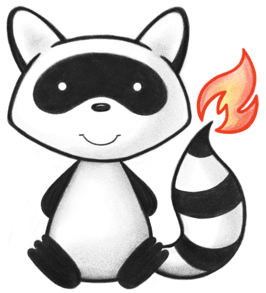
001package org.hl7.fhir.r4.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.exceptions.FHIRException; 033import org.hl7.fhir.r4.context.SimpleWorkerContext; 034import org.hl7.fhir.r4.model.Coding; 035import org.hl7.fhir.r4.model.ConceptMap; 036import org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent; 037import org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent; 038import org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent; 039import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence; 040import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 041 042@MarkedToMoveToAdjunctPackage 043public class ConceptMapEngine { 044 045 private SimpleWorkerContext context; 046 047 public ConceptMapEngine(SimpleWorkerContext context) { 048 this.context = context; 049 } 050 051 public Coding translate(Coding source, String url) throws FHIRException { 052 ConceptMap cm = context.fetchResource(ConceptMap.class, url); 053 if (cm == null) 054 throw new FHIRException("Unable to find ConceptMap '" + url + "'"); 055 if (source.hasSystem()) 056 return translateBySystem(cm, source.getSystem(), source.getCode()); 057 else 058 return translateByJustCode(cm, source.getCode()); 059 } 060 061 private Coding translateByJustCode(ConceptMap cm, String code) throws FHIRException { 062 SourceElementComponent ct = null; 063 ConceptMapGroupComponent cg = null; 064 for (ConceptMapGroupComponent g : cm.getGroup()) { 065 for (SourceElementComponent e : g.getElement()) { 066 if (code.equals(e.getCode())) { 067 if (ct != null) 068 throw new FHIRException("Unable to process translate " + code 069 + " because multiple candidate matches were found in concept map " + cm.getUrl()); 070 ct = e; 071 cg = g; 072 } 073 } 074 } 075 if (ct == null) 076 return null; 077 TargetElementComponent tt = null; 078 for (TargetElementComponent t : ct.getTarget()) { 079 if (!t.hasDependsOn() && !t.hasProduct() && isOkEquivalence(t.getEquivalence())) { 080 if (tt != null) 081 throw new FHIRException("Unable to process translate " + code 082 + " because multiple targets were found in concept map " + cm.getUrl()); 083 tt = t; 084 } 085 } 086 if (tt == null) 087 return null; 088 return new Coding().setSystem(cg.getTarget()).setVersion(cg.getTargetVersion()).setCode(tt.getCode()) 089 .setDisplay(tt.getDisplay()); 090 } 091 092 private boolean isOkEquivalence(ConceptMapEquivalence equivalence) { 093 return equivalence != null && equivalence != ConceptMapEquivalence.DISJOINT 094 && equivalence != ConceptMapEquivalence.UNMATCHED; 095 } 096 097 private Coding translateBySystem(ConceptMap cm, String system, String code) { 098 throw new Error("Not done yet"); 099 } 100 101}