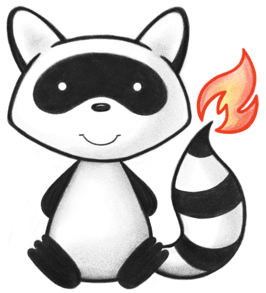
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.File; 033import java.io.FileInputStream; 034import java.io.FileNotFoundException; 035import java.io.IOException; 036import java.text.SimpleDateFormat; 037import java.util.Date; 038import java.util.UUID; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.r4.formats.IParser; 043import org.hl7.fhir.r4.formats.JsonParser; 044import org.hl7.fhir.r4.formats.XmlParser; 045import org.hl7.fhir.r4.model.Bundle; 046import org.hl7.fhir.r4.model.Bundle.BundleEntryComponent; 047import org.hl7.fhir.r4.model.Bundle.BundleType; 048import org.hl7.fhir.r4.model.Bundle.HTTPVerb; 049import org.hl7.fhir.r4.model.Resource; 050import org.hl7.fhir.r4.utils.client.FHIRToolingClient; 051import org.hl7.fhir.utilities.IniFile; 052import org.hl7.fhir.utilities.Utilities; 053 054public class BatchLoader { 055 056 public static void main(String[] args) throws IOException, Exception { 057 if (args.length < 3) { 058 System.out.println( 059 "Batch uploader takes 3 parameters in order: server base url, file/folder to upload, and batch size"); 060 } else { 061 String server = args[0]; 062 String file = args[1]; 063 int size = Integer.parseInt(args[2]); 064 if (file.endsWith(".xml")) { 065 throw new FHIRException("Unimplemented file type " + file); 066 } else if (file.endsWith(".json")) { 067 throw new FHIRException("Unimplemented file type " + file); 068// } else if (file.endsWith(".zip")) { 069// LoadZipFile(server, file, p, size, 0, -1); 070 } else if (new File(file).isDirectory()) { 071 LoadDirectory(server, file, size); 072 } else 073 throw new FHIRException("Unknown file type " + file); 074 } 075 } 076 077 private static void LoadDirectory(String server, String folder, int size) throws IOException, Exception { 078 System.out.print("Connecting to " + server + ".. "); 079 FHIRToolingClient client = new FHIRToolingClient(server, "fhir/batch-loader"); 080 System.out.println("Done"); 081 082 IniFile ini = new IniFile(Utilities.path(folder, "batch-load-progress.ini")); 083 for (File f : new File(folder).listFiles()) { 084 if (f.getName().endsWith(".json") || f.getName().endsWith(".xml")) { 085 if (!ini.getBooleanProperty("finished", f.getName())) { 086 sendFile(client, f, size, ini); 087 } 088 } 089 } 090 } 091 092 private static void sendFile(FHIRToolingClient client, File f, int size, IniFile ini) 093 throws FHIRFormatError, FileNotFoundException, IOException { 094 long ms = System.currentTimeMillis(); 095 System.out.print("Loading " + f.getName() + ".. "); 096 IParser parser = f.getName().endsWith(".json") ? new JsonParser() : new XmlParser(); 097 Resource res = parser.parse(new FileInputStream(f)); 098 System.out.println(" done: (" + Long.toString(System.currentTimeMillis() - ms) + " ms)"); 099 100 if (res instanceof Bundle) { 101 Bundle bnd = (Bundle) res; 102 int cursor = ini.hasProperty("progress", f.getName()) ? ini.getIntegerProperty("progress", f.getName()) : 0; 103 while (cursor < bnd.getEntry().size()) { 104 Bundle bt = new Bundle(); 105 bt.setType(BundleType.BATCH); 106 bt.setId(UUID.randomUUID().toString().toLowerCase()); 107 for (int i = cursor; i < Math.min(bnd.getEntry().size(), cursor + size); i++) { 108 BundleEntryComponent be = bt.addEntry(); 109 be.setResource(bnd.getEntry().get(i).getResource()); 110 be.getRequest().setMethod(HTTPVerb.PUT); 111 be.getRequest().setUrl(be.getResource().getResourceType().toString() + "/" + be.getResource().getId()); 112 } 113 System.out.print(f.getName() + " (" + cursor + "/" + bnd.getEntry().size() + "): "); 114 ms = System.currentTimeMillis(); 115 Bundle resp = client.transaction(bt); 116 117 int ncursor = cursor + size; 118 for (int i = 0; i < resp.getEntry().size(); i++) { 119 BundleEntryComponent t = resp.getEntry().get(i); 120 if (!t.getResponse().getStatus().startsWith("2")) { 121 System.out.println("failed status at " + Integer.toString(i) + ": " + t.getResponse().getStatus()); 122 ncursor = cursor + i - 1; 123 break; 124 } 125 } 126 cursor = ncursor; 127 System.out.println(" .. done: (" + Long.toString(System.currentTimeMillis() - ms) + " ms) " 128 + SimpleDateFormat.getInstance().format(new Date())); 129 ini.setIntegerProperty("progress", f.getName(), cursor, null); 130 ini.save(); 131 } 132 ini.setBooleanProperty("finished", f.getName(), true, null); 133 ini.save(); 134 } else { 135 client.update(res); 136 ini.setBooleanProperty("finished", f.getName(), true, null); 137 ini.save(); 138 } 139 } 140// 141// private static void LoadZipFile(String server, String file, IParser p, int size, int start, int end) throws IOException, Exception { 142// System.out.println("Load Zip file "+file); 143// Bundle b = new Bundle(); 144// b.setType(BundleType.COLLECTION); 145// b.setId(UUID.randomUUID().toString().toLowerCase()); 146// ZipInputStream zip = new ZipInputStream(new FileInputStream(file)); 147// ZipEntry entry; 148// while((entry = zip.getNextEntry())!=null) 149// { 150// try { 151// Resource r = p.parse(zip); 152// b.addEntry().setResource(r); 153// } catch (Exception e) { 154// throw new Exception("Error parsing "+entry.getName()+": "+e.getMessage(), e); 155// } 156// } 157// loadBundle(server, b, size, start, end); 158// } 159// 160// 161// private static int loadBundle(String server, Bundle b, int size, int start, int end) throws URISyntaxException { 162// System.out.println("Post to "+server+". size = "+Integer.toString(size)+", start = "+Integer.toString(start)+", total = "+Integer.toString(b.getEntry().size())); 163// FHIRToolingClient client = new FHIRToolingClient(server); 164// int c = start; 165// if (end == -1) 166// end = b.getEntry().size(); 167// while (c < end) { 168// Bundle bt = new Bundle(); 169// bt.setType(BundleType.BATCH); 170// bt.setId(UUID.randomUUID().toString().toLowerCase()); 171// for (int i = c; i < Math.min(b.getEntry().size(), c+size); i++) { 172// BundleEntryComponent be = bt.addEntry(); 173// be.setResource(b.getEntry().get(i).getResource()); 174// be.getRequest().setMethod(HTTPVerb.PUT); 175// be.getRequest().setUrl(be.getResource().getResourceType().toString()+"/"+be.getResource().getId()); 176// } 177// System.out.print(" posting.."); 178// long ms = System.currentTimeMillis(); 179// Bundle resp = client.transaction(bt); 180// 181// for (int i = 0; i < resp.getEntry().size(); i++) { 182// BundleEntryComponent t = resp.getEntry().get(i); 183// if (!t.getResponse().getStatus().startsWith("2")) { 184// System.out.println("failed status at "+Integer.toString(i)+": "+t.getResponse().getStatus()); 185// return c+i; 186// } 187// } 188// c = c + size; 189// System.out.println(" ..done: "+Integer.toString(c)+". ("+Long.toString(System.currentTimeMillis()-ms)+" ms)"); 190// } 191// System.out.println(" done"); 192// return c; 193// } 194 195}