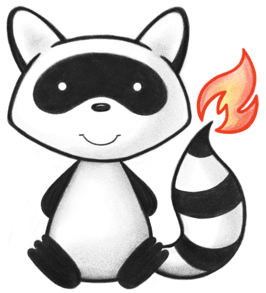
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ObservationCategory { 037 038 /** 039 * Observations generated by physical exam findings including direct 040 * observations made by a clinician and use of simple instruments and the result 041 * of simple maneuvers performed directly on the patient's body. 042 */ 043 EXAM, 044 /** 045 * Observations generated by imaging. The scope includes observations, plain 046 * x-ray, ultrasound, CT, MRI, angiography, echocardiography, nuclear medicine. 047 */ 048 IMAGING, 049 /** 050 * The results of observations generated by laboratories. Laboratory results are 051 * typically generated by laboratories providing analytic services in areas such 052 * as chemistry, hematology, serology, histology, cytology, anatomic pathology, 053 * microbiology, and/or virology. These observations are based on analysis of 054 * specimens obtained from the patient and submitted to the laboratory. 055 */ 056 LABORATORY, 057 /** 058 * Observations generated by other procedures. This category includes 059 * observations resulting from interventional and non-interventional procedures 060 * excluding lab and imaging (e.g. cardiology catheterization, endoscopy, 061 * electrodiagnostics, etc.). Procedure results are typically generated by a 062 * clinician to provide more granular information about component observations 063 * made during a procedure, such as where a gastroenterologist reports the size 064 * of a polyp observed during a colonoscopy. 065 */ 066 PROCEDURE, 067 /** 068 * The Social History Observations define the patient's occupational, personal 069 * (e.g. lifestyle), social, and environmental history and health risk factors, 070 * as well as administrative data such as marital status, race, ethnicity and 071 * religious affiliation. 072 */ 073 SOCIALHISTORY, 074 /** 075 * Assessment tool/survey instrument observations (e.g. Apgar Scores, Montreal 076 * Cognitive Assessment (MoCA)) 077 */ 078 SURVEY, 079 /** 080 * Observations generated by non-interventional treatment protocols (e.g. 081 * occupational, physical, radiation, nutritional and medication therapy) 082 */ 083 THERAPY, 084 /** 085 * Clinical observations measure the body's basic functions such as such as 086 * blood pressure, heart rate, respiratory rate, height, weight, body mass 087 * index, head circumference, pulse oximetry, temperature, and body surface 088 * area. 089 */ 090 VITALSIGNS, 091 /** 092 * added to help the parsers 093 */ 094 NULL; 095 096 public static V3ObservationCategory fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("exam".equals(codeString)) 100 return EXAM; 101 if ("imaging".equals(codeString)) 102 return IMAGING; 103 if ("laboratory".equals(codeString)) 104 return LABORATORY; 105 if ("procedure".equals(codeString)) 106 return PROCEDURE; 107 if ("social-history".equals(codeString)) 108 return SOCIALHISTORY; 109 if ("survey".equals(codeString)) 110 return SURVEY; 111 if ("therapy".equals(codeString)) 112 return THERAPY; 113 if ("vital-signs".equals(codeString)) 114 return VITALSIGNS; 115 throw new FHIRException("Unknown V3ObservationCategory code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case EXAM: 121 return "exam"; 122 case IMAGING: 123 return "imaging"; 124 case LABORATORY: 125 return "laboratory"; 126 case PROCEDURE: 127 return "procedure"; 128 case SOCIALHISTORY: 129 return "social-history"; 130 case SURVEY: 131 return "survey"; 132 case THERAPY: 133 return "therapy"; 134 case VITALSIGNS: 135 return "vital-signs"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getSystem() { 144 return "http://terminology.hl7.org/CodeSystem/v3-ObservationCategory"; 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case EXAM: 150 return "Observations generated by physical exam findings including direct observations made by a clinician and use of simple instruments and the result of simple maneuvers performed directly on the patient's body."; 151 case IMAGING: 152 return "Observations generated by imaging. The scope includes observations, plain x-ray, ultrasound, CT, MRI, angiography, echocardiography, nuclear medicine."; 153 case LABORATORY: 154 return "The results of observations generated by laboratories. Laboratory results are typically generated by laboratories providing analytic services in areas such as chemistry, hematology, serology, histology, cytology, anatomic pathology, microbiology, and/or virology. These observations are based on analysis of specimens obtained from the patient and submitted to the laboratory."; 155 case PROCEDURE: 156 return "Observations generated by other procedures. This category includes observations resulting from interventional and non-interventional procedures excluding lab and imaging (e.g. cardiology catheterization, endoscopy, electrodiagnostics, etc.). Procedure results are typically generated by a clinician to provide more granular information about component observations made during a procedure, such as where a gastroenterologist reports the size of a polyp observed during a colonoscopy."; 157 case SOCIALHISTORY: 158 return "The Social History Observations define the patient's occupational, personal (e.g. lifestyle), social, and environmental history and health risk factors, as well as administrative data such as marital status, race, ethnicity and religious affiliation."; 159 case SURVEY: 160 return "Assessment tool/survey instrument observations (e.g. Apgar Scores, Montreal Cognitive Assessment (MoCA))"; 161 case THERAPY: 162 return "Observations generated by non-interventional treatment protocols (e.g. occupational, physical, radiation, nutritional and medication therapy)"; 163 case VITALSIGNS: 164 return "Clinical observations measure the body's basic functions such as such as blood pressure, heart rate, respiratory rate, height, weight, body mass index, head circumference, pulse oximetry, temperature, and body surface area."; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 172 public String getDisplay() { 173 switch (this) { 174 case EXAM: 175 return "Exam"; 176 case IMAGING: 177 return "Imaging"; 178 case LABORATORY: 179 return "Laboratory"; 180 case PROCEDURE: 181 return "Procedure"; 182 case SOCIALHISTORY: 183 return "Social History"; 184 case SURVEY: 185 return "Survey"; 186 case THERAPY: 187 return "Therapy"; 188 case VITALSIGNS: 189 return "Vital Signs"; 190 case NULL: 191 return null; 192 default: 193 return "?"; 194 } 195 } 196 197}