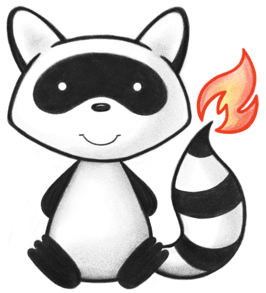
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3GTSAbbreviation { 037 038 /** 039 * Every morning at institution specified times. 040 */ 041 AM, 042 /** 043 * At bedtime (institution specified time). 044 */ 045 BED, 046 /** 047 * Two times a day at institution specified time 048 */ 049 BID, 050 /** 051 * Regular business days (Monday to Friday excluding holidays) 052 */ 053 JB, 054 /** 055 * Regular weekends (Saturday and Sunday excluding holidays) 056 */ 057 JE, 058 /** 059 * Holidays 060 */ 061 JH, 062 /** 063 * Christian Holidays (Roman/Gregorian [Western] Tradition.) 064 */ 065 _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN, 066 /** 067 * Easter Sunday. The Easter date is a rather complex calculation based on 068 * Astronomical tables describing full moon dates. Details can be found at 069 * [http://www.assa.org.au/edm.html, and 070 * http://aa.usno.navy.mil/AA/faq/docs/easter.html]. Note that the Christian 071 * Orthodox Holidays are based on the Julian calendar. 072 */ 073 JHCHREAS, 074 /** 075 * Good Friday, is the Friday right before Easter Sunday. 076 */ 077 JHCHRGFR, 078 /** 079 * New Year's Day (January 1) 080 */ 081 JHCHRNEW, 082 /** 083 * Pentecost Sunday, is seven weeks after Easter (the 50th day of Easter). 084 */ 085 JHCHRPEN, 086 /** 087 * Christmas Eve (December 24) 088 */ 089 JHCHRXME, 090 /** 091 * Christmas Day (December 25) 092 */ 093 JHCHRXMS, 094 /** 095 * Description:The Netherlands National Holidays. 096 */ 097 JHNNL, 098 /** 099 * Description:Liberation day (May 5 every five years) 100 */ 101 JHNNLLD, 102 /** 103 * Description:Queen's day (April 30) 104 */ 105 JHNNLQD, 106 /** 107 * Description:Sinterklaas (December 5) 108 */ 109 JHNNLSK, 110 /** 111 * United States National Holidays (public holidays for federal employees 112 * established by U.S. Federal law 5 U.S.C. 6103). 113 */ 114 JHNUS, 115 /** 116 * Columbus Day, the second Monday in October. 117 */ 118 JHNUSCLM, 119 /** 120 * Independence Day (4th of July) 121 */ 122 JHNUSIND, 123 /** 124 * Alternative Monday after 4th of July Weekend [5 U.S.C. 6103(b)]. 125 */ 126 JHNUSIND1, 127 /** 128 * Alternative Friday before 4th of July Weekend [5 U.S.C. 6103(b)]. 129 */ 130 JHNUSIND5, 131 /** 132 * Labor Day, the first Monday in September. 133 */ 134 JHNUSLBR, 135 /** 136 * Memorial Day, the last Monday in May. 137 */ 138 JHNUSMEM, 139 /** 140 * Friday before Memorial Day Weekend 141 */ 142 JHNUSMEM5, 143 /** 144 * Saturday of Memorial Day Weekend 145 */ 146 JHNUSMEM6, 147 /** 148 * Dr. Martin Luther King, Jr. Day, the third Monday in January. 149 */ 150 JHNUSMLK, 151 /** 152 * Washington's Birthday (Presidential Day) the third Monday in February. 153 */ 154 JHNUSPRE, 155 /** 156 * Thanksgiving Day, the fourth Thursday in November. 157 */ 158 JHNUSTKS, 159 /** 160 * Friday after Thanksgiving. 161 */ 162 JHNUSTKS5, 163 /** 164 * Veteran's Day, November 11. 165 */ 166 JHNUSVET, 167 /** 168 * Monthly at institution specified time. 169 */ 170 MO, 171 /** 172 * Every afternoon at institution specified times. 173 */ 174 PM, 175 /** 176 * Every hour at institution specified times. 177 */ 178 Q1H, 179 /** 180 * Every 2 hours at institution specified times. 181 */ 182 Q2H, 183 /** 184 * Every 3 hours at institution specified times. 185 */ 186 Q3H, 187 /** 188 * Every 4 hours at institution specified time 189 */ 190 Q4H, 191 /** 192 * Every 6 hours at institution specified time 193 */ 194 Q6H, 195 /** 196 * Every 8 hours at institution specified times. 197 */ 198 Q8H, 199 /** 200 * Every day at institution specified times. 201 */ 202 QD, 203 /** 204 * Four times a day at institution specified time 205 */ 206 QID, 207 /** 208 * Every other day at institution specified times. 209 */ 210 QOD, 211 /** 212 * Three times a day at institution specified time 213 */ 214 TID, 215 /** 216 * Weekly at institution specified time. 217 */ 218 WK, 219 /** 220 * added to help the parsers 221 */ 222 NULL; 223 224 public static V3GTSAbbreviation fromCode(String codeString) throws FHIRException { 225 if (codeString == null || "".equals(codeString)) 226 return null; 227 if ("AM".equals(codeString)) 228 return AM; 229 if ("BED".equals(codeString)) 230 return BED; 231 if ("BID".equals(codeString)) 232 return BID; 233 if ("JB".equals(codeString)) 234 return JB; 235 if ("JE".equals(codeString)) 236 return JE; 237 if ("JH".equals(codeString)) 238 return JH; 239 if ("_GTSAbbreviationHolidaysChristianRoman".equals(codeString)) 240 return _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN; 241 if ("JHCHREAS".equals(codeString)) 242 return JHCHREAS; 243 if ("JHCHRGFR".equals(codeString)) 244 return JHCHRGFR; 245 if ("JHCHRNEW".equals(codeString)) 246 return JHCHRNEW; 247 if ("JHCHRPEN".equals(codeString)) 248 return JHCHRPEN; 249 if ("JHCHRXME".equals(codeString)) 250 return JHCHRXME; 251 if ("JHCHRXMS".equals(codeString)) 252 return JHCHRXMS; 253 if ("JHNNL".equals(codeString)) 254 return JHNNL; 255 if ("JHNNLLD".equals(codeString)) 256 return JHNNLLD; 257 if ("JHNNLQD".equals(codeString)) 258 return JHNNLQD; 259 if ("JHNNLSK".equals(codeString)) 260 return JHNNLSK; 261 if ("JHNUS".equals(codeString)) 262 return JHNUS; 263 if ("JHNUSCLM".equals(codeString)) 264 return JHNUSCLM; 265 if ("JHNUSIND".equals(codeString)) 266 return JHNUSIND; 267 if ("JHNUSIND1".equals(codeString)) 268 return JHNUSIND1; 269 if ("JHNUSIND5".equals(codeString)) 270 return JHNUSIND5; 271 if ("JHNUSLBR".equals(codeString)) 272 return JHNUSLBR; 273 if ("JHNUSMEM".equals(codeString)) 274 return JHNUSMEM; 275 if ("JHNUSMEM5".equals(codeString)) 276 return JHNUSMEM5; 277 if ("JHNUSMEM6".equals(codeString)) 278 return JHNUSMEM6; 279 if ("JHNUSMLK".equals(codeString)) 280 return JHNUSMLK; 281 if ("JHNUSPRE".equals(codeString)) 282 return JHNUSPRE; 283 if ("JHNUSTKS".equals(codeString)) 284 return JHNUSTKS; 285 if ("JHNUSTKS5".equals(codeString)) 286 return JHNUSTKS5; 287 if ("JHNUSVET".equals(codeString)) 288 return JHNUSVET; 289 if ("MO".equals(codeString)) 290 return MO; 291 if ("PM".equals(codeString)) 292 return PM; 293 if ("Q1H".equals(codeString)) 294 return Q1H; 295 if ("Q2H".equals(codeString)) 296 return Q2H; 297 if ("Q3H".equals(codeString)) 298 return Q3H; 299 if ("Q4H".equals(codeString)) 300 return Q4H; 301 if ("Q6H".equals(codeString)) 302 return Q6H; 303 if ("Q8H".equals(codeString)) 304 return Q8H; 305 if ("QD".equals(codeString)) 306 return QD; 307 if ("QID".equals(codeString)) 308 return QID; 309 if ("QOD".equals(codeString)) 310 return QOD; 311 if ("TID".equals(codeString)) 312 return TID; 313 if ("WK".equals(codeString)) 314 return WK; 315 throw new FHIRException("Unknown V3GTSAbbreviation code '" + codeString + "'"); 316 } 317 318 public String toCode() { 319 switch (this) { 320 case AM: 321 return "AM"; 322 case BED: 323 return "BED"; 324 case BID: 325 return "BID"; 326 case JB: 327 return "JB"; 328 case JE: 329 return "JE"; 330 case JH: 331 return "JH"; 332 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: 333 return "_GTSAbbreviationHolidaysChristianRoman"; 334 case JHCHREAS: 335 return "JHCHREAS"; 336 case JHCHRGFR: 337 return "JHCHRGFR"; 338 case JHCHRNEW: 339 return "JHCHRNEW"; 340 case JHCHRPEN: 341 return "JHCHRPEN"; 342 case JHCHRXME: 343 return "JHCHRXME"; 344 case JHCHRXMS: 345 return "JHCHRXMS"; 346 case JHNNL: 347 return "JHNNL"; 348 case JHNNLLD: 349 return "JHNNLLD"; 350 case JHNNLQD: 351 return "JHNNLQD"; 352 case JHNNLSK: 353 return "JHNNLSK"; 354 case JHNUS: 355 return "JHNUS"; 356 case JHNUSCLM: 357 return "JHNUSCLM"; 358 case JHNUSIND: 359 return "JHNUSIND"; 360 case JHNUSIND1: 361 return "JHNUSIND1"; 362 case JHNUSIND5: 363 return "JHNUSIND5"; 364 case JHNUSLBR: 365 return "JHNUSLBR"; 366 case JHNUSMEM: 367 return "JHNUSMEM"; 368 case JHNUSMEM5: 369 return "JHNUSMEM5"; 370 case JHNUSMEM6: 371 return "JHNUSMEM6"; 372 case JHNUSMLK: 373 return "JHNUSMLK"; 374 case JHNUSPRE: 375 return "JHNUSPRE"; 376 case JHNUSTKS: 377 return "JHNUSTKS"; 378 case JHNUSTKS5: 379 return "JHNUSTKS5"; 380 case JHNUSVET: 381 return "JHNUSVET"; 382 case MO: 383 return "MO"; 384 case PM: 385 return "PM"; 386 case Q1H: 387 return "Q1H"; 388 case Q2H: 389 return "Q2H"; 390 case Q3H: 391 return "Q3H"; 392 case Q4H: 393 return "Q4H"; 394 case Q6H: 395 return "Q6H"; 396 case Q8H: 397 return "Q8H"; 398 case QD: 399 return "QD"; 400 case QID: 401 return "QID"; 402 case QOD: 403 return "QOD"; 404 case TID: 405 return "TID"; 406 case WK: 407 return "WK"; 408 case NULL: 409 return null; 410 default: 411 return "?"; 412 } 413 } 414 415 public String getSystem() { 416 return "http://terminology.hl7.org/CodeSystem/v3-GTSAbbreviation"; 417 } 418 419 public String getDefinition() { 420 switch (this) { 421 case AM: 422 return "Every morning at institution specified times."; 423 case BED: 424 return "At bedtime (institution specified time)."; 425 case BID: 426 return "Two times a day at institution specified time"; 427 case JB: 428 return "Regular business days (Monday to Friday excluding holidays)"; 429 case JE: 430 return "Regular weekends (Saturday and Sunday excluding holidays)"; 431 case JH: 432 return "Holidays"; 433 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: 434 return "Christian Holidays (Roman/Gregorian [Western] Tradition.)"; 435 case JHCHREAS: 436 return "Easter Sunday. The Easter date is a rather complex calculation based on Astronomical tables describing full moon dates. Details can be found at [http://www.assa.org.au/edm.html, and http://aa.usno.navy.mil/AA/faq/docs/easter.html]. Note that the Christian Orthodox Holidays are based on the Julian calendar."; 437 case JHCHRGFR: 438 return "Good Friday, is the Friday right before Easter Sunday."; 439 case JHCHRNEW: 440 return "New Year's Day (January 1)"; 441 case JHCHRPEN: 442 return "Pentecost Sunday, is seven weeks after Easter (the 50th day of Easter)."; 443 case JHCHRXME: 444 return "Christmas Eve (December 24)"; 445 case JHCHRXMS: 446 return "Christmas Day (December 25)"; 447 case JHNNL: 448 return "Description:The Netherlands National Holidays."; 449 case JHNNLLD: 450 return "Description:Liberation day (May 5 every five years)"; 451 case JHNNLQD: 452 return "Description:Queen's day (April 30)"; 453 case JHNNLSK: 454 return "Description:Sinterklaas (December 5)"; 455 case JHNUS: 456 return "United States National Holidays (public holidays for federal employees established by U.S. Federal law 5 U.S.C. 6103)."; 457 case JHNUSCLM: 458 return "Columbus Day, the second Monday in October."; 459 case JHNUSIND: 460 return "Independence Day (4th of July)"; 461 case JHNUSIND1: 462 return "Alternative Monday after 4th of July Weekend [5 U.S.C. 6103(b)]."; 463 case JHNUSIND5: 464 return "Alternative Friday before 4th of July Weekend [5 U.S.C. 6103(b)]."; 465 case JHNUSLBR: 466 return "Labor Day, the first Monday in September."; 467 case JHNUSMEM: 468 return "Memorial Day, the last Monday in May."; 469 case JHNUSMEM5: 470 return "Friday before Memorial Day Weekend"; 471 case JHNUSMEM6: 472 return "Saturday of Memorial Day Weekend"; 473 case JHNUSMLK: 474 return "Dr. Martin Luther King, Jr. Day, the third Monday in January."; 475 case JHNUSPRE: 476 return "Washington's Birthday (Presidential Day) the third Monday in February."; 477 case JHNUSTKS: 478 return "Thanksgiving Day, the fourth Thursday in November."; 479 case JHNUSTKS5: 480 return "Friday after Thanksgiving."; 481 case JHNUSVET: 482 return "Veteran's Day, November 11."; 483 case MO: 484 return "Monthly at institution specified time."; 485 case PM: 486 return "Every afternoon at institution specified times."; 487 case Q1H: 488 return "Every hour at institution specified times."; 489 case Q2H: 490 return "Every 2 hours at institution specified times."; 491 case Q3H: 492 return "Every 3 hours at institution specified times."; 493 case Q4H: 494 return "Every 4 hours at institution specified time"; 495 case Q6H: 496 return "Every 6 hours at institution specified time"; 497 case Q8H: 498 return "Every 8 hours at institution specified times."; 499 case QD: 500 return "Every day at institution specified times."; 501 case QID: 502 return "Four times a day at institution specified time"; 503 case QOD: 504 return "Every other day at institution specified times."; 505 case TID: 506 return "Three times a day at institution specified time"; 507 case WK: 508 return "Weekly at institution specified time."; 509 case NULL: 510 return null; 511 default: 512 return "?"; 513 } 514 } 515 516 public String getDisplay() { 517 switch (this) { 518 case AM: 519 return "AM"; 520 case BED: 521 return "at bedtime"; 522 case BID: 523 return "BID"; 524 case JB: 525 return "JB"; 526 case JE: 527 return "JE"; 528 case JH: 529 return "GTSAbbreviationHolidays"; 530 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: 531 return "GTSAbbreviationHolidaysChristianRoman"; 532 case JHCHREAS: 533 return "JHCHREAS"; 534 case JHCHRGFR: 535 return "JHCHRGFR"; 536 case JHCHRNEW: 537 return "JHCHRNEW"; 538 case JHCHRPEN: 539 return "JHCHRPEN"; 540 case JHCHRXME: 541 return "JHCHRXME"; 542 case JHCHRXMS: 543 return "JHCHRXMS"; 544 case JHNNL: 545 return "The Netherlands National Holidays"; 546 case JHNNLLD: 547 return "Liberation day (May 5 every five years)"; 548 case JHNNLQD: 549 return "Queen's day (April 30)"; 550 case JHNNLSK: 551 return "Sinterklaas (December 5)"; 552 case JHNUS: 553 return "GTSAbbreviationHolidaysUSNational"; 554 case JHNUSCLM: 555 return "JHNUSCLM"; 556 case JHNUSIND: 557 return "JHNUSIND"; 558 case JHNUSIND1: 559 return "JHNUSIND1"; 560 case JHNUSIND5: 561 return "JHNUSIND5"; 562 case JHNUSLBR: 563 return "JHNUSLBR"; 564 case JHNUSMEM: 565 return "JHNUSMEM"; 566 case JHNUSMEM5: 567 return "JHNUSMEM5"; 568 case JHNUSMEM6: 569 return "JHNUSMEM6"; 570 case JHNUSMLK: 571 return "JHNUSMLK"; 572 case JHNUSPRE: 573 return "JHNUSPRE"; 574 case JHNUSTKS: 575 return "JHNUSTKS"; 576 case JHNUSTKS5: 577 return "JHNUSTKS5"; 578 case JHNUSVET: 579 return "JHNUSVET"; 580 case MO: 581 return "monthly"; 582 case PM: 583 return "PM"; 584 case Q1H: 585 return "every hour"; 586 case Q2H: 587 return "every 2 hours"; 588 case Q3H: 589 return "every 3 hours"; 590 case Q4H: 591 return "Q4H"; 592 case Q6H: 593 return "Q6H"; 594 case Q8H: 595 return "every 8 hours"; 596 case QD: 597 return "QD"; 598 case QID: 599 return "QID"; 600 case QOD: 601 return "QOD"; 602 case TID: 603 return "TID"; 604 case WK: 605 return "weekly"; 606 case NULL: 607 return null; 608 default: 609 return "?"; 610 } 611 } 612 613}