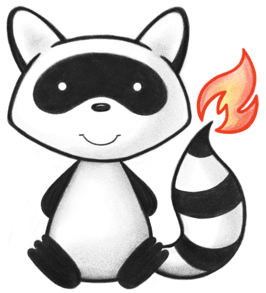
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum W3cProvenanceActivityType { 037 038 /** 039 * The completion of production of a new entity by an activity. This entity did 040 * not exist before generation and becomes available for usage after this 041 * generation. Given that a generation is the completion of production of an 042 * entity, it is instantaneous. 043 */ 044 GENERATION, 045 /** 046 * the beginning of utilizing an entity by an activity. Before usage, the 047 * activity had not begun to utilize this entity and could not have been 048 * affected by the entity. (Note: This definition is formulated for a given 049 * usage; it is permitted for an activity to have used a same entity multiple 050 * times.) Given that a usage is the beginning of utilizing an entity, it is 051 * instantaneous. 052 */ 053 USAGE, 054 /** 055 * The exchange of some unspecified entity by two activities, one activity using 056 * some entity generated by the other. A communication implies that activity a2 057 * is dependent on another activity, a1, by way of some unspecified entity that 058 * is generated by a1 and used by a2. 059 */ 060 COMMUNICATION, 061 /** 062 * When an activity is deemed to have been started by an entity, known as 063 * trigger. The activity did not exist before its start. Any usage, generation, 064 * or invalidation involving an activity follows the activity's start. A start 065 * may refer to a trigger entity that set off the activity, or to an activity, 066 * known as starter, that generated the trigger. Given that a start is when an 067 * activity is deemed to have started, it is instantaneous. 068 */ 069 START, 070 /** 071 * When an activity is deemed to have been ended by an entity, known as trigger. 072 * The activity no longer exists after its end. Any usage, generation, or 073 * invalidation involving an activity precedes the activity's end. An end may 074 * refer to a trigger entity that terminated the activity, or to an activity, 075 * known as ender that generated the trigger. 076 */ 077 END, 078 /** 079 * The start of the destruction, cessation, or expiry of an existing entity by 080 * an activity. The entity is no longer available for use (or further 081 * invalidation) after invalidation. Any generation or usage of an entity 082 * precedes its invalidation. Given that an invalidation is the start of 083 * destruction, cessation, or expiry, it is instantaneous. 084 */ 085 INVALIDATION, 086 /** 087 * A transformation of an entity into another, an update of an entity resulting 088 * in a new one, or the construction of a new entity based on a pre-existing 089 * entity. For an entity to be transformed from, created from, or resulting from 090 * an update to another, there must be some underpinning activity or activities 091 * performing the necessary action(s) resulting in such a derivation. A 092 * derivation can be described at various levels of precision. In its simplest 093 * form, derivation relates two entities. Optionally, attributes can be added to 094 * represent further information about the derivation. If the derivation is the 095 * result of a single known activity, then this activity can also be optionally 096 * expressed. To provide a completely accurate description of the derivation, 097 * the generation and usage of the generated and used entities, respectively, 098 * can be provided, so as to make the derivation path, through usage, activity, 099 * and generation, explicit. Optional information such as activity, generation, 100 * and usage can be linked to derivations to aid analysis of provenance and to 101 * facilitate provenance-based reproducibility. 102 */ 103 DERIVATION, 104 /** 105 * A derivation for which the resulting entity is a revised version of some 106 * original. The implication here is that the resulting entity contains 107 * substantial content from the original. A revision relation is a kind of 108 * derivation relation from a revised entity to a preceding entity. 109 */ 110 REVISION, 111 /** 112 * The repeat of (some or all of) an entity, such as text or image, by someone 113 * who might or might not be its original author. A quotation relation is a kind 114 * of derivation relation, for which an entity was derived from a preceding 115 * entity by copying, or 'quoting', some or all of it. 116 */ 117 QUOTATION, 118 /** 119 * Refers to something produced by some agent with direct experience and 120 * knowledge about the topic, at the time of the topic's study, without benefit 121 * from hindsight. Because of the directness of primary sources, they 'speak for 122 * themselves' in ways that cannot be captured through the filter of secondary 123 * sources. As such, it is important for secondary sources to reference those 124 * primary sources from which they were derived, so that their reliability can 125 * be investigated. It is also important to note that a given entity might be a 126 * primary source for one entity but not another. It is the reason why Primary 127 * Source is defined as a relation as opposed to a subtype of Entity. 128 */ 129 PRIMARYSOURCE, 130 /** 131 * Ascribing of an entity (object/document) to an agent. 132 */ 133 ATTRIBUTION, 134 /** 135 * An aggregating activity that results in composition of an entity, which 136 * provides a structure to some constituents that must themselves be entities. 137 * These constituents are said to be member of the collections. 138 */ 139 COLLECTION, 140 /** 141 * added to help the parsers 142 */ 143 NULL; 144 145 public static W3cProvenanceActivityType fromCode(String codeString) throws FHIRException { 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("Generation".equals(codeString)) 149 return GENERATION; 150 if ("Usage".equals(codeString)) 151 return USAGE; 152 if ("Communication".equals(codeString)) 153 return COMMUNICATION; 154 if ("Start".equals(codeString)) 155 return START; 156 if ("End".equals(codeString)) 157 return END; 158 if ("Invalidation".equals(codeString)) 159 return INVALIDATION; 160 if ("Derivation".equals(codeString)) 161 return DERIVATION; 162 if ("Revision".equals(codeString)) 163 return REVISION; 164 if ("Quotation".equals(codeString)) 165 return QUOTATION; 166 if ("Primary-Source".equals(codeString)) 167 return PRIMARYSOURCE; 168 if ("Attribution".equals(codeString)) 169 return ATTRIBUTION; 170 if ("Collection".equals(codeString)) 171 return COLLECTION; 172 throw new FHIRException("Unknown W3cProvenanceActivityType code '" + codeString + "'"); 173 } 174 175 public String toCode() { 176 switch (this) { 177 case GENERATION: 178 return "Generation"; 179 case USAGE: 180 return "Usage"; 181 case COMMUNICATION: 182 return "Communication"; 183 case START: 184 return "Start"; 185 case END: 186 return "End"; 187 case INVALIDATION: 188 return "Invalidation"; 189 case DERIVATION: 190 return "Derivation"; 191 case REVISION: 192 return "Revision"; 193 case QUOTATION: 194 return "Quotation"; 195 case PRIMARYSOURCE: 196 return "Primary-Source"; 197 case ATTRIBUTION: 198 return "Attribution"; 199 case COLLECTION: 200 return "Collection"; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getSystem() { 209 return "http://hl7.org/fhir/w3c-provenance-activity-type"; 210 } 211 212 public String getDefinition() { 213 switch (this) { 214 case GENERATION: 215 return "The completion of production of a new entity by an activity. This entity did not exist before generation and becomes available for usage after this generation. Given that a generation is the completion of production of an entity, it is instantaneous."; 216 case USAGE: 217 return "the beginning of utilizing an entity by an activity. Before usage, the activity had not begun to utilize this entity and could not have been affected by the entity. (Note: This definition is formulated for a given usage; it is permitted for an activity to have used a same entity multiple times.) Given that a usage is the beginning of utilizing an entity, it is instantaneous."; 218 case COMMUNICATION: 219 return "The exchange of some unspecified entity by two activities, one activity using some entity generated by the other. A communication implies that activity a2 is dependent on another activity, a1, by way of some unspecified entity that is generated by a1 and used by a2."; 220 case START: 221 return "When an activity is deemed to have been started by an entity, known as trigger. The activity did not exist before its start. Any usage, generation, or invalidation involving an activity follows the activity's start. A start may refer to a trigger entity that set off the activity, or to an activity, known as starter, that generated the trigger. Given that a start is when an activity is deemed to have started, it is instantaneous."; 222 case END: 223 return "When an activity is deemed to have been ended by an entity, known as trigger. The activity no longer exists after its end. Any usage, generation, or invalidation involving an activity precedes the activity's end. An end may refer to a trigger entity that terminated the activity, or to an activity, known as ender that generated the trigger."; 224 case INVALIDATION: 225 return "The start of the destruction, cessation, or expiry of an existing entity by an activity. The entity is no longer available for use (or further invalidation) after invalidation. Any generation or usage of an entity precedes its invalidation. Given that an invalidation is the start of destruction, cessation, or expiry, it is instantaneous."; 226 case DERIVATION: 227 return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a pre-existing entity. For an entity to be transformed from, created from, or resulting from an update to another, there must be some underpinning activity or activities performing the necessary action(s) resulting in such a derivation. A derivation can be described at various levels of precision. In its simplest form, derivation relates two entities. Optionally, attributes can be added to represent further information about the derivation. If the derivation is the result of a single known activity, then this activity can also be optionally expressed. To provide a completely accurate description of the derivation, the generation and usage of the generated and used entities, respectively, can be provided, so as to make the derivation path, through usage, activity, and generation, explicit. Optional information such as activity, generation, and usage can be linked to derivations to aid analysis of provenance and to facilitate provenance-based reproducibility."; 228 case REVISION: 229 return "A derivation for which the resulting entity is a revised version of some original. The implication here is that the resulting entity contains substantial content from the original. A revision relation is a kind of derivation relation from a revised entity to a preceding entity."; 230 case QUOTATION: 231 return "The repeat of (some or all of) an entity, such as text or image, by someone who might or might not be its original author. A quotation relation is a kind of derivation relation, for which an entity was derived from a preceding entity by copying, or 'quoting', some or all of it."; 232 case PRIMARYSOURCE: 233 return "Refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight. Because of the directness of primary sources, they 'speak for themselves' in ways that cannot be captured through the filter of secondary sources. As such, it is important for secondary sources to reference those primary sources from which they were derived, so that their reliability can be investigated. It is also important to note that a given entity might be a primary source for one entity but not another. It is the reason why Primary Source is defined as a relation as opposed to a subtype of Entity."; 234 case ATTRIBUTION: 235 return "Ascribing of an entity (object/document) to an agent."; 236 case COLLECTION: 237 return " An aggregating activity that results in composition of an entity, which provides a structure to some constituents that must themselves be entities. These constituents are said to be member of the collections."; 238 case NULL: 239 return null; 240 default: 241 return "?"; 242 } 243 } 244 245 public String getDisplay() { 246 switch (this) { 247 case GENERATION: 248 return "wasGeneratedBy"; 249 case USAGE: 250 return "used"; 251 case COMMUNICATION: 252 return "wasInformedBy"; 253 case START: 254 return "wasStartedBy"; 255 case END: 256 return "wasEndedBy"; 257 case INVALIDATION: 258 return "wasInvalidatedBy"; 259 case DERIVATION: 260 return "wasDerivedFrom"; 261 case REVISION: 262 return "wasRevisionOf"; 263 case QUOTATION: 264 return "wasQuotedFrom"; 265 case PRIMARYSOURCE: 266 return "wasPrimarySourceOf"; 267 case ATTRIBUTION: 268 return "wasAttributedTo"; 269 case COLLECTION: 270 return "isCollectionOf"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 278}