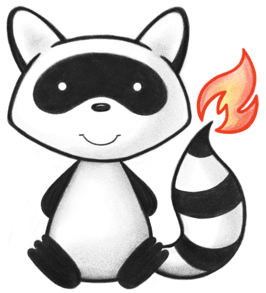
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3TimingEvent { 037 038 /** 039 * before meal (from lat. ante cibus) 040 */ 041 AC, 042 /** 043 * before lunch (from lat. ante cibus diurnus) 044 */ 045 ACD, 046 /** 047 * before breakfast (from lat. ante cibus matutinus) 048 */ 049 ACM, 050 /** 051 * before dinner (from lat. ante cibus vespertinus) 052 */ 053 ACV, 054 /** 055 * Description: meal (from lat. ante cibus) 056 */ 057 C, 058 /** 059 * Description: lunch (from lat. cibus diurnus) 060 */ 061 CD, 062 /** 063 * Description: breakfast (from lat. cibus matutinus) 064 */ 065 CM, 066 /** 067 * Description: dinner (from lat. cibus vespertinus) 068 */ 069 CV, 070 /** 071 * Description: Prior to beginning a regular period of extended sleep (this 072 * would exclude naps). Note that this might occur at different times of day 073 * depending on a person's regular sleep schedule. 074 */ 075 HS, 076 /** 077 * between meals (from lat. inter cibus) 078 */ 079 IC, 080 /** 081 * between lunch and dinner 082 */ 083 ICD, 084 /** 085 * between breakfast and lunch 086 */ 087 ICM, 088 /** 089 * between dinner and the hour of sleep 090 */ 091 ICV, 092 /** 093 * after meal (from lat. post cibus) 094 */ 095 PC, 096 /** 097 * after lunch (from lat. post cibus diurnus) 098 */ 099 PCD, 100 /** 101 * after breakfast (from lat. post cibus matutinus) 102 */ 103 PCM, 104 /** 105 * after dinner (from lat. post cibus vespertinus) 106 */ 107 PCV, 108 /** 109 * Description: Upon waking up from a regular period of sleep, in order to start 110 * regular activities (this would exclude waking up from a nap or temporarily 111 * waking up during a period of sleep) 112 * 113 * 114 * Usage Notes: e.g. 115 * 116 * Take pulse rate on waking in management of thyrotoxicosis. 117 * 118 * Take BP on waking in management of hypertension 119 * 120 * Take basal body temperature on waking in establishing date of ovulation 121 */ 122 WAKE, 123 /** 124 * added to help the parsers 125 */ 126 NULL; 127 128 public static V3TimingEvent fromCode(String codeString) throws FHIRException { 129 if (codeString == null || "".equals(codeString)) 130 return null; 131 if ("AC".equals(codeString)) 132 return AC; 133 if ("ACD".equals(codeString)) 134 return ACD; 135 if ("ACM".equals(codeString)) 136 return ACM; 137 if ("ACV".equals(codeString)) 138 return ACV; 139 if ("C".equals(codeString)) 140 return C; 141 if ("CD".equals(codeString)) 142 return CD; 143 if ("CM".equals(codeString)) 144 return CM; 145 if ("CV".equals(codeString)) 146 return CV; 147 if ("HS".equals(codeString)) 148 return HS; 149 if ("IC".equals(codeString)) 150 return IC; 151 if ("ICD".equals(codeString)) 152 return ICD; 153 if ("ICM".equals(codeString)) 154 return ICM; 155 if ("ICV".equals(codeString)) 156 return ICV; 157 if ("PC".equals(codeString)) 158 return PC; 159 if ("PCD".equals(codeString)) 160 return PCD; 161 if ("PCM".equals(codeString)) 162 return PCM; 163 if ("PCV".equals(codeString)) 164 return PCV; 165 if ("WAKE".equals(codeString)) 166 return WAKE; 167 throw new FHIRException("Unknown V3TimingEvent code '" + codeString + "'"); 168 } 169 170 public String toCode() { 171 switch (this) { 172 case AC: 173 return "AC"; 174 case ACD: 175 return "ACD"; 176 case ACM: 177 return "ACM"; 178 case ACV: 179 return "ACV"; 180 case C: 181 return "C"; 182 case CD: 183 return "CD"; 184 case CM: 185 return "CM"; 186 case CV: 187 return "CV"; 188 case HS: 189 return "HS"; 190 case IC: 191 return "IC"; 192 case ICD: 193 return "ICD"; 194 case ICM: 195 return "ICM"; 196 case ICV: 197 return "ICV"; 198 case PC: 199 return "PC"; 200 case PCD: 201 return "PCD"; 202 case PCM: 203 return "PCM"; 204 case PCV: 205 return "PCV"; 206 case WAKE: 207 return "WAKE"; 208 case NULL: 209 return null; 210 default: 211 return "?"; 212 } 213 } 214 215 public String getSystem() { 216 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 217 } 218 219 public String getDefinition() { 220 switch (this) { 221 case AC: 222 return "before meal (from lat. ante cibus)"; 223 case ACD: 224 return "before lunch (from lat. ante cibus diurnus)"; 225 case ACM: 226 return "before breakfast (from lat. ante cibus matutinus)"; 227 case ACV: 228 return "before dinner (from lat. ante cibus vespertinus)"; 229 case C: 230 return "Description: meal (from lat. ante cibus)"; 231 case CD: 232 return "Description: lunch (from lat. cibus diurnus)"; 233 case CM: 234 return "Description: breakfast (from lat. cibus matutinus)"; 235 case CV: 236 return "Description: dinner (from lat. cibus vespertinus)"; 237 case HS: 238 return "Description: Prior to beginning a regular period of extended sleep (this would exclude naps). Note that this might occur at different times of day depending on a person's regular sleep schedule."; 239 case IC: 240 return "between meals (from lat. inter cibus)"; 241 case ICD: 242 return "between lunch and dinner"; 243 case ICM: 244 return "between breakfast and lunch"; 245 case ICV: 246 return "between dinner and the hour of sleep"; 247 case PC: 248 return "after meal (from lat. post cibus)"; 249 case PCD: 250 return "after lunch (from lat. post cibus diurnus)"; 251 case PCM: 252 return "after breakfast (from lat. post cibus matutinus)"; 253 case PCV: 254 return "after dinner (from lat. post cibus vespertinus)"; 255 case WAKE: 256 return "Description: Upon waking up from a regular period of sleep, in order to start regular activities (this would exclude waking up from a nap or temporarily waking up during a period of sleep)\r\n\n \n Usage Notes: e.g.\r\n\n Take pulse rate on waking in management of thyrotoxicosis.\r\n\n Take BP on waking in management of hypertension\r\n\n Take basal body temperature on waking in establishing date of ovulation"; 257 case NULL: 258 return null; 259 default: 260 return "?"; 261 } 262 } 263 264 public String getDisplay() { 265 switch (this) { 266 case AC: 267 return "AC"; 268 case ACD: 269 return "ACD"; 270 case ACM: 271 return "ACM"; 272 case ACV: 273 return "ACV"; 274 case C: 275 return "C"; 276 case CD: 277 return "CD"; 278 case CM: 279 return "CM"; 280 case CV: 281 return "CV"; 282 case HS: 283 return "HS"; 284 case IC: 285 return "IC"; 286 case ICD: 287 return "ICD"; 288 case ICM: 289 return "ICM"; 290 case ICV: 291 return "ICV"; 292 case PC: 293 return "PC"; 294 case PCD: 295 return "PCD"; 296 case PCM: 297 return "PCM"; 298 case PCV: 299 return "PCV"; 300 case WAKE: 301 return "WAKE"; 302 case NULL: 303 return null; 304 default: 305 return "?"; 306 } 307 } 308 309}