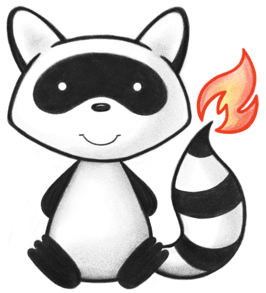
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ActPriority { 037 038 /** 039 * As soon as possible, next highest priority after stat. 040 */ 041 A, 042 /** 043 * Filler should contact the placer as soon as results are available, even for 044 * preliminary results. (Was "C" in HL7 version 2.3's reporting priority.) 045 */ 046 CR, 047 /** 048 * Filler should contact the placer (or target) to schedule the service. (Was 049 * "C" in HL7 version 2.3's TQ-priority component.) 050 */ 051 CS, 052 /** 053 * Filler should contact the placer to schedule the service. (Was "C" in HL7 054 * version 2.3's TQ-priority component.) 055 */ 056 CSP, 057 /** 058 * Filler should contact the service recipient (target) to schedule the service. 059 * (Was "C" in HL7 version 2.3's TQ-priority component.) 060 */ 061 CSR, 062 /** 063 * Beneficial to the patient but not essential for survival. 064 */ 065 EL, 066 /** 067 * An unforeseen combination of circumstances or the resulting state that calls 068 * for immediate action. 069 */ 070 EM, 071 /** 072 * Used to indicate that a service is to be performed prior to a scheduled 073 * surgery. When ordering a service and using the pre-op priority, a check is 074 * done to see the amount of time that must be allowed for performance of the 075 * service. When the order is placed, a message can be generated indicating the 076 * time needed for the service so that it is not ordered in conflict with a 077 * scheduled operation. 078 */ 079 P, 080 /** 081 * An "as needed" order should be accompanied by a description of what 082 * constitutes a need. This description is represented by an observation service 083 * predicate as a precondition. 084 */ 085 PRN, 086 /** 087 * Routine service, do at usual work hours. 088 */ 089 R, 090 /** 091 * A report should be prepared and sent as quickly as possible. 092 */ 093 RR, 094 /** 095 * With highest priority (e.g., emergency). 096 */ 097 S, 098 /** 099 * It is critical to come as close as possible to the requested time (e.g., for 100 * a through antimicrobial level). 101 */ 102 T, 103 /** 104 * Drug is to be used as directed by the prescriber. 105 */ 106 UD, 107 /** 108 * Calls for prompt action. 109 */ 110 UR, 111 /** 112 * added to help the parsers 113 */ 114 NULL; 115 116 public static V3ActPriority fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("A".equals(codeString)) 120 return A; 121 if ("CR".equals(codeString)) 122 return CR; 123 if ("CS".equals(codeString)) 124 return CS; 125 if ("CSP".equals(codeString)) 126 return CSP; 127 if ("CSR".equals(codeString)) 128 return CSR; 129 if ("EL".equals(codeString)) 130 return EL; 131 if ("EM".equals(codeString)) 132 return EM; 133 if ("P".equals(codeString)) 134 return P; 135 if ("PRN".equals(codeString)) 136 return PRN; 137 if ("R".equals(codeString)) 138 return R; 139 if ("RR".equals(codeString)) 140 return RR; 141 if ("S".equals(codeString)) 142 return S; 143 if ("T".equals(codeString)) 144 return T; 145 if ("UD".equals(codeString)) 146 return UD; 147 if ("UR".equals(codeString)) 148 return UR; 149 throw new FHIRException("Unknown V3ActPriority code '" + codeString + "'"); 150 } 151 152 public String toCode() { 153 switch (this) { 154 case A: 155 return "A"; 156 case CR: 157 return "CR"; 158 case CS: 159 return "CS"; 160 case CSP: 161 return "CSP"; 162 case CSR: 163 return "CSR"; 164 case EL: 165 return "EL"; 166 case EM: 167 return "EM"; 168 case P: 169 return "P"; 170 case PRN: 171 return "PRN"; 172 case R: 173 return "R"; 174 case RR: 175 return "RR"; 176 case S: 177 return "S"; 178 case T: 179 return "T"; 180 case UD: 181 return "UD"; 182 case UR: 183 return "UR"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getSystem() { 192 return "http://terminology.hl7.org/CodeSystem/v3-ActPriority"; 193 } 194 195 public String getDefinition() { 196 switch (this) { 197 case A: 198 return "As soon as possible, next highest priority after stat."; 199 case CR: 200 return "Filler should contact the placer as soon as results are available, even for preliminary results. (Was \"C\" in HL7 version 2.3's reporting priority.)"; 201 case CS: 202 return "Filler should contact the placer (or target) to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 203 case CSP: 204 return "Filler should contact the placer to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 205 case CSR: 206 return "Filler should contact the service recipient (target) to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 207 case EL: 208 return "Beneficial to the patient but not essential for survival."; 209 case EM: 210 return "An unforeseen combination of circumstances or the resulting state that calls for immediate action."; 211 case P: 212 return "Used to indicate that a service is to be performed prior to a scheduled surgery. When ordering a service and using the pre-op priority, a check is done to see the amount of time that must be allowed for performance of the service. When the order is placed, a message can be generated indicating the time needed for the service so that it is not ordered in conflict with a scheduled operation."; 213 case PRN: 214 return "An \"as needed\" order should be accompanied by a description of what constitutes a need. This description is represented by an observation service predicate as a precondition."; 215 case R: 216 return "Routine service, do at usual work hours."; 217 case RR: 218 return "A report should be prepared and sent as quickly as possible."; 219 case S: 220 return "With highest priority (e.g., emergency)."; 221 case T: 222 return "It is critical to come as close as possible to the requested time (e.g., for a through antimicrobial level)."; 223 case UD: 224 return "Drug is to be used as directed by the prescriber."; 225 case UR: 226 return "Calls for prompt action."; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 234 public String getDisplay() { 235 switch (this) { 236 case A: 237 return "ASAP"; 238 case CR: 239 return "callback results"; 240 case CS: 241 return "callback for scheduling"; 242 case CSP: 243 return "callback placer for scheduling"; 244 case CSR: 245 return "contact recipient for scheduling"; 246 case EL: 247 return "elective"; 248 case EM: 249 return "emergency"; 250 case P: 251 return "preop"; 252 case PRN: 253 return "as needed"; 254 case R: 255 return "routine"; 256 case RR: 257 return "rush reporting"; 258 case S: 259 return "stat"; 260 case T: 261 return "timing critical"; 262 case UD: 263 return "use as directed"; 264 case UR: 265 return "urgent"; 266 case NULL: 267 return null; 268 default: 269 return "?"; 270 } 271 } 272 273}