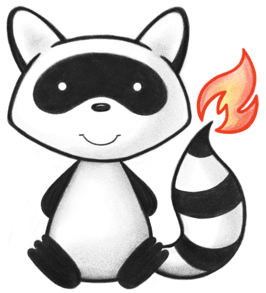
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3PersonDisabilityType { 037 038 /** 039 * Vision impaired 040 */ 041 _1, 042 /** 043 * Hearing impaired 044 */ 045 _2, 046 /** 047 * Speech impaired 048 */ 049 _3, 050 /** 051 * Mentally impaired 052 */ 053 _4, 054 /** 055 * Mobility impaired 056 */ 057 _5, 058 /** 059 * A crib is required to move the person 060 */ 061 CB, 062 /** 063 * Person requires crutches to ambulate 064 */ 065 CR, 066 /** 067 * A gurney is required to move the person 068 */ 069 G, 070 /** 071 * Person requires a wheelchair to be ambulatory 072 */ 073 WC, 074 /** 075 * Person requires a walker to ambulate 076 */ 077 WK, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static V3PersonDisabilityType fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("1".equals(codeString)) 087 return _1; 088 if ("2".equals(codeString)) 089 return _2; 090 if ("3".equals(codeString)) 091 return _3; 092 if ("4".equals(codeString)) 093 return _4; 094 if ("5".equals(codeString)) 095 return _5; 096 if ("CB".equals(codeString)) 097 return CB; 098 if ("CR".equals(codeString)) 099 return CR; 100 if ("G".equals(codeString)) 101 return G; 102 if ("WC".equals(codeString)) 103 return WC; 104 if ("WK".equals(codeString)) 105 return WK; 106 throw new FHIRException("Unknown V3PersonDisabilityType code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case _1: 112 return "1"; 113 case _2: 114 return "2"; 115 case _3: 116 return "3"; 117 case _4: 118 return "4"; 119 case _5: 120 return "5"; 121 case CB: 122 return "CB"; 123 case CR: 124 return "CR"; 125 case G: 126 return "G"; 127 case WC: 128 return "WC"; 129 case WK: 130 return "WK"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getSystem() { 139 return "http://terminology.hl7.org/CodeSystem/v3-PersonDisabilityType"; 140 } 141 142 public String getDefinition() { 143 switch (this) { 144 case _1: 145 return "Vision impaired"; 146 case _2: 147 return "Hearing impaired"; 148 case _3: 149 return "Speech impaired"; 150 case _4: 151 return "Mentally impaired"; 152 case _5: 153 return "Mobility impaired"; 154 case CB: 155 return "A crib is required to move the person"; 156 case CR: 157 return "Person requires crutches to ambulate"; 158 case G: 159 return "A gurney is required to move the person"; 160 case WC: 161 return "Person requires a wheelchair to be ambulatory"; 162 case WK: 163 return "Person requires a walker to ambulate"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDisplay() { 172 switch (this) { 173 case _1: 174 return "Vision impaired"; 175 case _2: 176 return "Hearing impaired"; 177 case _3: 178 return "Speech impaired"; 179 case _4: 180 return "Mentally impaired"; 181 case _5: 182 return "Mobility impaired"; 183 case CB: 184 return "Requires crib"; 185 case CR: 186 return "Requires crutches"; 187 case G: 188 return "Requires gurney"; 189 case WC: 190 return "Requires wheelchair"; 191 case WK: 192 return "Requires walker"; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200}