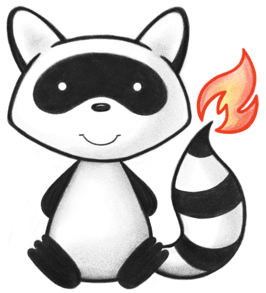
001package org.hl7.fhir.r4.utils; 002 003import java.io.File; 004import java.io.FileOutputStream; 005import java.io.FileNotFoundException; 006import java.io.IOException; 007import java.util.Arrays; 008import java.util.Set; 009import java.util.HashSet; 010 011import org.hl7.fhir.r4.formats.IParser.OutputStyle; 012import org.hl7.fhir.r4.formats.JsonParser; 013import org.hl7.fhir.r4.model.Resource; 014import org.hl7.fhir.r4.model.MetadataResource; 015import org.hl7.fhir.r4.model.Parameters; 016import org.hl7.fhir.utilities.FileUtilities; 017import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 018 019/** 020 * Used to take an overload dump from tx.fhir.org and turn it into a parameters resource 021 * 022 * @author grahamegrieve 023 * 024 */ 025@Deprecated 026public class ParametersBuilder { 027 028 public static void main(String[] args) throws FileNotFoundException, IOException { 029 new ParametersBuilder(args[0], args[1]).process(args[2]); 030 } 031 032 033 034 private String folder; 035 private String baseId; 036 037 protected ParametersBuilder(String folder, String baseId) { 038 super(); 039 this.folder = folder; 040 this.baseId = baseId; 041 } 042 043 private void process(String output) throws FileNotFoundException, IOException { 044 Parameters p = new Parameters(); 045 Set<String> ids = new HashSet<>(); 046 for (File f : ManagedFileAccess.file(folder).listFiles()) { 047 if (f.getName().startsWith(baseId)) { 048 if (f.getName().startsWith(baseId)) { 049 byte[] cnt = FileUtilities.fileToBytes(f); 050 cnt = shaveZeros(cnt); // bug in tx.fhir.org 051 MetadataResource r = (MetadataResource) new JsonParser().parse(cnt); 052 if (!ids.contains(r.getUrl()+"|"+r.getVersion())) { 053 ids.add(r.getUrl()+"|"+r.getVersion()); 054 p.addParameter().setName("tx-resource").setResource(r); 055 } 056 } 057 } 058 } 059 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(output), p); 060 } 061 062 private byte[] shaveZeros(byte[] cnt) { 063 for (int i = 0; i < cnt.length; i++) { 064 if (cnt[i] == 0) { 065 return Arrays.copyOf(cnt, i-1); 066 } 067 } 068 return cnt; 069 } 070}