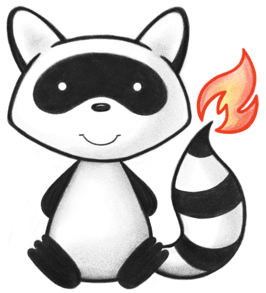
001package org.hl7.fhir.r4.utils.sql; 002 003import org.hl7.fhir.r4.utils.sql.Column; 004import org.hl7.fhir.r4.utils.sql.ColumnKind; 005import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 006 007@MarkedToMoveToAdjunctPackage 008public class Column { 009 010 private String name; 011 private int length; 012 private String type; 013 private ColumnKind kind; 014 private boolean isColl; 015 private boolean duplicateReported; 016 017 protected Column() { 018 super(); 019 } 020 021 protected Column(String name, boolean isColl, String type, ColumnKind kind) { 022 super(); 023 this.name = name; 024 this.isColl = isColl; 025 this.type = type; 026 this.kind = kind; 027 } 028 029 public String getName() { 030 return name; 031 } 032 public int getLength() { 033 return length; 034 } 035 public ColumnKind getKind() { 036 return kind; 037 } 038 039 public void setName(String name) { 040 this.name = name; 041 } 042 043 public void setLength(int length) { 044 this.length = length; 045 } 046 047 public void setKind(ColumnKind kind) { 048 this.kind = kind; 049 } 050 051 public String getType() { 052 return type; 053 } 054 055 public void setType(String type) { 056 this.type = type; 057 } 058 059 public boolean isColl() { 060 return isColl; 061 } 062 063 public void setColl(boolean isColl) { 064 this.isColl = isColl; 065 } 066 067 public String diff(Column other) { 068 if (!name.equals(other.name)) { 069 return "Names differ: '"+name+"' vs '"+other.name+"'"; 070 } 071 if (kind != ColumnKind.Null && other.kind != ColumnKind.Null) { 072 if (length != other.length) { 073 return "Lengths differ: '"+length+"' vs '"+other.length+"'"; 074 } 075 if (kind != other.kind) { 076 return "Kinds differ: '"+kind+"' vs '"+other.kind+"'"; 077 } 078 if (isColl != other.isColl) { 079 return "Collection status differs: '"+isColl+"' vs '"+other.isColl+"'"; 080 } 081 } else if (kind == ColumnKind.Null) { 082 kind = other.kind; 083 length = other.length; 084 isColl = other.isColl; 085 } 086 return null; 087 } 088 089 public boolean isDuplicateReported() { 090 return duplicateReported; 091 } 092 093 public void setDuplicateReported(boolean duplicateReported) { 094 this.duplicateReported = duplicateReported; 095 } 096 097 @Override 098 public String toString() { 099 return "Column [name=" + name + ", length=" + length + ", type=" + type + ", kind=" + kind + ", isColl=" + isColl 100 + "]"; 101 } 102 103 104}