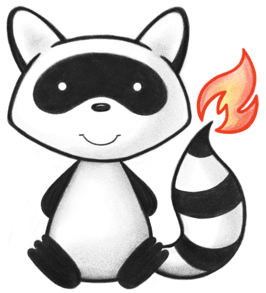
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3HtmlLinkType { 037 038 /** 039 * Designates substitute versions for the document in which the link occurs. 040 * When used together with the lang attribute, it implies a translated version 041 * of the document. When used together with the media attribute, it implies a 042 * version designed for a different medium (or media). 043 */ 044 ALTERNATE, 045 /** 046 * Refers to a document serving as an appendix in a collection of documents. 047 */ 048 APPENDIX, 049 /** 050 * Refers to a bookmark. A bookmark is a link to a key entry point within an 051 * extended document. The title attribute may be used, for example, to label the 052 * bookmark. Note that several bookmarks may be defined in each document. 053 */ 054 BOOKMARK, 055 /** 056 * Refers to a document serving as a chapter in a collection of documents. 057 */ 058 CHAPTER, 059 /** 060 * Refers to a document serving as a table of contents. Some user agents also 061 * support the synonym ToC (from "Table of Contents"). 062 */ 063 CONTENTS, 064 /** 065 * Refers to a copyright statement for the current document. 066 */ 067 COPYRIGHT, 068 /** 069 * Refers to a document providing a glossary of terms that pertain to the 070 * current document. 071 */ 072 GLOSSARY, 073 /** 074 * Refers to a document offering help (more information, links to other sources 075 * of information, etc.). 076 */ 077 HELP, 078 /** 079 * Refers to a document providing an index for the current document. 080 */ 081 INDEX, 082 /** 083 * Refers to the next document in a linear sequence of documents. User agents 084 * may choose to preload the "next" document, to reduce the perceived load time. 085 */ 086 NEXT, 087 /** 088 * Refers to the previous document in an ordered series of documents. Some user 089 * agents also support the synonym "Previous". 090 */ 091 PREV, 092 /** 093 * Refers to a document serving as a section in a collection of documents. 094 */ 095 SECTION, 096 /** 097 * Refers to the first document in a collection of documents. This link type 098 * tells search engines which document is considered by the author to be the 099 * starting point of the collection. 100 */ 101 START, 102 /** 103 * Refers to an external style sheet. See the section on external style sheets 104 * for details. This is used together with the link type "Alternate" for 105 * user-selectable alternate style sheets. 106 */ 107 STYLESHEET, 108 /** 109 * Refers to a document serving as a subsection in a collection of documents. 110 */ 111 SUBSECTION, 112 /** 113 * added to help the parsers 114 */ 115 NULL; 116 117 public static V3HtmlLinkType fromCode(String codeString) throws FHIRException { 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("alternate".equals(codeString)) 121 return ALTERNATE; 122 if ("appendix".equals(codeString)) 123 return APPENDIX; 124 if ("bookmark".equals(codeString)) 125 return BOOKMARK; 126 if ("chapter".equals(codeString)) 127 return CHAPTER; 128 if ("contents".equals(codeString)) 129 return CONTENTS; 130 if ("copyright".equals(codeString)) 131 return COPYRIGHT; 132 if ("glossary".equals(codeString)) 133 return GLOSSARY; 134 if ("help".equals(codeString)) 135 return HELP; 136 if ("index".equals(codeString)) 137 return INDEX; 138 if ("next".equals(codeString)) 139 return NEXT; 140 if ("prev".equals(codeString)) 141 return PREV; 142 if ("section".equals(codeString)) 143 return SECTION; 144 if ("start".equals(codeString)) 145 return START; 146 if ("stylesheet".equals(codeString)) 147 return STYLESHEET; 148 if ("subsection".equals(codeString)) 149 return SUBSECTION; 150 throw new FHIRException("Unknown V3HtmlLinkType code '" + codeString + "'"); 151 } 152 153 public String toCode() { 154 switch (this) { 155 case ALTERNATE: 156 return "alternate"; 157 case APPENDIX: 158 return "appendix"; 159 case BOOKMARK: 160 return "bookmark"; 161 case CHAPTER: 162 return "chapter"; 163 case CONTENTS: 164 return "contents"; 165 case COPYRIGHT: 166 return "copyright"; 167 case GLOSSARY: 168 return "glossary"; 169 case HELP: 170 return "help"; 171 case INDEX: 172 return "index"; 173 case NEXT: 174 return "next"; 175 case PREV: 176 return "prev"; 177 case SECTION: 178 return "section"; 179 case START: 180 return "start"; 181 case STYLESHEET: 182 return "stylesheet"; 183 case SUBSECTION: 184 return "subsection"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 192 public String getSystem() { 193 return "http://terminology.hl7.org/CodeSystem/v3-HtmlLinkType"; 194 } 195 196 public String getDefinition() { 197 switch (this) { 198 case ALTERNATE: 199 return "Designates substitute versions for the document in which the link occurs. When used together with the lang attribute, it implies a translated version of the document. When used together with the media attribute, it implies a version designed for a different medium (or media)."; 200 case APPENDIX: 201 return "Refers to a document serving as an appendix in a collection of documents."; 202 case BOOKMARK: 203 return "Refers to a bookmark. A bookmark is a link to a key entry point within an extended document. The title attribute may be used, for example, to label the bookmark. Note that several bookmarks may be defined in each document."; 204 case CHAPTER: 205 return "Refers to a document serving as a chapter in a collection of documents."; 206 case CONTENTS: 207 return "Refers to a document serving as a table of contents. Some user agents also support the synonym ToC (from \"Table of Contents\")."; 208 case COPYRIGHT: 209 return "Refers to a copyright statement for the current document."; 210 case GLOSSARY: 211 return "Refers to a document providing a glossary of terms that pertain to the current document."; 212 case HELP: 213 return "Refers to a document offering help (more information, links to other sources of information, etc.)."; 214 case INDEX: 215 return "Refers to a document providing an index for the current document."; 216 case NEXT: 217 return "Refers to the next document in a linear sequence of documents. User agents may choose to preload the \"next\" document, to reduce the perceived load time."; 218 case PREV: 219 return "Refers to the previous document in an ordered series of documents. Some user agents also support the synonym \"Previous\"."; 220 case SECTION: 221 return "Refers to a document serving as a section in a collection of documents."; 222 case START: 223 return "Refers to the first document in a collection of documents. This link type tells search engines which document is considered by the author to be the starting point of the collection."; 224 case STYLESHEET: 225 return "Refers to an external style sheet. See the section on external style sheets for details. This is used together with the link type \"Alternate\" for user-selectable alternate style sheets."; 226 case SUBSECTION: 227 return "Refers to a document serving as a subsection in a collection of documents."; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 235 public String getDisplay() { 236 switch (this) { 237 case ALTERNATE: 238 return "alternate"; 239 case APPENDIX: 240 return "appendix"; 241 case BOOKMARK: 242 return "bookmark"; 243 case CHAPTER: 244 return "chapter"; 245 case CONTENTS: 246 return "contents"; 247 case COPYRIGHT: 248 return "copyright"; 249 case GLOSSARY: 250 return "glossary"; 251 case HELP: 252 return "help"; 253 case INDEX: 254 return "index"; 255 case NEXT: 256 return "next"; 257 case PREV: 258 return "prev"; 259 case SECTION: 260 return "section"; 261 case START: 262 return "start"; 263 case STYLESHEET: 264 return "stylesheet"; 265 case SUBSECTION: 266 return "subsection"; 267 case NULL: 268 return null; 269 default: 270 return "?"; 271 } 272 } 273 274}