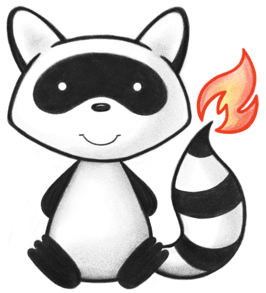
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.r4.hapi.ctx; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.FhirVersionEnum; 025import ca.uhn.fhir.context.RuntimeResourceDefinition; 026import ca.uhn.fhir.fhirpath.IFhirPath; 027import ca.uhn.fhir.i18n.Msg; 028import ca.uhn.fhir.model.api.IFhirVersion; 029import ca.uhn.fhir.model.primitive.IdDt; 030import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 031import ca.uhn.fhir.util.ReflectionUtil; 032import org.apache.commons.lang3.StringUtils; 033import org.hl7.fhir.instance.model.api.IBaseCoding; 034import org.hl7.fhir.instance.model.api.IBaseReference; 035import org.hl7.fhir.instance.model.api.IBaseResource; 036import org.hl7.fhir.instance.model.api.IIdType; 037import org.hl7.fhir.instance.model.api.IPrimitiveType; 038import org.hl7.fhir.r4.hapi.fluentpath.FhirPathR4; 039import org.hl7.fhir.r4.hapi.rest.server.R4BundleFactory; 040import org.hl7.fhir.r4.model.Coding; 041import org.hl7.fhir.r4.model.IdType; 042import org.hl7.fhir.r4.model.Reference; 043import org.hl7.fhir.r4.model.Resource; 044import org.hl7.fhir.r4.model.StructureDefinition; 045 046import java.io.InputStream; 047import java.util.Date; 048import java.util.List; 049 050public class FhirR4 implements IFhirVersion { 051 052 private String myId; 053 054 @Override 055 public IFhirPath createFhirPathExecutor(FhirContext theFhirContext) { 056 return new FhirPathR4(theFhirContext); 057 } 058 059 @Override 060 public IBaseResource generateProfile(RuntimeResourceDefinition theRuntimeResourceDefinition, String theServerBase) { 061 StructureDefinition retVal = new StructureDefinition(); 062 063 RuntimeResourceDefinition def = theRuntimeResourceDefinition; 064 065 myId = def.getId(); 066 if (StringUtils.isBlank(myId)) { 067 myId = theRuntimeResourceDefinition.getName().toLowerCase(); 068 } 069 070 retVal.setId(new IdDt(myId)); 071 return retVal; 072 } 073 074 @SuppressWarnings("rawtypes") 075 @Override 076 public Class<List> getContainedType() { 077 return List.class; 078 } 079 080 @Override 081 public InputStream getFhirVersionPropertiesFile() { 082 String path = "org/hl7/fhir/r4/hapi/model/fhirversion.properties"; 083 InputStream str = FhirR4.class.getResourceAsStream("/" + path); 084 if (str == null) { 085 str = FhirR4.class.getResourceAsStream(path); 086 } 087 if (str == null) { 088 throw new ConfigurationException(Msg.code(257) + "Can not find model property file on classpath: " + path); 089 } 090 return str; 091 } 092 093 @Override 094 public IPrimitiveType<Date> getLastUpdated(IBaseResource theResource) { 095 return ((Resource) theResource).getMeta().getLastUpdatedElement(); 096 } 097 098 @Override 099 public String getPathToSchemaDefinitions() { 100 return "/org/hl7/fhir/r4/model/schema"; 101 } 102 103 @Override 104 public Class<? extends IBaseReference> getResourceReferenceType() { 105 return Reference.class; 106 } 107 108 @Override 109 public Object getServerVersion() { 110 return ReflectionUtil.newInstanceOfFhirServerType("org.hl7.fhir.r4.hapi.ctx.FhirServerR4"); 111 } 112 113 @Override 114 public FhirVersionEnum getVersion() { 115 return FhirVersionEnum.R4; 116 } 117 118 @Override 119 public IVersionSpecificBundleFactory newBundleFactory(FhirContext theContext) { 120 return new R4BundleFactory(theContext); 121 } 122 123 @Override 124 public IBaseCoding newCodingDt() { 125 return new Coding(); 126 } 127 128 @Override 129 public IIdType newIdType() { 130 return new IdType(); 131 } 132}