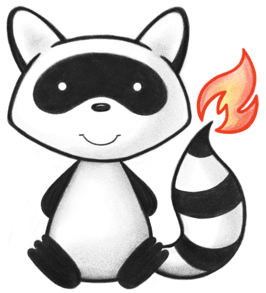
001package org.hl7.fhir.r4.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.ArrayList; 033import java.util.HashSet; 034import java.util.List; 035import java.util.Set; 036 037import org.hl7.fhir.r4.model.StructureDefinition; 038import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 039 040@MarkedToMoveToAdjunctPackage 041public class ValidationProfileSet { 042 043 public static class ProfileRegistration { 044 private String profile; 045 private boolean errorOnMissing; 046 047 public ProfileRegistration(String profile, boolean errorOnMissing) { 048 super(); 049 this.profile = profile; 050 this.errorOnMissing = errorOnMissing; 051 } 052 053 public String getProfile() { 054 return profile; 055 } 056 057 public boolean isErrorOnMissing() { 058 return errorOnMissing; 059 } 060 061 } 062 063 private List<ProfileRegistration> canonical = new ArrayList<ProfileRegistration>(); 064 private List<StructureDefinition> definitions = new ArrayList<StructureDefinition>(); 065 066 public ValidationProfileSet(String profile, boolean isError) { 067 super(); 068 canonical.add(new ProfileRegistration(profile, isError)); 069 } 070 071 public ValidationProfileSet() { 072 super(); 073 } 074 075 public ValidationProfileSet(StructureDefinition profile) { 076 super(); 077 definitions.add(profile); 078 canonical.add(new ProfileRegistration(profile.getUrl(), true)); 079 } 080 081 public ValidationProfileSet(List<String> profiles, boolean isError) { 082 super(); 083 if (profiles != null) 084 for (String p : profiles) 085 canonical.add(new ProfileRegistration(p, isError)); 086 } 087 088 public List<String> getCanonicalUrls() { 089 List<String> res = new ArrayList<String>(); 090 for (ProfileRegistration c : canonical) { 091 res.add(c.getProfile()); 092 } 093 return res; 094 } 095 096 public List<StructureDefinition> getDefinitions() { 097 return definitions; 098 } 099 100 public boolean empty() { 101 return canonical.isEmpty() && definitions.isEmpty(); 102 } 103 104 public List<String> getCanonicalAll() { 105 Set<String> res = new HashSet<String>(); 106 res.addAll(getCanonicalUrls()); 107 for (StructureDefinition sd : definitions) 108 res.add(sd.getUrl()); 109 return new ArrayList<String>(res); 110 } 111 112 public List<ProfileRegistration> getCanonical() { 113 return canonical; 114 } 115 116 public StructureDefinition fetch(String effectiveProfile) { 117 for (StructureDefinition sd : definitions) 118 if (effectiveProfile.equals(sd.getUrl())) 119 return sd; 120 return null; 121 } 122 123}