a handling caveat controlling access.\r\n\n Maps to FHIR NOPAT: Typically, this is used on an Alert resource, when the alert records information on patient abuse or non-compliance.\r\n\n FHIR print name is \"keep information from patient\". Maps to the French realm - code: INVISIBLE_PATIENT.\r\n\n \n displayName: Document non visible par le patient\n codingScheme: 1.2.250.1.213.1.1.4.13\n \n French use case: A label for documents that the author chose to hide from the patient until the content can be disclose to the patient in a face to face meeting between a healthcare professional and the patient (in French law some results like cancer diagnosis or AIDS diagnosis must be announced to the patient by a healthcare professional and should not be find out by the patient alone)."; 13620 case NOPERSISTP: 13621 return "Prohibition on collection of the information beyond time necessary to accomplish authorized purpose of use is prohibited."; 13622 case NORDSCLCD: 13623 return "Prohibition on redisclosure without patient consent directive."; 13624 case NORDSCLCDS: 13625 return "Prohibition on redisclosure without a consent directive from the information subject."; 13626 case NORDSCLW: 13627 return "Prohibition on disclosure without authorization under jurisdictional law."; 13628 case NORELINK: 13629 return "Prohibition on associating de-identified or pseudonymized information with other information in a manner that could or does result in disclosing information intended to be masked."; 13630 case NOREUSE: 13631 return "Prohibition on use of the information beyond the purpose of use initially authorized."; 13632 case NOVIP: 13633 return "Prohibition on disclosure except to principals with access permission to specific VIP information."; 13634 case ORCON: 13635 return "Prohibition on disclosure except as permitted by the information originator."; 13636 case _ACTPRODUCTACQUISITIONCODE: 13637 return "The method that a product is obtained for use by the subject of the supply act (e.g. patient). Product examples are consumable or durable goods."; 13638 case LOAN: 13639 return "Temporary supply of a product without transfer of ownership for the product."; 13640 case RENT: 13641 return "Temporary supply of a product with financial compensation, without transfer of ownership for the product."; 13642 case TRANSFER: 13643 return "Transfer of ownership for a product."; 13644 case SALE: 13645 return "Transfer of ownership for a product for financial compensation."; 13646 case _ACTSPECIMENTRANSPORTCODE: 13647 return "Transportation of a specimen."; 13648 case SREC: 13649 return "Description:Specimen has been received by the participating organization/department."; 13650 case SSTOR: 13651 return "Description:Specimen has been placed into storage at a participating location."; 13652 case STRAN: 13653 return "Description:Specimen has been put in transit to a participating receiver."; 13654 case _ACTSPECIMENTREATMENTCODE: 13655 return "Set of codes related to specimen treatments"; 13656 case ACID: 13657 return "The lowering of specimen pH through the addition of an acid"; 13658 case ALK: 13659 return "The act rendering alkaline by impregnating with an alkali; a conferring of alkaline qualities."; 13660 case DEFB: 13661 return "The removal of fibrin from whole blood or plasma through physical or chemical means"; 13662 case FILT: 13663 return "The passage of a liquid through a filter, accomplished by gravity, pressure or vacuum (suction)."; 13664 case LDLP: 13665 return "LDL Precipitation"; 13666 case NEUT: 13667 return "The act or process by which an acid and a base are combined in such proportions that the resulting compound is neutral."; 13668 case RECA: 13669 return "The addition of calcium back to a specimen after it was removed by chelating agents"; 13670 case UFIL: 13671 return "The filtration of a colloidal substance through a semipermeable medium that allows only the passage of small molecules."; 13672 case _ACTSUBSTANCEADMINISTRATIONCODE: 13673 return "Description: Describes the type of substance administration being performed. This should not be used to carry codes for identification of products. Use an associated role or entity to carry such information."; 13674 case DRUG: 13675 return "The introduction of a drug into a subject with the intention of altering its biologic state with the intent of improving its health status."; 13676 case FD: 13677 return "Description: The introduction of material into a subject with the intent of providing nutrition or other dietary supplements (e.g. minerals or vitamins)."; 13678 case IMMUNIZ: 13679 return "The introduction of an immunogen with the intent of stimulating an immune response, aimed at preventing subsequent infections by more viable agents."; 13680 case BOOSTER: 13681 return "An additional immunization administration within a series intended to bolster or enhance immunity."; 13682 case INITIMMUNIZ: 13683 return "The first immunization administration in a series intended to produce immunity"; 13684 case _ACTTASKCODE: 13685 return "Description: A task or action that a user may perform in a clinical information system (e.g., medication order entry, laboratory test results review, problem list entry)."; 13686 case OE: 13687 return "A clinician creates a request for a service to be performed for a given patient."; 13688 case LABOE: 13689 return "A clinician creates a request for a laboratory test to be done for a given patient."; 13690 case MEDOE: 13691 return "A clinician creates a request for the administration of one or more medications to a given patient."; 13692 case PATDOC: 13693 return "A person enters documentation about a given patient."; 13694 case ALLERLREV: 13695 return "Description: A person reviews a list of known allergies of a given patient."; 13696 case CLINNOTEE: 13697 return "A clinician enters a clinical note about a given patient"; 13698 case DIAGLISTE: 13699 return "A clinician enters a diagnosis for a given patient."; 13700 case DISCHINSTE: 13701 return "A person provides a discharge instruction to a patient."; 13702 case DISCHSUME: 13703 return "A clinician enters a discharge summary for a given patient."; 13704 case PATEDUE: 13705 return "A person provides a patient-specific education handout to a patient."; 13706 case PATREPE: 13707 return "A pathologist enters a report for a given patient."; 13708 case PROBLISTE: 13709 return "A clinician enters a problem for a given patient."; 13710 case RADREPE: 13711 return "A radiologist enters a report for a given patient."; 13712 case IMMLREV: 13713 return "Description: A person reviews a list of immunizations due or received for a given patient."; 13714 case REMLREV: 13715 return "Description: A person reviews a list of health care reminders for a given patient."; 13716 case WELLREMLREV: 13717 return "Description: A person reviews a list of wellness or preventive care reminders for a given patient."; 13718 case PATINFO: 13719 return "A person (e.g., clinician, the patient herself) reviews patient information in the electronic medical record."; 13720 case ALLERLE: 13721 return "Description: A person enters a known allergy for a given patient."; 13722 case CDSREV: 13723 return "A person reviews a recommendation/assessment provided automatically by a clinical decision support application for a given patient."; 13724 case CLINNOTEREV: 13725 return "A person reviews a clinical note of a given patient."; 13726 case DISCHSUMREV: 13727 return "A person reviews a discharge summary of a given patient."; 13728 case DIAGLISTREV: 13729 return "A person reviews a list of diagnoses of a given patient."; 13730 case IMMLE: 13731 return "Description: A person enters an immunization due or received for a given patient."; 13732 case LABRREV: 13733 return "A person reviews a list of laboratory results of a given patient."; 13734 case MICRORREV: 13735 return "A person reviews a list of microbiology results of a given patient."; 13736 case MICROORGRREV: 13737 return "A person reviews organisms of microbiology results of a given patient."; 13738 case MICROSENSRREV: 13739 return "A person reviews the sensitivity test of microbiology results of a given patient."; 13740 case MLREV: 13741 return "A person reviews a list of medication orders submitted to a given patient"; 13742 case MARWLREV: 13743 return "A clinician reviews a work list of medications to be administered to a given patient."; 13744 case OREV: 13745 return "A person reviews a list of orders submitted to a given patient."; 13746 case PATREPREV: 13747 return "A person reviews a pathology report of a given patient."; 13748 case PROBLISTREV: 13749 return "A person reviews a list of problems of a given patient."; 13750 case RADREPREV: 13751 return "A person reviews a radiology report of a given patient."; 13752 case REMLE: 13753 return "Description: A person enters a health care reminder for a given patient."; 13754 case WELLREMLE: 13755 return "Description: A person enters a wellness or preventive care reminder for a given patient."; 13756 case RISKASSESS: 13757 return "A person reviews a Risk Assessment Instrument report of a given patient."; 13758 case FALLRISK: 13759 return "A person reviews a Falls Risk Assessment Instrument report of a given patient."; 13760 case _ACTTRANSPORTATIONMODECODE: 13761 return "Characterizes how a transportation act was or will be carried out.\r\n\n \n Examples: Via private transport, via public transit, via courier."; 13762 case _ACTPATIENTTRANSPORTATIONMODECODE: 13763 return "Definition: Characterizes how a patient was or will be transported to the site of a patient encounter.\r\n\n \n Examples: Via ambulance, via public transit, on foot."; 13764 case AFOOT: 13765 return "pedestrian transport"; 13766 case AMBT: 13767 return "ambulance transport"; 13768 case AMBAIR: 13769 return "fixed-wing ambulance transport"; 13770 case AMBGRND: 13771 return "ground ambulance transport"; 13772 case AMBHELO: 13773 return "helicopter ambulance transport"; 13774 case LAWENF: 13775 return "law enforcement transport"; 13776 case PRVTRN: 13777 return "private transport"; 13778 case PUBTRN: 13779 return "public transport"; 13780 case _OBSERVATIONTYPE: 13781 return "Identifies the kinds of observations that can be performed"; 13782 case _ACTSPECOBSCODE: 13783 return "Identifies the type of observation that is made about a specimen that may affect its processing, analysis or further result interpretation"; 13784 case ARTBLD: 13785 return "Describes the artificial blood identifier that is associated with the specimen."; 13786 case DILUTION: 13787 return "An observation that reports the dilution of a sample."; 13788 case AUTOHIGH: 13789 return "The dilution of a sample performed by automated equipment. The value is specified by the equipment"; 13790 case AUTOLOW: 13791 return "The dilution of a sample performed by automated equipment. The value is specified by the equipment"; 13792 case PRE: 13793 return "The dilution of the specimen made prior to being loaded onto analytical equipment"; 13794 case RERUN: 13795 return "The value of the dilution of a sample after it had been analyzed at a prior dilution value"; 13796 case EVNFCTS: 13797 return "Domain provides codes that qualify the ActLabObsEnvfctsCode domain. (Environmental Factors)"; 13798 case INTFR: 13799 return "An observation that relates to factors that may potentially cause interference with the observation"; 13800 case FIBRIN: 13801 return "The Fibrin Index of the specimen. In the case of only differentiating between Absent and Present, recommend using 0 and 1"; 13802 case HEMOLYSIS: 13803 return "An observation of the hemolysis index of the specimen in g/L"; 13804 case ICTERUS: 13805 return "An observation that describes the icterus index of the specimen. It is recommended to use mMol/L of bilirubin"; 13806 case LIPEMIA: 13807 return "An observation used to describe the Lipemia Index of the specimen. It is recommended to use the optical turbidity at 600 nm (in absorbance units)."; 13808 case VOLUME: 13809 return "An observation that reports the volume of a sample."; 13810 case AVAILABLE: 13811 return "The available quantity of specimen. This is the current quantity minus any planned consumption (e.g., tests that are planned)"; 13812 case CONSUMPTION: 13813 return "The quantity of specimen that is used each time the equipment uses this substance"; 13814 case CURRENT: 13815 return "The current quantity of the specimen, i.e., initial quantity minus what has been actually used."; 13816 case INITIAL: 13817 return "The initial quantity of the specimen in inventory"; 13818 case _ANNOTATIONTYPE: 13819 return "AnnotationType"; 13820 case _ACTPATIENTANNOTATIONTYPE: 13821 return "Description:Provides a categorization for annotations recorded directly against the patient ."; 13822 case ANNDI: 13823 return "Description:A note that is specific to a patient's diagnostic images, either historical, current or planned."; 13824 case ANNGEN: 13825 return "Description:A general or uncategorized note."; 13826 case ANNIMM: 13827 return "A note that is specific to a patient's immunizations, either historical, current or planned."; 13828 case ANNLAB: 13829 return "Description:A note that is specific to a patient's laboratory results, either historical, current or planned."; 13830 case ANNMED: 13831 return "Description:A note that is specific to a patient's medications, either historical, current or planned."; 13832 case _GENETICOBSERVATIONTYPE: 13833 return "Description: None provided"; 13834 case GENE: 13835 return "Description: A DNA segment that contributes to phenotype/function. In the absence of demonstrated function a gene may be characterized by sequence, transcription or homology"; 13836 case _IMMUNIZATIONOBSERVATIONTYPE: 13837 return "Description: Observation codes which describe characteristics of the immunization material."; 13838 case OBSANTC: 13839 return "Description: Indicates the valid antigen count."; 13840 case OBSANTV: 13841 return "Description: Indicates whether an antigen is valid or invalid."; 13842 case _INDIVIDUALCASESAFETYREPORTTYPE: 13843 return "A code that is used to indicate the type of case safety report received from sender. The current code example reference is from the International Conference on Harmonisation (ICH) Expert Workgroup guideline on Clinical Safety Data Management: Data Elements for Transmission of Individual Case Safety Reports. The unknown/unavailable option allows the transmission of information from a secondary sender where the initial sender did not specify the type of report.\r\n\n Example concepts include: Spontaneous, Report from study, Other."; 13844 case PATADVEVNT: 13845 return "Indicates that the ICSR is describing problems that a patient experienced after receiving a vaccine product."; 13846 case VACPROBLEM: 13847 return "Indicates that the ICSR is describing a problem with the actual vaccine product such as physical defects (cloudy, particulate matter) or inability to confer immunity."; 13848 case _LOINCOBSERVATIONACTCONTEXTAGETYPE: 13849 return "Definition:The set of LOINC codes for the act of determining the period of time that has elapsed since an entity was born or created."; 13850 case _216119: 13851 return "Definition:Estimated age."; 13852 case _216127: 13853 return "Definition:Reported age."; 13854 case _295535: 13855 return "Definition:Calculated age."; 13856 case _305250: 13857 return "Definition:General specification of age with no implied method of determination."; 13858 case _309724: 13859 return "Definition:Age at onset of associated adverse event; no implied method of determination."; 13860 case _MEDICATIONOBSERVATIONTYPE: 13861 return "MedicationObservationType"; 13862 case REPHALFLIFE: 13863 return "Description:This observation represents an 'average' or 'expected' half-life typical of the product."; 13864 case SPLCOATING: 13865 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, indicating whether it has one or more coatings such as sugar coating, film coating, or enteric coating. Only coatings to the external surface or the dosage form should be considered (for example, coatings to individual pellets or granules inside a capsule or tablet are excluded from consideration).\r\n\n \n Constraints: The Observation.value must be a Boolean (BL) with true for the presence or false for the absence of one or more coatings on a solid dosage form."; 13866 case SPLCOLOR: 13867 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, specifying the color or colors that most predominantly define the appearance of the dose form. SPLCOLOR is not an FDA specification for the actual color of solid dosage forms or the names of colors that can appear in labeling.\r\n\n \n Constraints: The Observation.value must be a single coded value or a list of multiple coded values, specifying one or more distinct colors that approximate of the color(s) of distinct areas of the solid dosage form, such as the different sides of a tablet or one-part capsule, or the different halves of a two-part capsule. Bands on banded capsules, regardless of the color, are not considered when assigning an SPLCOLOR. Imprints on the dosage form, regardless of their color are not considered when assigning an SPLCOLOR. If more than one color exists on a particular side or half, then the most predominant color on that side or half is recorded. If the gelatin capsule shell is colorless and transparent, use the predominant color of the contents that appears through the colorless and transparent capsule shell. Colors can include: Black;Gray;White;Red;Pink;Purple;Green;Yellow;Orange;Brown;Blue;Turquoise."; 13868 case SPLIMAGE: 13869 return "Description: A characteristic representing a single file reference that contains two or more views of the same dosage form of the product; in most cases this should represent front and back views of the dosage form, but occasionally additional views might be needed in order to capture all of the important physical characteristics of the dosage form. Any imprint and/or symbol should be clearly identifiable, and the viewer should not normally need to rotate the image in order to read it. Images that are submitted with SPL should be included in the same directory as the SPL file."; 13870 case SPLIMPRINT: 13871 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, specifying the alphanumeric text that appears on the solid dosage form, including text that is embossed, debossed, engraved or printed with ink. The presence of other non-textual distinguishing marks or symbols is recorded by SPLSYMBOL.\r\n\n \n Examples: Included in SPLIMPRINT are alphanumeric text that appears on the bands of banded capsules and logos and other symbols that can be interpreted as letters or numbers.\r\n\n \n Constraints: The Observation.value must be of type Character String (ST). Excluded from SPLIMPRINT are internal and external cut-outs in the form of alphanumeric text and the letter 'R' with a circle around it (when referring to a registered trademark) and the letters 'TM' (when referring to a 'trade mark'). To record text, begin on either side or part of the dosage form. Start at the top left and progress as one would normally read a book. Enter a semicolon to show separation between words or line divisions."; 13872 case SPLSCORING: 13873 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, specifying the number of equal pieces that the solid dosage form can be divided into using score line(s). \r\n\n \n Example: One score line creating two equal pieces is given a value of 2, two parallel score lines creating three equal pieces is given a value of 3.\r\n\n \n Constraints: Whether three parallel score lines create four equal pieces or two intersecting score lines create two equal pieces using one score line and four equal pieces using both score lines, both have the scoring value of 4. Solid dosage forms that are not scored are given a value of 1. Solid dosage forms that can only be divided into unequal pieces are given a null-value with nullFlavor other (OTH)."; 13874 case SPLSHAPE: 13875 return "Description: A characteristic of an oral solid dosage form of a medicinal product, specifying the two dimensional representation of the solid dose form, in terms of the outside perimeter of a solid dosage form when the dosage form, resting on a flat surface, is viewed from directly above, including slight rounding of corners. SPLSHAPE does not include embossing, scoring, debossing, or internal cut-outs. SPLSHAPE is independent of the orientation of the imprint and logo. Shapes can include: Triangle (3 sided); Square; Round; Semicircle; Pentagon (5 sided); Diamond; Double circle; Bullet; Hexagon (6 sided); Rectangle; Gear; Capsule; Heptagon (7 sided); Trapezoid; Oval; Clover; Octagon (8 sided); Tear; Freeform."; 13876 case SPLSIZE: 13877 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, specifying the longest single dimension of the solid dosage form as a physical quantity in the dimension of length (e.g., 3 mm). The length is should be specified in millimeters and should be rounded to the nearest whole millimeter.\r\n\n \n Example: SPLSIZE for a rectangular shaped tablet is the length and SPLSIZE for a round shaped tablet is the diameter."; 13878 case SPLSYMBOL: 13879 return "Definition: A characteristic of an oral solid dosage form of a medicinal product, to describe whether or not the medicinal product has a mark or symbol appearing on it for easy and definite recognition. Score lines, letters, numbers, and internal and external cut-outs are not considered marks or symbols. See SPLSCORING and SPLIMPRINT for these characteristics.\r\n\n \n Constraints: The Observation.value must be a Boolean (BL) with <u>true</u> indicating the presence and <u>false</u> for the absence of marks or symbols.\r\n\n \n Example:"; 13880 case _OBSERVATIONISSUETRIGGERCODEDOBSERVATIONTYPE: 13881 return "Distinguishes the kinds of coded observations that could be the trigger for clinical issue detection. These are observations that are not measurable, but instead can be defined with codes. Coded observation types include: Allergy, Intolerance, Medical Condition, Pregnancy status, etc."; 13882 case _CASETRANSMISSIONMODE: 13883 return "Code for the mechanism by which disease was acquired by the living subject involved in the public health case. Includes sexually transmitted, airborne, bloodborne, vectorborne, foodborne, zoonotic, nosocomial, mechanical, dermal, congenital, environmental exposure, indeterminate."; 13884 case AIRTRNS: 13885 return "Communication of an agent from a living subject or environmental source to a living subject through indirect contact via oral or nasal inhalation."; 13886 case ANANTRNS: 13887 return "Communication of an agent from one animal to another proximate animal."; 13888 case ANHUMTRNS: 13889 return "Communication of an agent from an animal to a proximate person."; 13890 case BDYFLDTRNS: 13891 return "Communication of an agent from one living subject to another living subject through direct contact with any body fluid."; 13892 case BLDTRNS: 13893 return "Communication of an agent to a living subject through direct contact with blood or blood products whether the contact with blood is part of a therapeutic procedure or not."; 13894 case DERMTRNS: 13895 return "Communication of an agent from a living subject or environmental source to a living subject via agent migration through intact skin."; 13896 case ENVTRNS: 13897 return "Communication of an agent from an environmental surface or source to a living subject by direct contact."; 13898 case FECTRNS: 13899 return "Communication of an agent from a living subject or environmental source to a living subject through oral contact with material contaminated by person or animal fecal material."; 13900 case FOMTRNS: 13901 return "Communication of an agent from an non-living material to a living subject through direct contact."; 13902 case FOODTRNS: 13903 return "Communication of an agent from a food source to a living subject via oral consumption."; 13904 case HUMHUMTRNS: 13905 return "Communication of an agent from a person to a proximate person."; 13906 case INDTRNS: 13907 return "Communication of an agent to a living subject via an undetermined route."; 13908 case LACTTRNS: 13909 return "Communication of an agent from one living subject to another living subject through direct contact with mammalian milk or colostrum."; 13910 case NOSTRNS: 13911 return "Communication of an agent from any entity to a living subject while the living subject is in the patient role in a healthcare facility."; 13912 case PARTRNS: 13913 return "Communication of an agent from a living subject or environmental source to a living subject where the acquisition of the agent is not via the alimentary canal."; 13914 case PLACTRNS: 13915 return "Communication of an agent from a living subject to the progeny of that living subject via agent migration across the maternal-fetal placental membranes while in utero."; 13916 case SEXTRNS: 13917 return "Communication of an agent from one living subject to another living subject through direct contact with genital or oral tissues as part of a sexual act."; 13918 case TRNSFTRNS: 13919 return "Communication of an agent from one living subject to another living subject through direct contact with blood or blood products where the contact with blood is part of a therapeutic procedure."; 13920 case VECTRNS: 13921 return "Communication of an agent from a living subject acting as a required intermediary in the agent transmission process to a recipient living subject via direct contact."; 13922 case WATTRNS: 13923 return "Communication of an agent from a contaminated water source to a living subject whether the water is ingested as a food or not. The route of entry of the water may be through any bodily orifice."; 13924 case _OBSERVATIONQUALITYMEASUREATTRIBUTE: 13925 return "Codes used to define various metadata aspects of a health quality measure."; 13926 case AGGREGATE: 13927 return "Indicates that the observation is carrying out an aggregation calculation, contained in the value element."; 13928 case CMPMSRMTH: 13929 return "Indicates what method is used in a quality measure to combine the component measure results included in an composite measure."; 13930 case CMPMSRSCRWGHT: 13931 return "An attribute of a quality measure describing the weight this component measure score is to carry in determining the overall composite measure final score. The value is real value greater than 0 and less than 1.0. Each component measure score will be multiplied by its CMPMSRSCRWGHT and then summed with the other component measures to determine the final overall composite measure score. The sum across all CMPMSRSCRWGHT values within a single composite measure SHALL be 1.0. The value assigned is scoped to the composite measure referencing this component measure only."; 13932 case COPY: 13933 return "Identifies the organization(s) who own the intellectual property represented by the eMeasure."; 13934 case CRS: 13935 return "Summary of relevant clinical guidelines or other clinical recommendations supporting this eMeasure."; 13936 case DEF: 13937 return "Description of individual terms, provided as needed."; 13938 case DISC: 13939 return "Disclaimer information for the eMeasure."; 13940 case FINALDT: 13941 return "The timestamp when the eMeasure was last packaged in the Measure Authoring Tool."; 13942 case GUIDE: 13943 return "Used to allow measure developers to provide additional guidance for implementers to understand greater specificity than could be provided in the logic for data criteria."; 13944 case IDUR: 13945 return "Information on whether an increase or decrease in score is the preferred result \n(e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range)."; 13946 case ITMCNT: 13947 return "Describes the items counted by the measure (e.g., patients, encounters, procedures, etc.)"; 13948 case KEY: 13949 return "A significant word that aids in discoverability."; 13950 case MEDT: 13951 return "The end date of the measurement period."; 13952 case MSD: 13953 return "The start date of the measurement period."; 13954 case MSRADJ: 13955 return "The method of adjusting for clinical severity and conditions present at the start of care that can influence patient outcomes for making valid comparisons of outcome measures across providers. Indicates whether an eMeasure is subject to the statistical process for reducing, removing, or clarifying the influences of confounding factors to allow more useful comparisons."; 13956 case MSRAGG: 13957 return "Describes how to combine information calculated based on logic in each of several populations into one summarized result. It can also be used to describe how to risk adjust the data based on supplemental data elements described in the eMeasure. (e.g., pneumonia hospital measures antibiotic selection in the ICU versus non-ICU and then the roll-up of the two). \r\n\n \n Open Issue: The description does NOT align well with the definition used in the HQMF specfication; correct the MSGAGG definition, and the possible distinction of MSRAGG as a child of AGGREGATE."; 13958 case MSRIMPROV: 13959 return "Information on whether an increase or decrease in score is the preferred result. This should reflect information on which way is better, an increase or decrease in score."; 13960 case MSRJUR: 13961 return "The list of jurisdiction(s) for which the measure applies."; 13962 case MSRRPTR: 13963 return "Type of person or organization that is expected to report the issue."; 13964 case MSRRPTTIME: 13965 return "The maximum time that may elapse following completion of the measure until the measure report must be sent to the receiver."; 13966 case MSRSCORE: 13967 return "Indicates how the calculation is performed for the eMeasure \n(e.g., proportion, continuous variable, ratio)"; 13968 case MSRSET: 13969 return "Location(s) in which care being measured is rendered\r\n\n Usage Note: MSRSET is used rather than RoleCode because the setting applies to what is being measured, as opposed to participating directly in the health quality measure documantion itself)."; 13970 case MSRTOPIC: 13971 return "health quality measure topic type"; 13972 case MSRTP: 13973 return "The time period for which the eMeasure applies."; 13974 case MSRTYPE: 13975 return "Indicates whether the eMeasure is used to examine a process or an outcome over time \n(e.g., Structure, Process, Outcome)."; 13976 case RAT: 13977 return "Succinct statement of the need for the measure. Usually includes statements pertaining to Importance criterion: impact, gap in care and evidence."; 13978 case REF: 13979 return "Identifies bibliographic citations or references to clinical practice guidelines, sources of evidence, or other relevant materials supporting the intent and rationale of the eMeasure."; 13980 case SDE: 13981 return "Comparison of results across strata can be used to show where disparities exist or where there is a need to expose differences in results. For example, Centers for Medicare & Medicaid Services (CMS) in the U.S. defines four required Supplemental Data Elements (payer, ethnicity, race, and gender), which are variables used to aggregate data into various subgroups. Additional supplemental data elements required for risk adjustment or other purposes of data aggregation can be included in the Supplemental Data Element section."; 13982 case STRAT: 13983 return "Describes the strata for which the measure is to be evaluated. There are three examples of reasons for stratification based on existing work. These include: (1) evaluate the measure based on different age groupings within the population described in the measure (e.g., evaluate the whole [age 14-25] and each sub-stratum [14-19] and [20-25]); (2) evaluate the eMeasure based on either a specific condition, a specific discharge location, or both; (3) evaluate the eMeasure based on different locations within a facility (e.g., evaluate the overall rate for all intensive care units and also some strata include additional findings [specific birth weights for neonatal intensive care units])."; 13984 case TRANF: 13985 return "Can be a URL or hyperlinks that link to the transmission formats that are specified for a particular reporting program."; 13986 case USE: 13987 return "Usage notes."; 13988 case _OBSERVATIONSEQUENCETYPE: 13989 return "ObservationSequenceType"; 13990 case TIMEABSOLUTE: 13991 return "A sequence of values in the \"absolute\" time domain. This is the same time domain that all HL7 timestamps use. It is time as measured by the Gregorian calendar"; 13992 case TIMERELATIVE: 13993 return "A sequence of values in a \"relative\" time domain. The time is measured relative to the earliest effective time in the Observation Series containing this sequence."; 13994 case _OBSERVATIONSERIESTYPE: 13995 return "ObservationSeriesType"; 13996 case _ECGOBSERVATIONSERIESTYPE: 13997 return "ECGObservationSeriesType"; 13998 case REPRESENTATIVEBEAT: 13999 return "This Observation Series type contains waveforms of a \"representative beat\" (a.k.a. \"median beat\" or \"average beat\"). The waveform samples are measured in relative time, relative to the beginning of the beat as defined by the Observation Series effective time. The waveforms are not directly acquired from the subject, but rather algorithmically derived from the \"rhythm\" waveforms."; 14000 case RHYTHM: 14001 return "This Observation type contains ECG \"rhythm\" waveforms. The waveform samples are measured in absolute time (a.k.a. \"subject time\" or \"effective time\"). These waveforms are usually \"raw\" with some minimal amount of noise reduction and baseline filtering applied."; 14002 case _PATIENTIMMUNIZATIONRELATEDOBSERVATIONTYPE: 14003 return "Description: Reporting codes that are related to an immunization event."; 14004 case CLSSRM: 14005 return "Description: The class room associated with the patient during the immunization event."; 14006 case GRADE: 14007 return "Description: The school grade or level the patient was in when immunized."; 14008 case SCHL: 14009 return "Description: The school the patient attended when immunized."; 14010 case SCHLDIV: 14011 return "Description: The school division or district associated with the patient during the immunization event."; 14012 case TEACHER: 14013 return "Description: The patient's teacher when immunized."; 14014 case _POPULATIONINCLUSIONOBSERVATIONTYPE: 14015 return "Observation types for specifying criteria used to assert that a subject is included in a particular population."; 14016 case DENEX: 14017 return "Criteria which specify subjects who should be removed from the eMeasure population and denominator before determining if numerator criteria are met. Denominator exclusions are used in proportion and ratio measures to help narrow the denominator."; 14018 case DENEXCEP: 14019 return "Criteria which specify the removal of a subject, procedure or unit of measurement from the denominator, only if the numerator criteria are not met. Denominator exceptions allow for adjustment of the calculated score for those providers with higher risk populations. Denominator exceptions are used only in proportion eMeasures. They are not appropriate for ratio or continuous variable eMeasures. Denominator exceptions allow for the exercise of clinical judgment and should be specifically defined where capturing the information in a structured manner fits the clinical workflow. Generic denominator exception reasons used in proportion eMeasures fall into three general categories:\r\n\n \n Medical reasons\n Patient (or subject) reasons\n System reasons"; 14020 case DENOM: 14021 return "Criteria for specifying the entities to be evaluated by a specific quality measure, based on a shared common set of characteristics (within a specific measurement set to which a given measure belongs). The denominator can be the same as the initial population, or it may be a subset of the initial population to further constrain it for the purpose of the eMeasure. Different measures within an eMeasure set may have different denominators. Continuous Variable eMeasures do not have a denominator, but instead define a measure population."; 14022 case IPOP: 14023 return "Criteria for specifying the entities to be evaluated by a specific quality measure, based on a shared common set of characteristics (within a specific measurement set to which a given measure belongs)."; 14024 case IPPOP: 14025 return "Criteria for specifying the patients to be evaluated by a specific quality measure, based on a shared common set of characteristics (within a specific measurement set to which a given measure belongs). Details often include information based upon specific age groups, diagnoses, diagnostic and procedure codes, and enrollment periods."; 14026 case MSROBS: 14027 return "Defines the observation to be performed for each patient or event in the measure population. Measure observations for each case in the population are aggregated to determine the overall measure score for the population.\r\n\n \n Examples: \n \r\n\n \n the median time from arrival in the Emergency Room to departure\n the median time from decision to admit to a hospital to the actual admission for Emergency Room patients"; 14028 case MSRPOPL: 14029 return "Criteria for specifying\nthe measure population as a narrative description (e.g., all patients seen in the Emergency Department during the measurement period). This is used only in continuous variable eMeasures."; 14030 case MSRPOPLEX: 14031 return "Criteria for specifying subjects who should be removed from the eMeasure's Initial Population and Measure Population. Measure Population Exclusions are used in Continuous Variable measures to help narrow the Measure Population before determining the value(s) of the continuous variable(s)."; 14032 case NUMER: 14033 return "Criteria for specifying the processes or outcomes expected for each patient, procedure, or other unit of measurement defined in the denominator for proportion measures, or related to (but not directly derived from) the denominator for ratio measures (e.g., a numerator listing the number of central line blood stream infections and a denominator indicating the days per thousand of central line usage in a specific time period)."; 14034 case NUMEX: 14035 return "Criteria for specifying instances that should not be included in the numerator data. (e.g., if the number of central line blood stream infections per 1000 catheter days were to exclude infections with a specific bacterium, that bacterium would be listed as a numerator exclusion). Numerator Exclusions are used only in ratio eMeasures."; 14036 case _PREFERENCEOBSERVATIONTYPE: 14037 return "Types of observations that can be made about Preferences."; 14038 case PREFSTRENGTH: 14039 return "An observation about how important a preference is to the target of the preference."; 14040 case ADVERSEREACTION: 14041 return "Indicates that the observation is of an unexpected negative occurrence in the subject suspected to result from the subject's exposure to one or more agents. Observation values would be the symptom resulting from the reaction."; 14042 case ASSERTION: 14043 return "Description:Refines classCode OBS to indicate an observation in which observation.value contains a finding or other nominalized statement, where the encoded information in Observation.value is not altered by Observation.code. For instance, observation.code=\"ASSERTION\" and observation.value=\"fracture of femur present\" is an assertion of a clinical finding of femur fracture."; 14044 case CASESER: 14045 return "Definition:An observation that provides a characterization of the level of harm to an investigation subject as a result of a reaction or event."; 14046 case CDIO: 14047 return "An observation that states whether the disease was likely acquired outside the jurisdiction of observation, and if so, the nature of the inter-jurisdictional relationship.\r\n\n \n OpenIssue: This code could be moved to LOINC if it can be done before there are significant implemenations using it."; 14048 case CRIT: 14049 return "A clinical judgment as to the worst case result of a future exposure (including substance administration). When the worst case result is assessed to have a life-threatening or organ system threatening potential, it is considered to be of high criticality."; 14050 case CTMO: 14051 return "An observation that states the mechanism by which disease was acquired by the living subject involved in the public health case.\r\n\n \n OpenIssue: This code could be moved to LOINC if it can be done before there are significant implemenations using it."; 14052 case DX: 14053 return "Includes all codes defining types of indications such as diagnosis, symptom and other indications such as contrast agents for lab tests."; 14054 case ADMDX: 14055 return "Admitting diagnosis are the diagnoses documented for administrative purposes as the basis for a hospital admission."; 14056 case DISDX: 14057 return "Discharge diagnosis are the diagnoses documented for administrative purposes as the time of hospital discharge."; 14058 case INTDX: 14059 return "Intermediate diagnoses are those diagnoses documented for administrative purposes during the course of a hospital stay."; 14060 case NOI: 14061 return "The type of injury that the injury coding specifies."; 14062 case GISTIER: 14063 return "Description: Accuracy determined as per the GIS tier code system."; 14064 case HHOBS: 14065 return "Indicates that the observation is of a person?s living situation in a household including the household composition and circumstances."; 14066 case ISSUE: 14067 return "There is a clinical issue for the therapy that makes continuation of the therapy inappropriate.\r\n\n \n Open Issue: The definition of this code does not correctly represent the concept space of its specializations (children)"; 14068 case _ACTADMINISTRATIVEDETECTEDISSUECODE: 14069 return "Identifies types of detectyed issues for Act class \"ALRT\" for the administrative and patient administrative acts domains."; 14070 case _ACTADMINISTRATIVEAUTHORIZATIONDETECTEDISSUECODE: 14071 return "ActAdministrativeAuthorizationDetectedIssueCode"; 14072 case NAT: 14073 return "The requesting party has insufficient authorization to invoke the interaction."; 14074 case SUPPRESSED: 14075 return "Description: One or more records in the query response have been suppressed due to consent or privacy restrictions."; 14076 case VALIDAT: 14077 return "Description:The specified element did not pass business-rule validation."; 14078 case KEY204: 14079 return "The ID of the patient, order, etc., was not found. Used for transactions other than additions, e.g. transfer of a non-existent patient."; 14080 case KEY205: 14081 return "The ID of the patient, order, etc., already exists. Used in response to addition transactions (Admit, New Order, etc.)."; 14082 case COMPLY: 14083 return "There may be an issue with the patient complying with the intentions of the proposed therapy"; 14084 case DUPTHPY: 14085 return "The proposed therapy appears to duplicate an existing therapy"; 14086 case DUPTHPCLS: 14087 return "Description:The proposed therapy appears to have the same intended therapeutic benefit as an existing therapy, though the specific mechanisms of action vary."; 14088 case DUPTHPGEN: 14089 return "Description:The proposed therapy appears to have the same intended therapeutic benefit as an existing therapy and uses the same mechanisms of action as the existing therapy."; 14090 case ABUSE: 14091 return "Description:The proposed therapy is frequently misused or abused and therefore should be used with caution and/or monitoring."; 14092 case FRAUD: 14093 return "Description:The request is suspected to have a fraudulent basis."; 14094 case PLYDOC: 14095 return "A similar or identical therapy was recently ordered by a different practitioner."; 14096 case PLYPHRM: 14097 return "This patient was recently supplied a similar or identical therapy from a different pharmacy or supplier."; 14098 case DOSE: 14099 return "Proposed dosage instructions for therapy differ from standard practice."; 14100 case DOSECOND: 14101 return "Description:Proposed dosage is inappropriate due to patient's medical condition."; 14102 case DOSEDUR: 14103 return "Proposed length of therapy differs from standard practice."; 14104 case DOSEDURH: 14105 return "Proposed length of therapy is longer than standard practice"; 14106 case DOSEDURHIND: 14107 return "Proposed length of therapy is longer than standard practice for the identified indication or diagnosis"; 14108 case DOSEDURL: 14109 return "Proposed length of therapy is shorter than that necessary for therapeutic effect"; 14110 case DOSEDURLIND: 14111 return "Proposed length of therapy is shorter than standard practice for the identified indication or diagnosis"; 14112 case DOSEH: 14113 return "Proposed dosage exceeds standard practice"; 14114 case DOSEHINDA: 14115 return "Proposed dosage exceeds standard practice for the patient's age"; 14116 case DOSEHIND: 14117 return "High Dose for Indication Alert"; 14118 case DOSEHINDSA: 14119 return "Proposed dosage exceeds standard practice for the patient's height or body surface area"; 14120 case DOSEHINDW: 14121 return "Proposed dosage exceeds standard practice for the patient's weight"; 14122 case DOSEIVL: 14123 return "Proposed dosage interval/timing differs from standard practice"; 14124 case DOSEIVLIND: 14125 return "Proposed dosage interval/timing differs from standard practice for the identified indication or diagnosis"; 14126 case DOSEL: 14127 return "Proposed dosage is below suggested therapeutic levels"; 14128 case DOSELINDA: 14129 return "Proposed dosage is below suggested therapeutic levels for the patient's age"; 14130 case DOSELIND: 14131 return "Low Dose for Indication Alert"; 14132 case DOSELINDSA: 14133 return "Proposed dosage is below suggested therapeutic levels for the patient's height or body surface area"; 14134 case DOSELINDW: 14135 return "Proposed dosage is below suggested therapeutic levels for the patient's weight"; 14136 case MDOSE: 14137 return "Description:The maximum quantity of this drug allowed to be administered within a particular time-range (month, year, lifetime) has been reached or exceeded."; 14138 case OBSA: 14139 return "Proposed therapy may be inappropriate or contraindicated due to conditions or characteristics of the patient"; 14140 case AGE: 14141 return "Proposed therapy may be inappropriate or contraindicated due to patient age"; 14142 case ADALRT: 14143 return "Proposed therapy is outside of the standard practice for an adult patient."; 14144 case GEALRT: 14145 return "Proposed therapy is outside of standard practice for a geriatric patient."; 14146 case PEALRT: 14147 return "Proposed therapy is outside of the standard practice for a pediatric patient."; 14148 case COND: 14149 return "Proposed therapy may be inappropriate or contraindicated due to an existing/recent patient condition or diagnosis"; 14150 case HGHT: 14151 return ""; 14152 case LACT: 14153 return "Proposed therapy may be inappropriate or contraindicated when breast-feeding"; 14154 case PREG: 14155 return "Proposed therapy may be inappropriate or contraindicated during pregnancy"; 14156 case WGHT: 14157 return ""; 14158 case CREACT: 14159 return "Description:Proposed therapy may be inappropriate or contraindicated because of a common but non-patient specific reaction to the product.\r\n\n \n Example:There is no record of a specific sensitivity for the patient, but the presence of the sensitivity is common and therefore caution is warranted."; 14160 case GEN: 14161 return "Proposed therapy may be inappropriate or contraindicated due to patient genetic indicators."; 14162 case GEND: 14163 return "Proposed therapy may be inappropriate or contraindicated due to patient gender."; 14164 case LAB: 14165 return "Proposed therapy may be inappropriate or contraindicated due to recent lab test results"; 14166 case REACT: 14167 return "Proposed therapy may be inappropriate or contraindicated based on the potential for a patient reaction to the proposed product"; 14168 case ALGY: 14169 return "Proposed therapy may be inappropriate or contraindicated because of a recorded patient allergy to the proposed product. (Allergies are immune based reactions.)"; 14170 case INT: 14171 return "Proposed therapy may be inappropriate or contraindicated because of a recorded patient intolerance to the proposed product. (Intolerances are non-immune based sensitivities.)"; 14172 case RREACT: 14173 return "Proposed therapy may be inappropriate or contraindicated because of a potential patient reaction to a cross-sensitivity related product."; 14174 case RALG: 14175 return "Proposed therapy may be inappropriate or contraindicated because of a recorded patient allergy to a cross-sensitivity related product. (Allergies are immune based reactions.)"; 14176 case RAR: 14177 return "Proposed therapy may be inappropriate or contraindicated because of a recorded prior adverse reaction to a cross-sensitivity related product."; 14178 case RINT: 14179 return "Proposed therapy may be inappropriate or contraindicated because of a recorded patient intolerance to a cross-sensitivity related product. (Intolerances are non-immune based sensitivities.)"; 14180 case BUS: 14181 return "Description:A local business rule relating multiple elements has been violated."; 14182 case CODEINVAL: 14183 return "Description:The specified code is not valid against the list of codes allowed for the element."; 14184 case CODEDEPREC: 14185 return "Description:The specified code has been deprecated and should no longer be used. Select another code from the code system."; 14186 case FORMAT: 14187 return "Description:The element does not follow the formatting or type rules defined for the field."; 14188 case ILLEGAL: 14189 return "Description:The request is missing elements or contains elements which cause it to not meet the legal standards for actioning."; 14190 case LENRANGE: 14191 return "Description:The length of the data specified falls out of the range defined for the element."; 14192 case LENLONG: 14193 return "Description:The length of the data specified is greater than the maximum length defined for the element."; 14194 case LENSHORT: 14195 return "Description:The length of the data specified is less than the minimum length defined for the element."; 14196 case MISSCOND: 14197 return "Description:The specified element must be specified with a non-null value under certain conditions. In this case, the conditions are true but the element is still missing or null."; 14198 case MISSMAND: 14199 return "Description:The specified element is mandatory and was not included in the instance."; 14200 case NODUPS: 14201 return "Description:More than one element with the same value exists in the set. Duplicates not permission in this set in a set."; 14202 case NOPERSIST: 14203 return "Description: Element in submitted message will not persist in data storage based on detected issue."; 14204 case REPRANGE: 14205 return "Description:The number of repeating elements falls outside the range of the allowed number of repetitions."; 14206 case MAXOCCURS: 14207 return "Description:The number of repeating elements is above the maximum number of repetitions allowed."; 14208 case MINOCCURS: 14209 return "Description:The number of repeating elements is below the minimum number of repetitions allowed."; 14210 case _ACTADMINISTRATIVERULEDETECTEDISSUECODE: 14211 return "ActAdministrativeRuleDetectedIssueCode"; 14212 case KEY206: 14213 return "Description: Metadata associated with the identification (e.g. name or gender) does not match the identification being verified."; 14214 case OBSOLETE: 14215 return "Description: One or more records in the query response have a status of 'obsolete'."; 14216 case _ACTSUPPLIEDITEMDETECTEDISSUECODE: 14217 return "Identifies types of detected issues regarding the administration or supply of an item to a patient."; 14218 case _ADMINISTRATIONDETECTEDISSUECODE: 14219 return "Administration of the proposed therapy may be inappropriate or contraindicated as proposed"; 14220 case _APPROPRIATENESSDETECTEDISSUECODE: 14221 return "AppropriatenessDetectedIssueCode"; 14222 case _INTERACTIONDETECTEDISSUECODE: 14223 return "InteractionDetectedIssueCode"; 14224 case FOOD: 14225 return "Proposed therapy may interact with certain foods"; 14226 case TPROD: 14227 return "Proposed therapy may interact with an existing or recent therapeutic product"; 14228 case DRG: 14229 return "Proposed therapy may interact with an existing or recent drug therapy"; 14230 case NHP: 14231 return "Proposed therapy may interact with existing or recent natural health product therapy"; 14232 case NONRX: 14233 return "Proposed therapy may interact with a non-prescription drug (e.g. alcohol, tobacco, Aspirin)"; 14234 case PREVINEF: 14235 return "Definition:The same or similar treatment has previously been attempted with the patient without achieving a positive effect."; 14236 case DACT: 14237 return "Description:Proposed therapy may be contraindicated or ineffective based on an existing or recent drug therapy."; 14238 case TIME: 14239 return "Description:Proposed therapy may be inappropriate or ineffective based on the proposed start or end time."; 14240 case ALRTENDLATE: 14241 return "Definition:Proposed therapy may be inappropriate or ineffective because the end of administration is too close to another planned therapy."; 14242 case ALRTSTRTLATE: 14243 return "Definition:Proposed therapy may be inappropriate or ineffective because the start of administration is too late after the onset of the condition."; 14244 case _TIMINGDETECTEDISSUECODE: 14245 return "Proposed therapy may be inappropriate or ineffective based on the proposed start or end time."; 14246 case ENDLATE: 14247 return "Proposed therapy may be inappropriate or ineffective because the end of administration is too close to another planned therapy"; 14248 case STRTLATE: 14249 return "Proposed therapy may be inappropriate or ineffective because the start of administration is too late after the onset of the condition"; 14250 case _SUPPLYDETECTEDISSUECODE: 14251 return "Supplying the product at this time may be inappropriate or indicate compliance issues with the associated therapy"; 14252 case ALLDONE: 14253 return "Definition:The requested action has already been performed and so this request has no effect"; 14254 case FULFIL: 14255 return "Definition:The therapy being performed is in some way out of alignment with the requested therapy."; 14256 case NOTACTN: 14257 return "Definition:The status of the request being fulfilled has changed such that it is no longer actionable. This may be because the request has expired, has already been completely fulfilled or has been otherwise stopped or disabled. (Not used for 'suspended' orders.)"; 14258 case NOTEQUIV: 14259 return "Definition:The therapy being performed is not sufficiently equivalent to the therapy which was requested."; 14260 case NOTEQUIVGEN: 14261 return "Definition:The therapy being performed is not generically equivalent (having the identical biological action) to the therapy which was requested."; 14262 case NOTEQUIVTHER: 14263 return "Definition:The therapy being performed is not therapeutically equivalent (having the same overall patient effect) to the therapy which was requested."; 14264 case TIMING: 14265 return "Definition:The therapy is being performed at a time which diverges from the time the therapy was requested"; 14266 case INTERVAL: 14267 return "Definition:The therapy action is being performed outside the bounds of the time period requested"; 14268 case MINFREQ: 14269 return "Definition:The therapy action is being performed too soon after the previous occurrence based on the requested frequency"; 14270 case HELD: 14271 return "Definition:There should be no actions taken in fulfillment of a request that has been held or suspended."; 14272 case TOOLATE: 14273 return "The patient is receiving a subsequent fill significantly later than would be expected based on the amount previously supplied and the therapy dosage instructions"; 14274 case TOOSOON: 14275 return "The patient is receiving a subsequent fill significantly earlier than would be expected based on the amount previously supplied and the therapy dosage instructions"; 14276 case HISTORIC: 14277 return "Description: While the record was accepted in the repository, there is a more recent version of a record of this type."; 14278 case PATPREF: 14279 return "Definition:The proposed therapy goes against preferences or consent constraints recorded in the patient's record."; 14280 case PATPREFALT: 14281 return "Definition:The proposed therapy goes against preferences or consent constraints recorded in the patient's record. An alternate therapy meeting those constraints is available."; 14282 case KSUBJ: 14283 return "Categorization of types of observation that capture the main clinical knowledge subject which may be a medication, a laboratory test, a disease."; 14284 case KSUBT: 14285 return "Categorization of types of observation that capture a knowledge subtopic which might be treatment, etiology, or prognosis."; 14286 case OINT: 14287 return "Hypersensitivity resulting in an adverse reaction upon exposure to an agent."; 14288 case ALG: 14289 return "Hypersensitivity to an agent caused by an immunologic response to an initial exposure"; 14290 case DALG: 14291 return "An allergy to a pharmaceutical product."; 14292 case EALG: 14293 return "An allergy to a substance other than a drug or a food. E.g. Latex, pollen, etc."; 14294 case FALG: 14295 return "An allergy to a substance generally consumed for nutritional purposes."; 14296 case DINT: 14297 return "Hypersensitivity resulting in an adverse reaction upon exposure to a drug."; 14298 case DNAINT: 14299 return "Hypersensitivity to an agent caused by a mechanism other than an immunologic response to an initial exposure"; 14300 case EINT: 14301 return "Hypersensitivity resulting in an adverse reaction upon exposure to environmental conditions."; 14302 case ENAINT: 14303 return "Hypersensitivity to an agent caused by a mechanism other than an immunologic response to an initial exposure"; 14304 case FINT: 14305 return "Hypersensitivity resulting in an adverse reaction upon exposure to food."; 14306 case FNAINT: 14307 return "Hypersensitivity to an agent caused by a mechanism other than an immunologic response to an initial exposure"; 14308 case NAINT: 14309 return "Hypersensitivity to an agent caused by a mechanism other than an immunologic response to an initial exposure"; 14310 case SEV: 14311 return "A subjective evaluation of the seriousness or intensity associated with another observation."; 14312 case _FDALABELDATA: 14313 return "FDA label data"; 14314 case FDACOATING: 14315 return "FDA label coating"; 14316 case FDACOLOR: 14317 return "FDA label color"; 14318 case FDAIMPRINTCD: 14319 return "FDA label imprint code"; 14320 case FDALOGO: 14321 return "FDA label logo"; 14322 case FDASCORING: 14323 return "FDA label scoring"; 14324 case FDASHAPE: 14325 return "FDA label shape"; 14326 case FDASIZE: 14327 return "FDA label size"; 14328 case _ROIOVERLAYSHAPE: 14329 return "Shape of the region on the object being referenced"; 14330 case CIRCLE: 14331 return "A circle defined by two (column,row) pairs. The first point is the center of the circle and the second point is a point on the perimeter of the circle."; 14332 case ELLIPSE: 14333 return "An ellipse defined by four (column,row) pairs, the first two points specifying the endpoints of the major axis and the second two points specifying the endpoints of the minor axis."; 14334 case POINT: 14335 return "A single point denoted by a single (column,row) pair, or multiple points each denoted by a (column,row) pair."; 14336 case POLY: 14337 return "A series of connected line segments with ordered vertices denoted by (column,row) pairs; if the first and last vertices are the same, it is a closed polygon."; 14338 case C: 14339 return "Description:Indicates that result data has been corrected."; 14340 case DIET: 14341 return "Code set to define specialized/allowed diets"; 14342 case BR: 14343 return "A diet exclusively composed of oatmeal, semolina, or rice, to be extremely easy to eat and digest."; 14344 case DM: 14345 return "A diet that uses carbohydrates sparingly. Typically with a restriction in daily energy content (e.g. 1600-2000 kcal)."; 14346 case FAST: 14347 return "No enteral intake of foot or liquids whatsoever, no smoking. Typically 6 to 8 hours before anesthesia."; 14348 case FORMULA: 14349 return "A diet consisting of a formula feeding, either for an infant or an adult, to provide nutrition either orally or through the gastrointestinal tract via tube, catheter or stoma."; 14350 case GF: 14351 return "Gluten free diet for celiac disease."; 14352 case LF: 14353 return "A diet low in fat, particularly to patients with hepatic diseases."; 14354 case LP: 14355 return "A low protein diet for patients with renal failure."; 14356 case LQ: 14357 return "A strictly liquid diet, that can be fully absorbed in the intestine, and therefore may not contain fiber. Used before enteral surgeries."; 14358 case LS: 14359 return "A diet low in sodium for patients with congestive heart failure and/or renal failure."; 14360 case N: 14361 return "A normal diet, i.e. no special preparations or restrictions for medical reasons. This is notwithstanding any preferences the patient might have regarding special foods, such as vegetarian, kosher, etc."; 14362 case NF: 14363 return "A no fat diet for acute hepatic diseases."; 14364 case PAF: 14365 return "Phenylketonuria diet."; 14366 case PAR: 14367 return "Patient is supplied with parenteral nutrition, typically described in terms of i.v. medications."; 14368 case RD: 14369 return "A diet that seeks to reduce body fat, typically low energy content (800-1600 kcal)."; 14370 case SCH: 14371 return "A diet that avoids ingredients that might cause digestion problems, e.g., avoid excessive fat, avoid too much fiber (cabbage, peas, beans)."; 14372 case SUPPLEMENT: 14373 return "A diet that is not intended to be complete but is added to other diets."; 14374 case T: 14375 return "This is not really a diet, since it contains little nutritional value, but is essentially just water. Used before coloscopy examinations."; 14376 case VLI: 14377 return "Diet with low content of the amino-acids valin, leucin, and isoleucin, for \"maple syrup disease.\""; 14378 case DRUGPRG: 14379 return "Definition: A public or government health program that administers and funds coverage for prescription drugs to assist program eligible who meet financial and health status criteria."; 14380 case F: 14381 return "Description:Indicates that a result is complete. No further results are to come. This maps to the 'complete' state in the observation result status code."; 14382 case PRLMN: 14383 return "Description:Indicates that a result is incomplete. There are further results to come. This maps to the 'active' state in the observation result status code."; 14384 case SECOBS: 14385 return "An observation identifying security metadata about an IT resource (data, information object, service, or system capability), which may be used to make access control decisions. Security metadata are used to name security labels. \r\n\n \n Rationale: According to ISO/TS 22600-3:2009(E) A.9.1.7 SECURITY LABEL MATCHING, Security label matching compares the initiator's clearance to the target's security label. All of the following must be true for authorization to be granted:\r\n\n \n The security policy identifiers shall be identical\n The classification level of the initiator shall be greater than or equal to that of the target (that is, there shall be at least one value in the classification list of the clearance greater than or equal to the classification of the target), and \n For each security category in the target label, there shall be a security category of the same type in the initiator's clearance and the initiator's classification level shall dominate that of the target.\n \n \n Examples: SecurityObservationType security label fields include:\r\n\n \n Confidentiality classification\n Compartment category\n Sensitivity category\n Security mechanisms used to ensure data integrity or to perform authorized data transformation\n Indicators of an IT resource completeness, veracity, reliability, trustworthiness, or provenance.\n \n \n Usage Note: SecurityObservationType codes designate security label field types, which are valued with an applicable SecurityObservationValue code as the \"security label tag\"."; 14386 case SECCATOBS: 14387 return "Type of security metadata observation made about the category of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions. Security category metadata is defined by ISO/IEC 2382-8:1998(E/F)/ T-REC-X.812-1995 as: \"A nonhierarchical grouping of sensitive information used to control access to data more finely than with hierarchical security classification alone.\"\r\n\n \n Rationale: A security category observation supports requirement to specify the type of IT resource to facilitate application of appropriate levels of information security according to a range of levels of impact or consequences that might result from the unauthorized disclosure, modification, or use of the information or information system. A resource is assigned to a specific category of information (e.g., privacy, medical, proprietary, financial, investigative, contractor sensitive, security management) defined by an organization or in some instances, by a specific law, Executive Order, directive, policy, or regulation. [FIPS 199]\r\n\n \n Examples: Types of security categories include:\r\n\n \n Compartment: A division of data into isolated blocks with separate security controls for the purpose of reducing risk. (ISO 2382-8). A security label tag that \"segments\" an IT resource by indicating that access and use is restricted to members of a defined community or project. (HL7 Healthcare Classification System) \n Sensitivity: The characteristic of an IT resource which implies its value or importance and may include its vulnerability. (ISO 7492-2) Privacy metadata for information perceived as undesirable to share. (HL7 Healthcare Classification System)"; 14388 case SECCLASSOBS: 14389 return "Type of security metadata observation made about the classification of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions. Security classification is defined by ISO/IEC 2382-8:1998(E/F)/ T-REC-X.812-1995 as: \"The determination of which specific degree of protection against access the data or information requires, together with a designation of that degree of protection.\" Security classification metadata is based on an analysis of applicable policies and the risk of financial, reputational, or other harm that could result from unauthorized disclosure.\r\n\n \n Rationale: A security classification observation may indicate that the confidentiality level indicated by an Act or Role confidentiality attribute has been overridden by the entity responsible for ascribing the SecurityClassificationObservationValue. This supports the business requirement for increasing or decreasing the level of confidentiality (classification or declassification) based on parameters beyond the original assignment of an Act or Role confidentiality.\r\n\n \n Examples: Types of security classification include: HL7 Confidentiality Codes such as very restricted, unrestricted, and normal. Intelligence community examples include top secret, secret, and confidential.\r\n\n \n Usage Note: Security classification observation type codes designate security label field types, which are valued with an applicable SecurityClassificationObservationValue code as the \"security label tag\"."; 14390 case SECCONOBS: 14391 return "Type of security metadata observation made about the control of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions. Security control metadata convey instructions to users and receivers for secure distribution, transmission, and storage; dictate obligations or mandated actions; specify any action prohibited by refrain policy such as dissemination controls; and stipulate the permissible purpose of use of an IT resource. \r\n\n \n Rationale: A security control observation supports requirement to specify applicable management, operational, and technical controls (i.e., safeguards or countermeasures) prescribed for an information system to protect the confidentiality, integrity, and availability of the system and its information. [FIPS 199]\r\n\n \n Examples: Types of security control metadata include: \r\n\n \n handling caveats\n dissemination controls\n obligations\n refrain policies\n purpose of use constraints"; 14392 case SECINTOBS: 14393 return "Type of security metadata observation made about the integrity of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions.\r\n\n \n Rationale: A security integrity observation supports the requirement to guard against improper information modification or destruction, and includes ensuring information non-repudiation and authenticity. (44 U.S.C., SEC. 3542)\r\n\n \n Examples: Types of security integrity metadata include: \r\n\n \n Integrity status, which indicates the completeness or workflow status of an IT resource (data, information object, service, or system capability)\n Integrity confidence, which indicates the reliability and trustworthiness of an IT resource\n Integrity control, which indicates pertinent handling caveats, obligations, refrain policies, and purpose of use for the resource\n Data integrity, which indicate the security mechanisms used to ensure that the accuracy and consistency are preserved regardless of changes made (ISO/IEC DIS 2382-8)\n Alteration integrity, which indicate the security mechanisms used for authorized transformations of the resource\n Integrity provenance, which indicates the entity responsible for a report or assertion relayed \"second-hand\" about an IT resource"; 14394 case SECALTINTOBS: 14395 return "Type of security metadata observation made about the alteration integrity of an IT resource (data, information object, service, or system capability), which indicates the mechanism used for authorized transformations of the resource.\r\n\n \n Examples: Types of security alteration integrity observation metadata, which may value the observation with a code used to indicate the mechanism used for authorized transformation of an IT resource, including: \r\n\n \n translation\n syntactic transformation\n semantic mapping\n redaction\n masking\n pseudonymization\n anonymization"; 14396 case SECDATINTOBS: 14397 return "Type of security metadata observation made about the data integrity of an IT resource (data, information object, service, or system capability), which indicates the security mechanism used to preserve resource accuracy and consistency. Data integrity is defined by ISO 22600-23.3.21 as: \"The property that data has not been altered or destroyed in an unauthorized manner\", and by ISO/IEC 2382-8: The property of data whose accuracy and consistency are preserved regardless of changes made.\"\r\n\n \n Examples: Types of security data integrity observation metadata, which may value the observation, include cryptographic hash function and digital signature."; 14398 case SECINTCONOBS: 14399 return "Type of security metadata observation made about the integrity confidence of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions.\r\n\n \n Examples: Types of security integrity confidence observation metadata, which may value the observation, include highly reliable, uncertain reliability, and not reliable.\r\n\n \n Usage Note: A security integrity confidence observation on an Act may indicate that a valued Act.uncertaintycode attribute has been overridden by the entity responsible for ascribing the SecurityIntegrityConfidenceObservationValue. This supports the business requirements for increasing or decreasing the assessment of the reliability or trustworthiness of an IT resource based on parameters beyond the original assignment of an Act statement level of uncertainty."; 14400 case SECINTPRVOBS: 14401 return "Type of security metadata observation made about the provenance integrity of an IT resource (data, information object, service, or system capability), which indicates the lifecycle completeness of an IT resource in terms of workflow status such as its creation, modification, suspension, and deletion; locations in which the resource has been collected or archived, from which it may be retrieved, and the history of its distribution and disclosure. Integrity provenance metadata about an IT resource may be used to assess its veracity, reliability, and trustworthiness.\r\n\n \n Examples: Types of security integrity provenance observation metadata, which may value the observation about an IT resource, include: \r\n\n \n completeness or workflow status, such as authentication\n the entity responsible for original authoring or informing about an IT resource\n the entity responsible for a report or assertion about an IT resource relayed â??second-handâ??\n the entity responsible for excerpting, transforming, or compiling an IT resource"; 14402 case SECINTPRVABOBS: 14403 return "Type of security metadata observation made about the integrity provenance of an IT resource (data, information object, service, or system capability), which indicates the entity that made assertions about the resource. The asserting entity may not be the original informant about the resource.\r\n\n \n Examples: Types of security integrity provenance asserted by observation metadata, which may value the observation, including: \r\n\n \n assertions about an IT resource by a patient\n assertions about an IT resource by a clinician\n assertions about an IT resource by a device"; 14404 case SECINTPRVRBOBS: 14405 return "Type of security metadata observation made about the integrity provenance of an IT resource (data, information object, service, or system capability), which indicates the entity that reported the existence of the resource. The reporting entity may not be the original author of the resource.\r\n\n \n Examples: Types of security integrity provenance reported by observation metadata, which may value the observation, include: \r\n\n \n reports about an IT resource by a patient\n reports about an IT resource by a clinician\n reports about an IT resource by a device"; 14406 case SECINTSTOBS: 14407 return "Type of security metadata observation made about the integrity status of an IT resource (data, information object, service, or system capability), which may be used to make access control decisions. Indicates the completeness of an IT resource in terms of workflow status, which may impact users that are authorized to access and use the resource.\r\n\n \n Examples: Types of security integrity status observation metadata, which may value the observation, include codes from the HL7 DocumentCompletion code system such as legally authenticated, in progress, and incomplete."; 14408 case SECTRSTOBS: 14409 return "An observation identifying trust metadata about an IT resource (data, information object, service, or system capability), which may be used as a trust attribute to populate a computable trust policy, trust credential, trust assertion, or trust label field in a security label or trust policy, which are principally used for authentication, authorization, and access control decisions."; 14410 case TRSTACCRDOBS: 14411 return "Type of security metadata observation made about the formal declaration by an authority or neutral third party that validates the technical, security, trust, and business practice conformance of Trust Agents to facilitate security, interoperability, and trust among participants within a security domain or trust framework."; 14412 case TRSTAGREOBS: 14413 return "Type of security metadata observation made about privacy and security requirements with which a security domain must comply. [ISO IEC 10181-1]"; 14414 case TRSTCERTOBS: 14415 return "Type of security metadata observation made about a set of security-relevant data issued by a security authority or trusted third party, together with security information which is used to provide the integrity and data origin authentication services for an IT resource (data, information object, service, or system capability). [Based on ISO IEC 10181-1]\r\n\n \n For example,\n \r\n\n \n A Certificate Policy (CP), which is a named set of rules that indicates the applicability of a certificate to a particular community and/or class of application with common security requirements. For example, a particular Certificate Policy might indicate the applicability of a type of certificate to the authentication of electronic data interchange transactions for the trading of goods within a given price range. [Trust Service Principles and Criteria for Certification Authorities Version 2.0 March 2011 Copyright 2011 by Canadian Institute of Chartered Accountants.\n A Certificate Practice Statement (CSP), which is a statement of the practices which an Authority employs in issuing and managing certificates. [Trust Service Principles and Criteria for Certification Authorities Version 2.0 March 2011 Copyright 2011 by Canadian Institute of Chartered Accountants.]"; 14416 case TRSTFWKOBS: 14417 return "Type of security metadata observation made about a complete set of contracts, regulations or commitments that enable participating actors to rely on certain assertions by other actors to fulfill their information security requirements. [Kantara Initiative]"; 14418 case TRSTLOAOBS: 14419 return "Type of security metadata observation made about the digital quality or reliability of a trust assertion, activity, capability, information exchange, mechanism, process, or protocol."; 14420 case TRSTMECOBS: 14421 return "Type of security metadata observation made about a security architecture system component that supports enforcement of security policies."; 14422 case SUBSIDFFS: 14423 return "Definition: A government health program that provides coverage on a fee for service basis for health services to persons meeting eligibility criteria such as income, location of residence, access to other coverages, health condition, and age, the cost of which is to some extent subsidized by public funds.\r\n\n \n Discussion: The structure and business processes for underwriting and administering a subsidized fee for service program is further specified by the Underwriter and Payer Role.class and Role.code."; 14424 case WRKCOMP: 14425 return "Definition: Government mandated program providing coverage, disability income, and vocational rehabilitation for injuries sustained in the work place or in the course of employment. Employers may either self-fund the program, purchase commercial coverage, or pay a premium to a government entity that administers the program. Employees may be required to pay premiums toward the cost of coverage as well."; 14426 case _ACTPROCEDURECODE: 14427 return "An identifying code for healthcare interventions/procedures."; 14428 case _ACTBILLABLESERVICECODE: 14429 return "Definition: An identifying code for billable services, as opposed to codes for similar services used to identify them for functional purposes."; 14430 case _HL7DEFINEDACTCODES: 14431 return "Domain provides the root for HL7-defined detailed or rich codes for the Act classes."; 14432 case COPAY: 14433 return ""; 14434 case DEDUCT: 14435 return ""; 14436 case DOSEIND: 14437 return ""; 14438 case PRA: 14439 return ""; 14440 case STORE: 14441 return "The act of putting something away for safe keeping. The \"something\" may be physical object such as a specimen, or information, such as observations regarding a specimen."; 14442 case NULL: 14443 return null; 14444 default: 14445 return "?"; 14446 } 14447 } 14448 14449 public String getDisplay() { 14450 switch (this) { 14451 case _ACTACCOUNTCODE: 14452 return "ActAccountCode"; 14453 case ACCTRECEIVABLE: 14454 return "account receivable"; 14455 case CASH: 14456 return "Cash"; 14457 case CC: 14458 return "credit card"; 14459 case AE: 14460 return "American Express"; 14461 case DN: 14462 return "Diner's Club"; 14463 case DV: 14464 return "Discover Card"; 14465 case MC: 14466 return "Master Card"; 14467 case V: 14468 return "Visa"; 14469 case PBILLACCT: 14470 return "patient billing account"; 14471 case _ACTADJUDICATIONCODE: 14472 return "ActAdjudicationCode"; 14473 case _ACTADJUDICATIONGROUPCODE: 14474 return "ActAdjudicationGroupCode"; 14475 case CONT: 14476 return "contract"; 14477 case DAY: 14478 return "day"; 14479 case LOC: 14480 return "location"; 14481 case MONTH: 14482 return "month"; 14483 case PERIOD: 14484 return "period"; 14485 case PROV: 14486 return "provider"; 14487 case WEEK: 14488 return "week"; 14489 case YEAR: 14490 return "year"; 14491 case AA: 14492 return "adjudicated with adjustments"; 14493 case ANF: 14494 return "adjudicated with adjustments and no financial impact"; 14495 case AR: 14496 return "adjudicated as refused"; 14497 case AS: 14498 return "adjudicated as submitted"; 14499 case _ACTADJUDICATIONRESULTACTIONCODE: 14500 return "ActAdjudicationResultActionCode"; 14501 case DISPLAY: 14502 return "Display"; 14503 case FORM: 14504 return "Print on Form"; 14505 case _ACTBILLABLEMODIFIERCODE: 14506 return "ActBillableModifierCode"; 14507 case CPTM: 14508 return "CPT modifier codes"; 14509 case HCPCSA: 14510 return "HCPCS Level II and Carrier-assigned"; 14511 case _ACTBILLINGARRANGEMENTCODE: 14512 return "ActBillingArrangementCode"; 14513 case BLK: 14514 return "block funding"; 14515 case CAP: 14516 return "capitation funding"; 14517 case CONTF: 14518 return "contract funding"; 14519 case FINBILL: 14520 return "financial"; 14521 case ROST: 14522 return "roster funding"; 14523 case SESS: 14524 return "sessional funding"; 14525 case FFS: 14526 return "fee for service"; 14527 case FFPS: 14528 return "first fill, part fill, partial strength"; 14529 case FFCS: 14530 return "first fill complete, partial strength"; 14531 case TFS: 14532 return "trial fill partial strength"; 14533 case _ACTBOUNDEDROICODE: 14534 return "ActBoundedROICode"; 14535 case ROIFS: 14536 return "fully specified ROI"; 14537 case ROIPS: 14538 return "partially specified ROI"; 14539 case _ACTCAREPROVISIONCODE: 14540 return "act care provision"; 14541 case _ACTCREDENTIALEDCARECODE: 14542 return "act credentialed care"; 14543 case _ACTCREDENTIALEDCAREPROVISIONPERSONCODE: 14544 return "act credentialed care provision peron"; 14545 case CACC: 14546 return "certified anatomic pathology and clinical pathology care"; 14547 case CAIC: 14548 return "certified allergy and immunology care"; 14549 case CAMC: 14550 return "certified aerospace medicine care"; 14551 case CANC: 14552 return "certified anesthesiology care"; 14553 case CAPC: 14554 return "certified anatomic pathology care"; 14555 case CBGC: 14556 return "certified clinical biochemical genetics care"; 14557 case CCCC: 14558 return "certified clinical cytogenetics care"; 14559 case CCGC: 14560 return "certified clinical genetics (M.D.) care"; 14561 case CCPC: 14562 return "certified clinical pathology care"; 14563 case CCSC: 14564 return "certified colon and rectal surgery care"; 14565 case CDEC: 14566 return "certified dermatology care"; 14567 case CDRC: 14568 return "certified diagnostic radiology care"; 14569 case CEMC: 14570 return "certified emergency medicine care"; 14571 case CFPC: 14572 return "certified family practice care"; 14573 case CIMC: 14574 return "certified internal medicine care"; 14575 case CMGC: 14576 return "certified clinical molecular genetics care"; 14577 case CNEC: 14578 return "certified neurology care"; 14579 case CNMC: 14580 return "certified nuclear medicine care"; 14581 case CNQC: 14582 return "certified neurology with special qualifications in child neurology care"; 14583 case CNSC: 14584 return "certified neurological surgery care"; 14585 case COGC: 14586 return "certified obstetrics and gynecology care"; 14587 case COMC: 14588 return "certified occupational medicine care"; 14589 case COPC: 14590 return "certified ophthalmology care"; 14591 case COSC: 14592 return "certified orthopaedic surgery care"; 14593 case COTC: 14594 return "certified otolaryngology care"; 14595 case CPEC: 14596 return "certified pediatrics care"; 14597 case CPGC: 14598 return "certified Ph.D. medical genetics care"; 14599 case CPHC: 14600 return "certified public health and general preventive medicine care"; 14601 case CPRC: 14602 return "certified physical medicine and rehabilitation care"; 14603 case CPSC: 14604 return "certified plastic surgery care"; 14605 case CPYC: 14606 return "certified psychiatry care"; 14607 case CROC: 14608 return "certified radiation oncology care"; 14609 case CRPC: 14610 return "certified radiological physics care"; 14611 case CSUC: 14612 return "certified surgery care"; 14613 case CTSC: 14614 return "certified thoracic surgery care"; 14615 case CURC: 14616 return "certified urology care"; 14617 case CVSC: 14618 return "certified vascular surgery care"; 14619 case LGPC: 14620 return "licensed general physician care"; 14621 case _ACTCREDENTIALEDCAREPROVISIONPROGRAMCODE: 14622 return "act credentialed care provision program"; 14623 case AALC: 14624 return "accredited assisted living care"; 14625 case AAMC: 14626 return "accredited ambulatory care"; 14627 case ABHC: 14628 return "accredited behavioral health care"; 14629 case ACAC: 14630 return "accredited critical access hospital care"; 14631 case ACHC: 14632 return "accredited hospital care"; 14633 case AHOC: 14634 return "accredited home care"; 14635 case ALTC: 14636 return "accredited long term care"; 14637 case AOSC: 14638 return "accredited office-based surgery care"; 14639 case CACS: 14640 return "certified acute coronary syndrome care"; 14641 case CAMI: 14642 return "certified acute myocardial infarction care"; 14643 case CAST: 14644 return "certified asthma care"; 14645 case CBAR: 14646 return "certified bariatric surgery care"; 14647 case CCAD: 14648 return "certified coronary artery disease care"; 14649 case CCAR: 14650 return "certified cardiac care"; 14651 case CDEP: 14652 return "certified depression care"; 14653 case CDGD: 14654 return "certified digestive/gastrointestinal disorders care"; 14655 case CDIA: 14656 return "certified diabetes care"; 14657 case CEPI: 14658 return "certified epilepsy care"; 14659 case CFEL: 14660 return "certified frail elderly care"; 14661 case CHFC: 14662 return "certified heart failure care"; 14663 case CHRO: 14664 return "certified high risk obstetrics care"; 14665 case CHYP: 14666 return "certified hyperlipidemia care"; 14667 case CMIH: 14668 return "certified migraine headache care"; 14669 case CMSC: 14670 return "certified multiple sclerosis care"; 14671 case COJR: 14672 return "certified orthopedic joint replacement care"; 14673 case CONC: 14674 return "certified oncology care"; 14675 case COPD: 14676 return "certified chronic obstructive pulmonary disease care"; 14677 case CORT: 14678 return "certified organ transplant care"; 14679 case CPAD: 14680 return "certified parkinsons disease care"; 14681 case CPND: 14682 return "certified pneumonia disease care"; 14683 case CPST: 14684 return "certified primary stroke center care"; 14685 case CSDM: 14686 return "certified stroke disease management care"; 14687 case CSIC: 14688 return "certified sickle cell care"; 14689 case CSLD: 14690 return "certified sleep disorders care"; 14691 case CSPT: 14692 return "certified spine treatment care"; 14693 case CTBU: 14694 return "certified trauma/burn center care"; 14695 case CVDC: 14696 return "certified vascular diseases care"; 14697 case CWMA: 14698 return "certified wound management care"; 14699 case CWOH: 14700 return "certified women's health care"; 14701 case _ACTENCOUNTERCODE: 14702 return "ActEncounterCode"; 14703 case AMB: 14704 return "ambulatory"; 14705 case EMER: 14706 return "emergency"; 14707 case FLD: 14708 return "field"; 14709 case HH: 14710 return "home health"; 14711 case IMP: 14712 return "inpatient encounter"; 14713 case ACUTE: 14714 return "inpatient acute"; 14715 case NONAC: 14716 return "inpatient non-acute"; 14717 case OBSENC: 14718 return "observation encounter"; 14719 case PRENC: 14720 return "pre-admission"; 14721 case SS: 14722 return "short stay"; 14723 case VR: 14724 return "virtual"; 14725 case _ACTMEDICALSERVICECODE: 14726 return "ActMedicalServiceCode"; 14727 case ALC: 14728 return "Alternative Level of Care"; 14729 case CARD: 14730 return "Cardiology"; 14731 case CHR: 14732 return "Chronic"; 14733 case DNTL: 14734 return "Dental"; 14735 case DRGRHB: 14736 return "Drug Rehab"; 14737 case GENRL: 14738 return "General"; 14739 case MED: 14740 return "Medical"; 14741 case OBS: 14742 return "Obstetrics"; 14743 case ONC: 14744 return "Oncology"; 14745 case PALL: 14746 return "Palliative"; 14747 case PED: 14748 return "Pediatrics"; 14749 case PHAR: 14750 return "Pharmaceutical"; 14751 case PHYRHB: 14752 return "Physical Rehab"; 14753 case PSYCH: 14754 return "Psychiatric"; 14755 case SURG: 14756 return "Surgical"; 14757 case _ACTCLAIMATTACHMENTCATEGORYCODE: 14758 return "ActClaimAttachmentCategoryCode"; 14759 case AUTOATTCH: 14760 return "auto attachment"; 14761 case DOCUMENT: 14762 return "document"; 14763 case HEALTHREC: 14764 return "health record"; 14765 case IMG: 14766 return "image attachment"; 14767 case LABRESULTS: 14768 return "lab results"; 14769 case MODEL: 14770 return "model"; 14771 case WIATTCH: 14772 return "work injury report attachment"; 14773 case XRAY: 14774 return "x-ray"; 14775 case _ACTCONSENTTYPE: 14776 return "ActConsentType"; 14777 case ICOL: 14778 return "information collection"; 14779 case IDSCL: 14780 return "information disclosure"; 14781 case INFA: 14782 return "information access"; 14783 case INFAO: 14784 return "access only"; 14785 case INFASO: 14786 return "access and save only"; 14787 case IRDSCL: 14788 return "information redisclosure"; 14789 case RESEARCH: 14790 return "research information access"; 14791 case RSDID: 14792 return "de-identified information access"; 14793 case RSREID: 14794 return "re-identifiable information access"; 14795 case _ACTCONTAINERREGISTRATIONCODE: 14796 return "ActContainerRegistrationCode"; 14797 case ID: 14798 return "Identified"; 14799 case IP: 14800 return "In Position"; 14801 case L: 14802 return "Left Equipment"; 14803 case M: 14804 return "Missing"; 14805 case O: 14806 return "In Process"; 14807 case R: 14808 return "Process Completed"; 14809 case X: 14810 return "Container Unavailable"; 14811 case _ACTCONTROLVARIABLE: 14812 return "ActControlVariable"; 14813 case AUTO: 14814 return "auto-repeat permission"; 14815 case ENDC: 14816 return "endogenous content"; 14817 case REFLEX: 14818 return "reflex permission"; 14819 case _ACTCOVERAGECONFIRMATIONCODE: 14820 return "ActCoverageConfirmationCode"; 14821 case _ACTCOVERAGEAUTHORIZATIONCONFIRMATIONCODE: 14822 return "ActCoverageAuthorizationConfirmationCode"; 14823 case AUTH: 14824 return "Authorized"; 14825 case NAUTH: 14826 return "Not Authorized"; 14827 case _ACTCOVERAGEELIGIBILITYCONFIRMATIONCODE: 14828 return "ActCoverageEligibilityConfirmationCode"; 14829 case ELG: 14830 return "Eligible"; 14831 case NELG: 14832 return "Not Eligible"; 14833 case _ACTCOVERAGELIMITCODE: 14834 return "ActCoverageLimitCode"; 14835 case _ACTCOVERAGEQUANTITYLIMITCODE: 14836 return "ActCoverageQuantityLimitCode"; 14837 case COVPRD: 14838 return "coverage period"; 14839 case LFEMX: 14840 return "life time maximum"; 14841 case NETAMT: 14842 return "Net Amount"; 14843 case PRDMX: 14844 return "period maximum"; 14845 case UNITPRICE: 14846 return "Unit Price"; 14847 case UNITQTY: 14848 return "Unit Quantity"; 14849 case COVMX: 14850 return "coverage maximum"; 14851 case _ACTCOVEREDPARTYLIMITCODE: 14852 return "ActCoveredPartyLimitCode"; 14853 case _ACTCOVERAGETYPECODE: 14854 return "ActCoverageTypeCode"; 14855 case _ACTINSURANCEPOLICYCODE: 14856 return "ActInsurancePolicyCode"; 14857 case EHCPOL: 14858 return "extended healthcare"; 14859 case HSAPOL: 14860 return "health spending account"; 14861 case AUTOPOL: 14862 return "automobile"; 14863 case COL: 14864 return "collision coverage policy"; 14865 case UNINSMOT: 14866 return "uninsured motorist policy"; 14867 case PUBLICPOL: 14868 return "public healthcare"; 14869 case DENTPRG: 14870 return "dental program"; 14871 case DISEASEPRG: 14872 return "public health program"; 14873 case CANPRG: 14874 return "women's cancer detection program"; 14875 case ENDRENAL: 14876 return "end renal program"; 14877 case HIVAIDS: 14878 return "HIV-AIDS program"; 14879 case MANDPOL: 14880 return "mandatory health program"; 14881 case MENTPRG: 14882 return "mental health program"; 14883 case SAFNET: 14884 return "safety net clinic program"; 14885 case SUBPRG: 14886 return "substance use program"; 14887 case SUBSIDIZ: 14888 return "subsidized health program"; 14889 case SUBSIDMC: 14890 return "subsidized managed care program"; 14891 case SUBSUPP: 14892 return "subsidized supplemental health program"; 14893 case WCBPOL: 14894 return "worker's compensation"; 14895 case _ACTINSURANCETYPECODE: 14896 return "ActInsuranceTypeCode"; 14897 case _ACTHEALTHINSURANCETYPECODE: 14898 return "ActHealthInsuranceTypeCode"; 14899 case DENTAL: 14900 return "dental care policy"; 14901 case DISEASE: 14902 return "disease specific policy"; 14903 case DRUGPOL: 14904 return "drug policy"; 14905 case HIP: 14906 return "health insurance plan policy"; 14907 case LTC: 14908 return "long term care policy"; 14909 case MCPOL: 14910 return "managed care policy"; 14911 case POS: 14912 return "point of service policy"; 14913 case HMO: 14914 return "health maintenance organization policy"; 14915 case PPO: 14916 return "preferred provider organization policy"; 14917 case MENTPOL: 14918 return "mental health policy"; 14919 case SUBPOL: 14920 return "substance use policy"; 14921 case VISPOL: 14922 return "vision care policy"; 14923 case DIS: 14924 return "disability insurance policy"; 14925 case EWB: 14926 return "employee welfare benefit plan policy"; 14927 case FLEXP: 14928 return "flexible benefit plan policy"; 14929 case LIFE: 14930 return "life insurance policy"; 14931 case ANNU: 14932 return "annuity policy"; 14933 case TLIFE: 14934 return "term life insurance policy"; 14935 case ULIFE: 14936 return "universal life insurance policy"; 14937 case PNC: 14938 return "property and casualty insurance policy"; 14939 case REI: 14940 return "reinsurance policy"; 14941 case SURPL: 14942 return "surplus line insurance policy"; 14943 case UMBRL: 14944 return "umbrella liability insurance policy"; 14945 case _ACTPROGRAMTYPECODE: 14946 return "ActProgramTypeCode"; 14947 case CHAR: 14948 return "charity program"; 14949 case CRIME: 14950 return "crime victim program"; 14951 case EAP: 14952 return "employee assistance program"; 14953 case GOVEMP: 14954 return "government employee health program"; 14955 case HIRISK: 14956 return "high risk pool program"; 14957 case IND: 14958 return "indigenous peoples health program"; 14959 case MILITARY: 14960 return "military health program"; 14961 case RETIRE: 14962 return "retiree health program"; 14963 case SOCIAL: 14964 return "social service program"; 14965 case VET: 14966 return "veteran health program"; 14967 case _ACTDETECTEDISSUEMANAGEMENTCODE: 14968 return "ActDetectedIssueManagementCode"; 14969 case _ACTADMINISTRATIVEDETECTEDISSUEMANAGEMENTCODE: 14970 return "ActAdministrativeDetectedIssueManagementCode"; 14971 case _AUTHORIZATIONISSUEMANAGEMENTCODE: 14972 return "Authorization Issue Management Code"; 14973 case EMAUTH: 14974 return "emergency authorization override"; 14975 case _21: 14976 return "authorization confirmed"; 14977 case _1: 14978 return "Therapy Appropriate"; 14979 case _19: 14980 return "Consulted Supplier"; 14981 case _2: 14982 return "Assessed Patient"; 14983 case _22: 14984 return "appropriate indication or diagnosis"; 14985 case _23: 14986 return "prior therapy documented"; 14987 case _3: 14988 return "Patient Explanation"; 14989 case _4: 14990 return "Consulted Other Source"; 14991 case _5: 14992 return "Consulted Prescriber"; 14993 case _6: 14994 return "Prescriber Declined Change"; 14995 case _7: 14996 return "Interacting Therapy No Longer Active/Planned"; 14997 case _14: 14998 return "Supply Appropriate"; 14999 case _15: 15000 return "Replacement"; 15001 case _16: 15002 return "Vacation Supply"; 15003 case _17: 15004 return "Weekend Supply"; 15005 case _18: 15006 return "Leave of Absence"; 15007 case _20: 15008 return "additional quantity on separate dispense"; 15009 case _8: 15010 return "Other Action Taken"; 15011 case _10: 15012 return "Provided Patient Education"; 15013 case _11: 15014 return "Added Concurrent Therapy"; 15015 case _12: 15016 return "Temporarily Suspended Concurrent Therapy"; 15017 case _13: 15018 return "Stopped Concurrent Therapy"; 15019 case _9: 15020 return "Instituted Ongoing Monitoring Program"; 15021 case _ACTEXPOSURECODE: 15022 return "ActExposureCode"; 15023 case CHLDCARE: 15024 return "Day care - Child care Interaction"; 15025 case CONVEYNC: 15026 return "Common Conveyance Interaction"; 15027 case HLTHCARE: 15028 return "Health Care Interaction - Not Patient Care"; 15029 case HOMECARE: 15030 return "Care Giver Interaction"; 15031 case HOSPPTNT: 15032 return "Hospital Patient Interaction"; 15033 case HOSPVSTR: 15034 return "Hospital Visitor Interaction"; 15035 case HOUSEHLD: 15036 return "Household Interaction"; 15037 case INMATE: 15038 return "Inmate Interaction"; 15039 case INTIMATE: 15040 return "Intimate Interaction"; 15041 case LTRMCARE: 15042 return "Long Term Care Facility Interaction"; 15043 case PLACE: 15044 return "Common Space Interaction"; 15045 case PTNTCARE: 15046 return "Health Care Interaction - Patient Care"; 15047 case SCHOOL2: 15048 return "School Interaction"; 15049 case SOCIAL2: 15050 return "Social/Extended Family Interaction"; 15051 case SUBSTNCE: 15052 return "Common Substance Interaction"; 15053 case TRAVINT: 15054 return "Common Travel Interaction"; 15055 case WORK2: 15056 return "Work Interaction"; 15057 case _ACTFINANCIALTRANSACTIONCODE: 15058 return "ActFinancialTransactionCode"; 15059 case CHRG: 15060 return "Standard Charge"; 15061 case REV: 15062 return "Standard Charge Reversal"; 15063 case _ACTINCIDENTCODE: 15064 return "ActIncidentCode"; 15065 case MVA: 15066 return "Motor vehicle accident"; 15067 case SCHOOL: 15068 return "School Accident"; 15069 case SPT: 15070 return "Sporting Accident"; 15071 case WPA: 15072 return "Workplace accident"; 15073 case _ACTINFORMATIONACCESSCODE: 15074 return "ActInformationAccessCode"; 15075 case ACADR: 15076 return "adverse drug reaction access"; 15077 case ACALL: 15078 return "all access"; 15079 case ACALLG: 15080 return "allergy access"; 15081 case ACCONS: 15082 return "informational consent access"; 15083 case ACDEMO: 15084 return "demographics access"; 15085 case ACDI: 15086 return "diagnostic imaging access"; 15087 case ACIMMUN: 15088 return "immunization access"; 15089 case ACLAB: 15090 return "lab test result access"; 15091 case ACMED: 15092 return "medication access"; 15093 case ACMEDC: 15094 return "medical condition access"; 15095 case ACMEN: 15096 return "mental health access"; 15097 case ACOBS: 15098 return "common observations access"; 15099 case ACPOLPRG: 15100 return "policy or program information access"; 15101 case ACPROV: 15102 return "provider information access"; 15103 case ACPSERV: 15104 return "professional service access"; 15105 case ACSUBSTAB: 15106 return "substance abuse access"; 15107 case _ACTINFORMATIONACCESSCONTEXTCODE: 15108 return "ActInformationAccessContextCode"; 15109 case INFAUT: 15110 return "authorized information transfer"; 15111 case INFCON: 15112 return "after explicit consent"; 15113 case INFCRT: 15114 return "only on court order"; 15115 case INFDNG: 15116 return "only if danger to others"; 15117 case INFEMER: 15118 return "only in an emergency"; 15119 case INFPWR: 15120 return "only if public welfare risk"; 15121 case INFREG: 15122 return "regulatory information transfer"; 15123 case _ACTINFORMATIONCATEGORYCODE: 15124 return "ActInformationCategoryCode"; 15125 case ALLCAT: 15126 return "all categories"; 15127 case ALLGCAT: 15128 return "allergy category"; 15129 case ARCAT: 15130 return "adverse drug reaction category"; 15131 case COBSCAT: 15132 return "common observation category"; 15133 case DEMOCAT: 15134 return "demographics category"; 15135 case DICAT: 15136 return "diagnostic image category"; 15137 case IMMUCAT: 15138 return "immunization category"; 15139 case LABCAT: 15140 return "lab test category"; 15141 case MEDCCAT: 15142 return "medical condition category"; 15143 case MENCAT: 15144 return "mental health category"; 15145 case PSVCCAT: 15146 return "professional service category"; 15147 case RXCAT: 15148 return "medication category"; 15149 case _ACTINVOICEELEMENTCODE: 15150 return "ActInvoiceElementCode"; 15151 case _ACTINVOICEADJUDICATIONPAYMENTCODE: 15152 return "ActInvoiceAdjudicationPaymentCode"; 15153 case _ACTINVOICEADJUDICATIONPAYMENTGROUPCODE: 15154 return "ActInvoiceAdjudicationPaymentGroupCode"; 15155 case ALEC: 15156 return "alternate electronic"; 15157 case BONUS: 15158 return "bonus"; 15159 case CFWD: 15160 return "carry forward adjusment"; 15161 case EDU: 15162 return "education fees"; 15163 case EPYMT: 15164 return "early payment fee"; 15165 case GARN: 15166 return "garnishee"; 15167 case INVOICE: 15168 return "submitted invoice"; 15169 case PINV: 15170 return "paper invoice"; 15171 case PPRD: 15172 return "prior period adjustment"; 15173 case PROA: 15174 return "professional association deduction"; 15175 case RECOV: 15176 return "recovery"; 15177 case RETRO: 15178 return "retro adjustment"; 15179 case TRAN: 15180 return "transaction fee"; 15181 case _ACTINVOICEADJUDICATIONPAYMENTSUMMARYCODE: 15182 return "ActInvoiceAdjudicationPaymentSummaryCode"; 15183 case INVTYPE: 15184 return "invoice type"; 15185 case PAYEE: 15186 return "payee"; 15187 case PAYOR: 15188 return "payor"; 15189 case SENDAPP: 15190 return "sending application"; 15191 case _ACTINVOICEDETAILCODE: 15192 return "ActInvoiceDetailCode"; 15193 case _ACTINVOICEDETAILCLINICALPRODUCTCODE: 15194 return "ActInvoiceDetailClinicalProductCode"; 15195 case UNSPSC: 15196 return "United Nations Standard Products and Services Classification"; 15197 case _ACTINVOICEDETAILDRUGPRODUCTCODE: 15198 return "ActInvoiceDetailDrugProductCode"; 15199 case GTIN: 15200 return "Global Trade Item Number"; 15201 case UPC: 15202 return "Universal Product Code"; 15203 case _ACTINVOICEDETAILGENERICCODE: 15204 return "ActInvoiceDetailGenericCode"; 15205 case _ACTINVOICEDETAILGENERICADJUDICATORCODE: 15206 return "ActInvoiceDetailGenericAdjudicatorCode"; 15207 case COIN: 15208 return "coinsurance"; 15209 case COPAYMENT: 15210 return "patient co-pay"; 15211 case DEDUCTIBLE: 15212 return "deductible"; 15213 case PAY: 15214 return "payment"; 15215 case SPEND: 15216 return "spend down"; 15217 case COINS: 15218 return "co-insurance"; 15219 case _ACTINVOICEDETAILGENERICMODIFIERCODE: 15220 return "ActInvoiceDetailGenericModifierCode"; 15221 case AFTHRS: 15222 return "non-normal hours"; 15223 case ISOL: 15224 return "isolation allowance"; 15225 case OOO: 15226 return "out of office"; 15227 case _ACTINVOICEDETAILGENERICPROVIDERCODE: 15228 return "ActInvoiceDetailGenericProviderCode"; 15229 case CANCAPT: 15230 return "cancelled appointment"; 15231 case DSC: 15232 return "discount"; 15233 case ESA: 15234 return "extraordinary service assessment"; 15235 case FFSTOP: 15236 return "fee for service top off"; 15237 case FNLFEE: 15238 return "final fee"; 15239 case FRSTFEE: 15240 return "first fee"; 15241 case MARKUP: 15242 return "markup or up-charge"; 15243 case MISSAPT: 15244 return "missed appointment"; 15245 case PERFEE: 15246 return "periodic fee"; 15247 case PERMBNS: 15248 return "performance bonus"; 15249 case RESTOCK: 15250 return "restocking fee"; 15251 case TRAVEL: 15252 return "travel"; 15253 case URGENT: 15254 return "urgent"; 15255 case _ACTINVOICEDETAILTAXCODE: 15256 return "ActInvoiceDetailTaxCode"; 15257 case FST: 15258 return "federal sales tax"; 15259 case HST: 15260 return "harmonized sales Tax"; 15261 case PST: 15262 return "provincial/state sales tax"; 15263 case _ACTINVOICEDETAILPREFERREDACCOMMODATIONCODE: 15264 return "ActInvoiceDetailPreferredAccommodationCode"; 15265 case _ACTENCOUNTERACCOMMODATIONCODE: 15266 return "ActEncounterAccommodationCode"; 15267 case _HL7ACCOMMODATIONCODE: 15268 return "HL7AccommodationCode"; 15269 case I: 15270 return "Isolation"; 15271 case P: 15272 return "Private"; 15273 case S: 15274 return "Suite"; 15275 case SP: 15276 return "Semi-private"; 15277 case W: 15278 return "Ward"; 15279 case _ACTINVOICEDETAILCLINICALSERVICECODE: 15280 return "ActInvoiceDetailClinicalServiceCode"; 15281 case _ACTINVOICEGROUPCODE: 15282 return "ActInvoiceGroupCode"; 15283 case _ACTINVOICEINTERGROUPCODE: 15284 return "ActInvoiceInterGroupCode"; 15285 case CPNDDRGING: 15286 return "compound drug invoice group"; 15287 case CPNDINDING: 15288 return "compound ingredient invoice group"; 15289 case CPNDSUPING: 15290 return "compound supply invoice group"; 15291 case DRUGING: 15292 return "drug invoice group"; 15293 case FRAMEING: 15294 return "frame invoice group"; 15295 case LENSING: 15296 return "lens invoice group"; 15297 case PRDING: 15298 return "product invoice group"; 15299 case _ACTINVOICEROOTGROUPCODE: 15300 return "ActInvoiceRootGroupCode"; 15301 case CPINV: 15302 return "clinical product invoice"; 15303 case CSINV: 15304 return "clinical service invoice"; 15305 case CSPINV: 15306 return "clinical service and product"; 15307 case FININV: 15308 return "financial invoice"; 15309 case OHSINV: 15310 return "oral health service"; 15311 case PAINV: 15312 return "preferred accommodation invoice"; 15313 case RXCINV: 15314 return "Rx compound invoice"; 15315 case RXDINV: 15316 return "Rx dispense invoice"; 15317 case SBFINV: 15318 return "sessional or block fee invoice"; 15319 case VRXINV: 15320 return "vision dispense invoice"; 15321 case _ACTINVOICEELEMENTSUMMARYCODE: 15322 return "ActInvoiceElementSummaryCode"; 15323 case _INVOICEELEMENTADJUDICATED: 15324 return "InvoiceElementAdjudicated"; 15325 case ADNFPPELAT: 15326 return "adjud. nullified prior-period electronic amount"; 15327 case ADNFPPELCT: 15328 return "adjud. nullified prior-period electronic count"; 15329 case ADNFPPMNAT: 15330 return "adjud. nullified prior-period manual amount"; 15331 case ADNFPPMNCT: 15332 return "adjud. nullified prior-period manual count"; 15333 case ADNFSPELAT: 15334 return "adjud. nullified same-period electronic amount"; 15335 case ADNFSPELCT: 15336 return "adjud. nullified same-period electronic count"; 15337 case ADNFSPMNAT: 15338 return "adjud. nullified same-period manual amount"; 15339 case ADNFSPMNCT: 15340 return "adjud. nullified same-period manual count"; 15341 case ADNPPPELAT: 15342 return "adjud. non-payee payable prior-period electronic amount"; 15343 case ADNPPPELCT: 15344 return "adjud. non-payee payable prior-period electronic count"; 15345 case ADNPPPMNAT: 15346 return "adjud. non-payee payable prior-period manual amount"; 15347 case ADNPPPMNCT: 15348 return "adjud. non-payee payable prior-period manual count"; 15349 case ADNPSPELAT: 15350 return "adjud. non-payee payable same-period electronic amount"; 15351 case ADNPSPELCT: 15352 return "adjud. non-payee payable same-period electronic count"; 15353 case ADNPSPMNAT: 15354 return "adjud. non-payee payable same-period manual amount"; 15355 case ADNPSPMNCT: 15356 return "adjud. non-payee payable same-period manual count"; 15357 case ADPPPPELAT: 15358 return "adjud. payee payable prior-period electronic amount"; 15359 case ADPPPPELCT: 15360 return "adjud. payee payable prior-period electronic count"; 15361 case ADPPPPMNAT: 15362 return "adjud. payee payable prior-period manual amout"; 15363 case ADPPPPMNCT: 15364 return "adjud. payee payable prior-period manual count"; 15365 case ADPPSPELAT: 15366 return "adjud. payee payable same-period electronic amount"; 15367 case ADPPSPELCT: 15368 return "adjud. payee payable same-period electronic count"; 15369 case ADPPSPMNAT: 15370 return "adjud. payee payable same-period manual amount"; 15371 case ADPPSPMNCT: 15372 return "adjud. payee payable same-period manual count"; 15373 case ADRFPPELAT: 15374 return "adjud. refused prior-period electronic amount"; 15375 case ADRFPPELCT: 15376 return "adjud. refused prior-period electronic count"; 15377 case ADRFPPMNAT: 15378 return "adjud. refused prior-period manual amount"; 15379 case ADRFPPMNCT: 15380 return "adjud. refused prior-period manual count"; 15381 case ADRFSPELAT: 15382 return "adjud. refused same-period electronic amount"; 15383 case ADRFSPELCT: 15384 return "adjud. refused same-period electronic count"; 15385 case ADRFSPMNAT: 15386 return "adjud. refused same-period manual amount"; 15387 case ADRFSPMNCT: 15388 return "adjud. refused same-period manual count"; 15389 case _INVOICEELEMENTPAID: 15390 return "InvoiceElementPaid"; 15391 case PDNFPPELAT: 15392 return "paid nullified prior-period electronic amount"; 15393 case PDNFPPELCT: 15394 return "paid nullified prior-period electronic count"; 15395 case PDNFPPMNAT: 15396 return "paid nullified prior-period manual amount"; 15397 case PDNFPPMNCT: 15398 return "paid nullified prior-period manual count"; 15399 case PDNFSPELAT: 15400 return "paid nullified same-period electronic amount"; 15401 case PDNFSPELCT: 15402 return "paid nullified same-period electronic count"; 15403 case PDNFSPMNAT: 15404 return "paid nullified same-period manual amount"; 15405 case PDNFSPMNCT: 15406 return "paid nullified same-period manual count"; 15407 case PDNPPPELAT: 15408 return "paid non-payee payable prior-period electronic amount"; 15409 case PDNPPPELCT: 15410 return "paid non-payee payable prior-period electronic count"; 15411 case PDNPPPMNAT: 15412 return "paid non-payee payable prior-period manual amount"; 15413 case PDNPPPMNCT: 15414 return "paid non-payee payable prior-period manual count"; 15415 case PDNPSPELAT: 15416 return "paid non-payee payable same-period electronic amount"; 15417 case PDNPSPELCT: 15418 return "paid non-payee payable same-period electronic count"; 15419 case PDNPSPMNAT: 15420 return "paid non-payee payable same-period manual amount"; 15421 case PDNPSPMNCT: 15422 return "paid non-payee payable same-period manual count"; 15423 case PDPPPPELAT: 15424 return "paid payee payable prior-period electronic amount"; 15425 case PDPPPPELCT: 15426 return "paid payee payable prior-period electronic count"; 15427 case PDPPPPMNAT: 15428 return "paid payee payable prior-period manual amount"; 15429 case PDPPPPMNCT: 15430 return "paid payee payable prior-period manual count"; 15431 case PDPPSPELAT: 15432 return "paid payee payable same-period electronic amount"; 15433 case PDPPSPELCT: 15434 return "paid payee payable same-period electronic count"; 15435 case PDPPSPMNAT: 15436 return "paid payee payable same-period manual amount"; 15437 case PDPPSPMNCT: 15438 return "paid payee payable same-period manual count"; 15439 case _INVOICEELEMENTSUBMITTED: 15440 return "InvoiceElementSubmitted"; 15441 case SBBLELAT: 15442 return "submitted billed electronic amount"; 15443 case SBBLELCT: 15444 return "submitted billed electronic count"; 15445 case SBNFELAT: 15446 return "submitted nullified electronic amount"; 15447 case SBNFELCT: 15448 return "submitted cancelled electronic count"; 15449 case SBPDELAT: 15450 return "submitted pending electronic amount"; 15451 case SBPDELCT: 15452 return "submitted pending electronic count"; 15453 case _ACTINVOICEOVERRIDECODE: 15454 return "ActInvoiceOverrideCode"; 15455 case COVGE: 15456 return "coverage problem"; 15457 case EFORM: 15458 return "electronic form to follow"; 15459 case FAX: 15460 return "fax to follow"; 15461 case GFTH: 15462 return "good faith indicator"; 15463 case LATE: 15464 return "late invoice"; 15465 case MANUAL: 15466 return "manual review"; 15467 case OOJ: 15468 return "out of jurisdiction"; 15469 case ORTHO: 15470 return "orthodontic service"; 15471 case PAPER: 15472 return "paper documentation to follow"; 15473 case PIE: 15474 return "public insurance exhausted"; 15475 case PYRDELAY: 15476 return "delayed by a previous payor"; 15477 case REFNR: 15478 return "referral not required"; 15479 case REPSERV: 15480 return "repeated service"; 15481 case UNRELAT: 15482 return "unrelated service"; 15483 case VERBAUTH: 15484 return "verbal authorization"; 15485 case _ACTLISTCODE: 15486 return "ActListCode"; 15487 case _ACTOBSERVATIONLIST: 15488 return "ActObservationList"; 15489 case CARELIST: 15490 return "care plan"; 15491 case CONDLIST: 15492 return "condition list"; 15493 case INTOLIST: 15494 return "intolerance list"; 15495 case PROBLIST: 15496 return "problem list"; 15497 case RISKLIST: 15498 return "risk factors"; 15499 case GOALLIST: 15500 return "goal list"; 15501 case _ACTTHERAPYDURATIONWORKINGLISTCODE: 15502 return "ActTherapyDurationWorkingListCode"; 15503 case _ACTMEDICATIONTHERAPYDURATIONWORKINGLISTCODE: 15504 return "act medication therapy duration working list"; 15505 case ACU: 15506 return "short term/acute"; 15507 case CHRON: 15508 return "continuous/chronic"; 15509 case ONET: 15510 return "one time"; 15511 case PRN: 15512 return "as needed"; 15513 case MEDLIST: 15514 return "medication list"; 15515 case CURMEDLIST: 15516 return "current medication list"; 15517 case DISCMEDLIST: 15518 return "discharge medication list"; 15519 case HISTMEDLIST: 15520 return "medication history"; 15521 case _ACTMONITORINGPROTOCOLCODE: 15522 return "ActMonitoringProtocolCode"; 15523 case CTLSUB: 15524 return "Controlled Substance"; 15525 case INV: 15526 return "investigational"; 15527 case LU: 15528 return "limited use"; 15529 case OTC: 15530 return "non prescription medicine"; 15531 case RX: 15532 return "prescription only medicine"; 15533 case SA: 15534 return "special authorization"; 15535 case SAC: 15536 return "special access"; 15537 case _ACTNONOBSERVATIONINDICATIONCODE: 15538 return "ActNonObservationIndicationCode"; 15539 case IND01: 15540 return "imaging study requiring contrast"; 15541 case IND02: 15542 return "colonoscopy prep"; 15543 case IND03: 15544 return "prophylaxis"; 15545 case IND04: 15546 return "surgical prophylaxis"; 15547 case IND05: 15548 return "pregnancy prophylaxis"; 15549 case _ACTOBSERVATIONVERIFICATIONTYPE: 15550 return "act observation verification"; 15551 case VFPAPER: 15552 return "verify paper"; 15553 case _ACTPAYMENTCODE: 15554 return "ActPaymentCode"; 15555 case ACH: 15556 return "Automated Clearing House"; 15557 case CHK: 15558 return "Cheque"; 15559 case DDP: 15560 return "Direct Deposit"; 15561 case NON: 15562 return "Non-Payment Data"; 15563 case _ACTPHARMACYSUPPLYTYPE: 15564 return "ActPharmacySupplyType"; 15565 case DF: 15566 return "Daily Fill"; 15567 case EM: 15568 return "Emergency Supply"; 15569 case SO: 15570 return "Script Owing"; 15571 case FF: 15572 return "First Fill"; 15573 case FFC: 15574 return "First Fill - Complete"; 15575 case FFP: 15576 return "First Fill - Part Fill"; 15577 case FFSS: 15578 return "first fill, partial strength"; 15579 case TF: 15580 return "Trial Fill"; 15581 case FS: 15582 return "Floor stock"; 15583 case MS: 15584 return "Manufacturer Sample"; 15585 case RF: 15586 return "Refill"; 15587 case UD: 15588 return "Unit Dose"; 15589 case RFC: 15590 return "Refill - Complete"; 15591 case RFCS: 15592 return "refill complete partial strength"; 15593 case RFF: 15594 return "Refill (First fill this facility)"; 15595 case RFFS: 15596 return "refill partial strength (first fill this facility)"; 15597 case RFP: 15598 return "Refill - Part Fill"; 15599 case RFPS: 15600 return "refill part fill partial strength"; 15601 case RFS: 15602 return "refill partial strength"; 15603 case TB: 15604 return "Trial Balance"; 15605 case TBS: 15606 return "trial balance partial strength"; 15607 case UDE: 15608 return "unit dose equivalent"; 15609 case _ACTPOLICYTYPE: 15610 return "ActPolicyType"; 15611 case _ACTPRIVACYPOLICY: 15612 return "ActPrivacyPolicy"; 15613 case _ACTCONSENTDIRECTIVE: 15614 return "ActConsentDirective"; 15615 case EMRGONLY: 15616 return "emergency only"; 15617 case GRANTORCHOICE: 15618 return "grantor choice"; 15619 case IMPLIED: 15620 return "implied consent"; 15621 case IMPLIEDD: 15622 return "implied consent with opportunity to dissent"; 15623 case NOCONSENT: 15624 return "no consent"; 15625 case NOPP: 15626 return "notice of privacy practices"; 15627 case OPTIN: 15628 return "opt-in"; 15629 case OPTINR: 15630 return "opt-in with restrictions"; 15631 case OPTOUT: 15632 return "op-out"; 15633 case OPTOUTE: 15634 return "opt-out with exceptions"; 15635 case _ACTPRIVACYLAW: 15636 return "ActPrivacyLaw"; 15637 case _ACTUSPRIVACYLAW: 15638 return "_ActUSPrivacyLaw"; 15639 case _42CFRPART2: 15640 return "42 CFR Part2"; 15641 case COMMONRULE: 15642 return "Common Rule"; 15643 case HIPAANOPP: 15644 return "HIPAA notice of privacy practices"; 15645 case HIPAAPSYNOTES: 15646 return "HIPAA psychotherapy notes"; 15647 case HIPAASELFPAY: 15648 return "HIPAA self-pay"; 15649 case TITLE38SECTION7332: 15650 return "Title 38 Section 7332"; 15651 case _INFORMATIONSENSITIVITYPOLICY: 15652 return "InformationSensitivityPolicy"; 15653 case _ACTINFORMATIONSENSITIVITYPOLICY: 15654 return "ActInformationSensitivityPolicy"; 15655 case ETH: 15656 return "substance abuse information sensitivity"; 15657 case GDIS: 15658 return "genetic disease information sensitivity"; 15659 case HIV: 15660 return "HIV/AIDS information sensitivity"; 15661 case MST: 15662 return "military sexual trauma information sensitivity"; 15663 case SCA: 15664 return "sickle cell anemia information sensitivity"; 15665 case SDV: 15666 return "sexual assault, abuse, or domestic violence information sensitivity"; 15667 case SEX: 15668 return "sexuality and reproductive health information sensitivity"; 15669 case SPI: 15670 return "specially protected information sensitivity"; 15671 case BH: 15672 return "behavioral health information sensitivity"; 15673 case COGN: 15674 return "cognitive disability information sensitivity"; 15675 case DVD: 15676 return "developmental disability information sensitivity"; 15677 case EMOTDIS: 15678 return "emotional disturbance information sensitivity"; 15679 case MH: 15680 return "mental health information sensitivity"; 15681 case PSY: 15682 return "psychiatry disorder information sensitivity"; 15683 case PSYTHPN: 15684 return "psychotherapy note information sensitivity"; 15685 case SUD: 15686 return "substance use disorder information sensitivity"; 15687 case ETHUD: 15688 return "alcohol use disorder information sensitivity"; 15689 case OPIOIDUD: 15690 return "opioid use disorder information sensitivity"; 15691 case STD: 15692 return "sexually transmitted disease information sensitivity"; 15693 case TBOO: 15694 return "taboo"; 15695 case VIO: 15696 return "violence information sensitivity"; 15697 case SICKLE: 15698 return "sickle cell"; 15699 case _ENTITYSENSITIVITYPOLICYTYPE: 15700 return "EntityInformationSensitivityPolicy"; 15701 case DEMO: 15702 return "all demographic information sensitivity"; 15703 case DOB: 15704 return "date of birth information sensitivity"; 15705 case GENDER: 15706 return "gender and sexual orientation information sensitivity"; 15707 case LIVARG: 15708 return "living arrangement information sensitivity"; 15709 case MARST: 15710 return "marital status information sensitivity"; 15711 case RACE: 15712 return "race information sensitivity"; 15713 case REL: 15714 return "religion information sensitivity"; 15715 case _ROLEINFORMATIONSENSITIVITYPOLICY: 15716 return "RoleInformationSensitivityPolicy"; 15717 case B: 15718 return "business information sensitivity"; 15719 case EMPL: 15720 return "employer information sensitivity"; 15721 case LOCIS: 15722 return "location information sensitivity"; 15723 case SSP: 15724 return "sensitive service provider information sensitivity"; 15725 case ADOL: 15726 return "adolescent information sensitivity"; 15727 case CEL: 15728 return "celebrity information sensitivity"; 15729 case DIA: 15730 return "diagnosis information sensitivity"; 15731 case DRGIS: 15732 return "drug information sensitivity"; 15733 case EMP: 15734 return "employee information sensitivity"; 15735 case PDS: 15736 return "patient default information sensitivity"; 15737 case PHY: 15738 return "physician requested information sensitivity"; 15739 case PRS: 15740 return "patient requested information sensitivity"; 15741 case COMPT: 15742 return "compartment"; 15743 case ACOCOMPT: 15744 return "accountable care organization compartment"; 15745 case CTCOMPT: 15746 return "care team compartment"; 15747 case FMCOMPT: 15748 return "financial management compartment"; 15749 case HRCOMPT: 15750 return "human resource compartment"; 15751 case LRCOMPT: 15752 return "legitimate relationship compartment"; 15753 case PACOMPT: 15754 return "patient administration compartment"; 15755 case RESCOMPT: 15756 return "research project compartment"; 15757 case RMGTCOMPT: 15758 return "records management compartment"; 15759 case ACTTRUSTPOLICYTYPE: 15760 return "trust policy"; 15761 case TRSTACCRD: 15762 return "trust accreditation"; 15763 case TRSTAGRE: 15764 return "trust agreement"; 15765 case TRSTASSUR: 15766 return "trust assurance"; 15767 case TRSTCERT: 15768 return "trust certificate"; 15769 case TRSTFWK: 15770 return "trust framework"; 15771 case TRSTMEC: 15772 return "trust mechanism"; 15773 case COVPOL: 15774 return "benefit policy"; 15775 case SECURITYPOLICY: 15776 return "security policy"; 15777 case AUTHPOL: 15778 return "authorization policy"; 15779 case ACCESSCONSCHEME: 15780 return "access control scheme"; 15781 case DELEPOL: 15782 return "delegation policy"; 15783 case OBLIGATIONPOLICY: 15784 return "obligation policy"; 15785 case ANONY: 15786 return "anonymize"; 15787 case AOD: 15788 return "accounting of disclosure"; 15789 case AUDIT: 15790 return "audit"; 15791 case AUDTR: 15792 return "audit trail"; 15793 case CPLYCC: 15794 return "comply with confidentiality code"; 15795 case CPLYCD: 15796 return "comply with consent directive"; 15797 case CPLYJPP: 15798 return "comply with jurisdictional privacy policy"; 15799 case CPLYOPP: 15800 return "comply with organizational privacy policy"; 15801 case CPLYOSP: 15802 return "comply with organizational security policy"; 15803 case CPLYPOL: 15804 return "comply with policy"; 15805 case DECLASSIFYLABEL: 15806 return "declassify security label"; 15807 case DEID: 15808 return "deidentify"; 15809 case DELAU: 15810 return "delete after use"; 15811 case DOWNGRDLABEL: 15812 return "downgrade security label"; 15813 case DRIVLABEL: 15814 return "derive security label"; 15815 case ENCRYPT: 15816 return "encrypt"; 15817 case ENCRYPTR: 15818 return "encrypt at rest"; 15819 case ENCRYPTT: 15820 return "encrypt in transit"; 15821 case ENCRYPTU: 15822 return "encrypt in use"; 15823 case HUAPRV: 15824 return "human approval"; 15825 case LABEL: 15826 return "assign security label"; 15827 case MASK: 15828 return "mask"; 15829 case MINEC: 15830 return "minimum necessary"; 15831 case PERSISTLABEL: 15832 return "persist security label"; 15833 case PRIVMARK: 15834 return "privacy mark"; 15835 case PSEUD: 15836 return "pseudonymize"; 15837 case REDACT: 15838 return "redact"; 15839 case UPGRDLABEL: 15840 return "upgrade security label"; 15841 case REFRAINPOLICY: 15842 return "refrain policy"; 15843 case NOAUTH: 15844 return "no disclosure without subject authorization"; 15845 case NOCOLLECT: 15846 return "no collection"; 15847 case NODSCLCD: 15848 return "no disclosure without consent directive"; 15849 case NODSCLCDS: 15850 return "no disclosure without information subject's consent directive"; 15851 case NOINTEGRATE: 15852 return "no integration"; 15853 case NOLIST: 15854 return "no unlisted entity disclosure"; 15855 case NOMOU: 15856 return "no disclosure without MOU"; 15857 case NOORGPOL: 15858 return "no disclosure without organizational authorization"; 15859 case NOPAT: 15860 return "no disclosure to patient, family or caregivers without attending provider's authorization"; 15861 case NOPERSISTP: 15862 return "no collection beyond purpose of use"; 15863 case NORDSCLCD: 15864 return "no redisclosure without consent directive"; 15865 case NORDSCLCDS: 15866 return "no redisclosure without information subject's consent directive"; 15867 case NORDSCLW: 15868 return "no disclosure without jurisdictional authorization"; 15869 case NORELINK: 15870 return "no relinking"; 15871 case NOREUSE: 15872 return "no reuse beyond purpose of use"; 15873 case NOVIP: 15874 return "no unauthorized VIP disclosure"; 15875 case ORCON: 15876 return "no disclosure without originator authorization"; 15877 case _ACTPRODUCTACQUISITIONCODE: 15878 return "ActProductAcquisitionCode"; 15879 case LOAN: 15880 return "Loan"; 15881 case RENT: 15882 return "Rent"; 15883 case TRANSFER: 15884 return "Transfer"; 15885 case SALE: 15886 return "Sale"; 15887 case _ACTSPECIMENTRANSPORTCODE: 15888 return "ActSpecimenTransportCode"; 15889 case SREC: 15890 return "specimen received"; 15891 case SSTOR: 15892 return "specimen in storage"; 15893 case STRAN: 15894 return "specimen in transit"; 15895 case _ACTSPECIMENTREATMENTCODE: 15896 return "ActSpecimenTreatmentCode"; 15897 case ACID: 15898 return "Acidification"; 15899 case ALK: 15900 return "Alkalization"; 15901 case DEFB: 15902 return "Defibrination"; 15903 case FILT: 15904 return "Filtration"; 15905 case LDLP: 15906 return "LDL Precipitation"; 15907 case NEUT: 15908 return "Neutralization"; 15909 case RECA: 15910 return "Recalcification"; 15911 case UFIL: 15912 return "Ultrafiltration"; 15913 case _ACTSUBSTANCEADMINISTRATIONCODE: 15914 return "ActSubstanceAdministrationCode"; 15915 case DRUG: 15916 return "Drug therapy"; 15917 case FD: 15918 return "food"; 15919 case IMMUNIZ: 15920 return "Immunization"; 15921 case BOOSTER: 15922 return "Booster Immunization"; 15923 case INITIMMUNIZ: 15924 return "Initial Immunization"; 15925 case _ACTTASKCODE: 15926 return "ActTaskCode"; 15927 case OE: 15928 return "order entry task"; 15929 case LABOE: 15930 return "laboratory test order entry task"; 15931 case MEDOE: 15932 return "medication order entry task"; 15933 case PATDOC: 15934 return "patient documentation task"; 15935 case ALLERLREV: 15936 return "allergy list review"; 15937 case CLINNOTEE: 15938 return "clinical note entry task"; 15939 case DIAGLISTE: 15940 return "diagnosis list entry task"; 15941 case DISCHINSTE: 15942 return "discharge instruction entry"; 15943 case DISCHSUME: 15944 return "discharge summary entry task"; 15945 case PATEDUE: 15946 return "patient education entry"; 15947 case PATREPE: 15948 return "pathology report entry task"; 15949 case PROBLISTE: 15950 return "problem list entry task"; 15951 case RADREPE: 15952 return "radiology report entry task"; 15953 case IMMLREV: 15954 return "immunization list review"; 15955 case REMLREV: 15956 return "reminder list review"; 15957 case WELLREMLREV: 15958 return "wellness reminder list review"; 15959 case PATINFO: 15960 return "patient information review task"; 15961 case ALLERLE: 15962 return "allergy list entry"; 15963 case CDSREV: 15964 return "clinical decision support intervention review"; 15965 case CLINNOTEREV: 15966 return "clinical note review task"; 15967 case DISCHSUMREV: 15968 return "discharge summary review task"; 15969 case DIAGLISTREV: 15970 return "diagnosis list review task"; 15971 case IMMLE: 15972 return "immunization list entry"; 15973 case LABRREV: 15974 return "laboratory results review task"; 15975 case MICRORREV: 15976 return "microbiology results review task"; 15977 case MICROORGRREV: 15978 return "microbiology organisms results review task"; 15979 case MICROSENSRREV: 15980 return "microbiology sensitivity test results review task"; 15981 case MLREV: 15982 return "medication list review task"; 15983 case MARWLREV: 15984 return "medication administration record work list review task"; 15985 case OREV: 15986 return "orders review task"; 15987 case PATREPREV: 15988 return "pathology report review task"; 15989 case PROBLISTREV: 15990 return "problem list review task"; 15991 case RADREPREV: 15992 return "radiology report review task"; 15993 case REMLE: 15994 return "reminder list entry"; 15995 case WELLREMLE: 15996 return "wellness reminder list entry"; 15997 case RISKASSESS: 15998 return "risk assessment instrument task"; 15999 case FALLRISK: 16000 return "falls risk assessment instrument task"; 16001 case _ACTTRANSPORTATIONMODECODE: 16002 return "ActTransportationModeCode"; 16003 case _ACTPATIENTTRANSPORTATIONMODECODE: 16004 return "ActPatientTransportationModeCode"; 16005 case AFOOT: 16006 return "pedestrian transport"; 16007 case AMBT: 16008 return "ambulance transport"; 16009 case AMBAIR: 16010 return "fixed-wing ambulance transport"; 16011 case AMBGRND: 16012 return "ground ambulance transport"; 16013 case AMBHELO: 16014 return "helicopter ambulance transport"; 16015 case LAWENF: 16016 return "law enforcement transport"; 16017 case PRVTRN: 16018 return "private transport"; 16019 case PUBTRN: 16020 return "public transport"; 16021 case _OBSERVATIONTYPE: 16022 return "ObservationType"; 16023 case _ACTSPECOBSCODE: 16024 return "ActSpecObsCode"; 16025 case ARTBLD: 16026 return "ActSpecObsArtBldCode"; 16027 case DILUTION: 16028 return "ActSpecObsDilutionCode"; 16029 case AUTOHIGH: 16030 return "Auto-High Dilution"; 16031 case AUTOLOW: 16032 return "Auto-Low Dilution"; 16033 case PRE: 16034 return "Pre-Dilution"; 16035 case RERUN: 16036 return "Rerun Dilution"; 16037 case EVNFCTS: 16038 return "ActSpecObsEvntfctsCode"; 16039 case INTFR: 16040 return "ActSpecObsInterferenceCode"; 16041 case FIBRIN: 16042 return "Fibrin"; 16043 case HEMOLYSIS: 16044 return "Hemolysis"; 16045 case ICTERUS: 16046 return "Icterus"; 16047 case LIPEMIA: 16048 return "Lipemia"; 16049 case VOLUME: 16050 return "ActSpecObsVolumeCode"; 16051 case AVAILABLE: 16052 return "Available Volume"; 16053 case CONSUMPTION: 16054 return "Consumption Volume"; 16055 case CURRENT: 16056 return "Current Volume"; 16057 case INITIAL: 16058 return "Initial Volume"; 16059 case _ANNOTATIONTYPE: 16060 return "AnnotationType"; 16061 case _ACTPATIENTANNOTATIONTYPE: 16062 return "ActPatientAnnotationType"; 16063 case ANNDI: 16064 return "diagnostic image note"; 16065 case ANNGEN: 16066 return "general note"; 16067 case ANNIMM: 16068 return "immunization note"; 16069 case ANNLAB: 16070 return "laboratory note"; 16071 case ANNMED: 16072 return "medication note"; 16073 case _GENETICOBSERVATIONTYPE: 16074 return "GeneticObservationType"; 16075 case GENE: 16076 return "gene"; 16077 case _IMMUNIZATIONOBSERVATIONTYPE: 16078 return "ImmunizationObservationType"; 16079 case OBSANTC: 16080 return "antigen count"; 16081 case OBSANTV: 16082 return "antigen validity"; 16083 case _INDIVIDUALCASESAFETYREPORTTYPE: 16084 return "Individual Case Safety Report Type"; 16085 case PATADVEVNT: 16086 return "patient adverse event"; 16087 case VACPROBLEM: 16088 return "vaccine product problem"; 16089 case _LOINCOBSERVATIONACTCONTEXTAGETYPE: 16090 return "LOINCObservationActContextAgeType"; 16091 case _216119: 16092 return "age patient qn est"; 16093 case _216127: 16094 return "age patient qn reported"; 16095 case _295535: 16096 return "age patient qn calc"; 16097 case _305250: 16098 return "age patient qn definition"; 16099 case _309724: 16100 return "age at onset of adverse event"; 16101 case _MEDICATIONOBSERVATIONTYPE: 16102 return "MedicationObservationType"; 16103 case REPHALFLIFE: 16104 return "representative half-life"; 16105 case SPLCOATING: 16106 return "coating"; 16107 case SPLCOLOR: 16108 return "color"; 16109 case SPLIMAGE: 16110 return "image"; 16111 case SPLIMPRINT: 16112 return "imprint"; 16113 case SPLSCORING: 16114 return "scoring"; 16115 case SPLSHAPE: 16116 return "shape"; 16117 case SPLSIZE: 16118 return "size"; 16119 case SPLSYMBOL: 16120 return "symbol"; 16121 case _OBSERVATIONISSUETRIGGERCODEDOBSERVATIONTYPE: 16122 return "ObservationIssueTriggerCodedObservationType"; 16123 case _CASETRANSMISSIONMODE: 16124 return "case transmission mode"; 16125 case AIRTRNS: 16126 return "airborne transmission"; 16127 case ANANTRNS: 16128 return "animal to animal transmission"; 16129 case ANHUMTRNS: 16130 return "animal to human transmission"; 16131 case BDYFLDTRNS: 16132 return "body fluid contact transmission"; 16133 case BLDTRNS: 16134 return "blood borne transmission"; 16135 case DERMTRNS: 16136 return "transdermal transmission"; 16137 case ENVTRNS: 16138 return "environmental exposure transmission"; 16139 case FECTRNS: 16140 return "fecal-oral transmission"; 16141 case FOMTRNS: 16142 return "fomite transmission"; 16143 case FOODTRNS: 16144 return "food-borne transmission"; 16145 case HUMHUMTRNS: 16146 return "human to human transmission"; 16147 case INDTRNS: 16148 return "indeterminate disease transmission mode"; 16149 case LACTTRNS: 16150 return "lactation transmission"; 16151 case NOSTRNS: 16152 return "nosocomial transmission"; 16153 case PARTRNS: 16154 return "parenteral transmission"; 16155 case PLACTRNS: 16156 return "transplacental transmission"; 16157 case SEXTRNS: 16158 return "sexual transmission"; 16159 case TRNSFTRNS: 16160 return "transfusion transmission"; 16161 case VECTRNS: 16162 return "vector-borne transmission"; 16163 case WATTRNS: 16164 return "water-borne transmission"; 16165 case _OBSERVATIONQUALITYMEASUREATTRIBUTE: 16166 return "ObservationQualityMeasureAttribute"; 16167 case AGGREGATE: 16168 return "aggregate measure observation"; 16169 case CMPMSRMTH: 16170 return "composite measure method"; 16171 case CMPMSRSCRWGHT: 16172 return "component measure scoring weight"; 16173 case COPY: 16174 return "copyright"; 16175 case CRS: 16176 return "clinical recommendation statement"; 16177 case DEF: 16178 return "definition"; 16179 case DISC: 16180 return "disclaimer"; 16181 case FINALDT: 16182 return "finalized date/time"; 16183 case GUIDE: 16184 return "guidance"; 16185 case IDUR: 16186 return "improvement notation"; 16187 case ITMCNT: 16188 return "items counted"; 16189 case KEY: 16190 return "keyword"; 16191 case MEDT: 16192 return "measurement end date"; 16193 case MSD: 16194 return "measurement start date"; 16195 case MSRADJ: 16196 return "risk adjustment"; 16197 case MSRAGG: 16198 return "rate aggregation"; 16199 case MSRIMPROV: 16200 return "health quality measure improvement notation"; 16201 case MSRJUR: 16202 return "jurisdiction"; 16203 case MSRRPTR: 16204 return "reporter type"; 16205 case MSRRPTTIME: 16206 return "timeframe for reporting"; 16207 case MSRSCORE: 16208 return "measure scoring"; 16209 case MSRSET: 16210 return "health quality measure care setting"; 16211 case MSRTOPIC: 16212 return "health quality measure topic type"; 16213 case MSRTP: 16214 return "measurement period"; 16215 case MSRTYPE: 16216 return "measure type"; 16217 case RAT: 16218 return "rationale"; 16219 case REF: 16220 return "reference"; 16221 case SDE: 16222 return "supplemental data elements"; 16223 case STRAT: 16224 return "stratification"; 16225 case TRANF: 16226 return "transmission format"; 16227 case USE: 16228 return "notice of use"; 16229 case _OBSERVATIONSEQUENCETYPE: 16230 return "ObservationSequenceType"; 16231 case TIMEABSOLUTE: 16232 return "absolute time sequence"; 16233 case TIMERELATIVE: 16234 return "relative time sequence"; 16235 case _OBSERVATIONSERIESTYPE: 16236 return "ObservationSeriesType"; 16237 case _ECGOBSERVATIONSERIESTYPE: 16238 return "ECGObservationSeriesType"; 16239 case REPRESENTATIVEBEAT: 16240 return "ECG representative beat waveforms"; 16241 case RHYTHM: 16242 return "ECG rhythm waveforms"; 16243 case _PATIENTIMMUNIZATIONRELATEDOBSERVATIONTYPE: 16244 return "PatientImmunizationRelatedObservationType"; 16245 case CLSSRM: 16246 return "classroom"; 16247 case GRADE: 16248 return "grade"; 16249 case SCHL: 16250 return "school"; 16251 case SCHLDIV: 16252 return "school division"; 16253 case TEACHER: 16254 return "teacher"; 16255 case _POPULATIONINCLUSIONOBSERVATIONTYPE: 16256 return "PopulationInclusionObservationType"; 16257 case DENEX: 16258 return "denominator exclusions"; 16259 case DENEXCEP: 16260 return "denominator exceptions"; 16261 case DENOM: 16262 return "denominator"; 16263 case IPOP: 16264 return "initial population"; 16265 case IPPOP: 16266 return "initial patient population"; 16267 case MSROBS: 16268 return "measure observation"; 16269 case MSRPOPL: 16270 return "measure population"; 16271 case MSRPOPLEX: 16272 return "measure population exclusions"; 16273 case NUMER: 16274 return "numerator"; 16275 case NUMEX: 16276 return "numerator exclusions"; 16277 case _PREFERENCEOBSERVATIONTYPE: 16278 return "_PreferenceObservationType"; 16279 case PREFSTRENGTH: 16280 return "preference strength"; 16281 case ADVERSEREACTION: 16282 return "Adverse Reaction"; 16283 case ASSERTION: 16284 return "Assertion"; 16285 case CASESER: 16286 return "case seriousness criteria"; 16287 case CDIO: 16288 return "case disease imported observation"; 16289 case CRIT: 16290 return "criticality"; 16291 case CTMO: 16292 return "case transmission mode observation"; 16293 case DX: 16294 return "ObservationDiagnosisTypes"; 16295 case ADMDX: 16296 return "admitting diagnosis"; 16297 case DISDX: 16298 return "discharge diagnosis"; 16299 case INTDX: 16300 return "intermediate diagnosis"; 16301 case NOI: 16302 return "nature of injury"; 16303 case GISTIER: 16304 return "GIS tier"; 16305 case HHOBS: 16306 return "household situation observation"; 16307 case ISSUE: 16308 return "detected issue"; 16309 case _ACTADMINISTRATIVEDETECTEDISSUECODE: 16310 return "ActAdministrativeDetectedIssueCode"; 16311 case _ACTADMINISTRATIVEAUTHORIZATIONDETECTEDISSUECODE: 16312 return "ActAdministrativeAuthorizationDetectedIssueCode"; 16313 case NAT: 16314 return "Insufficient authorization"; 16315 case SUPPRESSED: 16316 return "record suppressed"; 16317 case VALIDAT: 16318 return "validation issue"; 16319 case KEY204: 16320 return "Unknown key identifier"; 16321 case KEY205: 16322 return "Duplicate key identifier"; 16323 case COMPLY: 16324 return "Compliance Alert"; 16325 case DUPTHPY: 16326 return "Duplicate Therapy Alert"; 16327 case DUPTHPCLS: 16328 return "duplicate therapeutic alass alert"; 16329 case DUPTHPGEN: 16330 return "duplicate generic alert"; 16331 case ABUSE: 16332 return "commonly abused/misused alert"; 16333 case FRAUD: 16334 return "potential fraud"; 16335 case PLYDOC: 16336 return "Poly-orderer Alert"; 16337 case PLYPHRM: 16338 return "Poly-supplier Alert"; 16339 case DOSE: 16340 return "Dosage problem"; 16341 case DOSECOND: 16342 return "dosage-condition alert"; 16343 case DOSEDUR: 16344 return "Dose-Duration Alert"; 16345 case DOSEDURH: 16346 return "Dose-Duration High Alert"; 16347 case DOSEDURHIND: 16348 return "Dose-Duration High for Indication Alert"; 16349 case DOSEDURL: 16350 return "Dose-Duration Low Alert"; 16351 case DOSEDURLIND: 16352 return "Dose-Duration Low for Indication Alert"; 16353 case DOSEH: 16354 return "High Dose Alert"; 16355 case DOSEHINDA: 16356 return "High Dose for Age Alert"; 16357 case DOSEHIND: 16358 return "High Dose for Indication Alert"; 16359 case DOSEHINDSA: 16360 return "High Dose for Height/Surface Area Alert"; 16361 case DOSEHINDW: 16362 return "High Dose for Weight Alert"; 16363 case DOSEIVL: 16364 return "Dose-Interval Alert"; 16365 case DOSEIVLIND: 16366 return "Dose-Interval for Indication Alert"; 16367 case DOSEL: 16368 return "Low Dose Alert"; 16369 case DOSELINDA: 16370 return "Low Dose for Age Alert"; 16371 case DOSELIND: 16372 return "Low Dose for Indication Alert"; 16373 case DOSELINDSA: 16374 return "Low Dose for Height/Surface Area Alert"; 16375 case DOSELINDW: 16376 return "Low Dose for Weight Alert"; 16377 case MDOSE: 16378 return "maximum dosage reached"; 16379 case OBSA: 16380 return "Observation Alert"; 16381 case AGE: 16382 return "Age Alert"; 16383 case ADALRT: 16384 return "adult alert"; 16385 case GEALRT: 16386 return "geriatric alert"; 16387 case PEALRT: 16388 return "pediatric alert"; 16389 case COND: 16390 return "Condition Alert"; 16391 case HGHT: 16392 return "HGHT"; 16393 case LACT: 16394 return "Lactation Alert"; 16395 case PREG: 16396 return "Pregnancy Alert"; 16397 case WGHT: 16398 return "WGHT"; 16399 case CREACT: 16400 return "common reaction alert"; 16401 case GEN: 16402 return "Genetic Alert"; 16403 case GEND: 16404 return "Gender Alert"; 16405 case LAB: 16406 return "Lab Alert"; 16407 case REACT: 16408 return "Reaction Alert"; 16409 case ALGY: 16410 return "Allergy Alert"; 16411 case INT: 16412 return "Intolerance Alert"; 16413 case RREACT: 16414 return "Related Reaction Alert"; 16415 case RALG: 16416 return "Related Allergy Alert"; 16417 case RAR: 16418 return "Related Prior Reaction Alert"; 16419 case RINT: 16420 return "Related Intolerance Alert"; 16421 case BUS: 16422 return "business constraint violation"; 16423 case CODEINVAL: 16424 return "code is not valid"; 16425 case CODEDEPREC: 16426 return "code has been deprecated"; 16427 case FORMAT: 16428 return "invalid format"; 16429 case ILLEGAL: 16430 return "illegal"; 16431 case LENRANGE: 16432 return "length out of range"; 16433 case LENLONG: 16434 return "length is too long"; 16435 case LENSHORT: 16436 return "length is too short"; 16437 case MISSCOND: 16438 return "conditional element missing"; 16439 case MISSMAND: 16440 return "mandatory element missing"; 16441 case NODUPS: 16442 return "duplicate values are not permitted"; 16443 case NOPERSIST: 16444 return "element will not be persisted"; 16445 case REPRANGE: 16446 return "repetitions out of range"; 16447 case MAXOCCURS: 16448 return "repetitions above maximum"; 16449 case MINOCCURS: 16450 return "repetitions below minimum"; 16451 case _ACTADMINISTRATIVERULEDETECTEDISSUECODE: 16452 return "ActAdministrativeRuleDetectedIssueCode"; 16453 case KEY206: 16454 return "non-matching identification"; 16455 case OBSOLETE: 16456 return "obsolete record returned"; 16457 case _ACTSUPPLIEDITEMDETECTEDISSUECODE: 16458 return "ActSuppliedItemDetectedIssueCode"; 16459 case _ADMINISTRATIONDETECTEDISSUECODE: 16460 return "AdministrationDetectedIssueCode"; 16461 case _APPROPRIATENESSDETECTEDISSUECODE: 16462 return "AppropriatenessDetectedIssueCode"; 16463 case _INTERACTIONDETECTEDISSUECODE: 16464 return "InteractionDetectedIssueCode"; 16465 case FOOD: 16466 return "Food Interaction Alert"; 16467 case TPROD: 16468 return "Therapeutic Product Alert"; 16469 case DRG: 16470 return "Drug Interaction Alert"; 16471 case NHP: 16472 return "Natural Health Product Alert"; 16473 case NONRX: 16474 return "Non-Prescription Interaction Alert"; 16475 case PREVINEF: 16476 return "previously ineffective"; 16477 case DACT: 16478 return "drug action detected issue"; 16479 case TIME: 16480 return "timing detected issue"; 16481 case ALRTENDLATE: 16482 return "end too late alert"; 16483 case ALRTSTRTLATE: 16484 return "start too late alert"; 16485 case _TIMINGDETECTEDISSUECODE: 16486 return "TimingDetectedIssueCode"; 16487 case ENDLATE: 16488 return "End Too Late Alert"; 16489 case STRTLATE: 16490 return "Start Too Late Alert"; 16491 case _SUPPLYDETECTEDISSUECODE: 16492 return "SupplyDetectedIssueCode"; 16493 case ALLDONE: 16494 return "already performed"; 16495 case FULFIL: 16496 return "fulfillment alert"; 16497 case NOTACTN: 16498 return "no longer actionable"; 16499 case NOTEQUIV: 16500 return "not equivalent alert"; 16501 case NOTEQUIVGEN: 16502 return "not generically equivalent alert"; 16503 case NOTEQUIVTHER: 16504 return "not therapeutically equivalent alert"; 16505 case TIMING: 16506 return "event timing incorrect alert"; 16507 case INTERVAL: 16508 return "outside requested time"; 16509 case MINFREQ: 16510 return "too soon within frequency based on the usage"; 16511 case HELD: 16512 return "held/suspended alert"; 16513 case TOOLATE: 16514 return "Refill Too Late Alert"; 16515 case TOOSOON: 16516 return "Refill Too Soon Alert"; 16517 case HISTORIC: 16518 return "record recorded as historical"; 16519 case PATPREF: 16520 return "violates stated preferences"; 16521 case PATPREFALT: 16522 return "violates stated preferences, alternate available"; 16523 case KSUBJ: 16524 return "knowledge subject"; 16525 case KSUBT: 16526 return "knowledge subtopic"; 16527 case OINT: 16528 return "intolerance"; 16529 case ALG: 16530 return "Allergy"; 16531 case DALG: 16532 return "Drug Allergy"; 16533 case EALG: 16534 return "Environmental Allergy"; 16535 case FALG: 16536 return "Food Allergy"; 16537 case DINT: 16538 return "Drug Intolerance"; 16539 case DNAINT: 16540 return "Drug Non-Allergy Intolerance"; 16541 case EINT: 16542 return "Environmental Intolerance"; 16543 case ENAINT: 16544 return "Environmental Non-Allergy Intolerance"; 16545 case FINT: 16546 return "Food Intolerance"; 16547 case FNAINT: 16548 return "Food Non-Allergy Intolerance"; 16549 case NAINT: 16550 return "Non-Allergy Intolerance"; 16551 case SEV: 16552 return "Severity Observation"; 16553 case _FDALABELDATA: 16554 return "FDALabelData"; 16555 case FDACOATING: 16556 return "coating"; 16557 case FDACOLOR: 16558 return "color"; 16559 case FDAIMPRINTCD: 16560 return "imprint code"; 16561 case FDALOGO: 16562 return "logo"; 16563 case FDASCORING: 16564 return "scoring"; 16565 case FDASHAPE: 16566 return "shape"; 16567 case FDASIZE: 16568 return "size"; 16569 case _ROIOVERLAYSHAPE: 16570 return "ROIOverlayShape"; 16571 case CIRCLE: 16572 return "circle"; 16573 case ELLIPSE: 16574 return "ellipse"; 16575 case POINT: 16576 return "point"; 16577 case POLY: 16578 return "polyline"; 16579 case C: 16580 return "corrected"; 16581 case DIET: 16582 return "Diet"; 16583 case BR: 16584 return "breikost (GE)"; 16585 case DM: 16586 return "diabetes mellitus diet"; 16587 case FAST: 16588 return "fasting"; 16589 case FORMULA: 16590 return "formula diet"; 16591 case GF: 16592 return "gluten free"; 16593 case LF: 16594 return "low fat"; 16595 case LP: 16596 return "low protein"; 16597 case LQ: 16598 return "liquid"; 16599 case LS: 16600 return "low sodium"; 16601 case N: 16602 return "normal diet"; 16603 case NF: 16604 return "no fat"; 16605 case PAF: 16606 return "phenylalanine free"; 16607 case PAR: 16608 return "parenteral"; 16609 case RD: 16610 return "reduction diet"; 16611 case SCH: 16612 return "schonkost (GE)"; 16613 case SUPPLEMENT: 16614 return "nutritional supplement"; 16615 case T: 16616 return "tea only"; 16617 case VLI: 16618 return "low valin, leucin, isoleucin"; 16619 case DRUGPRG: 16620 return "drug program"; 16621 case F: 16622 return "final"; 16623 case PRLMN: 16624 return "preliminary"; 16625 case SECOBS: 16626 return "SecurityObservationType"; 16627 case SECCATOBS: 16628 return "security category observation"; 16629 case SECCLASSOBS: 16630 return "security classification observation"; 16631 case SECCONOBS: 16632 return "security control observation"; 16633 case SECINTOBS: 16634 return "security integrity observation"; 16635 case SECALTINTOBS: 16636 return "security alteration integrity observation"; 16637 case SECDATINTOBS: 16638 return "security data integrity observation"; 16639 case SECINTCONOBS: 16640 return "security integrity confidence observation"; 16641 case SECINTPRVOBS: 16642 return "security integrity provenance observation"; 16643 case SECINTPRVABOBS: 16644 return "security integrity provenance asserted by observation"; 16645 case SECINTPRVRBOBS: 16646 return "security integrity provenance reported by observation"; 16647 case SECINTSTOBS: 16648 return "security integrity status observation"; 16649 case SECTRSTOBS: 16650 return "SECTRSTOBS"; 16651 case TRSTACCRDOBS: 16652 return "trust accreditation observation"; 16653 case TRSTAGREOBS: 16654 return "trust agreement observation"; 16655 case TRSTCERTOBS: 16656 return "trust certificate observation"; 16657 case TRSTFWKOBS: 16658 return "trust framework observation"; 16659 case TRSTLOAOBS: 16660 return "trust assurance observation"; 16661 case TRSTMECOBS: 16662 return "trust mechanism observation"; 16663 case SUBSIDFFS: 16664 return "subsidized fee for service program"; 16665 case WRKCOMP: 16666 return "(workers compensation program"; 16667 case _ACTPROCEDURECODE: 16668 return "ActProcedureCode"; 16669 case _ACTBILLABLESERVICECODE: 16670 return "ActBillableServiceCode"; 16671 case _HL7DEFINEDACTCODES: 16672 return "HL7DefinedActCodes"; 16673 case COPAY: 16674 return "COPAY"; 16675 case DEDUCT: 16676 return "DEDUCT"; 16677 case DOSEIND: 16678 return "DOSEIND"; 16679 case PRA: 16680 return "PRA"; 16681 case STORE: 16682 return "Storage"; 16683 case NULL: 16684 return null; 16685 default: 16686 return "?"; 16687 } 16688 } 16689 16690}