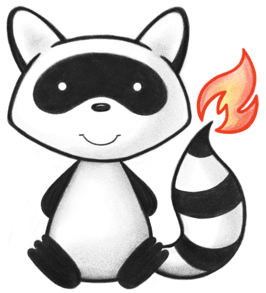
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.io.ByteArrayInputStream; 033import java.io.FileOutputStream; 034import java.io.OutputStream; 035import java.nio.file.Files; 036import java.nio.file.Paths; 037import java.security.KeyFactory; 038import java.security.PrivateKey; 039import java.security.PublicKey; 040import java.security.spec.PKCS8EncodedKeySpec; 041import java.security.spec.X509EncodedKeySpec; 042import java.util.Collections; 043 044import javax.xml.crypto.dsig.CanonicalizationMethod; 045import javax.xml.crypto.dsig.DigestMethod; 046import javax.xml.crypto.dsig.Reference; 047import javax.xml.crypto.dsig.SignatureMethod; 048import javax.xml.crypto.dsig.SignedInfo; 049import javax.xml.crypto.dsig.Transform; 050import javax.xml.crypto.dsig.XMLSignature; 051import javax.xml.crypto.dsig.XMLSignatureFactory; 052import javax.xml.crypto.dsig.dom.DOMSignContext; 053import javax.xml.crypto.dsig.keyinfo.KeyInfo; 054import javax.xml.crypto.dsig.keyinfo.KeyInfoFactory; 055import javax.xml.crypto.dsig.keyinfo.KeyValue; 056import javax.xml.crypto.dsig.spec.C14NMethodParameterSpec; 057import javax.xml.crypto.dsig.spec.TransformParameterSpec; 058import javax.xml.parsers.DocumentBuilder; 059import javax.xml.parsers.DocumentBuilderFactory; 060 061import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 062import org.hl7.fhir.utilities.Utilities; 063import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 064import org.hl7.fhir.utilities.xml.XMLUtil; 065import org.hl7.fhir.utilities.xml.XmlGenerator; 066import org.w3c.dom.Document; 067 068@MarkedToMoveToAdjunctPackage 069public class DigitalSignatures { 070 071 public static PrivateKey getPrivateKey(String filename) throws Exception { 072 073 byte[] keyBytes = Files.readAllBytes(Paths.get(filename)); 074 075 PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(keyBytes); 076 KeyFactory kf = KeyFactory.getInstance("RSA"); 077 return kf.generatePrivate(spec); 078 } 079 080 public static PublicKey getPublicKey(String filename) throws Exception { 081 082 byte[] keyBytes = Files.readAllBytes(Paths.get(filename)); 083 084 X509EncodedKeySpec spec = new X509EncodedKeySpec(keyBytes); 085 KeyFactory kf = KeyFactory.getInstance("RSA"); 086 return kf.generatePublic(spec); 087 } 088 089 public static void main(String[] args) throws Exception { 090 // http://docs.oracle.com/javase/7/docs/technotes/guides/security/xmldsig/XMLDigitalSignature.html 091 // 092 byte[] inputXml = "<Envelope xmlns=\"urn:envelope\">\r\n</Envelope>\r\n".getBytes(); 093 // load the document that's going to be signed 094 DocumentBuilderFactory dbf = XMLUtil.newXXEProtectedDocumentBuilderFactory(); 095 dbf.setNamespaceAware(true); 096 DocumentBuilder builder = dbf.newDocumentBuilder(); 097 Document doc = builder.parse(new ByteArrayInputStream(inputXml)); 098 099// // create a key pair 100// KeyPairGenerator kpg = KeyPairGenerator.getInstance("RSA"); 101// kpg.initialize(512); 102// KeyPair kp = kpg.generateKeyPair(); 103 PublicKey pub = getPublicKey("C:\\work\\fhirserver\\tests\\signatures\\public_key.der"); 104 PrivateKey priv = getPrivateKey("C:\\work\\fhirserver\\tests\\signatures\\private_key.der"); 105 106 // sign the document 107 DOMSignContext dsc = new DOMSignContext(priv, doc.getDocumentElement()); 108 XMLSignatureFactory fac = XMLSignatureFactory.getInstance("DOM"); 109 110 Reference ref = fac.newReference("", fac.newDigestMethod(DigestMethod.SHA1, null), 111 Collections.singletonList(fac.newTransform(Transform.ENVELOPED, (TransformParameterSpec) null)), null, null); 112 SignedInfo si = fac.newSignedInfo( 113 fac.newCanonicalizationMethod(CanonicalizationMethod.INCLUSIVE, (C14NMethodParameterSpec) null), 114 fac.newSignatureMethod(SignatureMethod.RSA_SHA1, null), Collections.singletonList(ref)); 115 116 KeyInfoFactory kif = fac.getKeyInfoFactory(); 117 KeyValue kv = kif.newKeyValue(pub); 118 KeyInfo ki = kif.newKeyInfo(Collections.singletonList(kv)); 119 XMLSignature signature = fac.newXMLSignature(si, ki); 120 signature.sign(dsc); 121 122 OutputStream os = ManagedFileAccess.outStream(Utilities.path("[tmp]", "java-digsig.xml")); 123 new XmlGenerator().generate(doc.getDocumentElement(), os); 124 } 125}