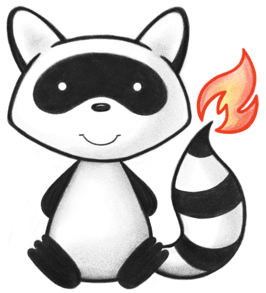
001package org.hl7.fhir.r4.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032/* 033Copyright (c) 2011+, HL7, Inc 034All rights reserved. 035 036Redistribution and use in source and binary forms, with or without modification, 037are permitted provided that the following conditions are met: 038 039 * Redistributions of source code must retain the above copyright notice, this 040 list of conditions and the following disclaimer. 041 * Redistributions in binary form must reproduce the above copyright notice, 042 this list of conditions and the following disclaimer in the documentation 043 and/or other materials provided with the distribution. 044 * Neither the name of HL7 nor the names of its contributors may be used to 045 endorse or promote products derived from this software without specific 046 prior written permission. 047 048THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 049ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 050WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 051IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 052INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 053NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 054PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 055WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 056ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 057POSSIBILITY OF SUCH DAMAGE. 058 059*/ 060 061import java.io.File; 062import java.io.FileInputStream; 063import java.io.FileOutputStream; 064import java.io.IOException; 065import java.util.HashMap; 066import java.util.Map; 067 068import org.apache.commons.io.IOUtils; 069import org.hl7.fhir.exceptions.FHIRFormatError; 070import org.hl7.fhir.r4.context.IWorkerContext; 071import org.hl7.fhir.r4.formats.IParser.OutputStyle; 072import org.hl7.fhir.r4.formats.JsonParser; 073import org.hl7.fhir.r4.model.MetadataResource; 074import org.hl7.fhir.r4.model.OperationOutcome; 075import org.hl7.fhir.r4.model.Parameters; 076import org.hl7.fhir.r4.model.Resource; 077import org.hl7.fhir.r4.model.ValueSet; 078import org.hl7.fhir.r4.terminologies.ValueSetExpander.TerminologyServiceErrorClass; 079import org.hl7.fhir.r4.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 080import org.hl7.fhir.r4.utils.ToolingExtensions; 081import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 082import org.hl7.fhir.utilities.Utilities; 083import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 084import org.hl7.fhir.utilities.xhtml.XhtmlComposer; 085 086@MarkedToMoveToAdjunctPackage 087public class ValueSetExpansionCache implements ValueSetExpanderFactory { 088 089 public class CacheAwareExpander implements ValueSetExpander { 090 091 @Override 092 public ValueSetExpansionOutcome expand(ValueSet source, Parameters expParams) throws ETooCostly, IOException { 093 String cacheKey = makeCacheKey(source, expParams); 094 if (expansions.containsKey(cacheKey)) 095 return expansions.get(cacheKey); 096 ValueSetExpander vse = new ValueSetExpanderSimple(context); 097 ValueSetExpansionOutcome vso = vse.expand(source, expParams); 098 if (vso.getError() != null) { 099 // well, we'll see if the designated server can expand it, and if it can, we'll 100 // cache it locally 101 vso = context.expandVS(source, false, expParams == null || !expParams.getParameterBool("excludeNested")); 102 if (cacheFolder != null) { 103 FileOutputStream s = ManagedFileAccess.outStream(Utilities.path(cacheFolder, makeFileName(source.getUrl()))); 104 context.newXmlParser().setOutputStyle(OutputStyle.PRETTY).compose(s, vso.getValueset()); 105 s.close(); 106 } 107 } 108 if (vso.getValueset() != null) 109 expansions.put(cacheKey, vso); 110 return vso; 111 } 112 113 private String makeCacheKey(ValueSet source, Parameters expParams) throws IOException { 114 if (expParams == null) 115 return source.getUrl(); 116 JsonParser p = new JsonParser(); 117 String r = p.composeString(expParams); 118 return source.getUrl() + " " + r.hashCode(); 119 } 120 121 } 122 123 private static final String VS_ID_EXT = "http://tools/cache"; 124 125 private final Map<String, ValueSetExpansionOutcome> expansions = new HashMap<String, ValueSetExpansionOutcome>(); 126 private final Map<String, MetadataResource> canonicals = new HashMap<String, MetadataResource>(); 127 private final IWorkerContext context; 128 private final String cacheFolder; 129 130 private Object lock; 131 132 public ValueSetExpansionCache(IWorkerContext context, Object lock) { 133 super(); 134 cacheFolder = null; 135 this.lock = lock; 136 this.context = context; 137 } 138 139 public ValueSetExpansionCache(IWorkerContext context, String cacheFolder, Object lock) 140 throws FHIRFormatError, IOException { 141 super(); 142 this.context = context; 143 this.cacheFolder = cacheFolder; 144 this.lock = lock; 145 if (this.cacheFolder != null) 146 loadCache(); 147 } 148 149 private String makeFileName(String url) { 150 return url.replace("$", "").replace(":", "").replace("|", ".").replace("//", "/").replace("/", "_") + ".xml"; 151 } 152 153 private void loadCache() throws FHIRFormatError, IOException { 154 File[] files = ManagedFileAccess.file(cacheFolder).listFiles(); 155 for (File f : files) { 156 if (f.getName().endsWith(".xml")) { 157 final FileInputStream is = ManagedFileAccess.inStream(f); 158 try { 159 Resource r = context.newXmlParser().setOutputStyle(OutputStyle.PRETTY).parse(is); 160 if (r instanceof OperationOutcome) { 161 OperationOutcome oo = (OperationOutcome) r; 162 expansions.put(ToolingExtensions.getExtension(oo, VS_ID_EXT).getValue().toString(), 163 new ValueSetExpansionOutcome( 164 new XhtmlComposer(XhtmlComposer.XML, false).composePlainText(oo.getText().getDiv()), 165 TerminologyServiceErrorClass.UNKNOWN)); 166 } else if (r instanceof ValueSet) { 167 ValueSet vs = (ValueSet) r; 168 if (vs.hasExpansion()) 169 expansions.put(vs.getUrl(), new ValueSetExpansionOutcome(vs)); 170 else { 171 canonicals.put(vs.getUrl(), vs); 172 if (vs.hasVersion()) 173 canonicals.put(vs.getUrl() + "|" + vs.getVersion(), vs); 174 } 175 } else if (r instanceof MetadataResource) { 176 MetadataResource md = (MetadataResource) r; 177 canonicals.put(md.getUrl(), md); 178 if (md.hasVersion()) 179 canonicals.put(md.getUrl() + "|" + md.getVersion(), md); 180 } 181 } finally { 182 IOUtils.closeQuietly(is); 183 } 184 } 185 } 186 } 187 188 @Override 189 public ValueSetExpander getExpander() { 190 return new CacheAwareExpander(); 191 // return new ValueSetExpander(valuesets, codesystems); 192 } 193 194 public MetadataResource getStoredResource(String canonicalUri) { 195 synchronized (lock) { 196 return canonicals.get(canonicalUri); 197 } 198 } 199 200 public void storeResource(MetadataResource md) throws IOException { 201 synchronized (lock) { 202 canonicals.put(md.getUrl(), md); 203 if (md.hasVersion()) 204 canonicals.put(md.getUrl() + "|" + md.getVersion(), md); 205 } 206 if (cacheFolder != null) { 207 FileOutputStream s = ManagedFileAccess.outStream( 208 Utilities.path(cacheFolder, makeFileName(md.getUrl() + "|" + md.getVersion()))); 209 context.newXmlParser().setOutputStyle(OutputStyle.PRETTY).compose(s, md); 210 s.close(); 211 } 212 } 213 214}