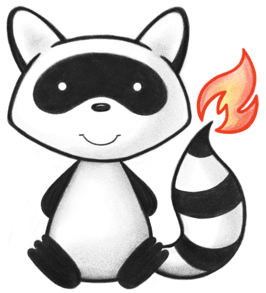
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3NullFlavor { 037 038 /** 039 * Description:The value is exceptional (missing, omitted, incomplete, 040 * improper). No information as to the reason for being an exceptional value is 041 * provided. This is the most general exceptional value. It is also the default 042 * exceptional value. 043 */ 044 NI, 045 /** 046 * Description:The value as represented in the instance is not a member of the 047 * set of permitted data values in the constrained value domain of a variable. 048 */ 049 INV, 050 /** 051 * Description:An actual value may exist, but it must be derived from the 052 * provided information (usually an EXPR generic data type extension will be 053 * used to convey the derivation expressionexpression . 054 */ 055 DER, 056 /** 057 * Description:The actual value is not a member of the set of permitted data 058 * values in the constrained value domain of a variable. (e.g., concept not 059 * provided by required code system). 060 * 061 * 062 * Usage Notes: This flavor and its specializations are most commonly used with 063 * the CD datatype and its flavors. However, it may apply to *any* datatype 064 * where the constraints of the type are tighter than can be conveyed. For 065 * example, a PQ that is for a true measured amount whose units are not 066 * supported in UCUM, a need to convey a REAL when the type has been constrained 067 * to INT, etc. 068 * 069 * With coded datatypes, this null flavor may only be used if the vocabulary 070 * binding has a coding strength of CNE. By definition, all local codes and 071 * original text are part of the value set if the coding strength is CWE. 072 */ 073 OTH, 074 /** 075 * Negative infinity of numbers. 076 */ 077 NINF, 078 /** 079 * Positive infinity of numbers. 080 */ 081 PINF, 082 /** 083 * Description: The actual value has not yet been encoded within the approved 084 * value domain. 085 * 086 * 087 * Example: Original text or a local code has been specified but translation or 088 * encoding to the approved value set has not yet occurred due to limitations of 089 * the sending system. Original text has been captured for a PQ, but not attempt 090 * has been made to split the value and unit or to encode the unit in UCUM. 091 * 092 * 093 * Usage Notes: If it is known that it is not possible to encode the concept, 094 * OTH should be used instead. However, use of UNC does not necessarily 095 * guarantee the concept will be encodable, only that encoding has not been 096 * attempted. 097 * 098 * Data type properties such as original text and translations may be present 099 * when this null flavor is included. 100 */ 101 UNC, 102 /** 103 * There is information on this item available but it has not been provided by 104 * the sender due to security, privacy or other reasons. There may be an 105 * alternate mechanism for gaining access to this information. 106 * 107 * Note: using this null flavor does provide information that may be a breach of 108 * confidentiality, even though no detail data is provided. Its primary purpose 109 * is for those circumstances where it is necessary to inform the receiver that 110 * the information does exist without providing any detail. 111 */ 112 MSK, 113 /** 114 * Known to have no proper value (e.g., last menstrual period for a male). 115 */ 116 NA, 117 /** 118 * Description:A proper value is applicable, but not known. 119 * 120 * 121 * Usage Notes: This means the actual value is not known. If the only thing that 122 * is unknown is how to properly express the value in the necessary constraints 123 * (value set, datatype, etc.), then the OTH or UNC flavor should be used. No 124 * properties should be included for a datatype with this property unless: 125 * 126 * 127 * Those properties themselves directly translate to a semantic of "unknown". 128 * (E.g. a local code sent as a translation that conveys 'unknown') Those 129 * properties further qualify the nature of what is unknown. (E.g. specifying a 130 * use code of "H" and a URL prefix of "tel:" to convey that it is the home 131 * phone number that is unknown.) 132 */ 133 UNK, 134 /** 135 * Information was sought but not found (e.g., patient was asked but didn't 136 * know) 137 */ 138 ASKU, 139 /** 140 * Information is not available at this time but it is expected that it will be 141 * available later. 142 */ 143 NAV, 144 /** 145 * This information has not been sought (e.g., patient was not asked) 146 */ 147 NASK, 148 /** 149 * Information is not available at this time (with no expectation regarding 150 * whether it will or will not be available in the future). 151 */ 152 NAVU, 153 /** 154 * Description:The specific quantity is not known, but is known to be non-zero 155 * and is not specified because it makes up the bulk of the material. e.g. 'Add 156 * 10mg of ingredient X, 50mg of ingredient Y, and sufficient quantity of water 157 * to 100mL.' The null flavor would be used to express the quantity of water. 158 */ 159 QS, 160 /** 161 * The content is greater than zero, but too small to be quantified. 162 */ 163 TRC, 164 /** 165 * Value is not present in a message. This is only defined in messages, never in 166 * application data! All values not present in the message must be replaced by 167 * the applicable default, or no-information (NI) as the default of all 168 * defaults. 169 */ 170 NP, 171 /** 172 * added to help the parsers 173 */ 174 NULL; 175 176 public static V3NullFlavor fromCode(String codeString) throws FHIRException { 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("NI".equals(codeString)) 180 return NI; 181 if ("INV".equals(codeString)) 182 return INV; 183 if ("DER".equals(codeString)) 184 return DER; 185 if ("OTH".equals(codeString)) 186 return OTH; 187 if ("NINF".equals(codeString)) 188 return NINF; 189 if ("PINF".equals(codeString)) 190 return PINF; 191 if ("UNC".equals(codeString)) 192 return UNC; 193 if ("MSK".equals(codeString)) 194 return MSK; 195 if ("NA".equals(codeString)) 196 return NA; 197 if ("UNK".equals(codeString)) 198 return UNK; 199 if ("ASKU".equals(codeString)) 200 return ASKU; 201 if ("NAV".equals(codeString)) 202 return NAV; 203 if ("NASK".equals(codeString)) 204 return NASK; 205 if ("NAVU".equals(codeString)) 206 return NAVU; 207 if ("QS".equals(codeString)) 208 return QS; 209 if ("TRC".equals(codeString)) 210 return TRC; 211 if ("NP".equals(codeString)) 212 return NP; 213 throw new FHIRException("Unknown V3NullFlavor code '" + codeString + "'"); 214 } 215 216 public String toCode() { 217 switch (this) { 218 case NI: 219 return "NI"; 220 case INV: 221 return "INV"; 222 case DER: 223 return "DER"; 224 case OTH: 225 return "OTH"; 226 case NINF: 227 return "NINF"; 228 case PINF: 229 return "PINF"; 230 case UNC: 231 return "UNC"; 232 case MSK: 233 return "MSK"; 234 case NA: 235 return "NA"; 236 case UNK: 237 return "UNK"; 238 case ASKU: 239 return "ASKU"; 240 case NAV: 241 return "NAV"; 242 case NASK: 243 return "NASK"; 244 case NAVU: 245 return "NAVU"; 246 case QS: 247 return "QS"; 248 case TRC: 249 return "TRC"; 250 case NP: 251 return "NP"; 252 case NULL: 253 return null; 254 default: 255 return "?"; 256 } 257 } 258 259 public String getSystem() { 260 return "http://terminology.hl7.org/CodeSystem/v3-NullFlavor"; 261 } 262 263 public String getDefinition() { 264 switch (this) { 265 case NI: 266 return "Description:The value is exceptional (missing, omitted, incomplete, improper). No information as to the reason for being an exceptional value is provided. This is the most general exceptional value. It is also the default exceptional value."; 267 case INV: 268 return "Description:The value as represented in the instance is not a member of the set of permitted data values in the constrained value domain of a variable."; 269 case DER: 270 return "Description:An actual value may exist, but it must be derived from the provided information (usually an EXPR generic data type extension will be used to convey the derivation expressionexpression ."; 271 case OTH: 272 return "Description:The actual value is not a member of the set of permitted data values in the constrained value domain of a variable. (e.g., concept not provided by required code system).\r\n\n \n Usage Notes: This flavor and its specializations are most commonly used with the CD datatype and its flavors. However, it may apply to *any* datatype where the constraints of the type are tighter than can be conveyed. For example, a PQ that is for a true measured amount whose units are not supported in UCUM, a need to convey a REAL when the type has been constrained to INT, etc.\r\n\n With coded datatypes, this null flavor may only be used if the vocabulary binding has a coding strength of CNE. By definition, all local codes and original text are part of the value set if the coding strength is CWE."; 273 case NINF: 274 return "Negative infinity of numbers."; 275 case PINF: 276 return "Positive infinity of numbers."; 277 case UNC: 278 return "Description: The actual value has not yet been encoded within the approved value domain.\r\n\n \n Example: Original text or a local code has been specified but translation or encoding to the approved value set has not yet occurred due to limitations of the sending system. Original text has been captured for a PQ, but not attempt has been made to split the value and unit or to encode the unit in UCUM.\r\n\n \n Usage Notes: If it is known that it is not possible to encode the concept, OTH should be used instead. However, use of UNC does not necessarily guarantee the concept will be encodable, only that encoding has not been attempted.\r\n\n Data type properties such as original text and translations may be present when this null flavor is included."; 279 case MSK: 280 return "There is information on this item available but it has not been provided by the sender due to security, privacy or other reasons. There may be an alternate mechanism for gaining access to this information.\r\n\n Note: using this null flavor does provide information that may be a breach of confidentiality, even though no detail data is provided. Its primary purpose is for those circumstances where it is necessary to inform the receiver that the information does exist without providing any detail."; 281 case NA: 282 return "Known to have no proper value (e.g., last menstrual period for a male)."; 283 case UNK: 284 return "Description:A proper value is applicable, but not known.\r\n\n \n Usage Notes: This means the actual value is not known. If the only thing that is unknown is how to properly express the value in the necessary constraints (value set, datatype, etc.), then the OTH or UNC flavor should be used. No properties should be included for a datatype with this property unless:\r\n\n \n Those properties themselves directly translate to a semantic of \"unknown\". (E.g. a local code sent as a translation that conveys 'unknown')\n Those properties further qualify the nature of what is unknown. (E.g. specifying a use code of \"H\" and a URL prefix of \"tel:\" to convey that it is the home phone number that is unknown.)"; 285 case ASKU: 286 return "Information was sought but not found (e.g., patient was asked but didn't know)"; 287 case NAV: 288 return "Information is not available at this time but it is expected that it will be available later."; 289 case NASK: 290 return "This information has not been sought (e.g., patient was not asked)"; 291 case NAVU: 292 return "Information is not available at this time (with no expectation regarding whether it will or will not be available in the future)."; 293 case QS: 294 return "Description:The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material. e.g. 'Add 10mg of ingredient X, 50mg of ingredient Y, and sufficient quantity of water to 100mL.' The null flavor would be used to express the quantity of water."; 295 case TRC: 296 return "The content is greater than zero, but too small to be quantified."; 297 case NP: 298 return "Value is not present in a message. This is only defined in messages, never in application data! All values not present in the message must be replaced by the applicable default, or no-information (NI) as the default of all defaults."; 299 case NULL: 300 return null; 301 default: 302 return "?"; 303 } 304 } 305 306 public String getDisplay() { 307 switch (this) { 308 case NI: 309 return "NoInformation"; 310 case INV: 311 return "invalid"; 312 case DER: 313 return "derived"; 314 case OTH: 315 return "other"; 316 case NINF: 317 return "negative infinity"; 318 case PINF: 319 return "positive infinity"; 320 case UNC: 321 return "un-encoded"; 322 case MSK: 323 return "masked"; 324 case NA: 325 return "not applicable"; 326 case UNK: 327 return "unknown"; 328 case ASKU: 329 return "asked but unknown"; 330 case NAV: 331 return "temporarily unavailable"; 332 case NASK: 333 return "not asked"; 334 case NAVU: 335 return "Not available"; 336 case QS: 337 return "Sufficient Quantity"; 338 case TRC: 339 return "trace"; 340 case NP: 341 return "not present"; 342 case NULL: 343 return null; 344 default: 345 return "?"; 346 } 347 } 348 349}