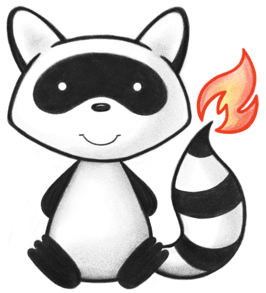
001package org.hl7.fhir.r4.utils.formats; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import java.util.Stack; 033 034import org.w3c.dom.Document; 035import org.w3c.dom.Element; 036import org.w3c.dom.Node; 037import org.w3c.dom.UserDataHandler; 038import org.w3c.dom.events.Event; 039import org.w3c.dom.events.EventListener; 040import org.w3c.dom.events.EventTarget; 041import org.w3c.dom.events.MutationEvent; 042import org.xml.sax.Attributes; 043import org.xml.sax.Locator; 044import org.xml.sax.SAXException; 045import org.xml.sax.XMLReader; 046import org.xml.sax.helpers.LocatorImpl; 047import org.xml.sax.helpers.XMLFilterImpl; 048 049// http://javacoalface.blogspot.com.au/2011/04/line-and-column-numbers-in-xml-dom.html 050 051@Deprecated 052public class XmlLocationAnnotator extends XMLFilterImpl { 053 054 private Locator locator; 055 private Stack<Locator> locatorStack = new Stack<Locator>(); 056 private Stack<Element> elementStack = new Stack<Element>(); 057 private UserDataHandler dataHandler = new LocationDataHandler(); 058 059 public XmlLocationAnnotator(XMLReader xmlReader, Document dom) { 060 super(xmlReader); 061 062 // Add listener to DOM, so we know which node was added. 063 EventListener modListener = new EventListener() { 064 @Override 065 public void handleEvent(Event e) { 066 EventTarget target = ((MutationEvent) e).getTarget(); 067 elementStack.push((Element) target); 068 } 069 }; 070 ((EventTarget) dom).addEventListener("DOMNodeInserted", modListener, true); 071 } 072 073 @Override 074 public void setDocumentLocator(Locator locator) { 075 super.setDocumentLocator(locator); 076 this.locator = locator; 077 } 078 079 @Override 080 public void startElement(String uri, String localName, String qName, Attributes atts) throws SAXException { 081 super.startElement(uri, localName, qName, atts); 082 083 // Keep snapshot of start location, 084 // for later when end of element is found. 085 locatorStack.push(new LocatorImpl(locator)); 086 } 087 088 @Override 089 public void endElement(String uri, String localName, String qName) throws SAXException { 090 091 // Mutation event fired by the adding of element end, 092 // and so lastAddedElement will be set. 093 super.endElement(uri, localName, qName); 094 095 if (locatorStack.size() > 0) { 096 Locator startLocator = locatorStack.pop(); 097 098 XmlLocationData location = new XmlLocationData(startLocator.getSystemId(), startLocator.getLineNumber(), 099 startLocator.getColumnNumber(), locator.getLineNumber(), locator.getColumnNumber()); 100 Element lastAddedElement = elementStack.pop(); 101 102 lastAddedElement.setUserData(XmlLocationData.LOCATION_DATA_KEY, location, dataHandler); 103 } 104 } 105 106 // Ensure location data copied to any new DOM node. 107 private class LocationDataHandler implements UserDataHandler { 108 109 @Override 110 public void handle(short operation, String key, Object data, Node src, Node dst) { 111 112 if (src != null && dst != null) { 113 XmlLocationData locatonData = (XmlLocationData) src.getUserData(XmlLocationData.LOCATION_DATA_KEY); 114 115 if (locatonData != null) { 116 dst.setUserData(XmlLocationData.LOCATION_DATA_KEY, locatonData, dataHandler); 117 } 118 } 119 } 120 } 121}