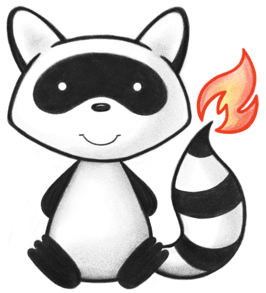
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ManagedParticipationStatus { 037 038 /** 039 * The 'typical' state. Excludes "nullified" which represents the termination 040 * state of a participation instance that was created in error. 041 */ 042 NORMAL, 043 /** 044 * The state representing the fact that the Participation is in progress. 045 */ 046 ACTIVE, 047 /** 048 * The terminal state resulting from cancellation of the Participation prior to 049 * activation. 050 */ 051 CANCELLED, 052 /** 053 * The terminal state representing the successful completion of the 054 * Participation. 055 */ 056 COMPLETED, 057 /** 058 * The state representing that fact that the Participation has not yet become 059 * active. 060 */ 061 PENDING, 062 /** 063 * The state representing the termination of a Participation instance that was 064 * created in error. 065 */ 066 NULLIFIED, 067 /** 068 * added to help the parsers 069 */ 070 NULL; 071 072 public static V3ManagedParticipationStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("normal".equals(codeString)) 076 return NORMAL; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("cancelled".equals(codeString)) 080 return CANCELLED; 081 if ("completed".equals(codeString)) 082 return COMPLETED; 083 if ("pending".equals(codeString)) 084 return PENDING; 085 if ("nullified".equals(codeString)) 086 return NULLIFIED; 087 throw new FHIRException("Unknown V3ManagedParticipationStatus code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case NORMAL: 093 return "normal"; 094 case ACTIVE: 095 return "active"; 096 case CANCELLED: 097 return "cancelled"; 098 case COMPLETED: 099 return "completed"; 100 case PENDING: 101 return "pending"; 102 case NULLIFIED: 103 return "nullified"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 return "http://terminology.hl7.org/CodeSystem/v3-ManagedParticipationStatus"; 113 } 114 115 public String getDefinition() { 116 switch (this) { 117 case NORMAL: 118 return "The 'typical' state. Excludes \"nullified\" which represents the termination state of a participation instance that was created in error."; 119 case ACTIVE: 120 return "The state representing the fact that the Participation is in progress."; 121 case CANCELLED: 122 return "The terminal state resulting from cancellation of the Participation prior to activation."; 123 case COMPLETED: 124 return "The terminal state representing the successful completion of the Participation."; 125 case PENDING: 126 return "The state representing that fact that the Participation has not yet become active."; 127 case NULLIFIED: 128 return "The state representing the termination of a Participation instance that was created in error."; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getDisplay() { 137 switch (this) { 138 case NORMAL: 139 return "normal"; 140 case ACTIVE: 141 return "active"; 142 case CANCELLED: 143 return "cancelled"; 144 case COMPLETED: 145 return "completed"; 146 case PENDING: 147 return "pending"; 148 case NULLIFIED: 149 return "nullified"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157}