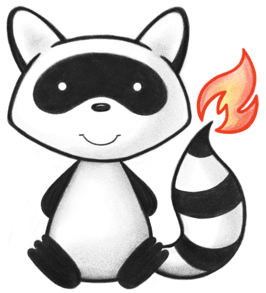
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3LivingArrangement { 037 038 /** 039 * Definition: Living arrangements lacking a permanent residence. 040 */ 041 HL, 042 /** 043 * Nomadic 044 */ 045 M, 046 /** 047 * Transient 048 */ 049 T, 050 /** 051 * Institution 052 */ 053 I, 054 /** 055 * Definition: A group living arrangement specifically for the care of those in 056 * need of temporary and crisis housing assistance. Examples include domestic 057 * violence shelters, shelters for displaced or homeless individuals, Salvation 058 * Army, Jesus House, etc. Community based services may be provided. 059 */ 060 CS, 061 /** 062 * Group Home 063 */ 064 G, 065 /** 066 * Nursing Home 067 */ 068 N, 069 /** 070 * Extended care facility 071 */ 072 X, 073 /** 074 * Definition: A living arrangement within a private residence for single 075 * family. 076 */ 077 PR, 078 /** 079 * Independent Household 080 */ 081 H, 082 /** 083 * Retirement Community 084 */ 085 R, 086 /** 087 * Definition: Assisted living in a single family residence for persons with 088 * physical, behavioral, or functional health, or socio-economic challenges. 089 * There may or may not be on-site supervision but the housing is designed to 090 * assist the client with developing independent living skills. Community based 091 * services may be provided. 092 */ 093 SL, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static V3LivingArrangement fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("HL".equals(codeString)) 103 return HL; 104 if ("M".equals(codeString)) 105 return M; 106 if ("T".equals(codeString)) 107 return T; 108 if ("I".equals(codeString)) 109 return I; 110 if ("CS".equals(codeString)) 111 return CS; 112 if ("G".equals(codeString)) 113 return G; 114 if ("N".equals(codeString)) 115 return N; 116 if ("X".equals(codeString)) 117 return X; 118 if ("PR".equals(codeString)) 119 return PR; 120 if ("H".equals(codeString)) 121 return H; 122 if ("R".equals(codeString)) 123 return R; 124 if ("SL".equals(codeString)) 125 return SL; 126 throw new FHIRException("Unknown V3LivingArrangement code '" + codeString + "'"); 127 } 128 129 public String toCode() { 130 switch (this) { 131 case HL: 132 return "HL"; 133 case M: 134 return "M"; 135 case T: 136 return "T"; 137 case I: 138 return "I"; 139 case CS: 140 return "CS"; 141 case G: 142 return "G"; 143 case N: 144 return "N"; 145 case X: 146 return "X"; 147 case PR: 148 return "PR"; 149 case H: 150 return "H"; 151 case R: 152 return "R"; 153 case SL: 154 return "SL"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getSystem() { 163 return "http://terminology.hl7.org/CodeSystem/v3-LivingArrangement"; 164 } 165 166 public String getDefinition() { 167 switch (this) { 168 case HL: 169 return "Definition: Living arrangements lacking a permanent residence."; 170 case M: 171 return "Nomadic"; 172 case T: 173 return "Transient"; 174 case I: 175 return "Institution"; 176 case CS: 177 return "Definition: A group living arrangement specifically for the care of those in need of temporary and crisis housing assistance. Examples include domestic violence shelters, shelters for displaced or homeless individuals, Salvation Army, Jesus House, etc. Community based services may be provided."; 178 case G: 179 return "Group Home"; 180 case N: 181 return "Nursing Home"; 182 case X: 183 return "Extended care facility"; 184 case PR: 185 return "Definition: A living arrangement within a private residence for single family."; 186 case H: 187 return "Independent Household"; 188 case R: 189 return "Retirement Community"; 190 case SL: 191 return "Definition: Assisted living in a single family residence for persons with physical, behavioral, or functional health, or socio-economic challenges. There may or may not be on-site supervision but the housing is designed to assist the client with developing independent living skills. Community based services may be provided."; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 199 public String getDisplay() { 200 switch (this) { 201 case HL: 202 return "homeless"; 203 case M: 204 return "Nomadic"; 205 case T: 206 return "Transient"; 207 case I: 208 return "Institution"; 209 case CS: 210 return "community shelter"; 211 case G: 212 return "Group Home"; 213 case N: 214 return "Nursing Home"; 215 case X: 216 return "Extended care facility"; 217 case PR: 218 return "private residence"; 219 case H: 220 return "Independent Household"; 221 case R: 222 return "Retirement Community"; 223 case SL: 224 return "supported living"; 225 case NULL: 226 return null; 227 default: 228 return "?"; 229 } 230 } 231 232}