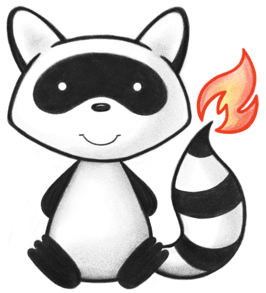
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3TargetAwareness { 037 038 /** 039 * Target person has been informed about the issue but currently denies it. 040 */ 041 D, 042 /** 043 * Target person is fully aware of the issue. 044 */ 045 F, 046 /** 047 * Target person is not capable of comprehending the issue. 048 */ 049 I, 050 /** 051 * Target person is marginally aware of the issue. 052 */ 053 M, 054 /** 055 * Target person is partially aware of the issue. 056 */ 057 P, 058 /** 059 * Target person has not yet been informed of the issue. 060 */ 061 U, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static V3TargetAwareness fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("D".equals(codeString)) 071 return D; 072 if ("F".equals(codeString)) 073 return F; 074 if ("I".equals(codeString)) 075 return I; 076 if ("M".equals(codeString)) 077 return M; 078 if ("P".equals(codeString)) 079 return P; 080 if ("U".equals(codeString)) 081 return U; 082 throw new FHIRException("Unknown V3TargetAwareness code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case D: 088 return "D"; 089 case F: 090 return "F"; 091 case I: 092 return "I"; 093 case M: 094 return "M"; 095 case P: 096 return "P"; 097 case U: 098 return "U"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 return "http://terminology.hl7.org/CodeSystem/v3-TargetAwareness"; 108 } 109 110 public String getDefinition() { 111 switch (this) { 112 case D: 113 return "Target person has been informed about the issue but currently denies it."; 114 case F: 115 return "Target person is fully aware of the issue."; 116 case I: 117 return "Target person is not capable of comprehending the issue."; 118 case M: 119 return "Target person is marginally aware of the issue."; 120 case P: 121 return "Target person is partially aware of the issue."; 122 case U: 123 return "Target person has not yet been informed of the issue."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case D: 134 return "denying"; 135 case F: 136 return "full awareness"; 137 case I: 138 return "incapable"; 139 case M: 140 return "marginal"; 141 case P: 142 return "partial"; 143 case U: 144 return "uninformed"; 145 case NULL: 146 return null; 147 default: 148 return "?"; 149 } 150 } 151 152}