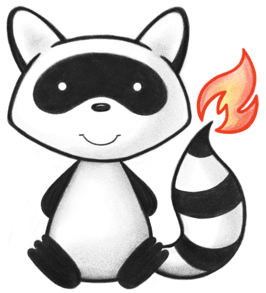
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3RoleLinkType { 037 038 /** 039 * An action taken with respect to a subject Entity by a regulatory or 040 * authoritative body with supervisory capacity over that entity. The action is 041 * taken in response to behavior by the subject Entity that body finds to be 042 * undesirable. 043 * 044 * Suspension, license restrictions, monetary fine, letter of reprimand, 045 * mandated training, mandated supervision, etc.Examples: 046 */ 047 REL, 048 /** 049 * This relationship indicates the source Role is available to the target Role 050 * as a backup. An entity in a backup role will be available as a substitute or 051 * replacement in the event that the entity assigned the role is unavailable. In 052 * medical roles where it is critical that the function be performed and there 053 * is a possibility that the individual assigned may be ill or otherwise 054 * indisposed, another individual is assigned to cover for the individual 055 * originally assigned the role. A backup may be required to be identified, but 056 * unless the backup is actually used, he/she would not assume the assigned 057 * entity role. 058 */ 059 BACKUP, 060 /** 061 * This relationship indicates the target Role provides or receives information 062 * regarding the target role. For example, AssignedEntity is a contact for a 063 * ServiceDeliveryLocation. 064 */ 065 CONT, 066 /** 067 * The source Role has direct authority over the target role in a chain of 068 * authority. 069 */ 070 DIRAUTH, 071 /** 072 * Description: The source role provides identification for the target role. The 073 * source role must be IDENT. The player entity of the source role is 074 * constrained to be the same as (i.e. the equivalent of, or equal to) the 075 * player of the target role if present. If the player is absent from the source 076 * role, then it is assumed to be the same as the player of the target role. 077 */ 078 IDENT, 079 /** 080 * The source Role has indirect authority over the target role in a chain of 081 * authority. 082 */ 083 INDAUTH, 084 /** 085 * The target Role is part of the Source Role. 086 */ 087 PART, 088 /** 089 * This relationship indicates that the source Role replaces (or subsumes) the 090 * target Role. Allows for new identifiers and/or new effective time for a 091 * registry entry or a certification, etc. 092 */ 093 REPL, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static V3RoleLinkType fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("REL".equals(codeString)) 103 return REL; 104 if ("BACKUP".equals(codeString)) 105 return BACKUP; 106 if ("CONT".equals(codeString)) 107 return CONT; 108 if ("DIRAUTH".equals(codeString)) 109 return DIRAUTH; 110 if ("IDENT".equals(codeString)) 111 return IDENT; 112 if ("INDAUTH".equals(codeString)) 113 return INDAUTH; 114 if ("PART".equals(codeString)) 115 return PART; 116 if ("REPL".equals(codeString)) 117 return REPL; 118 throw new FHIRException("Unknown V3RoleLinkType code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case REL: 124 return "REL"; 125 case BACKUP: 126 return "BACKUP"; 127 case CONT: 128 return "CONT"; 129 case DIRAUTH: 130 return "DIRAUTH"; 131 case IDENT: 132 return "IDENT"; 133 case INDAUTH: 134 return "INDAUTH"; 135 case PART: 136 return "PART"; 137 case REPL: 138 return "REPL"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 return "http://terminology.hl7.org/CodeSystem/v3-RoleLinkType"; 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case REL: 153 return "An action taken with respect to a subject Entity by a regulatory or authoritative body with supervisory capacity over that entity. The action is taken in response to behavior by the subject Entity that body finds to be undesirable.\r\n\n Suspension, license restrictions, monetary fine, letter of reprimand, mandated training, mandated supervision, etc.Examples:"; 154 case BACKUP: 155 return "This relationship indicates the source Role is available to the target Role as a backup. An entity in a backup role will be available as a substitute or replacement in the event that the entity assigned the role is unavailable. In medical roles where it is critical that the function be performed and there is a possibility that the individual assigned may be ill or otherwise indisposed, another individual is assigned to cover for the individual originally assigned the role. A backup may be required to be identified, but unless the backup is actually used, he/she would not assume the assigned entity role."; 156 case CONT: 157 return "This relationship indicates the target Role provides or receives information regarding the target role. For example, AssignedEntity is a contact for a ServiceDeliveryLocation."; 158 case DIRAUTH: 159 return "The source Role has direct authority over the target role in a chain of authority."; 160 case IDENT: 161 return "Description: The source role provides identification for the target role. The source role must be IDENT. The player entity of the source role is constrained to be the same as (i.e. the equivalent of, or equal to) the player of the target role if present. If the player is absent from the source role, then it is assumed to be the same as the player of the target role."; 162 case INDAUTH: 163 return "The source Role has indirect authority over the target role in a chain of authority."; 164 case PART: 165 return "The target Role is part of the Source Role."; 166 case REPL: 167 return "This relationship indicates that the source Role replaces (or subsumes) the target Role. Allows for new identifiers and/or new effective time for a registry entry or a certification, etc."; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 175 public String getDisplay() { 176 switch (this) { 177 case REL: 178 return "related"; 179 case BACKUP: 180 return "is backup for"; 181 case CONT: 182 return "has contact"; 183 case DIRAUTH: 184 return "has direct authority over"; 185 case IDENT: 186 return "Identification"; 187 case INDAUTH: 188 return "has indirect authority over"; 189 case PART: 190 return "has part"; 191 case REPL: 192 return "replaces"; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200}