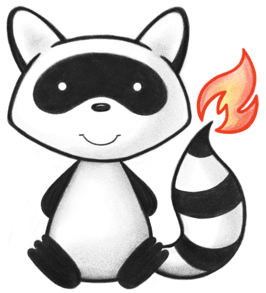
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3EntityStatus { 037 038 /** 039 * The 'typical' state. Excludes "nullified" which represents the termination 040 * state of an Entity record instance that was created in error. 041 */ 042 NORMAL, 043 /** 044 * The state representing the fact that the Entity record is currently active. 045 */ 046 ACTIVE, 047 /** 048 * Definition: The state representing the fact that the entity is inactive. 049 */ 050 INACTIVE, 051 /** 052 * The state representing the normal termination of an Entity record. 053 */ 054 TERMINATED, 055 /** 056 * The state representing the termination of an Entity record instance that was 057 * created in error. 058 */ 059 NULLIFIED, 060 /** 061 * added to help the parsers 062 */ 063 NULL; 064 065 public static V3EntityStatus fromCode(String codeString) throws FHIRException { 066 if (codeString == null || "".equals(codeString)) 067 return null; 068 if ("normal".equals(codeString)) 069 return NORMAL; 070 if ("active".equals(codeString)) 071 return ACTIVE; 072 if ("inactive".equals(codeString)) 073 return INACTIVE; 074 if ("terminated".equals(codeString)) 075 return TERMINATED; 076 if ("nullified".equals(codeString)) 077 return NULLIFIED; 078 throw new FHIRException("Unknown V3EntityStatus code '" + codeString + "'"); 079 } 080 081 public String toCode() { 082 switch (this) { 083 case NORMAL: 084 return "normal"; 085 case ACTIVE: 086 return "active"; 087 case INACTIVE: 088 return "inactive"; 089 case TERMINATED: 090 return "terminated"; 091 case NULLIFIED: 092 return "nullified"; 093 case NULL: 094 return null; 095 default: 096 return "?"; 097 } 098 } 099 100 public String getSystem() { 101 return "http://terminology.hl7.org/CodeSystem/v3-EntityStatus"; 102 } 103 104 public String getDefinition() { 105 switch (this) { 106 case NORMAL: 107 return "The 'typical' state. Excludes \"nullified\" which represents the termination state of an Entity record instance that was created in error."; 108 case ACTIVE: 109 return "The state representing the fact that the Entity record is currently active."; 110 case INACTIVE: 111 return "Definition: The state representing the fact that the entity is inactive."; 112 case TERMINATED: 113 return "The state representing the normal termination of an Entity record."; 114 case NULLIFIED: 115 return "The state representing the termination of an Entity record instance that was created in error."; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDisplay() { 124 switch (this) { 125 case NORMAL: 126 return "normal"; 127 case ACTIVE: 128 return "active"; 129 case INACTIVE: 130 return "inactive"; 131 case TERMINATED: 132 return "terminated"; 133 case NULLIFIED: 134 return "nullified"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142}