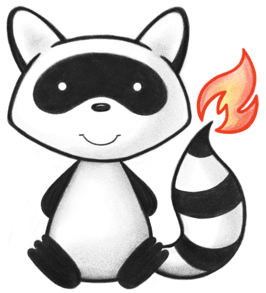
001package org.hl7.fhir.r4.utils.client.network; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.stream.Collectors; 006 007import org.hl7.fhir.exceptions.FHIRException; 008 009import org.hl7.fhir.utilities.http.HTTPHeader; 010 011/** 012 * Generic Implementation of Client Headers. 013 * 014 * Stores a list of headers for HTTP calls to the TX server. Users can implement 015 * their own instance if they desire specific, custom behavior. 016 */ 017public class ClientHeaders { 018 019 private final ArrayList<HTTPHeader> headers; 020 021 public ClientHeaders() { 022 this.headers = new ArrayList<>(); 023 } 024 025 public ClientHeaders(ArrayList<HTTPHeader> headers) { 026 this.headers = headers; 027 } 028 029 public ArrayList<HTTPHeader> headers() { 030 return headers; 031 } 032 033 /** 034 * Add a header to the list of stored headers for network operations. 035 * 036 * @param header {@link HTTPHeader} to add to the list. 037 * @throws FHIRException if the header being added is a duplicate. 038 */ 039 public ClientHeaders addHeader(HTTPHeader header) throws FHIRException { 040 if (headers.contains(header)) { 041 throw new FHIRException("Attempting to add duplicate header, <" + header.getName() + ", " + header.getValue() + ">."); 042 } 043 headers.add(header); 044 return this; 045 } 046 047 /** 048 * Add a header to the list of stored headers for network operations. 049 * 050 * @param headerList {@link List} of {@link HTTPHeader} to add. 051 * @throws FHIRException if any of the headers being added is a duplicate. 052 */ 053 public ClientHeaders addHeaders(List<HTTPHeader> headerList) throws FHIRException { 054 headerList.forEach(this::addHeader); 055 return this; 056 } 057 058 /** 059 * Removes the passed in header from the list of stored headers. 060 * 061 * @param header {@link HTTPHeader} to remove from the list. 062 * @throws FHIRException if the header passed in does not exist within the 063 * stored list. 064 */ 065 public ClientHeaders removeHeader(HTTPHeader header) throws FHIRException { 066 if (!headers.remove(header)) { 067 throw new FHIRException("Attempting to remove header, <" + header.getName() + ", " + header.getValue() 068 + ">, from GenericClientHeaders that is not currently stored."); 069 } 070 return this; 071 } 072 073 /** 074 * Removes the passed in headers from the list of stored headers. 075 * 076 * @param headerList {@link List} of {@link HTTPHeader} to remove. 077 * @throws FHIRException if any of the headers passed in does not exist within 078 * the stored list. 079 */ 080 public ClientHeaders removeHeaders(List<HTTPHeader> headerList) throws FHIRException { 081 headerList.forEach(this::removeHeader); 082 return this; 083 } 084 085 /** 086 * Clears all stored {@link HTTPHeader}. 087 */ 088 public ClientHeaders clearHeaders() { 089 headers.clear(); 090 return this; 091 } 092 093 @Override 094 public String toString() { 095 return this.headers.stream().map(header -> "\t" + header.getName() + ":" + header.getValue()) 096 .collect(Collectors.joining(",\n", "{\n", "\n}")); 097 } 098}