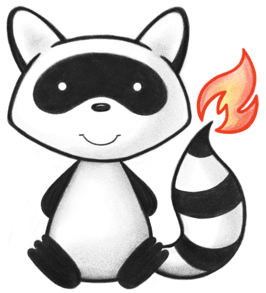
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3WorkClassificationODH { 037 038 /** 039 * A situation in which an individual serves in a government-sponsored military 040 * force. 041 */ 042 PWAF, 043 /** 044 * A situation in which an individual works for a national government 045 * organization, not including armed forces, and receives a paid salary or wage. 046 */ 047 PWFG, 048 /** 049 * A situation in which an individual works for a government organization with 050 * jurisdiction below the level of state/provincial/territorial/tribal 051 * government (e.g., city, town, township), not armed forces, and receives a 052 * paid salary or wage. 053 */ 054 PWLG, 055 /** 056 * A situation in which an individual works for a business (not government) that 057 * they do not own and receives a paid salary or wage. 058 */ 059 PWNSE, 060 /** 061 * A situation in which an individual earns a salary or wage working for himself 062 * or herself instead of working for an employer. 063 */ 064 PWSE, 065 /** 066 * A situation in which an individual works for a government organization with 067 * jurisdiction immediately below the level of national government (between 068 * national government and local government), not armed forces and receives a 069 * paid salary or wage. Often called a state, provincial, territorial, or tribal 070 * government. 071 */ 072 PWSG, 073 /** 074 * A situation in which an individual works for a business (not government) that 075 * they do not own without receiving a paid salary or wage. 076 */ 077 UWNSE, 078 /** 079 * A situation in which an individual works for himself or herself without 080 * receiving a paid salary or wage. 081 */ 082 UWSE, 083 /** 084 * A situation in which an individual chooses to do something, especially for 085 * other people or for an organization, willingly and without being forced or 086 * compensated to do it. This can include formal activity undertaken through 087 * public, private and voluntary organizations as well as informal community 088 * participation. 089 */ 090 VW, 091 /** 092 * added to help the parsers 093 */ 094 NULL; 095 096 public static V3WorkClassificationODH fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("PWAF".equals(codeString)) 100 return PWAF; 101 if ("PWFG".equals(codeString)) 102 return PWFG; 103 if ("PWLG".equals(codeString)) 104 return PWLG; 105 if ("PWNSE".equals(codeString)) 106 return PWNSE; 107 if ("PWSE".equals(codeString)) 108 return PWSE; 109 if ("PWSG".equals(codeString)) 110 return PWSG; 111 if ("UWNSE".equals(codeString)) 112 return UWNSE; 113 if ("UWSE".equals(codeString)) 114 return UWSE; 115 if ("VW".equals(codeString)) 116 return VW; 117 throw new FHIRException("Unknown V3WorkClassificationODH code '" + codeString + "'"); 118 } 119 120 public String toCode() { 121 switch (this) { 122 case PWAF: 123 return "PWAF"; 124 case PWFG: 125 return "PWFG"; 126 case PWLG: 127 return "PWLG"; 128 case PWNSE: 129 return "PWNSE"; 130 case PWSE: 131 return "PWSE"; 132 case PWSG: 133 return "PWSG"; 134 case UWNSE: 135 return "UWNSE"; 136 case UWSE: 137 return "UWSE"; 138 case VW: 139 return "VW"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getSystem() { 148 return "http://terminology.hl7.org/CodeSystem/v3-WorkClassificationODH"; 149 } 150 151 public String getDefinition() { 152 switch (this) { 153 case PWAF: 154 return "A situation in which an individual serves in a government-sponsored military force."; 155 case PWFG: 156 return "A situation in which an individual works for a national government organization, not including armed forces, and receives a paid salary or wage."; 157 case PWLG: 158 return "A situation in which an individual works for a government organization with jurisdiction below the level of state/provincial/territorial/tribal government (e.g., city, town, township), not armed forces, and receives a paid salary or wage."; 159 case PWNSE: 160 return "A situation in which an individual works for a business (not government) that they do not own and receives a paid salary or wage."; 161 case PWSE: 162 return "A situation in which an individual earns a salary or wage working for himself or herself instead of working for an employer."; 163 case PWSG: 164 return "A situation in which an individual works for a government organization with jurisdiction immediately below the level of national government (between national government and local government), not armed forces and receives a paid salary or wage. Often called a state, provincial, territorial, or tribal government."; 165 case UWNSE: 166 return "A situation in which an individual works for a business (not government) that they do not own without receiving a paid salary or wage."; 167 case UWSE: 168 return "A situation in which an individual works for himself or herself without receiving a paid salary or wage."; 169 case VW: 170 return "A situation in which an individual chooses to do something, especially for other people or for an organization, willingly and without being forced or compensated to do it. This can include formal activity undertaken through public, private and voluntary organizations as well as informal community participation."; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDisplay() { 179 switch (this) { 180 case PWAF: 181 return "Paid work, Armed Forces"; 182 case PWFG: 183 return "Paid work, national government, not armed forces"; 184 case PWLG: 185 return "Paid work, local government, not armed forces"; 186 case PWNSE: 187 return "Paid non-governmental work, not self-employed"; 188 case PWSE: 189 return "Paid work, self-employed"; 190 case PWSG: 191 return "Paid work, state government, not armed forces"; 192 case UWNSE: 193 return "Unpaid non-governmental work, not self-employed"; 194 case UWSE: 195 return "Unpaid work, self-employed"; 196 case VW: 197 return "Voluntary work"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 205}