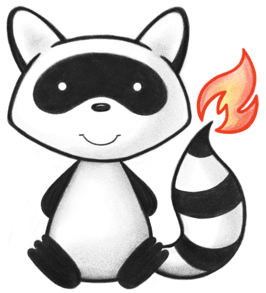
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * This resource allows for the definition of some activity to be performed, 052 * independent of a particular patient, practitioner, or other performance 053 * context. 054 */ 055@ResourceDef(name = "ActivityDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ActivityDefinition") 056@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "status", "experimental", 057 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 058 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 059 "endorser", "relatedArtifact", "library", "kind", "profile", "code", "intent", "priority", "doNotPerform", 060 "timing[x]", "location", "participant", "product[x]", "quantity", "dosage", "bodySite", "specimenRequirement", 061 "observationRequirement", "observationResultRequirement", "transform", "dynamicValue" }) 062public class ActivityDefinition extends MetadataResource { 063 064 public enum ActivityDefinitionKind { 065 /** 066 * A booking of a healthcare event among patient(s), practitioner(s), related 067 * person(s) and/or device(s) for a specific date/time. This may result in one 068 * or more Encounter(s). 069 */ 070 APPOINTMENT, 071 /** 072 * A reply to an appointment request for a patient and/or practitioner(s), such 073 * as a confirmation or rejection. 074 */ 075 APPOINTMENTRESPONSE, 076 /** 077 * Healthcare plan for patient or group. 078 */ 079 CAREPLAN, 080 /** 081 * Claim, Pre-determination or Pre-authorization. 082 */ 083 CLAIM, 084 /** 085 * A request for information to be sent to a receiver. 086 */ 087 COMMUNICATIONREQUEST, 088 /** 089 * Legal Agreement. 090 */ 091 CONTRACT, 092 /** 093 * Medical device request. 094 */ 095 DEVICEREQUEST, 096 /** 097 * Enrollment request. 098 */ 099 ENROLLMENTREQUEST, 100 /** 101 * Guidance or advice relating to an immunization. 102 */ 103 IMMUNIZATIONRECOMMENDATION, 104 /** 105 * Ordering of medication for patient or group. 106 */ 107 MEDICATIONREQUEST, 108 /** 109 * Diet, formula or nutritional supplement request. 110 */ 111 NUTRITIONORDER, 112 /** 113 * A record of a request for service such as diagnostic investigations, 114 * treatments, or operations to be performed. 115 */ 116 SERVICEREQUEST, 117 /** 118 * Request for a medication, substance or device. 119 */ 120 SUPPLYREQUEST, 121 /** 122 * A task to be performed. 123 */ 124 TASK, 125 /** 126 * Prescription for vision correction products for a patient. 127 */ 128 VISIONPRESCRIPTION, 129 /** 130 * added to help the parsers with the generic types 131 */ 132 NULL; 133 134 public static ActivityDefinitionKind fromCode(String codeString) throws FHIRException { 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("Appointment".equals(codeString)) 138 return APPOINTMENT; 139 if ("AppointmentResponse".equals(codeString)) 140 return APPOINTMENTRESPONSE; 141 if ("CarePlan".equals(codeString)) 142 return CAREPLAN; 143 if ("Claim".equals(codeString)) 144 return CLAIM; 145 if ("CommunicationRequest".equals(codeString)) 146 return COMMUNICATIONREQUEST; 147 if ("Contract".equals(codeString)) 148 return CONTRACT; 149 if ("DeviceRequest".equals(codeString)) 150 return DEVICEREQUEST; 151 if ("EnrollmentRequest".equals(codeString)) 152 return ENROLLMENTREQUEST; 153 if ("ImmunizationRecommendation".equals(codeString)) 154 return IMMUNIZATIONRECOMMENDATION; 155 if ("MedicationRequest".equals(codeString)) 156 return MEDICATIONREQUEST; 157 if ("NutritionOrder".equals(codeString)) 158 return NUTRITIONORDER; 159 if ("ServiceRequest".equals(codeString)) 160 return SERVICEREQUEST; 161 if ("SupplyRequest".equals(codeString)) 162 return SUPPLYREQUEST; 163 if ("Task".equals(codeString)) 164 return TASK; 165 if ("VisionPrescription".equals(codeString)) 166 return VISIONPRESCRIPTION; 167 if (Configuration.isAcceptInvalidEnums()) 168 return null; 169 else 170 throw new FHIRException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 171 } 172 173 public String toCode() { 174 switch (this) { 175 case APPOINTMENT: 176 return "Appointment"; 177 case APPOINTMENTRESPONSE: 178 return "AppointmentResponse"; 179 case CAREPLAN: 180 return "CarePlan"; 181 case CLAIM: 182 return "Claim"; 183 case COMMUNICATIONREQUEST: 184 return "CommunicationRequest"; 185 case CONTRACT: 186 return "Contract"; 187 case DEVICEREQUEST: 188 return "DeviceRequest"; 189 case ENROLLMENTREQUEST: 190 return "EnrollmentRequest"; 191 case IMMUNIZATIONRECOMMENDATION: 192 return "ImmunizationRecommendation"; 193 case MEDICATIONREQUEST: 194 return "MedicationRequest"; 195 case NUTRITIONORDER: 196 return "NutritionOrder"; 197 case SERVICEREQUEST: 198 return "ServiceRequest"; 199 case SUPPLYREQUEST: 200 return "SupplyRequest"; 201 case TASK: 202 return "Task"; 203 case VISIONPRESCRIPTION: 204 return "VisionPrescription"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getSystem() { 213 switch (this) { 214 case APPOINTMENT: 215 return "http://hl7.org/fhir/request-resource-types"; 216 case APPOINTMENTRESPONSE: 217 return "http://hl7.org/fhir/request-resource-types"; 218 case CAREPLAN: 219 return "http://hl7.org/fhir/request-resource-types"; 220 case CLAIM: 221 return "http://hl7.org/fhir/request-resource-types"; 222 case COMMUNICATIONREQUEST: 223 return "http://hl7.org/fhir/request-resource-types"; 224 case CONTRACT: 225 return "http://hl7.org/fhir/request-resource-types"; 226 case DEVICEREQUEST: 227 return "http://hl7.org/fhir/request-resource-types"; 228 case ENROLLMENTREQUEST: 229 return "http://hl7.org/fhir/request-resource-types"; 230 case IMMUNIZATIONRECOMMENDATION: 231 return "http://hl7.org/fhir/request-resource-types"; 232 case MEDICATIONREQUEST: 233 return "http://hl7.org/fhir/request-resource-types"; 234 case NUTRITIONORDER: 235 return "http://hl7.org/fhir/request-resource-types"; 236 case SERVICEREQUEST: 237 return "http://hl7.org/fhir/request-resource-types"; 238 case SUPPLYREQUEST: 239 return "http://hl7.org/fhir/request-resource-types"; 240 case TASK: 241 return "http://hl7.org/fhir/request-resource-types"; 242 case VISIONPRESCRIPTION: 243 return "http://hl7.org/fhir/request-resource-types"; 244 case NULL: 245 return null; 246 default: 247 return "?"; 248 } 249 } 250 251 public String getDefinition() { 252 switch (this) { 253 case APPOINTMENT: 254 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 255 case APPOINTMENTRESPONSE: 256 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 257 case CAREPLAN: 258 return "Healthcare plan for patient or group."; 259 case CLAIM: 260 return "Claim, Pre-determination or Pre-authorization."; 261 case COMMUNICATIONREQUEST: 262 return "A request for information to be sent to a receiver."; 263 case CONTRACT: 264 return "Legal Agreement."; 265 case DEVICEREQUEST: 266 return "Medical device request."; 267 case ENROLLMENTREQUEST: 268 return "Enrollment request."; 269 case IMMUNIZATIONRECOMMENDATION: 270 return "Guidance or advice relating to an immunization."; 271 case MEDICATIONREQUEST: 272 return "Ordering of medication for patient or group."; 273 case NUTRITIONORDER: 274 return "Diet, formula or nutritional supplement request."; 275 case SERVICEREQUEST: 276 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 277 case SUPPLYREQUEST: 278 return "Request for a medication, substance or device."; 279 case TASK: 280 return "A task to be performed."; 281 case VISIONPRESCRIPTION: 282 return "Prescription for vision correction products for a patient."; 283 case NULL: 284 return null; 285 default: 286 return "?"; 287 } 288 } 289 290 public String getDisplay() { 291 switch (this) { 292 case APPOINTMENT: 293 return "Appointment"; 294 case APPOINTMENTRESPONSE: 295 return "AppointmentResponse"; 296 case CAREPLAN: 297 return "CarePlan"; 298 case CLAIM: 299 return "Claim"; 300 case COMMUNICATIONREQUEST: 301 return "CommunicationRequest"; 302 case CONTRACT: 303 return "Contract"; 304 case DEVICEREQUEST: 305 return "DeviceRequest"; 306 case ENROLLMENTREQUEST: 307 return "EnrollmentRequest"; 308 case IMMUNIZATIONRECOMMENDATION: 309 return "ImmunizationRecommendation"; 310 case MEDICATIONREQUEST: 311 return "MedicationRequest"; 312 case NUTRITIONORDER: 313 return "NutritionOrder"; 314 case SERVICEREQUEST: 315 return "ServiceRequest"; 316 case SUPPLYREQUEST: 317 return "SupplyRequest"; 318 case TASK: 319 return "Task"; 320 case VISIONPRESCRIPTION: 321 return "VisionPrescription"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 } 329 330 public static class ActivityDefinitionKindEnumFactory implements EnumFactory<ActivityDefinitionKind> { 331 public ActivityDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 332 if (codeString == null || "".equals(codeString)) 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("Appointment".equals(codeString)) 336 return ActivityDefinitionKind.APPOINTMENT; 337 if ("AppointmentResponse".equals(codeString)) 338 return ActivityDefinitionKind.APPOINTMENTRESPONSE; 339 if ("CarePlan".equals(codeString)) 340 return ActivityDefinitionKind.CAREPLAN; 341 if ("Claim".equals(codeString)) 342 return ActivityDefinitionKind.CLAIM; 343 if ("CommunicationRequest".equals(codeString)) 344 return ActivityDefinitionKind.COMMUNICATIONREQUEST; 345 if ("Contract".equals(codeString)) 346 return ActivityDefinitionKind.CONTRACT; 347 if ("DeviceRequest".equals(codeString)) 348 return ActivityDefinitionKind.DEVICEREQUEST; 349 if ("EnrollmentRequest".equals(codeString)) 350 return ActivityDefinitionKind.ENROLLMENTREQUEST; 351 if ("ImmunizationRecommendation".equals(codeString)) 352 return ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION; 353 if ("MedicationRequest".equals(codeString)) 354 return ActivityDefinitionKind.MEDICATIONREQUEST; 355 if ("NutritionOrder".equals(codeString)) 356 return ActivityDefinitionKind.NUTRITIONORDER; 357 if ("ServiceRequest".equals(codeString)) 358 return ActivityDefinitionKind.SERVICEREQUEST; 359 if ("SupplyRequest".equals(codeString)) 360 return ActivityDefinitionKind.SUPPLYREQUEST; 361 if ("Task".equals(codeString)) 362 return ActivityDefinitionKind.TASK; 363 if ("VisionPrescription".equals(codeString)) 364 return ActivityDefinitionKind.VISIONPRESCRIPTION; 365 throw new IllegalArgumentException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 366 } 367 368 public Enumeration<ActivityDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 369 if (code == null) 370 return null; 371 if (code.isEmpty()) 372 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NULL, code); 373 String codeString = code.asStringValue(); 374 if (codeString == null || "".equals(codeString)) 375 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NULL, code); 376 if ("Appointment".equals(codeString)) 377 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENT, code); 378 if ("AppointmentResponse".equals(codeString)) 379 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.APPOINTMENTRESPONSE, code); 380 if ("CarePlan".equals(codeString)) 381 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CAREPLAN, code); 382 if ("Claim".equals(codeString)) 383 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CLAIM, code); 384 if ("CommunicationRequest".equals(codeString)) 385 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.COMMUNICATIONREQUEST, code); 386 if ("Contract".equals(codeString)) 387 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.CONTRACT, code); 388 if ("DeviceRequest".equals(codeString)) 389 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.DEVICEREQUEST, code); 390 if ("EnrollmentRequest".equals(codeString)) 391 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.ENROLLMENTREQUEST, code); 392 if ("ImmunizationRecommendation".equals(codeString)) 393 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION, code); 394 if ("MedicationRequest".equals(codeString)) 395 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.MEDICATIONREQUEST, code); 396 if ("NutritionOrder".equals(codeString)) 397 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.NUTRITIONORDER, code); 398 if ("ServiceRequest".equals(codeString)) 399 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SERVICEREQUEST, code); 400 if ("SupplyRequest".equals(codeString)) 401 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.SUPPLYREQUEST, code); 402 if ("Task".equals(codeString)) 403 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.TASK, code); 404 if ("VisionPrescription".equals(codeString)) 405 return new Enumeration<ActivityDefinitionKind>(this, ActivityDefinitionKind.VISIONPRESCRIPTION, code); 406 throw new FHIRException("Unknown ActivityDefinitionKind code '" + codeString + "'"); 407 } 408 409 public String toCode(ActivityDefinitionKind code) { 410 if (code == ActivityDefinitionKind.APPOINTMENT) 411 return "Appointment"; 412 if (code == ActivityDefinitionKind.APPOINTMENTRESPONSE) 413 return "AppointmentResponse"; 414 if (code == ActivityDefinitionKind.CAREPLAN) 415 return "CarePlan"; 416 if (code == ActivityDefinitionKind.CLAIM) 417 return "Claim"; 418 if (code == ActivityDefinitionKind.COMMUNICATIONREQUEST) 419 return "CommunicationRequest"; 420 if (code == ActivityDefinitionKind.CONTRACT) 421 return "Contract"; 422 if (code == ActivityDefinitionKind.DEVICEREQUEST) 423 return "DeviceRequest"; 424 if (code == ActivityDefinitionKind.ENROLLMENTREQUEST) 425 return "EnrollmentRequest"; 426 if (code == ActivityDefinitionKind.IMMUNIZATIONRECOMMENDATION) 427 return "ImmunizationRecommendation"; 428 if (code == ActivityDefinitionKind.MEDICATIONREQUEST) 429 return "MedicationRequest"; 430 if (code == ActivityDefinitionKind.NUTRITIONORDER) 431 return "NutritionOrder"; 432 if (code == ActivityDefinitionKind.SERVICEREQUEST) 433 return "ServiceRequest"; 434 if (code == ActivityDefinitionKind.SUPPLYREQUEST) 435 return "SupplyRequest"; 436 if (code == ActivityDefinitionKind.TASK) 437 return "Task"; 438 if (code == ActivityDefinitionKind.VISIONPRESCRIPTION) 439 return "VisionPrescription"; 440 return "?"; 441 } 442 443 public String toSystem(ActivityDefinitionKind code) { 444 return code.getSystem(); 445 } 446 } 447 448 public enum RequestIntent { 449 /** 450 * The request is a suggestion made by someone/something that does not have an 451 * intention to ensure it occurs and without providing an authorization to act. 452 */ 453 PROPOSAL, 454 /** 455 * The request represents an intention to ensure something occurs without 456 * providing an authorization for others to act. 457 */ 458 PLAN, 459 /** 460 * The request represents a legally binding instruction authored by a Patient or 461 * RelatedPerson. 462 */ 463 DIRECTIVE, 464 /** 465 * The request represents a request/demand and authorization for action by a 466 * Practitioner. 467 */ 468 ORDER, 469 /** 470 * The request represents an original authorization for action. 471 */ 472 ORIGINALORDER, 473 /** 474 * The request represents an automatically generated supplemental authorization 475 * for action based on a parent authorization together with initial results of 476 * the action taken against that parent authorization. 477 */ 478 REFLEXORDER, 479 /** 480 * The request represents the view of an authorization instantiated by a 481 * fulfilling system representing the details of the fulfiller's intention to 482 * act upon a submitted order. 483 */ 484 FILLERORDER, 485 /** 486 * An order created in fulfillment of a broader order that represents the 487 * authorization for a single activity occurrence. E.g. The administration of a 488 * single dose of a drug. 489 */ 490 INSTANCEORDER, 491 /** 492 * The request represents a component or option for a RequestGroup that 493 * establishes timing, conditionality and/or other constraints among a set of 494 * requests. Refer to [[[RequestGroup]]] for additional information on how this 495 * status is used. 496 */ 497 OPTION, 498 /** 499 * added to help the parsers with the generic types 500 */ 501 NULL; 502 503 public static RequestIntent fromCode(String codeString) throws FHIRException { 504 if (codeString == null || "".equals(codeString)) 505 return null; 506 if ("proposal".equals(codeString)) 507 return PROPOSAL; 508 if ("plan".equals(codeString)) 509 return PLAN; 510 if ("directive".equals(codeString)) 511 return DIRECTIVE; 512 if ("order".equals(codeString)) 513 return ORDER; 514 if ("original-order".equals(codeString)) 515 return ORIGINALORDER; 516 if ("reflex-order".equals(codeString)) 517 return REFLEXORDER; 518 if ("filler-order".equals(codeString)) 519 return FILLERORDER; 520 if ("instance-order".equals(codeString)) 521 return INSTANCEORDER; 522 if ("option".equals(codeString)) 523 return OPTION; 524 if (Configuration.isAcceptInvalidEnums()) 525 return null; 526 else 527 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 528 } 529 530 public String toCode() { 531 switch (this) { 532 case PROPOSAL: 533 return "proposal"; 534 case PLAN: 535 return "plan"; 536 case DIRECTIVE: 537 return "directive"; 538 case ORDER: 539 return "order"; 540 case ORIGINALORDER: 541 return "original-order"; 542 case REFLEXORDER: 543 return "reflex-order"; 544 case FILLERORDER: 545 return "filler-order"; 546 case INSTANCEORDER: 547 return "instance-order"; 548 case OPTION: 549 return "option"; 550 case NULL: 551 return null; 552 default: 553 return "?"; 554 } 555 } 556 557 public String getSystem() { 558 switch (this) { 559 case PROPOSAL: 560 return "http://hl7.org/fhir/request-intent"; 561 case PLAN: 562 return "http://hl7.org/fhir/request-intent"; 563 case DIRECTIVE: 564 return "http://hl7.org/fhir/request-intent"; 565 case ORDER: 566 return "http://hl7.org/fhir/request-intent"; 567 case ORIGINALORDER: 568 return "http://hl7.org/fhir/request-intent"; 569 case REFLEXORDER: 570 return "http://hl7.org/fhir/request-intent"; 571 case FILLERORDER: 572 return "http://hl7.org/fhir/request-intent"; 573 case INSTANCEORDER: 574 return "http://hl7.org/fhir/request-intent"; 575 case OPTION: 576 return "http://hl7.org/fhir/request-intent"; 577 case NULL: 578 return null; 579 default: 580 return "?"; 581 } 582 } 583 584 public String getDefinition() { 585 switch (this) { 586 case PROPOSAL: 587 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 588 case PLAN: 589 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 590 case DIRECTIVE: 591 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 592 case ORDER: 593 return "The request represents a request/demand and authorization for action by a Practitioner."; 594 case ORIGINALORDER: 595 return "The request represents an original authorization for action."; 596 case REFLEXORDER: 597 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 598 case FILLERORDER: 599 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 600 case INSTANCEORDER: 601 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 602 case OPTION: 603 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 604 case NULL: 605 return null; 606 default: 607 return "?"; 608 } 609 } 610 611 public String getDisplay() { 612 switch (this) { 613 case PROPOSAL: 614 return "Proposal"; 615 case PLAN: 616 return "Plan"; 617 case DIRECTIVE: 618 return "Directive"; 619 case ORDER: 620 return "Order"; 621 case ORIGINALORDER: 622 return "Original Order"; 623 case REFLEXORDER: 624 return "Reflex Order"; 625 case FILLERORDER: 626 return "Filler Order"; 627 case INSTANCEORDER: 628 return "Instance Order"; 629 case OPTION: 630 return "Option"; 631 case NULL: 632 return null; 633 default: 634 return "?"; 635 } 636 } 637 } 638 639 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 640 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 641 if (codeString == null || "".equals(codeString)) 642 if (codeString == null || "".equals(codeString)) 643 return null; 644 if ("proposal".equals(codeString)) 645 return RequestIntent.PROPOSAL; 646 if ("plan".equals(codeString)) 647 return RequestIntent.PLAN; 648 if ("directive".equals(codeString)) 649 return RequestIntent.DIRECTIVE; 650 if ("order".equals(codeString)) 651 return RequestIntent.ORDER; 652 if ("original-order".equals(codeString)) 653 return RequestIntent.ORIGINALORDER; 654 if ("reflex-order".equals(codeString)) 655 return RequestIntent.REFLEXORDER; 656 if ("filler-order".equals(codeString)) 657 return RequestIntent.FILLERORDER; 658 if ("instance-order".equals(codeString)) 659 return RequestIntent.INSTANCEORDER; 660 if ("option".equals(codeString)) 661 return RequestIntent.OPTION; 662 throw new IllegalArgumentException("Unknown RequestIntent code '" + codeString + "'"); 663 } 664 665 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 666 if (code == null) 667 return null; 668 if (code.isEmpty()) 669 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 670 String codeString = code.asStringValue(); 671 if (codeString == null || "".equals(codeString)) 672 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 673 if ("proposal".equals(codeString)) 674 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 675 if ("plan".equals(codeString)) 676 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 677 if ("directive".equals(codeString)) 678 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 679 if ("order".equals(codeString)) 680 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 681 if ("original-order".equals(codeString)) 682 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 683 if ("reflex-order".equals(codeString)) 684 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 685 if ("filler-order".equals(codeString)) 686 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 687 if ("instance-order".equals(codeString)) 688 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 689 if ("option".equals(codeString)) 690 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 691 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 692 } 693 694 public String toCode(RequestIntent code) { 695 if (code == RequestIntent.PROPOSAL) 696 return "proposal"; 697 if (code == RequestIntent.PLAN) 698 return "plan"; 699 if (code == RequestIntent.DIRECTIVE) 700 return "directive"; 701 if (code == RequestIntent.ORDER) 702 return "order"; 703 if (code == RequestIntent.ORIGINALORDER) 704 return "original-order"; 705 if (code == RequestIntent.REFLEXORDER) 706 return "reflex-order"; 707 if (code == RequestIntent.FILLERORDER) 708 return "filler-order"; 709 if (code == RequestIntent.INSTANCEORDER) 710 return "instance-order"; 711 if (code == RequestIntent.OPTION) 712 return "option"; 713 return "?"; 714 } 715 716 public String toSystem(RequestIntent code) { 717 return code.getSystem(); 718 } 719 } 720 721 public enum RequestPriority { 722 /** 723 * The request has normal priority. 724 */ 725 ROUTINE, 726 /** 727 * The request should be actioned promptly - higher priority than routine. 728 */ 729 URGENT, 730 /** 731 * The request should be actioned as soon as possible - higher priority than 732 * urgent. 733 */ 734 ASAP, 735 /** 736 * The request should be actioned immediately - highest possible priority. E.g. 737 * an emergency. 738 */ 739 STAT, 740 /** 741 * added to help the parsers with the generic types 742 */ 743 NULL; 744 745 public static RequestPriority fromCode(String codeString) throws FHIRException { 746 if (codeString == null || "".equals(codeString)) 747 return null; 748 if ("routine".equals(codeString)) 749 return ROUTINE; 750 if ("urgent".equals(codeString)) 751 return URGENT; 752 if ("asap".equals(codeString)) 753 return ASAP; 754 if ("stat".equals(codeString)) 755 return STAT; 756 if (Configuration.isAcceptInvalidEnums()) 757 return null; 758 else 759 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 760 } 761 762 public String toCode() { 763 switch (this) { 764 case ROUTINE: 765 return "routine"; 766 case URGENT: 767 return "urgent"; 768 case ASAP: 769 return "asap"; 770 case STAT: 771 return "stat"; 772 case NULL: 773 return null; 774 default: 775 return "?"; 776 } 777 } 778 779 public String getSystem() { 780 switch (this) { 781 case ROUTINE: 782 return "http://hl7.org/fhir/request-priority"; 783 case URGENT: 784 return "http://hl7.org/fhir/request-priority"; 785 case ASAP: 786 return "http://hl7.org/fhir/request-priority"; 787 case STAT: 788 return "http://hl7.org/fhir/request-priority"; 789 case NULL: 790 return null; 791 default: 792 return "?"; 793 } 794 } 795 796 public String getDefinition() { 797 switch (this) { 798 case ROUTINE: 799 return "The request has normal priority."; 800 case URGENT: 801 return "The request should be actioned promptly - higher priority than routine."; 802 case ASAP: 803 return "The request should be actioned as soon as possible - higher priority than urgent."; 804 case STAT: 805 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 806 case NULL: 807 return null; 808 default: 809 return "?"; 810 } 811 } 812 813 public String getDisplay() { 814 switch (this) { 815 case ROUTINE: 816 return "Routine"; 817 case URGENT: 818 return "Urgent"; 819 case ASAP: 820 return "ASAP"; 821 case STAT: 822 return "STAT"; 823 case NULL: 824 return null; 825 default: 826 return "?"; 827 } 828 } 829 } 830 831 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 832 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 833 if (codeString == null || "".equals(codeString)) 834 if (codeString == null || "".equals(codeString)) 835 return null; 836 if ("routine".equals(codeString)) 837 return RequestPriority.ROUTINE; 838 if ("urgent".equals(codeString)) 839 return RequestPriority.URGENT; 840 if ("asap".equals(codeString)) 841 return RequestPriority.ASAP; 842 if ("stat".equals(codeString)) 843 return RequestPriority.STAT; 844 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 845 } 846 847 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 848 if (code == null) 849 return null; 850 if (code.isEmpty()) 851 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 852 String codeString = code.asStringValue(); 853 if (codeString == null || "".equals(codeString)) 854 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 855 if ("routine".equals(codeString)) 856 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 857 if ("urgent".equals(codeString)) 858 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 859 if ("asap".equals(codeString)) 860 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 861 if ("stat".equals(codeString)) 862 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 863 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 864 } 865 866 public String toCode(RequestPriority code) { 867 if (code == RequestPriority.ROUTINE) 868 return "routine"; 869 if (code == RequestPriority.URGENT) 870 return "urgent"; 871 if (code == RequestPriority.ASAP) 872 return "asap"; 873 if (code == RequestPriority.STAT) 874 return "stat"; 875 return "?"; 876 } 877 878 public String toSystem(RequestPriority code) { 879 return code.getSystem(); 880 } 881 } 882 883 public enum ActivityParticipantType { 884 /** 885 * The participant is the patient under evaluation. 886 */ 887 PATIENT, 888 /** 889 * The participant is a practitioner involved in the patient's care. 890 */ 891 PRACTITIONER, 892 /** 893 * The participant is a person related to the patient. 894 */ 895 RELATEDPERSON, 896 /** 897 * The participant is a system or device used in the care of the patient. 898 */ 899 DEVICE, 900 /** 901 * added to help the parsers with the generic types 902 */ 903 NULL; 904 905 public static ActivityParticipantType fromCode(String codeString) throws FHIRException { 906 if (codeString == null || "".equals(codeString)) 907 return null; 908 if ("patient".equals(codeString)) 909 return PATIENT; 910 if ("practitioner".equals(codeString)) 911 return PRACTITIONER; 912 if ("related-person".equals(codeString)) 913 return RELATEDPERSON; 914 if ("device".equals(codeString)) 915 return DEVICE; 916 if (Configuration.isAcceptInvalidEnums()) 917 return null; 918 else 919 throw new FHIRException("Unknown ActivityParticipantType code '" + codeString + "'"); 920 } 921 922 public String toCode() { 923 switch (this) { 924 case PATIENT: 925 return "patient"; 926 case PRACTITIONER: 927 return "practitioner"; 928 case RELATEDPERSON: 929 return "related-person"; 930 case DEVICE: 931 return "device"; 932 case NULL: 933 return null; 934 default: 935 return "?"; 936 } 937 } 938 939 public String getSystem() { 940 switch (this) { 941 case PATIENT: 942 return "http://hl7.org/fhir/action-participant-type"; 943 case PRACTITIONER: 944 return "http://hl7.org/fhir/action-participant-type"; 945 case RELATEDPERSON: 946 return "http://hl7.org/fhir/action-participant-type"; 947 case DEVICE: 948 return "http://hl7.org/fhir/action-participant-type"; 949 case NULL: 950 return null; 951 default: 952 return "?"; 953 } 954 } 955 956 public String getDefinition() { 957 switch (this) { 958 case PATIENT: 959 return "The participant is the patient under evaluation."; 960 case PRACTITIONER: 961 return "The participant is a practitioner involved in the patient's care."; 962 case RELATEDPERSON: 963 return "The participant is a person related to the patient."; 964 case DEVICE: 965 return "The participant is a system or device used in the care of the patient."; 966 case NULL: 967 return null; 968 default: 969 return "?"; 970 } 971 } 972 973 public String getDisplay() { 974 switch (this) { 975 case PATIENT: 976 return "Patient"; 977 case PRACTITIONER: 978 return "Practitioner"; 979 case RELATEDPERSON: 980 return "Related Person"; 981 case DEVICE: 982 return "Device"; 983 case NULL: 984 return null; 985 default: 986 return "?"; 987 } 988 } 989 } 990 991 public static class ActivityParticipantTypeEnumFactory implements EnumFactory<ActivityParticipantType> { 992 public ActivityParticipantType fromCode(String codeString) throws IllegalArgumentException { 993 if (codeString == null || "".equals(codeString)) 994 if (codeString == null || "".equals(codeString)) 995 return null; 996 if ("patient".equals(codeString)) 997 return ActivityParticipantType.PATIENT; 998 if ("practitioner".equals(codeString)) 999 return ActivityParticipantType.PRACTITIONER; 1000 if ("related-person".equals(codeString)) 1001 return ActivityParticipantType.RELATEDPERSON; 1002 if ("device".equals(codeString)) 1003 return ActivityParticipantType.DEVICE; 1004 throw new IllegalArgumentException("Unknown ActivityParticipantType code '" + codeString + "'"); 1005 } 1006 1007 public Enumeration<ActivityParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 1008 if (code == null) 1009 return null; 1010 if (code.isEmpty()) 1011 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.NULL, code); 1012 String codeString = code.asStringValue(); 1013 if (codeString == null || "".equals(codeString)) 1014 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.NULL, code); 1015 if ("patient".equals(codeString)) 1016 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PATIENT, code); 1017 if ("practitioner".equals(codeString)) 1018 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.PRACTITIONER, code); 1019 if ("related-person".equals(codeString)) 1020 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.RELATEDPERSON, code); 1021 if ("device".equals(codeString)) 1022 return new Enumeration<ActivityParticipantType>(this, ActivityParticipantType.DEVICE, code); 1023 throw new FHIRException("Unknown ActivityParticipantType code '" + codeString + "'"); 1024 } 1025 1026 public String toCode(ActivityParticipantType code) { 1027 if (code == ActivityParticipantType.PATIENT) 1028 return "patient"; 1029 if (code == ActivityParticipantType.PRACTITIONER) 1030 return "practitioner"; 1031 if (code == ActivityParticipantType.RELATEDPERSON) 1032 return "related-person"; 1033 if (code == ActivityParticipantType.DEVICE) 1034 return "device"; 1035 return "?"; 1036 } 1037 1038 public String toSystem(ActivityParticipantType code) { 1039 return code.getSystem(); 1040 } 1041 } 1042 1043 @Block() 1044 public static class ActivityDefinitionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 1045 /** 1046 * The type of participant in the action. 1047 */ 1048 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1049 @Description(shortDefinition = "patient | practitioner | related-person | device", formalDefinition = "The type of participant in the action.") 1050 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-type") 1051 protected Enumeration<ActivityParticipantType> type; 1052 1053 /** 1054 * The role the participant should play in performing the described action. 1055 */ 1056 @Child(name = "role", type = { 1057 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1058 @Description(shortDefinition = "E.g. Nurse, Surgeon, Parent, etc.", formalDefinition = "The role the participant should play in performing the described action.") 1059 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-role") 1060 protected CodeableConcept role; 1061 1062 private static final long serialVersionUID = -1450932564L; 1063 1064 /** 1065 * Constructor 1066 */ 1067 public ActivityDefinitionParticipantComponent() { 1068 super(); 1069 } 1070 1071 /** 1072 * Constructor 1073 */ 1074 public ActivityDefinitionParticipantComponent(Enumeration<ActivityParticipantType> type) { 1075 super(); 1076 this.type = type; 1077 } 1078 1079 /** 1080 * @return {@link #type} (The type of participant in the action.). This is the 1081 * underlying object with id, value and extensions. The accessor 1082 * "getType" gives direct access to the value 1083 */ 1084 public Enumeration<ActivityParticipantType> getTypeElement() { 1085 if (this.type == null) 1086 if (Configuration.errorOnAutoCreate()) 1087 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.type"); 1088 else if (Configuration.doAutoCreate()) 1089 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); // bb 1090 return this.type; 1091 } 1092 1093 public boolean hasTypeElement() { 1094 return this.type != null && !this.type.isEmpty(); 1095 } 1096 1097 public boolean hasType() { 1098 return this.type != null && !this.type.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #type} (The type of participant in the action.). This is 1103 * the underlying object with id, value and extensions. The 1104 * accessor "getType" gives direct access to the value 1105 */ 1106 public ActivityDefinitionParticipantComponent setTypeElement(Enumeration<ActivityParticipantType> value) { 1107 this.type = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return The type of participant in the action. 1113 */ 1114 public ActivityParticipantType getType() { 1115 return this.type == null ? null : this.type.getValue(); 1116 } 1117 1118 /** 1119 * @param value The type of participant in the action. 1120 */ 1121 public ActivityDefinitionParticipantComponent setType(ActivityParticipantType value) { 1122 if (this.type == null) 1123 this.type = new Enumeration<ActivityParticipantType>(new ActivityParticipantTypeEnumFactory()); 1124 this.type.setValue(value); 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #role} (The role the participant should play in performing the 1130 * described action.) 1131 */ 1132 public CodeableConcept getRole() { 1133 if (this.role == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create ActivityDefinitionParticipantComponent.role"); 1136 else if (Configuration.doAutoCreate()) 1137 this.role = new CodeableConcept(); // cc 1138 return this.role; 1139 } 1140 1141 public boolean hasRole() { 1142 return this.role != null && !this.role.isEmpty(); 1143 } 1144 1145 /** 1146 * @param value {@link #role} (The role the participant should play in 1147 * performing the described action.) 1148 */ 1149 public ActivityDefinitionParticipantComponent setRole(CodeableConcept value) { 1150 this.role = value; 1151 return this; 1152 } 1153 1154 protected void listChildren(List<Property> children) { 1155 super.listChildren(children); 1156 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 1157 children.add(new Property("role", "CodeableConcept", 1158 "The role the participant should play in performing the described action.", 0, 1, role)); 1159 } 1160 1161 @Override 1162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1163 switch (_hash) { 1164 case 3575610: 1165 /* type */ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 1166 case 3506294: 1167 /* role */ return new Property("role", "CodeableConcept", 1168 "The role the participant should play in performing the described action.", 0, 1, role); 1169 default: 1170 return super.getNamedProperty(_hash, _name, _checkValid); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1177 switch (hash) { 1178 case 3575610: 1179 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ActivityParticipantType> 1180 case 3506294: 1181 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 1182 default: 1183 return super.getProperty(hash, name, checkValid); 1184 } 1185 1186 } 1187 1188 @Override 1189 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1190 switch (hash) { 1191 case 3575610: // type 1192 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 1193 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 1194 return value; 1195 case 3506294: // role 1196 this.role = castToCodeableConcept(value); // CodeableConcept 1197 return value; 1198 default: 1199 return super.setProperty(hash, name, value); 1200 } 1201 1202 } 1203 1204 @Override 1205 public Base setProperty(String name, Base value) throws FHIRException { 1206 if (name.equals("type")) { 1207 value = new ActivityParticipantTypeEnumFactory().fromType(castToCode(value)); 1208 this.type = (Enumeration) value; // Enumeration<ActivityParticipantType> 1209 } else if (name.equals("role")) { 1210 this.role = castToCodeableConcept(value); // CodeableConcept 1211 } else 1212 return super.setProperty(name, value); 1213 return value; 1214 } 1215 1216 @Override 1217 public Base makeProperty(int hash, String name) throws FHIRException { 1218 switch (hash) { 1219 case 3575610: 1220 return getTypeElement(); 1221 case 3506294: 1222 return getRole(); 1223 default: 1224 return super.makeProperty(hash, name); 1225 } 1226 1227 } 1228 1229 @Override 1230 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1231 switch (hash) { 1232 case 3575610: 1233 /* type */ return new String[] { "code" }; 1234 case 3506294: 1235 /* role */ return new String[] { "CodeableConcept" }; 1236 default: 1237 return super.getTypesForProperty(hash, name); 1238 } 1239 1240 } 1241 1242 @Override 1243 public Base addChild(String name) throws FHIRException { 1244 if (name.equals("type")) { 1245 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.type"); 1246 } else if (name.equals("role")) { 1247 this.role = new CodeableConcept(); 1248 return this.role; 1249 } else 1250 return super.addChild(name); 1251 } 1252 1253 public ActivityDefinitionParticipantComponent copy() { 1254 ActivityDefinitionParticipantComponent dst = new ActivityDefinitionParticipantComponent(); 1255 copyValues(dst); 1256 return dst; 1257 } 1258 1259 public void copyValues(ActivityDefinitionParticipantComponent dst) { 1260 super.copyValues(dst); 1261 dst.type = type == null ? null : type.copy(); 1262 dst.role = role == null ? null : role.copy(); 1263 } 1264 1265 @Override 1266 public boolean equalsDeep(Base other_) { 1267 if (!super.equalsDeep(other_)) 1268 return false; 1269 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 1270 return false; 1271 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 1272 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 1273 } 1274 1275 @Override 1276 public boolean equalsShallow(Base other_) { 1277 if (!super.equalsShallow(other_)) 1278 return false; 1279 if (!(other_ instanceof ActivityDefinitionParticipantComponent)) 1280 return false; 1281 ActivityDefinitionParticipantComponent o = (ActivityDefinitionParticipantComponent) other_; 1282 return compareValues(type, o.type, true); 1283 } 1284 1285 public boolean isEmpty() { 1286 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 1287 } 1288 1289 public String fhirType() { 1290 return "ActivityDefinition.participant"; 1291 1292 } 1293 1294 } 1295 1296 @Block() 1297 public static class ActivityDefinitionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 1298 /** 1299 * The path to the element to be customized. This is the path on the resource 1300 * that will hold the result of the calculation defined by the expression. The 1301 * specified path SHALL be a FHIRPath resolveable on the specified target type 1302 * of the ActivityDefinition, and SHALL consist only of identifiers, constant 1303 * indexers, and a restricted subset of functions. The path is allowed to 1304 * contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 1305 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 1306 * Profile](fhirpath.html#simple) for full details). 1307 */ 1308 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1309 @Description(shortDefinition = "The path to the element to be set dynamically", formalDefinition = "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).") 1310 protected StringType path; 1311 1312 /** 1313 * An expression specifying the value of the customized element. 1314 */ 1315 @Child(name = "expression", type = { 1316 Expression.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1317 @Description(shortDefinition = "An expression that provides the dynamic value for the customization", formalDefinition = "An expression specifying the value of the customized element.") 1318 protected Expression expression; 1319 1320 private static final long serialVersionUID = 1064529082L; 1321 1322 /** 1323 * Constructor 1324 */ 1325 public ActivityDefinitionDynamicValueComponent() { 1326 super(); 1327 } 1328 1329 /** 1330 * Constructor 1331 */ 1332 public ActivityDefinitionDynamicValueComponent(StringType path, Expression expression) { 1333 super(); 1334 this.path = path; 1335 this.expression = expression; 1336 } 1337 1338 /** 1339 * @return {@link #path} (The path to the element to be customized. This is the 1340 * path on the resource that will hold the result of the calculation 1341 * defined by the expression. The specified path SHALL be a FHIRPath 1342 * resolveable on the specified target type of the ActivityDefinition, 1343 * and SHALL consist only of identifiers, constant indexers, and a 1344 * restricted subset of functions. The path is allowed to contain 1345 * qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 1346 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 1347 * Profile](fhirpath.html#simple) for full details).). This is the 1348 * underlying object with id, value and extensions. The accessor 1349 * "getPath" gives direct access to the value 1350 */ 1351 public StringType getPathElement() { 1352 if (this.path == null) 1353 if (Configuration.errorOnAutoCreate()) 1354 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.path"); 1355 else if (Configuration.doAutoCreate()) 1356 this.path = new StringType(); // bb 1357 return this.path; 1358 } 1359 1360 public boolean hasPathElement() { 1361 return this.path != null && !this.path.isEmpty(); 1362 } 1363 1364 public boolean hasPath() { 1365 return this.path != null && !this.path.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #path} (The path to the element to be customized. This is 1370 * the path on the resource that will hold the result of the 1371 * calculation defined by the expression. The specified path SHALL 1372 * be a FHIRPath resolveable on the specified target type of the 1373 * ActivityDefinition, and SHALL consist only of identifiers, 1374 * constant indexers, and a restricted subset of functions. The 1375 * path is allowed to contain qualifiers (.) to traverse 1376 * sub-elements, as well as indexers ([x]) to traverse 1377 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1378 * Profile](fhirpath.html#simple) for full details).). This is the 1379 * underlying object with id, value and extensions. The accessor 1380 * "getPath" gives direct access to the value 1381 */ 1382 public ActivityDefinitionDynamicValueComponent setPathElement(StringType value) { 1383 this.path = value; 1384 return this; 1385 } 1386 1387 /** 1388 * @return The path to the element to be customized. This is the path on the 1389 * resource that will hold the result of the calculation defined by the 1390 * expression. The specified path SHALL be a FHIRPath resolveable on the 1391 * specified target type of the ActivityDefinition, and SHALL consist 1392 * only of identifiers, constant indexers, and a restricted subset of 1393 * functions. The path is allowed to contain qualifiers (.) to traverse 1394 * sub-elements, as well as indexers ([x]) to traverse 1395 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1396 * Profile](fhirpath.html#simple) for full details). 1397 */ 1398 public String getPath() { 1399 return this.path == null ? null : this.path.getValue(); 1400 } 1401 1402 /** 1403 * @param value The path to the element to be customized. This is the path on 1404 * the resource that will hold the result of the calculation 1405 * defined by the expression. The specified path SHALL be a 1406 * FHIRPath resolveable on the specified target type of the 1407 * ActivityDefinition, and SHALL consist only of identifiers, 1408 * constant indexers, and a restricted subset of functions. The 1409 * path is allowed to contain qualifiers (.) to traverse 1410 * sub-elements, as well as indexers ([x]) to traverse 1411 * multiple-cardinality sub-elements (see the [Simple FHIRPath 1412 * Profile](fhirpath.html#simple) for full details). 1413 */ 1414 public ActivityDefinitionDynamicValueComponent setPath(String value) { 1415 if (this.path == null) 1416 this.path = new StringType(); 1417 this.path.setValue(value); 1418 return this; 1419 } 1420 1421 /** 1422 * @return {@link #expression} (An expression specifying the value of the 1423 * customized element.) 1424 */ 1425 public Expression getExpression() { 1426 if (this.expression == null) 1427 if (Configuration.errorOnAutoCreate()) 1428 throw new Error("Attempt to auto-create ActivityDefinitionDynamicValueComponent.expression"); 1429 else if (Configuration.doAutoCreate()) 1430 this.expression = new Expression(); // cc 1431 return this.expression; 1432 } 1433 1434 public boolean hasExpression() { 1435 return this.expression != null && !this.expression.isEmpty(); 1436 } 1437 1438 /** 1439 * @param value {@link #expression} (An expression specifying the value of the 1440 * customized element.) 1441 */ 1442 public ActivityDefinitionDynamicValueComponent setExpression(Expression value) { 1443 this.expression = value; 1444 return this; 1445 } 1446 1447 protected void listChildren(List<Property> children) { 1448 super.listChildren(children); 1449 children.add(new Property("path", "string", 1450 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 1451 0, 1, path)); 1452 children.add(new Property("expression", "Expression", 1453 "An expression specifying the value of the customized element.", 0, 1, expression)); 1454 } 1455 1456 @Override 1457 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1458 switch (_hash) { 1459 case 3433509: 1460 /* path */ return new Property("path", "string", 1461 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 1462 0, 1, path); 1463 case -1795452264: 1464 /* expression */ return new Property("expression", "Expression", 1465 "An expression specifying the value of the customized element.", 0, 1, expression); 1466 default: 1467 return super.getNamedProperty(_hash, _name, _checkValid); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1474 switch (hash) { 1475 case 3433509: 1476 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1477 case -1795452264: 1478 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 1479 default: 1480 return super.getProperty(hash, name, checkValid); 1481 } 1482 1483 } 1484 1485 @Override 1486 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1487 switch (hash) { 1488 case 3433509: // path 1489 this.path = castToString(value); // StringType 1490 return value; 1491 case -1795452264: // expression 1492 this.expression = castToExpression(value); // Expression 1493 return value; 1494 default: 1495 return super.setProperty(hash, name, value); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base setProperty(String name, Base value) throws FHIRException { 1502 if (name.equals("path")) { 1503 this.path = castToString(value); // StringType 1504 } else if (name.equals("expression")) { 1505 this.expression = castToExpression(value); // Expression 1506 } else 1507 return super.setProperty(name, value); 1508 return value; 1509 } 1510 1511 @Override 1512 public Base makeProperty(int hash, String name) throws FHIRException { 1513 switch (hash) { 1514 case 3433509: 1515 return getPathElement(); 1516 case -1795452264: 1517 return getExpression(); 1518 default: 1519 return super.makeProperty(hash, name); 1520 } 1521 1522 } 1523 1524 @Override 1525 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1526 switch (hash) { 1527 case 3433509: 1528 /* path */ return new String[] { "string" }; 1529 case -1795452264: 1530 /* expression */ return new String[] { "Expression" }; 1531 default: 1532 return super.getTypesForProperty(hash, name); 1533 } 1534 1535 } 1536 1537 @Override 1538 public Base addChild(String name) throws FHIRException { 1539 if (name.equals("path")) { 1540 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.path"); 1541 } else if (name.equals("expression")) { 1542 this.expression = new Expression(); 1543 return this.expression; 1544 } else 1545 return super.addChild(name); 1546 } 1547 1548 public ActivityDefinitionDynamicValueComponent copy() { 1549 ActivityDefinitionDynamicValueComponent dst = new ActivityDefinitionDynamicValueComponent(); 1550 copyValues(dst); 1551 return dst; 1552 } 1553 1554 public void copyValues(ActivityDefinitionDynamicValueComponent dst) { 1555 super.copyValues(dst); 1556 dst.path = path == null ? null : path.copy(); 1557 dst.expression = expression == null ? null : expression.copy(); 1558 } 1559 1560 @Override 1561 public boolean equalsDeep(Base other_) { 1562 if (!super.equalsDeep(other_)) 1563 return false; 1564 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1565 return false; 1566 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1567 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 1568 } 1569 1570 @Override 1571 public boolean equalsShallow(Base other_) { 1572 if (!super.equalsShallow(other_)) 1573 return false; 1574 if (!(other_ instanceof ActivityDefinitionDynamicValueComponent)) 1575 return false; 1576 ActivityDefinitionDynamicValueComponent o = (ActivityDefinitionDynamicValueComponent) other_; 1577 return compareValues(path, o.path, true); 1578 } 1579 1580 public boolean isEmpty() { 1581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 1582 } 1583 1584 public String fhirType() { 1585 return "ActivityDefinition.dynamicValue"; 1586 1587 } 1588 1589 } 1590 1591 /** 1592 * A formal identifier that is used to identify this activity definition when it 1593 * is represented in other formats, or referenced in a specification, model, 1594 * design or an instance. 1595 */ 1596 @Child(name = "identifier", type = { 1597 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1598 @Description(shortDefinition = "Additional identifier for the activity definition", formalDefinition = "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1599 protected List<Identifier> identifier; 1600 1601 /** 1602 * An explanatory or alternate title for the activity definition giving 1603 * additional information about its content. 1604 */ 1605 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1606 @Description(shortDefinition = "Subordinate title of the activity definition", formalDefinition = "An explanatory or alternate title for the activity definition giving additional information about its content.") 1607 protected StringType subtitle; 1608 1609 /** 1610 * A code or group definition that describes the intended subject of the 1611 * activity being defined. 1612 */ 1613 @Child(name = "subject", type = { CodeableConcept.class, 1614 Group.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1615 @Description(shortDefinition = "Type of individual the activity definition is intended for", formalDefinition = "A code or group definition that describes the intended subject of the activity being defined.") 1616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 1617 protected Type subject; 1618 1619 /** 1620 * Explanation of why this activity definition is needed and why it has been 1621 * designed as it has. 1622 */ 1623 @Child(name = "purpose", type = { 1624 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1625 @Description(shortDefinition = "Why this activity definition is defined", formalDefinition = "Explanation of why this activity definition is needed and why it has been designed as it has.") 1626 protected MarkdownType purpose; 1627 1628 /** 1629 * A detailed description of how the activity definition is used from a clinical 1630 * perspective. 1631 */ 1632 @Child(name = "usage", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1633 @Description(shortDefinition = "Describes the clinical usage of the activity definition", formalDefinition = "A detailed description of how the activity definition is used from a clinical perspective.") 1634 protected StringType usage; 1635 1636 /** 1637 * A copyright statement relating to the activity definition and/or its 1638 * contents. Copyright statements are generally legal restrictions on the use 1639 * and publishing of the activity definition. 1640 */ 1641 @Child(name = "copyright", type = { 1642 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1643 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.") 1644 protected MarkdownType copyright; 1645 1646 /** 1647 * The date on which the resource content was approved by the publisher. 1648 * Approval happens once when the content is officially approved for usage. 1649 */ 1650 @Child(name = "approvalDate", type = { 1651 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1652 @Description(shortDefinition = "When the activity definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1653 protected DateType approvalDate; 1654 1655 /** 1656 * The date on which the resource content was last reviewed. Review happens 1657 * periodically after approval but does not change the original approval date. 1658 */ 1659 @Child(name = "lastReviewDate", type = { 1660 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1661 @Description(shortDefinition = "When the activity definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1662 protected DateType lastReviewDate; 1663 1664 /** 1665 * The period during which the activity definition content was or is planned to 1666 * be in active use. 1667 */ 1668 @Child(name = "effectivePeriod", type = { 1669 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1670 @Description(shortDefinition = "When the activity definition is expected to be used", formalDefinition = "The period during which the activity definition content was or is planned to be in active use.") 1671 protected Period effectivePeriod; 1672 1673 /** 1674 * Descriptive topics related to the content of the activity. Topics provide a 1675 * high-level categorization of the activity that can be useful for filtering 1676 * and searching. 1677 */ 1678 @Child(name = "topic", type = { 1679 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1680 @Description(shortDefinition = "E.g. Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.") 1681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 1682 protected List<CodeableConcept> topic; 1683 1684 /** 1685 * An individiual or organization primarily involved in the creation and 1686 * maintenance of the content. 1687 */ 1688 @Child(name = "author", type = { 1689 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1690 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 1691 protected List<ContactDetail> author; 1692 1693 /** 1694 * An individual or organization primarily responsible for internal coherence of 1695 * the content. 1696 */ 1697 @Child(name = "editor", type = { 1698 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1699 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 1700 protected List<ContactDetail> editor; 1701 1702 /** 1703 * An individual or organization primarily responsible for review of some aspect 1704 * of the content. 1705 */ 1706 @Child(name = "reviewer", type = { 1707 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1708 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 1709 protected List<ContactDetail> reviewer; 1710 1711 /** 1712 * An individual or organization responsible for officially endorsing the 1713 * content for use in some setting. 1714 */ 1715 @Child(name = "endorser", type = { 1716 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1717 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 1718 protected List<ContactDetail> endorser; 1719 1720 /** 1721 * Related artifacts such as additional documentation, justification, or 1722 * bibliographic references. 1723 */ 1724 @Child(name = "relatedArtifact", type = { 1725 RelatedArtifact.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1726 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 1727 protected List<RelatedArtifact> relatedArtifact; 1728 1729 /** 1730 * A reference to a Library resource containing any formal logic used by the 1731 * activity definition. 1732 */ 1733 @Child(name = "library", type = { 1734 CanonicalType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1735 @Description(shortDefinition = "Logic used by the activity definition", formalDefinition = "A reference to a Library resource containing any formal logic used by the activity definition.") 1736 protected List<CanonicalType> library; 1737 1738 /** 1739 * A description of the kind of resource the activity definition is 1740 * representing. For example, a MedicationRequest, a ServiceRequest, or a 1741 * CommunicationRequest. Typically, but not always, this is a Request resource. 1742 */ 1743 @Child(name = "kind", type = { CodeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 1744 @Description(shortDefinition = "Kind of resource", formalDefinition = "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.") 1745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-resource-types") 1746 protected Enumeration<ActivityDefinitionKind> kind; 1747 1748 /** 1749 * A profile to which the target of the activity definition is expected to 1750 * conform. 1751 */ 1752 @Child(name = "profile", type = { 1753 CanonicalType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1754 @Description(shortDefinition = "What profile the resource needs to conform to", formalDefinition = "A profile to which the target of the activity definition is expected to conform.") 1755 protected CanonicalType profile; 1756 1757 /** 1758 * Detailed description of the type of activity; e.g. What lab test, what 1759 * procedure, what kind of encounter. 1760 */ 1761 @Child(name = "code", type = { 1762 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 1763 @Description(shortDefinition = "Detail type of activity", formalDefinition = "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.") 1764 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 1765 protected CodeableConcept code; 1766 1767 /** 1768 * Indicates the level of authority/intentionality associated with the activity 1769 * and where the request should fit into the workflow chain. 1770 */ 1771 @Child(name = "intent", type = { CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1772 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.") 1773 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 1774 protected Enumeration<RequestIntent> intent; 1775 1776 /** 1777 * Indicates how quickly the activity should be addressed with respect to other 1778 * requests. 1779 */ 1780 @Child(name = "priority", type = { CodeType.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 1781 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the activity should be addressed with respect to other requests.") 1782 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1783 protected Enumeration<RequestPriority> priority; 1784 1785 /** 1786 * Set this to true if the definition is to indicate that a particular activity 1787 * should NOT be performed. If true, this element should be interpreted to 1788 * reinforce a negative coding. For example NPO as a code with a doNotPerform of 1789 * true would still indicate to NOT perform the action. 1790 */ 1791 @Child(name = "doNotPerform", type = { 1792 BooleanType.class }, order = 21, min = 0, max = 1, modifier = true, summary = true) 1793 @Description(shortDefinition = "True if the activity should not be performed", formalDefinition = "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.") 1794 protected BooleanType doNotPerform; 1795 1796 /** 1797 * The period, timing or frequency upon which the described activity is to 1798 * occur. 1799 */ 1800 @Child(name = "timing", type = { Timing.class, DateTimeType.class, Age.class, Period.class, Range.class, 1801 Duration.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 1802 @Description(shortDefinition = "When activity is to occur", formalDefinition = "The period, timing or frequency upon which the described activity is to occur.") 1803 protected Type timing; 1804 1805 /** 1806 * Identifies the facility where the activity will occur; e.g. home, hospital, 1807 * specific clinic, etc. 1808 */ 1809 @Child(name = "location", type = { Location.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 1810 @Description(shortDefinition = "Where it should happen", formalDefinition = "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.") 1811 protected Reference location; 1812 1813 /** 1814 * The actual object that is the target of the reference (Identifies the 1815 * facility where the activity will occur; e.g. home, hospital, specific clinic, 1816 * etc.) 1817 */ 1818 protected Location locationTarget; 1819 1820 /** 1821 * Indicates who should participate in performing the action described. 1822 */ 1823 @Child(name = "participant", type = {}, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1824 @Description(shortDefinition = "Who should participate in the action", formalDefinition = "Indicates who should participate in performing the action described.") 1825 protected List<ActivityDefinitionParticipantComponent> participant; 1826 1827 /** 1828 * Identifies the food, drug or other product being consumed or supplied in the 1829 * activity. 1830 */ 1831 @Child(name = "product", type = { Medication.class, Substance.class, 1832 CodeableConcept.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 1833 @Description(shortDefinition = "What's administered/supplied", formalDefinition = "Identifies the food, drug or other product being consumed or supplied in the activity.") 1834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1835 protected Type product; 1836 1837 /** 1838 * Identifies the quantity expected to be consumed at once (per dose, per meal, 1839 * etc.). 1840 */ 1841 @Child(name = "quantity", type = { Quantity.class }, order = 26, min = 0, max = 1, modifier = false, summary = false) 1842 @Description(shortDefinition = "How much is administered/consumed/supplied", formalDefinition = "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).") 1843 protected Quantity quantity; 1844 1845 /** 1846 * Provides detailed dosage instructions in the same way that they are described 1847 * for MedicationRequest resources. 1848 */ 1849 @Child(name = "dosage", type = { 1850 Dosage.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1851 @Description(shortDefinition = "Detailed dosage instructions", formalDefinition = "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.") 1852 protected List<Dosage> dosage; 1853 1854 /** 1855 * Indicates the sites on the subject's body where the procedure should be 1856 * performed (I.e. the target sites). 1857 */ 1858 @Child(name = "bodySite", type = { 1859 CodeableConcept.class }, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1860 @Description(shortDefinition = "What part of body to perform on", formalDefinition = "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).") 1861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1862 protected List<CodeableConcept> bodySite; 1863 1864 /** 1865 * Defines specimen requirements for the action to be performed, such as 1866 * required specimens for a lab test. 1867 */ 1868 @Child(name = "specimenRequirement", type = { 1869 SpecimenDefinition.class }, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1870 @Description(shortDefinition = "What specimens are required to perform this action", formalDefinition = "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.") 1871 protected List<Reference> specimenRequirement; 1872 /** 1873 * The actual objects that are the target of the reference (Defines specimen 1874 * requirements for the action to be performed, such as required specimens for a 1875 * lab test.) 1876 */ 1877 protected List<SpecimenDefinition> specimenRequirementTarget; 1878 1879 /** 1880 * Defines observation requirements for the action to be performed, such as body 1881 * weight or surface area. 1882 */ 1883 @Child(name = "observationRequirement", type = { 1884 ObservationDefinition.class }, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1885 @Description(shortDefinition = "What observations are required to perform this action", formalDefinition = "Defines observation requirements for the action to be performed, such as body weight or surface area.") 1886 protected List<Reference> observationRequirement; 1887 /** 1888 * The actual objects that are the target of the reference (Defines observation 1889 * requirements for the action to be performed, such as body weight or surface 1890 * area.) 1891 */ 1892 protected List<ObservationDefinition> observationRequirementTarget; 1893 1894 /** 1895 * Defines the observations that are expected to be produced by the action. 1896 */ 1897 @Child(name = "observationResultRequirement", type = { 1898 ObservationDefinition.class }, order = 31, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1899 @Description(shortDefinition = "What observations must be produced by this action", formalDefinition = "Defines the observations that are expected to be produced by the action.") 1900 protected List<Reference> observationResultRequirement; 1901 /** 1902 * The actual objects that are the target of the reference (Defines the 1903 * observations that are expected to be produced by the action.) 1904 */ 1905 protected List<ObservationDefinition> observationResultRequirementTarget; 1906 1907 /** 1908 * A reference to a StructureMap resource that defines a transform that can be 1909 * executed to produce the intent resource using the ActivityDefinition instance 1910 * as the input. 1911 */ 1912 @Child(name = "transform", type = { 1913 CanonicalType.class }, order = 32, min = 0, max = 1, modifier = false, summary = false) 1914 @Description(shortDefinition = "Transform to apply the template", formalDefinition = "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.") 1915 protected CanonicalType transform; 1916 1917 /** 1918 * Dynamic values that will be evaluated to produce values for elements of the 1919 * resulting resource. For example, if the dosage of a medication must be 1920 * computed based on the patient's weight, a dynamic value would be used to 1921 * specify an expression that calculated the weight, and the path on the request 1922 * resource that would contain the result. 1923 */ 1924 @Child(name = "dynamicValue", type = {}, order = 33, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1925 @Description(shortDefinition = "Dynamic aspects of the definition", formalDefinition = "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.") 1926 protected List<ActivityDefinitionDynamicValueComponent> dynamicValue; 1927 1928 private static final long serialVersionUID = 1488459022L; 1929 1930 /** 1931 * Constructor 1932 */ 1933 public ActivityDefinition() { 1934 super(); 1935 } 1936 1937 /** 1938 * Constructor 1939 */ 1940 public ActivityDefinition(Enumeration<PublicationStatus> status) { 1941 super(); 1942 this.status = status; 1943 } 1944 1945 /** 1946 * @return {@link #url} (An absolute URI that is used to identify this activity 1947 * definition when it is referenced in a specification, model, design or 1948 * an instance; also called its canonical identifier. This SHOULD be 1949 * globally unique and SHOULD be a literal address at which at which an 1950 * authoritative instance of this activity definition is (or will be) 1951 * published. This URL can be the target of a canonical reference. It 1952 * SHALL remain the same when the activity definition is stored on 1953 * different servers.). This is the underlying object with id, value and 1954 * extensions. The accessor "getUrl" gives direct access to the value 1955 */ 1956 public UriType getUrlElement() { 1957 if (this.url == null) 1958 if (Configuration.errorOnAutoCreate()) 1959 throw new Error("Attempt to auto-create ActivityDefinition.url"); 1960 else if (Configuration.doAutoCreate()) 1961 this.url = new UriType(); // bb 1962 return this.url; 1963 } 1964 1965 public boolean hasUrlElement() { 1966 return this.url != null && !this.url.isEmpty(); 1967 } 1968 1969 public boolean hasUrl() { 1970 return this.url != null && !this.url.isEmpty(); 1971 } 1972 1973 /** 1974 * @param value {@link #url} (An absolute URI that is used to identify this 1975 * activity definition when it is referenced in a specification, 1976 * model, design or an instance; also called its canonical 1977 * identifier. This SHOULD be globally unique and SHOULD be a 1978 * literal address at which at which an authoritative instance of 1979 * this activity definition is (or will be) published. This URL can 1980 * be the target of a canonical reference. It SHALL remain the same 1981 * when the activity definition is stored on different servers.). 1982 * This is the underlying object with id, value and extensions. The 1983 * accessor "getUrl" gives direct access to the value 1984 */ 1985 public ActivityDefinition setUrlElement(UriType value) { 1986 this.url = value; 1987 return this; 1988 } 1989 1990 /** 1991 * @return An absolute URI that is used to identify this activity definition 1992 * when it is referenced in a specification, model, design or an 1993 * instance; also called its canonical identifier. This SHOULD be 1994 * globally unique and SHOULD be a literal address at which at which an 1995 * authoritative instance of this activity definition is (or will be) 1996 * published. This URL can be the target of a canonical reference. It 1997 * SHALL remain the same when the activity definition is stored on 1998 * different servers. 1999 */ 2000 public String getUrl() { 2001 return this.url == null ? null : this.url.getValue(); 2002 } 2003 2004 /** 2005 * @param value An absolute URI that is used to identify this activity 2006 * definition when it is referenced in a specification, model, 2007 * design or an instance; also called its canonical identifier. 2008 * This SHOULD be globally unique and SHOULD be a literal address 2009 * at which at which an authoritative instance of this activity 2010 * definition is (or will be) published. This URL can be the target 2011 * of a canonical reference. It SHALL remain the same when the 2012 * activity definition is stored on different servers. 2013 */ 2014 public ActivityDefinition setUrl(String value) { 2015 if (Utilities.noString(value)) 2016 this.url = null; 2017 else { 2018 if (this.url == null) 2019 this.url = new UriType(); 2020 this.url.setValue(value); 2021 } 2022 return this; 2023 } 2024 2025 /** 2026 * @return {@link #identifier} (A formal identifier that is used to identify 2027 * this activity definition when it is represented in other formats, or 2028 * referenced in a specification, model, design or an instance.) 2029 */ 2030 public List<Identifier> getIdentifier() { 2031 if (this.identifier == null) 2032 this.identifier = new ArrayList<Identifier>(); 2033 return this.identifier; 2034 } 2035 2036 /** 2037 * @return Returns a reference to <code>this</code> for easy method chaining 2038 */ 2039 public ActivityDefinition setIdentifier(List<Identifier> theIdentifier) { 2040 this.identifier = theIdentifier; 2041 return this; 2042 } 2043 2044 public boolean hasIdentifier() { 2045 if (this.identifier == null) 2046 return false; 2047 for (Identifier item : this.identifier) 2048 if (!item.isEmpty()) 2049 return true; 2050 return false; 2051 } 2052 2053 public Identifier addIdentifier() { // 3 2054 Identifier t = new Identifier(); 2055 if (this.identifier == null) 2056 this.identifier = new ArrayList<Identifier>(); 2057 this.identifier.add(t); 2058 return t; 2059 } 2060 2061 public ActivityDefinition addIdentifier(Identifier t) { // 3 2062 if (t == null) 2063 return this; 2064 if (this.identifier == null) 2065 this.identifier = new ArrayList<Identifier>(); 2066 this.identifier.add(t); 2067 return this; 2068 } 2069 2070 /** 2071 * @return The first repetition of repeating field {@link #identifier}, creating 2072 * it if it does not already exist 2073 */ 2074 public Identifier getIdentifierFirstRep() { 2075 if (getIdentifier().isEmpty()) { 2076 addIdentifier(); 2077 } 2078 return getIdentifier().get(0); 2079 } 2080 2081 /** 2082 * @return {@link #version} (The identifier that is used to identify this 2083 * version of the activity definition when it is referenced in a 2084 * specification, model, design or instance. This is an arbitrary value 2085 * managed by the activity definition author and is not expected to be 2086 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2087 * if a managed version is not available. There is also no expectation 2088 * that versions can be placed in a lexicographical sequence. To provide 2089 * a version consistent with the Decision Support Service specification, 2090 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 2091 * information on versioning knowledge assets, refer to the Decision 2092 * Support Service specification. Note that a version is required for 2093 * non-experimental active assets.). This is the underlying object with 2094 * id, value and extensions. The accessor "getVersion" gives direct 2095 * access to the value 2096 */ 2097 public StringType getVersionElement() { 2098 if (this.version == null) 2099 if (Configuration.errorOnAutoCreate()) 2100 throw new Error("Attempt to auto-create ActivityDefinition.version"); 2101 else if (Configuration.doAutoCreate()) 2102 this.version = new StringType(); // bb 2103 return this.version; 2104 } 2105 2106 public boolean hasVersionElement() { 2107 return this.version != null && !this.version.isEmpty(); 2108 } 2109 2110 public boolean hasVersion() { 2111 return this.version != null && !this.version.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #version} (The identifier that is used to identify this 2116 * version of the activity definition when it is referenced in a 2117 * specification, model, design or instance. This is an arbitrary 2118 * value managed by the activity definition author and is not 2119 * expected to be globally unique. For example, it might be a 2120 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2121 * There is also no expectation that versions can be placed in a 2122 * lexicographical sequence. To provide a version consistent with 2123 * the Decision Support Service specification, use the format 2124 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2125 * versioning knowledge assets, refer to the Decision Support 2126 * Service specification. Note that a version is required for 2127 * non-experimental active assets.). This is the underlying object 2128 * with id, value and extensions. The accessor "getVersion" gives 2129 * direct access to the value 2130 */ 2131 public ActivityDefinition setVersionElement(StringType value) { 2132 this.version = value; 2133 return this; 2134 } 2135 2136 /** 2137 * @return The identifier that is used to identify this version of the activity 2138 * definition when it is referenced in a specification, model, design or 2139 * instance. This is an arbitrary value managed by the activity 2140 * definition author and is not expected to be globally unique. For 2141 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2142 * is not available. There is also no expectation that versions can be 2143 * placed in a lexicographical sequence. To provide a version consistent 2144 * with the Decision Support Service specification, use the format 2145 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 2146 * knowledge assets, refer to the Decision Support Service 2147 * specification. Note that a version is required for non-experimental 2148 * active assets. 2149 */ 2150 public String getVersion() { 2151 return this.version == null ? null : this.version.getValue(); 2152 } 2153 2154 /** 2155 * @param value The identifier that is used to identify this version of the 2156 * activity definition when it is referenced in a specification, 2157 * model, design or instance. This is an arbitrary value managed by 2158 * the activity definition author and is not expected to be 2159 * globally unique. For example, it might be a timestamp (e.g. 2160 * yyyymmdd) if a managed version is not available. There is also 2161 * no expectation that versions can be placed in a lexicographical 2162 * sequence. To provide a version consistent with the Decision 2163 * Support Service specification, use the format 2164 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2165 * versioning knowledge assets, refer to the Decision Support 2166 * Service specification. Note that a version is required for 2167 * non-experimental active assets. 2168 */ 2169 public ActivityDefinition setVersion(String value) { 2170 if (Utilities.noString(value)) 2171 this.version = null; 2172 else { 2173 if (this.version == null) 2174 this.version = new StringType(); 2175 this.version.setValue(value); 2176 } 2177 return this; 2178 } 2179 2180 /** 2181 * @return {@link #name} (A natural language name identifying the activity 2182 * definition. This name should be usable as an identifier for the 2183 * module by machine processing applications such as code generation.). 2184 * This is the underlying object with id, value and extensions. The 2185 * accessor "getName" gives direct access to the value 2186 */ 2187 public StringType getNameElement() { 2188 if (this.name == null) 2189 if (Configuration.errorOnAutoCreate()) 2190 throw new Error("Attempt to auto-create ActivityDefinition.name"); 2191 else if (Configuration.doAutoCreate()) 2192 this.name = new StringType(); // bb 2193 return this.name; 2194 } 2195 2196 public boolean hasNameElement() { 2197 return this.name != null && !this.name.isEmpty(); 2198 } 2199 2200 public boolean hasName() { 2201 return this.name != null && !this.name.isEmpty(); 2202 } 2203 2204 /** 2205 * @param value {@link #name} (A natural language name identifying the activity 2206 * definition. This name should be usable as an identifier for the 2207 * module by machine processing applications such as code 2208 * generation.). This is the underlying object with id, value and 2209 * extensions. The accessor "getName" gives direct access to the 2210 * value 2211 */ 2212 public ActivityDefinition setNameElement(StringType value) { 2213 this.name = value; 2214 return this; 2215 } 2216 2217 /** 2218 * @return A natural language name identifying the activity definition. This 2219 * name should be usable as an identifier for the module by machine 2220 * processing applications such as code generation. 2221 */ 2222 public String getName() { 2223 return this.name == null ? null : this.name.getValue(); 2224 } 2225 2226 /** 2227 * @param value A natural language name identifying the activity definition. 2228 * This name should be usable as an identifier for the module by 2229 * machine processing applications such as code generation. 2230 */ 2231 public ActivityDefinition setName(String value) { 2232 if (Utilities.noString(value)) 2233 this.name = null; 2234 else { 2235 if (this.name == null) 2236 this.name = new StringType(); 2237 this.name.setValue(value); 2238 } 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #title} (A short, descriptive, user-friendly title for the 2244 * activity definition.). This is the underlying object with id, value 2245 * and extensions. The accessor "getTitle" gives direct access to the 2246 * value 2247 */ 2248 public StringType getTitleElement() { 2249 if (this.title == null) 2250 if (Configuration.errorOnAutoCreate()) 2251 throw new Error("Attempt to auto-create ActivityDefinition.title"); 2252 else if (Configuration.doAutoCreate()) 2253 this.title = new StringType(); // bb 2254 return this.title; 2255 } 2256 2257 public boolean hasTitleElement() { 2258 return this.title != null && !this.title.isEmpty(); 2259 } 2260 2261 public boolean hasTitle() { 2262 return this.title != null && !this.title.isEmpty(); 2263 } 2264 2265 /** 2266 * @param value {@link #title} (A short, descriptive, user-friendly title for 2267 * the activity definition.). This is the underlying object with 2268 * id, value and extensions. The accessor "getTitle" gives direct 2269 * access to the value 2270 */ 2271 public ActivityDefinition setTitleElement(StringType value) { 2272 this.title = value; 2273 return this; 2274 } 2275 2276 /** 2277 * @return A short, descriptive, user-friendly title for the activity 2278 * definition. 2279 */ 2280 public String getTitle() { 2281 return this.title == null ? null : this.title.getValue(); 2282 } 2283 2284 /** 2285 * @param value A short, descriptive, user-friendly title for the activity 2286 * definition. 2287 */ 2288 public ActivityDefinition setTitle(String value) { 2289 if (Utilities.noString(value)) 2290 this.title = null; 2291 else { 2292 if (this.title == null) 2293 this.title = new StringType(); 2294 this.title.setValue(value); 2295 } 2296 return this; 2297 } 2298 2299 /** 2300 * @return {@link #subtitle} (An explanatory or alternate title for the activity 2301 * definition giving additional information about its content.). This is 2302 * the underlying object with id, value and extensions. The accessor 2303 * "getSubtitle" gives direct access to the value 2304 */ 2305 public StringType getSubtitleElement() { 2306 if (this.subtitle == null) 2307 if (Configuration.errorOnAutoCreate()) 2308 throw new Error("Attempt to auto-create ActivityDefinition.subtitle"); 2309 else if (Configuration.doAutoCreate()) 2310 this.subtitle = new StringType(); // bb 2311 return this.subtitle; 2312 } 2313 2314 public boolean hasSubtitleElement() { 2315 return this.subtitle != null && !this.subtitle.isEmpty(); 2316 } 2317 2318 public boolean hasSubtitle() { 2319 return this.subtitle != null && !this.subtitle.isEmpty(); 2320 } 2321 2322 /** 2323 * @param value {@link #subtitle} (An explanatory or alternate title for the 2324 * activity definition giving additional information about its 2325 * content.). This is the underlying object with id, value and 2326 * extensions. The accessor "getSubtitle" gives direct access to 2327 * the value 2328 */ 2329 public ActivityDefinition setSubtitleElement(StringType value) { 2330 this.subtitle = value; 2331 return this; 2332 } 2333 2334 /** 2335 * @return An explanatory or alternate title for the activity definition giving 2336 * additional information about its content. 2337 */ 2338 public String getSubtitle() { 2339 return this.subtitle == null ? null : this.subtitle.getValue(); 2340 } 2341 2342 /** 2343 * @param value An explanatory or alternate title for the activity definition 2344 * giving additional information about its content. 2345 */ 2346 public ActivityDefinition setSubtitle(String value) { 2347 if (Utilities.noString(value)) 2348 this.subtitle = null; 2349 else { 2350 if (this.subtitle == null) 2351 this.subtitle = new StringType(); 2352 this.subtitle.setValue(value); 2353 } 2354 return this; 2355 } 2356 2357 /** 2358 * @return {@link #status} (The status of this activity definition. Enables 2359 * tracking the life-cycle of the content.). This is the underlying 2360 * object with id, value and extensions. The accessor "getStatus" gives 2361 * direct access to the value 2362 */ 2363 public Enumeration<PublicationStatus> getStatusElement() { 2364 if (this.status == null) 2365 if (Configuration.errorOnAutoCreate()) 2366 throw new Error("Attempt to auto-create ActivityDefinition.status"); 2367 else if (Configuration.doAutoCreate()) 2368 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2369 return this.status; 2370 } 2371 2372 public boolean hasStatusElement() { 2373 return this.status != null && !this.status.isEmpty(); 2374 } 2375 2376 public boolean hasStatus() { 2377 return this.status != null && !this.status.isEmpty(); 2378 } 2379 2380 /** 2381 * @param value {@link #status} (The status of this activity definition. Enables 2382 * tracking the life-cycle of the content.). This is the underlying 2383 * object with id, value and extensions. The accessor "getStatus" 2384 * gives direct access to the value 2385 */ 2386 public ActivityDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2387 this.status = value; 2388 return this; 2389 } 2390 2391 /** 2392 * @return The status of this activity definition. Enables tracking the 2393 * life-cycle of the content. 2394 */ 2395 public PublicationStatus getStatus() { 2396 return this.status == null ? null : this.status.getValue(); 2397 } 2398 2399 /** 2400 * @param value The status of this activity definition. Enables tracking the 2401 * life-cycle of the content. 2402 */ 2403 public ActivityDefinition setStatus(PublicationStatus value) { 2404 if (this.status == null) 2405 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2406 this.status.setValue(value); 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #experimental} (A Boolean value to indicate that this activity 2412 * definition is authored for testing purposes (or 2413 * education/evaluation/marketing) and is not intended to be used for 2414 * genuine usage.). This is the underlying object with id, value and 2415 * extensions. The accessor "getExperimental" gives direct access to the 2416 * value 2417 */ 2418 public BooleanType getExperimentalElement() { 2419 if (this.experimental == null) 2420 if (Configuration.errorOnAutoCreate()) 2421 throw new Error("Attempt to auto-create ActivityDefinition.experimental"); 2422 else if (Configuration.doAutoCreate()) 2423 this.experimental = new BooleanType(); // bb 2424 return this.experimental; 2425 } 2426 2427 public boolean hasExperimentalElement() { 2428 return this.experimental != null && !this.experimental.isEmpty(); 2429 } 2430 2431 public boolean hasExperimental() { 2432 return this.experimental != null && !this.experimental.isEmpty(); 2433 } 2434 2435 /** 2436 * @param value {@link #experimental} (A Boolean value to indicate that this 2437 * activity definition is authored for testing purposes (or 2438 * education/evaluation/marketing) and is not intended to be used 2439 * for genuine usage.). This is the underlying object with id, 2440 * value and extensions. The accessor "getExperimental" gives 2441 * direct access to the value 2442 */ 2443 public ActivityDefinition setExperimentalElement(BooleanType value) { 2444 this.experimental = value; 2445 return this; 2446 } 2447 2448 /** 2449 * @return A Boolean value to indicate that this activity definition is authored 2450 * for testing purposes (or education/evaluation/marketing) and is not 2451 * intended to be used for genuine usage. 2452 */ 2453 public boolean getExperimental() { 2454 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2455 } 2456 2457 /** 2458 * @param value A Boolean value to indicate that this activity definition is 2459 * authored for testing purposes (or 2460 * education/evaluation/marketing) and is not intended to be used 2461 * for genuine usage. 2462 */ 2463 public ActivityDefinition setExperimental(boolean value) { 2464 if (this.experimental == null) 2465 this.experimental = new BooleanType(); 2466 this.experimental.setValue(value); 2467 return this; 2468 } 2469 2470 /** 2471 * @return {@link #subject} (A code or group definition that describes the 2472 * intended subject of the activity being defined.) 2473 */ 2474 public Type getSubject() { 2475 return this.subject; 2476 } 2477 2478 /** 2479 * @return {@link #subject} (A code or group definition that describes the 2480 * intended subject of the activity being defined.) 2481 */ 2482 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2483 if (this.subject == null) 2484 this.subject = new CodeableConcept(); 2485 if (!(this.subject instanceof CodeableConcept)) 2486 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2487 + this.subject.getClass().getName() + " was encountered"); 2488 return (CodeableConcept) this.subject; 2489 } 2490 2491 public boolean hasSubjectCodeableConcept() { 2492 return this != null && this.subject instanceof CodeableConcept; 2493 } 2494 2495 /** 2496 * @return {@link #subject} (A code or group definition that describes the 2497 * intended subject of the activity being defined.) 2498 */ 2499 public Reference getSubjectReference() throws FHIRException { 2500 if (this.subject == null) 2501 this.subject = new Reference(); 2502 if (!(this.subject instanceof Reference)) 2503 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 2504 + " was encountered"); 2505 return (Reference) this.subject; 2506 } 2507 2508 public boolean hasSubjectReference() { 2509 return this != null && this.subject instanceof Reference; 2510 } 2511 2512 public boolean hasSubject() { 2513 return this.subject != null && !this.subject.isEmpty(); 2514 } 2515 2516 /** 2517 * @param value {@link #subject} (A code or group definition that describes the 2518 * intended subject of the activity being defined.) 2519 */ 2520 public ActivityDefinition setSubject(Type value) { 2521 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2522 throw new Error("Not the right type for ActivityDefinition.subject[x]: " + value.fhirType()); 2523 this.subject = value; 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #date} (The date (and optionally time) when the activity 2529 * definition was published. The date must change when the business 2530 * version changes and it must change if the status code changes. In 2531 * addition, it should change when the substantive content of the 2532 * activity definition changes.). This is the underlying object with id, 2533 * value and extensions. The accessor "getDate" gives direct access to 2534 * the value 2535 */ 2536 public DateTimeType getDateElement() { 2537 if (this.date == null) 2538 if (Configuration.errorOnAutoCreate()) 2539 throw new Error("Attempt to auto-create ActivityDefinition.date"); 2540 else if (Configuration.doAutoCreate()) 2541 this.date = new DateTimeType(); // bb 2542 return this.date; 2543 } 2544 2545 public boolean hasDateElement() { 2546 return this.date != null && !this.date.isEmpty(); 2547 } 2548 2549 public boolean hasDate() { 2550 return this.date != null && !this.date.isEmpty(); 2551 } 2552 2553 /** 2554 * @param value {@link #date} (The date (and optionally time) when the activity 2555 * definition was published. The date must change when the business 2556 * version changes and it must change if the status code changes. 2557 * In addition, it should change when the substantive content of 2558 * the activity definition changes.). This is the underlying object 2559 * with id, value and extensions. The accessor "getDate" gives 2560 * direct access to the value 2561 */ 2562 public ActivityDefinition setDateElement(DateTimeType value) { 2563 this.date = value; 2564 return this; 2565 } 2566 2567 /** 2568 * @return The date (and optionally time) when the activity definition was 2569 * published. The date must change when the business version changes and 2570 * it must change if the status code changes. In addition, it should 2571 * change when the substantive content of the activity definition 2572 * changes. 2573 */ 2574 public Date getDate() { 2575 return this.date == null ? null : this.date.getValue(); 2576 } 2577 2578 /** 2579 * @param value The date (and optionally time) when the activity definition was 2580 * published. The date must change when the business version 2581 * changes and it must change if the status code changes. In 2582 * addition, it should change when the substantive content of the 2583 * activity definition changes. 2584 */ 2585 public ActivityDefinition setDate(Date value) { 2586 if (value == null) 2587 this.date = null; 2588 else { 2589 if (this.date == null) 2590 this.date = new DateTimeType(); 2591 this.date.setValue(value); 2592 } 2593 return this; 2594 } 2595 2596 /** 2597 * @return {@link #publisher} (The name of the organization or individual that 2598 * published the activity definition.). This is the underlying object 2599 * with id, value and extensions. The accessor "getPublisher" gives 2600 * direct access to the value 2601 */ 2602 public StringType getPublisherElement() { 2603 if (this.publisher == null) 2604 if (Configuration.errorOnAutoCreate()) 2605 throw new Error("Attempt to auto-create ActivityDefinition.publisher"); 2606 else if (Configuration.doAutoCreate()) 2607 this.publisher = new StringType(); // bb 2608 return this.publisher; 2609 } 2610 2611 public boolean hasPublisherElement() { 2612 return this.publisher != null && !this.publisher.isEmpty(); 2613 } 2614 2615 public boolean hasPublisher() { 2616 return this.publisher != null && !this.publisher.isEmpty(); 2617 } 2618 2619 /** 2620 * @param value {@link #publisher} (The name of the organization or individual 2621 * that published the activity definition.). This is the underlying 2622 * object with id, value and extensions. The accessor 2623 * "getPublisher" gives direct access to the value 2624 */ 2625 public ActivityDefinition setPublisherElement(StringType value) { 2626 this.publisher = value; 2627 return this; 2628 } 2629 2630 /** 2631 * @return The name of the organization or individual that published the 2632 * activity definition. 2633 */ 2634 public String getPublisher() { 2635 return this.publisher == null ? null : this.publisher.getValue(); 2636 } 2637 2638 /** 2639 * @param value The name of the organization or individual that published the 2640 * activity definition. 2641 */ 2642 public ActivityDefinition setPublisher(String value) { 2643 if (Utilities.noString(value)) 2644 this.publisher = null; 2645 else { 2646 if (this.publisher == null) 2647 this.publisher = new StringType(); 2648 this.publisher.setValue(value); 2649 } 2650 return this; 2651 } 2652 2653 /** 2654 * @return {@link #contact} (Contact details to assist a user in finding and 2655 * communicating with the publisher.) 2656 */ 2657 public List<ContactDetail> getContact() { 2658 if (this.contact == null) 2659 this.contact = new ArrayList<ContactDetail>(); 2660 return this.contact; 2661 } 2662 2663 /** 2664 * @return Returns a reference to <code>this</code> for easy method chaining 2665 */ 2666 public ActivityDefinition setContact(List<ContactDetail> theContact) { 2667 this.contact = theContact; 2668 return this; 2669 } 2670 2671 public boolean hasContact() { 2672 if (this.contact == null) 2673 return false; 2674 for (ContactDetail item : this.contact) 2675 if (!item.isEmpty()) 2676 return true; 2677 return false; 2678 } 2679 2680 public ContactDetail addContact() { // 3 2681 ContactDetail t = new ContactDetail(); 2682 if (this.contact == null) 2683 this.contact = new ArrayList<ContactDetail>(); 2684 this.contact.add(t); 2685 return t; 2686 } 2687 2688 public ActivityDefinition addContact(ContactDetail t) { // 3 2689 if (t == null) 2690 return this; 2691 if (this.contact == null) 2692 this.contact = new ArrayList<ContactDetail>(); 2693 this.contact.add(t); 2694 return this; 2695 } 2696 2697 /** 2698 * @return The first repetition of repeating field {@link #contact}, creating it 2699 * if it does not already exist 2700 */ 2701 public ContactDetail getContactFirstRep() { 2702 if (getContact().isEmpty()) { 2703 addContact(); 2704 } 2705 return getContact().get(0); 2706 } 2707 2708 /** 2709 * @return {@link #description} (A free text natural language description of the 2710 * activity definition from a consumer's perspective.). This is the 2711 * underlying object with id, value and extensions. The accessor 2712 * "getDescription" gives direct access to the value 2713 */ 2714 public MarkdownType getDescriptionElement() { 2715 if (this.description == null) 2716 if (Configuration.errorOnAutoCreate()) 2717 throw new Error("Attempt to auto-create ActivityDefinition.description"); 2718 else if (Configuration.doAutoCreate()) 2719 this.description = new MarkdownType(); // bb 2720 return this.description; 2721 } 2722 2723 public boolean hasDescriptionElement() { 2724 return this.description != null && !this.description.isEmpty(); 2725 } 2726 2727 public boolean hasDescription() { 2728 return this.description != null && !this.description.isEmpty(); 2729 } 2730 2731 /** 2732 * @param value {@link #description} (A free text natural language description 2733 * of the activity definition from a consumer's perspective.). This 2734 * is the underlying object with id, value and extensions. The 2735 * accessor "getDescription" gives direct access to the value 2736 */ 2737 public ActivityDefinition setDescriptionElement(MarkdownType value) { 2738 this.description = value; 2739 return this; 2740 } 2741 2742 /** 2743 * @return A free text natural language description of the activity definition 2744 * from a consumer's perspective. 2745 */ 2746 public String getDescription() { 2747 return this.description == null ? null : this.description.getValue(); 2748 } 2749 2750 /** 2751 * @param value A free text natural language description of the activity 2752 * definition from a consumer's perspective. 2753 */ 2754 public ActivityDefinition setDescription(String value) { 2755 if (value == null) 2756 this.description = null; 2757 else { 2758 if (this.description == null) 2759 this.description = new MarkdownType(); 2760 this.description.setValue(value); 2761 } 2762 return this; 2763 } 2764 2765 /** 2766 * @return {@link #useContext} (The content was developed with a focus and 2767 * intent of supporting the contexts that are listed. These contexts may 2768 * be general categories (gender, age, ...) or may be references to 2769 * specific programs (insurance plans, studies, ...) and may be used to 2770 * assist with indexing and searching for appropriate activity 2771 * definition instances.) 2772 */ 2773 public List<UsageContext> getUseContext() { 2774 if (this.useContext == null) 2775 this.useContext = new ArrayList<UsageContext>(); 2776 return this.useContext; 2777 } 2778 2779 /** 2780 * @return Returns a reference to <code>this</code> for easy method chaining 2781 */ 2782 public ActivityDefinition setUseContext(List<UsageContext> theUseContext) { 2783 this.useContext = theUseContext; 2784 return this; 2785 } 2786 2787 public boolean hasUseContext() { 2788 if (this.useContext == null) 2789 return false; 2790 for (UsageContext item : this.useContext) 2791 if (!item.isEmpty()) 2792 return true; 2793 return false; 2794 } 2795 2796 public UsageContext addUseContext() { // 3 2797 UsageContext t = new UsageContext(); 2798 if (this.useContext == null) 2799 this.useContext = new ArrayList<UsageContext>(); 2800 this.useContext.add(t); 2801 return t; 2802 } 2803 2804 public ActivityDefinition addUseContext(UsageContext t) { // 3 2805 if (t == null) 2806 return this; 2807 if (this.useContext == null) 2808 this.useContext = new ArrayList<UsageContext>(); 2809 this.useContext.add(t); 2810 return this; 2811 } 2812 2813 /** 2814 * @return The first repetition of repeating field {@link #useContext}, creating 2815 * it if it does not already exist 2816 */ 2817 public UsageContext getUseContextFirstRep() { 2818 if (getUseContext().isEmpty()) { 2819 addUseContext(); 2820 } 2821 return getUseContext().get(0); 2822 } 2823 2824 /** 2825 * @return {@link #jurisdiction} (A legal or geographic region in which the 2826 * activity definition is intended to be used.) 2827 */ 2828 public List<CodeableConcept> getJurisdiction() { 2829 if (this.jurisdiction == null) 2830 this.jurisdiction = new ArrayList<CodeableConcept>(); 2831 return this.jurisdiction; 2832 } 2833 2834 /** 2835 * @return Returns a reference to <code>this</code> for easy method chaining 2836 */ 2837 public ActivityDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2838 this.jurisdiction = theJurisdiction; 2839 return this; 2840 } 2841 2842 public boolean hasJurisdiction() { 2843 if (this.jurisdiction == null) 2844 return false; 2845 for (CodeableConcept item : this.jurisdiction) 2846 if (!item.isEmpty()) 2847 return true; 2848 return false; 2849 } 2850 2851 public CodeableConcept addJurisdiction() { // 3 2852 CodeableConcept t = new CodeableConcept(); 2853 if (this.jurisdiction == null) 2854 this.jurisdiction = new ArrayList<CodeableConcept>(); 2855 this.jurisdiction.add(t); 2856 return t; 2857 } 2858 2859 public ActivityDefinition addJurisdiction(CodeableConcept t) { // 3 2860 if (t == null) 2861 return this; 2862 if (this.jurisdiction == null) 2863 this.jurisdiction = new ArrayList<CodeableConcept>(); 2864 this.jurisdiction.add(t); 2865 return this; 2866 } 2867 2868 /** 2869 * @return The first repetition of repeating field {@link #jurisdiction}, 2870 * creating it if it does not already exist 2871 */ 2872 public CodeableConcept getJurisdictionFirstRep() { 2873 if (getJurisdiction().isEmpty()) { 2874 addJurisdiction(); 2875 } 2876 return getJurisdiction().get(0); 2877 } 2878 2879 /** 2880 * @return {@link #purpose} (Explanation of why this activity definition is 2881 * needed and why it has been designed as it has.). This is the 2882 * underlying object with id, value and extensions. The accessor 2883 * "getPurpose" gives direct access to the value 2884 */ 2885 public MarkdownType getPurposeElement() { 2886 if (this.purpose == null) 2887 if (Configuration.errorOnAutoCreate()) 2888 throw new Error("Attempt to auto-create ActivityDefinition.purpose"); 2889 else if (Configuration.doAutoCreate()) 2890 this.purpose = new MarkdownType(); // bb 2891 return this.purpose; 2892 } 2893 2894 public boolean hasPurposeElement() { 2895 return this.purpose != null && !this.purpose.isEmpty(); 2896 } 2897 2898 public boolean hasPurpose() { 2899 return this.purpose != null && !this.purpose.isEmpty(); 2900 } 2901 2902 /** 2903 * @param value {@link #purpose} (Explanation of why this activity definition is 2904 * needed and why it has been designed as it has.). This is the 2905 * underlying object with id, value and extensions. The accessor 2906 * "getPurpose" gives direct access to the value 2907 */ 2908 public ActivityDefinition setPurposeElement(MarkdownType value) { 2909 this.purpose = value; 2910 return this; 2911 } 2912 2913 /** 2914 * @return Explanation of why this activity definition is needed and why it has 2915 * been designed as it has. 2916 */ 2917 public String getPurpose() { 2918 return this.purpose == null ? null : this.purpose.getValue(); 2919 } 2920 2921 /** 2922 * @param value Explanation of why this activity definition is needed and why it 2923 * has been designed as it has. 2924 */ 2925 public ActivityDefinition setPurpose(String value) { 2926 if (value == null) 2927 this.purpose = null; 2928 else { 2929 if (this.purpose == null) 2930 this.purpose = new MarkdownType(); 2931 this.purpose.setValue(value); 2932 } 2933 return this; 2934 } 2935 2936 /** 2937 * @return {@link #usage} (A detailed description of how the activity definition 2938 * is used from a clinical perspective.). This is the underlying object 2939 * with id, value and extensions. The accessor "getUsage" gives direct 2940 * access to the value 2941 */ 2942 public StringType getUsageElement() { 2943 if (this.usage == null) 2944 if (Configuration.errorOnAutoCreate()) 2945 throw new Error("Attempt to auto-create ActivityDefinition.usage"); 2946 else if (Configuration.doAutoCreate()) 2947 this.usage = new StringType(); // bb 2948 return this.usage; 2949 } 2950 2951 public boolean hasUsageElement() { 2952 return this.usage != null && !this.usage.isEmpty(); 2953 } 2954 2955 public boolean hasUsage() { 2956 return this.usage != null && !this.usage.isEmpty(); 2957 } 2958 2959 /** 2960 * @param value {@link #usage} (A detailed description of how the activity 2961 * definition is used from a clinical perspective.). This is the 2962 * underlying object with id, value and extensions. The accessor 2963 * "getUsage" gives direct access to the value 2964 */ 2965 public ActivityDefinition setUsageElement(StringType value) { 2966 this.usage = value; 2967 return this; 2968 } 2969 2970 /** 2971 * @return A detailed description of how the activity definition is used from a 2972 * clinical perspective. 2973 */ 2974 public String getUsage() { 2975 return this.usage == null ? null : this.usage.getValue(); 2976 } 2977 2978 /** 2979 * @param value A detailed description of how the activity definition is used 2980 * from a clinical perspective. 2981 */ 2982 public ActivityDefinition setUsage(String value) { 2983 if (Utilities.noString(value)) 2984 this.usage = null; 2985 else { 2986 if (this.usage == null) 2987 this.usage = new StringType(); 2988 this.usage.setValue(value); 2989 } 2990 return this; 2991 } 2992 2993 /** 2994 * @return {@link #copyright} (A copyright statement relating to the activity 2995 * definition and/or its contents. Copyright statements are generally 2996 * legal restrictions on the use and publishing of the activity 2997 * definition.). This is the underlying object with id, value and 2998 * extensions. The accessor "getCopyright" gives direct access to the 2999 * value 3000 */ 3001 public MarkdownType getCopyrightElement() { 3002 if (this.copyright == null) 3003 if (Configuration.errorOnAutoCreate()) 3004 throw new Error("Attempt to auto-create ActivityDefinition.copyright"); 3005 else if (Configuration.doAutoCreate()) 3006 this.copyright = new MarkdownType(); // bb 3007 return this.copyright; 3008 } 3009 3010 public boolean hasCopyrightElement() { 3011 return this.copyright != null && !this.copyright.isEmpty(); 3012 } 3013 3014 public boolean hasCopyright() { 3015 return this.copyright != null && !this.copyright.isEmpty(); 3016 } 3017 3018 /** 3019 * @param value {@link #copyright} (A copyright statement relating to the 3020 * activity definition and/or its contents. Copyright statements 3021 * are generally legal restrictions on the use and publishing of 3022 * the activity definition.). This is the underlying object with 3023 * id, value and extensions. The accessor "getCopyright" gives 3024 * direct access to the value 3025 */ 3026 public ActivityDefinition setCopyrightElement(MarkdownType value) { 3027 this.copyright = value; 3028 return this; 3029 } 3030 3031 /** 3032 * @return A copyright statement relating to the activity definition and/or its 3033 * contents. Copyright statements are generally legal restrictions on 3034 * the use and publishing of the activity definition. 3035 */ 3036 public String getCopyright() { 3037 return this.copyright == null ? null : this.copyright.getValue(); 3038 } 3039 3040 /** 3041 * @param value A copyright statement relating to the activity definition and/or 3042 * its contents. Copyright statements are generally legal 3043 * restrictions on the use and publishing of the activity 3044 * definition. 3045 */ 3046 public ActivityDefinition setCopyright(String value) { 3047 if (value == null) 3048 this.copyright = null; 3049 else { 3050 if (this.copyright == null) 3051 this.copyright = new MarkdownType(); 3052 this.copyright.setValue(value); 3053 } 3054 return this; 3055 } 3056 3057 /** 3058 * @return {@link #approvalDate} (The date on which the resource content was 3059 * approved by the publisher. Approval happens once when the content is 3060 * officially approved for usage.). This is the underlying object with 3061 * id, value and extensions. The accessor "getApprovalDate" gives direct 3062 * access to the value 3063 */ 3064 public DateType getApprovalDateElement() { 3065 if (this.approvalDate == null) 3066 if (Configuration.errorOnAutoCreate()) 3067 throw new Error("Attempt to auto-create ActivityDefinition.approvalDate"); 3068 else if (Configuration.doAutoCreate()) 3069 this.approvalDate = new DateType(); // bb 3070 return this.approvalDate; 3071 } 3072 3073 public boolean hasApprovalDateElement() { 3074 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3075 } 3076 3077 public boolean hasApprovalDate() { 3078 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3079 } 3080 3081 /** 3082 * @param value {@link #approvalDate} (The date on which the resource content 3083 * was approved by the publisher. Approval happens once when the 3084 * content is officially approved for usage.). This is the 3085 * underlying object with id, value and extensions. The accessor 3086 * "getApprovalDate" gives direct access to the value 3087 */ 3088 public ActivityDefinition setApprovalDateElement(DateType value) { 3089 this.approvalDate = value; 3090 return this; 3091 } 3092 3093 /** 3094 * @return The date on which the resource content was approved by the publisher. 3095 * Approval happens once when the content is officially approved for 3096 * usage. 3097 */ 3098 public Date getApprovalDate() { 3099 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3100 } 3101 3102 /** 3103 * @param value The date on which the resource content was approved by the 3104 * publisher. Approval happens once when the content is officially 3105 * approved for usage. 3106 */ 3107 public ActivityDefinition setApprovalDate(Date value) { 3108 if (value == null) 3109 this.approvalDate = null; 3110 else { 3111 if (this.approvalDate == null) 3112 this.approvalDate = new DateType(); 3113 this.approvalDate.setValue(value); 3114 } 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #lastReviewDate} (The date on which the resource content was 3120 * last reviewed. Review happens periodically after approval but does 3121 * not change the original approval date.). This is the underlying 3122 * object with id, value and extensions. The accessor 3123 * "getLastReviewDate" gives direct access to the value 3124 */ 3125 public DateType getLastReviewDateElement() { 3126 if (this.lastReviewDate == null) 3127 if (Configuration.errorOnAutoCreate()) 3128 throw new Error("Attempt to auto-create ActivityDefinition.lastReviewDate"); 3129 else if (Configuration.doAutoCreate()) 3130 this.lastReviewDate = new DateType(); // bb 3131 return this.lastReviewDate; 3132 } 3133 3134 public boolean hasLastReviewDateElement() { 3135 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3136 } 3137 3138 public boolean hasLastReviewDate() { 3139 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3140 } 3141 3142 /** 3143 * @param value {@link #lastReviewDate} (The date on which the resource content 3144 * was last reviewed. Review happens periodically after approval 3145 * but does not change the original approval date.). This is the 3146 * underlying object with id, value and extensions. The accessor 3147 * "getLastReviewDate" gives direct access to the value 3148 */ 3149 public ActivityDefinition setLastReviewDateElement(DateType value) { 3150 this.lastReviewDate = value; 3151 return this; 3152 } 3153 3154 /** 3155 * @return The date on which the resource content was last reviewed. Review 3156 * happens periodically after approval but does not change the original 3157 * approval date. 3158 */ 3159 public Date getLastReviewDate() { 3160 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3161 } 3162 3163 /** 3164 * @param value The date on which the resource content was last reviewed. Review 3165 * happens periodically after approval but does not change the 3166 * original approval date. 3167 */ 3168 public ActivityDefinition setLastReviewDate(Date value) { 3169 if (value == null) 3170 this.lastReviewDate = null; 3171 else { 3172 if (this.lastReviewDate == null) 3173 this.lastReviewDate = new DateType(); 3174 this.lastReviewDate.setValue(value); 3175 } 3176 return this; 3177 } 3178 3179 /** 3180 * @return {@link #effectivePeriod} (The period during which the activity 3181 * definition content was or is planned to be in active use.) 3182 */ 3183 public Period getEffectivePeriod() { 3184 if (this.effectivePeriod == null) 3185 if (Configuration.errorOnAutoCreate()) 3186 throw new Error("Attempt to auto-create ActivityDefinition.effectivePeriod"); 3187 else if (Configuration.doAutoCreate()) 3188 this.effectivePeriod = new Period(); // cc 3189 return this.effectivePeriod; 3190 } 3191 3192 public boolean hasEffectivePeriod() { 3193 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3194 } 3195 3196 /** 3197 * @param value {@link #effectivePeriod} (The period during which the activity 3198 * definition content was or is planned to be in active use.) 3199 */ 3200 public ActivityDefinition setEffectivePeriod(Period value) { 3201 this.effectivePeriod = value; 3202 return this; 3203 } 3204 3205 /** 3206 * @return {@link #topic} (Descriptive topics related to the content of the 3207 * activity. Topics provide a high-level categorization of the activity 3208 * that can be useful for filtering and searching.) 3209 */ 3210 public List<CodeableConcept> getTopic() { 3211 if (this.topic == null) 3212 this.topic = new ArrayList<CodeableConcept>(); 3213 return this.topic; 3214 } 3215 3216 /** 3217 * @return Returns a reference to <code>this</code> for easy method chaining 3218 */ 3219 public ActivityDefinition setTopic(List<CodeableConcept> theTopic) { 3220 this.topic = theTopic; 3221 return this; 3222 } 3223 3224 public boolean hasTopic() { 3225 if (this.topic == null) 3226 return false; 3227 for (CodeableConcept item : this.topic) 3228 if (!item.isEmpty()) 3229 return true; 3230 return false; 3231 } 3232 3233 public CodeableConcept addTopic() { // 3 3234 CodeableConcept t = new CodeableConcept(); 3235 if (this.topic == null) 3236 this.topic = new ArrayList<CodeableConcept>(); 3237 this.topic.add(t); 3238 return t; 3239 } 3240 3241 public ActivityDefinition addTopic(CodeableConcept t) { // 3 3242 if (t == null) 3243 return this; 3244 if (this.topic == null) 3245 this.topic = new ArrayList<CodeableConcept>(); 3246 this.topic.add(t); 3247 return this; 3248 } 3249 3250 /** 3251 * @return The first repetition of repeating field {@link #topic}, creating it 3252 * if it does not already exist 3253 */ 3254 public CodeableConcept getTopicFirstRep() { 3255 if (getTopic().isEmpty()) { 3256 addTopic(); 3257 } 3258 return getTopic().get(0); 3259 } 3260 3261 /** 3262 * @return {@link #author} (An individiual or organization primarily involved in 3263 * the creation and maintenance of the content.) 3264 */ 3265 public List<ContactDetail> getAuthor() { 3266 if (this.author == null) 3267 this.author = new ArrayList<ContactDetail>(); 3268 return this.author; 3269 } 3270 3271 /** 3272 * @return Returns a reference to <code>this</code> for easy method chaining 3273 */ 3274 public ActivityDefinition setAuthor(List<ContactDetail> theAuthor) { 3275 this.author = theAuthor; 3276 return this; 3277 } 3278 3279 public boolean hasAuthor() { 3280 if (this.author == null) 3281 return false; 3282 for (ContactDetail item : this.author) 3283 if (!item.isEmpty()) 3284 return true; 3285 return false; 3286 } 3287 3288 public ContactDetail addAuthor() { // 3 3289 ContactDetail t = new ContactDetail(); 3290 if (this.author == null) 3291 this.author = new ArrayList<ContactDetail>(); 3292 this.author.add(t); 3293 return t; 3294 } 3295 3296 public ActivityDefinition addAuthor(ContactDetail t) { // 3 3297 if (t == null) 3298 return this; 3299 if (this.author == null) 3300 this.author = new ArrayList<ContactDetail>(); 3301 this.author.add(t); 3302 return this; 3303 } 3304 3305 /** 3306 * @return The first repetition of repeating field {@link #author}, creating it 3307 * if it does not already exist 3308 */ 3309 public ContactDetail getAuthorFirstRep() { 3310 if (getAuthor().isEmpty()) { 3311 addAuthor(); 3312 } 3313 return getAuthor().get(0); 3314 } 3315 3316 /** 3317 * @return {@link #editor} (An individual or organization primarily responsible 3318 * for internal coherence of the content.) 3319 */ 3320 public List<ContactDetail> getEditor() { 3321 if (this.editor == null) 3322 this.editor = new ArrayList<ContactDetail>(); 3323 return this.editor; 3324 } 3325 3326 /** 3327 * @return Returns a reference to <code>this</code> for easy method chaining 3328 */ 3329 public ActivityDefinition setEditor(List<ContactDetail> theEditor) { 3330 this.editor = theEditor; 3331 return this; 3332 } 3333 3334 public boolean hasEditor() { 3335 if (this.editor == null) 3336 return false; 3337 for (ContactDetail item : this.editor) 3338 if (!item.isEmpty()) 3339 return true; 3340 return false; 3341 } 3342 3343 public ContactDetail addEditor() { // 3 3344 ContactDetail t = new ContactDetail(); 3345 if (this.editor == null) 3346 this.editor = new ArrayList<ContactDetail>(); 3347 this.editor.add(t); 3348 return t; 3349 } 3350 3351 public ActivityDefinition addEditor(ContactDetail t) { // 3 3352 if (t == null) 3353 return this; 3354 if (this.editor == null) 3355 this.editor = new ArrayList<ContactDetail>(); 3356 this.editor.add(t); 3357 return this; 3358 } 3359 3360 /** 3361 * @return The first repetition of repeating field {@link #editor}, creating it 3362 * if it does not already exist 3363 */ 3364 public ContactDetail getEditorFirstRep() { 3365 if (getEditor().isEmpty()) { 3366 addEditor(); 3367 } 3368 return getEditor().get(0); 3369 } 3370 3371 /** 3372 * @return {@link #reviewer} (An individual or organization primarily 3373 * responsible for review of some aspect of the content.) 3374 */ 3375 public List<ContactDetail> getReviewer() { 3376 if (this.reviewer == null) 3377 this.reviewer = new ArrayList<ContactDetail>(); 3378 return this.reviewer; 3379 } 3380 3381 /** 3382 * @return Returns a reference to <code>this</code> for easy method chaining 3383 */ 3384 public ActivityDefinition setReviewer(List<ContactDetail> theReviewer) { 3385 this.reviewer = theReviewer; 3386 return this; 3387 } 3388 3389 public boolean hasReviewer() { 3390 if (this.reviewer == null) 3391 return false; 3392 for (ContactDetail item : this.reviewer) 3393 if (!item.isEmpty()) 3394 return true; 3395 return false; 3396 } 3397 3398 public ContactDetail addReviewer() { // 3 3399 ContactDetail t = new ContactDetail(); 3400 if (this.reviewer == null) 3401 this.reviewer = new ArrayList<ContactDetail>(); 3402 this.reviewer.add(t); 3403 return t; 3404 } 3405 3406 public ActivityDefinition addReviewer(ContactDetail t) { // 3 3407 if (t == null) 3408 return this; 3409 if (this.reviewer == null) 3410 this.reviewer = new ArrayList<ContactDetail>(); 3411 this.reviewer.add(t); 3412 return this; 3413 } 3414 3415 /** 3416 * @return The first repetition of repeating field {@link #reviewer}, creating 3417 * it if it does not already exist 3418 */ 3419 public ContactDetail getReviewerFirstRep() { 3420 if (getReviewer().isEmpty()) { 3421 addReviewer(); 3422 } 3423 return getReviewer().get(0); 3424 } 3425 3426 /** 3427 * @return {@link #endorser} (An individual or organization responsible for 3428 * officially endorsing the content for use in some setting.) 3429 */ 3430 public List<ContactDetail> getEndorser() { 3431 if (this.endorser == null) 3432 this.endorser = new ArrayList<ContactDetail>(); 3433 return this.endorser; 3434 } 3435 3436 /** 3437 * @return Returns a reference to <code>this</code> for easy method chaining 3438 */ 3439 public ActivityDefinition setEndorser(List<ContactDetail> theEndorser) { 3440 this.endorser = theEndorser; 3441 return this; 3442 } 3443 3444 public boolean hasEndorser() { 3445 if (this.endorser == null) 3446 return false; 3447 for (ContactDetail item : this.endorser) 3448 if (!item.isEmpty()) 3449 return true; 3450 return false; 3451 } 3452 3453 public ContactDetail addEndorser() { // 3 3454 ContactDetail t = new ContactDetail(); 3455 if (this.endorser == null) 3456 this.endorser = new ArrayList<ContactDetail>(); 3457 this.endorser.add(t); 3458 return t; 3459 } 3460 3461 public ActivityDefinition addEndorser(ContactDetail t) { // 3 3462 if (t == null) 3463 return this; 3464 if (this.endorser == null) 3465 this.endorser = new ArrayList<ContactDetail>(); 3466 this.endorser.add(t); 3467 return this; 3468 } 3469 3470 /** 3471 * @return The first repetition of repeating field {@link #endorser}, creating 3472 * it if it does not already exist 3473 */ 3474 public ContactDetail getEndorserFirstRep() { 3475 if (getEndorser().isEmpty()) { 3476 addEndorser(); 3477 } 3478 return getEndorser().get(0); 3479 } 3480 3481 /** 3482 * @return {@link #relatedArtifact} (Related artifacts such as additional 3483 * documentation, justification, or bibliographic references.) 3484 */ 3485 public List<RelatedArtifact> getRelatedArtifact() { 3486 if (this.relatedArtifact == null) 3487 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3488 return this.relatedArtifact; 3489 } 3490 3491 /** 3492 * @return Returns a reference to <code>this</code> for easy method chaining 3493 */ 3494 public ActivityDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3495 this.relatedArtifact = theRelatedArtifact; 3496 return this; 3497 } 3498 3499 public boolean hasRelatedArtifact() { 3500 if (this.relatedArtifact == null) 3501 return false; 3502 for (RelatedArtifact item : this.relatedArtifact) 3503 if (!item.isEmpty()) 3504 return true; 3505 return false; 3506 } 3507 3508 public RelatedArtifact addRelatedArtifact() { // 3 3509 RelatedArtifact t = new RelatedArtifact(); 3510 if (this.relatedArtifact == null) 3511 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3512 this.relatedArtifact.add(t); 3513 return t; 3514 } 3515 3516 public ActivityDefinition addRelatedArtifact(RelatedArtifact t) { // 3 3517 if (t == null) 3518 return this; 3519 if (this.relatedArtifact == null) 3520 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3521 this.relatedArtifact.add(t); 3522 return this; 3523 } 3524 3525 /** 3526 * @return The first repetition of repeating field {@link #relatedArtifact}, 3527 * creating it if it does not already exist 3528 */ 3529 public RelatedArtifact getRelatedArtifactFirstRep() { 3530 if (getRelatedArtifact().isEmpty()) { 3531 addRelatedArtifact(); 3532 } 3533 return getRelatedArtifact().get(0); 3534 } 3535 3536 /** 3537 * @return {@link #library} (A reference to a Library resource containing any 3538 * formal logic used by the activity definition.) 3539 */ 3540 public List<CanonicalType> getLibrary() { 3541 if (this.library == null) 3542 this.library = new ArrayList<CanonicalType>(); 3543 return this.library; 3544 } 3545 3546 /** 3547 * @return Returns a reference to <code>this</code> for easy method chaining 3548 */ 3549 public ActivityDefinition setLibrary(List<CanonicalType> theLibrary) { 3550 this.library = theLibrary; 3551 return this; 3552 } 3553 3554 public boolean hasLibrary() { 3555 if (this.library == null) 3556 return false; 3557 for (CanonicalType item : this.library) 3558 if (!item.isEmpty()) 3559 return true; 3560 return false; 3561 } 3562 3563 /** 3564 * @return {@link #library} (A reference to a Library resource containing any 3565 * formal logic used by the activity definition.) 3566 */ 3567 public CanonicalType addLibraryElement() {// 2 3568 CanonicalType t = new CanonicalType(); 3569 if (this.library == null) 3570 this.library = new ArrayList<CanonicalType>(); 3571 this.library.add(t); 3572 return t; 3573 } 3574 3575 /** 3576 * @param value {@link #library} (A reference to a Library resource containing 3577 * any formal logic used by the activity definition.) 3578 */ 3579 public ActivityDefinition addLibrary(String value) { // 1 3580 CanonicalType t = new CanonicalType(); 3581 t.setValue(value); 3582 if (this.library == null) 3583 this.library = new ArrayList<CanonicalType>(); 3584 this.library.add(t); 3585 return this; 3586 } 3587 3588 /** 3589 * @param value {@link #library} (A reference to a Library resource containing 3590 * any formal logic used by the activity definition.) 3591 */ 3592 public boolean hasLibrary(String value) { 3593 if (this.library == null) 3594 return false; 3595 for (CanonicalType v : this.library) 3596 if (v.getValue().equals(value)) // canonical(Library) 3597 return true; 3598 return false; 3599 } 3600 3601 /** 3602 * @return {@link #kind} (A description of the kind of resource the activity 3603 * definition is representing. For example, a MedicationRequest, a 3604 * ServiceRequest, or a CommunicationRequest. Typically, but not always, 3605 * this is a Request resource.). This is the underlying object with id, 3606 * value and extensions. The accessor "getKind" gives direct access to 3607 * the value 3608 */ 3609 public Enumeration<ActivityDefinitionKind> getKindElement() { 3610 if (this.kind == null) 3611 if (Configuration.errorOnAutoCreate()) 3612 throw new Error("Attempt to auto-create ActivityDefinition.kind"); 3613 else if (Configuration.doAutoCreate()) 3614 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); // bb 3615 return this.kind; 3616 } 3617 3618 public boolean hasKindElement() { 3619 return this.kind != null && !this.kind.isEmpty(); 3620 } 3621 3622 public boolean hasKind() { 3623 return this.kind != null && !this.kind.isEmpty(); 3624 } 3625 3626 /** 3627 * @param value {@link #kind} (A description of the kind of resource the 3628 * activity definition is representing. For example, a 3629 * MedicationRequest, a ServiceRequest, or a CommunicationRequest. 3630 * Typically, but not always, this is a Request resource.). This is 3631 * the underlying object with id, value and extensions. The 3632 * accessor "getKind" gives direct access to the value 3633 */ 3634 public ActivityDefinition setKindElement(Enumeration<ActivityDefinitionKind> value) { 3635 this.kind = value; 3636 return this; 3637 } 3638 3639 /** 3640 * @return A description of the kind of resource the activity definition is 3641 * representing. For example, a MedicationRequest, a ServiceRequest, or 3642 * a CommunicationRequest. Typically, but not always, this is a Request 3643 * resource. 3644 */ 3645 public ActivityDefinitionKind getKind() { 3646 return this.kind == null ? null : this.kind.getValue(); 3647 } 3648 3649 /** 3650 * @param value A description of the kind of resource the activity definition is 3651 * representing. For example, a MedicationRequest, a 3652 * ServiceRequest, or a CommunicationRequest. Typically, but not 3653 * always, this is a Request resource. 3654 */ 3655 public ActivityDefinition setKind(ActivityDefinitionKind value) { 3656 if (value == null) 3657 this.kind = null; 3658 else { 3659 if (this.kind == null) 3660 this.kind = new Enumeration<ActivityDefinitionKind>(new ActivityDefinitionKindEnumFactory()); 3661 this.kind.setValue(value); 3662 } 3663 return this; 3664 } 3665 3666 /** 3667 * @return {@link #profile} (A profile to which the target of the activity 3668 * definition is expected to conform.). This is the underlying object 3669 * with id, value and extensions. The accessor "getProfile" gives direct 3670 * access to the value 3671 */ 3672 public CanonicalType getProfileElement() { 3673 if (this.profile == null) 3674 if (Configuration.errorOnAutoCreate()) 3675 throw new Error("Attempt to auto-create ActivityDefinition.profile"); 3676 else if (Configuration.doAutoCreate()) 3677 this.profile = new CanonicalType(); // bb 3678 return this.profile; 3679 } 3680 3681 public boolean hasProfileElement() { 3682 return this.profile != null && !this.profile.isEmpty(); 3683 } 3684 3685 public boolean hasProfile() { 3686 return this.profile != null && !this.profile.isEmpty(); 3687 } 3688 3689 /** 3690 * @param value {@link #profile} (A profile to which the target of the activity 3691 * definition is expected to conform.). This is the underlying 3692 * object with id, value and extensions. The accessor "getProfile" 3693 * gives direct access to the value 3694 */ 3695 public ActivityDefinition setProfileElement(CanonicalType value) { 3696 this.profile = value; 3697 return this; 3698 } 3699 3700 /** 3701 * @return A profile to which the target of the activity definition is expected 3702 * to conform. 3703 */ 3704 public String getProfile() { 3705 return this.profile == null ? null : this.profile.getValue(); 3706 } 3707 3708 /** 3709 * @param value A profile to which the target of the activity definition is 3710 * expected to conform. 3711 */ 3712 public ActivityDefinition setProfile(String value) { 3713 if (Utilities.noString(value)) 3714 this.profile = null; 3715 else { 3716 if (this.profile == null) 3717 this.profile = new CanonicalType(); 3718 this.profile.setValue(value); 3719 } 3720 return this; 3721 } 3722 3723 /** 3724 * @return {@link #code} (Detailed description of the type of activity; e.g. 3725 * What lab test, what procedure, what kind of encounter.) 3726 */ 3727 public CodeableConcept getCode() { 3728 if (this.code == null) 3729 if (Configuration.errorOnAutoCreate()) 3730 throw new Error("Attempt to auto-create ActivityDefinition.code"); 3731 else if (Configuration.doAutoCreate()) 3732 this.code = new CodeableConcept(); // cc 3733 return this.code; 3734 } 3735 3736 public boolean hasCode() { 3737 return this.code != null && !this.code.isEmpty(); 3738 } 3739 3740 /** 3741 * @param value {@link #code} (Detailed description of the type of activity; 3742 * e.g. What lab test, what procedure, what kind of encounter.) 3743 */ 3744 public ActivityDefinition setCode(CodeableConcept value) { 3745 this.code = value; 3746 return this; 3747 } 3748 3749 /** 3750 * @return {@link #intent} (Indicates the level of authority/intentionality 3751 * associated with the activity and where the request should fit into 3752 * the workflow chain.). This is the underlying object with id, value 3753 * and extensions. The accessor "getIntent" gives direct access to the 3754 * value 3755 */ 3756 public Enumeration<RequestIntent> getIntentElement() { 3757 if (this.intent == null) 3758 if (Configuration.errorOnAutoCreate()) 3759 throw new Error("Attempt to auto-create ActivityDefinition.intent"); 3760 else if (Configuration.doAutoCreate()) 3761 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 3762 return this.intent; 3763 } 3764 3765 public boolean hasIntentElement() { 3766 return this.intent != null && !this.intent.isEmpty(); 3767 } 3768 3769 public boolean hasIntent() { 3770 return this.intent != null && !this.intent.isEmpty(); 3771 } 3772 3773 /** 3774 * @param value {@link #intent} (Indicates the level of authority/intentionality 3775 * associated with the activity and where the request should fit 3776 * into the workflow chain.). This is the underlying object with 3777 * id, value and extensions. The accessor "getIntent" gives direct 3778 * access to the value 3779 */ 3780 public ActivityDefinition setIntentElement(Enumeration<RequestIntent> value) { 3781 this.intent = value; 3782 return this; 3783 } 3784 3785 /** 3786 * @return Indicates the level of authority/intentionality associated with the 3787 * activity and where the request should fit into the workflow chain. 3788 */ 3789 public RequestIntent getIntent() { 3790 return this.intent == null ? null : this.intent.getValue(); 3791 } 3792 3793 /** 3794 * @param value Indicates the level of authority/intentionality associated with 3795 * the activity and where the request should fit into the workflow 3796 * chain. 3797 */ 3798 public ActivityDefinition setIntent(RequestIntent value) { 3799 if (value == null) 3800 this.intent = null; 3801 else { 3802 if (this.intent == null) 3803 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 3804 this.intent.setValue(value); 3805 } 3806 return this; 3807 } 3808 3809 /** 3810 * @return {@link #priority} (Indicates how quickly the activity should be 3811 * addressed with respect to other requests.). This is the underlying 3812 * object with id, value and extensions. The accessor "getPriority" 3813 * gives direct access to the value 3814 */ 3815 public Enumeration<RequestPriority> getPriorityElement() { 3816 if (this.priority == null) 3817 if (Configuration.errorOnAutoCreate()) 3818 throw new Error("Attempt to auto-create ActivityDefinition.priority"); 3819 else if (Configuration.doAutoCreate()) 3820 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3821 return this.priority; 3822 } 3823 3824 public boolean hasPriorityElement() { 3825 return this.priority != null && !this.priority.isEmpty(); 3826 } 3827 3828 public boolean hasPriority() { 3829 return this.priority != null && !this.priority.isEmpty(); 3830 } 3831 3832 /** 3833 * @param value {@link #priority} (Indicates how quickly the activity should be 3834 * addressed with respect to other requests.). This is the 3835 * underlying object with id, value and extensions. The accessor 3836 * "getPriority" gives direct access to the value 3837 */ 3838 public ActivityDefinition setPriorityElement(Enumeration<RequestPriority> value) { 3839 this.priority = value; 3840 return this; 3841 } 3842 3843 /** 3844 * @return Indicates how quickly the activity should be addressed with respect 3845 * to other requests. 3846 */ 3847 public RequestPriority getPriority() { 3848 return this.priority == null ? null : this.priority.getValue(); 3849 } 3850 3851 /** 3852 * @param value Indicates how quickly the activity should be addressed with 3853 * respect to other requests. 3854 */ 3855 public ActivityDefinition setPriority(RequestPriority value) { 3856 if (value == null) 3857 this.priority = null; 3858 else { 3859 if (this.priority == null) 3860 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 3861 this.priority.setValue(value); 3862 } 3863 return this; 3864 } 3865 3866 /** 3867 * @return {@link #doNotPerform} (Set this to true if the definition is to 3868 * indicate that a particular activity should NOT be performed. If true, 3869 * this element should be interpreted to reinforce a negative coding. 3870 * For example NPO as a code with a doNotPerform of true would still 3871 * indicate to NOT perform the action.). This is the underlying object 3872 * with id, value and extensions. The accessor "getDoNotPerform" gives 3873 * direct access to the value 3874 */ 3875 public BooleanType getDoNotPerformElement() { 3876 if (this.doNotPerform == null) 3877 if (Configuration.errorOnAutoCreate()) 3878 throw new Error("Attempt to auto-create ActivityDefinition.doNotPerform"); 3879 else if (Configuration.doAutoCreate()) 3880 this.doNotPerform = new BooleanType(); // bb 3881 return this.doNotPerform; 3882 } 3883 3884 public boolean hasDoNotPerformElement() { 3885 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3886 } 3887 3888 public boolean hasDoNotPerform() { 3889 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 3890 } 3891 3892 /** 3893 * @param value {@link #doNotPerform} (Set this to true if the definition is to 3894 * indicate that a particular activity should NOT be performed. If 3895 * true, this element should be interpreted to reinforce a negative 3896 * coding. For example NPO as a code with a doNotPerform of true 3897 * would still indicate to NOT perform the action.). This is the 3898 * underlying object with id, value and extensions. The accessor 3899 * "getDoNotPerform" gives direct access to the value 3900 */ 3901 public ActivityDefinition setDoNotPerformElement(BooleanType value) { 3902 this.doNotPerform = value; 3903 return this; 3904 } 3905 3906 /** 3907 * @return Set this to true if the definition is to indicate that a particular 3908 * activity should NOT be performed. If true, this element should be 3909 * interpreted to reinforce a negative coding. For example NPO as a code 3910 * with a doNotPerform of true would still indicate to NOT perform the 3911 * action. 3912 */ 3913 public boolean getDoNotPerform() { 3914 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 3915 } 3916 3917 /** 3918 * @param value Set this to true if the definition is to indicate that a 3919 * particular activity should NOT be performed. If true, this 3920 * element should be interpreted to reinforce a negative coding. 3921 * For example NPO as a code with a doNotPerform of true would 3922 * still indicate to NOT perform the action. 3923 */ 3924 public ActivityDefinition setDoNotPerform(boolean value) { 3925 if (this.doNotPerform == null) 3926 this.doNotPerform = new BooleanType(); 3927 this.doNotPerform.setValue(value); 3928 return this; 3929 } 3930 3931 /** 3932 * @return {@link #timing} (The period, timing or frequency upon which the 3933 * described activity is to occur.) 3934 */ 3935 public Type getTiming() { 3936 return this.timing; 3937 } 3938 3939 /** 3940 * @return {@link #timing} (The period, timing or frequency upon which the 3941 * described activity is to occur.) 3942 */ 3943 public Timing getTimingTiming() throws FHIRException { 3944 if (this.timing == null) 3945 this.timing = new Timing(); 3946 if (!(this.timing instanceof Timing)) 3947 throw new FHIRException( 3948 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 3949 return (Timing) this.timing; 3950 } 3951 3952 public boolean hasTimingTiming() { 3953 return this != null && this.timing instanceof Timing; 3954 } 3955 3956 /** 3957 * @return {@link #timing} (The period, timing or frequency upon which the 3958 * described activity is to occur.) 3959 */ 3960 public DateTimeType getTimingDateTimeType() throws FHIRException { 3961 if (this.timing == null) 3962 this.timing = new DateTimeType(); 3963 if (!(this.timing instanceof DateTimeType)) 3964 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3965 + this.timing.getClass().getName() + " was encountered"); 3966 return (DateTimeType) this.timing; 3967 } 3968 3969 public boolean hasTimingDateTimeType() { 3970 return this != null && this.timing instanceof DateTimeType; 3971 } 3972 3973 /** 3974 * @return {@link #timing} (The period, timing or frequency upon which the 3975 * described activity is to occur.) 3976 */ 3977 public Age getTimingAge() throws FHIRException { 3978 if (this.timing == null) 3979 this.timing = new Age(); 3980 if (!(this.timing instanceof Age)) 3981 throw new FHIRException( 3982 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 3983 return (Age) this.timing; 3984 } 3985 3986 public boolean hasTimingAge() { 3987 return this != null && this.timing instanceof Age; 3988 } 3989 3990 /** 3991 * @return {@link #timing} (The period, timing or frequency upon which the 3992 * described activity is to occur.) 3993 */ 3994 public Period getTimingPeriod() throws FHIRException { 3995 if (this.timing == null) 3996 this.timing = new Period(); 3997 if (!(this.timing instanceof Period)) 3998 throw new FHIRException( 3999 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 4000 return (Period) this.timing; 4001 } 4002 4003 public boolean hasTimingPeriod() { 4004 return this != null && this.timing instanceof Period; 4005 } 4006 4007 /** 4008 * @return {@link #timing} (The period, timing or frequency upon which the 4009 * described activity is to occur.) 4010 */ 4011 public Range getTimingRange() throws FHIRException { 4012 if (this.timing == null) 4013 this.timing = new Range(); 4014 if (!(this.timing instanceof Range)) 4015 throw new FHIRException( 4016 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 4017 return (Range) this.timing; 4018 } 4019 4020 public boolean hasTimingRange() { 4021 return this != null && this.timing instanceof Range; 4022 } 4023 4024 /** 4025 * @return {@link #timing} (The period, timing or frequency upon which the 4026 * described activity is to occur.) 4027 */ 4028 public Duration getTimingDuration() throws FHIRException { 4029 if (this.timing == null) 4030 this.timing = new Duration(); 4031 if (!(this.timing instanceof Duration)) 4032 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 4033 + " was encountered"); 4034 return (Duration) this.timing; 4035 } 4036 4037 public boolean hasTimingDuration() { 4038 return this != null && this.timing instanceof Duration; 4039 } 4040 4041 public boolean hasTiming() { 4042 return this.timing != null && !this.timing.isEmpty(); 4043 } 4044 4045 /** 4046 * @param value {@link #timing} (The period, timing or frequency upon which the 4047 * described activity is to occur.) 4048 */ 4049 public ActivityDefinition setTiming(Type value) { 4050 if (value != null && !(value instanceof Timing || value instanceof DateTimeType || value instanceof Age 4051 || value instanceof Period || value instanceof Range || value instanceof Duration)) 4052 throw new Error("Not the right type for ActivityDefinition.timing[x]: " + value.fhirType()); 4053 this.timing = value; 4054 return this; 4055 } 4056 4057 /** 4058 * @return {@link #location} (Identifies the facility where the activity will 4059 * occur; e.g. home, hospital, specific clinic, etc.) 4060 */ 4061 public Reference getLocation() { 4062 if (this.location == null) 4063 if (Configuration.errorOnAutoCreate()) 4064 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4065 else if (Configuration.doAutoCreate()) 4066 this.location = new Reference(); // cc 4067 return this.location; 4068 } 4069 4070 public boolean hasLocation() { 4071 return this.location != null && !this.location.isEmpty(); 4072 } 4073 4074 /** 4075 * @param value {@link #location} (Identifies the facility where the activity 4076 * will occur; e.g. home, hospital, specific clinic, etc.) 4077 */ 4078 public ActivityDefinition setLocation(Reference value) { 4079 this.location = value; 4080 return this; 4081 } 4082 4083 /** 4084 * @return {@link #location} The actual object that is the target of the 4085 * reference. The reference library doesn't populate this, but you can 4086 * use it to hold the resource if you resolve it. (Identifies the 4087 * facility where the activity will occur; e.g. home, hospital, specific 4088 * clinic, etc.) 4089 */ 4090 public Location getLocationTarget() { 4091 if (this.locationTarget == null) 4092 if (Configuration.errorOnAutoCreate()) 4093 throw new Error("Attempt to auto-create ActivityDefinition.location"); 4094 else if (Configuration.doAutoCreate()) 4095 this.locationTarget = new Location(); // aa 4096 return this.locationTarget; 4097 } 4098 4099 /** 4100 * @param value {@link #location} The actual object that is the target of the 4101 * reference. The reference library doesn't use these, but you can 4102 * use it to hold the resource if you resolve it. (Identifies the 4103 * facility where the activity will occur; e.g. home, hospital, 4104 * specific clinic, etc.) 4105 */ 4106 public ActivityDefinition setLocationTarget(Location value) { 4107 this.locationTarget = value; 4108 return this; 4109 } 4110 4111 /** 4112 * @return {@link #participant} (Indicates who should participate in performing 4113 * the action described.) 4114 */ 4115 public List<ActivityDefinitionParticipantComponent> getParticipant() { 4116 if (this.participant == null) 4117 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4118 return this.participant; 4119 } 4120 4121 /** 4122 * @return Returns a reference to <code>this</code> for easy method chaining 4123 */ 4124 public ActivityDefinition setParticipant(List<ActivityDefinitionParticipantComponent> theParticipant) { 4125 this.participant = theParticipant; 4126 return this; 4127 } 4128 4129 public boolean hasParticipant() { 4130 if (this.participant == null) 4131 return false; 4132 for (ActivityDefinitionParticipantComponent item : this.participant) 4133 if (!item.isEmpty()) 4134 return true; 4135 return false; 4136 } 4137 4138 public ActivityDefinitionParticipantComponent addParticipant() { // 3 4139 ActivityDefinitionParticipantComponent t = new ActivityDefinitionParticipantComponent(); 4140 if (this.participant == null) 4141 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4142 this.participant.add(t); 4143 return t; 4144 } 4145 4146 public ActivityDefinition addParticipant(ActivityDefinitionParticipantComponent t) { // 3 4147 if (t == null) 4148 return this; 4149 if (this.participant == null) 4150 this.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 4151 this.participant.add(t); 4152 return this; 4153 } 4154 4155 /** 4156 * @return The first repetition of repeating field {@link #participant}, 4157 * creating it if it does not already exist 4158 */ 4159 public ActivityDefinitionParticipantComponent getParticipantFirstRep() { 4160 if (getParticipant().isEmpty()) { 4161 addParticipant(); 4162 } 4163 return getParticipant().get(0); 4164 } 4165 4166 /** 4167 * @return {@link #product} (Identifies the food, drug or other product being 4168 * consumed or supplied in the activity.) 4169 */ 4170 public Type getProduct() { 4171 return this.product; 4172 } 4173 4174 /** 4175 * @return {@link #product} (Identifies the food, drug or other product being 4176 * consumed or supplied in the activity.) 4177 */ 4178 public Reference getProductReference() throws FHIRException { 4179 if (this.product == null) 4180 this.product = new Reference(); 4181 if (!(this.product instanceof Reference)) 4182 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.product.getClass().getName() 4183 + " was encountered"); 4184 return (Reference) this.product; 4185 } 4186 4187 public boolean hasProductReference() { 4188 return this != null && this.product instanceof Reference; 4189 } 4190 4191 /** 4192 * @return {@link #product} (Identifies the food, drug or other product being 4193 * consumed or supplied in the activity.) 4194 */ 4195 public CodeableConcept getProductCodeableConcept() throws FHIRException { 4196 if (this.product == null) 4197 this.product = new CodeableConcept(); 4198 if (!(this.product instanceof CodeableConcept)) 4199 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 4200 + this.product.getClass().getName() + " was encountered"); 4201 return (CodeableConcept) this.product; 4202 } 4203 4204 public boolean hasProductCodeableConcept() { 4205 return this != null && this.product instanceof CodeableConcept; 4206 } 4207 4208 public boolean hasProduct() { 4209 return this.product != null && !this.product.isEmpty(); 4210 } 4211 4212 /** 4213 * @param value {@link #product} (Identifies the food, drug or other product 4214 * being consumed or supplied in the activity.) 4215 */ 4216 public ActivityDefinition setProduct(Type value) { 4217 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 4218 throw new Error("Not the right type for ActivityDefinition.product[x]: " + value.fhirType()); 4219 this.product = value; 4220 return this; 4221 } 4222 4223 /** 4224 * @return {@link #quantity} (Identifies the quantity expected to be consumed at 4225 * once (per dose, per meal, etc.).) 4226 */ 4227 public Quantity getQuantity() { 4228 if (this.quantity == null) 4229 if (Configuration.errorOnAutoCreate()) 4230 throw new Error("Attempt to auto-create ActivityDefinition.quantity"); 4231 else if (Configuration.doAutoCreate()) 4232 this.quantity = new Quantity(); // cc 4233 return this.quantity; 4234 } 4235 4236 public boolean hasQuantity() { 4237 return this.quantity != null && !this.quantity.isEmpty(); 4238 } 4239 4240 /** 4241 * @param value {@link #quantity} (Identifies the quantity expected to be 4242 * consumed at once (per dose, per meal, etc.).) 4243 */ 4244 public ActivityDefinition setQuantity(Quantity value) { 4245 this.quantity = value; 4246 return this; 4247 } 4248 4249 /** 4250 * @return {@link #dosage} (Provides detailed dosage instructions in the same 4251 * way that they are described for MedicationRequest resources.) 4252 */ 4253 public List<Dosage> getDosage() { 4254 if (this.dosage == null) 4255 this.dosage = new ArrayList<Dosage>(); 4256 return this.dosage; 4257 } 4258 4259 /** 4260 * @return Returns a reference to <code>this</code> for easy method chaining 4261 */ 4262 public ActivityDefinition setDosage(List<Dosage> theDosage) { 4263 this.dosage = theDosage; 4264 return this; 4265 } 4266 4267 public boolean hasDosage() { 4268 if (this.dosage == null) 4269 return false; 4270 for (Dosage item : this.dosage) 4271 if (!item.isEmpty()) 4272 return true; 4273 return false; 4274 } 4275 4276 public Dosage addDosage() { // 3 4277 Dosage t = new Dosage(); 4278 if (this.dosage == null) 4279 this.dosage = new ArrayList<Dosage>(); 4280 this.dosage.add(t); 4281 return t; 4282 } 4283 4284 public ActivityDefinition addDosage(Dosage t) { // 3 4285 if (t == null) 4286 return this; 4287 if (this.dosage == null) 4288 this.dosage = new ArrayList<Dosage>(); 4289 this.dosage.add(t); 4290 return this; 4291 } 4292 4293 /** 4294 * @return The first repetition of repeating field {@link #dosage}, creating it 4295 * if it does not already exist 4296 */ 4297 public Dosage getDosageFirstRep() { 4298 if (getDosage().isEmpty()) { 4299 addDosage(); 4300 } 4301 return getDosage().get(0); 4302 } 4303 4304 /** 4305 * @return {@link #bodySite} (Indicates the sites on the subject's body where 4306 * the procedure should be performed (I.e. the target sites).) 4307 */ 4308 public List<CodeableConcept> getBodySite() { 4309 if (this.bodySite == null) 4310 this.bodySite = new ArrayList<CodeableConcept>(); 4311 return this.bodySite; 4312 } 4313 4314 /** 4315 * @return Returns a reference to <code>this</code> for easy method chaining 4316 */ 4317 public ActivityDefinition setBodySite(List<CodeableConcept> theBodySite) { 4318 this.bodySite = theBodySite; 4319 return this; 4320 } 4321 4322 public boolean hasBodySite() { 4323 if (this.bodySite == null) 4324 return false; 4325 for (CodeableConcept item : this.bodySite) 4326 if (!item.isEmpty()) 4327 return true; 4328 return false; 4329 } 4330 4331 public CodeableConcept addBodySite() { // 3 4332 CodeableConcept t = new CodeableConcept(); 4333 if (this.bodySite == null) 4334 this.bodySite = new ArrayList<CodeableConcept>(); 4335 this.bodySite.add(t); 4336 return t; 4337 } 4338 4339 public ActivityDefinition addBodySite(CodeableConcept t) { // 3 4340 if (t == null) 4341 return this; 4342 if (this.bodySite == null) 4343 this.bodySite = new ArrayList<CodeableConcept>(); 4344 this.bodySite.add(t); 4345 return this; 4346 } 4347 4348 /** 4349 * @return The first repetition of repeating field {@link #bodySite}, creating 4350 * it if it does not already exist 4351 */ 4352 public CodeableConcept getBodySiteFirstRep() { 4353 if (getBodySite().isEmpty()) { 4354 addBodySite(); 4355 } 4356 return getBodySite().get(0); 4357 } 4358 4359 /** 4360 * @return {@link #specimenRequirement} (Defines specimen requirements for the 4361 * action to be performed, such as required specimens for a lab test.) 4362 */ 4363 public List<Reference> getSpecimenRequirement() { 4364 if (this.specimenRequirement == null) 4365 this.specimenRequirement = new ArrayList<Reference>(); 4366 return this.specimenRequirement; 4367 } 4368 4369 /** 4370 * @return Returns a reference to <code>this</code> for easy method chaining 4371 */ 4372 public ActivityDefinition setSpecimenRequirement(List<Reference> theSpecimenRequirement) { 4373 this.specimenRequirement = theSpecimenRequirement; 4374 return this; 4375 } 4376 4377 public boolean hasSpecimenRequirement() { 4378 if (this.specimenRequirement == null) 4379 return false; 4380 for (Reference item : this.specimenRequirement) 4381 if (!item.isEmpty()) 4382 return true; 4383 return false; 4384 } 4385 4386 public Reference addSpecimenRequirement() { // 3 4387 Reference t = new Reference(); 4388 if (this.specimenRequirement == null) 4389 this.specimenRequirement = new ArrayList<Reference>(); 4390 this.specimenRequirement.add(t); 4391 return t; 4392 } 4393 4394 public ActivityDefinition addSpecimenRequirement(Reference t) { // 3 4395 if (t == null) 4396 return this; 4397 if (this.specimenRequirement == null) 4398 this.specimenRequirement = new ArrayList<Reference>(); 4399 this.specimenRequirement.add(t); 4400 return this; 4401 } 4402 4403 /** 4404 * @return The first repetition of repeating field {@link #specimenRequirement}, 4405 * creating it if it does not already exist 4406 */ 4407 public Reference getSpecimenRequirementFirstRep() { 4408 if (getSpecimenRequirement().isEmpty()) { 4409 addSpecimenRequirement(); 4410 } 4411 return getSpecimenRequirement().get(0); 4412 } 4413 4414 /** 4415 * @deprecated Use Reference#setResource(IBaseResource) instead 4416 */ 4417 @Deprecated 4418 public List<SpecimenDefinition> getSpecimenRequirementTarget() { 4419 if (this.specimenRequirementTarget == null) 4420 this.specimenRequirementTarget = new ArrayList<SpecimenDefinition>(); 4421 return this.specimenRequirementTarget; 4422 } 4423 4424 /** 4425 * @deprecated Use Reference#setResource(IBaseResource) instead 4426 */ 4427 @Deprecated 4428 public SpecimenDefinition addSpecimenRequirementTarget() { 4429 SpecimenDefinition r = new SpecimenDefinition(); 4430 if (this.specimenRequirementTarget == null) 4431 this.specimenRequirementTarget = new ArrayList<SpecimenDefinition>(); 4432 this.specimenRequirementTarget.add(r); 4433 return r; 4434 } 4435 4436 /** 4437 * @return {@link #observationRequirement} (Defines observation requirements for 4438 * the action to be performed, such as body weight or surface area.) 4439 */ 4440 public List<Reference> getObservationRequirement() { 4441 if (this.observationRequirement == null) 4442 this.observationRequirement = new ArrayList<Reference>(); 4443 return this.observationRequirement; 4444 } 4445 4446 /** 4447 * @return Returns a reference to <code>this</code> for easy method chaining 4448 */ 4449 public ActivityDefinition setObservationRequirement(List<Reference> theObservationRequirement) { 4450 this.observationRequirement = theObservationRequirement; 4451 return this; 4452 } 4453 4454 public boolean hasObservationRequirement() { 4455 if (this.observationRequirement == null) 4456 return false; 4457 for (Reference item : this.observationRequirement) 4458 if (!item.isEmpty()) 4459 return true; 4460 return false; 4461 } 4462 4463 public Reference addObservationRequirement() { // 3 4464 Reference t = new Reference(); 4465 if (this.observationRequirement == null) 4466 this.observationRequirement = new ArrayList<Reference>(); 4467 this.observationRequirement.add(t); 4468 return t; 4469 } 4470 4471 public ActivityDefinition addObservationRequirement(Reference t) { // 3 4472 if (t == null) 4473 return this; 4474 if (this.observationRequirement == null) 4475 this.observationRequirement = new ArrayList<Reference>(); 4476 this.observationRequirement.add(t); 4477 return this; 4478 } 4479 4480 /** 4481 * @return The first repetition of repeating field 4482 * {@link #observationRequirement}, creating it if it does not already 4483 * exist 4484 */ 4485 public Reference getObservationRequirementFirstRep() { 4486 if (getObservationRequirement().isEmpty()) { 4487 addObservationRequirement(); 4488 } 4489 return getObservationRequirement().get(0); 4490 } 4491 4492 /** 4493 * @deprecated Use Reference#setResource(IBaseResource) instead 4494 */ 4495 @Deprecated 4496 public List<ObservationDefinition> getObservationRequirementTarget() { 4497 if (this.observationRequirementTarget == null) 4498 this.observationRequirementTarget = new ArrayList<ObservationDefinition>(); 4499 return this.observationRequirementTarget; 4500 } 4501 4502 /** 4503 * @deprecated Use Reference#setResource(IBaseResource) instead 4504 */ 4505 @Deprecated 4506 public ObservationDefinition addObservationRequirementTarget() { 4507 ObservationDefinition r = new ObservationDefinition(); 4508 if (this.observationRequirementTarget == null) 4509 this.observationRequirementTarget = new ArrayList<ObservationDefinition>(); 4510 this.observationRequirementTarget.add(r); 4511 return r; 4512 } 4513 4514 /** 4515 * @return {@link #observationResultRequirement} (Defines the observations that 4516 * are expected to be produced by the action.) 4517 */ 4518 public List<Reference> getObservationResultRequirement() { 4519 if (this.observationResultRequirement == null) 4520 this.observationResultRequirement = new ArrayList<Reference>(); 4521 return this.observationResultRequirement; 4522 } 4523 4524 /** 4525 * @return Returns a reference to <code>this</code> for easy method chaining 4526 */ 4527 public ActivityDefinition setObservationResultRequirement(List<Reference> theObservationResultRequirement) { 4528 this.observationResultRequirement = theObservationResultRequirement; 4529 return this; 4530 } 4531 4532 public boolean hasObservationResultRequirement() { 4533 if (this.observationResultRequirement == null) 4534 return false; 4535 for (Reference item : this.observationResultRequirement) 4536 if (!item.isEmpty()) 4537 return true; 4538 return false; 4539 } 4540 4541 public Reference addObservationResultRequirement() { // 3 4542 Reference t = new Reference(); 4543 if (this.observationResultRequirement == null) 4544 this.observationResultRequirement = new ArrayList<Reference>(); 4545 this.observationResultRequirement.add(t); 4546 return t; 4547 } 4548 4549 public ActivityDefinition addObservationResultRequirement(Reference t) { // 3 4550 if (t == null) 4551 return this; 4552 if (this.observationResultRequirement == null) 4553 this.observationResultRequirement = new ArrayList<Reference>(); 4554 this.observationResultRequirement.add(t); 4555 return this; 4556 } 4557 4558 /** 4559 * @return The first repetition of repeating field 4560 * {@link #observationResultRequirement}, creating it if it does not 4561 * already exist 4562 */ 4563 public Reference getObservationResultRequirementFirstRep() { 4564 if (getObservationResultRequirement().isEmpty()) { 4565 addObservationResultRequirement(); 4566 } 4567 return getObservationResultRequirement().get(0); 4568 } 4569 4570 /** 4571 * @deprecated Use Reference#setResource(IBaseResource) instead 4572 */ 4573 @Deprecated 4574 public List<ObservationDefinition> getObservationResultRequirementTarget() { 4575 if (this.observationResultRequirementTarget == null) 4576 this.observationResultRequirementTarget = new ArrayList<ObservationDefinition>(); 4577 return this.observationResultRequirementTarget; 4578 } 4579 4580 /** 4581 * @deprecated Use Reference#setResource(IBaseResource) instead 4582 */ 4583 @Deprecated 4584 public ObservationDefinition addObservationResultRequirementTarget() { 4585 ObservationDefinition r = new ObservationDefinition(); 4586 if (this.observationResultRequirementTarget == null) 4587 this.observationResultRequirementTarget = new ArrayList<ObservationDefinition>(); 4588 this.observationResultRequirementTarget.add(r); 4589 return r; 4590 } 4591 4592 /** 4593 * @return {@link #transform} (A reference to a StructureMap resource that 4594 * defines a transform that can be executed to produce the intent 4595 * resource using the ActivityDefinition instance as the input.). This 4596 * is the underlying object with id, value and extensions. The accessor 4597 * "getTransform" gives direct access to the value 4598 */ 4599 public CanonicalType getTransformElement() { 4600 if (this.transform == null) 4601 if (Configuration.errorOnAutoCreate()) 4602 throw new Error("Attempt to auto-create ActivityDefinition.transform"); 4603 else if (Configuration.doAutoCreate()) 4604 this.transform = new CanonicalType(); // bb 4605 return this.transform; 4606 } 4607 4608 public boolean hasTransformElement() { 4609 return this.transform != null && !this.transform.isEmpty(); 4610 } 4611 4612 public boolean hasTransform() { 4613 return this.transform != null && !this.transform.isEmpty(); 4614 } 4615 4616 /** 4617 * @param value {@link #transform} (A reference to a StructureMap resource that 4618 * defines a transform that can be executed to produce the intent 4619 * resource using the ActivityDefinition instance as the input.). 4620 * This is the underlying object with id, value and extensions. The 4621 * accessor "getTransform" gives direct access to the value 4622 */ 4623 public ActivityDefinition setTransformElement(CanonicalType value) { 4624 this.transform = value; 4625 return this; 4626 } 4627 4628 /** 4629 * @return A reference to a StructureMap resource that defines a transform that 4630 * can be executed to produce the intent resource using the 4631 * ActivityDefinition instance as the input. 4632 */ 4633 public String getTransform() { 4634 return this.transform == null ? null : this.transform.getValue(); 4635 } 4636 4637 /** 4638 * @param value A reference to a StructureMap resource that defines a transform 4639 * that can be executed to produce the intent resource using the 4640 * ActivityDefinition instance as the input. 4641 */ 4642 public ActivityDefinition setTransform(String value) { 4643 if (Utilities.noString(value)) 4644 this.transform = null; 4645 else { 4646 if (this.transform == null) 4647 this.transform = new CanonicalType(); 4648 this.transform.setValue(value); 4649 } 4650 return this; 4651 } 4652 4653 /** 4654 * @return {@link #dynamicValue} (Dynamic values that will be evaluated to 4655 * produce values for elements of the resulting resource. For example, 4656 * if the dosage of a medication must be computed based on the patient's 4657 * weight, a dynamic value would be used to specify an expression that 4658 * calculated the weight, and the path on the request resource that 4659 * would contain the result.) 4660 */ 4661 public List<ActivityDefinitionDynamicValueComponent> getDynamicValue() { 4662 if (this.dynamicValue == null) 4663 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4664 return this.dynamicValue; 4665 } 4666 4667 /** 4668 * @return Returns a reference to <code>this</code> for easy method chaining 4669 */ 4670 public ActivityDefinition setDynamicValue(List<ActivityDefinitionDynamicValueComponent> theDynamicValue) { 4671 this.dynamicValue = theDynamicValue; 4672 return this; 4673 } 4674 4675 public boolean hasDynamicValue() { 4676 if (this.dynamicValue == null) 4677 return false; 4678 for (ActivityDefinitionDynamicValueComponent item : this.dynamicValue) 4679 if (!item.isEmpty()) 4680 return true; 4681 return false; 4682 } 4683 4684 public ActivityDefinitionDynamicValueComponent addDynamicValue() { // 3 4685 ActivityDefinitionDynamicValueComponent t = new ActivityDefinitionDynamicValueComponent(); 4686 if (this.dynamicValue == null) 4687 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4688 this.dynamicValue.add(t); 4689 return t; 4690 } 4691 4692 public ActivityDefinition addDynamicValue(ActivityDefinitionDynamicValueComponent t) { // 3 4693 if (t == null) 4694 return this; 4695 if (this.dynamicValue == null) 4696 this.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 4697 this.dynamicValue.add(t); 4698 return this; 4699 } 4700 4701 /** 4702 * @return The first repetition of repeating field {@link #dynamicValue}, 4703 * creating it if it does not already exist 4704 */ 4705 public ActivityDefinitionDynamicValueComponent getDynamicValueFirstRep() { 4706 if (getDynamicValue().isEmpty()) { 4707 addDynamicValue(); 4708 } 4709 return getDynamicValue().get(0); 4710 } 4711 4712 protected void listChildren(List<Property> children) { 4713 super.listChildren(children); 4714 children.add(new Property("url", "uri", 4715 "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 4716 0, 1, url)); 4717 children.add(new Property("identifier", "Identifier", 4718 "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4719 0, java.lang.Integer.MAX_VALUE, identifier)); 4720 children.add(new Property("version", "string", 4721 "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 4722 0, 1, version)); 4723 children.add(new Property("name", "string", 4724 "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4725 0, 1, name)); 4726 children.add(new Property("title", "string", 4727 "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title)); 4728 children.add(new Property("subtitle", "string", 4729 "An explanatory or alternate title for the activity definition giving additional information about its content.", 4730 0, 1, subtitle)); 4731 children.add(new Property("status", "code", 4732 "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 4733 children.add(new Property("experimental", "boolean", 4734 "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4735 0, 1, experimental)); 4736 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4737 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4738 subject)); 4739 children.add(new Property("date", "dateTime", 4740 "The date (and optionally time) when the activity definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 4741 0, 1, date)); 4742 children.add(new Property("publisher", "string", 4743 "The name of the organization or individual that published the activity definition.", 0, 1, publisher)); 4744 children.add(new Property("contact", "ContactDetail", 4745 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4746 java.lang.Integer.MAX_VALUE, contact)); 4747 children.add(new Property("description", "markdown", 4748 "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, 4749 description)); 4750 children.add(new Property("useContext", "UsageContext", 4751 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 4752 0, java.lang.Integer.MAX_VALUE, useContext)); 4753 children.add(new Property("jurisdiction", "CodeableConcept", 4754 "A legal or geographic region in which the activity definition is intended to be used.", 0, 4755 java.lang.Integer.MAX_VALUE, jurisdiction)); 4756 children.add(new Property("purpose", "markdown", 4757 "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, 4758 purpose)); 4759 children.add(new Property("usage", "string", 4760 "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage)); 4761 children.add(new Property("copyright", "markdown", 4762 "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 4763 0, 1, copyright)); 4764 children.add(new Property("approvalDate", "date", 4765 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4766 0, 1, approvalDate)); 4767 children.add(new Property("lastReviewDate", "date", 4768 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4769 0, 1, lastReviewDate)); 4770 children.add(new Property("effectivePeriod", "Period", 4771 "The period during which the activity definition content was or is planned to be in active use.", 0, 1, 4772 effectivePeriod)); 4773 children.add(new Property("topic", "CodeableConcept", 4774 "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 4775 0, java.lang.Integer.MAX_VALUE, topic)); 4776 children.add(new Property("author", "ContactDetail", 4777 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4778 java.lang.Integer.MAX_VALUE, author)); 4779 children.add(new Property("editor", "ContactDetail", 4780 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4781 java.lang.Integer.MAX_VALUE, editor)); 4782 children.add(new Property("reviewer", "ContactDetail", 4783 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4784 java.lang.Integer.MAX_VALUE, reviewer)); 4785 children.add(new Property("endorser", "ContactDetail", 4786 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4787 java.lang.Integer.MAX_VALUE, endorser)); 4788 children.add(new Property("relatedArtifact", "RelatedArtifact", 4789 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4790 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4791 children.add(new Property("library", "canonical(Library)", 4792 "A reference to a Library resource containing any formal logic used by the activity definition.", 0, 4793 java.lang.Integer.MAX_VALUE, library)); 4794 children.add(new Property("kind", "code", 4795 "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 4796 0, 1, kind)); 4797 children.add(new Property("profile", "canonical(StructureDefinition)", 4798 "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile)); 4799 children.add(new Property("code", "CodeableConcept", 4800 "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 0, 4801 1, code)); 4802 children.add(new Property("intent", "code", 4803 "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 4804 0, 1, intent)); 4805 children.add(new Property("priority", "code", 4806 "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority)); 4807 children.add(new Property("doNotPerform", "boolean", 4808 "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 4809 0, 1, doNotPerform)); 4810 children.add(new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 4811 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing)); 4812 children.add(new Property("location", "Reference(Location)", 4813 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 4814 location)); 4815 children.add(new Property("participant", "", "Indicates who should participate in performing the action described.", 4816 0, java.lang.Integer.MAX_VALUE, participant)); 4817 children.add(new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 4818 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product)); 4819 children.add(new Property("quantity", "SimpleQuantity", 4820 "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity)); 4821 children.add(new Property("dosage", "Dosage", 4822 "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 4823 0, java.lang.Integer.MAX_VALUE, dosage)); 4824 children.add(new Property("bodySite", "CodeableConcept", 4825 "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 0, 4826 java.lang.Integer.MAX_VALUE, bodySite)); 4827 children.add(new Property("specimenRequirement", "Reference(SpecimenDefinition)", 4828 "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, 4829 java.lang.Integer.MAX_VALUE, specimenRequirement)); 4830 children.add(new Property("observationRequirement", "Reference(ObservationDefinition)", 4831 "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, 4832 java.lang.Integer.MAX_VALUE, observationRequirement)); 4833 children.add(new Property("observationResultRequirement", "Reference(ObservationDefinition)", 4834 "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, 4835 observationResultRequirement)); 4836 children.add(new Property("transform", "canonical(StructureMap)", 4837 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4838 0, 1, transform)); 4839 children.add(new Property("dynamicValue", "", 4840 "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 4841 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 4842 } 4843 4844 @Override 4845 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4846 switch (_hash) { 4847 case 116079: 4848 /* url */ return new Property("url", "uri", 4849 "An absolute URI that is used to identify this activity definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this activity definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the activity definition is stored on different servers.", 4850 0, 1, url); 4851 case -1618432855: 4852 /* identifier */ return new Property("identifier", "Identifier", 4853 "A formal identifier that is used to identify this activity definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4854 0, java.lang.Integer.MAX_VALUE, identifier); 4855 case 351608024: 4856 /* version */ return new Property("version", "string", 4857 "The identifier that is used to identify this version of the activity definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the activity definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 4858 0, 1, version); 4859 case 3373707: 4860 /* name */ return new Property("name", "string", 4861 "A natural language name identifying the activity definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4862 0, 1, name); 4863 case 110371416: 4864 /* title */ return new Property("title", "string", 4865 "A short, descriptive, user-friendly title for the activity definition.", 0, 1, title); 4866 case -2060497896: 4867 /* subtitle */ return new Property("subtitle", "string", 4868 "An explanatory or alternate title for the activity definition giving additional information about its content.", 4869 0, 1, subtitle); 4870 case -892481550: 4871 /* status */ return new Property("status", "code", 4872 "The status of this activity definition. Enables tracking the life-cycle of the content.", 0, 1, status); 4873 case -404562712: 4874 /* experimental */ return new Property("experimental", "boolean", 4875 "A Boolean value to indicate that this activity definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4876 0, 1, experimental); 4877 case -573640748: 4878 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4879 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4880 subject); 4881 case -1867885268: 4882 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4883 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4884 subject); 4885 case -1257122603: 4886 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4887 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4888 subject); 4889 case 772938623: 4890 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4891 "A code or group definition that describes the intended subject of the activity being defined.", 0, 1, 4892 subject); 4893 case 3076014: 4894 /* date */ return new Property("date", "dateTime", 4895 "The date (and optionally time) when the activity definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the activity definition changes.", 4896 0, 1, date); 4897 case 1447404028: 4898 /* publisher */ return new Property("publisher", "string", 4899 "The name of the organization or individual that published the activity definition.", 0, 1, publisher); 4900 case 951526432: 4901 /* contact */ return new Property("contact", "ContactDetail", 4902 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4903 java.lang.Integer.MAX_VALUE, contact); 4904 case -1724546052: 4905 /* description */ return new Property("description", "markdown", 4906 "A free text natural language description of the activity definition from a consumer's perspective.", 0, 1, 4907 description); 4908 case -669707736: 4909 /* useContext */ return new Property("useContext", "UsageContext", 4910 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate activity definition instances.", 4911 0, java.lang.Integer.MAX_VALUE, useContext); 4912 case -507075711: 4913 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4914 "A legal or geographic region in which the activity definition is intended to be used.", 0, 4915 java.lang.Integer.MAX_VALUE, jurisdiction); 4916 case -220463842: 4917 /* purpose */ return new Property("purpose", "markdown", 4918 "Explanation of why this activity definition is needed and why it has been designed as it has.", 0, 1, 4919 purpose); 4920 case 111574433: 4921 /* usage */ return new Property("usage", "string", 4922 "A detailed description of how the activity definition is used from a clinical perspective.", 0, 1, usage); 4923 case 1522889671: 4924 /* copyright */ return new Property("copyright", "markdown", 4925 "A copyright statement relating to the activity definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the activity definition.", 4926 0, 1, copyright); 4927 case 223539345: 4928 /* approvalDate */ return new Property("approvalDate", "date", 4929 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4930 0, 1, approvalDate); 4931 case -1687512484: 4932 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4933 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4934 0, 1, lastReviewDate); 4935 case -403934648: 4936 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4937 "The period during which the activity definition content was or is planned to be in active use.", 0, 1, 4938 effectivePeriod); 4939 case 110546223: 4940 /* topic */ return new Property("topic", "CodeableConcept", 4941 "Descriptive topics related to the content of the activity. Topics provide a high-level categorization of the activity that can be useful for filtering and searching.", 4942 0, java.lang.Integer.MAX_VALUE, topic); 4943 case -1406328437: 4944 /* author */ return new Property("author", "ContactDetail", 4945 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4946 java.lang.Integer.MAX_VALUE, author); 4947 case -1307827859: 4948 /* editor */ return new Property("editor", "ContactDetail", 4949 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4950 java.lang.Integer.MAX_VALUE, editor); 4951 case -261190139: 4952 /* reviewer */ return new Property("reviewer", "ContactDetail", 4953 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4954 java.lang.Integer.MAX_VALUE, reviewer); 4955 case 1740277666: 4956 /* endorser */ return new Property("endorser", "ContactDetail", 4957 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4958 java.lang.Integer.MAX_VALUE, endorser); 4959 case 666807069: 4960 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4961 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4962 java.lang.Integer.MAX_VALUE, relatedArtifact); 4963 case 166208699: 4964 /* library */ return new Property("library", "canonical(Library)", 4965 "A reference to a Library resource containing any formal logic used by the activity definition.", 0, 4966 java.lang.Integer.MAX_VALUE, library); 4967 case 3292052: 4968 /* kind */ return new Property("kind", "code", 4969 "A description of the kind of resource the activity definition is representing. For example, a MedicationRequest, a ServiceRequest, or a CommunicationRequest. Typically, but not always, this is a Request resource.", 4970 0, 1, kind); 4971 case -309425751: 4972 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 4973 "A profile to which the target of the activity definition is expected to conform.", 0, 1, profile); 4974 case 3059181: 4975 /* code */ return new Property("code", "CodeableConcept", 4976 "Detailed description of the type of activity; e.g. What lab test, what procedure, what kind of encounter.", 4977 0, 1, code); 4978 case -1183762788: 4979 /* intent */ return new Property("intent", "code", 4980 "Indicates the level of authority/intentionality associated with the activity and where the request should fit into the workflow chain.", 4981 0, 1, intent); 4982 case -1165461084: 4983 /* priority */ return new Property("priority", "code", 4984 "Indicates how quickly the activity should be addressed with respect to other requests.", 0, 1, priority); 4985 case -1788508167: 4986 /* doNotPerform */ return new Property("doNotPerform", "boolean", 4987 "Set this to true if the definition is to indicate that a particular activity should NOT be performed. If true, this element should be interpreted to reinforce a negative coding. For example NPO as a code with a doNotPerform of true would still indicate to NOT perform the action.", 4988 0, 1, doNotPerform); 4989 case 164632566: 4990 /* timing[x] */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 4991 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4992 case -873664438: 4993 /* timing */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 4994 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4995 case -497554124: 4996 /* timingTiming */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 4997 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 4998 case -1837458939: 4999 /* timingDateTime */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5000 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5001 case 164607061: 5002 /* timingAge */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5003 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5004 case -615615829: 5005 /* timingPeriod */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5006 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5007 case -710871277: 5008 /* timingRange */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5009 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5010 case -1327253506: 5011 /* timingDuration */ return new Property("timing[x]", "Timing|dateTime|Age|Period|Range|Duration", 5012 "The period, timing or frequency upon which the described activity is to occur.", 0, 1, timing); 5013 case 1901043637: 5014 /* location */ return new Property("location", "Reference(Location)", 5015 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, 5016 location); 5017 case 767422259: 5018 /* participant */ return new Property("participant", "", 5019 "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, 5020 participant); 5021 case 1753005361: 5022 /* product[x] */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5023 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5024 case -309474065: 5025 /* product */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5026 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5027 case -669667556: 5028 /* productReference */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5029 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5030 case 906854066: 5031 /* productCodeableConcept */ return new Property("product[x]", "Reference(Medication|Substance)|CodeableConcept", 5032 "Identifies the food, drug or other product being consumed or supplied in the activity.", 0, 1, product); 5033 case -1285004149: 5034 /* quantity */ return new Property("quantity", "SimpleQuantity", 5035 "Identifies the quantity expected to be consumed at once (per dose, per meal, etc.).", 0, 1, quantity); 5036 case -1326018889: 5037 /* dosage */ return new Property("dosage", "Dosage", 5038 "Provides detailed dosage instructions in the same way that they are described for MedicationRequest resources.", 5039 0, java.lang.Integer.MAX_VALUE, dosage); 5040 case 1702620169: 5041 /* bodySite */ return new Property("bodySite", "CodeableConcept", 5042 "Indicates the sites on the subject's body where the procedure should be performed (I.e. the target sites).", 5043 0, java.lang.Integer.MAX_VALUE, bodySite); 5044 case 1498467355: 5045 /* specimenRequirement */ return new Property("specimenRequirement", "Reference(SpecimenDefinition)", 5046 "Defines specimen requirements for the action to be performed, such as required specimens for a lab test.", 0, 5047 java.lang.Integer.MAX_VALUE, specimenRequirement); 5048 case 362354807: 5049 /* observationRequirement */ return new Property("observationRequirement", "Reference(ObservationDefinition)", 5050 "Defines observation requirements for the action to be performed, such as body weight or surface area.", 0, 5051 java.lang.Integer.MAX_VALUE, observationRequirement); 5052 case 395230490: 5053 /* observationResultRequirement */ return new Property("observationResultRequirement", 5054 "Reference(ObservationDefinition)", 5055 "Defines the observations that are expected to be produced by the action.", 0, java.lang.Integer.MAX_VALUE, 5056 observationResultRequirement); 5057 case 1052666732: 5058 /* transform */ return new Property("transform", "canonical(StructureMap)", 5059 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 5060 0, 1, transform); 5061 case 572625010: 5062 /* dynamicValue */ return new Property("dynamicValue", "", 5063 "Dynamic values that will be evaluated to produce values for elements of the resulting resource. For example, if the dosage of a medication must be computed based on the patient's weight, a dynamic value would be used to specify an expression that calculated the weight, and the path on the request resource that would contain the result.", 5064 0, java.lang.Integer.MAX_VALUE, dynamicValue); 5065 default: 5066 return super.getNamedProperty(_hash, _name, _checkValid); 5067 } 5068 5069 } 5070 5071 @Override 5072 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5073 switch (hash) { 5074 case 116079: 5075 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5076 case -1618432855: 5077 /* identifier */ return this.identifier == null ? new Base[0] 5078 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5079 case 351608024: 5080 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5081 case 3373707: 5082 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5083 case 110371416: 5084 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5085 case -2060497896: 5086 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 5087 case -892481550: 5088 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5089 case -404562712: 5090 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5091 case -1867885268: 5092 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 5093 case 3076014: 5094 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5095 case 1447404028: 5096 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5097 case 951526432: 5098 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5099 case -1724546052: 5100 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5101 case -669707736: 5102 /* useContext */ return this.useContext == null ? new Base[0] 5103 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5104 case -507075711: 5105 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5106 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5107 case -220463842: 5108 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5109 case 111574433: 5110 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 5111 case 1522889671: 5112 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5113 case 223539345: 5114 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5115 case -1687512484: 5116 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5117 case -403934648: 5118 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5119 case 110546223: 5120 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5121 case -1406328437: 5122 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5123 case -1307827859: 5124 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5125 case -261190139: 5126 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5127 case 1740277666: 5128 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5129 case 666807069: 5130 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 5131 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5132 case 166208699: 5133 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 5134 case 3292052: 5135 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActivityDefinitionKind> 5136 case -309425751: 5137 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 5138 case 3059181: 5139 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 5140 case -1183762788: 5141 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<RequestIntent> 5142 case -1165461084: 5143 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 5144 case -1788508167: 5145 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 5146 case -873664438: 5147 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 5148 case 1901043637: 5149 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 5150 case 767422259: 5151 /* participant */ return this.participant == null ? new Base[0] 5152 : this.participant.toArray(new Base[this.participant.size()]); // ActivityDefinitionParticipantComponent 5153 case -309474065: 5154 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 5155 case -1285004149: 5156 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 5157 case -1326018889: 5158 /* dosage */ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 5159 case 1702620169: 5160 /* bodySite */ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 5161 case 1498467355: 5162 /* specimenRequirement */ return this.specimenRequirement == null ? new Base[0] 5163 : this.specimenRequirement.toArray(new Base[this.specimenRequirement.size()]); // Reference 5164 case 362354807: 5165 /* observationRequirement */ return this.observationRequirement == null ? new Base[0] 5166 : this.observationRequirement.toArray(new Base[this.observationRequirement.size()]); // Reference 5167 case 395230490: 5168 /* observationResultRequirement */ return this.observationResultRequirement == null ? new Base[0] 5169 : this.observationResultRequirement.toArray(new Base[this.observationResultRequirement.size()]); // Reference 5170 case 1052666732: 5171 /* transform */ return this.transform == null ? new Base[0] : new Base[] { this.transform }; // CanonicalType 5172 case 572625010: 5173 /* dynamicValue */ return this.dynamicValue == null ? new Base[0] 5174 : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // ActivityDefinitionDynamicValueComponent 5175 default: 5176 return super.getProperty(hash, name, checkValid); 5177 } 5178 5179 } 5180 5181 @Override 5182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5183 switch (hash) { 5184 case 116079: // url 5185 this.url = castToUri(value); // UriType 5186 return value; 5187 case -1618432855: // identifier 5188 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5189 return value; 5190 case 351608024: // version 5191 this.version = castToString(value); // StringType 5192 return value; 5193 case 3373707: // name 5194 this.name = castToString(value); // StringType 5195 return value; 5196 case 110371416: // title 5197 this.title = castToString(value); // StringType 5198 return value; 5199 case -2060497896: // subtitle 5200 this.subtitle = castToString(value); // StringType 5201 return value; 5202 case -892481550: // status 5203 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5204 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5205 return value; 5206 case -404562712: // experimental 5207 this.experimental = castToBoolean(value); // BooleanType 5208 return value; 5209 case -1867885268: // subject 5210 this.subject = castToType(value); // Type 5211 return value; 5212 case 3076014: // date 5213 this.date = castToDateTime(value); // DateTimeType 5214 return value; 5215 case 1447404028: // publisher 5216 this.publisher = castToString(value); // StringType 5217 return value; 5218 case 951526432: // contact 5219 this.getContact().add(castToContactDetail(value)); // ContactDetail 5220 return value; 5221 case -1724546052: // description 5222 this.description = castToMarkdown(value); // MarkdownType 5223 return value; 5224 case -669707736: // useContext 5225 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5226 return value; 5227 case -507075711: // jurisdiction 5228 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5229 return value; 5230 case -220463842: // purpose 5231 this.purpose = castToMarkdown(value); // MarkdownType 5232 return value; 5233 case 111574433: // usage 5234 this.usage = castToString(value); // StringType 5235 return value; 5236 case 1522889671: // copyright 5237 this.copyright = castToMarkdown(value); // MarkdownType 5238 return value; 5239 case 223539345: // approvalDate 5240 this.approvalDate = castToDate(value); // DateType 5241 return value; 5242 case -1687512484: // lastReviewDate 5243 this.lastReviewDate = castToDate(value); // DateType 5244 return value; 5245 case -403934648: // effectivePeriod 5246 this.effectivePeriod = castToPeriod(value); // Period 5247 return value; 5248 case 110546223: // topic 5249 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 5250 return value; 5251 case -1406328437: // author 5252 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 5253 return value; 5254 case -1307827859: // editor 5255 this.getEditor().add(castToContactDetail(value)); // ContactDetail 5256 return value; 5257 case -261190139: // reviewer 5258 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 5259 return value; 5260 case 1740277666: // endorser 5261 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 5262 return value; 5263 case 666807069: // relatedArtifact 5264 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 5265 return value; 5266 case 166208699: // library 5267 this.getLibrary().add(castToCanonical(value)); // CanonicalType 5268 return value; 5269 case 3292052: // kind 5270 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 5271 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 5272 return value; 5273 case -309425751: // profile 5274 this.profile = castToCanonical(value); // CanonicalType 5275 return value; 5276 case 3059181: // code 5277 this.code = castToCodeableConcept(value); // CodeableConcept 5278 return value; 5279 case -1183762788: // intent 5280 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5281 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5282 return value; 5283 case -1165461084: // priority 5284 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5285 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5286 return value; 5287 case -1788508167: // doNotPerform 5288 this.doNotPerform = castToBoolean(value); // BooleanType 5289 return value; 5290 case -873664438: // timing 5291 this.timing = castToType(value); // Type 5292 return value; 5293 case 1901043637: // location 5294 this.location = castToReference(value); // Reference 5295 return value; 5296 case 767422259: // participant 5297 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); // ActivityDefinitionParticipantComponent 5298 return value; 5299 case -309474065: // product 5300 this.product = castToType(value); // Type 5301 return value; 5302 case -1285004149: // quantity 5303 this.quantity = castToQuantity(value); // Quantity 5304 return value; 5305 case -1326018889: // dosage 5306 this.getDosage().add(castToDosage(value)); // Dosage 5307 return value; 5308 case 1702620169: // bodySite 5309 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 5310 return value; 5311 case 1498467355: // specimenRequirement 5312 this.getSpecimenRequirement().add(castToReference(value)); // Reference 5313 return value; 5314 case 362354807: // observationRequirement 5315 this.getObservationRequirement().add(castToReference(value)); // Reference 5316 return value; 5317 case 395230490: // observationResultRequirement 5318 this.getObservationResultRequirement().add(castToReference(value)); // Reference 5319 return value; 5320 case 1052666732: // transform 5321 this.transform = castToCanonical(value); // CanonicalType 5322 return value; 5323 case 572625010: // dynamicValue 5324 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); // ActivityDefinitionDynamicValueComponent 5325 return value; 5326 default: 5327 return super.setProperty(hash, name, value); 5328 } 5329 5330 } 5331 5332 @Override 5333 public Base setProperty(String name, Base value) throws FHIRException { 5334 if (name.equals("url")) { 5335 this.url = castToUri(value); // UriType 5336 } else if (name.equals("identifier")) { 5337 this.getIdentifier().add(castToIdentifier(value)); 5338 } else if (name.equals("version")) { 5339 this.version = castToString(value); // StringType 5340 } else if (name.equals("name")) { 5341 this.name = castToString(value); // StringType 5342 } else if (name.equals("title")) { 5343 this.title = castToString(value); // StringType 5344 } else if (name.equals("subtitle")) { 5345 this.subtitle = castToString(value); // StringType 5346 } else if (name.equals("status")) { 5347 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5348 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5349 } else if (name.equals("experimental")) { 5350 this.experimental = castToBoolean(value); // BooleanType 5351 } else if (name.equals("subject[x]")) { 5352 this.subject = castToType(value); // Type 5353 } else if (name.equals("date")) { 5354 this.date = castToDateTime(value); // DateTimeType 5355 } else if (name.equals("publisher")) { 5356 this.publisher = castToString(value); // StringType 5357 } else if (name.equals("contact")) { 5358 this.getContact().add(castToContactDetail(value)); 5359 } else if (name.equals("description")) { 5360 this.description = castToMarkdown(value); // MarkdownType 5361 } else if (name.equals("useContext")) { 5362 this.getUseContext().add(castToUsageContext(value)); 5363 } else if (name.equals("jurisdiction")) { 5364 this.getJurisdiction().add(castToCodeableConcept(value)); 5365 } else if (name.equals("purpose")) { 5366 this.purpose = castToMarkdown(value); // MarkdownType 5367 } else if (name.equals("usage")) { 5368 this.usage = castToString(value); // StringType 5369 } else if (name.equals("copyright")) { 5370 this.copyright = castToMarkdown(value); // MarkdownType 5371 } else if (name.equals("approvalDate")) { 5372 this.approvalDate = castToDate(value); // DateType 5373 } else if (name.equals("lastReviewDate")) { 5374 this.lastReviewDate = castToDate(value); // DateType 5375 } else if (name.equals("effectivePeriod")) { 5376 this.effectivePeriod = castToPeriod(value); // Period 5377 } else if (name.equals("topic")) { 5378 this.getTopic().add(castToCodeableConcept(value)); 5379 } else if (name.equals("author")) { 5380 this.getAuthor().add(castToContactDetail(value)); 5381 } else if (name.equals("editor")) { 5382 this.getEditor().add(castToContactDetail(value)); 5383 } else if (name.equals("reviewer")) { 5384 this.getReviewer().add(castToContactDetail(value)); 5385 } else if (name.equals("endorser")) { 5386 this.getEndorser().add(castToContactDetail(value)); 5387 } else if (name.equals("relatedArtifact")) { 5388 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 5389 } else if (name.equals("library")) { 5390 this.getLibrary().add(castToCanonical(value)); 5391 } else if (name.equals("kind")) { 5392 value = new ActivityDefinitionKindEnumFactory().fromType(castToCode(value)); 5393 this.kind = (Enumeration) value; // Enumeration<ActivityDefinitionKind> 5394 } else if (name.equals("profile")) { 5395 this.profile = castToCanonical(value); // CanonicalType 5396 } else if (name.equals("code")) { 5397 this.code = castToCodeableConcept(value); // CodeableConcept 5398 } else if (name.equals("intent")) { 5399 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5400 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5401 } else if (name.equals("priority")) { 5402 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5403 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5404 } else if (name.equals("doNotPerform")) { 5405 this.doNotPerform = castToBoolean(value); // BooleanType 5406 } else if (name.equals("timing[x]")) { 5407 this.timing = castToType(value); // Type 5408 } else if (name.equals("location")) { 5409 this.location = castToReference(value); // Reference 5410 } else if (name.equals("participant")) { 5411 this.getParticipant().add((ActivityDefinitionParticipantComponent) value); 5412 } else if (name.equals("product[x]")) { 5413 this.product = castToType(value); // Type 5414 } else if (name.equals("quantity")) { 5415 this.quantity = castToQuantity(value); // Quantity 5416 } else if (name.equals("dosage")) { 5417 this.getDosage().add(castToDosage(value)); 5418 } else if (name.equals("bodySite")) { 5419 this.getBodySite().add(castToCodeableConcept(value)); 5420 } else if (name.equals("specimenRequirement")) { 5421 this.getSpecimenRequirement().add(castToReference(value)); 5422 } else if (name.equals("observationRequirement")) { 5423 this.getObservationRequirement().add(castToReference(value)); 5424 } else if (name.equals("observationResultRequirement")) { 5425 this.getObservationResultRequirement().add(castToReference(value)); 5426 } else if (name.equals("transform")) { 5427 this.transform = castToCanonical(value); // CanonicalType 5428 } else if (name.equals("dynamicValue")) { 5429 this.getDynamicValue().add((ActivityDefinitionDynamicValueComponent) value); 5430 } else 5431 return super.setProperty(name, value); 5432 return value; 5433 } 5434 5435 @Override 5436 public Base makeProperty(int hash, String name) throws FHIRException { 5437 switch (hash) { 5438 case 116079: 5439 return getUrlElement(); 5440 case -1618432855: 5441 return addIdentifier(); 5442 case 351608024: 5443 return getVersionElement(); 5444 case 3373707: 5445 return getNameElement(); 5446 case 110371416: 5447 return getTitleElement(); 5448 case -2060497896: 5449 return getSubtitleElement(); 5450 case -892481550: 5451 return getStatusElement(); 5452 case -404562712: 5453 return getExperimentalElement(); 5454 case -573640748: 5455 return getSubject(); 5456 case -1867885268: 5457 return getSubject(); 5458 case 3076014: 5459 return getDateElement(); 5460 case 1447404028: 5461 return getPublisherElement(); 5462 case 951526432: 5463 return addContact(); 5464 case -1724546052: 5465 return getDescriptionElement(); 5466 case -669707736: 5467 return addUseContext(); 5468 case -507075711: 5469 return addJurisdiction(); 5470 case -220463842: 5471 return getPurposeElement(); 5472 case 111574433: 5473 return getUsageElement(); 5474 case 1522889671: 5475 return getCopyrightElement(); 5476 case 223539345: 5477 return getApprovalDateElement(); 5478 case -1687512484: 5479 return getLastReviewDateElement(); 5480 case -403934648: 5481 return getEffectivePeriod(); 5482 case 110546223: 5483 return addTopic(); 5484 case -1406328437: 5485 return addAuthor(); 5486 case -1307827859: 5487 return addEditor(); 5488 case -261190139: 5489 return addReviewer(); 5490 case 1740277666: 5491 return addEndorser(); 5492 case 666807069: 5493 return addRelatedArtifact(); 5494 case 166208699: 5495 return addLibraryElement(); 5496 case 3292052: 5497 return getKindElement(); 5498 case -309425751: 5499 return getProfileElement(); 5500 case 3059181: 5501 return getCode(); 5502 case -1183762788: 5503 return getIntentElement(); 5504 case -1165461084: 5505 return getPriorityElement(); 5506 case -1788508167: 5507 return getDoNotPerformElement(); 5508 case 164632566: 5509 return getTiming(); 5510 case -873664438: 5511 return getTiming(); 5512 case 1901043637: 5513 return getLocation(); 5514 case 767422259: 5515 return addParticipant(); 5516 case 1753005361: 5517 return getProduct(); 5518 case -309474065: 5519 return getProduct(); 5520 case -1285004149: 5521 return getQuantity(); 5522 case -1326018889: 5523 return addDosage(); 5524 case 1702620169: 5525 return addBodySite(); 5526 case 1498467355: 5527 return addSpecimenRequirement(); 5528 case 362354807: 5529 return addObservationRequirement(); 5530 case 395230490: 5531 return addObservationResultRequirement(); 5532 case 1052666732: 5533 return getTransformElement(); 5534 case 572625010: 5535 return addDynamicValue(); 5536 default: 5537 return super.makeProperty(hash, name); 5538 } 5539 5540 } 5541 5542 @Override 5543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5544 switch (hash) { 5545 case 116079: 5546 /* url */ return new String[] { "uri" }; 5547 case -1618432855: 5548 /* identifier */ return new String[] { "Identifier" }; 5549 case 351608024: 5550 /* version */ return new String[] { "string" }; 5551 case 3373707: 5552 /* name */ return new String[] { "string" }; 5553 case 110371416: 5554 /* title */ return new String[] { "string" }; 5555 case -2060497896: 5556 /* subtitle */ return new String[] { "string" }; 5557 case -892481550: 5558 /* status */ return new String[] { "code" }; 5559 case -404562712: 5560 /* experimental */ return new String[] { "boolean" }; 5561 case -1867885268: 5562 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 5563 case 3076014: 5564 /* date */ return new String[] { "dateTime" }; 5565 case 1447404028: 5566 /* publisher */ return new String[] { "string" }; 5567 case 951526432: 5568 /* contact */ return new String[] { "ContactDetail" }; 5569 case -1724546052: 5570 /* description */ return new String[] { "markdown" }; 5571 case -669707736: 5572 /* useContext */ return new String[] { "UsageContext" }; 5573 case -507075711: 5574 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5575 case -220463842: 5576 /* purpose */ return new String[] { "markdown" }; 5577 case 111574433: 5578 /* usage */ return new String[] { "string" }; 5579 case 1522889671: 5580 /* copyright */ return new String[] { "markdown" }; 5581 case 223539345: 5582 /* approvalDate */ return new String[] { "date" }; 5583 case -1687512484: 5584 /* lastReviewDate */ return new String[] { "date" }; 5585 case -403934648: 5586 /* effectivePeriod */ return new String[] { "Period" }; 5587 case 110546223: 5588 /* topic */ return new String[] { "CodeableConcept" }; 5589 case -1406328437: 5590 /* author */ return new String[] { "ContactDetail" }; 5591 case -1307827859: 5592 /* editor */ return new String[] { "ContactDetail" }; 5593 case -261190139: 5594 /* reviewer */ return new String[] { "ContactDetail" }; 5595 case 1740277666: 5596 /* endorser */ return new String[] { "ContactDetail" }; 5597 case 666807069: 5598 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 5599 case 166208699: 5600 /* library */ return new String[] { "canonical" }; 5601 case 3292052: 5602 /* kind */ return new String[] { "code" }; 5603 case -309425751: 5604 /* profile */ return new String[] { "canonical" }; 5605 case 3059181: 5606 /* code */ return new String[] { "CodeableConcept" }; 5607 case -1183762788: 5608 /* intent */ return new String[] { "code" }; 5609 case -1165461084: 5610 /* priority */ return new String[] { "code" }; 5611 case -1788508167: 5612 /* doNotPerform */ return new String[] { "boolean" }; 5613 case -873664438: 5614 /* timing */ return new String[] { "Timing", "dateTime", "Age", "Period", "Range", "Duration" }; 5615 case 1901043637: 5616 /* location */ return new String[] { "Reference" }; 5617 case 767422259: 5618 /* participant */ return new String[] {}; 5619 case -309474065: 5620 /* product */ return new String[] { "Reference", "CodeableConcept" }; 5621 case -1285004149: 5622 /* quantity */ return new String[] { "SimpleQuantity" }; 5623 case -1326018889: 5624 /* dosage */ return new String[] { "Dosage" }; 5625 case 1702620169: 5626 /* bodySite */ return new String[] { "CodeableConcept" }; 5627 case 1498467355: 5628 /* specimenRequirement */ return new String[] { "Reference" }; 5629 case 362354807: 5630 /* observationRequirement */ return new String[] { "Reference" }; 5631 case 395230490: 5632 /* observationResultRequirement */ return new String[] { "Reference" }; 5633 case 1052666732: 5634 /* transform */ return new String[] { "canonical" }; 5635 case 572625010: 5636 /* dynamicValue */ return new String[] {}; 5637 default: 5638 return super.getTypesForProperty(hash, name); 5639 } 5640 5641 } 5642 5643 @Override 5644 public Base addChild(String name) throws FHIRException { 5645 if (name.equals("url")) { 5646 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.url"); 5647 } else if (name.equals("identifier")) { 5648 return addIdentifier(); 5649 } else if (name.equals("version")) { 5650 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.version"); 5651 } else if (name.equals("name")) { 5652 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.name"); 5653 } else if (name.equals("title")) { 5654 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.title"); 5655 } else if (name.equals("subtitle")) { 5656 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.subtitle"); 5657 } else if (name.equals("status")) { 5658 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.status"); 5659 } else if (name.equals("experimental")) { 5660 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.experimental"); 5661 } else if (name.equals("subjectCodeableConcept")) { 5662 this.subject = new CodeableConcept(); 5663 return this.subject; 5664 } else if (name.equals("subjectReference")) { 5665 this.subject = new Reference(); 5666 return this.subject; 5667 } else if (name.equals("date")) { 5668 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.date"); 5669 } else if (name.equals("publisher")) { 5670 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.publisher"); 5671 } else if (name.equals("contact")) { 5672 return addContact(); 5673 } else if (name.equals("description")) { 5674 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.description"); 5675 } else if (name.equals("useContext")) { 5676 return addUseContext(); 5677 } else if (name.equals("jurisdiction")) { 5678 return addJurisdiction(); 5679 } else if (name.equals("purpose")) { 5680 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.purpose"); 5681 } else if (name.equals("usage")) { 5682 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.usage"); 5683 } else if (name.equals("copyright")) { 5684 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.copyright"); 5685 } else if (name.equals("approvalDate")) { 5686 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.approvalDate"); 5687 } else if (name.equals("lastReviewDate")) { 5688 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.lastReviewDate"); 5689 } else if (name.equals("effectivePeriod")) { 5690 this.effectivePeriod = new Period(); 5691 return this.effectivePeriod; 5692 } else if (name.equals("topic")) { 5693 return addTopic(); 5694 } else if (name.equals("author")) { 5695 return addAuthor(); 5696 } else if (name.equals("editor")) { 5697 return addEditor(); 5698 } else if (name.equals("reviewer")) { 5699 return addReviewer(); 5700 } else if (name.equals("endorser")) { 5701 return addEndorser(); 5702 } else if (name.equals("relatedArtifact")) { 5703 return addRelatedArtifact(); 5704 } else if (name.equals("library")) { 5705 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.library"); 5706 } else if (name.equals("kind")) { 5707 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.kind"); 5708 } else if (name.equals("profile")) { 5709 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.profile"); 5710 } else if (name.equals("code")) { 5711 this.code = new CodeableConcept(); 5712 return this.code; 5713 } else if (name.equals("intent")) { 5714 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.intent"); 5715 } else if (name.equals("priority")) { 5716 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.priority"); 5717 } else if (name.equals("doNotPerform")) { 5718 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.doNotPerform"); 5719 } else if (name.equals("timingTiming")) { 5720 this.timing = new Timing(); 5721 return this.timing; 5722 } else if (name.equals("timingDateTime")) { 5723 this.timing = new DateTimeType(); 5724 return this.timing; 5725 } else if (name.equals("timingAge")) { 5726 this.timing = new Age(); 5727 return this.timing; 5728 } else if (name.equals("timingPeriod")) { 5729 this.timing = new Period(); 5730 return this.timing; 5731 } else if (name.equals("timingRange")) { 5732 this.timing = new Range(); 5733 return this.timing; 5734 } else if (name.equals("timingDuration")) { 5735 this.timing = new Duration(); 5736 return this.timing; 5737 } else if (name.equals("location")) { 5738 this.location = new Reference(); 5739 return this.location; 5740 } else if (name.equals("participant")) { 5741 return addParticipant(); 5742 } else if (name.equals("productReference")) { 5743 this.product = new Reference(); 5744 return this.product; 5745 } else if (name.equals("productCodeableConcept")) { 5746 this.product = new CodeableConcept(); 5747 return this.product; 5748 } else if (name.equals("quantity")) { 5749 this.quantity = new Quantity(); 5750 return this.quantity; 5751 } else if (name.equals("dosage")) { 5752 return addDosage(); 5753 } else if (name.equals("bodySite")) { 5754 return addBodySite(); 5755 } else if (name.equals("specimenRequirement")) { 5756 return addSpecimenRequirement(); 5757 } else if (name.equals("observationRequirement")) { 5758 return addObservationRequirement(); 5759 } else if (name.equals("observationResultRequirement")) { 5760 return addObservationResultRequirement(); 5761 } else if (name.equals("transform")) { 5762 throw new FHIRException("Cannot call addChild on a singleton property ActivityDefinition.transform"); 5763 } else if (name.equals("dynamicValue")) { 5764 return addDynamicValue(); 5765 } else 5766 return super.addChild(name); 5767 } 5768 5769 public String fhirType() { 5770 return "ActivityDefinition"; 5771 5772 } 5773 5774 public ActivityDefinition copy() { 5775 ActivityDefinition dst = new ActivityDefinition(); 5776 copyValues(dst); 5777 return dst; 5778 } 5779 5780 public void copyValues(ActivityDefinition dst) { 5781 super.copyValues(dst); 5782 dst.url = url == null ? null : url.copy(); 5783 if (identifier != null) { 5784 dst.identifier = new ArrayList<Identifier>(); 5785 for (Identifier i : identifier) 5786 dst.identifier.add(i.copy()); 5787 } 5788 ; 5789 dst.version = version == null ? null : version.copy(); 5790 dst.name = name == null ? null : name.copy(); 5791 dst.title = title == null ? null : title.copy(); 5792 dst.subtitle = subtitle == null ? null : subtitle.copy(); 5793 dst.status = status == null ? null : status.copy(); 5794 dst.experimental = experimental == null ? null : experimental.copy(); 5795 dst.subject = subject == null ? null : subject.copy(); 5796 dst.date = date == null ? null : date.copy(); 5797 dst.publisher = publisher == null ? null : publisher.copy(); 5798 if (contact != null) { 5799 dst.contact = new ArrayList<ContactDetail>(); 5800 for (ContactDetail i : contact) 5801 dst.contact.add(i.copy()); 5802 } 5803 ; 5804 dst.description = description == null ? null : description.copy(); 5805 if (useContext != null) { 5806 dst.useContext = new ArrayList<UsageContext>(); 5807 for (UsageContext i : useContext) 5808 dst.useContext.add(i.copy()); 5809 } 5810 ; 5811 if (jurisdiction != null) { 5812 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5813 for (CodeableConcept i : jurisdiction) 5814 dst.jurisdiction.add(i.copy()); 5815 } 5816 ; 5817 dst.purpose = purpose == null ? null : purpose.copy(); 5818 dst.usage = usage == null ? null : usage.copy(); 5819 dst.copyright = copyright == null ? null : copyright.copy(); 5820 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5821 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5822 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5823 if (topic != null) { 5824 dst.topic = new ArrayList<CodeableConcept>(); 5825 for (CodeableConcept i : topic) 5826 dst.topic.add(i.copy()); 5827 } 5828 ; 5829 if (author != null) { 5830 dst.author = new ArrayList<ContactDetail>(); 5831 for (ContactDetail i : author) 5832 dst.author.add(i.copy()); 5833 } 5834 ; 5835 if (editor != null) { 5836 dst.editor = new ArrayList<ContactDetail>(); 5837 for (ContactDetail i : editor) 5838 dst.editor.add(i.copy()); 5839 } 5840 ; 5841 if (reviewer != null) { 5842 dst.reviewer = new ArrayList<ContactDetail>(); 5843 for (ContactDetail i : reviewer) 5844 dst.reviewer.add(i.copy()); 5845 } 5846 ; 5847 if (endorser != null) { 5848 dst.endorser = new ArrayList<ContactDetail>(); 5849 for (ContactDetail i : endorser) 5850 dst.endorser.add(i.copy()); 5851 } 5852 ; 5853 if (relatedArtifact != null) { 5854 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5855 for (RelatedArtifact i : relatedArtifact) 5856 dst.relatedArtifact.add(i.copy()); 5857 } 5858 ; 5859 if (library != null) { 5860 dst.library = new ArrayList<CanonicalType>(); 5861 for (CanonicalType i : library) 5862 dst.library.add(i.copy()); 5863 } 5864 ; 5865 dst.kind = kind == null ? null : kind.copy(); 5866 dst.profile = profile == null ? null : profile.copy(); 5867 dst.code = code == null ? null : code.copy(); 5868 dst.intent = intent == null ? null : intent.copy(); 5869 dst.priority = priority == null ? null : priority.copy(); 5870 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 5871 dst.timing = timing == null ? null : timing.copy(); 5872 dst.location = location == null ? null : location.copy(); 5873 if (participant != null) { 5874 dst.participant = new ArrayList<ActivityDefinitionParticipantComponent>(); 5875 for (ActivityDefinitionParticipantComponent i : participant) 5876 dst.participant.add(i.copy()); 5877 } 5878 ; 5879 dst.product = product == null ? null : product.copy(); 5880 dst.quantity = quantity == null ? null : quantity.copy(); 5881 if (dosage != null) { 5882 dst.dosage = new ArrayList<Dosage>(); 5883 for (Dosage i : dosage) 5884 dst.dosage.add(i.copy()); 5885 } 5886 ; 5887 if (bodySite != null) { 5888 dst.bodySite = new ArrayList<CodeableConcept>(); 5889 for (CodeableConcept i : bodySite) 5890 dst.bodySite.add(i.copy()); 5891 } 5892 ; 5893 if (specimenRequirement != null) { 5894 dst.specimenRequirement = new ArrayList<Reference>(); 5895 for (Reference i : specimenRequirement) 5896 dst.specimenRequirement.add(i.copy()); 5897 } 5898 ; 5899 if (observationRequirement != null) { 5900 dst.observationRequirement = new ArrayList<Reference>(); 5901 for (Reference i : observationRequirement) 5902 dst.observationRequirement.add(i.copy()); 5903 } 5904 ; 5905 if (observationResultRequirement != null) { 5906 dst.observationResultRequirement = new ArrayList<Reference>(); 5907 for (Reference i : observationResultRequirement) 5908 dst.observationResultRequirement.add(i.copy()); 5909 } 5910 ; 5911 dst.transform = transform == null ? null : transform.copy(); 5912 if (dynamicValue != null) { 5913 dst.dynamicValue = new ArrayList<ActivityDefinitionDynamicValueComponent>(); 5914 for (ActivityDefinitionDynamicValueComponent i : dynamicValue) 5915 dst.dynamicValue.add(i.copy()); 5916 } 5917 ; 5918 } 5919 5920 protected ActivityDefinition typedCopy() { 5921 return copy(); 5922 } 5923 5924 @Override 5925 public boolean equalsDeep(Base other_) { 5926 if (!super.equalsDeep(other_)) 5927 return false; 5928 if (!(other_ instanceof ActivityDefinition)) 5929 return false; 5930 ActivityDefinition o = (ActivityDefinition) other_; 5931 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 5932 && compareDeep(subject, o.subject, true) && compareDeep(purpose, o.purpose, true) 5933 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 5934 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5935 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 5936 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 5937 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5938 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 5939 && compareDeep(kind, o.kind, true) && compareDeep(profile, o.profile, true) && compareDeep(code, o.code, true) 5940 && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 5941 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(timing, o.timing, true) 5942 && compareDeep(location, o.location, true) && compareDeep(participant, o.participant, true) 5943 && compareDeep(product, o.product, true) && compareDeep(quantity, o.quantity, true) 5944 && compareDeep(dosage, o.dosage, true) && compareDeep(bodySite, o.bodySite, true) 5945 && compareDeep(specimenRequirement, o.specimenRequirement, true) 5946 && compareDeep(observationRequirement, o.observationRequirement, true) 5947 && compareDeep(observationResultRequirement, o.observationResultRequirement, true) 5948 && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true); 5949 } 5950 5951 @Override 5952 public boolean equalsShallow(Base other_) { 5953 if (!super.equalsShallow(other_)) 5954 return false; 5955 if (!(other_ instanceof ActivityDefinition)) 5956 return false; 5957 ActivityDefinition o = (ActivityDefinition) other_; 5958 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 5959 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 5960 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5961 && compareValues(kind, o.kind, true) && compareValues(intent, o.intent, true) 5962 && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true); 5963 } 5964 5965 public boolean isEmpty() { 5966 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, subject, purpose, usage, 5967 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 5968 relatedArtifact, library, kind, profile, code, intent, priority, doNotPerform, timing, location, participant, 5969 product, quantity, dosage, bodySite, specimenRequirement, observationRequirement, observationResultRequirement, 5970 transform, dynamicValue); 5971 } 5972 5973 @Override 5974 public ResourceType getResourceType() { 5975 return ResourceType.ActivityDefinition; 5976 } 5977 5978 /** 5979 * Search parameter: <b>date</b> 5980 * <p> 5981 * Description: <b>The activity definition publication date</b><br> 5982 * Type: <b>date</b><br> 5983 * Path: <b>ActivityDefinition.date</b><br> 5984 * </p> 5985 */ 5986 @SearchParamDefinition(name = "date", path = "ActivityDefinition.date", description = "The activity definition publication date", type = "date") 5987 public static final String SP_DATE = "date"; 5988 /** 5989 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5990 * <p> 5991 * Description: <b>The activity definition publication date</b><br> 5992 * Type: <b>date</b><br> 5993 * Path: <b>ActivityDefinition.date</b><br> 5994 * </p> 5995 */ 5996 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5997 SP_DATE); 5998 5999 /** 6000 * Search parameter: <b>identifier</b> 6001 * <p> 6002 * Description: <b>External identifier for the activity definition</b><br> 6003 * Type: <b>token</b><br> 6004 * Path: <b>ActivityDefinition.identifier</b><br> 6005 * </p> 6006 */ 6007 @SearchParamDefinition(name = "identifier", path = "ActivityDefinition.identifier", description = "External identifier for the activity definition", type = "token") 6008 public static final String SP_IDENTIFIER = "identifier"; 6009 /** 6010 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6011 * <p> 6012 * Description: <b>External identifier for the activity definition</b><br> 6013 * Type: <b>token</b><br> 6014 * Path: <b>ActivityDefinition.identifier</b><br> 6015 * </p> 6016 */ 6017 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6018 SP_IDENTIFIER); 6019 6020 /** 6021 * Search parameter: <b>successor</b> 6022 * <p> 6023 * Description: <b>What resource is being referenced</b><br> 6024 * Type: <b>reference</b><br> 6025 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6026 * </p> 6027 */ 6028 @SearchParamDefinition(name = "successor", path = "ActivityDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 6029 public static final String SP_SUCCESSOR = "successor"; 6030 /** 6031 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 6032 * <p> 6033 * Description: <b>What resource is being referenced</b><br> 6034 * Type: <b>reference</b><br> 6035 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6036 * </p> 6037 */ 6038 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6039 SP_SUCCESSOR); 6040 6041 /** 6042 * Constant for fluent queries to be used to add include statements. Specifies 6043 * the path value of "<b>ActivityDefinition:successor</b>". 6044 */ 6045 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 6046 "ActivityDefinition:successor").toLocked(); 6047 6048 /** 6049 * Search parameter: <b>context-type-value</b> 6050 * <p> 6051 * Description: <b>A use context type and value assigned to the activity 6052 * definition</b><br> 6053 * Type: <b>composite</b><br> 6054 * Path: <b></b><br> 6055 * </p> 6056 */ 6057 @SearchParamDefinition(name = "context-type-value", path = "ActivityDefinition.useContext", description = "A use context type and value assigned to the activity definition", type = "composite", compositeOf = { 6058 "context-type", "context" }) 6059 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6060 /** 6061 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6062 * <p> 6063 * Description: <b>A use context type and value assigned to the activity 6064 * definition</b><br> 6065 * Type: <b>composite</b><br> 6066 * Path: <b></b><br> 6067 * </p> 6068 */ 6069 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 6070 SP_CONTEXT_TYPE_VALUE); 6071 6072 /** 6073 * Search parameter: <b>jurisdiction</b> 6074 * <p> 6075 * Description: <b>Intended jurisdiction for the activity definition</b><br> 6076 * Type: <b>token</b><br> 6077 * Path: <b>ActivityDefinition.jurisdiction</b><br> 6078 * </p> 6079 */ 6080 @SearchParamDefinition(name = "jurisdiction", path = "ActivityDefinition.jurisdiction", description = "Intended jurisdiction for the activity definition", type = "token") 6081 public static final String SP_JURISDICTION = "jurisdiction"; 6082 /** 6083 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6084 * <p> 6085 * Description: <b>Intended jurisdiction for the activity definition</b><br> 6086 * Type: <b>token</b><br> 6087 * Path: <b>ActivityDefinition.jurisdiction</b><br> 6088 * </p> 6089 */ 6090 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6091 SP_JURISDICTION); 6092 6093 /** 6094 * Search parameter: <b>description</b> 6095 * <p> 6096 * Description: <b>The description of the activity definition</b><br> 6097 * Type: <b>string</b><br> 6098 * Path: <b>ActivityDefinition.description</b><br> 6099 * </p> 6100 */ 6101 @SearchParamDefinition(name = "description", path = "ActivityDefinition.description", description = "The description of the activity definition", type = "string") 6102 public static final String SP_DESCRIPTION = "description"; 6103 /** 6104 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6105 * <p> 6106 * Description: <b>The description of the activity definition</b><br> 6107 * Type: <b>string</b><br> 6108 * Path: <b>ActivityDefinition.description</b><br> 6109 * </p> 6110 */ 6111 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 6112 SP_DESCRIPTION); 6113 6114 /** 6115 * Search parameter: <b>derived-from</b> 6116 * <p> 6117 * Description: <b>What resource is being referenced</b><br> 6118 * Type: <b>reference</b><br> 6119 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6120 * </p> 6121 */ 6122 @SearchParamDefinition(name = "derived-from", path = "ActivityDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 6123 public static final String SP_DERIVED_FROM = "derived-from"; 6124 /** 6125 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6126 * <p> 6127 * Description: <b>What resource is being referenced</b><br> 6128 * Type: <b>reference</b><br> 6129 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6130 * </p> 6131 */ 6132 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6133 SP_DERIVED_FROM); 6134 6135 /** 6136 * Constant for fluent queries to be used to add include statements. Specifies 6137 * the path value of "<b>ActivityDefinition:derived-from</b>". 6138 */ 6139 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 6140 "ActivityDefinition:derived-from").toLocked(); 6141 6142 /** 6143 * Search parameter: <b>context-type</b> 6144 * <p> 6145 * Description: <b>A type of use context assigned to the activity 6146 * definition</b><br> 6147 * Type: <b>token</b><br> 6148 * Path: <b>ActivityDefinition.useContext.code</b><br> 6149 * </p> 6150 */ 6151 @SearchParamDefinition(name = "context-type", path = "ActivityDefinition.useContext.code", description = "A type of use context assigned to the activity definition", type = "token") 6152 public static final String SP_CONTEXT_TYPE = "context-type"; 6153 /** 6154 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6155 * <p> 6156 * Description: <b>A type of use context assigned to the activity 6157 * definition</b><br> 6158 * Type: <b>token</b><br> 6159 * Path: <b>ActivityDefinition.useContext.code</b><br> 6160 * </p> 6161 */ 6162 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6163 SP_CONTEXT_TYPE); 6164 6165 /** 6166 * Search parameter: <b>predecessor</b> 6167 * <p> 6168 * Description: <b>What resource is being referenced</b><br> 6169 * Type: <b>reference</b><br> 6170 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6171 * </p> 6172 */ 6173 @SearchParamDefinition(name = "predecessor", path = "ActivityDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 6174 public static final String SP_PREDECESSOR = "predecessor"; 6175 /** 6176 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6177 * <p> 6178 * Description: <b>What resource is being referenced</b><br> 6179 * Type: <b>reference</b><br> 6180 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6181 * </p> 6182 */ 6183 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6184 SP_PREDECESSOR); 6185 6186 /** 6187 * Constant for fluent queries to be used to add include statements. Specifies 6188 * the path value of "<b>ActivityDefinition:predecessor</b>". 6189 */ 6190 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 6191 "ActivityDefinition:predecessor").toLocked(); 6192 6193 /** 6194 * Search parameter: <b>title</b> 6195 * <p> 6196 * Description: <b>The human-friendly name of the activity definition</b><br> 6197 * Type: <b>string</b><br> 6198 * Path: <b>ActivityDefinition.title</b><br> 6199 * </p> 6200 */ 6201 @SearchParamDefinition(name = "title", path = "ActivityDefinition.title", description = "The human-friendly name of the activity definition", type = "string") 6202 public static final String SP_TITLE = "title"; 6203 /** 6204 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6205 * <p> 6206 * Description: <b>The human-friendly name of the activity definition</b><br> 6207 * Type: <b>string</b><br> 6208 * Path: <b>ActivityDefinition.title</b><br> 6209 * </p> 6210 */ 6211 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 6212 SP_TITLE); 6213 6214 /** 6215 * Search parameter: <b>composed-of</b> 6216 * <p> 6217 * Description: <b>What resource is being referenced</b><br> 6218 * Type: <b>reference</b><br> 6219 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6220 * </p> 6221 */ 6222 @SearchParamDefinition(name = "composed-of", path = "ActivityDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 6223 public static final String SP_COMPOSED_OF = "composed-of"; 6224 /** 6225 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6226 * <p> 6227 * Description: <b>What resource is being referenced</b><br> 6228 * Type: <b>reference</b><br> 6229 * Path: <b>ActivityDefinition.relatedArtifact.resource</b><br> 6230 * </p> 6231 */ 6232 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6233 SP_COMPOSED_OF); 6234 6235 /** 6236 * Constant for fluent queries to be used to add include statements. Specifies 6237 * the path value of "<b>ActivityDefinition:composed-of</b>". 6238 */ 6239 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 6240 "ActivityDefinition:composed-of").toLocked(); 6241 6242 /** 6243 * Search parameter: <b>version</b> 6244 * <p> 6245 * Description: <b>The business version of the activity definition</b><br> 6246 * Type: <b>token</b><br> 6247 * Path: <b>ActivityDefinition.version</b><br> 6248 * </p> 6249 */ 6250 @SearchParamDefinition(name = "version", path = "ActivityDefinition.version", description = "The business version of the activity definition", type = "token") 6251 public static final String SP_VERSION = "version"; 6252 /** 6253 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6254 * <p> 6255 * Description: <b>The business version of the activity definition</b><br> 6256 * Type: <b>token</b><br> 6257 * Path: <b>ActivityDefinition.version</b><br> 6258 * </p> 6259 */ 6260 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6261 SP_VERSION); 6262 6263 /** 6264 * Search parameter: <b>url</b> 6265 * <p> 6266 * Description: <b>The uri that identifies the activity definition</b><br> 6267 * Type: <b>uri</b><br> 6268 * Path: <b>ActivityDefinition.url</b><br> 6269 * </p> 6270 */ 6271 @SearchParamDefinition(name = "url", path = "ActivityDefinition.url", description = "The uri that identifies the activity definition", type = "uri") 6272 public static final String SP_URL = "url"; 6273 /** 6274 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6275 * <p> 6276 * Description: <b>The uri that identifies the activity definition</b><br> 6277 * Type: <b>uri</b><br> 6278 * Path: <b>ActivityDefinition.url</b><br> 6279 * </p> 6280 */ 6281 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6282 6283 /** 6284 * Search parameter: <b>context-quantity</b> 6285 * <p> 6286 * Description: <b>A quantity- or range-valued use context assigned to the 6287 * activity definition</b><br> 6288 * Type: <b>quantity</b><br> 6289 * Path: <b>ActivityDefinition.useContext.valueQuantity, 6290 * ActivityDefinition.useContext.valueRange</b><br> 6291 * </p> 6292 */ 6293 @SearchParamDefinition(name = "context-quantity", path = "(ActivityDefinition.useContext.value as Quantity) | (ActivityDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the activity definition", type = "quantity") 6294 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6295 /** 6296 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6297 * <p> 6298 * Description: <b>A quantity- or range-valued use context assigned to the 6299 * activity definition</b><br> 6300 * Type: <b>quantity</b><br> 6301 * Path: <b>ActivityDefinition.useContext.valueQuantity, 6302 * ActivityDefinition.useContext.valueRange</b><br> 6303 * </p> 6304 */ 6305 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6306 SP_CONTEXT_QUANTITY); 6307 6308 /** 6309 * Search parameter: <b>effective</b> 6310 * <p> 6311 * Description: <b>The time during which the activity definition is intended to 6312 * be in use</b><br> 6313 * Type: <b>date</b><br> 6314 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 6315 * </p> 6316 */ 6317 @SearchParamDefinition(name = "effective", path = "ActivityDefinition.effectivePeriod", description = "The time during which the activity definition is intended to be in use", type = "date") 6318 public static final String SP_EFFECTIVE = "effective"; 6319 /** 6320 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6321 * <p> 6322 * Description: <b>The time during which the activity definition is intended to 6323 * be in use</b><br> 6324 * Type: <b>date</b><br> 6325 * Path: <b>ActivityDefinition.effectivePeriod</b><br> 6326 * </p> 6327 */ 6328 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6329 SP_EFFECTIVE); 6330 6331 /** 6332 * Search parameter: <b>depends-on</b> 6333 * <p> 6334 * Description: <b>What resource is being referenced</b><br> 6335 * Type: <b>reference</b><br> 6336 * Path: <b>ActivityDefinition.relatedArtifact.resource, 6337 * ActivityDefinition.library</b><br> 6338 * </p> 6339 */ 6340 @SearchParamDefinition(name = "depends-on", path = "ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library", description = "What resource is being referenced", type = "reference") 6341 public static final String SP_DEPENDS_ON = "depends-on"; 6342 /** 6343 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6344 * <p> 6345 * Description: <b>What resource is being referenced</b><br> 6346 * Type: <b>reference</b><br> 6347 * Path: <b>ActivityDefinition.relatedArtifact.resource, 6348 * ActivityDefinition.library</b><br> 6349 * </p> 6350 */ 6351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6352 SP_DEPENDS_ON); 6353 6354 /** 6355 * Constant for fluent queries to be used to add include statements. Specifies 6356 * the path value of "<b>ActivityDefinition:depends-on</b>". 6357 */ 6358 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 6359 "ActivityDefinition:depends-on").toLocked(); 6360 6361 /** 6362 * Search parameter: <b>name</b> 6363 * <p> 6364 * Description: <b>Computationally friendly name of the activity 6365 * definition</b><br> 6366 * Type: <b>string</b><br> 6367 * Path: <b>ActivityDefinition.name</b><br> 6368 * </p> 6369 */ 6370 @SearchParamDefinition(name = "name", path = "ActivityDefinition.name", description = "Computationally friendly name of the activity definition", type = "string") 6371 public static final String SP_NAME = "name"; 6372 /** 6373 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6374 * <p> 6375 * Description: <b>Computationally friendly name of the activity 6376 * definition</b><br> 6377 * Type: <b>string</b><br> 6378 * Path: <b>ActivityDefinition.name</b><br> 6379 * </p> 6380 */ 6381 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6382 SP_NAME); 6383 6384 /** 6385 * Search parameter: <b>context</b> 6386 * <p> 6387 * Description: <b>A use context assigned to the activity definition</b><br> 6388 * Type: <b>token</b><br> 6389 * Path: <b>ActivityDefinition.useContext.valueCodeableConcept</b><br> 6390 * </p> 6391 */ 6392 @SearchParamDefinition(name = "context", path = "(ActivityDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the activity definition", type = "token") 6393 public static final String SP_CONTEXT = "context"; 6394 /** 6395 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6396 * <p> 6397 * Description: <b>A use context assigned to the activity definition</b><br> 6398 * Type: <b>token</b><br> 6399 * Path: <b>ActivityDefinition.useContext.valueCodeableConcept</b><br> 6400 * </p> 6401 */ 6402 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6403 SP_CONTEXT); 6404 6405 /** 6406 * Search parameter: <b>publisher</b> 6407 * <p> 6408 * Description: <b>Name of the publisher of the activity definition</b><br> 6409 * Type: <b>string</b><br> 6410 * Path: <b>ActivityDefinition.publisher</b><br> 6411 * </p> 6412 */ 6413 @SearchParamDefinition(name = "publisher", path = "ActivityDefinition.publisher", description = "Name of the publisher of the activity definition", type = "string") 6414 public static final String SP_PUBLISHER = "publisher"; 6415 /** 6416 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6417 * <p> 6418 * Description: <b>Name of the publisher of the activity definition</b><br> 6419 * Type: <b>string</b><br> 6420 * Path: <b>ActivityDefinition.publisher</b><br> 6421 * </p> 6422 */ 6423 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6424 SP_PUBLISHER); 6425 6426 /** 6427 * Search parameter: <b>topic</b> 6428 * <p> 6429 * Description: <b>Topics associated with the module</b><br> 6430 * Type: <b>token</b><br> 6431 * Path: <b>ActivityDefinition.topic</b><br> 6432 * </p> 6433 */ 6434 @SearchParamDefinition(name = "topic", path = "ActivityDefinition.topic", description = "Topics associated with the module", type = "token") 6435 public static final String SP_TOPIC = "topic"; 6436 /** 6437 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6438 * <p> 6439 * Description: <b>Topics associated with the module</b><br> 6440 * Type: <b>token</b><br> 6441 * Path: <b>ActivityDefinition.topic</b><br> 6442 * </p> 6443 */ 6444 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6445 SP_TOPIC); 6446 6447 /** 6448 * Search parameter: <b>context-type-quantity</b> 6449 * <p> 6450 * Description: <b>A use context type and quantity- or range-based value 6451 * assigned to the activity definition</b><br> 6452 * Type: <b>composite</b><br> 6453 * Path: <b></b><br> 6454 * </p> 6455 */ 6456 @SearchParamDefinition(name = "context-type-quantity", path = "ActivityDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the activity definition", type = "composite", compositeOf = { 6457 "context-type", "context-quantity" }) 6458 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6459 /** 6460 * <b>Fluent Client</b> search parameter constant for 6461 * <b>context-type-quantity</b> 6462 * <p> 6463 * Description: <b>A use context type and quantity- or range-based value 6464 * assigned to the activity definition</b><br> 6465 * Type: <b>composite</b><br> 6466 * Path: <b></b><br> 6467 * </p> 6468 */ 6469 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6470 SP_CONTEXT_TYPE_QUANTITY); 6471 6472 /** 6473 * Search parameter: <b>status</b> 6474 * <p> 6475 * Description: <b>The current status of the activity definition</b><br> 6476 * Type: <b>token</b><br> 6477 * Path: <b>ActivityDefinition.status</b><br> 6478 * </p> 6479 */ 6480 @SearchParamDefinition(name = "status", path = "ActivityDefinition.status", description = "The current status of the activity definition", type = "token") 6481 public static final String SP_STATUS = "status"; 6482 /** 6483 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6484 * <p> 6485 * Description: <b>The current status of the activity definition</b><br> 6486 * Type: <b>token</b><br> 6487 * Path: <b>ActivityDefinition.status</b><br> 6488 * </p> 6489 */ 6490 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6491 SP_STATUS); 6492 6493}