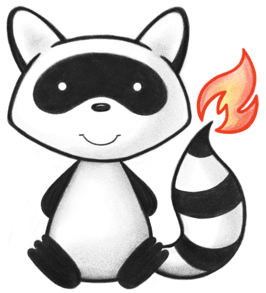
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3DocumentCompletion { 037 038 /** 039 * A completion status in which a document has been signed manually or 040 * electronically by one or more individuals who attest to its accuracy. No 041 * explicit determination is made that the assigned individual has performed the 042 * authentication. While the standard allows multiple instances of 043 * authentication, it would be typical to have a single instance of 044 * authentication, usually by the assigned individual. 045 */ 046 AU, 047 /** 048 * A completion status in which information has been orally recorded but not yet 049 * transcribed. 050 */ 051 DI, 052 /** 053 * A completion status in which document content, other than dictation, has been 054 * received but has not been translated into the final electronic format. 055 * Examples include paper documents, whether hand-written or typewritten, and 056 * intermediate electronic forms, such as voice to text. 057 */ 058 DO, 059 /** 060 * A completion status in which information is known to be missing from a 061 * transcribed document. 062 */ 063 IN, 064 /** 065 * A workflow status where the material has been assigned to personnel to 066 * perform the task of transcription. The document remains in this state until 067 * the document is transcribed. 068 */ 069 IP, 070 /** 071 * A completion status in which a document has been signed manually or 072 * electronically by the individual who is legally responsible for that 073 * document. This is the most mature state in the workflow progression. 074 */ 075 LA, 076 /** 077 * A completion status in which a document was created in error or was placed in 078 * the wrong chart. The document is no longer available. 079 */ 080 NU, 081 /** 082 * A completion status in which a document is transcribed but not authenticated. 083 */ 084 PA, 085 /** 086 * A completion status where the document is complete and there is no 087 * expectation that the document will be signed. 088 */ 089 UC, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 095 public static V3DocumentCompletion fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("AU".equals(codeString)) 099 return AU; 100 if ("DI".equals(codeString)) 101 return DI; 102 if ("DO".equals(codeString)) 103 return DO; 104 if ("IN".equals(codeString)) 105 return IN; 106 if ("IP".equals(codeString)) 107 return IP; 108 if ("LA".equals(codeString)) 109 return LA; 110 if ("NU".equals(codeString)) 111 return NU; 112 if ("PA".equals(codeString)) 113 return PA; 114 if ("UC".equals(codeString)) 115 return UC; 116 throw new FHIRException("Unknown V3DocumentCompletion code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case AU: 122 return "AU"; 123 case DI: 124 return "DI"; 125 case DO: 126 return "DO"; 127 case IN: 128 return "IN"; 129 case IP: 130 return "IP"; 131 case LA: 132 return "LA"; 133 case NU: 134 return "NU"; 135 case PA: 136 return "PA"; 137 case UC: 138 return "UC"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 return "http://terminology.hl7.org/CodeSystem/v3-DocumentCompletion"; 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case AU: 153 return "A completion status in which a document has been signed manually or electronically by one or more individuals who attest to its accuracy. No explicit determination is made that the assigned individual has performed the authentication. While the standard allows multiple instances of authentication, it would be typical to have a single instance of authentication, usually by the assigned individual."; 154 case DI: 155 return "A completion status in which information has been orally recorded but not yet transcribed."; 156 case DO: 157 return "A completion status in which document content, other than dictation, has been received but has not been translated into the final electronic format. Examples include paper documents, whether hand-written or typewritten, and intermediate electronic forms, such as voice to text."; 158 case IN: 159 return "A completion status in which information is known to be missing from a transcribed document."; 160 case IP: 161 return "A workflow status where the material has been assigned to personnel to perform the task of transcription. The document remains in this state until the document is transcribed."; 162 case LA: 163 return "A completion status in which a document has been signed manually or electronically by the individual who is legally responsible for that document. This is the most mature state in the workflow progression."; 164 case NU: 165 return "A completion status in which a document was created in error or was placed in the wrong chart. The document is no longer available."; 166 case PA: 167 return "A completion status in which a document is transcribed but not authenticated."; 168 case UC: 169 return "A completion status where the document is complete and there is no expectation that the document will be signed."; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 177 public String getDisplay() { 178 switch (this) { 179 case AU: 180 return "authenticated"; 181 case DI: 182 return "dictated"; 183 case DO: 184 return "documented"; 185 case IN: 186 return "incomplete"; 187 case IP: 188 return "in progress"; 189 case LA: 190 return "legally authenticated"; 191 case NU: 192 return "nullified document"; 193 case PA: 194 return "pre-authenticated"; 195 case UC: 196 return "unsigned completed document"; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204}