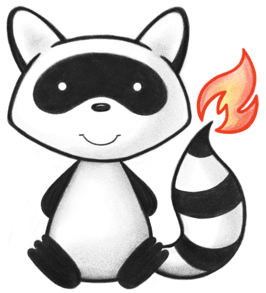
001package org.hl7.fhir.r4.utils.client.network; 002 003import java.io.ByteArrayOutputStream; 004import java.io.IOException; 005import java.io.OutputStreamWriter; 006import java.nio.charset.StandardCharsets; 007import java.util.Map; 008 009import org.hl7.fhir.r4.formats.IParser; 010import org.hl7.fhir.r4.formats.JsonParser; 011import org.hl7.fhir.r4.formats.XmlParser; 012import org.hl7.fhir.r4.model.Resource; 013import org.hl7.fhir.r4.utils.client.EFhirClientException; 014 015public class ByteUtils { 016 017 public static <T extends Resource> byte[] resourceToByteArray(T resource, boolean pretty, boolean isJson, boolean noXhtml) { 018 ByteArrayOutputStream baos = null; 019 byte[] byteArray = null; 020 try { 021 baos = new ByteArrayOutputStream(); 022 IParser parser = null; 023 if (isJson) { 024 parser = new JsonParser(); 025 } else { 026 parser = new XmlParser(); 027 } 028 parser.setOutputStyle(pretty ? IParser.OutputStyle.PRETTY : IParser.OutputStyle.NORMAL); 029 if (noXhtml) { 030 parser.setSuppressXhtml("Narrative removed"); 031 } 032 parser.compose(baos, resource); 033 baos.close(); 034 byteArray = baos.toByteArray(); 035 baos.close(); 036 } catch (Exception e) { 037 try { 038 baos.close(); 039 } catch (Exception ex) { 040 throw new EFhirClientException(0, "Error closing output stream", ex); 041 } 042 throw new EFhirClientException(0, "Error converting output stream to byte array", e); 043 } 044 return byteArray; 045 } 046 047 public static byte[] encodeFormSubmission(Map<String, String> parameters, String resourceName, Resource resource, 048 String boundary) throws IOException { 049 ByteArrayOutputStream b = new ByteArrayOutputStream(); 050 OutputStreamWriter w = new OutputStreamWriter(b, StandardCharsets.UTF_8); 051 for (String name : parameters.keySet()) { 052 w.write("--"); 053 w.write(boundary); 054 w.write("\r\nContent-Disposition: form-data; name=\"" + name + "\"\r\n\r\n"); 055 w.write(parameters.get(name) + "\r\n"); 056 } 057 w.write("--"); 058 w.write(boundary); 059 w.write("\r\nContent-Disposition: form-data; name=\"" + resourceName + "\"\r\n\r\n"); 060 w.close(); 061 JsonParser json = new JsonParser(); 062 json.setOutputStyle(IParser.OutputStyle.NORMAL); 063 json.compose(b, resource); 064 b.close(); 065 w = new OutputStreamWriter(b, StandardCharsets.UTF_8); 066 w.write("\r\n--"); 067 w.write(boundary); 068 w.write("--"); 069 w.close(); 070 return b.toByteArray(); 071 } 072}