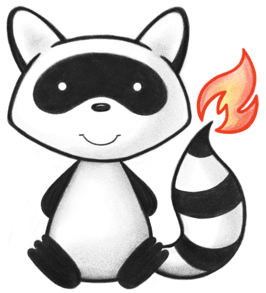
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3CompressionAlgorithm { 037 038 /** 039 * bzip-2 compression format. See [http://www.bzip.org/] for more information. 040 */ 041 BZ, 042 /** 043 * The deflate compressed data format as specified in RFC 1951 044 * [http://www.ietf.org/rfc/rfc1951.txt]. 045 */ 046 DF, 047 /** 048 * A compressed data format that is compatible with the widely used GZIP utility 049 * as specified in RFC 1952 [http://www.ietf.org/rfc/rfc1952.txt] (uses the 050 * deflate algorithm). 051 */ 052 GZ, 053 /** 054 * Original UNIX compress algorithm and file format using the LZC algorithm (a 055 * variant of LZW). Patent encumbered and less efficient than deflate. 056 */ 057 Z, 058 /** 059 * 7z compression file format. See [http://www.7-zip.org/7z.html] for more 060 * information. 061 */ 062 Z7, 063 /** 064 * A compressed data format that also uses the deflate algorithm. Specified as 065 * RFC 1950 [http://www.ietf.org/rfc/rfc1952.txt] 066 */ 067 ZL, 068 /** 069 * added to help the parsers 070 */ 071 NULL; 072 073 public static V3CompressionAlgorithm fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("BZ".equals(codeString)) 077 return BZ; 078 if ("DF".equals(codeString)) 079 return DF; 080 if ("GZ".equals(codeString)) 081 return GZ; 082 if ("Z".equals(codeString)) 083 return Z; 084 if ("Z7".equals(codeString)) 085 return Z7; 086 if ("ZL".equals(codeString)) 087 return ZL; 088 throw new FHIRException("Unknown V3CompressionAlgorithm code '" + codeString + "'"); 089 } 090 091 public String toCode() { 092 switch (this) { 093 case BZ: 094 return "BZ"; 095 case DF: 096 return "DF"; 097 case GZ: 098 return "GZ"; 099 case Z: 100 return "Z"; 101 case Z7: 102 return "Z7"; 103 case ZL: 104 return "ZL"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 return "http://terminology.hl7.org/CodeSystem/v3-CompressionAlgorithm"; 114 } 115 116 public String getDefinition() { 117 switch (this) { 118 case BZ: 119 return "bzip-2 compression format. See [http://www.bzip.org/] for more information."; 120 case DF: 121 return "The deflate compressed data format as specified in RFC 1951 [http://www.ietf.org/rfc/rfc1951.txt]."; 122 case GZ: 123 return "A compressed data format that is compatible with the widely used GZIP utility as specified in RFC 1952 [http://www.ietf.org/rfc/rfc1952.txt] (uses the deflate algorithm)."; 124 case Z: 125 return "Original UNIX compress algorithm and file format using the LZC algorithm (a variant of LZW). Patent encumbered and less efficient than deflate."; 126 case Z7: 127 return "7z compression file format. See [http://www.7-zip.org/7z.html] for more information."; 128 case ZL: 129 return "A compressed data format that also uses the deflate algorithm. Specified as RFC 1950 [http://www.ietf.org/rfc/rfc1952.txt]"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDisplay() { 138 switch (this) { 139 case BZ: 140 return "bzip"; 141 case DF: 142 return "deflate"; 143 case GZ: 144 return "gzip"; 145 case Z: 146 return "compress"; 147 case Z7: 148 return "Z7"; 149 case ZL: 150 return "zlib"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158}