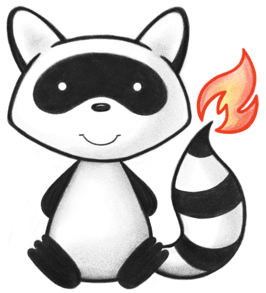
001package org.hl7.fhir.r4.utils.sql; 002 003import java.math.BigDecimal; 004import java.util.Date; 005 006import org.hl7.fhir.r4.model.Base; 007import org.hl7.fhir.r4.utils.sql.Value; 008import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 009 010 011/** 012 * String value is always provided, and a more specific value may also be provided 013 */ 014 015@MarkedToMoveToAdjunctPackage 016public class Value { 017 018 private String valueString; 019 private Boolean valueBoolean; 020 private Date valueDate; 021 private Integer valueInt; 022 private BigDecimal valueDecimal; 023 private byte[] valueBinary; 024 private Base valueComplex; 025 026 private Value() { 027 super(); 028 } 029 030 public static Value makeString(String s) { 031 Value v = new Value(); 032 v.valueString = s; 033 return v; 034 } 035 036 public static Value makeBoolean(String s, Boolean b) { 037 Value v = new Value(); 038 v.valueString = s; 039 v.valueBoolean = b; 040 return v; 041 } 042 043 public static Value makeDate(String s, Date d) { 044 Value v = new Value(); 045 v.valueString = s; 046 v.valueDate = d; 047 return v; 048 } 049 050 public static Value makeInteger(String s, Integer i) { 051 Value v = new Value(); 052 v.valueString = s; 053 v.valueInt = i; 054 return v; 055 } 056 057 058 public static Value makeDecimal(String s, BigDecimal bigDecimal) { 059 Value v = new Value(); 060 v.valueString = s; 061 v.valueDecimal = bigDecimal; 062 return v; 063 } 064 065 public static Value makeBinary(String s, byte[] b) { 066 Value v = new Value(); 067 v.valueString = s; 068 v.valueBinary = b; 069 return v; 070 } 071 072 public static Value makeComplex(Base b) { 073 Value v = new Value(); 074 v.valueComplex = b; 075 return v; 076 } 077 public String getValueString() { 078 return valueString; 079 } 080 081 public Date getValueDate() { 082 return valueDate; 083 } 084 085 public Integer getValueInt() { 086 return valueInt; 087 } 088 089 public BigDecimal getValueDecimal() { 090 return valueDecimal; 091 } 092 093 public byte[] getValueBinary() { 094 return valueBinary; 095 } 096 097 public Boolean getValueBoolean() { 098 return valueBoolean; 099 } 100 101 public Base getValueComplex() { 102 return valueComplex; 103 } 104 105 public boolean hasValueString() { 106 return valueString != null; 107 } 108 109 public boolean hasValueDate() { 110 return valueDate != null; 111 } 112 113 public boolean hasValueInt() { 114 return valueInt != null; 115 } 116 117 public boolean hasValueDecimal() { 118 return valueDecimal != null; 119 } 120 121 public boolean hasValueBinary() { 122 return valueBinary != null; 123 } 124 125 public boolean hasValueBoolean() { 126 return valueBoolean != null; 127 } 128 129 public boolean hasValueComplex() { 130 return valueComplex != null; 131 } 132}