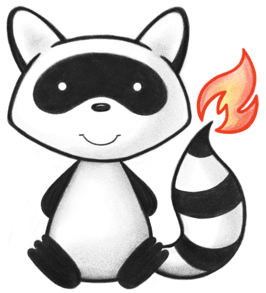
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3IntegrityCheckAlgorithm { 037 038 /** 039 * This algorithm is defined in FIPS PUB 180-1: Secure Hash Standard. As of 040 * April 17, 1995. 041 */ 042 SHA1, 043 /** 044 * This algorithm is defined in FIPS PUB 180-2: Secure Hash Standard. 045 */ 046 SHA256, 047 /** 048 * added to help the parsers 049 */ 050 NULL; 051 052 public static V3IntegrityCheckAlgorithm fromCode(String codeString) throws FHIRException { 053 if (codeString == null || "".equals(codeString)) 054 return null; 055 if ("SHA-1".equals(codeString)) 056 return SHA1; 057 if ("SHA-256".equals(codeString)) 058 return SHA256; 059 throw new FHIRException("Unknown V3IntegrityCheckAlgorithm code '" + codeString + "'"); 060 } 061 062 public String toCode() { 063 switch (this) { 064 case SHA1: 065 return "SHA-1"; 066 case SHA256: 067 return "SHA-256"; 068 case NULL: 069 return null; 070 default: 071 return "?"; 072 } 073 } 074 075 public String getSystem() { 076 return "http://terminology.hl7.org/CodeSystem/v3-IntegrityCheckAlgorithm"; 077 } 078 079 public String getDefinition() { 080 switch (this) { 081 case SHA1: 082 return "This algorithm is defined in FIPS PUB 180-1: Secure Hash Standard. As of April 17, 1995."; 083 case SHA256: 084 return "This algorithm is defined in FIPS PUB 180-2: Secure Hash Standard."; 085 case NULL: 086 return null; 087 default: 088 return "?"; 089 } 090 } 091 092 public String getDisplay() { 093 switch (this) { 094 case SHA1: 095 return "secure hash algorithm - 1"; 096 case SHA256: 097 return "secure hash algorithm - 256"; 098 case NULL: 099 return null; 100 default: 101 return "?"; 102 } 103 } 104 105}