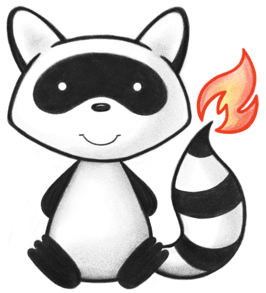
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ObservationValue { 037 038 /** 039 * Codes specify the category of observation, evidence, or document used to 040 * assess for services, e.g., discharge planning, or to establish eligibility 041 * for coverage under a policy or program. The type of evidence is coded as 042 * observation values. 043 */ 044 _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE, 045 /** 046 * Code specifying financial indicators used to assess or establish eligibility 047 * for coverage under a policy or program; e.g., pay stub; tax or income 048 * document; asset document; living expenses. 049 */ 050 _ACTFINANCIALSTATUSOBSERVATIONVALUE, 051 /** 052 * Codes specifying asset indicators used to assess or establish eligibility for 053 * coverage under a policy or program. 054 */ 055 ASSET, 056 /** 057 * Indicator of annuity ownership or status as beneficiary. 058 */ 059 ANNUITY, 060 /** 061 * Indicator of real property ownership, e.g., deed or real estate contract. 062 */ 063 PROP, 064 /** 065 * Indicator of retirement investment account ownership. 066 */ 067 RETACCT, 068 /** 069 * Indicator of status as trust beneficiary. 070 */ 071 TRUST, 072 /** 073 * Code specifying income indicators used to assess or establish eligibility for 074 * coverage under a policy or program; e.g., pay or pension check, child support 075 * payments received or provided, and taxes paid. 076 */ 077 INCOME, 078 /** 079 * Indicator of child support payments received or provided. 080 */ 081 CHILD, 082 /** 083 * Indicator of disability income replacement payment. 084 */ 085 DISABL, 086 /** 087 * Indicator of investment income, e.g., dividend check, annuity payment; real 088 * estate rent, investment divestiture proceeds; trust or endowment check. 089 */ 090 INVEST, 091 /** 092 * Indicator of paid employment, e.g., letter of hire, contract, employer 093 * letter; copy of pay check or pay stub. 094 */ 095 PAY, 096 /** 097 * Indicator of retirement payment, e.g., pension check. 098 */ 099 RETIRE, 100 /** 101 * Indicator of spousal or partner support payments received or provided; e.g., 102 * alimony payment; support stipulations in a divorce settlement. 103 */ 104 SPOUSAL, 105 /** 106 * Indicator of income supplement, e.g., gifting, parental income support; 107 * stipend, or grant. 108 */ 109 SUPPLE, 110 /** 111 * Indicator of tax obligation or payment, e.g., statement of taxable income. 112 */ 113 TAX, 114 /** 115 * Codes specifying living expense indicators used to assess or establish 116 * eligibility for coverage under a policy or program. 117 */ 118 LIVEXP, 119 /** 120 * Indicator of clothing expenses. 121 */ 122 CLOTH, 123 /** 124 * Indicator of transportation expenses. 125 */ 126 FOOD, 127 /** 128 * Indicator of health expenses; including medication costs, health service 129 * costs, financial participations, and health coverage premiums. 130 */ 131 HEALTH, 132 /** 133 * Indicator of housing expense, e.g., household appliances, fixtures, 134 * furnishings, and maintenance and repairs. 135 */ 136 HOUSE, 137 /** 138 * Indicator of legal expenses. 139 */ 140 LEGAL, 141 /** 142 * Indicator of mortgage amount, interest, and payments. 143 */ 144 MORTG, 145 /** 146 * Indicator of rental or lease payments. 147 */ 148 RENT, 149 /** 150 * Indicator of transportation expenses. 151 */ 152 SUNDRY, 153 /** 154 * Indicator of transportation expenses, e.g., vehicle payments, vehicle 155 * insurance, vehicle fuel, and vehicle maintenance and repairs. 156 */ 157 TRANS, 158 /** 159 * Indicator of transportation expenses. 160 */ 161 UTIL, 162 /** 163 * Code specifying eligibility indicators used to assess or establish 164 * eligibility for coverage under a policy or program eligibility status, e.g., 165 * certificates of creditable coverage; student enrollment; adoption, marriage 166 * or birth certificate. 167 */ 168 ELSTAT, 169 /** 170 * Indicator of adoption. 171 */ 172 ADOPT, 173 /** 174 * Indicator of birth. 175 */ 176 BTHCERT, 177 /** 178 * Indicator of creditable coverage. 179 */ 180 CCOC, 181 /** 182 * Indicator of driving status. 183 */ 184 DRLIC, 185 /** 186 * Indicator of foster child status. 187 */ 188 FOSTER, 189 /** 190 * Indicator of status as covered member under a policy or program, e.g., member 191 * id card or coverage document. 192 */ 193 MEMBER, 194 /** 195 * Indicator of military status. 196 */ 197 MIL, 198 /** 199 * Indicator of marriage status. 200 */ 201 MRGCERT, 202 /** 203 * Indicator of citizenship. 204 */ 205 PASSPORT, 206 /** 207 * Indicator of student status. 208 */ 209 STUDENRL, 210 /** 211 * Code specifying non-clinical indicators related to health status used to 212 * assess or establish eligibility for coverage under a policy or program, e.g., 213 * pregnancy, disability, drug use, mental health issues. 214 */ 215 HLSTAT, 216 /** 217 * Indication of disability. 218 */ 219 DISABLE, 220 /** 221 * Indication of drug use. 222 */ 223 DRUG, 224 /** 225 * Indication of IV drug use . 226 */ 227 IVDRG, 228 /** 229 * Non-clinical report of pregnancy. 230 */ 231 PGNT, 232 /** 233 * Code specifying observations related to living dependency, such as dependent 234 * upon spouse for activities of daily living. 235 */ 236 LIVDEP, 237 /** 238 * Continued living in private residence requires functional and health care 239 * assistance from one or more relatives. 240 */ 241 RELDEP, 242 /** 243 * Continued living in private residence requires functional and health care 244 * assistance from spouse or life partner. 245 */ 246 SPSDEP, 247 /** 248 * Continued living in private residence requires functional and health care 249 * assistance from one or more unrelated persons. 250 */ 251 URELDEP, 252 /** 253 * Code specifying observations related to living situation for a person in a 254 * private residence. 255 */ 256 LIVSIT, 257 /** 258 * Living alone. Maps to PD1-2 Living arrangement (IS) 00742 [A] 259 */ 260 ALONE, 261 /** 262 * Living with one or more dependent children requiring moderate supervision. 263 */ 264 DEPCHD, 265 /** 266 * Living with disabled spouse requiring functional and health care assistance 267 */ 268 DEPSPS, 269 /** 270 * Living with one or more dependent children requiring intensive supervision 271 */ 272 DEPYGCHD, 273 /** 274 * Living with family. Maps to PD1-2 Living arrangement (IS) 00742 [F] 275 */ 276 FAM, 277 /** 278 * Living with one or more relatives. Maps to PD1-2 Living arrangement (IS) 279 * 00742 [R] 280 */ 281 RELAT, 282 /** 283 * Living only with spouse or life partner. Maps to PD1-2 Living arrangement 284 * (IS) 00742 [S] 285 */ 286 SPS, 287 /** 288 * Living with one or more unrelated persons. 289 */ 290 UNREL, 291 /** 292 * Code specifying observations or indicators related to socio-economic status 293 * used to assess to assess for services, e.g., discharge planning, or to 294 * establish eligibility for coverage under a policy or program. 295 */ 296 SOECSTAT, 297 /** 298 * Indication of abuse victim. 299 */ 300 ABUSE, 301 /** 302 * Indication of status as homeless. 303 */ 304 HMLESS, 305 /** 306 * Indication of status as illegal immigrant. 307 */ 308 ILGIM, 309 /** 310 * Indication of status as incarcerated. 311 */ 312 INCAR, 313 /** 314 * Indication of probation status. 315 */ 316 PROB, 317 /** 318 * Indication of refugee status. 319 */ 320 REFUG, 321 /** 322 * Indication of unemployed status. 323 */ 324 UNEMPL, 325 /** 326 * Indicates the result of a particular allergy test. E.g. Negative, Mild, 327 * Moderate, Severe 328 */ 329 _ALLERGYTESTVALUE, 330 /** 331 * Description:Patient exhibits no reaction to the challenge agent. 332 */ 333 A0, 334 /** 335 * Description:Patient exhibits a minimal reaction to the challenge agent. 336 */ 337 A1, 338 /** 339 * Description:Patient exhibits a mild reaction to the challenge agent. 340 */ 341 A2, 342 /** 343 * Description:Patient exhibits moderate reaction to the challenge agent. 344 */ 345 A3, 346 /** 347 * Description:Patient exhibits a severe reaction to the challenge agent. 348 */ 349 A4, 350 /** 351 * Observation values that communicate the method used in a quality measure to 352 * combine the component measure results included in an composite measure. 353 */ 354 _COMPOSITEMEASURESCORING, 355 /** 356 * Code specifying that the measure uses all-or-nothing scoring. All-or-nothing 357 * scoring places an individual in the numerator of the composite measure if and 358 * only if they are in the numerator of all component measures in which they are 359 * in the denominator. 360 */ 361 ALLORNONESCR, 362 /** 363 * Code specifying that the measure uses linear scoring. Linear scoring computes 364 * the fraction of component measures in which the individual appears in the 365 * numerator, giving equal weight to each component measure. 366 */ 367 LINEARSCR, 368 /** 369 * Code specifying that the measure uses opportunity-based scoring. In 370 * opportunity-based scoring the measure score is determined by combining the 371 * denominator and numerator of each component measure to determine an overall 372 * composite score. 373 */ 374 OPPORSCR, 375 /** 376 * Code specifying that the measure uses weighted scoring. Weighted scoring 377 * assigns a factor to each component measure to weight that measure's 378 * contribution to the overall score. 379 */ 380 WEIGHTSCR, 381 /** 382 * Description:Coded observation values for coverage limitations, for e.g., 383 * types of claims or types of parties covered under a policy or program. 384 */ 385 _COVERAGELIMITOBSERVATIONVALUE, 386 /** 387 * Description:Coded observation values for types of covered parties under a 388 * policy or program based on their personal relationships or employment status. 389 */ 390 _COVERAGELEVELOBSERVATIONVALUE, 391 /** 392 * Description:Child over an age as specified by coverage policy or program, 393 * e.g., student, differently abled, and income dependent. 394 */ 395 ADC, 396 /** 397 * Description:Dependent biological, adopted, foster child as specified by 398 * coverage policy or program. 399 */ 400 CHD, 401 /** 402 * Description:Person requiring functional and/or financial assistance from 403 * another person as specified by coverage policy or program. 404 */ 405 DEP, 406 /** 407 * Description:Persons registered as a family unit in a domestic partner 408 * registry as specified by law and by coverage policy or program. 409 */ 410 DP, 411 /** 412 * Description:An individual employed by an employer who receive remuneration in 413 * wages, salary, commission, tips, piece-rates, or pay-in-kind through the 414 * employeraTMs payment system (i.e., not a contractor) as specified by coverage 415 * policy or program. 416 */ 417 ECH, 418 /** 419 * Description:As specified by coverage policy or program. 420 */ 421 FLY, 422 /** 423 * Description:Person as specified by coverage policy or program. 424 */ 425 IND, 426 /** 427 * Description:A pair of people of the same gender who live together as a family 428 * as specified by coverage policy or program, e.g., Naomi and Ruth from the 429 * Book of Ruth; Socrates and Alcibiades 430 */ 431 SSP, 432 /** 433 * A clinical judgment as to the worst case result of a future exposure 434 * (including substance administration). When the worst case result is assessed 435 * to have a life-threatening or organ system threatening potential, it is 436 * considered to be of high criticality. 437 */ 438 _CRITICALITYOBSERVATIONVALUE, 439 /** 440 * Worst case result of a future exposure is assessed to be life-threatening or 441 * having high potential for organ system failure. 442 */ 443 CRITH, 444 /** 445 * Worst case result of a future exposure is not assessed to be life-threatening 446 * or having high potential for organ system failure. 447 */ 448 CRITL, 449 /** 450 * Unable to assess the worst case result of a future exposure. 451 */ 452 CRITU, 453 /** 454 * Concepts representing whether a person does or does not currently have a job 455 * or is not currently in the labor pool seeking employment. 456 */ 457 _EMPLOYMENTSTATUS, 458 /** 459 * Individuals who, during the last week: a) did any work for at least 1 hour as 460 * paid or unpaid employees of a business or government organization; worked in 461 * their own businesses, professions, or on their own farms; or b) were not 462 * working, but who have a job or business from which the individual was 463 * temporarily absent because of vacation, illness, bad weather, childcare 464 * problems, maternity or paternity leave, labor-management dispute, job 465 * training, or other family or personal reasons, regardless of whether or not 466 * they were paid for the time off or were seeking other jobs. 467 */ 468 EMPLOYED, 469 /** 470 * Persons not classified as employed or unemployed, meaning those who have no 471 * job and are not looking for one. 472 */ 473 NOTINLABORFORCE, 474 /** 475 * Persons who currently have no employment, but are available for work and have 476 * made specific efforts to find employment. 477 */ 478 UNEMPLOYED, 479 /** 480 * Description: The domain contains genetic analysis specific observation 481 * values, e.g. Homozygote, Heterozygote, etc. 482 */ 483 _GENETICOBSERVATIONVALUE, 484 /** 485 * Description: An individual having different alleles at one or more loci 486 * regarding a specific character 487 */ 488 HOMOZYGOTE, 489 /** 490 * Observation values used to indicate the type of scoring (e.g. proportion, 491 * ratio) used by a health quality measure. 492 */ 493 _OBSERVATIONMEASURESCORING, 494 /** 495 * A measure in which either short-term cross-section or long-term longitudinal 496 * analysis is performed over a group of subjects defined by a set of common 497 * properties or defining characteristics (e.g., Male smokers between the ages 498 * of 40 and 50 years, exposure to treatment, exposure duration). 499 */ 500 COHORT, 501 /** 502 * A measure score in which each individual value for the measure can fall 503 * anywhere along a continuous scale (e.g., mean time to thrombolytics which 504 * aggregates the time in minutes from a case presenting with chest pain to the 505 * time of administration of thrombolytics). 506 */ 507 CONTVAR, 508 /** 509 * A score derived by dividing the number of cases that meet a criterion for 510 * quality (the numerator) by the number of eligible cases within a given time 511 * frame (the denominator) where the numerator cases are a subset of the 512 * denominator cases (e.g., percentage of eligible women with a mammogram 513 * performed in the last year). 514 */ 515 PROPOR, 516 /** 517 * A score that may have a value of zero or greater that is derived by dividing 518 * a count of one type of data by a count of another type of data (e.g., the 519 * number of patients with central lines who develop infection divided by the 520 * number of central line days). 521 */ 522 RATIO, 523 /** 524 * Observation values used to indicate what kind of health quality measure is 525 * used. 526 */ 527 _OBSERVATIONMEASURETYPE, 528 /** 529 * A measure that is composed from one or more other measures and indicates an 530 * overall summary of those measures. 531 */ 532 COMPOSITE, 533 /** 534 * A measure related to the efficiency of medical treatment. 535 */ 536 EFFICIENCY, 537 /** 538 * A measure related to the level of patient engagement or patient experience of 539 * care. 540 */ 541 EXPERIENCE, 542 /** 543 * A measure that indicates the result of the performance (or non-performance) 544 * of a function or process. 545 */ 546 OUTCOME, 547 /** 548 * A measure that evaluates the change over time of a physiologic state 549 * observable that is associated with a specific long-term health outcome. 550 */ 551 INTERMOM, 552 /** 553 * A measure that is a comparison of patient reported outcomes for a single or 554 * multiple patients collected via an instrument specifically designed to obtain 555 * input directly from patients. 556 */ 557 PROPM, 558 /** 559 * A measure which focuses on a process which leads to a certain outcome, 560 * meaning that a scientific basis exists for believing that the process, when 561 * executed well, will increase the probability of achieving a desired outcome. 562 */ 563 PROCESS, 564 /** 565 * A measure that assesses the use of one or more processes where the expected 566 * health benefit exceeds the expected negative consequences. 567 */ 568 APPROPRIATE, 569 /** 570 * A measure related to the extent of use of clinical resources or cost of care. 571 */ 572 RESOURCE, 573 /** 574 * A measure related to the structure of patient care. 575 */ 576 STRUCTURE, 577 /** 578 * Observation values used to assert various populations that a subject falls 579 * into. 580 */ 581 _OBSERVATIONPOPULATIONINCLUSION, 582 /** 583 * Patients who should be removed from the eMeasure population and denominator 584 * before determining if numerator criteria are met. Denominator exclusions are 585 * used in proportion and ratio measures to help narrow the denominator. 586 */ 587 DENEX, 588 /** 589 * Denominator exceptions are those conditions that should remove a patient, 590 * procedure or unit of measurement from the denominator only if the numerator 591 * criteria are not met. Denominator exceptions allow for adjustment of the 592 * calculated score for those providers with higher risk populations. 593 * Denominator exceptions are used only in proportion eMeasures. They are not 594 * appropriate for ratio or continuous variable eMeasures. Denominator 595 * exceptions allow for the exercise of clinical judgment and should be 596 * specifically defined where capturing the information in a structured manner 597 * fits the clinical workflow. Generic denominator exception reasons used in 598 * proportion eMeasures fall into three general categories: 599 * 600 * 601 * Medical reasons Patient reasons System reasons 602 */ 603 DENEXCEP, 604 /** 605 * It can be the same as the initial patient population or a subset of the 606 * initial patient population to further constrain the population for the 607 * purpose of the eMeasure. Different measures within an eMeasure set may have 608 * different Denominators. Continuous Variable eMeasures do not have a 609 * Denominator, but instead define a Measure Population. 610 */ 611 DENOM, 612 /** 613 * The initial population refers to all entities to be evaluated by a specific 614 * quality measure who share a common set of specified characteristics within a 615 * specific measurement set to which a given measure belongs. 616 */ 617 IP, 618 /** 619 * The initial patient population refers to all patients to be evaluated by a 620 * specific quality measure who share a common set of specified characteristics 621 * within a specific measurement set to which a given measure belongs. Details 622 * often include information based upon specific age groups, diagnoses, 623 * diagnostic and procedure codes, and enrollment periods. 624 */ 625 IPP, 626 /** 627 * Measure population is used only in continuous variable eMeasures. It is a 628 * narrative description of the eMeasure population. (e.g., all patients seen in 629 * the Emergency Department during the measurement period). 630 */ 631 MSRPOPL, 632 /** 633 * Numerators are used in proportion and ratio eMeasures. In proportion measures 634 * the numerator criteria are the processes or outcomes expected for each 635 * patient, procedure, or other unit of measurement defined in the denominator. 636 * In ratio measures the numerator is related, but not directly derived from the 637 * denominator (e.g., a numerator listing the number of central line blood 638 * stream infections and a denominator indicating the days per thousand of 639 * central line usage in a specific time period). 640 */ 641 NUMER, 642 /** 643 * Numerator Exclusions are used only in ratio eMeasures to define instances 644 * that should not be included in the numerator data. (e.g., if the number of 645 * central line blood stream infections per 1000 catheter days were to exclude 646 * infections with a specific bacterium, that bacterium would be listed as a 647 * numerator exclusion.) 648 */ 649 NUMEX, 650 /** 651 * PartialCompletionScale 652 */ 653 _PARTIALCOMPLETIONSCALE, 654 /** 655 * Value for Act.partialCompletionCode attribute that implies 81-99% completion 656 */ 657 G, 658 /** 659 * Value for Act.partialCompletionCode attribute that implies 61-80% completion 660 */ 661 LE, 662 /** 663 * Value for Act.partialCompletionCode attribute that implies 41-60% completion 664 */ 665 ME, 666 /** 667 * Value for Act.partialCompletionCode attribute that implies 1-20% completion 668 */ 669 MI, 670 /** 671 * Value for Act.partialCompletionCode attribute that implies 0% completion 672 */ 673 N, 674 /** 675 * Value for Act.partialCompletionCode attribute that implies 21-40% completion 676 */ 677 S, 678 /** 679 * Observation values used to indicate security observation metadata. 680 */ 681 _SECURITYOBSERVATIONVALUE, 682 /** 683 * Abstract security observation values used to indicate security integrity 684 * metadata. 685 * 686 * 687 * Examples: Codes conveying integrity status, integrity confidence, and 688 * provenance. 689 */ 690 _SECINTOBV, 691 /** 692 * Abstract security metadata observation values used to indicate mechanism used 693 * for authorized alteration of an IT resource (data, information object, 694 * service, or system capability) 695 */ 696 _SECALTINTOBV, 697 /** 698 * Security metadata observation values used to indicate the use of a more 699 * abstract version of the content, e.g., replacing exact value of an age or 700 * date field with a range, or remove the left digits of a credit card number or 701 * SSN. 702 */ 703 ABSTRED, 704 /** 705 * Security metadata observation values used to indicate the use of an 706 * algorithmic combination of actual values with the result of an aggregate 707 * function, e.g., average, sum, or count in order to limit disclosure of an IT 708 * resource (data, information object, service, or system capability) to the 709 * minimum necessary. 710 */ 711 AGGRED, 712 /** 713 * Security metadata observation value conveying the alteration integrity of an 714 * IT resource (data, information object, service, or system capability) by used 715 * to indicate the mechanism by which software systems can strip portions of the 716 * resource that could allow the identification of the source of the information 717 * or the information subject. No key to relink the data is retained. 718 */ 719 ANONYED, 720 /** 721 * Security metadata observation value used to indicate that the IT resource 722 * semantic content has been transformed from one encoding to another. 723 * 724 * 725 * Usage Note: "MAP" code does not indicate the semantic fidelity of the 726 * transformed content. 727 * 728 * To indicate semantic fidelity for maps of HL7 to other code systems, this 729 * security alteration integrity observation may be further specified using an 730 * Act valued with Value Set: MapRelationship (2.16.840.1.113883.1.11.11052). 731 * 732 * Semantic fidelity of the mapped IT Resource may also be indicated using a 733 * SecurityIntegrityConfidenceObservation. 734 */ 735 MAPPED, 736 /** 737 * Security metadata observation value conveying the alteration integrity of an 738 * IT resource (data, information object, service, or system capability) by 739 * indicating the mechanism by which software systems can make data 740 * unintelligible (that is, as unreadable and unusable by algorithmically 741 * transforming plaintext into ciphertext) such that it can only be accessed or 742 * used by authorized users. An authorized user may be provided a key to decrypt 743 * per license or "shared secret". 744 * 745 * 746 * Usage Note: "MASKED" may be used, per applicable policy, as a flag to 747 * indicate to a user or receiver that some portion of an IT resource has been 748 * further encrypted, and may be accessed only by an authorized user or receiver 749 * to which a decryption key is provided. 750 */ 751 MASKED, 752 /** 753 * Security metadata observation value conveying the alteration integrity of an 754 * IT resource (data, information object, service, or system capability), by 755 * indicating the mechanism by which software systems can strip portions of the 756 * resource that could allow the identification of the source of the information 757 * or the information subject. Custodian may retain a key to relink data 758 * necessary to reidentify the information subject. 759 * 760 * 761 * Rationale: Personal data which has been processed to make it impossible to 762 * know whose data it is. Used particularly for secondary use of health data. In 763 * some cases, it may be possible for authorized individuals to restore the 764 * identity of the individual, e.g.,for public health case management. Based on 765 * ISO/TS 25237:2008 Health informaticsâ??Pseudonymization 766 */ 767 PSEUDED, 768 /** 769 * Security metadata observation value used to indicate the mechanism by which 770 * software systems can filter an IT resource (data, information object, 771 * service, or system capability) to remove any portion of the resource that is 772 * not authorized to be access, used, or disclosed. 773 * 774 * 775 * Usage Note: "REDACTED" may be used, per applicable policy, as a flag to 776 * indicate to a user or receiver that some portion of an IT resource has 777 * filtered and not included in the content accessed or received. 778 */ 779 REDACTED, 780 /** 781 * Metadata observation used to indicate that some information has been removed 782 * from the source object when the view this object contains was constructed 783 * because of configuration options when the view was created. The content may 784 * not be suitable for use as the basis of a record update 785 * 786 * 787 * Usage Note: This is not suitable to be used when information is removed for 788 * security reasons - see the code REDACTED for this use. 789 */ 790 SUBSETTED, 791 /** 792 * Security metadata observation value used to indicate that the IT resource 793 * syntax has been transformed from one syntactical representation to another. 794 * 795 * 796 * Usage Note: "SYNTAC" code does not indicate the syntactical correctness of 797 * the syntactically transformed IT resource. 798 */ 799 SYNTAC, 800 /** 801 * Security metadata observation value used to indicate that the IT resource has 802 * been translated from one human language to another. 803 * 804 * 805 * Usage Note: "TRSLT" does not indicate the fidelity of the translation or the 806 * languages translated. 807 * 808 * The fidelity of the IT Resource translation may be indicated using a 809 * SecurityIntegrityConfidenceObservation. 810 * 811 * To indicate languages, use the Value Set:HumanLanguage 812 * (2.16.840.1.113883.1.11.11526) 813 */ 814 TRSLT, 815 /** 816 * Security metadata observation value conveying the alteration integrity of an 817 * IT resource (data, information object, service, or system capability) which 818 * indicates that the resource only retains versions of an IT resource for 819 * access and use per applicable policy 820 * 821 * 822 * Usage Note: When this code is used, expectation is that the system has 823 * removed historical versions of the data that falls outside the time period 824 * deemed to be the effective time of the applicable version. 825 */ 826 VERSIONED, 827 /** 828 * Abstract security observation values used to indicate data integrity 829 * metadata. 830 * 831 * 832 * Examples: Codes conveying the mechanism used to preserve the accuracy and 833 * consistency of an IT resource such as a digital signature and a cryptographic 834 * hash function. 835 */ 836 _SECDATINTOBV, 837 /** 838 * Security metadata observation value used to indicate the mechanism by which 839 * software systems can establish that data was not modified in transit. 840 * 841 * 842 * Rationale: This definition is intended to align with the ISO 22600-2 3.3.19 843 * definition of cryptographic checkvalue: Information which is derived by 844 * performing a cryptographic transformation (see cryptography) on the data 845 * unit. The derivation of the checkvalue may be performed in one or more steps 846 * and is a result of a mathematical function of the key and a data unit. It is 847 * usually used to check the integrity of a data unit. 848 * 849 * 850 * Examples: 851 * 852 * 853 * 854 * SHA-1 SHA-2 (Secure Hash Algorithm) 855 */ 856 CRYTOHASH, 857 /** 858 * Security metadata observation value used to indicate the mechanism by which 859 * software systems use digital signature to establish that data has not been 860 * modified. 861 * 862 * 863 * Rationale: This definition is intended to align with the ISO 22600-2 3.3.26 864 * definition of digital signature: Data appended to, or a cryptographic 865 * transformation (see cryptography) of, a data unit that allows a recipient of 866 * the data unit to prove the source and integrity of the data unit and protect 867 * against forgery e.g., by the recipient. 868 */ 869 DIGSIG, 870 /** 871 * Abstract security observation value used to indicate integrity confidence 872 * metadata. 873 * 874 * 875 * Examples: Codes conveying the level of reliability and trustworthiness of an 876 * IT resource. 877 */ 878 _SECINTCONOBV, 879 /** 880 * Security metadata observation value used to indicate that the veracity or 881 * trustworthiness of an IT resource (data, information object, service, or 882 * system capability) for a specified purpose of use is perceived to be or 883 * deemed by policy to be very high. 884 */ 885 HRELIABLE, 886 /** 887 * Security metadata observation value used to indicate that the veracity or 888 * trustworthiness of an IT resource (data, information object, service, or 889 * system capability) for a specified purpose of use is perceived to be or 890 * deemed by policy to be adequate. 891 */ 892 RELIABLE, 893 /** 894 * Security metadata observation value used to indicate that the veracity or 895 * trustworthiness of an IT resource (data, information object, service, or 896 * system capability) for a specified purpose of use is perceived to be or 897 * deemed by policy to be uncertain. 898 */ 899 UNCERTREL, 900 /** 901 * Security metadata observation value used to indicate that the veracity or 902 * trustworthiness of an IT resource (data, information object, service, or 903 * system capability) for a specified purpose of use is perceived to be or 904 * deemed by policy to be inadequate. 905 */ 906 UNRELIABLE, 907 /** 908 * Abstract security metadata observation value used to indicate the provenance 909 * of an IT resource (data, information object, service, or system capability). 910 * 911 * 912 * Examples: Codes conveying the provenance metadata about the entity reporting 913 * an IT resource. 914 */ 915 _SECINTPRVOBV, 916 /** 917 * Abstract security provenance metadata observation value used to indicate the 918 * entity that asserted an IT resource (data, information object, service, or 919 * system capability). 920 * 921 * 922 * Examples: Codes conveying the provenance metadata about the entity asserting 923 * the resource. 924 */ 925 _SECINTPRVABOBV, 926 /** 927 * Security provenance metadata observation value used to indicate that an IT 928 * resource (data, information object, service, or system capability) was 929 * asserted by a clinician. 930 */ 931 CLINAST, 932 /** 933 * Security provenance metadata observation value used to indicate that an IT 934 * resource (data, information object, service, or system capability) was 935 * asserted by a device. 936 */ 937 DEVAST, 938 /** 939 * Security provenance metadata observation value used to indicate that an IT 940 * resource (data, information object, service, or system capability) was 941 * asserted by a healthcare professional. 942 */ 943 HCPAST, 944 /** 945 * Security provenance metadata observation value used to indicate that an IT 946 * resource (data, information object, service, or system capability) was 947 * asserted by a patient acquaintance. 948 */ 949 PACQAST, 950 /** 951 * Security provenance metadata observation value used to indicate that an IT 952 * resource (data, information object, service, or system capability) was 953 * asserted by a patient. 954 */ 955 PATAST, 956 /** 957 * Security provenance metadata observation value used to indicate that an IT 958 * resource (data, information object, service, or system capability) was 959 * asserted by a payer. 960 */ 961 PAYAST, 962 /** 963 * Security provenance metadata observation value used to indicate that an IT 964 * resource (data, information object, service, or system capability) was 965 * asserted by a professional. 966 */ 967 PROAST, 968 /** 969 * Security provenance metadata observation value used to indicate that an IT 970 * resource (data, information object, service, or system capability) was 971 * asserted by a substitute decision maker. 972 */ 973 SDMAST, 974 /** 975 * Abstract security provenance metadata observation value used to indicate the 976 * entity that reported the resource (data, information object, service, or 977 * system capability). 978 * 979 * 980 * Examples: Codes conveying the provenance metadata about the entity reporting 981 * an IT resource. 982 */ 983 _SECINTPRVRBOBV, 984 /** 985 * Security provenance metadata observation value used to indicate that an IT 986 * resource (data, information object, service, or system capability) was 987 * reported by a clinician. 988 */ 989 CLINRPT, 990 /** 991 * Security provenance metadata observation value used to indicate that an IT 992 * resource (data, information object, service, or system capability) was 993 * reported by a device. 994 */ 995 DEVRPT, 996 /** 997 * Security provenance metadata observation value used to indicate that an IT 998 * resource (data, information object, service, or system capability) was 999 * reported by a healthcare professional. 1000 */ 1001 HCPRPT, 1002 /** 1003 * Security provenance metadata observation value used to indicate that an IT 1004 * resource (data, information object, service, or system capability) was 1005 * reported by a patient acquaintance. 1006 */ 1007 PACQRPT, 1008 /** 1009 * Security provenance metadata observation value used to indicate that an IT 1010 * resource (data, information object, service, or system capability) was 1011 * reported by a patient. 1012 */ 1013 PATRPT, 1014 /** 1015 * Security provenance metadata observation value used to indicate that an IT 1016 * resource (data, information object, service, or system capability) was 1017 * reported by a payer. 1018 */ 1019 PAYRPT, 1020 /** 1021 * Security provenance metadata observation value used to indicate that an IT 1022 * resource (data, information object, service, or system capability) was 1023 * reported by a professional. 1024 */ 1025 PRORPT, 1026 /** 1027 * Security provenance metadata observation value used to indicate that an IT 1028 * resource (data, information object, service, or system capability) was 1029 * reported by a substitute decision maker. 1030 */ 1031 SDMRPT, 1032 /** 1033 * Observation value used to indicate aspects of trust applicable to an IT 1034 * resource (data, information object, service, or system capability). 1035 */ 1036 SECTRSTOBV, 1037 /** 1038 * Values for security trust accreditation metadata observation made about the 1039 * formal declaration by an authority or neutral third party that validates the 1040 * technical, security, trust, and business practice conformance of Trust Agents 1041 * to facilitate security, interoperability, and trust among participants within 1042 * a security domain or trust framework. 1043 */ 1044 TRSTACCRDOBV, 1045 /** 1046 * Values for security trust agreement metadata observation made about privacy 1047 * and security requirements with which a security domain must comply. [ISO IEC 1048 * 10181-1] [ISO IEC 10181-1] 1049 */ 1050 TRSTAGREOBV, 1051 /** 1052 * Values for security trust certificate metadata observation made about a set 1053 * of security-relevant data issued by a security authority or trusted third 1054 * party, together with security information which is used to provide the 1055 * integrity and data origin authentication services for an IT resource (data, 1056 * information object, service, or system capability). [Based on ISO IEC 1057 * 10181-1] 1058 * 1059 * For example, a Certificate Policy (CP), which is a named set of rules that 1060 * indicates the applicability of a certificate to a particular community and/or 1061 * class of application with common security requirements. A particular 1062 * Certificate Policy might indicate the applicability of a type of certificate 1063 * to the authentication of electronic data interchange transactions for the 1064 * trading of goods within a given price range. Another example is Cross 1065 * Certification with Federal Bridge. 1066 */ 1067 TRSTCERTOBV, 1068 /** 1069 * Values for security trust assurance metadata observation made about the 1070 * digital quality or reliability of a trust assertion, activity, capability, 1071 * information exchange, mechanism, process, or protocol. 1072 */ 1073 TRSTLOAOBV, 1074 /** 1075 * The value assigned as the indicator of the digital quality or reliability of 1076 * the verification and validation process used to verify the claimed identity 1077 * of an entity by securely associating an identifier and its authenticator. 1078 * [Based on ISO 7498-2] 1079 * 1080 * For example, the degree of confidence in the vetting process used to 1081 * establish the identity of the individual to whom the credential was issued, 1082 * and 2) the degree of confidence that the individual who uses the credential 1083 * is the individual to whom the credential was issued. [OMB M-04-04 1084 * E-Authentication Guidance for Federal Agencies] 1085 */ 1086 LOAAN, 1087 /** 1088 * Indicator of low digital quality or reliability of the digital reliability of 1089 * the verification and validation process used to verify the claimed identity 1090 * of an entity by securely associating an identifier and its authenticator. 1091 * [Based on ISO 7498-2] 1092 * 1093 * The degree of confidence in the vetting process used to establish the 1094 * identity of the individual to whom the credential was issued, and 2) the 1095 * degree of confidence that the individual who uses the credential is the 1096 * individual to whom the credential was issued. [OMB M-04-04 E-Authentication 1097 * Guidance for Federal Agencies] 1098 * 1099 * Low authentication level of assurance indicates that the relying party may 1100 * have little or no confidence in the asserted identity's validity. Level 1 1101 * requires little or no confidence in the asserted identity. No identity 1102 * proofing is required at this level, but the authentication mechanism should 1103 * provide some assurance that the same claimant is accessing the protected 1104 * transaction or data. A wide range of available authentication technologies 1105 * can be employed and any of the token methods of Levels 2, 3, or 4, including 1106 * Personal Identification Numbers (PINs), may be used. To be authenticated, the 1107 * claimant must prove control of the token through a secure authentication 1108 * protocol. At Level 1, long-term shared authentication secrets may be revealed 1109 * to verifiers. Assertions issued about claimants as a result of a successful 1110 * authentication are either cryptographically authenticated by relying parties 1111 * (using approved methods) or are obtained directly from a trusted party via a 1112 * secure authentication protocol. [Summary of the technical requirements 1113 * specified in NIST SP 800-63 for the four levels of assurance defined by the 1114 * December 2003, the Office of Management and Budget (OMB) issued Memorandum 1115 * M-04-04, E-Authentication Guidance for Federal Agencies.] 1116 */ 1117 LOAAN1, 1118 /** 1119 * Indicator of basic digital quality or reliability of the digital reliability 1120 * of the verification and validation process used to verify the claimed 1121 * identity of an entity by securely associating an identifier and its 1122 * authenticator. [Based on ISO 7498-2] 1123 * 1124 * The degree of confidence in the vetting process used to establish the 1125 * identity of the individual to whom the credential was issued, and 2) the 1126 * degree of confidence that the individual who uses the credential is the 1127 * individual to whom the credential was issued. [OMB M-04-04 E-Authentication 1128 * Guidance for Federal Agencies] 1129 * 1130 * Basic authentication level of assurance indicates that the relying party may 1131 * have some confidence in the asserted identity's validity. Level 2 requires 1132 * confidence that the asserted identity is accurate. Level 2 provides for 1133 * single-factor remote network authentication, including identity-proofing 1134 * requirements for presentation of identifying materials or information. A wide 1135 * range of available authentication technologies can be employed, including any 1136 * of the token methods of Levels 3 or 4, as well as passwords. Successful 1137 * authentication requires that the claimant prove through a secure 1138 * authentication protocol that the claimant controls the token. Eavesdropper, 1139 * replay, and online guessing attacks are prevented. Long-term shared 1140 * authentication secrets, if used, are never revealed to any party except the 1141 * claimant and verifiers operated by the CSP; however, session (temporary) 1142 * shared secrets may be provided to independent verifiers by the CSP. Approved 1143 * cryptographic techniques are required. Assertions issued about claimants as a 1144 * result of a successful authentication are either cryptographically 1145 * authenticated by relying parties (using approved methods) or are obtained 1146 * directly from a trusted party via a secure authentication protocol. [Summary 1147 * of the technical requirements specified in NIST SP 800-63 for the four levels 1148 * of assurance defined by the December 2003, the Office of Management and 1149 * Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal 1150 * Agencies.] 1151 */ 1152 LOAAN2, 1153 /** 1154 * Indicator of medium digital quality or reliability of the digital reliability 1155 * of verification and validation of the process used to verify the claimed 1156 * identity of an entity by securely associating an identifier and its 1157 * authenticator. [Based on ISO 7498-2] 1158 * 1159 * The degree of confidence in the vetting process used to establish the 1160 * identity of the individual to whom the credential was issued, and 2) the 1161 * degree of confidence that the individual who uses the credential is the 1162 * individual to whom the credential was issued. [OMB M-04-04 E-Authentication 1163 * Guidance for Federal Agencies] 1164 * 1165 * Medium authentication level of assurance indicates that the relying party may 1166 * have high confidence in the asserted identity's validity. Level 3 is 1167 * appropriate for transactions that need high confidence in the accuracy of the 1168 * asserted identity. Level 3 provides multifactor remote network 1169 * authentication. At this level, identity-proofing procedures require 1170 * verification of identifying materials and information. Authentication is 1171 * based on proof of possession of a key or password through a cryptographic 1172 * protocol. Cryptographic strength mechanisms should protect the primary 1173 * authentication token (a cryptographic key) against compromise by the protocol 1174 * threats, including eavesdropper, replay, online guessing, verifier 1175 * impersonation, and man-in-the-middle attacks. A minimum of two authentication 1176 * factors is required. Three kinds of tokens may be used: 1177 * 1178 * 1179 * "soft" cryptographic token, which has the key stored on a general-purpose 1180 * computer, "hard" cryptographic token, which has the key stored on a special 1181 * hardware device, and "one-time password" device token, which has symmetric 1182 * key stored on a personal hardware device that is a cryptographic module 1183 * validated at FIPS 140-2 Level 1 or higher. Validation testing of 1184 * cryptographic modules and algorithms for conformance to Federal Information 1185 * Processing Standard (FIPS) 140-2, Security Requirements for Cryptographic 1186 * Modules, is managed by NIST. 1187 * 1188 * Authentication requires that the claimant prove control of the token through 1189 * a secure authentication protocol. The token must be unlocked with a password 1190 * or biometric representation, or a password must be used in a secure 1191 * authentication protocol, to establish two-factor authentication. Long-term 1192 * shared authentication secrets, if used, are never revealed to any party 1193 * except the claimant and verifiers operated directly by the CSP; however, 1194 * session (temporary) shared secrets may be provided to independent verifiers 1195 * by the CSP. Approved cryptographic techniques are used for all operations. 1196 * Assertions issued about claimants as a result of a successful authentication 1197 * are either cryptographically authenticated by relying parties (using approved 1198 * methods) or are obtained directly from a trusted party via a secure 1199 * authentication protocol. [Summary of the technical requirements specified in 1200 * NIST SP 800-63 for the four levels of assurance defined by the December 2003, 1201 * the Office of Management and Budget (OMB) issued Memorandum M-04-04, 1202 * E-Authentication Guidance for Federal Agencies.] 1203 */ 1204 LOAAN3, 1205 /** 1206 * Indicator of high digital quality or reliability of the digital reliability 1207 * of the verification and validation process used to verify the claimed 1208 * identity of an entity by securely associating an identifier and its 1209 * authenticator. [Based on ISO 7498-2] 1210 * 1211 * The degree of confidence in the vetting process used to establish the 1212 * identity of the individual to whom the credential was issued, and 2) the 1213 * degree of confidence that the individual who uses the credential is the 1214 * individual to whom the credential was issued. [OMB M-04-04 E-Authentication 1215 * Guidance for Federal Agencies] 1216 * 1217 * High authentication level of assurance indicates that the relying party may 1218 * have very high confidence in the asserted identity's validity. Level 4 is for 1219 * transactions that need very high confidence in the accuracy of the asserted 1220 * identity. Level 4 provides the highest practical assurance of remote network 1221 * authentication. Authentication is based on proof of possession of a key 1222 * through a cryptographic protocol. This level is similar to Level 3 except 1223 * that only â??hardâ?? cryptographic tokens are allowed, cryptographic module 1224 * validation requirements are strengthened, and subsequent critical data 1225 * transfers must be authenticated via a key that is bound to the authentication 1226 * process. The token should be a hardware cryptographic module validated at 1227 * FIPS 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 1228 * physical security. This level requires a physical token, which cannot readily 1229 * be copied, and operator authentication at Level 2 and higher, and ensures 1230 * good, two-factor remote authentication. 1231 * 1232 * Level 4 requires strong cryptographic authentication of all parties and all 1233 * sensitive data transfers between the parties. Either public key or symmetric 1234 * key technology may be used. Authentication requires that the claimant prove 1235 * through a secure authentication protocol that the claimant controls the 1236 * token. Eavesdropper, replay, online guessing, verifier impersonation, and 1237 * man-in-the-middle attacks are prevented. Long-term shared authentication 1238 * secrets, if used, are never revealed to any party except the claimant and 1239 * verifiers operated directly by the CSP; however, session (temporary) shared 1240 * secrets may be provided to independent verifiers by the CSP. Strong approved 1241 * cryptographic techniques are used for all operations. All sensitive data 1242 * transfers are cryptographically authenticated using keys bound to the 1243 * authentication process. [Summary of the technical requirements specified in 1244 * NIST SP 800-63 for the four levels of assurance defined by the December 2003, 1245 * the Office of Management and Budget (OMB) issued Memorandum M-04-04, 1246 * E-Authentication Guidance for Federal Agencies.] 1247 */ 1248 LOAAN4, 1249 /** 1250 * The value assigned as the indicator of the digital quality or reliability of 1251 * a defined sequence of messages between a Claimant and a Verifier that 1252 * demonstrates that the Claimant has possession and control of a valid token to 1253 * establish his/her identity, and optionally, demonstrates to the Claimant that 1254 * he or she is communicating with the intended Verifier. [Based on NIST SP 1255 * 800-63-2] 1256 */ 1257 LOAAP, 1258 /** 1259 * Indicator of the low digital quality or reliability of a defined sequence of 1260 * messages between a Claimant and a Verifier that demonstrates that the 1261 * Claimant has possession and control of a valid token to establish his/her 1262 * identity, and optionally, demonstrates to the Claimant that he or she is 1263 * communicating with the intended Verifier. [Based on NIST SP 800-63-2] 1264 * 1265 * Low authentication process level of assurance indicates that (1) long-term 1266 * shared authentication secrets may be revealed to verifiers; and (2) 1267 * assertions and assertion references require protection from 1268 * manufacture/modification and reuse attacks. [Summary of the technical 1269 * requirements specified in NIST SP 800-63 for the four levels of assurance 1270 * defined by the December 2003, the Office of Management and Budget (OMB) 1271 * issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 1272 */ 1273 LOAAP1, 1274 /** 1275 * Indicator of the basic digital quality or reliability of a defined sequence 1276 * of messages between a Claimant and a Verifier that demonstrates that the 1277 * Claimant has possession and control of a valid token to establish his/her 1278 * identity, and optionally, demonstrates to the Claimant that he or she is 1279 * communicating with the intended Verifier. [Based on NIST SP 800-63-2] 1280 * 1281 * Basic authentication process level of assurance indicates that long-term 1282 * shared authentication secrets are never revealed to any other party except 1283 * Credential Service Provider (CSP). Sessions (temporary) shared secrets may be 1284 * provided to independent verifiers by CSP. Long-term shared authentication 1285 * secrets, if used, are never revealed to any other party except Verifiers 1286 * operated by the Credential Service Provider (CSP); however, session 1287 * (temporary) shared secrets may be provided to independent Verifiers by the 1288 * CSP. In addition to Level 1 requirements, assertions are resistant to 1289 * disclosure, redirection, capture and substitution attacks. Approved 1290 * cryptographic techniques are required. [Summary of the technical requirements 1291 * specified in NIST SP 800-63 for the four levels of assurance defined by the 1292 * December 2003, the Office of Management and Budget (OMB) issued Memorandum 1293 * M-04-04, E-Authentication Guidance for Federal Agencies.] 1294 */ 1295 LOAAP2, 1296 /** 1297 * Indicator of the medium digital quality or reliability of a defined sequence 1298 * of messages between a Claimant and a Verifier that demonstrates that the 1299 * Claimant has possession and control of a valid token to establish his/her 1300 * identity, and optionally, demonstrates to the Claimant that he or she is 1301 * communicating with the intended Verifier. [Based on NIST SP 800-63-2] 1302 * 1303 * Medium authentication process level of assurance indicates that the token can 1304 * be unlocked with password, biometric, or uses a secure multi-token 1305 * authentication protocol to establish two-factor authentication. Long-term 1306 * shared authentication secrets are never revealed to any party except the 1307 * Claimant and Credential Service Provider (CSP). 1308 * 1309 * Authentication requires that the Claimant prove, through a secure 1310 * authentication protocol, that he or she controls the token. The Claimant 1311 * unlocks the token with a password or biometric, or uses a secure multi-token 1312 * authentication protocol to establish two-factor authentication (through proof 1313 * of possession of a physical or software token in combination with some 1314 * memorized secret knowledge). Long-term shared authentication secrets, if 1315 * used, are never revealed to any party except the Claimant and Verifiers 1316 * operated directly by the CSP; however, session (temporary) shared secrets may 1317 * be provided to independent Verifiers by the CSP. In addition to Level 2 1318 * requirements, assertions are protected against repudiation by the Verifier. 1319 */ 1320 LOAAP3, 1321 /** 1322 * Indicator of the high digital quality or reliability of a defined sequence of 1323 * messages between a Claimant and a Verifier that demonstrates that the 1324 * Claimant has possession and control of a valid token to establish his/her 1325 * identity, and optionally, demonstrates to the Claimant that he or she is 1326 * communicating with the intended Verifier. [Based on NIST SP 800-63-2] 1327 * 1328 * High authentication process level of assurance indicates all sensitive data 1329 * transfer are cryptographically authenticated using keys bound to the 1330 * authentication process. Level 4 requires strong cryptographic authentication 1331 * of all communicating parties and all sensitive data transfers between the 1332 * parties. Either public key or symmetric key technology may be used. 1333 * Authentication requires that the Claimant prove through a secure 1334 * authentication protocol that he or she controls the token. All protocol 1335 * threats at Level 3 are required to be prevented at Level 4. Protocols shall 1336 * also be strongly resistant to man-in-the-middle attacks. Long-term shared 1337 * authentication secrets, if used, are never revealed to any party except the 1338 * Claimant and Verifiers operated directly by the CSP; however, session 1339 * (temporary) shared secrets may be provided to independent Verifiers by the 1340 * CSP. Approved cryptographic techniques are used for all operations. All 1341 * sensitive data transfers are cryptographically authenticated using keys bound 1342 * to the authentication process. [Summary of the technical requirements 1343 * specified in NIST SP 800-63 for the four levels of assurance defined by the 1344 * December 2003, the Office of Management and Budget (OMB) issued Memorandum 1345 * M-04-04, E-Authentication Guidance for Federal Agencies.] 1346 */ 1347 LOAAP4, 1348 /** 1349 * The value assigned as the indicator of the high quality or reliability of the 1350 * statement from a Verifier to a Relying Party (RP) that contains identity 1351 * information about a Subscriber. Assertions may also contain verified 1352 * attributes. 1353 */ 1354 LOAAS, 1355 /** 1356 * Indicator of the low quality or reliability of the statement from a Verifier 1357 * to a Relying Party (RP) that contains identity information about a 1358 * Subscriber. Assertions may also contain verified attributes. 1359 * 1360 * Assertions and assertion references require protection from modification and 1361 * reuse attacks. [Summary of the technical requirements specified in NIST SP 1362 * 800-63 for the four levels of assurance defined by the December 2003, the 1363 * Office of Management and Budget (OMB) issued Memorandum M-04-04, 1364 * E-Authentication Guidance for Federal Agencies.] 1365 */ 1366 LOAAS1, 1367 /** 1368 * Indicator of the basic quality or reliability of the statement from a 1369 * Verifier to a Relying Party (RP) that contains identity information about a 1370 * Subscriber. Assertions may also contain verified attributes. 1371 * 1372 * Assertions are resistant to disclosure, redirection, capture and substitution 1373 * attacks. Approved cryptographic techniques are required for all assertion 1374 * protocols. [Summary of the technical requirements specified in NIST SP 800-63 1375 * for the four levels of assurance defined by the December 2003, the Office of 1376 * Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication 1377 * Guidance for Federal Agencies.] 1378 */ 1379 LOAAS2, 1380 /** 1381 * Indicator of the medium quality or reliability of the statement from a 1382 * Verifier to a Relying Party (RP) that contains identity information about a 1383 * Subscriber. Assertions may also contain verified attributes. 1384 * 1385 * Assertions are protected against repudiation by the verifier. [Summary of the 1386 * technical requirements specified in NIST SP 800-63 for the four levels of 1387 * assurance defined by the December 2003, the Office of Management and Budget 1388 * (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal 1389 * Agencies.] 1390 */ 1391 LOAAS3, 1392 /** 1393 * Indicator of the high quality or reliability of the statement from a Verifier 1394 * to a Relying Party (RP) that contains identity information about a 1395 * Subscriber. Assertions may also contain verified attributes. 1396 * 1397 * Strongly resistant to man-in-the-middle attacks. "Bearer" assertions are not 1398 * used. "Holder-of-key" assertions may be used. RP maintains records of the 1399 * assertions. [Summary of the technical requirements specified in NIST SP 1400 * 800-63 for the four levels of assurance defined by the December 2003, the 1401 * Office of Management and Budget (OMB) issued Memorandum M-04-04, 1402 * E-Authentication Guidance for Federal Agencies.] 1403 */ 1404 LOAAS4, 1405 /** 1406 * Indicator of the digital quality or reliability of the activities performed 1407 * by the Credential Service Provider (CSP) subsequent to electronic 1408 * authentication registration, identity proofing and issuance activities to 1409 * manage and safeguard the integrity of an issued credential and its binding to 1410 * an identity. [Electronic Authentication Guideline - Recommendations of the 1411 * National Institute of Standards and Technology, NIST Special Publication 1412 * 800-63-1, Dec 2011] 1413 */ 1414 LOACM, 1415 /** 1416 * Indicator of the low digital quality or reliability of the activities 1417 * performed by the Credential Service Provider (CSP) subsequent to electronic 1418 * authentication registration, identity proofing and issuance activities to 1419 * manage and safeguard the integrity of an issued credential and its binding to 1420 * an identity. Little or no confidence that an individual has maintained 1421 * control over a token that has been entrusted to him or her and that that 1422 * token has not been compromised. Characteristics include weak identity binding 1423 * to tokens and plaintext passwords or secrets not transmitted across a 1424 * network. [Electronic Authentication Guideline - Recommendations of the 1425 * National Institute of Standards and Technology, NIST Special Publication 1426 * 800-63-1, Dec 2011] 1427 */ 1428 LOACM1, 1429 /** 1430 * Indicator of the basic digital quality or reliability of the activities 1431 * performed by the Credential Service Provider (CSP) subsequent to electronic 1432 * authentication registration, identity proofing and issuance activities to 1433 * manage and safeguard the integrity of an issued credential and its binding to 1434 * an identity. Some confidence that an individual has maintained control over a 1435 * token that has been entrusted to him or her and that that token has not been 1436 * compromised. Characteristics include: Verification must prove claimant 1437 * controls the token; token resists online guessing, replay, session hijacking, 1438 * and eavesdropping attacks; and token is at least weakly resistant to 1439 * man-in-the middle attacks. [Electronic Authentication Guideline - 1440 * Recommendations of the National Institute of Standards and Technology, NIST 1441 * Special Publication 800-63-1, Dec 2011] 1442 */ 1443 LOACM2, 1444 /** 1445 * Indicator of the medium digital quality or reliability of the activities 1446 * performed by the Credential Service Provider (CSP) subsequent to electronic 1447 * authentication registration, identity proofing and issuance activities to 1448 * manage and safeguard the integrity of an issued credential and itâ??s binding 1449 * to an identity. High confidence that an individual has maintained control 1450 * over a token that has been entrusted to him or her and that that token has 1451 * not been compromised. Characteristics include: Ownership of token verifiable 1452 * through security authentication protocol and credential management protects 1453 * against verifier impersonation attacks. [Electronic Authentication Guideline 1454 * - Recommendations of the National Institute of Standards and Technology, NIST 1455 * Special Publication 800-63-1, Dec 2011] 1456 */ 1457 LOACM3, 1458 /** 1459 * Indicator of the high digital quality or reliability of the activities 1460 * performed by the Credential Service Provider (CSP) subsequent to electronic 1461 * authentication registration, identity proofing and issuance activities to 1462 * manage and safeguard the integrity of an issued credential and itâ??s binding 1463 * to an identity. Very high confidence that an individual has maintained 1464 * control over a token that has been entrusted to him or her and that that 1465 * token has not been compromised. Characteristics include: Verifier can prove 1466 * control of token through a secure protocol; credential management supports 1467 * strong cryptographic authentication of all communication parties. [Electronic 1468 * Authentication Guideline - Recommendations of the National Institute of 1469 * Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 1470 */ 1471 LOACM4, 1472 /** 1473 * Indicator of the quality or reliability in the process of ascertaining that 1474 * an individual is who he or she claims to be. 1475 */ 1476 LOAID, 1477 /** 1478 * Indicator of low digital quality or reliability in the process of 1479 * ascertaining that an individual is who he or she claims to be. Requires that 1480 * a continuity of identity be maintained but does not require identity 1481 * proofing. [Based on Electronic Authentication Guideline - Recommendations of 1482 * the National Institute of Standards and Technology, NIST Special Publication 1483 * 800-63-1, Dec 2011] 1484 */ 1485 LOAID1, 1486 /** 1487 * Indicator of some digital quality or reliability in the process of 1488 * ascertaining that that an individual is who he or she claims to be. Requires 1489 * identity proofing via presentation of identifying material or information. 1490 * [Based on Electronic Authentication Guideline - Recommendations of the 1491 * National Institute of Standards and Technology, NIST Special Publication 1492 * 800-63-1, Dec 2011] 1493 */ 1494 LOAID2, 1495 /** 1496 * Indicator of high digital quality or reliability in the process of 1497 * ascertaining that an individual is who he or she claims to be. Requires 1498 * identity proofing procedures for verification of identifying materials and 1499 * information. [Based on Electronic Authentication Guideline - Recommendations 1500 * of the National Institute of Standards and Technology, NIST Special 1501 * Publication 800-63-1, Dec 2011] 1502 */ 1503 LOAID3, 1504 /** 1505 * Indicator of high digital quality or reliability in the process of 1506 * ascertaining that an individual is who he or she claims to be. Requires 1507 * identity proofing procedures for verification of identifying materials and 1508 * information. [Based on Electronic Authentication Guideline - Recommendations 1509 * of the National Institute of Standards and Technology, NIST Special 1510 * Publication 800-63-1, Dec 2011] 1511 */ 1512 LOAID4, 1513 /** 1514 * Indicator of the digital quality or reliability in the process of 1515 * establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 1516 */ 1517 LOANR, 1518 /** 1519 * Indicator of low digital quality or reliability in the process of 1520 * establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 1521 */ 1522 LOANR1, 1523 /** 1524 * Indicator of basic digital quality or reliability in the process of 1525 * establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 1526 */ 1527 LOANR2, 1528 /** 1529 * Indicator of medium digital quality or reliability in the process of 1530 * establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 1531 */ 1532 LOANR3, 1533 /** 1534 * Indicator of high digital quality or reliability in the process of 1535 * establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 1536 */ 1537 LOANR4, 1538 /** 1539 * Indicator of the digital quality or reliability of the information exchange 1540 * between network-connected devices where the information cannot be reliably 1541 * protected end-to-end by a single organizationâ??s security controls. [Based 1542 * on NIST SP 800-63-2] 1543 */ 1544 LOARA, 1545 /** 1546 * Indicator of low digital quality or reliability of the information exchange 1547 * between network-connected devices where the information cannot be reliably 1548 * protected end-to-end by a single organizationâ??s security controls. [Based 1549 * on NIST SP 800-63-2] 1550 */ 1551 LOARA1, 1552 /** 1553 * Indicator of basic digital quality or reliability of the information exchange 1554 * between network-connected devices where the information cannot be reliably 1555 * protected end-to-end by a single organizationâ??s security controls. [Based 1556 * on NIST SP 800-63-2] 1557 */ 1558 LOARA2, 1559 /** 1560 * Indicator of medium digital quality or reliability of the information 1561 * exchange between network-connected devices where the information cannot be 1562 * reliably protected end-to-end by a single organizationâ??s security controls. 1563 * [Based on NIST SP 800-63-2] 1564 */ 1565 LOARA3, 1566 /** 1567 * Indicator of high digital quality or reliability of the information exchange 1568 * between network-connected devices where the information cannot be reliably 1569 * protected end-to-end by a single organization's security controls. [Based on 1570 * NIST SP 800-63-2] 1571 */ 1572 LOARA4, 1573 /** 1574 * Indicator of the digital quality or reliability of single and multi-token 1575 * authentication. [Electronic Authentication Guideline - Recommendations of the 1576 * National Institute of Standards and Technology, NIST Special Publication 1577 * 800-63-1, Dec 2011] 1578 */ 1579 LOATK, 1580 /** 1581 * Indicator of the low digital quality or reliability of single and multi-token 1582 * authentication. Permits the use of any of the token methods of Levels 2, 3, 1583 * or 4. [Electronic Authentication Guideline - Recommendations of the National 1584 * Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 1585 * 2011] 1586 */ 1587 LOATK1, 1588 /** 1589 * Indicator of the basic digital quality or reliability of single and 1590 * multi-token authentication. Requires single factor authentication using 1591 * memorized secret tokens, pre-registered knowledge tokens, look-up secret 1592 * tokens, out of band tokens, or single factor one-time password devices. 1593 * [Electronic Authentication Guideline - Recommendations of the National 1594 * Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 1595 * 2011] 1596 */ 1597 LOATK2, 1598 /** 1599 * Indicator of the medium digital quality or reliability of single and 1600 * multi-token authentication. Requires two authentication factors. Provides 1601 * multi-factor remote network authentication. Permits multi-factor software 1602 * cryptographic token. [Electronic Authentication Guideline - Recommendations 1603 * of the National Institute of Standards and Technology, NIST Special 1604 * Publication 800-63-1, Dec 2011] 1605 */ 1606 LOATK3, 1607 /** 1608 * Indicator of the high digital quality or reliability of single and 1609 * multi-token authentication. Requires token that is a hardware cryptographic 1610 * module validated at validated at Federal Information Processing Standard 1611 * (FIPS) 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 1612 * physical security. Level 4 token requirements can be met by using the PIV 1613 * authentication key of a FIPS 201 compliant Personal Identity Verification 1614 * (PIV) Card. [Electronic Authentication Guideline - Recommendations of the 1615 * National Institute of Standards and Technology, NIST Special Publication 1616 * 800-63-1, Dec 2011] 1617 */ 1618 LOATK4, 1619 /** 1620 * Values for security trust mechanism metadata observation made about a 1621 * security architecture system component that supports enforcement of security 1622 * policies. 1623 */ 1624 TRSTMECOBV, 1625 /** 1626 * Potential values for observations of severity. 1627 */ 1628 _SEVERITYOBSERVATION, 1629 /** 1630 * Indicates the condition may be life-threatening or has the potential to cause 1631 * permanent injury. 1632 */ 1633 H, 1634 /** 1635 * Indicates the condition may result in some adverse consequences but is 1636 * unlikely to substantially affect the situation of the subject. 1637 */ 1638 L, 1639 /** 1640 * Indicates the condition may result in noticable adverse adverse consequences 1641 * but is unlikely to be life-threatening or cause permanent injury. 1642 */ 1643 M, 1644 /** 1645 * Contains codes for defining the observed, physical position of a subject, 1646 * such as during an observation, assessment, collection of a specimen, etc. ECG 1647 * waveforms and vital signs, such as blood pressure, are two examples where a 1648 * general, observed position typically needs to be noted. 1649 */ 1650 _SUBJECTBODYPOSITION, 1651 /** 1652 * Lying on the left side. 1653 */ 1654 LLD, 1655 /** 1656 * Lying with the front or ventral surface downward; lying face down. 1657 */ 1658 PRN, 1659 /** 1660 * Lying on the right side. 1661 */ 1662 RLD, 1663 /** 1664 * A semi-sitting position in bed with the head of the bed elevated 1665 * approximately 45 degrees. 1666 */ 1667 SFWL, 1668 /** 1669 * Resting the body on the buttocks, typically with upper torso erect or semi 1670 * erect. 1671 */ 1672 SIT, 1673 /** 1674 * To be stationary, upright, vertical, on one's legs. 1675 */ 1676 STN, 1677 /** 1678 * supine 1679 */ 1680 SUP, 1681 /** 1682 * Lying on the back, on an inclined plane, typically about 30-45 degrees with 1683 * head raised and feet lowered. 1684 */ 1685 RTRD, 1686 /** 1687 * Lying on the back, on an inclined plane, typically about 30-45 degrees, with 1688 * head lowered and feet raised. 1689 */ 1690 TRD, 1691 /** 1692 * Values for observations of verification act results 1693 * 1694 * 1695 * Examples: Verified, not verified, verified with warning. 1696 */ 1697 _VERIFICATIONOUTCOMEVALUE, 1698 /** 1699 * Definition: Coverage is in effect for healthcare service(s) and/or 1700 * product(s). 1701 */ 1702 ACT, 1703 /** 1704 * Definition: Coverage is in effect for healthcare service(s) and/or product(s) 1705 * - Pending Investigation 1706 */ 1707 ACTPEND, 1708 /** 1709 * Definition: Coverage is in effect for healthcare service(s) and/or 1710 * product(s). 1711 */ 1712 ELG, 1713 /** 1714 * Definition: Coverage is not in effect for healthcare service(s) and/or 1715 * product(s). 1716 */ 1717 INACT, 1718 /** 1719 * Definition: Coverage is not in effect for healthcare service(s) and/or 1720 * product(s) - Pending Investigation. 1721 */ 1722 INPNDINV, 1723 /** 1724 * Definition: Coverage is not in effect for healthcare service(s) and/or 1725 * product(s) - Pending Eligibility Update. 1726 */ 1727 INPNDUPD, 1728 /** 1729 * Definition: Coverage is not in effect for healthcare service(s) and/or 1730 * product(s). May optionally include reasons for the ineligibility. 1731 */ 1732 NELG, 1733 /** 1734 * Concepts that describe an individual's typical arrangement of working hours 1735 * for an occupation. 1736 */ 1737 _WORKSCHEDULE, 1738 /** 1739 * A person who is scheduled for work during daytime hours (for example between 1740 * 6am and 6pm) on a regular basis. 1741 */ 1742 DS, 1743 /** 1744 * Consistent Early morning schedule of 13 hours or less per shift (between 2 am 1745 * and 2 pm) 1746 */ 1747 EMS, 1748 /** 1749 * A person who is scheduled for work during evening hours (for example between 1750 * 2pm and midnight) on a regular basis. 1751 */ 1752 ES, 1753 /** 1754 * Scheduled for work during nighttime hours (for example between 9pm and 8am) 1755 * on a regular basis. 1756 */ 1757 NS, 1758 /** 1759 * Scheduled for work times that change periodically between days, and/or 1760 * evenings, and includes some night shifts. 1761 */ 1762 RSWN, 1763 /** 1764 * Scheduled for work days/times that change periodically between days, but does 1765 * not include night or evening work. 1766 */ 1767 RSWON, 1768 /** 1769 * Shift consisting of two distinct work periods each day that are separated by 1770 * a break of a few hours (for example 2 to 4 hours) 1771 */ 1772 SS, 1773 /** 1774 * Shifts of 17 or more hours. 1775 */ 1776 VLS, 1777 /** 1778 * Irregular, unpredictable hours scheduled on a short notice (for example, less 1779 * than 2 day notice): inconsistent schedule, on-call, as needed, as available. 1780 */ 1781 VS, 1782 /** 1783 * AnnotationValue 1784 */ 1785 _ANNOTATIONVALUE, 1786 /** 1787 * Description:Used in a patient care message to value simple clinical (non-lab) 1788 * observations. 1789 */ 1790 _COMMONCLINICALOBSERVATIONVALUE, 1791 /** 1792 * This domain is established as a parent to a variety of value domains being 1793 * defined to support the communication of Individual Case Safety Reports to 1794 * regulatory bodies. Arguably, this aggregation is not taxonomically pure, but 1795 * the grouping will facilitate the management of these domains. 1796 */ 1797 _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS, 1798 /** 1799 * Indicates the specific observation result which is the reason for the action 1800 * (prescription, lab test, etc.). E.g. Headache, Ear infection, planned 1801 * diagnostic image (requiring contrast agent), etc. 1802 */ 1803 _INDICATIONVALUE, 1804 /** 1805 * added to help the parsers 1806 */ 1807 NULL; 1808 1809 public static V3ObservationValue fromCode(String codeString) throws FHIRException { 1810 if (codeString == null || "".equals(codeString)) 1811 return null; 1812 if ("_ActCoverageAssessmentObservationValue".equals(codeString)) 1813 return _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE; 1814 if ("_ActFinancialStatusObservationValue".equals(codeString)) 1815 return _ACTFINANCIALSTATUSOBSERVATIONVALUE; 1816 if ("ASSET".equals(codeString)) 1817 return ASSET; 1818 if ("ANNUITY".equals(codeString)) 1819 return ANNUITY; 1820 if ("PROP".equals(codeString)) 1821 return PROP; 1822 if ("RETACCT".equals(codeString)) 1823 return RETACCT; 1824 if ("TRUST".equals(codeString)) 1825 return TRUST; 1826 if ("INCOME".equals(codeString)) 1827 return INCOME; 1828 if ("CHILD".equals(codeString)) 1829 return CHILD; 1830 if ("DISABL".equals(codeString)) 1831 return DISABL; 1832 if ("INVEST".equals(codeString)) 1833 return INVEST; 1834 if ("PAY".equals(codeString)) 1835 return PAY; 1836 if ("RETIRE".equals(codeString)) 1837 return RETIRE; 1838 if ("SPOUSAL".equals(codeString)) 1839 return SPOUSAL; 1840 if ("SUPPLE".equals(codeString)) 1841 return SUPPLE; 1842 if ("TAX".equals(codeString)) 1843 return TAX; 1844 if ("LIVEXP".equals(codeString)) 1845 return LIVEXP; 1846 if ("CLOTH".equals(codeString)) 1847 return CLOTH; 1848 if ("FOOD".equals(codeString)) 1849 return FOOD; 1850 if ("HEALTH".equals(codeString)) 1851 return HEALTH; 1852 if ("HOUSE".equals(codeString)) 1853 return HOUSE; 1854 if ("LEGAL".equals(codeString)) 1855 return LEGAL; 1856 if ("MORTG".equals(codeString)) 1857 return MORTG; 1858 if ("RENT".equals(codeString)) 1859 return RENT; 1860 if ("SUNDRY".equals(codeString)) 1861 return SUNDRY; 1862 if ("TRANS".equals(codeString)) 1863 return TRANS; 1864 if ("UTIL".equals(codeString)) 1865 return UTIL; 1866 if ("ELSTAT".equals(codeString)) 1867 return ELSTAT; 1868 if ("ADOPT".equals(codeString)) 1869 return ADOPT; 1870 if ("BTHCERT".equals(codeString)) 1871 return BTHCERT; 1872 if ("CCOC".equals(codeString)) 1873 return CCOC; 1874 if ("DRLIC".equals(codeString)) 1875 return DRLIC; 1876 if ("FOSTER".equals(codeString)) 1877 return FOSTER; 1878 if ("MEMBER".equals(codeString)) 1879 return MEMBER; 1880 if ("MIL".equals(codeString)) 1881 return MIL; 1882 if ("MRGCERT".equals(codeString)) 1883 return MRGCERT; 1884 if ("PASSPORT".equals(codeString)) 1885 return PASSPORT; 1886 if ("STUDENRL".equals(codeString)) 1887 return STUDENRL; 1888 if ("HLSTAT".equals(codeString)) 1889 return HLSTAT; 1890 if ("DISABLE".equals(codeString)) 1891 return DISABLE; 1892 if ("DRUG".equals(codeString)) 1893 return DRUG; 1894 if ("IVDRG".equals(codeString)) 1895 return IVDRG; 1896 if ("PGNT".equals(codeString)) 1897 return PGNT; 1898 if ("LIVDEP".equals(codeString)) 1899 return LIVDEP; 1900 if ("RELDEP".equals(codeString)) 1901 return RELDEP; 1902 if ("SPSDEP".equals(codeString)) 1903 return SPSDEP; 1904 if ("URELDEP".equals(codeString)) 1905 return URELDEP; 1906 if ("LIVSIT".equals(codeString)) 1907 return LIVSIT; 1908 if ("ALONE".equals(codeString)) 1909 return ALONE; 1910 if ("DEPCHD".equals(codeString)) 1911 return DEPCHD; 1912 if ("DEPSPS".equals(codeString)) 1913 return DEPSPS; 1914 if ("DEPYGCHD".equals(codeString)) 1915 return DEPYGCHD; 1916 if ("FAM".equals(codeString)) 1917 return FAM; 1918 if ("RELAT".equals(codeString)) 1919 return RELAT; 1920 if ("SPS".equals(codeString)) 1921 return SPS; 1922 if ("UNREL".equals(codeString)) 1923 return UNREL; 1924 if ("SOECSTAT".equals(codeString)) 1925 return SOECSTAT; 1926 if ("ABUSE".equals(codeString)) 1927 return ABUSE; 1928 if ("HMLESS".equals(codeString)) 1929 return HMLESS; 1930 if ("ILGIM".equals(codeString)) 1931 return ILGIM; 1932 if ("INCAR".equals(codeString)) 1933 return INCAR; 1934 if ("PROB".equals(codeString)) 1935 return PROB; 1936 if ("REFUG".equals(codeString)) 1937 return REFUG; 1938 if ("UNEMPL".equals(codeString)) 1939 return UNEMPL; 1940 if ("_AllergyTestValue".equals(codeString)) 1941 return _ALLERGYTESTVALUE; 1942 if ("A0".equals(codeString)) 1943 return A0; 1944 if ("A1".equals(codeString)) 1945 return A1; 1946 if ("A2".equals(codeString)) 1947 return A2; 1948 if ("A3".equals(codeString)) 1949 return A3; 1950 if ("A4".equals(codeString)) 1951 return A4; 1952 if ("_CompositeMeasureScoring".equals(codeString)) 1953 return _COMPOSITEMEASURESCORING; 1954 if ("ALLORNONESCR".equals(codeString)) 1955 return ALLORNONESCR; 1956 if ("LINEARSCR".equals(codeString)) 1957 return LINEARSCR; 1958 if ("OPPORSCR".equals(codeString)) 1959 return OPPORSCR; 1960 if ("WEIGHTSCR".equals(codeString)) 1961 return WEIGHTSCR; 1962 if ("_CoverageLimitObservationValue".equals(codeString)) 1963 return _COVERAGELIMITOBSERVATIONVALUE; 1964 if ("_CoverageLevelObservationValue".equals(codeString)) 1965 return _COVERAGELEVELOBSERVATIONVALUE; 1966 if ("ADC".equals(codeString)) 1967 return ADC; 1968 if ("CHD".equals(codeString)) 1969 return CHD; 1970 if ("DEP".equals(codeString)) 1971 return DEP; 1972 if ("DP".equals(codeString)) 1973 return DP; 1974 if ("ECH".equals(codeString)) 1975 return ECH; 1976 if ("FLY".equals(codeString)) 1977 return FLY; 1978 if ("IND".equals(codeString)) 1979 return IND; 1980 if ("SSP".equals(codeString)) 1981 return SSP; 1982 if ("_CriticalityObservationValue".equals(codeString)) 1983 return _CRITICALITYOBSERVATIONVALUE; 1984 if ("CRITH".equals(codeString)) 1985 return CRITH; 1986 if ("CRITL".equals(codeString)) 1987 return CRITL; 1988 if ("CRITU".equals(codeString)) 1989 return CRITU; 1990 if ("_EmploymentStatus".equals(codeString)) 1991 return _EMPLOYMENTSTATUS; 1992 if ("Employed".equals(codeString)) 1993 return EMPLOYED; 1994 if ("NotInLaborForce".equals(codeString)) 1995 return NOTINLABORFORCE; 1996 if ("Unemployed".equals(codeString)) 1997 return UNEMPLOYED; 1998 if ("_GeneticObservationValue".equals(codeString)) 1999 return _GENETICOBSERVATIONVALUE; 2000 if ("Homozygote".equals(codeString)) 2001 return HOMOZYGOTE; 2002 if ("_ObservationMeasureScoring".equals(codeString)) 2003 return _OBSERVATIONMEASURESCORING; 2004 if ("COHORT".equals(codeString)) 2005 return COHORT; 2006 if ("CONTVAR".equals(codeString)) 2007 return CONTVAR; 2008 if ("PROPOR".equals(codeString)) 2009 return PROPOR; 2010 if ("RATIO".equals(codeString)) 2011 return RATIO; 2012 if ("_ObservationMeasureType".equals(codeString)) 2013 return _OBSERVATIONMEASURETYPE; 2014 if ("COMPOSITE".equals(codeString)) 2015 return COMPOSITE; 2016 if ("EFFICIENCY".equals(codeString)) 2017 return EFFICIENCY; 2018 if ("EXPERIENCE".equals(codeString)) 2019 return EXPERIENCE; 2020 if ("OUTCOME".equals(codeString)) 2021 return OUTCOME; 2022 if ("INTERM-OM".equals(codeString)) 2023 return INTERMOM; 2024 if ("PRO-PM".equals(codeString)) 2025 return PROPM; 2026 if ("PROCESS".equals(codeString)) 2027 return PROCESS; 2028 if ("APPROPRIATE".equals(codeString)) 2029 return APPROPRIATE; 2030 if ("RESOURCE".equals(codeString)) 2031 return RESOURCE; 2032 if ("STRUCTURE".equals(codeString)) 2033 return STRUCTURE; 2034 if ("_ObservationPopulationInclusion".equals(codeString)) 2035 return _OBSERVATIONPOPULATIONINCLUSION; 2036 if ("DENEX".equals(codeString)) 2037 return DENEX; 2038 if ("DENEXCEP".equals(codeString)) 2039 return DENEXCEP; 2040 if ("DENOM".equals(codeString)) 2041 return DENOM; 2042 if ("IP".equals(codeString)) 2043 return IP; 2044 if ("IPP".equals(codeString)) 2045 return IPP; 2046 if ("MSRPOPL".equals(codeString)) 2047 return MSRPOPL; 2048 if ("NUMER".equals(codeString)) 2049 return NUMER; 2050 if ("NUMEX".equals(codeString)) 2051 return NUMEX; 2052 if ("_PartialCompletionScale".equals(codeString)) 2053 return _PARTIALCOMPLETIONSCALE; 2054 if ("G".equals(codeString)) 2055 return G; 2056 if ("LE".equals(codeString)) 2057 return LE; 2058 if ("ME".equals(codeString)) 2059 return ME; 2060 if ("MI".equals(codeString)) 2061 return MI; 2062 if ("N".equals(codeString)) 2063 return N; 2064 if ("S".equals(codeString)) 2065 return S; 2066 if ("_SecurityObservationValue".equals(codeString)) 2067 return _SECURITYOBSERVATIONVALUE; 2068 if ("_SECINTOBV".equals(codeString)) 2069 return _SECINTOBV; 2070 if ("_SECALTINTOBV".equals(codeString)) 2071 return _SECALTINTOBV; 2072 if ("ABSTRED".equals(codeString)) 2073 return ABSTRED; 2074 if ("AGGRED".equals(codeString)) 2075 return AGGRED; 2076 if ("ANONYED".equals(codeString)) 2077 return ANONYED; 2078 if ("MAPPED".equals(codeString)) 2079 return MAPPED; 2080 if ("MASKED".equals(codeString)) 2081 return MASKED; 2082 if ("PSEUDED".equals(codeString)) 2083 return PSEUDED; 2084 if ("REDACTED".equals(codeString)) 2085 return REDACTED; 2086 if ("SUBSETTED".equals(codeString)) 2087 return SUBSETTED; 2088 if ("SYNTAC".equals(codeString)) 2089 return SYNTAC; 2090 if ("TRSLT".equals(codeString)) 2091 return TRSLT; 2092 if ("VERSIONED".equals(codeString)) 2093 return VERSIONED; 2094 if ("_SECDATINTOBV".equals(codeString)) 2095 return _SECDATINTOBV; 2096 if ("CRYTOHASH".equals(codeString)) 2097 return CRYTOHASH; 2098 if ("DIGSIG".equals(codeString)) 2099 return DIGSIG; 2100 if ("_SECINTCONOBV".equals(codeString)) 2101 return _SECINTCONOBV; 2102 if ("HRELIABLE".equals(codeString)) 2103 return HRELIABLE; 2104 if ("RELIABLE".equals(codeString)) 2105 return RELIABLE; 2106 if ("UNCERTREL".equals(codeString)) 2107 return UNCERTREL; 2108 if ("UNRELIABLE".equals(codeString)) 2109 return UNRELIABLE; 2110 if ("_SECINTPRVOBV".equals(codeString)) 2111 return _SECINTPRVOBV; 2112 if ("_SECINTPRVABOBV".equals(codeString)) 2113 return _SECINTPRVABOBV; 2114 if ("CLINAST".equals(codeString)) 2115 return CLINAST; 2116 if ("DEVAST".equals(codeString)) 2117 return DEVAST; 2118 if ("HCPAST".equals(codeString)) 2119 return HCPAST; 2120 if ("PACQAST".equals(codeString)) 2121 return PACQAST; 2122 if ("PATAST".equals(codeString)) 2123 return PATAST; 2124 if ("PAYAST".equals(codeString)) 2125 return PAYAST; 2126 if ("PROAST".equals(codeString)) 2127 return PROAST; 2128 if ("SDMAST".equals(codeString)) 2129 return SDMAST; 2130 if ("_SECINTPRVRBOBV".equals(codeString)) 2131 return _SECINTPRVRBOBV; 2132 if ("CLINRPT".equals(codeString)) 2133 return CLINRPT; 2134 if ("DEVRPT".equals(codeString)) 2135 return DEVRPT; 2136 if ("HCPRPT".equals(codeString)) 2137 return HCPRPT; 2138 if ("PACQRPT".equals(codeString)) 2139 return PACQRPT; 2140 if ("PATRPT".equals(codeString)) 2141 return PATRPT; 2142 if ("PAYRPT".equals(codeString)) 2143 return PAYRPT; 2144 if ("PRORPT".equals(codeString)) 2145 return PRORPT; 2146 if ("SDMRPT".equals(codeString)) 2147 return SDMRPT; 2148 if ("SECTRSTOBV".equals(codeString)) 2149 return SECTRSTOBV; 2150 if ("TRSTACCRDOBV".equals(codeString)) 2151 return TRSTACCRDOBV; 2152 if ("TRSTAGREOBV".equals(codeString)) 2153 return TRSTAGREOBV; 2154 if ("TRSTCERTOBV".equals(codeString)) 2155 return TRSTCERTOBV; 2156 if ("TRSTLOAOBV".equals(codeString)) 2157 return TRSTLOAOBV; 2158 if ("LOAAN".equals(codeString)) 2159 return LOAAN; 2160 if ("LOAAN1".equals(codeString)) 2161 return LOAAN1; 2162 if ("LOAAN2".equals(codeString)) 2163 return LOAAN2; 2164 if ("LOAAN3".equals(codeString)) 2165 return LOAAN3; 2166 if ("LOAAN4".equals(codeString)) 2167 return LOAAN4; 2168 if ("LOAAP".equals(codeString)) 2169 return LOAAP; 2170 if ("LOAAP1".equals(codeString)) 2171 return LOAAP1; 2172 if ("LOAAP2".equals(codeString)) 2173 return LOAAP2; 2174 if ("LOAAP3".equals(codeString)) 2175 return LOAAP3; 2176 if ("LOAAP4".equals(codeString)) 2177 return LOAAP4; 2178 if ("LOAAS".equals(codeString)) 2179 return LOAAS; 2180 if ("LOAAS1".equals(codeString)) 2181 return LOAAS1; 2182 if ("LOAAS2".equals(codeString)) 2183 return LOAAS2; 2184 if ("LOAAS3".equals(codeString)) 2185 return LOAAS3; 2186 if ("LOAAS4".equals(codeString)) 2187 return LOAAS4; 2188 if ("LOACM".equals(codeString)) 2189 return LOACM; 2190 if ("LOACM1".equals(codeString)) 2191 return LOACM1; 2192 if ("LOACM2".equals(codeString)) 2193 return LOACM2; 2194 if ("LOACM3".equals(codeString)) 2195 return LOACM3; 2196 if ("LOACM4".equals(codeString)) 2197 return LOACM4; 2198 if ("LOAID".equals(codeString)) 2199 return LOAID; 2200 if ("LOAID1".equals(codeString)) 2201 return LOAID1; 2202 if ("LOAID2".equals(codeString)) 2203 return LOAID2; 2204 if ("LOAID3".equals(codeString)) 2205 return LOAID3; 2206 if ("LOAID4".equals(codeString)) 2207 return LOAID4; 2208 if ("LOANR".equals(codeString)) 2209 return LOANR; 2210 if ("LOANR1".equals(codeString)) 2211 return LOANR1; 2212 if ("LOANR2".equals(codeString)) 2213 return LOANR2; 2214 if ("LOANR3".equals(codeString)) 2215 return LOANR3; 2216 if ("LOANR4".equals(codeString)) 2217 return LOANR4; 2218 if ("LOARA".equals(codeString)) 2219 return LOARA; 2220 if ("LOARA1".equals(codeString)) 2221 return LOARA1; 2222 if ("LOARA2".equals(codeString)) 2223 return LOARA2; 2224 if ("LOARA3".equals(codeString)) 2225 return LOARA3; 2226 if ("LOARA4".equals(codeString)) 2227 return LOARA4; 2228 if ("LOATK".equals(codeString)) 2229 return LOATK; 2230 if ("LOATK1".equals(codeString)) 2231 return LOATK1; 2232 if ("LOATK2".equals(codeString)) 2233 return LOATK2; 2234 if ("LOATK3".equals(codeString)) 2235 return LOATK3; 2236 if ("LOATK4".equals(codeString)) 2237 return LOATK4; 2238 if ("TRSTMECOBV".equals(codeString)) 2239 return TRSTMECOBV; 2240 if ("_SeverityObservation".equals(codeString)) 2241 return _SEVERITYOBSERVATION; 2242 if ("H".equals(codeString)) 2243 return H; 2244 if ("L".equals(codeString)) 2245 return L; 2246 if ("M".equals(codeString)) 2247 return M; 2248 if ("_SubjectBodyPosition".equals(codeString)) 2249 return _SUBJECTBODYPOSITION; 2250 if ("LLD".equals(codeString)) 2251 return LLD; 2252 if ("PRN".equals(codeString)) 2253 return PRN; 2254 if ("RLD".equals(codeString)) 2255 return RLD; 2256 if ("SFWL".equals(codeString)) 2257 return SFWL; 2258 if ("SIT".equals(codeString)) 2259 return SIT; 2260 if ("STN".equals(codeString)) 2261 return STN; 2262 if ("SUP".equals(codeString)) 2263 return SUP; 2264 if ("RTRD".equals(codeString)) 2265 return RTRD; 2266 if ("TRD".equals(codeString)) 2267 return TRD; 2268 if ("_VerificationOutcomeValue".equals(codeString)) 2269 return _VERIFICATIONOUTCOMEVALUE; 2270 if ("ACT".equals(codeString)) 2271 return ACT; 2272 if ("ACTPEND".equals(codeString)) 2273 return ACTPEND; 2274 if ("ELG".equals(codeString)) 2275 return ELG; 2276 if ("INACT".equals(codeString)) 2277 return INACT; 2278 if ("INPNDINV".equals(codeString)) 2279 return INPNDINV; 2280 if ("INPNDUPD".equals(codeString)) 2281 return INPNDUPD; 2282 if ("NELG".equals(codeString)) 2283 return NELG; 2284 if ("_WorkSchedule".equals(codeString)) 2285 return _WORKSCHEDULE; 2286 if ("DS".equals(codeString)) 2287 return DS; 2288 if ("EMS".equals(codeString)) 2289 return EMS; 2290 if ("ES".equals(codeString)) 2291 return ES; 2292 if ("NS".equals(codeString)) 2293 return NS; 2294 if ("RSWN".equals(codeString)) 2295 return RSWN; 2296 if ("RSWON".equals(codeString)) 2297 return RSWON; 2298 if ("SS".equals(codeString)) 2299 return SS; 2300 if ("VLS".equals(codeString)) 2301 return VLS; 2302 if ("VS".equals(codeString)) 2303 return VS; 2304 if ("_AnnotationValue".equals(codeString)) 2305 return _ANNOTATIONVALUE; 2306 if ("_CommonClinicalObservationValue".equals(codeString)) 2307 return _COMMONCLINICALOBSERVATIONVALUE; 2308 if ("_IndividualCaseSafetyReportValueDomains".equals(codeString)) 2309 return _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS; 2310 if ("_IndicationValue".equals(codeString)) 2311 return _INDICATIONVALUE; 2312 throw new FHIRException("Unknown V3ObservationValue code '" + codeString + "'"); 2313 } 2314 2315 public String toCode() { 2316 switch (this) { 2317 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: 2318 return "_ActCoverageAssessmentObservationValue"; 2319 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: 2320 return "_ActFinancialStatusObservationValue"; 2321 case ASSET: 2322 return "ASSET"; 2323 case ANNUITY: 2324 return "ANNUITY"; 2325 case PROP: 2326 return "PROP"; 2327 case RETACCT: 2328 return "RETACCT"; 2329 case TRUST: 2330 return "TRUST"; 2331 case INCOME: 2332 return "INCOME"; 2333 case CHILD: 2334 return "CHILD"; 2335 case DISABL: 2336 return "DISABL"; 2337 case INVEST: 2338 return "INVEST"; 2339 case PAY: 2340 return "PAY"; 2341 case RETIRE: 2342 return "RETIRE"; 2343 case SPOUSAL: 2344 return "SPOUSAL"; 2345 case SUPPLE: 2346 return "SUPPLE"; 2347 case TAX: 2348 return "TAX"; 2349 case LIVEXP: 2350 return "LIVEXP"; 2351 case CLOTH: 2352 return "CLOTH"; 2353 case FOOD: 2354 return "FOOD"; 2355 case HEALTH: 2356 return "HEALTH"; 2357 case HOUSE: 2358 return "HOUSE"; 2359 case LEGAL: 2360 return "LEGAL"; 2361 case MORTG: 2362 return "MORTG"; 2363 case RENT: 2364 return "RENT"; 2365 case SUNDRY: 2366 return "SUNDRY"; 2367 case TRANS: 2368 return "TRANS"; 2369 case UTIL: 2370 return "UTIL"; 2371 case ELSTAT: 2372 return "ELSTAT"; 2373 case ADOPT: 2374 return "ADOPT"; 2375 case BTHCERT: 2376 return "BTHCERT"; 2377 case CCOC: 2378 return "CCOC"; 2379 case DRLIC: 2380 return "DRLIC"; 2381 case FOSTER: 2382 return "FOSTER"; 2383 case MEMBER: 2384 return "MEMBER"; 2385 case MIL: 2386 return "MIL"; 2387 case MRGCERT: 2388 return "MRGCERT"; 2389 case PASSPORT: 2390 return "PASSPORT"; 2391 case STUDENRL: 2392 return "STUDENRL"; 2393 case HLSTAT: 2394 return "HLSTAT"; 2395 case DISABLE: 2396 return "DISABLE"; 2397 case DRUG: 2398 return "DRUG"; 2399 case IVDRG: 2400 return "IVDRG"; 2401 case PGNT: 2402 return "PGNT"; 2403 case LIVDEP: 2404 return "LIVDEP"; 2405 case RELDEP: 2406 return "RELDEP"; 2407 case SPSDEP: 2408 return "SPSDEP"; 2409 case URELDEP: 2410 return "URELDEP"; 2411 case LIVSIT: 2412 return "LIVSIT"; 2413 case ALONE: 2414 return "ALONE"; 2415 case DEPCHD: 2416 return "DEPCHD"; 2417 case DEPSPS: 2418 return "DEPSPS"; 2419 case DEPYGCHD: 2420 return "DEPYGCHD"; 2421 case FAM: 2422 return "FAM"; 2423 case RELAT: 2424 return "RELAT"; 2425 case SPS: 2426 return "SPS"; 2427 case UNREL: 2428 return "UNREL"; 2429 case SOECSTAT: 2430 return "SOECSTAT"; 2431 case ABUSE: 2432 return "ABUSE"; 2433 case HMLESS: 2434 return "HMLESS"; 2435 case ILGIM: 2436 return "ILGIM"; 2437 case INCAR: 2438 return "INCAR"; 2439 case PROB: 2440 return "PROB"; 2441 case REFUG: 2442 return "REFUG"; 2443 case UNEMPL: 2444 return "UNEMPL"; 2445 case _ALLERGYTESTVALUE: 2446 return "_AllergyTestValue"; 2447 case A0: 2448 return "A0"; 2449 case A1: 2450 return "A1"; 2451 case A2: 2452 return "A2"; 2453 case A3: 2454 return "A3"; 2455 case A4: 2456 return "A4"; 2457 case _COMPOSITEMEASURESCORING: 2458 return "_CompositeMeasureScoring"; 2459 case ALLORNONESCR: 2460 return "ALLORNONESCR"; 2461 case LINEARSCR: 2462 return "LINEARSCR"; 2463 case OPPORSCR: 2464 return "OPPORSCR"; 2465 case WEIGHTSCR: 2466 return "WEIGHTSCR"; 2467 case _COVERAGELIMITOBSERVATIONVALUE: 2468 return "_CoverageLimitObservationValue"; 2469 case _COVERAGELEVELOBSERVATIONVALUE: 2470 return "_CoverageLevelObservationValue"; 2471 case ADC: 2472 return "ADC"; 2473 case CHD: 2474 return "CHD"; 2475 case DEP: 2476 return "DEP"; 2477 case DP: 2478 return "DP"; 2479 case ECH: 2480 return "ECH"; 2481 case FLY: 2482 return "FLY"; 2483 case IND: 2484 return "IND"; 2485 case SSP: 2486 return "SSP"; 2487 case _CRITICALITYOBSERVATIONVALUE: 2488 return "_CriticalityObservationValue"; 2489 case CRITH: 2490 return "CRITH"; 2491 case CRITL: 2492 return "CRITL"; 2493 case CRITU: 2494 return "CRITU"; 2495 case _EMPLOYMENTSTATUS: 2496 return "_EmploymentStatus"; 2497 case EMPLOYED: 2498 return "Employed"; 2499 case NOTINLABORFORCE: 2500 return "NotInLaborForce"; 2501 case UNEMPLOYED: 2502 return "Unemployed"; 2503 case _GENETICOBSERVATIONVALUE: 2504 return "_GeneticObservationValue"; 2505 case HOMOZYGOTE: 2506 return "Homozygote"; 2507 case _OBSERVATIONMEASURESCORING: 2508 return "_ObservationMeasureScoring"; 2509 case COHORT: 2510 return "COHORT"; 2511 case CONTVAR: 2512 return "CONTVAR"; 2513 case PROPOR: 2514 return "PROPOR"; 2515 case RATIO: 2516 return "RATIO"; 2517 case _OBSERVATIONMEASURETYPE: 2518 return "_ObservationMeasureType"; 2519 case COMPOSITE: 2520 return "COMPOSITE"; 2521 case EFFICIENCY: 2522 return "EFFICIENCY"; 2523 case EXPERIENCE: 2524 return "EXPERIENCE"; 2525 case OUTCOME: 2526 return "OUTCOME"; 2527 case INTERMOM: 2528 return "INTERM-OM"; 2529 case PROPM: 2530 return "PRO-PM"; 2531 case PROCESS: 2532 return "PROCESS"; 2533 case APPROPRIATE: 2534 return "APPROPRIATE"; 2535 case RESOURCE: 2536 return "RESOURCE"; 2537 case STRUCTURE: 2538 return "STRUCTURE"; 2539 case _OBSERVATIONPOPULATIONINCLUSION: 2540 return "_ObservationPopulationInclusion"; 2541 case DENEX: 2542 return "DENEX"; 2543 case DENEXCEP: 2544 return "DENEXCEP"; 2545 case DENOM: 2546 return "DENOM"; 2547 case IP: 2548 return "IP"; 2549 case IPP: 2550 return "IPP"; 2551 case MSRPOPL: 2552 return "MSRPOPL"; 2553 case NUMER: 2554 return "NUMER"; 2555 case NUMEX: 2556 return "NUMEX"; 2557 case _PARTIALCOMPLETIONSCALE: 2558 return "_PartialCompletionScale"; 2559 case G: 2560 return "G"; 2561 case LE: 2562 return "LE"; 2563 case ME: 2564 return "ME"; 2565 case MI: 2566 return "MI"; 2567 case N: 2568 return "N"; 2569 case S: 2570 return "S"; 2571 case _SECURITYOBSERVATIONVALUE: 2572 return "_SecurityObservationValue"; 2573 case _SECINTOBV: 2574 return "_SECINTOBV"; 2575 case _SECALTINTOBV: 2576 return "_SECALTINTOBV"; 2577 case ABSTRED: 2578 return "ABSTRED"; 2579 case AGGRED: 2580 return "AGGRED"; 2581 case ANONYED: 2582 return "ANONYED"; 2583 case MAPPED: 2584 return "MAPPED"; 2585 case MASKED: 2586 return "MASKED"; 2587 case PSEUDED: 2588 return "PSEUDED"; 2589 case REDACTED: 2590 return "REDACTED"; 2591 case SUBSETTED: 2592 return "SUBSETTED"; 2593 case SYNTAC: 2594 return "SYNTAC"; 2595 case TRSLT: 2596 return "TRSLT"; 2597 case VERSIONED: 2598 return "VERSIONED"; 2599 case _SECDATINTOBV: 2600 return "_SECDATINTOBV"; 2601 case CRYTOHASH: 2602 return "CRYTOHASH"; 2603 case DIGSIG: 2604 return "DIGSIG"; 2605 case _SECINTCONOBV: 2606 return "_SECINTCONOBV"; 2607 case HRELIABLE: 2608 return "HRELIABLE"; 2609 case RELIABLE: 2610 return "RELIABLE"; 2611 case UNCERTREL: 2612 return "UNCERTREL"; 2613 case UNRELIABLE: 2614 return "UNRELIABLE"; 2615 case _SECINTPRVOBV: 2616 return "_SECINTPRVOBV"; 2617 case _SECINTPRVABOBV: 2618 return "_SECINTPRVABOBV"; 2619 case CLINAST: 2620 return "CLINAST"; 2621 case DEVAST: 2622 return "DEVAST"; 2623 case HCPAST: 2624 return "HCPAST"; 2625 case PACQAST: 2626 return "PACQAST"; 2627 case PATAST: 2628 return "PATAST"; 2629 case PAYAST: 2630 return "PAYAST"; 2631 case PROAST: 2632 return "PROAST"; 2633 case SDMAST: 2634 return "SDMAST"; 2635 case _SECINTPRVRBOBV: 2636 return "_SECINTPRVRBOBV"; 2637 case CLINRPT: 2638 return "CLINRPT"; 2639 case DEVRPT: 2640 return "DEVRPT"; 2641 case HCPRPT: 2642 return "HCPRPT"; 2643 case PACQRPT: 2644 return "PACQRPT"; 2645 case PATRPT: 2646 return "PATRPT"; 2647 case PAYRPT: 2648 return "PAYRPT"; 2649 case PRORPT: 2650 return "PRORPT"; 2651 case SDMRPT: 2652 return "SDMRPT"; 2653 case SECTRSTOBV: 2654 return "SECTRSTOBV"; 2655 case TRSTACCRDOBV: 2656 return "TRSTACCRDOBV"; 2657 case TRSTAGREOBV: 2658 return "TRSTAGREOBV"; 2659 case TRSTCERTOBV: 2660 return "TRSTCERTOBV"; 2661 case TRSTLOAOBV: 2662 return "TRSTLOAOBV"; 2663 case LOAAN: 2664 return "LOAAN"; 2665 case LOAAN1: 2666 return "LOAAN1"; 2667 case LOAAN2: 2668 return "LOAAN2"; 2669 case LOAAN3: 2670 return "LOAAN3"; 2671 case LOAAN4: 2672 return "LOAAN4"; 2673 case LOAAP: 2674 return "LOAAP"; 2675 case LOAAP1: 2676 return "LOAAP1"; 2677 case LOAAP2: 2678 return "LOAAP2"; 2679 case LOAAP3: 2680 return "LOAAP3"; 2681 case LOAAP4: 2682 return "LOAAP4"; 2683 case LOAAS: 2684 return "LOAAS"; 2685 case LOAAS1: 2686 return "LOAAS1"; 2687 case LOAAS2: 2688 return "LOAAS2"; 2689 case LOAAS3: 2690 return "LOAAS3"; 2691 case LOAAS4: 2692 return "LOAAS4"; 2693 case LOACM: 2694 return "LOACM"; 2695 case LOACM1: 2696 return "LOACM1"; 2697 case LOACM2: 2698 return "LOACM2"; 2699 case LOACM3: 2700 return "LOACM3"; 2701 case LOACM4: 2702 return "LOACM4"; 2703 case LOAID: 2704 return "LOAID"; 2705 case LOAID1: 2706 return "LOAID1"; 2707 case LOAID2: 2708 return "LOAID2"; 2709 case LOAID3: 2710 return "LOAID3"; 2711 case LOAID4: 2712 return "LOAID4"; 2713 case LOANR: 2714 return "LOANR"; 2715 case LOANR1: 2716 return "LOANR1"; 2717 case LOANR2: 2718 return "LOANR2"; 2719 case LOANR3: 2720 return "LOANR3"; 2721 case LOANR4: 2722 return "LOANR4"; 2723 case LOARA: 2724 return "LOARA"; 2725 case LOARA1: 2726 return "LOARA1"; 2727 case LOARA2: 2728 return "LOARA2"; 2729 case LOARA3: 2730 return "LOARA3"; 2731 case LOARA4: 2732 return "LOARA4"; 2733 case LOATK: 2734 return "LOATK"; 2735 case LOATK1: 2736 return "LOATK1"; 2737 case LOATK2: 2738 return "LOATK2"; 2739 case LOATK3: 2740 return "LOATK3"; 2741 case LOATK4: 2742 return "LOATK4"; 2743 case TRSTMECOBV: 2744 return "TRSTMECOBV"; 2745 case _SEVERITYOBSERVATION: 2746 return "_SeverityObservation"; 2747 case H: 2748 return "H"; 2749 case L: 2750 return "L"; 2751 case M: 2752 return "M"; 2753 case _SUBJECTBODYPOSITION: 2754 return "_SubjectBodyPosition"; 2755 case LLD: 2756 return "LLD"; 2757 case PRN: 2758 return "PRN"; 2759 case RLD: 2760 return "RLD"; 2761 case SFWL: 2762 return "SFWL"; 2763 case SIT: 2764 return "SIT"; 2765 case STN: 2766 return "STN"; 2767 case SUP: 2768 return "SUP"; 2769 case RTRD: 2770 return "RTRD"; 2771 case TRD: 2772 return "TRD"; 2773 case _VERIFICATIONOUTCOMEVALUE: 2774 return "_VerificationOutcomeValue"; 2775 case ACT: 2776 return "ACT"; 2777 case ACTPEND: 2778 return "ACTPEND"; 2779 case ELG: 2780 return "ELG"; 2781 case INACT: 2782 return "INACT"; 2783 case INPNDINV: 2784 return "INPNDINV"; 2785 case INPNDUPD: 2786 return "INPNDUPD"; 2787 case NELG: 2788 return "NELG"; 2789 case _WORKSCHEDULE: 2790 return "_WorkSchedule"; 2791 case DS: 2792 return "DS"; 2793 case EMS: 2794 return "EMS"; 2795 case ES: 2796 return "ES"; 2797 case NS: 2798 return "NS"; 2799 case RSWN: 2800 return "RSWN"; 2801 case RSWON: 2802 return "RSWON"; 2803 case SS: 2804 return "SS"; 2805 case VLS: 2806 return "VLS"; 2807 case VS: 2808 return "VS"; 2809 case _ANNOTATIONVALUE: 2810 return "_AnnotationValue"; 2811 case _COMMONCLINICALOBSERVATIONVALUE: 2812 return "_CommonClinicalObservationValue"; 2813 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: 2814 return "_IndividualCaseSafetyReportValueDomains"; 2815 case _INDICATIONVALUE: 2816 return "_IndicationValue"; 2817 case NULL: 2818 return null; 2819 default: 2820 return "?"; 2821 } 2822 } 2823 2824 public String getSystem() { 2825 return "http://terminology.hl7.org/CodeSystem/v3-ObservationValue"; 2826 } 2827 2828 public String getDefinition() { 2829 switch (this) { 2830 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: 2831 return "Codes specify the category of observation, evidence, or document used to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program. The type of evidence is coded as observation values."; 2832 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: 2833 return "Code specifying financial indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay stub; tax or income document; asset document; living expenses."; 2834 case ASSET: 2835 return "Codes specifying asset indicators used to assess or establish eligibility for coverage under a policy or program."; 2836 case ANNUITY: 2837 return "Indicator of annuity ownership or status as beneficiary."; 2838 case PROP: 2839 return "Indicator of real property ownership, e.g., deed or real estate contract."; 2840 case RETACCT: 2841 return "Indicator of retirement investment account ownership."; 2842 case TRUST: 2843 return "Indicator of status as trust beneficiary."; 2844 case INCOME: 2845 return "Code specifying income indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay or pension check, child support payments received or provided, and taxes paid."; 2846 case CHILD: 2847 return "Indicator of child support payments received or provided."; 2848 case DISABL: 2849 return "Indicator of disability income replacement payment."; 2850 case INVEST: 2851 return "Indicator of investment income, e.g., dividend check, annuity payment; real estate rent, investment divestiture proceeds; trust or endowment check."; 2852 case PAY: 2853 return "Indicator of paid employment, e.g., letter of hire, contract, employer letter; copy of pay check or pay stub."; 2854 case RETIRE: 2855 return "Indicator of retirement payment, e.g., pension check."; 2856 case SPOUSAL: 2857 return "Indicator of spousal or partner support payments received or provided; e.g., alimony payment; support stipulations in a divorce settlement."; 2858 case SUPPLE: 2859 return "Indicator of income supplement, e.g., gifting, parental income support; stipend, or grant."; 2860 case TAX: 2861 return "Indicator of tax obligation or payment, e.g., statement of taxable income."; 2862 case LIVEXP: 2863 return "Codes specifying living expense indicators used to assess or establish eligibility for coverage under a policy or program."; 2864 case CLOTH: 2865 return "Indicator of clothing expenses."; 2866 case FOOD: 2867 return "Indicator of transportation expenses."; 2868 case HEALTH: 2869 return "Indicator of health expenses; including medication costs, health service costs, financial participations, and health coverage premiums."; 2870 case HOUSE: 2871 return "Indicator of housing expense, e.g., household appliances, fixtures, furnishings, and maintenance and repairs."; 2872 case LEGAL: 2873 return "Indicator of legal expenses."; 2874 case MORTG: 2875 return "Indicator of mortgage amount, interest, and payments."; 2876 case RENT: 2877 return "Indicator of rental or lease payments."; 2878 case SUNDRY: 2879 return "Indicator of transportation expenses."; 2880 case TRANS: 2881 return "Indicator of transportation expenses, e.g., vehicle payments, vehicle insurance, vehicle fuel, and vehicle maintenance and repairs."; 2882 case UTIL: 2883 return "Indicator of transportation expenses."; 2884 case ELSTAT: 2885 return "Code specifying eligibility indicators used to assess or establish eligibility for coverage under a policy or program eligibility status, e.g., certificates of creditable coverage; student enrollment; adoption, marriage or birth certificate."; 2886 case ADOPT: 2887 return "Indicator of adoption."; 2888 case BTHCERT: 2889 return "Indicator of birth."; 2890 case CCOC: 2891 return "Indicator of creditable coverage."; 2892 case DRLIC: 2893 return "Indicator of driving status."; 2894 case FOSTER: 2895 return "Indicator of foster child status."; 2896 case MEMBER: 2897 return "Indicator of status as covered member under a policy or program, e.g., member id card or coverage document."; 2898 case MIL: 2899 return "Indicator of military status."; 2900 case MRGCERT: 2901 return "Indicator of marriage status."; 2902 case PASSPORT: 2903 return "Indicator of citizenship."; 2904 case STUDENRL: 2905 return "Indicator of student status."; 2906 case HLSTAT: 2907 return "Code specifying non-clinical indicators related to health status used to assess or establish eligibility for coverage under a policy or program, e.g., pregnancy, disability, drug use, mental health issues."; 2908 case DISABLE: 2909 return "Indication of disability."; 2910 case DRUG: 2911 return "Indication of drug use."; 2912 case IVDRG: 2913 return "Indication of IV drug use ."; 2914 case PGNT: 2915 return "Non-clinical report of pregnancy."; 2916 case LIVDEP: 2917 return "Code specifying observations related to living dependency, such as dependent upon spouse for activities of daily living."; 2918 case RELDEP: 2919 return "Continued living in private residence requires functional and health care assistance from one or more relatives."; 2920 case SPSDEP: 2921 return "Continued living in private residence requires functional and health care assistance from spouse or life partner."; 2922 case URELDEP: 2923 return "Continued living in private residence requires functional and health care assistance from one or more unrelated persons."; 2924 case LIVSIT: 2925 return "Code specifying observations related to living situation for a person in a private residence."; 2926 case ALONE: 2927 return "Living alone. Maps to PD1-2 Living arrangement (IS) 00742 [A]"; 2928 case DEPCHD: 2929 return "Living with one or more dependent children requiring moderate supervision."; 2930 case DEPSPS: 2931 return "Living with disabled spouse requiring functional and health care assistance"; 2932 case DEPYGCHD: 2933 return "Living with one or more dependent children requiring intensive supervision"; 2934 case FAM: 2935 return "Living with family. Maps to PD1-2 Living arrangement (IS) 00742 [F]"; 2936 case RELAT: 2937 return "Living with one or more relatives. Maps to PD1-2 Living arrangement (IS) 00742 [R]"; 2938 case SPS: 2939 return "Living only with spouse or life partner. Maps to PD1-2 Living arrangement (IS) 00742 [S]"; 2940 case UNREL: 2941 return "Living with one or more unrelated persons."; 2942 case SOECSTAT: 2943 return "Code specifying observations or indicators related to socio-economic status used to assess to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program."; 2944 case ABUSE: 2945 return "Indication of abuse victim."; 2946 case HMLESS: 2947 return "Indication of status as homeless."; 2948 case ILGIM: 2949 return "Indication of status as illegal immigrant."; 2950 case INCAR: 2951 return "Indication of status as incarcerated."; 2952 case PROB: 2953 return "Indication of probation status."; 2954 case REFUG: 2955 return "Indication of refugee status."; 2956 case UNEMPL: 2957 return "Indication of unemployed status."; 2958 case _ALLERGYTESTVALUE: 2959 return "Indicates the result of a particular allergy test. E.g. Negative, Mild, Moderate, Severe"; 2960 case A0: 2961 return "Description:Patient exhibits no reaction to the challenge agent."; 2962 case A1: 2963 return "Description:Patient exhibits a minimal reaction to the challenge agent."; 2964 case A2: 2965 return "Description:Patient exhibits a mild reaction to the challenge agent."; 2966 case A3: 2967 return "Description:Patient exhibits moderate reaction to the challenge agent."; 2968 case A4: 2969 return "Description:Patient exhibits a severe reaction to the challenge agent."; 2970 case _COMPOSITEMEASURESCORING: 2971 return "Observation values that communicate the method used in a quality measure to combine the component measure results included in an composite measure."; 2972 case ALLORNONESCR: 2973 return "Code specifying that the measure uses all-or-nothing scoring. All-or-nothing scoring places an individual in the numerator of the composite measure if and only if they are in the numerator of all component measures in which they are in the denominator."; 2974 case LINEARSCR: 2975 return "Code specifying that the measure uses linear scoring. Linear scoring computes the fraction of component measures in which the individual appears in the numerator, giving equal weight to each component measure."; 2976 case OPPORSCR: 2977 return "Code specifying that the measure uses opportunity-based scoring. In opportunity-based scoring the measure score is determined by combining the denominator and numerator of each component measure to determine an overall composite score."; 2978 case WEIGHTSCR: 2979 return "Code specifying that the measure uses weighted scoring. Weighted scoring assigns a factor to each component measure to weight that measure's contribution to the overall score."; 2980 case _COVERAGELIMITOBSERVATIONVALUE: 2981 return "Description:Coded observation values for coverage limitations, for e.g., types of claims or types of parties covered under a policy or program."; 2982 case _COVERAGELEVELOBSERVATIONVALUE: 2983 return "Description:Coded observation values for types of covered parties under a policy or program based on their personal relationships or employment status."; 2984 case ADC: 2985 return "Description:Child over an age as specified by coverage policy or program, e.g., student, differently abled, and income dependent."; 2986 case CHD: 2987 return "Description:Dependent biological, adopted, foster child as specified by coverage policy or program."; 2988 case DEP: 2989 return "Description:Person requiring functional and/or financial assistance from another person as specified by coverage policy or program."; 2990 case DP: 2991 return "Description:Persons registered as a family unit in a domestic partner registry as specified by law and by coverage policy or program."; 2992 case ECH: 2993 return "Description:An individual employed by an employer who receive remuneration in wages, salary, commission, tips, piece-rates, or pay-in-kind through the employeraTMs payment system (i.e., not a contractor) as specified by coverage policy or program."; 2994 case FLY: 2995 return "Description:As specified by coverage policy or program."; 2996 case IND: 2997 return "Description:Person as specified by coverage policy or program."; 2998 case SSP: 2999 return "Description:A pair of people of the same gender who live together as a family as specified by coverage policy or program, e.g., Naomi and Ruth from the Book of Ruth; Socrates and Alcibiades"; 3000 case _CRITICALITYOBSERVATIONVALUE: 3001 return "A clinical judgment as to the worst case result of a future exposure (including substance administration). When the worst case result is assessed to have a life-threatening or organ system threatening potential, it is considered to be of high criticality."; 3002 case CRITH: 3003 return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 3004 case CRITL: 3005 return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 3006 case CRITU: 3007 return "Unable to assess the worst case result of a future exposure."; 3008 case _EMPLOYMENTSTATUS: 3009 return "Concepts representing whether a person does or does not currently have a job or is not currently in the labor pool seeking employment."; 3010 case EMPLOYED: 3011 return "Individuals who, during the last week: a) did any work for at least 1 hour as paid or unpaid employees of a business or government organization; worked in their own businesses, professions, or on their own farms; or b) were not working, but who have a job or business from which the individual was temporarily absent because of vacation, illness, bad weather, childcare problems, maternity or paternity leave, labor-management dispute, job training, or other family or personal reasons, regardless of whether or not they were paid for the time off or were seeking other jobs."; 3012 case NOTINLABORFORCE: 3013 return "Persons not classified as employed or unemployed, meaning those who have no job and are not looking for one."; 3014 case UNEMPLOYED: 3015 return "Persons who currently have no employment, but are available for work and have made specific efforts to find employment."; 3016 case _GENETICOBSERVATIONVALUE: 3017 return "Description: The domain contains genetic analysis specific observation values, e.g. Homozygote, Heterozygote, etc."; 3018 case HOMOZYGOTE: 3019 return "Description: An individual having different alleles at one or more loci regarding a specific character"; 3020 case _OBSERVATIONMEASURESCORING: 3021 return "Observation values used to indicate the type of scoring (e.g. proportion, ratio) used by a health quality measure."; 3022 case COHORT: 3023 return "A measure in which either short-term cross-section or long-term longitudinal analysis is performed over a group of subjects defined by a set of common properties or defining characteristics (e.g., Male smokers between the ages of 40 and 50 years, exposure to treatment, exposure duration)."; 3024 case CONTVAR: 3025 return "A measure score in which each individual value for the measure can fall anywhere along a continuous scale (e.g., mean time to thrombolytics which aggregates the time in minutes from a case presenting with chest pain to the time of administration of thrombolytics)."; 3026 case PROPOR: 3027 return "A score derived by dividing the number of cases that meet a criterion for quality (the numerator) by the number of eligible cases within a given time frame (the denominator) where the numerator cases are a subset of the denominator cases (e.g., percentage of eligible women with a mammogram performed in the last year)."; 3028 case RATIO: 3029 return "A score that may have a value of zero or greater that is derived by dividing a count of one type of data by a count of another type of data (e.g., the number of patients with central lines who develop infection divided by the number of central line days)."; 3030 case _OBSERVATIONMEASURETYPE: 3031 return "Observation values used to indicate what kind of health quality measure is used."; 3032 case COMPOSITE: 3033 return "A measure that is composed from one or more other measures and indicates an overall summary of those measures."; 3034 case EFFICIENCY: 3035 return "A measure related to the efficiency of medical treatment."; 3036 case EXPERIENCE: 3037 return "A measure related to the level of patient engagement or patient experience of care."; 3038 case OUTCOME: 3039 return "A measure that indicates the result of the performance (or non-performance) of a function or process."; 3040 case INTERMOM: 3041 return "A measure that evaluates the change over time of a physiologic state observable that is associated with a specific long-term health outcome."; 3042 case PROPM: 3043 return "A measure that is a comparison of patient reported outcomes for a single or multiple patients collected via an instrument specifically designed to obtain input directly from patients."; 3044 case PROCESS: 3045 return "A measure which focuses on a process which leads to a certain outcome, meaning that a scientific basis exists for believing that the process, when executed well, will increase the probability of achieving a desired outcome."; 3046 case APPROPRIATE: 3047 return "A measure that assesses the use of one or more processes where the expected health benefit exceeds the expected negative consequences."; 3048 case RESOURCE: 3049 return "A measure related to the extent of use of clinical resources or cost of care."; 3050 case STRUCTURE: 3051 return "A measure related to the structure of patient care."; 3052 case _OBSERVATIONPOPULATIONINCLUSION: 3053 return "Observation values used to assert various populations that a subject falls into."; 3054 case DENEX: 3055 return "Patients who should be removed from the eMeasure population and denominator before determining if numerator criteria are met. Denominator exclusions are used in proportion and ratio measures to help narrow the denominator."; 3056 case DENEXCEP: 3057 return "Denominator exceptions are those conditions that should remove a patient, procedure or unit of measurement from the denominator only if the numerator criteria are not met. Denominator exceptions allow for adjustment of the calculated score for those providers with higher risk populations. Denominator exceptions are used only in proportion eMeasures. They are not appropriate for ratio or continuous variable eMeasures. Denominator exceptions allow for the exercise of clinical judgment and should be specifically defined where capturing the information in a structured manner fits the clinical workflow. Generic denominator exception reasons used in proportion eMeasures fall into three general categories:\r\n\n \n Medical reasons\n Patient reasons\n System reasons"; 3058 case DENOM: 3059 return "It can be the same as the initial patient population or a subset of the initial patient population to further constrain the population for the purpose of the eMeasure. Different measures within an eMeasure set may have different Denominators. Continuous Variable eMeasures do not have a Denominator, but instead define a Measure Population."; 3060 case IP: 3061 return "The initial population refers to all entities to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs."; 3062 case IPP: 3063 return "The initial patient population refers to all patients to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs. Details often include information based upon specific age groups, diagnoses, diagnostic and procedure codes, and enrollment periods."; 3064 case MSRPOPL: 3065 return "Measure population is used only in continuous variable eMeasures. It is a narrative description of the eMeasure population. \n(e.g., all patients seen in the Emergency Department during the measurement period)."; 3066 case NUMER: 3067 return "Numerators are used in proportion and ratio eMeasures. In proportion measures the numerator criteria are the processes or outcomes expected for each patient, procedure, or other unit of measurement defined in the denominator. In ratio measures the numerator is related, but not directly derived from the denominator (e.g., a numerator listing the number of central line blood stream infections and a denominator indicating the days per thousand of central line usage in a specific time period)."; 3068 case NUMEX: 3069 return "Numerator Exclusions are used only in ratio eMeasures to define instances that should not be included in the numerator data. (e.g., if the number of central line blood stream infections per 1000 catheter days were to exclude infections with a specific bacterium, that bacterium would be listed as a numerator exclusion.)"; 3070 case _PARTIALCOMPLETIONSCALE: 3071 return "PartialCompletionScale"; 3072 case G: 3073 return "Value for Act.partialCompletionCode attribute that implies 81-99% completion"; 3074 case LE: 3075 return "Value for Act.partialCompletionCode attribute that implies 61-80% completion"; 3076 case ME: 3077 return "Value for Act.partialCompletionCode attribute that implies 41-60% completion"; 3078 case MI: 3079 return "Value for Act.partialCompletionCode attribute that implies 1-20% completion"; 3080 case N: 3081 return "Value for Act.partialCompletionCode attribute that implies 0% completion"; 3082 case S: 3083 return "Value for Act.partialCompletionCode attribute that implies 21-40% completion"; 3084 case _SECURITYOBSERVATIONVALUE: 3085 return "Observation values used to indicate security observation metadata."; 3086 case _SECINTOBV: 3087 return "Abstract security observation values used to indicate security integrity metadata.\r\n\n \n Examples: Codes conveying integrity status, integrity confidence, and provenance."; 3088 case _SECALTINTOBV: 3089 return "Abstract security metadata observation values used to indicate mechanism used for authorized alteration of an IT resource (data, information object, service, or system capability)"; 3090 case ABSTRED: 3091 return "Security metadata observation values used to indicate the use of a more abstract version of the content, e.g., replacing exact value of an age or date field with a range, or remove the left digits of a credit card number or SSN."; 3092 case AGGRED: 3093 return "Security metadata observation values used to indicate the use of an algorithmic combination of actual values with the result of an aggregate function, e.g., average, sum, or count in order to limit disclosure of an IT resource (data, information object, service, or system capability) to the minimum necessary."; 3094 case ANONYED: 3095 return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by used to indicate the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. No key to relink the data is retained."; 3096 case MAPPED: 3097 return "Security metadata observation value used to indicate that the IT resource semantic content has been transformed from one encoding to another.\r\n\n \n Usage Note: \"MAP\" code does not indicate the semantic fidelity of the transformed content.\r\n\n To indicate semantic fidelity for maps of HL7 to other code systems, this security alteration integrity observation may be further specified using an Act valued with Value Set: MapRelationship (2.16.840.1.113883.1.11.11052).\r\n\n Semantic fidelity of the mapped IT Resource may also be indicated using a SecurityIntegrityConfidenceObservation."; 3098 case MASKED: 3099 return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by indicating the mechanism by which software systems can make data unintelligible (that is, as unreadable and unusable by algorithmically transforming plaintext into ciphertext) such that it can only be accessed or used by authorized users. An authorized user may be provided a key to decrypt per license or \"shared secret\".\r\n\n \n Usage Note: \"MASKED\" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has been further encrypted, and may be accessed only by an authorized user or receiver to which a decryption key is provided."; 3100 case PSEUDED: 3101 return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability), by indicating the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. Custodian may retain a key to relink data necessary to reidentify the information subject.\r\n\n \n Rationale: Personal data which has been processed to make it impossible to know whose data it is. Used particularly for secondary use of health data. In some cases, it may be possible for authorized individuals to restore the identity of the individual, e.g.,for public health case management. Based on ISO/TS 25237:2008 Health informaticsâ??Pseudonymization"; 3102 case REDACTED: 3103 return "Security metadata observation value used to indicate the mechanism by which software systems can filter an IT resource (data, information object, service, or system capability) to remove any portion of the resource that is not authorized to be access, used, or disclosed.\r\n\n \n Usage Note: \"REDACTED\" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has filtered and not included in the content accessed or received."; 3104 case SUBSETTED: 3105 return "Metadata observation used to indicate that some information has been removed from the source object when the view this object contains was constructed because of configuration options when the view was created. The content may not be suitable for use as the basis of a record update\r\n\n \n Usage Note: This is not suitable to be used when information is removed for security reasons - see the code REDACTED for this use."; 3106 case SYNTAC: 3107 return "Security metadata observation value used to indicate that the IT resource syntax has been transformed from one syntactical representation to another. \r\n\n \n Usage Note: \"SYNTAC\" code does not indicate the syntactical correctness of the syntactically transformed IT resource."; 3108 case TRSLT: 3109 return "Security metadata observation value used to indicate that the IT resource has been translated from one human language to another. \r\n\n \n Usage Note: \"TRSLT\" does not indicate the fidelity of the translation or the languages translated.\r\n\n The fidelity of the IT Resource translation may be indicated using a SecurityIntegrityConfidenceObservation.\r\n\n To indicate languages, use the Value Set:HumanLanguage (2.16.840.1.113883.1.11.11526)"; 3110 case VERSIONED: 3111 return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) which indicates that the resource only retains versions of an IT resource for access and use per applicable policy\r\n\n \n Usage Note: When this code is used, expectation is that the system has removed historical versions of the data that falls outside the time period deemed to be the effective time of the applicable version."; 3112 case _SECDATINTOBV: 3113 return "Abstract security observation values used to indicate data integrity metadata.\r\n\n \n Examples: Codes conveying the mechanism used to preserve the accuracy and consistency of an IT resource such as a digital signature and a cryptographic hash function."; 3114 case CRYTOHASH: 3115 return "Security metadata observation value used to indicate the mechanism by which software systems can establish that data was not modified in transit.\r\n\n \n Rationale: This definition is intended to align with the ISO 22600-2 3.3.19 definition of cryptographic checkvalue: Information which is derived by performing a cryptographic transformation (see cryptography) on the data unit. The derivation of the checkvalue may be performed in one or more steps and is a result of a mathematical function of the key and a data unit. It is usually used to check the integrity of a data unit.\r\n\n \n Examples: \n \r\n\n \n SHA-1\n SHA-2 (Secure Hash Algorithm)"; 3116 case DIGSIG: 3117 return "Security metadata observation value used to indicate the mechanism by which software systems use digital signature to establish that data has not been modified. \r\n\n \n Rationale: This definition is intended to align with the ISO 22600-2 3.3.26 definition of digital signature: Data appended to, or a cryptographic transformation (see cryptography) of, a data unit that allows a recipient of the data unit to prove the source and integrity of the data unit and protect against forgery e.g., by the recipient."; 3118 case _SECINTCONOBV: 3119 return "Abstract security observation value used to indicate integrity confidence metadata.\r\n\n \n Examples: Codes conveying the level of reliability and trustworthiness of an IT resource."; 3120 case HRELIABLE: 3121 return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be very high."; 3122 case RELIABLE: 3123 return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be adequate."; 3124 case UNCERTREL: 3125 return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be uncertain."; 3126 case UNRELIABLE: 3127 return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be inadequate."; 3128 case _SECINTPRVOBV: 3129 return "Abstract security metadata observation value used to indicate the provenance of an IT resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity reporting an IT resource."; 3130 case _SECINTPRVABOBV: 3131 return "Abstract security provenance metadata observation value used to indicate the entity that asserted an IT resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity asserting the resource."; 3132 case CLINAST: 3133 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a clinician."; 3134 case DEVAST: 3135 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a device."; 3136 case HCPAST: 3137 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a healthcare professional."; 3138 case PACQAST: 3139 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient acquaintance."; 3140 case PATAST: 3141 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient."; 3142 case PAYAST: 3143 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a payer."; 3144 case PROAST: 3145 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a professional."; 3146 case SDMAST: 3147 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a substitute decision maker."; 3148 case _SECINTPRVRBOBV: 3149 return "Abstract security provenance metadata observation value used to indicate the entity that reported the resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity reporting an IT resource."; 3150 case CLINRPT: 3151 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a clinician."; 3152 case DEVRPT: 3153 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a device."; 3154 case HCPRPT: 3155 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a healthcare professional."; 3156 case PACQRPT: 3157 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient acquaintance."; 3158 case PATRPT: 3159 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient."; 3160 case PAYRPT: 3161 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a payer."; 3162 case PRORPT: 3163 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a professional."; 3164 case SDMRPT: 3165 return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a substitute decision maker."; 3166 case SECTRSTOBV: 3167 return "Observation value used to indicate aspects of trust applicable to an IT resource (data, information object, service, or system capability)."; 3168 case TRSTACCRDOBV: 3169 return "Values for security trust accreditation metadata observation made about the formal declaration by an authority or neutral third party that validates the technical, security, trust, and business practice conformance of Trust Agents to facilitate security, interoperability, and trust among participants within a security domain or trust framework."; 3170 case TRSTAGREOBV: 3171 return "Values for security trust agreement metadata observation made about privacy and security requirements with which a security domain must comply. [ISO IEC 10181-1]\n[ISO IEC 10181-1]"; 3172 case TRSTCERTOBV: 3173 return "Values for security trust certificate metadata observation made about a set of security-relevant data issued by a security authority or trusted third party, together with security information which is used to provide the integrity and data origin authentication services for an IT resource (data, information object, service, or system capability). [Based on ISO IEC 10181-1]\r\n\n For example, a Certificate Policy (CP), which is a named set of rules that indicates the applicability of a certificate to a particular community and/or class of application with common security requirements. A particular Certificate Policy might indicate the applicability of a type of certificate to the authentication of electronic data interchange transactions for the trading of goods within a given price range. Another example is Cross Certification with Federal Bridge."; 3174 case TRSTLOAOBV: 3175 return "Values for security trust assurance metadata observation made about the digital quality or reliability of a trust assertion, activity, capability, information exchange, mechanism, process, or protocol."; 3176 case LOAAN: 3177 return "The value assigned as the indicator of the digital quality or reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2]\r\n\n For example, the degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]"; 3178 case LOAAN1: 3179 return "Indicator of low digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] \r\n\n Low authentication level of assurance indicates that the relying party may have little or no confidence in the asserted identity's validity. Level 1 requires little or no confidence in the asserted identity. No identity proofing is required at this level, but the authentication mechanism should provide some assurance that the same claimant is accessing the protected transaction or data. A wide range of available authentication technologies can be employed and any of the token methods of Levels 2, 3, or 4, including Personal Identification Numbers (PINs), may be used. To be authenticated, the claimant must prove control of the token through a secure authentication protocol. At Level 1, long-term shared authentication secrets may be revealed to verifiers. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3180 case LOAAN2: 3181 return "Indicator of basic digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]\r\n\n Basic authentication level of assurance indicates that the relying party may have some confidence in the asserted identity's validity. Level 2 requires confidence that the asserted identity is accurate. Level 2 provides for single-factor remote network authentication, including identity-proofing requirements for presentation of identifying materials or information. A wide range of available authentication technologies can be employed, including any of the token methods of Levels 3 or 4, as well as passwords. Successful authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, and online guessing attacks are prevented. \nLong-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are required. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3182 case LOAAN3: 3183 return "Indicator of medium digital quality or reliability of the digital reliability of verification and validation of the process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] \r\n\n Medium authentication level of assurance indicates that the relying party may have high confidence in the asserted identity's validity. Level 3 is appropriate for transactions that need high confidence in the accuracy of the asserted identity. Level 3 provides multifactor remote network authentication. At this level, identity-proofing procedures require verification of identifying materials and information. Authentication is based on proof of possession of a key or password through a cryptographic protocol. Cryptographic strength mechanisms should protect the primary authentication token (a cryptographic key) against compromise by the protocol threats, including eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks. A minimum of two authentication factors is required. Three kinds of tokens may be used:\r\n\n \n \"soft\" cryptographic token, which has the key stored on a general-purpose computer, \n \"hard\" cryptographic token, which has the key stored on a special hardware device, and \n \"one-time password\" device token, which has symmetric key stored on a personal hardware device that is a cryptographic module validated at FIPS 140-2 Level 1 or higher. Validation testing of cryptographic modules and algorithms for conformance to Federal Information Processing Standard (FIPS) 140-2, Security Requirements for Cryptographic Modules, is managed by NIST.\n \n Authentication requires that the claimant prove control of the token through a secure authentication protocol. The token must be unlocked with a password or biometric representation, or a password must be used in a secure authentication protocol, to establish two-factor authentication. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are used for all operations. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3184 case LOAAN4: 3185 return "Indicator of high digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]\r\n\n High authentication level of assurance indicates that the relying party may have very high confidence in the asserted identity's validity. Level 4 is for transactions that need very high confidence in the accuracy of the asserted identity. Level 4 provides the highest practical assurance of remote network authentication. Authentication is based on proof of possession of a key through a cryptographic protocol. This level is similar to Level 3 except that only â??hardâ?? cryptographic tokens are allowed, cryptographic module validation requirements are strengthened, and subsequent critical data transfers must be authenticated via a key that is bound to the authentication process. The token should be a hardware cryptographic module validated at FIPS 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. This level requires a physical token, which cannot readily be copied, and operator authentication at Level 2 and higher, and ensures good, two-factor remote authentication.\r\n\n Level 4 requires strong cryptographic authentication of all parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks are prevented. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Strong approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3186 case LOAAP: 3187 return "The value assigned as the indicator of the digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]"; 3188 case LOAAP1: 3189 return "Indicator of the low digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Low authentication process level of assurance indicates that (1) long-term shared authentication secrets may be revealed to verifiers; and (2) assertions and assertion references require protection from manufacture/modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3190 case LOAAP2: 3191 return "Indicator of the basic digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Basic authentication process level of assurance indicates that long-term shared authentication secrets are never revealed to any other party except Credential Service Provider (CSP). Sessions (temporary) shared secrets may be provided to independent verifiers by CSP. Long-term shared authentication secrets, if used, are never revealed to any other party except Verifiers operated by the Credential Service Provider (CSP); however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 1 requirements, assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3192 case LOAAP3: 3193 return "Indicator of the medium digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Medium authentication process level of assurance indicates that the token can be unlocked with password, biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication. Long-term shared authentication secrets are never revealed to any party except the Claimant and Credential Service Provider (CSP).\r\n\n Authentication requires that the Claimant prove, through a secure authentication protocol, that he or she controls the token. The Claimant unlocks the token with a password or biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication (through proof of possession of a physical or software token in combination with some memorized secret knowledge). Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 2 requirements, assertions are protected against repudiation by the Verifier."; 3194 case LOAAP4: 3195 return "Indicator of the high digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n High authentication process level of assurance indicates all sensitive data transfer are cryptographically authenticated using keys bound to the authentication process. Level 4 requires strong cryptographic authentication of all communicating parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the Claimant prove through a secure authentication protocol that he or she controls the token. All protocol threats at Level 3 are required to be prevented at Level 4. Protocols shall also be strongly resistant to man-in-the-middle attacks. Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. Approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3196 case LOAAS: 3197 return "The value assigned as the indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes."; 3198 case LOAAS1: 3199 return "Indicator of the low quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions and assertion references require protection from modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3200 case LOAAS2: 3201 return "Indicator of the basic quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required for all assertion protocols. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3202 case LOAAS3: 3203 return "Indicator of the medium quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions are protected against repudiation by the verifier. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3204 case LOAAS4: 3205 return "Indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Strongly resistant to man-in-the-middle attacks. \"Bearer\" assertions are not used. \"Holder-of-key\" assertions may be used. RP maintains records of the assertions. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 3206 case LOACM: 3207 return "Indicator of the digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3208 case LOACM1: 3209 return "Indicator of the low digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Little or no confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include weak identity binding to tokens and plaintext passwords or secrets not transmitted across a network. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3210 case LOACM2: 3211 return "Indicator of the basic digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Some confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verification must prove claimant controls the token; token resists online guessing, replay, session hijacking, and eavesdropping attacks; and token is at least weakly resistant to man-in-the middle attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3212 case LOACM3: 3213 return "Indicator of the medium digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itâ??s binding to an identity. High confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Ownership of token verifiable through security authentication protocol and credential management protects against verifier impersonation attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3214 case LOACM4: 3215 return "Indicator of the high digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itâ??s binding to an identity. Very high confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verifier can prove control of token through a secure protocol; credential management supports strong cryptographic authentication of all communication parties. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3216 case LOAID: 3217 return "Indicator of the quality or reliability in the process of ascertaining that an individual is who he or she claims to be."; 3218 case LOAID1: 3219 return "Indicator of low digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires that a continuity of identity be maintained but does not require identity proofing. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3220 case LOAID2: 3221 return "Indicator of some digital quality or reliability in the process of ascertaining that that an individual is who he or she claims to be. Requires identity proofing via presentation of identifying material or information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3222 case LOAID3: 3223 return "Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3224 case LOAID4: 3225 return "Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3226 case LOANR: 3227 return "Indicator of the digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 3228 case LOANR1: 3229 return "Indicator of low digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 3230 case LOANR2: 3231 return "Indicator of basic digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 3232 case LOANR3: 3233 return "Indicator of medium digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 3234 case LOANR4: 3235 return "Indicator of high digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 3236 case LOARA: 3237 return "Indicator of the digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationâ??s security controls. [Based on NIST SP 800-63-2]"; 3238 case LOARA1: 3239 return "Indicator of low digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationâ??s security controls. [Based on NIST SP 800-63-2]"; 3240 case LOARA2: 3241 return "Indicator of basic digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationâ??s security controls. [Based on NIST SP 800-63-2]"; 3242 case LOARA3: 3243 return "Indicator of medium digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationâ??s security controls. [Based on NIST SP 800-63-2]"; 3244 case LOARA4: 3245 return "Indicator of high digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organization's security controls. [Based on NIST SP 800-63-2]"; 3246 case LOATK: 3247 return "Indicator of the digital quality or reliability of single and multi-token authentication. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3248 case LOATK1: 3249 return "Indicator of the low digital quality or reliability of single and multi-token authentication. Permits the use of any of the token methods of Levels 2, 3, or 4. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3250 case LOATK2: 3251 return "Indicator of the basic digital quality or reliability of single and multi-token authentication. Requires single factor authentication using memorized secret tokens, pre-registered knowledge tokens, look-up secret tokens, out of band tokens, or single factor one-time password devices. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3252 case LOATK3: 3253 return "Indicator of the medium digital quality or reliability of single and multi-token authentication. Requires two authentication factors. Provides multi-factor remote network authentication. Permits multi-factor software cryptographic token. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3254 case LOATK4: 3255 return "Indicator of the high digital quality or reliability of single and multi-token authentication. Requires token that is a hardware cryptographic module validated at validated at Federal Information Processing Standard (FIPS) 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. Level 4 token requirements can be met by using the PIV authentication key of a FIPS 201 compliant Personal Identity Verification (PIV) Card. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 3256 case TRSTMECOBV: 3257 return "Values for security trust mechanism metadata observation made about a security architecture system component that supports enforcement of security policies."; 3258 case _SEVERITYOBSERVATION: 3259 return "Potential values for observations of severity."; 3260 case H: 3261 return "Indicates the condition may be life-threatening or has the potential to cause permanent injury."; 3262 case L: 3263 return "Indicates the condition may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 3264 case M: 3265 return "Indicates the condition may result in noticable adverse adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 3266 case _SUBJECTBODYPOSITION: 3267 return "Contains codes for defining the observed, physical position of a subject, such as during an observation, assessment, collection of a specimen, etc. ECG waveforms and vital signs, such as blood pressure, are two examples where a general, observed position typically needs to be noted."; 3268 case LLD: 3269 return "Lying on the left side."; 3270 case PRN: 3271 return "Lying with the front or ventral surface downward; lying face down."; 3272 case RLD: 3273 return "Lying on the right side."; 3274 case SFWL: 3275 return "A semi-sitting position in bed with the head of the bed elevated approximately 45 degrees."; 3276 case SIT: 3277 return "Resting the body on the buttocks, typically with upper torso erect or semi erect."; 3278 case STN: 3279 return "To be stationary, upright, vertical, on one's legs."; 3280 case SUP: 3281 return "supine"; 3282 case RTRD: 3283 return "Lying on the back, on an inclined plane, typically about 30-45 degrees with head raised and feet lowered."; 3284 case TRD: 3285 return "Lying on the back, on an inclined plane, typically about 30-45 degrees, with head lowered and feet raised."; 3286 case _VERIFICATIONOUTCOMEVALUE: 3287 return "Values for observations of verification act results\r\n\n \n Examples: Verified, not verified, verified with warning."; 3288 case ACT: 3289 return "Definition: Coverage is in effect for healthcare service(s) and/or product(s)."; 3290 case ACTPEND: 3291 return "Definition: Coverage is in effect for healthcare service(s) and/or product(s) - Pending Investigation"; 3292 case ELG: 3293 return "Definition: Coverage is in effect for healthcare service(s) and/or product(s)."; 3294 case INACT: 3295 return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s)."; 3296 case INPNDINV: 3297 return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Investigation."; 3298 case INPNDUPD: 3299 return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Eligibility Update."; 3300 case NELG: 3301 return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s). May optionally include reasons for the ineligibility."; 3302 case _WORKSCHEDULE: 3303 return "Concepts that describe an individual's typical arrangement of working hours for an occupation."; 3304 case DS: 3305 return "A person who is scheduled for work during daytime hours (for example between 6am and 6pm) on a regular basis."; 3306 case EMS: 3307 return "Consistent Early morning schedule of 13 hours or less per shift (between 2 am and 2 pm)"; 3308 case ES: 3309 return "A person who is scheduled for work during evening hours (for example between 2pm and midnight) on a regular basis."; 3310 case NS: 3311 return "Scheduled for work during nighttime hours (for example between 9pm and 8am) on a regular basis."; 3312 case RSWN: 3313 return "Scheduled for work times that change periodically between days, and/or evenings, and includes some night shifts."; 3314 case RSWON: 3315 return "Scheduled for work days/times that change periodically between days, but does not include night or evening work."; 3316 case SS: 3317 return "Shift consisting of two distinct work periods each day that are separated by a break of a few hours (for example 2 to 4 hours)"; 3318 case VLS: 3319 return "Shifts of 17 or more hours."; 3320 case VS: 3321 return "Irregular, unpredictable hours scheduled on a short notice (for example, less than 2 day notice): inconsistent schedule, on-call, as needed, as available."; 3322 case _ANNOTATIONVALUE: 3323 return "AnnotationValue"; 3324 case _COMMONCLINICALOBSERVATIONVALUE: 3325 return "Description:Used in a patient care message to value simple clinical (non-lab) observations."; 3326 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: 3327 return "This domain is established as a parent to a variety of value domains being defined to support the communication of Individual Case Safety Reports to regulatory bodies. Arguably, this aggregation is not taxonomically pure, but the grouping will facilitate the management of these domains."; 3328 case _INDICATIONVALUE: 3329 return "Indicates the specific observation result which is the reason for the action (prescription, lab test, etc.). E.g. Headache, Ear infection, planned diagnostic image (requiring contrast agent), etc."; 3330 case NULL: 3331 return null; 3332 default: 3333 return "?"; 3334 } 3335 } 3336 3337 public String getDisplay() { 3338 switch (this) { 3339 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: 3340 return "ActCoverageAssessmentObservationValue"; 3341 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: 3342 return "ActFinancialStatusObservationValue"; 3343 case ASSET: 3344 return "asset"; 3345 case ANNUITY: 3346 return "annuity"; 3347 case PROP: 3348 return "real property"; 3349 case RETACCT: 3350 return "retirement investment account"; 3351 case TRUST: 3352 return "trust"; 3353 case INCOME: 3354 return "income"; 3355 case CHILD: 3356 return "child support"; 3357 case DISABL: 3358 return "disability pay"; 3359 case INVEST: 3360 return "investment income"; 3361 case PAY: 3362 return "paid employment"; 3363 case RETIRE: 3364 return "retirement pay"; 3365 case SPOUSAL: 3366 return "spousal or partner support"; 3367 case SUPPLE: 3368 return "income supplement"; 3369 case TAX: 3370 return "tax obligation"; 3371 case LIVEXP: 3372 return "living expense"; 3373 case CLOTH: 3374 return "clothing expense"; 3375 case FOOD: 3376 return "food expense"; 3377 case HEALTH: 3378 return "health expense"; 3379 case HOUSE: 3380 return "household expense"; 3381 case LEGAL: 3382 return "legal expense"; 3383 case MORTG: 3384 return "mortgage"; 3385 case RENT: 3386 return "rent"; 3387 case SUNDRY: 3388 return "sundry expense"; 3389 case TRANS: 3390 return "transportation expense"; 3391 case UTIL: 3392 return "utility expense"; 3393 case ELSTAT: 3394 return "eligibility indicator"; 3395 case ADOPT: 3396 return "adoption document"; 3397 case BTHCERT: 3398 return "birth certificate"; 3399 case CCOC: 3400 return "creditable coverage document"; 3401 case DRLIC: 3402 return "driver license"; 3403 case FOSTER: 3404 return "foster child document"; 3405 case MEMBER: 3406 return "program or policy member"; 3407 case MIL: 3408 return "military identification"; 3409 case MRGCERT: 3410 return "marriage certificate"; 3411 case PASSPORT: 3412 return "passport"; 3413 case STUDENRL: 3414 return "student enrollment"; 3415 case HLSTAT: 3416 return "health status"; 3417 case DISABLE: 3418 return "disabled"; 3419 case DRUG: 3420 return "drug use"; 3421 case IVDRG: 3422 return "IV drug use"; 3423 case PGNT: 3424 return "pregnant"; 3425 case LIVDEP: 3426 return "living dependency"; 3427 case RELDEP: 3428 return "relative dependent"; 3429 case SPSDEP: 3430 return "spouse dependent"; 3431 case URELDEP: 3432 return "unrelated person dependent"; 3433 case LIVSIT: 3434 return "living situation"; 3435 case ALONE: 3436 return "alone"; 3437 case DEPCHD: 3438 return "dependent children"; 3439 case DEPSPS: 3440 return "dependent spouse"; 3441 case DEPYGCHD: 3442 return "dependent young children"; 3443 case FAM: 3444 return "live with family"; 3445 case RELAT: 3446 return "relative"; 3447 case SPS: 3448 return "spouse only"; 3449 case UNREL: 3450 return "unrelated person"; 3451 case SOECSTAT: 3452 return "socio economic status"; 3453 case ABUSE: 3454 return "abuse victim"; 3455 case HMLESS: 3456 return "homeless"; 3457 case ILGIM: 3458 return "illegal immigrant"; 3459 case INCAR: 3460 return "incarcerated"; 3461 case PROB: 3462 return "probation"; 3463 case REFUG: 3464 return "refugee"; 3465 case UNEMPL: 3466 return "unemployed"; 3467 case _ALLERGYTESTVALUE: 3468 return "AllergyTestValue"; 3469 case A0: 3470 return "no reaction"; 3471 case A1: 3472 return "minimal reaction"; 3473 case A2: 3474 return "mild reaction"; 3475 case A3: 3476 return "moderate reaction"; 3477 case A4: 3478 return "severe reaction"; 3479 case _COMPOSITEMEASURESCORING: 3480 return "CompositeMeasureScoring"; 3481 case ALLORNONESCR: 3482 return "All-or-nothing Scoring"; 3483 case LINEARSCR: 3484 return "Linear Scoring"; 3485 case OPPORSCR: 3486 return "Opportunity Scoring"; 3487 case WEIGHTSCR: 3488 return "Weighted Scoring"; 3489 case _COVERAGELIMITOBSERVATIONVALUE: 3490 return "CoverageLimitObservationValue"; 3491 case _COVERAGELEVELOBSERVATIONVALUE: 3492 return "CoverageLevelObservationValue"; 3493 case ADC: 3494 return "adult child"; 3495 case CHD: 3496 return "child"; 3497 case DEP: 3498 return "dependent"; 3499 case DP: 3500 return "domestic partner"; 3501 case ECH: 3502 return "employee"; 3503 case FLY: 3504 return "family coverage"; 3505 case IND: 3506 return "individual"; 3507 case SSP: 3508 return "same sex partner"; 3509 case _CRITICALITYOBSERVATIONVALUE: 3510 return "CriticalityObservationValue"; 3511 case CRITH: 3512 return "high criticality"; 3513 case CRITL: 3514 return "low criticality"; 3515 case CRITU: 3516 return "unable to assess criticality"; 3517 case _EMPLOYMENTSTATUS: 3518 return "_EmploymentStatus"; 3519 case EMPLOYED: 3520 return "Employed"; 3521 case NOTINLABORFORCE: 3522 return "Not In Labor Force"; 3523 case UNEMPLOYED: 3524 return "Unemployed"; 3525 case _GENETICOBSERVATIONVALUE: 3526 return "GeneticObservationValue"; 3527 case HOMOZYGOTE: 3528 return "HOMO"; 3529 case _OBSERVATIONMEASURESCORING: 3530 return "ObservationMeasureScoring"; 3531 case COHORT: 3532 return "cohort measure scoring"; 3533 case CONTVAR: 3534 return "continuous variable measure scoring"; 3535 case PROPOR: 3536 return "proportion measure scoring"; 3537 case RATIO: 3538 return "ratio measure scoring"; 3539 case _OBSERVATIONMEASURETYPE: 3540 return "ObservationMeasureType"; 3541 case COMPOSITE: 3542 return "composite measure type"; 3543 case EFFICIENCY: 3544 return "efficiency measure type"; 3545 case EXPERIENCE: 3546 return "experience measure type"; 3547 case OUTCOME: 3548 return "outcome measure type"; 3549 case INTERMOM: 3550 return "intermediate clinical outcome measure"; 3551 case PROPM: 3552 return "intermediate clinical outcome measure"; 3553 case PROCESS: 3554 return "process measure type"; 3555 case APPROPRIATE: 3556 return "appropriate use process measure"; 3557 case RESOURCE: 3558 return "resource use measure type"; 3559 case STRUCTURE: 3560 return "structure measure type"; 3561 case _OBSERVATIONPOPULATIONINCLUSION: 3562 return "ObservationPopulationInclusion"; 3563 case DENEX: 3564 return "denominator exclusions"; 3565 case DENEXCEP: 3566 return "denominator exceptions"; 3567 case DENOM: 3568 return "denominator"; 3569 case IP: 3570 return "initial population"; 3571 case IPP: 3572 return "initial patient population"; 3573 case MSRPOPL: 3574 return "measure population"; 3575 case NUMER: 3576 return "numerator"; 3577 case NUMEX: 3578 return "numerator exclusions"; 3579 case _PARTIALCOMPLETIONSCALE: 3580 return "PartialCompletionScale"; 3581 case G: 3582 return "Great extent"; 3583 case LE: 3584 return "Large extent"; 3585 case ME: 3586 return "Medium extent"; 3587 case MI: 3588 return "Minimal extent"; 3589 case N: 3590 return "None"; 3591 case S: 3592 return "Some extent"; 3593 case _SECURITYOBSERVATIONVALUE: 3594 return "SecurityObservationValue"; 3595 case _SECINTOBV: 3596 return "security integrity"; 3597 case _SECALTINTOBV: 3598 return "alteration integrity"; 3599 case ABSTRED: 3600 return "abstracted"; 3601 case AGGRED: 3602 return "aggregated"; 3603 case ANONYED: 3604 return "anonymized"; 3605 case MAPPED: 3606 return "mapped"; 3607 case MASKED: 3608 return "masked"; 3609 case PSEUDED: 3610 return "pseudonymized"; 3611 case REDACTED: 3612 return "redacted"; 3613 case SUBSETTED: 3614 return "subsetted"; 3615 case SYNTAC: 3616 return "syntactic transform"; 3617 case TRSLT: 3618 return "translated"; 3619 case VERSIONED: 3620 return "versioned"; 3621 case _SECDATINTOBV: 3622 return "data integrity"; 3623 case CRYTOHASH: 3624 return "cryptographic hash function"; 3625 case DIGSIG: 3626 return "digital signature"; 3627 case _SECINTCONOBV: 3628 return "integrity confidence"; 3629 case HRELIABLE: 3630 return "highly reliable"; 3631 case RELIABLE: 3632 return "reliable"; 3633 case UNCERTREL: 3634 return "uncertain reliability"; 3635 case UNRELIABLE: 3636 return "unreliable"; 3637 case _SECINTPRVOBV: 3638 return "provenance"; 3639 case _SECINTPRVABOBV: 3640 return "provenance asserted by"; 3641 case CLINAST: 3642 return "clinician asserted"; 3643 case DEVAST: 3644 return "device asserted"; 3645 case HCPAST: 3646 return "healthcare professional asserted"; 3647 case PACQAST: 3648 return "patient acquaintance asserted"; 3649 case PATAST: 3650 return "patient asserted"; 3651 case PAYAST: 3652 return "payer asserted"; 3653 case PROAST: 3654 return "professional asserted"; 3655 case SDMAST: 3656 return "substitute decision maker asserted"; 3657 case _SECINTPRVRBOBV: 3658 return "provenance reported by"; 3659 case CLINRPT: 3660 return "clinician reported"; 3661 case DEVRPT: 3662 return "device reported"; 3663 case HCPRPT: 3664 return "healthcare professional reported"; 3665 case PACQRPT: 3666 return "patient acquaintance reported"; 3667 case PATRPT: 3668 return "patient reported"; 3669 case PAYRPT: 3670 return "payer reported"; 3671 case PRORPT: 3672 return "professional reported"; 3673 case SDMRPT: 3674 return "substitute decision maker reported"; 3675 case SECTRSTOBV: 3676 return "security trust observation"; 3677 case TRSTACCRDOBV: 3678 return "trust accreditation observation"; 3679 case TRSTAGREOBV: 3680 return "trust agreement observation"; 3681 case TRSTCERTOBV: 3682 return "trust certificate observation"; 3683 case TRSTLOAOBV: 3684 return "trust assurance observation"; 3685 case LOAAN: 3686 return "authentication level of assurance value"; 3687 case LOAAN1: 3688 return "low authentication level of assurance"; 3689 case LOAAN2: 3690 return "basic authentication level of assurance"; 3691 case LOAAN3: 3692 return "medium authentication level of assurance"; 3693 case LOAAN4: 3694 return "high authentication level of assurance"; 3695 case LOAAP: 3696 return "authentication process level of assurance value"; 3697 case LOAAP1: 3698 return "low authentication process level of assurance"; 3699 case LOAAP2: 3700 return "basic authentication process level of assurance"; 3701 case LOAAP3: 3702 return "medium authentication process level of assurance"; 3703 case LOAAP4: 3704 return "high authentication process level of assurance"; 3705 case LOAAS: 3706 return "assertion level of assurance value"; 3707 case LOAAS1: 3708 return "low assertion level of assurance"; 3709 case LOAAS2: 3710 return "basic assertion level of assurance"; 3711 case LOAAS3: 3712 return "medium assertion level of assurance"; 3713 case LOAAS4: 3714 return "high assertion level of assurance"; 3715 case LOACM: 3716 return "token and credential management level of assurance value)"; 3717 case LOACM1: 3718 return "low token and credential management level of assurance"; 3719 case LOACM2: 3720 return "basic token and credential management level of assurance"; 3721 case LOACM3: 3722 return "medium token and credential management level of assurance"; 3723 case LOACM4: 3724 return "high token and credential management level of assurance"; 3725 case LOAID: 3726 return "identity proofing level of assurance"; 3727 case LOAID1: 3728 return "low identity proofing level of assurance"; 3729 case LOAID2: 3730 return "basic identity proofing level of assurance"; 3731 case LOAID3: 3732 return "medium identity proofing level of assurance"; 3733 case LOAID4: 3734 return "high identity proofing level of assurance"; 3735 case LOANR: 3736 return "non-repudiation level of assurance value"; 3737 case LOANR1: 3738 return "low non-repudiation level of assurance"; 3739 case LOANR2: 3740 return "basic non-repudiation level of assurance"; 3741 case LOANR3: 3742 return "medium non-repudiation level of assurance"; 3743 case LOANR4: 3744 return "high non-repudiation level of assurance"; 3745 case LOARA: 3746 return "remote access level of assurance value"; 3747 case LOARA1: 3748 return "low remote access level of assurance"; 3749 case LOARA2: 3750 return "basic remote access level of assurance"; 3751 case LOARA3: 3752 return "medium remote access level of assurance"; 3753 case LOARA4: 3754 return "high remote access level of assurance"; 3755 case LOATK: 3756 return "token level of assurance value"; 3757 case LOATK1: 3758 return "low token level of assurance"; 3759 case LOATK2: 3760 return "basic token level of assurance"; 3761 case LOATK3: 3762 return "medium token level of assurance"; 3763 case LOATK4: 3764 return "high token level of assurance"; 3765 case TRSTMECOBV: 3766 return "none supplied 6"; 3767 case _SEVERITYOBSERVATION: 3768 return "SeverityObservation"; 3769 case H: 3770 return "High"; 3771 case L: 3772 return "Low"; 3773 case M: 3774 return "Moderate"; 3775 case _SUBJECTBODYPOSITION: 3776 return "_SubjectBodyPosition"; 3777 case LLD: 3778 return "left lateral decubitus"; 3779 case PRN: 3780 return "prone"; 3781 case RLD: 3782 return "right lateral decubitus"; 3783 case SFWL: 3784 return "Semi-Fowler's"; 3785 case SIT: 3786 return "sitting"; 3787 case STN: 3788 return "standing"; 3789 case SUP: 3790 return "supine"; 3791 case RTRD: 3792 return "reverse trendelenburg"; 3793 case TRD: 3794 return "trendelenburg"; 3795 case _VERIFICATIONOUTCOMEVALUE: 3796 return "verification outcome"; 3797 case ACT: 3798 return "active coverage"; 3799 case ACTPEND: 3800 return "active - pending investigation"; 3801 case ELG: 3802 return "eligible"; 3803 case INACT: 3804 return "inactive"; 3805 case INPNDINV: 3806 return "inactive - pending investigation"; 3807 case INPNDUPD: 3808 return "inactive - pending eligibility update"; 3809 case NELG: 3810 return "not eligible"; 3811 case _WORKSCHEDULE: 3812 return "_WorkSchedule"; 3813 case DS: 3814 return "daytime shift"; 3815 case EMS: 3816 return "early morning shift"; 3817 case ES: 3818 return "evening shift"; 3819 case NS: 3820 return "night shift"; 3821 case RSWN: 3822 return "rotating shift with nights"; 3823 case RSWON: 3824 return "rotating shift without nights"; 3825 case SS: 3826 return "split shift"; 3827 case VLS: 3828 return "very long shift"; 3829 case VS: 3830 return "variable shift"; 3831 case _ANNOTATIONVALUE: 3832 return "AnnotationValue"; 3833 case _COMMONCLINICALOBSERVATIONVALUE: 3834 return "common clinical observation"; 3835 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: 3836 return "Individual Case Safety Report Value Domains"; 3837 case _INDICATIONVALUE: 3838 return "IndicationValue"; 3839 case NULL: 3840 return null; 3841 default: 3842 return "?"; 3843 } 3844 } 3845 3846}