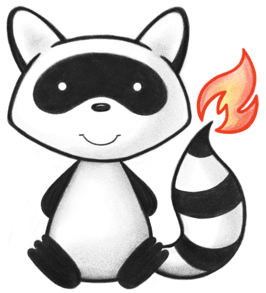
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.ArrayList; 033import java.util.List; 034 035import org.hl7.fhir.utilities.Utilities; 036 037public class TypesUtilities { 038 039 public enum TypeClassification { 040 PRIMITIVE, DATATYPE, METADATATYPE, SPECIAL; 041 042 public String toDisplay() { 043 switch (this) { 044 case DATATYPE: 045 return "Data Type"; 046 case METADATATYPE: 047 return "MetaDataType"; 048 case PRIMITIVE: 049 return "Primitive Type"; 050 case SPECIAL: 051 return "Special Type"; 052 } 053 return "??"; 054 } 055 } 056 057 public static class WildcardInformation { 058 private TypeClassification classification; 059 private String typeName; 060 private String comment; 061 062 public WildcardInformation(String typeName, String comment, TypeClassification classification) { 063 super(); 064 this.typeName = typeName; 065 this.comment = comment; 066 this.classification = classification; 067 } 068 069 public WildcardInformation(String typeName, TypeClassification classification) { 070 super(); 071 this.typeName = typeName; 072 this.classification = classification; 073 } 074 075 public String getTypeName() { 076 return typeName; 077 } 078 079 public String getComment() { 080 return comment; 081 } 082 083 public TypeClassification getClassification() { 084 return classification; 085 } 086 087 } 088 089 public static List<String> wildcardTypes() { 090 List<String> res = new ArrayList<String>(); 091 for (WildcardInformation wi : wildcards()) 092 res.add(wi.getTypeName()); 093 return res; 094 } 095 096 // this is the master list for what data types are allowed where the types = * 097 // that this list is incomplete means that the following types cannot have fixed 098 // values in a profile: 099 // Narrative 100 // Meta 101 // Any of the IDMP data types 102 // You have to walk into them to profile them. 103 // 104 public static List<WildcardInformation> wildcards() { 105 List<WildcardInformation> res = new ArrayList<WildcardInformation>(); 106 107 // primitive types 108 res.add(new WildcardInformation("base64Binary", TypeClassification.PRIMITIVE)); 109 res.add(new WildcardInformation("boolean", TypeClassification.PRIMITIVE)); 110 res.add(new WildcardInformation("canonical", TypeClassification.PRIMITIVE)); 111 res.add(new WildcardInformation("code", 112 "(only if the extension definition provides a <a href=\"terminologies.html#code\">fixed</a> binding to a suitable set of codes)", 113 TypeClassification.PRIMITIVE)); 114 res.add(new WildcardInformation("date", TypeClassification.PRIMITIVE)); 115 res.add(new WildcardInformation("dateTime", TypeClassification.PRIMITIVE)); 116 res.add(new WildcardInformation("decimal", TypeClassification.PRIMITIVE)); 117 res.add(new WildcardInformation("id", TypeClassification.PRIMITIVE)); 118 res.add(new WildcardInformation("instant", TypeClassification.PRIMITIVE)); 119 res.add(new WildcardInformation("integer", TypeClassification.PRIMITIVE)); 120 res.add(new WildcardInformation("markdown", TypeClassification.PRIMITIVE)); 121 res.add(new WildcardInformation("oid", TypeClassification.PRIMITIVE)); 122 res.add(new WildcardInformation("positiveInt", TypeClassification.PRIMITIVE)); 123 res.add(new WildcardInformation("string", TypeClassification.PRIMITIVE)); 124 res.add(new WildcardInformation("time", TypeClassification.PRIMITIVE)); 125 res.add(new WildcardInformation("unsignedInt", TypeClassification.PRIMITIVE)); 126 res.add(new WildcardInformation("uri", TypeClassification.PRIMITIVE)); 127 res.add(new WildcardInformation("url", TypeClassification.PRIMITIVE)); 128 res.add(new WildcardInformation("uuid", TypeClassification.PRIMITIVE)); 129 130 // Complex general purpose data types 131 res.add(new WildcardInformation("Address", TypeClassification.DATATYPE)); 132 res.add(new WildcardInformation("Age", TypeClassification.DATATYPE)); 133 res.add(new WildcardInformation("Annotation", TypeClassification.DATATYPE)); 134 res.add(new WildcardInformation("Attachment", TypeClassification.DATATYPE)); 135 res.add(new WildcardInformation("CodeableConcept", TypeClassification.DATATYPE)); 136 res.add(new WildcardInformation("Coding", TypeClassification.DATATYPE)); 137 res.add(new WildcardInformation("ContactPoint", TypeClassification.DATATYPE)); 138 res.add(new WildcardInformation("Count", TypeClassification.DATATYPE)); 139 res.add(new WildcardInformation("Distance", TypeClassification.DATATYPE)); 140 res.add(new WildcardInformation("Duration", TypeClassification.DATATYPE)); 141 res.add(new WildcardInformation("HumanName", TypeClassification.DATATYPE)); 142 res.add(new WildcardInformation("Identifier", TypeClassification.DATATYPE)); 143 res.add(new WildcardInformation("Money", TypeClassification.DATATYPE)); 144 res.add(new WildcardInformation("Period", TypeClassification.DATATYPE)); 145 res.add(new WildcardInformation("Quantity", TypeClassification.DATATYPE)); 146 res.add(new WildcardInformation("Range", TypeClassification.DATATYPE)); 147 res.add(new WildcardInformation("Ratio", TypeClassification.DATATYPE)); 148 res.add(new WildcardInformation("Reference", " - a reference to another resource", TypeClassification.DATATYPE)); 149 res.add(new WildcardInformation("SampledData", TypeClassification.DATATYPE)); 150 res.add(new WildcardInformation("Signature", TypeClassification.DATATYPE)); 151 res.add(new WildcardInformation("Timing", TypeClassification.DATATYPE)); 152 153 // metadata types 154 res.add(new WildcardInformation("ContactDetail", TypeClassification.METADATATYPE)); 155 res.add(new WildcardInformation("Contributor", TypeClassification.METADATATYPE)); 156 res.add(new WildcardInformation("DataRequirement", TypeClassification.METADATATYPE)); 157 res.add(new WildcardInformation("Expression", TypeClassification.METADATATYPE)); 158 res.add(new WildcardInformation("ParameterDefinition", TypeClassification.METADATATYPE)); 159 res.add(new WildcardInformation("RelatedArtifact", TypeClassification.METADATATYPE)); 160 res.add(new WildcardInformation("TriggerDefinition", TypeClassification.METADATATYPE)); 161 res.add(new WildcardInformation("UsageContext", TypeClassification.METADATATYPE)); 162 163 // special cases 164 res.add(new WildcardInformation("Dosage", TypeClassification.SPECIAL)); 165 res.add(new WildcardInformation("Meta", TypeClassification.SPECIAL)); 166 return res; 167 } 168 169 public static boolean isPrimitive(String code) { 170 return Utilities.existsInList(code, "boolean", "integer", "string", "decimal", "uri", "url", "canonical", 171 "base64Binary", "instant", "date", "dateTime", "time", "code", "oid", "id", "markdown", "unsignedInt", 172 "positiveInt", "xhtml"); 173 } 174}