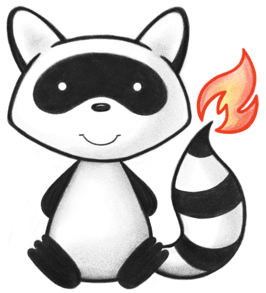
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3CodingRationale { 037 038 /** 039 * Description: Originally produced code. 040 */ 041 O, 042 /** 043 * Originally produced code, required by the specification describing the use of 044 * the coded concept. 045 */ 046 OR, 047 /** 048 * Description: Post-coded from free text source</description> 049 */ 050 P, 051 /** 052 * Post-coded from free text source, required by the specification describing 053 * the use of the coded concept. 054 */ 055 PR, 056 /** 057 * Description: Required standard code for HL7. 058 */ 059 R, 060 /** 061 * HL7 Specified or Mandated 062 */ 063 HL7, 064 /** 065 * Both HL7 mandated and the original code (precoordination) 066 */ 067 SH, 068 /** 069 * Source (or original) code 070 */ 071 SRC, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static V3CodingRationale fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("O".equals(codeString)) 081 return O; 082 if ("OR".equals(codeString)) 083 return OR; 084 if ("P".equals(codeString)) 085 return P; 086 if ("PR".equals(codeString)) 087 return PR; 088 if ("R".equals(codeString)) 089 return R; 090 if ("HL7".equals(codeString)) 091 return HL7; 092 if ("SH".equals(codeString)) 093 return SH; 094 if ("SRC".equals(codeString)) 095 return SRC; 096 throw new FHIRException("Unknown V3CodingRationale code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case O: 102 return "O"; 103 case OR: 104 return "OR"; 105 case P: 106 return "P"; 107 case PR: 108 return "PR"; 109 case R: 110 return "R"; 111 case HL7: 112 return "HL7"; 113 case SH: 114 return "SH"; 115 case SRC: 116 return "SRC"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 return "http://terminology.hl7.org/CodeSystem/v3-CodingRationale"; 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case O: 131 return "Description: Originally produced code."; 132 case OR: 133 return "Originally produced code, required by the specification describing the use of the coded concept."; 134 case P: 135 return "Description: Post-coded from free text source</description>"; 136 case PR: 137 return "Post-coded from free text source, required by the specification describing the use of the coded concept."; 138 case R: 139 return "Description: Required standard code for HL7."; 140 case HL7: 141 return "HL7 Specified or Mandated"; 142 case SH: 143 return "Both HL7 mandated and the original code (precoordination)"; 144 case SRC: 145 return "Source (or original) code"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getDisplay() { 154 switch (this) { 155 case O: 156 return "originally produced code"; 157 case OR: 158 return "original and required"; 159 case P: 160 return "post-coded"; 161 case PR: 162 return "post-coded and required"; 163 case R: 164 return "required"; 165 case HL7: 166 return "HL7 Specified or Mandated"; 167 case SH: 168 return "Both HL7 mandated and the original code"; 169 case SRC: 170 return "Source (or original) code"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178}