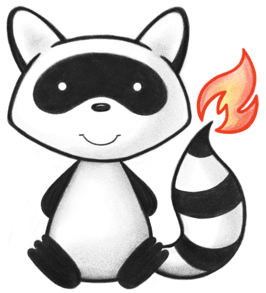
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityRisk { 037 038 /** 039 * A danger that can be associated with certain living subjects, including 040 * humans. 041 */ 042 AGG, 043 /** 044 * The dangers associated with normal biological materials. I.e. potential risk 045 * of unknown infections. Routine biological materials from living subjects. 046 */ 047 BIO, 048 /** 049 * Material is corrosive and may cause severe injury to skin, mucous membranes 050 * and eyes. Avoid any unprotected contact. 051 */ 052 COR, 053 /** 054 * The entity is at risk for escaping from containment or control. 055 */ 056 ESC, 057 /** 058 * Material is highly inflammable and in certain mixtures (with air) may lead to 059 * explosions. Keep away from fire, sparks and excessive heat. 060 */ 061 IFL, 062 /** 063 * Material is an explosive mixture. Keep away from fire, sparks, and heat. 064 */ 065 EXP, 066 /** 067 * Material known to be infectious with human pathogenic microorganisms. Those 068 * who handle this material must take precautions for their protection. 069 */ 070 INF, 071 /** 072 * Material contains microorganisms that is an environmental hazard. Must be 073 * handled with special care. 074 */ 075 BHZ, 076 /** 077 * Material is solid and sharp (e.g., cannulas). Dispose in hard container. 078 */ 079 INJ, 080 /** 081 * Material is poisonous to humans and/or animals. Special care must be taken to 082 * avoid incorporation, even of small amounts. 083 */ 084 POI, 085 /** 086 * Material is a source for ionizing radiation and must be handled with special 087 * care to avoid injury of those who handle it and to avoid environmental 088 * hazards. 089 */ 090 RAD, 091 /** 092 * added to help the parsers 093 */ 094 NULL; 095 096 public static V3EntityRisk fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("AGG".equals(codeString)) 100 return AGG; 101 if ("BIO".equals(codeString)) 102 return BIO; 103 if ("COR".equals(codeString)) 104 return COR; 105 if ("ESC".equals(codeString)) 106 return ESC; 107 if ("IFL".equals(codeString)) 108 return IFL; 109 if ("EXP".equals(codeString)) 110 return EXP; 111 if ("INF".equals(codeString)) 112 return INF; 113 if ("BHZ".equals(codeString)) 114 return BHZ; 115 if ("INJ".equals(codeString)) 116 return INJ; 117 if ("POI".equals(codeString)) 118 return POI; 119 if ("RAD".equals(codeString)) 120 return RAD; 121 throw new FHIRException("Unknown V3EntityRisk code '" + codeString + "'"); 122 } 123 124 public String toCode() { 125 switch (this) { 126 case AGG: 127 return "AGG"; 128 case BIO: 129 return "BIO"; 130 case COR: 131 return "COR"; 132 case ESC: 133 return "ESC"; 134 case IFL: 135 return "IFL"; 136 case EXP: 137 return "EXP"; 138 case INF: 139 return "INF"; 140 case BHZ: 141 return "BHZ"; 142 case INJ: 143 return "INJ"; 144 case POI: 145 return "POI"; 146 case RAD: 147 return "RAD"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getSystem() { 156 return "http://terminology.hl7.org/CodeSystem/v3-EntityRisk"; 157 } 158 159 public String getDefinition() { 160 switch (this) { 161 case AGG: 162 return "A danger that can be associated with certain living subjects, including humans."; 163 case BIO: 164 return "The dangers associated with normal biological materials. I.e. potential risk of unknown infections. Routine biological materials from living subjects."; 165 case COR: 166 return "Material is corrosive and may cause severe injury to skin, mucous membranes and eyes. Avoid any unprotected contact."; 167 case ESC: 168 return "The entity is at risk for escaping from containment or control."; 169 case IFL: 170 return "Material is highly inflammable and in certain mixtures (with air) may lead to explosions. Keep away from fire, sparks and excessive heat."; 171 case EXP: 172 return "Material is an explosive mixture. Keep away from fire, sparks, and heat."; 173 case INF: 174 return "Material known to be infectious with human pathogenic microorganisms. Those who handle this material must take precautions for their protection."; 175 case BHZ: 176 return "Material contains microorganisms that is an environmental hazard. Must be handled with special care."; 177 case INJ: 178 return "Material is solid and sharp (e.g., cannulas). Dispose in hard container."; 179 case POI: 180 return "Material is poisonous to humans and/or animals. Special care must be taken to avoid incorporation, even of small amounts."; 181 case RAD: 182 return "Material is a source for ionizing radiation and must be handled with special care to avoid injury of those who handle it and to avoid environmental hazards."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case AGG: 193 return "aggressive"; 194 case BIO: 195 return "Biological"; 196 case COR: 197 return "Corrosive"; 198 case ESC: 199 return "Escape Risk"; 200 case IFL: 201 return "inflammable"; 202 case EXP: 203 return "explosive"; 204 case INF: 205 return "infectious"; 206 case BHZ: 207 return "biohazard"; 208 case INJ: 209 return "injury hazard"; 210 case POI: 211 return "poison"; 212 case RAD: 213 return "radioactive"; 214 case NULL: 215 return null; 216 default: 217 return "?"; 218 } 219 } 220 221}