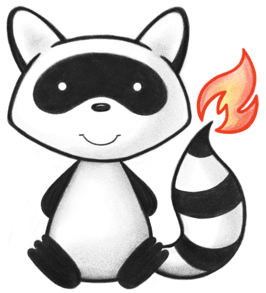
001package org.hl7.fhir.r4.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.FileNotFoundException; 033import java.io.IOException; 034 035import org.hl7.fhir.r4.model.Parameters; 036import org.hl7.fhir.r4.model.ValueSet; 037import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 038 039@MarkedToMoveToAdjunctPackage 040public interface ValueSetExpander { 041 public enum TerminologyServiceErrorClass { 042 UNKNOWN, NOSERVICE, SERVER_ERROR, VALUESET_UNSUPPORTED; 043 044 public boolean isInfrastructure() { 045 return this == NOSERVICE || this == SERVER_ERROR || this == VALUESET_UNSUPPORTED; 046 } 047 } 048 049 public class ETooCostly extends Exception { 050 051 public ETooCostly(String msg) { 052 super(msg); 053 } 054 055 } 056 057 /** 058 * Some value sets are just too big to expand. Instead of an expanded value set, 059 * you get back an interface that can test membership - usually on a server 060 * somewhere 061 * 062 * @author Grahame 063 */ 064 public class ValueSetExpansionOutcome { 065 private ValueSet valueset; 066 private String error; 067 private TerminologyServiceErrorClass errorClass; 068 private String txLink; 069 070 public ValueSetExpansionOutcome(ValueSet valueset) { 071 super(); 072 this.valueset = valueset; 073 this.error = null; 074 } 075 076 public ValueSetExpansionOutcome(ValueSet valueset, String error, TerminologyServiceErrorClass errorClass) { 077 super(); 078 this.valueset = valueset; 079 this.error = error; 080 this.errorClass = errorClass; 081 } 082 083 public ValueSetExpansionOutcome(ValueSetChecker service, String error, TerminologyServiceErrorClass errorClass) { 084 super(); 085 this.valueset = null; 086 this.error = error; 087 this.errorClass = errorClass; 088 } 089 090 public ValueSetExpansionOutcome(String error, TerminologyServiceErrorClass errorClass) { 091 this.valueset = null; 092 this.error = error; 093 this.errorClass = errorClass; 094 } 095 096 public ValueSet getValueset() { 097 return valueset; 098 } 099 100 public String getError() { 101 return error; 102 } 103 104 public TerminologyServiceErrorClass getErrorClass() { 105 return errorClass; 106 } 107 108 public String getTxLink() { 109 return txLink; 110 } 111 112 public ValueSetExpansionOutcome setTxLink(String txLink) { 113 this.txLink = txLink; 114 return this; 115 } 116 117 public boolean isOk() { 118 return error == null; 119 } 120 121 } 122 123 /** 124 * 125 * @param source the value set definition to expand 126 * @param profile a profile affecting the outcome. If you don't supply a 127 * profile, the default internal expansion profile will be used. 128 * 129 * @return 130 * @throws ETooCostly 131 * @throws FileNotFoundException 132 * @throws IOException 133 */ 134 public ValueSetExpansionOutcome expand(ValueSet source, Parameters parameters) 135 throws ETooCostly, FileNotFoundException, IOException; 136}