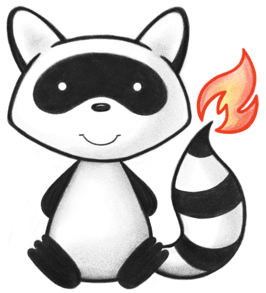
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3QueryParameterValue { 037 038 /** 039 * Description:Filter codes used to manage volume of dispenses returned by a 040 * parameter-based queries. 041 */ 042 _DISPENSEQUERYFILTERCODE, 043 /** 044 * Description:Returns all dispenses to date for a prescription. 045 */ 046 ALLDISP, 047 /** 048 * Description:Returns the most recent dispense for a prescription. 049 */ 050 LASTDISP, 051 /** 052 * Description:Returns no dispense for a prescription. 053 */ 054 NODISP, 055 /** 056 * Filter codes used to manage types of orders being returned by a 057 * parameter-based query. 058 */ 059 _ORDERFILTERCODE, 060 /** 061 * Return all orders. 062 */ 063 AO, 064 /** 065 * Return only those orders that do not have results. 066 */ 067 ONR, 068 /** 069 * Return only those orders that have results. 070 */ 071 OWR, 072 /** 073 * A "helper" vocabulary used to construct complex query filters based on how 074 * and whether a prescription has been dispensed. 075 */ 076 _PRESCRIPTIONDISPENSEFILTERCODE, 077 /** 078 * Filter to only include SubstanceAdministration orders which have no remaining 079 * quantity authorized to be dispensed. 080 */ 081 C, 082 /** 083 * Filter to only include SubstanceAdministration orders which have no 084 * fulfilling supply events performed. 085 */ 086 N, 087 /** 088 * Filter to only include SubstanceAdministration orders which have had at least 089 * one fulfilling supply event, but which still have outstanding quantity 090 * remaining to be authorized. 091 */ 092 R, 093 /** 094 * Description:Indicates how result sets should be filtered based on whether 095 * they have associated issues. 096 */ 097 _QUERYPARAMETERVALUE, 098 /** 099 * Description:Result set should not be filtered based on the presence of 100 * issues. 101 */ 102 ISSFA, 103 /** 104 * Description:Result set should be filtered to only include records with 105 * associated issues. 106 */ 107 ISSFI, 108 /** 109 * Description:Result set should be filtered to only include records with 110 * associated unmanaged issues. 111 */ 112 ISSFU, 113 /** 114 * added to help the parsers 115 */ 116 NULL; 117 118 public static V3QueryParameterValue fromCode(String codeString) throws FHIRException { 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("_DispenseQueryFilterCode".equals(codeString)) 122 return _DISPENSEQUERYFILTERCODE; 123 if ("ALLDISP".equals(codeString)) 124 return ALLDISP; 125 if ("LASTDISP".equals(codeString)) 126 return LASTDISP; 127 if ("NODISP".equals(codeString)) 128 return NODISP; 129 if ("_OrderFilterCode".equals(codeString)) 130 return _ORDERFILTERCODE; 131 if ("AO".equals(codeString)) 132 return AO; 133 if ("ONR".equals(codeString)) 134 return ONR; 135 if ("OWR".equals(codeString)) 136 return OWR; 137 if ("_PrescriptionDispenseFilterCode".equals(codeString)) 138 return _PRESCRIPTIONDISPENSEFILTERCODE; 139 if ("C".equals(codeString)) 140 return C; 141 if ("N".equals(codeString)) 142 return N; 143 if ("R".equals(codeString)) 144 return R; 145 if ("_QueryParameterValue".equals(codeString)) 146 return _QUERYPARAMETERVALUE; 147 if ("ISSFA".equals(codeString)) 148 return ISSFA; 149 if ("ISSFI".equals(codeString)) 150 return ISSFI; 151 if ("ISSFU".equals(codeString)) 152 return ISSFU; 153 throw new FHIRException("Unknown V3QueryParameterValue code '" + codeString + "'"); 154 } 155 156 public String toCode() { 157 switch (this) { 158 case _DISPENSEQUERYFILTERCODE: 159 return "_DispenseQueryFilterCode"; 160 case ALLDISP: 161 return "ALLDISP"; 162 case LASTDISP: 163 return "LASTDISP"; 164 case NODISP: 165 return "NODISP"; 166 case _ORDERFILTERCODE: 167 return "_OrderFilterCode"; 168 case AO: 169 return "AO"; 170 case ONR: 171 return "ONR"; 172 case OWR: 173 return "OWR"; 174 case _PRESCRIPTIONDISPENSEFILTERCODE: 175 return "_PrescriptionDispenseFilterCode"; 176 case C: 177 return "C"; 178 case N: 179 return "N"; 180 case R: 181 return "R"; 182 case _QUERYPARAMETERVALUE: 183 return "_QueryParameterValue"; 184 case ISSFA: 185 return "ISSFA"; 186 case ISSFI: 187 return "ISSFI"; 188 case ISSFU: 189 return "ISSFU"; 190 case NULL: 191 return null; 192 default: 193 return "?"; 194 } 195 } 196 197 public String getSystem() { 198 return "http://terminology.hl7.org/CodeSystem/v3-QueryParameterValue"; 199 } 200 201 public String getDefinition() { 202 switch (this) { 203 case _DISPENSEQUERYFILTERCODE: 204 return "Description:Filter codes used to manage volume of dispenses returned by a parameter-based queries."; 205 case ALLDISP: 206 return "Description:Returns all dispenses to date for a prescription."; 207 case LASTDISP: 208 return "Description:Returns the most recent dispense for a prescription."; 209 case NODISP: 210 return "Description:Returns no dispense for a prescription."; 211 case _ORDERFILTERCODE: 212 return "Filter codes used to manage types of orders being returned by a parameter-based query."; 213 case AO: 214 return "Return all orders."; 215 case ONR: 216 return "Return only those orders that do not have results."; 217 case OWR: 218 return "Return only those orders that have results."; 219 case _PRESCRIPTIONDISPENSEFILTERCODE: 220 return "A \"helper\" vocabulary used to construct complex query filters based on how and whether a prescription has been dispensed."; 221 case C: 222 return "Filter to only include SubstanceAdministration orders which have no remaining quantity authorized to be dispensed."; 223 case N: 224 return "Filter to only include SubstanceAdministration orders which have no fulfilling supply events performed."; 225 case R: 226 return "Filter to only include SubstanceAdministration orders which have had at least one fulfilling supply event, but which still have outstanding quantity remaining to be authorized."; 227 case _QUERYPARAMETERVALUE: 228 return "Description:Indicates how result sets should be filtered based on whether they have associated issues."; 229 case ISSFA: 230 return "Description:Result set should not be filtered based on the presence of issues."; 231 case ISSFI: 232 return "Description:Result set should be filtered to only include records with associated issues."; 233 case ISSFU: 234 return "Description:Result set should be filtered to only include records with associated unmanaged issues."; 235 case NULL: 236 return null; 237 default: 238 return "?"; 239 } 240 } 241 242 public String getDisplay() { 243 switch (this) { 244 case _DISPENSEQUERYFILTERCODE: 245 return "dispense query filter code"; 246 case ALLDISP: 247 return "all dispenses"; 248 case LASTDISP: 249 return "last dispense"; 250 case NODISP: 251 return "no dispense"; 252 case _ORDERFILTERCODE: 253 return "_OrderFilterCode"; 254 case AO: 255 return "all orders"; 256 case ONR: 257 return "orders without results"; 258 case OWR: 259 return "orders with results"; 260 case _PRESCRIPTIONDISPENSEFILTERCODE: 261 return "Prescription Dispense Filter Code"; 262 case C: 263 return "Completely dispensed"; 264 case N: 265 return "Never Dispensed"; 266 case R: 267 return "Dispensed with remaining fills"; 268 case _QUERYPARAMETERVALUE: 269 return "QueryParameterValue"; 270 case ISSFA: 271 return "all"; 272 case ISSFI: 273 return "with issues"; 274 case ISSFU: 275 return "with unmanaged issues"; 276 case NULL: 277 return null; 278 default: 279 return "?"; 280 } 281 } 282 283}