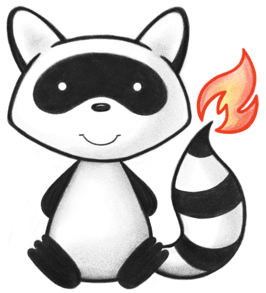
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3ProbabilityDistributionType { 037 038 /** 039 * The beta-distribution is used for data that is bounded on both sides and may 040 * or may not be skewed (e.g., occurs when probabilities are estimated.) Two 041 * parameters a and b are available to adjust the curve. The mean m and variance 042 * s2 relate as follows: m = a/ (a + b) and s2 = ab/((a + b)2 (a + b + 1)). 043 */ 044 B, 045 /** 046 * Used for data that describes extinction. The exponential distribution is a 047 * special form of g-distribution where a = 1, hence, the relationship to mean m 048 * and variance s2 are m = b and s2 = b2. 049 */ 050 E, 051 /** 052 * Used to describe the quotient of two c2 random variables. The F-distribution 053 * has two parameters n1 and n2, which are the numbers of degrees of freedom of 054 * the numerator and denominator variable respectively. The relationship to mean 055 * m and variance s2 are: m = n2 / (n2 - 2) and s2 = (2 n2 (n2 + n1 - 2)) / (n1 056 * (n2 - 2)2 (n2 - 4)). 057 */ 058 F, 059 /** 060 * The gamma-distribution used for data that is skewed and bounded to the right, 061 * i.e. where the maximum of the distribution curve is located near the origin. 062 * The g-distribution has a two parameters a and b. The relationship to mean m 063 * and variance s2 is m = a b and s2 = a b2. 064 */ 065 G, 066 /** 067 * The logarithmic normal distribution is used to transform skewed random 068 * variable X into a normally distributed random variable U = log X. The 069 * log-normal distribution can be specified with the properties mean m and 070 * standard deviation s. Note however that mean m and standard deviation s are 071 * the parameters of the raw value distribution, not the transformed parameters 072 * of the lognormal distribution that are conventionally referred to by the same 073 * letters. Those log-normal parameters mlog and slog relate to the mean m and 074 * standard deviation s of the data value through slog2 = log (s2/m2 + 1) and 075 * mlog = log m - slog2/2. 076 */ 077 LN, 078 /** 079 * This is the well-known bell-shaped normal distribution. Because of the 080 * central limit theorem, the normal distribution is the distribution of choice 081 * for an unbounded random variable that is an outcome of a combination of many 082 * stochastic processes. Even for values bounded on a single side (i.e. greater 083 * than 0) the normal distribution may be accurate enough if the mean is "far 084 * away" from the bound of the scale measured in terms of standard deviations. 085 */ 086 N, 087 /** 088 * Used to describe the quotient of a normal random variable and the square root 089 * of a c2 random variable. The t-distribution has one parameter n, the number 090 * of degrees of freedom. The relationship to mean m and variance s2 are: m = 0 091 * and s2 = n / (n - 2) 092 */ 093 T, 094 /** 095 * The uniform distribution assigns a constant probability over the entire 096 * interval of possible outcomes, while all outcomes outside this interval are 097 * assumed to have zero probability. The width of this interval is 2s sqrt(3). 098 * Thus, the uniform distribution assigns the probability densities f(x) = 099 * sqrt(2 s sqrt(3)) to values m - s sqrt(3) >= x <= m + s sqrt(3) and f(x) = 0 100 * otherwise. 101 */ 102 U, 103 /** 104 * Used to describe the sum of squares of random variables which occurs when a 105 * variance is estimated (rather than presumed) from the sample. The only 106 * parameter of the c2-distribution is n, so called the number of degrees of 107 * freedom (which is the number of independent parts in the sum). The 108 * c2-distribution is a special type of g-distribution with parameter a = n /2 109 * and b = 2. Hence, m = n and s2 = 2 n. 110 */ 111 X2, 112 /** 113 * added to help the parsers 114 */ 115 NULL; 116 117 public static V3ProbabilityDistributionType fromCode(String codeString) throws FHIRException { 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("B".equals(codeString)) 121 return B; 122 if ("E".equals(codeString)) 123 return E; 124 if ("F".equals(codeString)) 125 return F; 126 if ("G".equals(codeString)) 127 return G; 128 if ("LN".equals(codeString)) 129 return LN; 130 if ("N".equals(codeString)) 131 return N; 132 if ("T".equals(codeString)) 133 return T; 134 if ("U".equals(codeString)) 135 return U; 136 if ("X2".equals(codeString)) 137 return X2; 138 throw new FHIRException("Unknown V3ProbabilityDistributionType code '" + codeString + "'"); 139 } 140 141 public String toCode() { 142 switch (this) { 143 case B: 144 return "B"; 145 case E: 146 return "E"; 147 case F: 148 return "F"; 149 case G: 150 return "G"; 151 case LN: 152 return "LN"; 153 case N: 154 return "N"; 155 case T: 156 return "T"; 157 case U: 158 return "U"; 159 case X2: 160 return "X2"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getSystem() { 169 return "http://terminology.hl7.org/CodeSystem/v3-ProbabilityDistributionType"; 170 } 171 172 public String getDefinition() { 173 switch (this) { 174 case B: 175 return "The beta-distribution is used for data that is bounded on both sides and may or may not be skewed (e.g., occurs when probabilities are estimated.) Two parameters a and b are available to adjust the curve. The mean m and variance s2 relate as follows: m = a/ (a + b) and s2 = ab/((a + b)2 (a + b + 1))."; 176 case E: 177 return "Used for data that describes extinction. The exponential distribution is a special form of g-distribution where a = 1, hence, the relationship to mean m and variance s2 are m = b and s2 = b2."; 178 case F: 179 return "Used to describe the quotient of two c2 random variables. The F-distribution has two parameters n1 and n2, which are the numbers of degrees of freedom of the numerator and denominator variable respectively. The relationship to mean m and variance s2 are: m = n2 / (n2 - 2) and s2 = (2 n2 (n2 + n1 - 2)) / (n1 (n2 - 2)2 (n2 - 4))."; 180 case G: 181 return "The gamma-distribution used for data that is skewed and bounded to the right, i.e. where the maximum of the distribution curve is located near the origin. The g-distribution has a two parameters a and b. The relationship to mean m and variance s2 is m = a b and s2 = a b2."; 182 case LN: 183 return "The logarithmic normal distribution is used to transform skewed random variable X into a normally distributed random variable U = log X. The log-normal distribution can be specified with the properties mean m and standard deviation s. Note however that mean m and standard deviation s are the parameters of the raw value distribution, not the transformed parameters of the lognormal distribution that are conventionally referred to by the same letters. Those log-normal parameters mlog and slog relate to the mean m and standard deviation s of the data value through slog2 = log (s2/m2 + 1) and mlog = log m - slog2/2."; 184 case N: 185 return "This is the well-known bell-shaped normal distribution. Because of the central limit theorem, the normal distribution is the distribution of choice for an unbounded random variable that is an outcome of a combination of many stochastic processes. Even for values bounded on a single side (i.e. greater than 0) the normal distribution may be accurate enough if the mean is \"far away\" from the bound of the scale measured in terms of standard deviations."; 186 case T: 187 return "Used to describe the quotient of a normal random variable and the square root of a c2 random variable. The t-distribution has one parameter n, the number of degrees of freedom. The relationship to mean m and variance s2 are: m = 0 and s2 = n / (n - 2)"; 188 case U: 189 return "The uniform distribution assigns a constant probability over the entire interval of possible outcomes, while all outcomes outside this interval are assumed to have zero probability. The width of this interval is 2s sqrt(3). Thus, the uniform distribution assigns the probability densities f(x) = sqrt(2 s sqrt(3)) to values m - s sqrt(3) >= x <= m + s sqrt(3) and f(x) = 0 otherwise."; 190 case X2: 191 return "Used to describe the sum of squares of random variables which occurs when a variance is estimated (rather than presumed) from the sample. The only parameter of the c2-distribution is n, so called the number of degrees of freedom (which is the number of independent parts in the sum). The c2-distribution is a special type of g-distribution with parameter a = n /2 and b = 2. Hence, m = n and s2 = 2 n."; 192 case NULL: 193 return null; 194 default: 195 return "?"; 196 } 197 } 198 199 public String getDisplay() { 200 switch (this) { 201 case B: 202 return "beta"; 203 case E: 204 return "exponential"; 205 case F: 206 return "F"; 207 case G: 208 return "(gamma)"; 209 case LN: 210 return "log-normal"; 211 case N: 212 return "normal (Gaussian)"; 213 case T: 214 return "T"; 215 case U: 216 return "uniform"; 217 case X2: 218 return "chi square"; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 226}