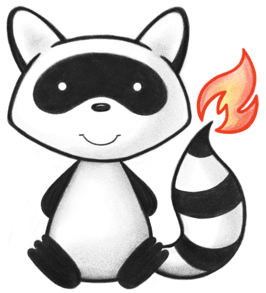
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityClass { 037 038 /** 039 * Corresponds to the Entity class 040 */ 041 ENT, 042 /** 043 * A health chart included to serve as a document receiving entity in the 044 * management of medical records. 045 */ 046 HCE, 047 /** 048 * Anything that essentially has the property of life, independent of current 049 * state (a dead human corpse is still essentially a living subject). 050 */ 051 LIV, 052 /** 053 * A subtype of living subject that includes all living things except the 054 * species Homo Sapiens. 055 */ 056 NLIV, 057 /** 058 * A living subject from the animal kingdom. 059 */ 060 ANM, 061 /** 062 * All single celled living organisms including protozoa, bacteria, yeast, 063 * viruses, etc. 064 */ 065 MIC, 066 /** 067 * A living subject from the order of plants. 068 */ 069 PLNT, 070 /** 071 * A living subject of the species homo sapiens. 072 */ 073 PSN, 074 /** 075 * Any thing that has extension in space and mass, may be of living or 076 * non-living origin. 077 */ 078 MAT, 079 /** 080 * A substance that is fully defined by an organic or inorganic chemical 081 * formula, includes mixtures of other chemical substances. Refine using, e.g., 082 * IUPAC codes. 083 */ 084 CHEM, 085 /** 086 * Naturally occurring, processed or manufactured entities that are primarily 087 * used as food for humans and animals. 088 */ 089 FOOD, 090 /** 091 * Corresponds to the ManufacturedMaterial class 092 */ 093 MMAT, 094 /** 095 * A container of other entities. 096 */ 097 CONT, 098 /** 099 * A type of container that can hold other containers or other holders. 100 */ 101 HOLD, 102 /** 103 * A subtype of ManufacturedMaterial used in an activity, without being 104 * substantially changed through that activity. The kind of device is identified 105 * by the code attribute inherited from Entity. 106 * 107 * 108 * Usage: This includes durable (reusable) medical equipment as well as 109 * disposable equipment. 110 */ 111 DEV, 112 /** 113 * A physical artifact that stores information about the granting of 114 * authorization. 115 */ 116 CER, 117 /** 118 * Class to contain unique attributes of diagnostic imaging equipment. 119 */ 120 MODDV, 121 /** 122 * A social or legal structure formed by human beings. 123 */ 124 ORG, 125 /** 126 * An agency of the people of a state often assuming some authority over a 127 * certain matter. Includes government, governmental agencies, associations. 128 */ 129 PUB, 130 /** 131 * A politically organized body of people bonded by territory, culture, or 132 * ethnicity, having sovereignty (to a certain extent) granted by other states 133 * (enclosing or neighboring states). This includes countries (nations), 134 * provinces (e.g., one of the United States of America or a French 135 * departement), counties or municipalities. Refine using, e.g., ISO country 136 * codes, FIPS-PUB state codes, etc. 137 */ 138 STATE, 139 /** 140 * A politically organized body of people bonded by territory and known as a 141 * nation. 142 */ 143 NAT, 144 /** 145 * A physical place or site with its containing structure. May be natural or 146 * man-made. The geographic position of a place may or may not be constant. 147 */ 148 PLC, 149 /** 150 * The territory of a city, town or other municipality. 151 */ 152 CITY, 153 /** 154 * The territory of a sovereign nation. 155 */ 156 COUNTRY, 157 /** 158 * The territory of a county, parish or other division of a state or province. 159 */ 160 COUNTY, 161 /** 162 * The territory of a state, province, department or other division of a 163 * sovereign country. 164 */ 165 PROVINCE, 166 /** 167 * A grouping of resources (personnel, material, or places) to be used for 168 * scheduling purposes. May be a pool of like-type resources, a team, or 169 * combination of personnel, material and places. 170 */ 171 RGRP, 172 /** 173 * added to help the parsers 174 */ 175 NULL; 176 177 public static V3EntityClass fromCode(String codeString) throws FHIRException { 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("ENT".equals(codeString)) 181 return ENT; 182 if ("HCE".equals(codeString)) 183 return HCE; 184 if ("LIV".equals(codeString)) 185 return LIV; 186 if ("NLIV".equals(codeString)) 187 return NLIV; 188 if ("ANM".equals(codeString)) 189 return ANM; 190 if ("MIC".equals(codeString)) 191 return MIC; 192 if ("PLNT".equals(codeString)) 193 return PLNT; 194 if ("PSN".equals(codeString)) 195 return PSN; 196 if ("MAT".equals(codeString)) 197 return MAT; 198 if ("CHEM".equals(codeString)) 199 return CHEM; 200 if ("FOOD".equals(codeString)) 201 return FOOD; 202 if ("MMAT".equals(codeString)) 203 return MMAT; 204 if ("CONT".equals(codeString)) 205 return CONT; 206 if ("HOLD".equals(codeString)) 207 return HOLD; 208 if ("DEV".equals(codeString)) 209 return DEV; 210 if ("CER".equals(codeString)) 211 return CER; 212 if ("MODDV".equals(codeString)) 213 return MODDV; 214 if ("ORG".equals(codeString)) 215 return ORG; 216 if ("PUB".equals(codeString)) 217 return PUB; 218 if ("STATE".equals(codeString)) 219 return STATE; 220 if ("NAT".equals(codeString)) 221 return NAT; 222 if ("PLC".equals(codeString)) 223 return PLC; 224 if ("CITY".equals(codeString)) 225 return CITY; 226 if ("COUNTRY".equals(codeString)) 227 return COUNTRY; 228 if ("COUNTY".equals(codeString)) 229 return COUNTY; 230 if ("PROVINCE".equals(codeString)) 231 return PROVINCE; 232 if ("RGRP".equals(codeString)) 233 return RGRP; 234 throw new FHIRException("Unknown V3EntityClass code '" + codeString + "'"); 235 } 236 237 public String toCode() { 238 switch (this) { 239 case ENT: 240 return "ENT"; 241 case HCE: 242 return "HCE"; 243 case LIV: 244 return "LIV"; 245 case NLIV: 246 return "NLIV"; 247 case ANM: 248 return "ANM"; 249 case MIC: 250 return "MIC"; 251 case PLNT: 252 return "PLNT"; 253 case PSN: 254 return "PSN"; 255 case MAT: 256 return "MAT"; 257 case CHEM: 258 return "CHEM"; 259 case FOOD: 260 return "FOOD"; 261 case MMAT: 262 return "MMAT"; 263 case CONT: 264 return "CONT"; 265 case HOLD: 266 return "HOLD"; 267 case DEV: 268 return "DEV"; 269 case CER: 270 return "CER"; 271 case MODDV: 272 return "MODDV"; 273 case ORG: 274 return "ORG"; 275 case PUB: 276 return "PUB"; 277 case STATE: 278 return "STATE"; 279 case NAT: 280 return "NAT"; 281 case PLC: 282 return "PLC"; 283 case CITY: 284 return "CITY"; 285 case COUNTRY: 286 return "COUNTRY"; 287 case COUNTY: 288 return "COUNTY"; 289 case PROVINCE: 290 return "PROVINCE"; 291 case RGRP: 292 return "RGRP"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getSystem() { 301 return "http://terminology.hl7.org/CodeSystem/v3-EntityClass"; 302 } 303 304 public String getDefinition() { 305 switch (this) { 306 case ENT: 307 return "Corresponds to the Entity class"; 308 case HCE: 309 return "A health chart included to serve as a document receiving entity in the management of medical records."; 310 case LIV: 311 return "Anything that essentially has the property of life, independent of current state (a dead human corpse is still essentially a living subject)."; 312 case NLIV: 313 return "A subtype of living subject that includes all living things except the species Homo Sapiens."; 314 case ANM: 315 return "A living subject from the animal kingdom."; 316 case MIC: 317 return "All single celled living organisms including protozoa, bacteria, yeast, viruses, etc."; 318 case PLNT: 319 return "A living subject from the order of plants."; 320 case PSN: 321 return "A living subject of the species homo sapiens."; 322 case MAT: 323 return "Any thing that has extension in space and mass, may be of living or non-living origin."; 324 case CHEM: 325 return "A substance that is fully defined by an organic or inorganic chemical formula, includes mixtures of other chemical substances. Refine using, e.g., IUPAC codes."; 326 case FOOD: 327 return "Naturally occurring, processed or manufactured entities that are primarily used as food for humans and animals."; 328 case MMAT: 329 return "Corresponds to the ManufacturedMaterial class"; 330 case CONT: 331 return "A container of other entities."; 332 case HOLD: 333 return "A type of container that can hold other containers or other holders."; 334 case DEV: 335 return "A subtype of ManufacturedMaterial used in an activity, without being substantially changed through that activity. The kind of device is identified by the code attribute inherited from Entity.\r\n\n \n Usage: This includes durable (reusable) medical equipment as well as disposable equipment."; 336 case CER: 337 return "A physical artifact that stores information about the granting of authorization."; 338 case MODDV: 339 return "Class to contain unique attributes of diagnostic imaging equipment."; 340 case ORG: 341 return "A social or legal structure formed by human beings."; 342 case PUB: 343 return "An agency of the people of a state often assuming some authority over a certain matter. Includes government, governmental agencies, associations."; 344 case STATE: 345 return "A politically organized body of people bonded by territory, culture, or ethnicity, having sovereignty (to a certain extent) granted by other states (enclosing or neighboring states). This includes countries (nations), provinces (e.g., one of the United States of America or a French departement), counties or municipalities. Refine using, e.g., ISO country codes, FIPS-PUB state codes, etc."; 346 case NAT: 347 return "A politically organized body of people bonded by territory and known as a nation."; 348 case PLC: 349 return "A physical place or site with its containing structure. May be natural or man-made. The geographic position of a place may or may not be constant."; 350 case CITY: 351 return "The territory of a city, town or other municipality."; 352 case COUNTRY: 353 return "The territory of a sovereign nation."; 354 case COUNTY: 355 return "The territory of a county, parish or other division of a state or province."; 356 case PROVINCE: 357 return "The territory of a state, province, department or other division of a sovereign country."; 358 case RGRP: 359 return "A grouping of resources (personnel, material, or places) to be used for scheduling purposes. May be a pool of like-type resources, a team, or combination of personnel, material and places."; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 367 public String getDisplay() { 368 switch (this) { 369 case ENT: 370 return "entity"; 371 case HCE: 372 return "health chart entity"; 373 case LIV: 374 return "living subject"; 375 case NLIV: 376 return "non-person living subject"; 377 case ANM: 378 return "animal"; 379 case MIC: 380 return "microorganism"; 381 case PLNT: 382 return "plant"; 383 case PSN: 384 return "person"; 385 case MAT: 386 return "material"; 387 case CHEM: 388 return "chemical substance"; 389 case FOOD: 390 return "food"; 391 case MMAT: 392 return "manufactured material"; 393 case CONT: 394 return "container"; 395 case HOLD: 396 return "holder"; 397 case DEV: 398 return "device"; 399 case CER: 400 return "certificate representation"; 401 case MODDV: 402 return "imaging modality"; 403 case ORG: 404 return "organization"; 405 case PUB: 406 return "public institution"; 407 case STATE: 408 return "state"; 409 case NAT: 410 return "Nation"; 411 case PLC: 412 return "place"; 413 case CITY: 414 return "city or town"; 415 case COUNTRY: 416 return "country"; 417 case COUNTY: 418 return "county or parish"; 419 case PROVINCE: 420 return "state or province"; 421 case RGRP: 422 return "group"; 423 case NULL: 424 return null; 425 default: 426 return "?"; 427 } 428 } 429 430}