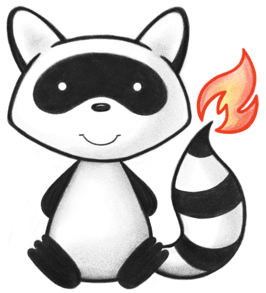
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Actual or potential/avoided event causing unintended physical injury 049 * resulting from or contributed to by medical care, a research study or other 050 * healthcare setting factors that requires additional monitoring, treatment, or 051 * hospitalization, or that results in death. 052 */ 053@ResourceDef(name = "AdverseEvent", profile = "http://hl7.org/fhir/StructureDefinition/AdverseEvent") 054public class AdverseEvent extends DomainResource { 055 056 public enum AdverseEventActuality { 057 /** 058 * The adverse event actually happened regardless of whether anyone was affected 059 * or harmed. 060 */ 061 ACTUAL, 062 /** 063 * A potential adverse event. 064 */ 065 POTENTIAL, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 071 public static AdverseEventActuality fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("actual".equals(codeString)) 075 return ACTUAL; 076 if ("potential".equals(codeString)) 077 return POTENTIAL; 078 if (Configuration.isAcceptInvalidEnums()) 079 return null; 080 else 081 throw new FHIRException("Unknown AdverseEventActuality code '" + codeString + "'"); 082 } 083 084 public String toCode() { 085 switch (this) { 086 case ACTUAL: 087 return "actual"; 088 case POTENTIAL: 089 return "potential"; 090 case NULL: 091 return null; 092 default: 093 return "?"; 094 } 095 } 096 097 public String getSystem() { 098 switch (this) { 099 case ACTUAL: 100 return "http://hl7.org/fhir/adverse-event-actuality"; 101 case POTENTIAL: 102 return "http://hl7.org/fhir/adverse-event-actuality"; 103 case NULL: 104 return null; 105 default: 106 return "?"; 107 } 108 } 109 110 public String getDefinition() { 111 switch (this) { 112 case ACTUAL: 113 return "The adverse event actually happened regardless of whether anyone was affected or harmed."; 114 case POTENTIAL: 115 return "A potential adverse event."; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDisplay() { 124 switch (this) { 125 case ACTUAL: 126 return "Adverse Event"; 127 case POTENTIAL: 128 return "Potential Adverse Event"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 } 136 137 public static class AdverseEventActualityEnumFactory implements EnumFactory<AdverseEventActuality> { 138 public AdverseEventActuality fromCode(String codeString) throws IllegalArgumentException { 139 if (codeString == null || "".equals(codeString)) 140 if (codeString == null || "".equals(codeString)) 141 return null; 142 if ("actual".equals(codeString)) 143 return AdverseEventActuality.ACTUAL; 144 if ("potential".equals(codeString)) 145 return AdverseEventActuality.POTENTIAL; 146 throw new IllegalArgumentException("Unknown AdverseEventActuality code '" + codeString + "'"); 147 } 148 149 public Enumeration<AdverseEventActuality> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.NULL, code); 157 if ("actual".equals(codeString)) 158 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.ACTUAL, code); 159 if ("potential".equals(codeString)) 160 return new Enumeration<AdverseEventActuality>(this, AdverseEventActuality.POTENTIAL, code); 161 throw new FHIRException("Unknown AdverseEventActuality code '" + codeString + "'"); 162 } 163 164 public String toCode(AdverseEventActuality code) { 165 if (code == AdverseEventActuality.NULL) 166 return null; 167 if (code == AdverseEventActuality.ACTUAL) 168 return "actual"; 169 if (code == AdverseEventActuality.POTENTIAL) 170 return "potential"; 171 return "?"; 172 } 173 174 public String toSystem(AdverseEventActuality code) { 175 return code.getSystem(); 176 } 177 } 178 179 @Block() 180 public static class AdverseEventSuspectEntityComponent extends BackboneElement implements IBaseBackboneElement { 181 /** 182 * Identifies the actual instance of what caused the adverse event. May be a 183 * substance, medication, medication administration, medication statement or a 184 * device. 185 */ 186 @Child(name = "instance", type = { Immunization.class, Procedure.class, Substance.class, Medication.class, 187 MedicationAdministration.class, MedicationStatement.class, 188 Device.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 189 @Description(shortDefinition = "Refers to the specific entity that caused the adverse event", formalDefinition = "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.") 190 protected Reference instance; 191 192 /** 193 * The actual object that is the target of the reference (Identifies the actual 194 * instance of what caused the adverse event. May be a substance, medication, 195 * medication administration, medication statement or a device.) 196 */ 197 protected Resource instanceTarget; 198 199 /** 200 * Information on the possible cause of the event. 201 */ 202 @Child(name = "causality", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 203 @Description(shortDefinition = "Information on the possible cause of the event", formalDefinition = "Information on the possible cause of the event.") 204 protected List<AdverseEventSuspectEntityCausalityComponent> causality; 205 206 private static final long serialVersionUID = 1245759325L; 207 208 /** 209 * Constructor 210 */ 211 public AdverseEventSuspectEntityComponent() { 212 super(); 213 } 214 215 /** 216 * Constructor 217 */ 218 public AdverseEventSuspectEntityComponent(Reference instance) { 219 super(); 220 this.instance = instance; 221 } 222 223 /** 224 * @return {@link #instance} (Identifies the actual instance of what caused the 225 * adverse event. May be a substance, medication, medication 226 * administration, medication statement or a device.) 227 */ 228 public Reference getInstance() { 229 if (this.instance == null) 230 if (Configuration.errorOnAutoCreate()) 231 throw new Error("Attempt to auto-create AdverseEventSuspectEntityComponent.instance"); 232 else if (Configuration.doAutoCreate()) 233 this.instance = new Reference(); // cc 234 return this.instance; 235 } 236 237 public boolean hasInstance() { 238 return this.instance != null && !this.instance.isEmpty(); 239 } 240 241 /** 242 * @param value {@link #instance} (Identifies the actual instance of what caused 243 * the adverse event. May be a substance, medication, medication 244 * administration, medication statement or a device.) 245 */ 246 public AdverseEventSuspectEntityComponent setInstance(Reference value) { 247 this.instance = value; 248 return this; 249 } 250 251 /** 252 * @return {@link #instance} The actual object that is the target of the 253 * reference. The reference library doesn't populate this, but you can 254 * use it to hold the resource if you resolve it. (Identifies the actual 255 * instance of what caused the adverse event. May be a substance, 256 * medication, medication administration, medication statement or a 257 * device.) 258 */ 259 public Resource getInstanceTarget() { 260 return this.instanceTarget; 261 } 262 263 /** 264 * @param value {@link #instance} The actual object that is the target of the 265 * reference. The reference library doesn't use these, but you can 266 * use it to hold the resource if you resolve it. (Identifies the 267 * actual instance of what caused the adverse event. May be a 268 * substance, medication, medication administration, medication 269 * statement or a device.) 270 */ 271 public AdverseEventSuspectEntityComponent setInstanceTarget(Resource value) { 272 this.instanceTarget = value; 273 return this; 274 } 275 276 /** 277 * @return {@link #causality} (Information on the possible cause of the event.) 278 */ 279 public List<AdverseEventSuspectEntityCausalityComponent> getCausality() { 280 if (this.causality == null) 281 this.causality = new ArrayList<AdverseEventSuspectEntityCausalityComponent>(); 282 return this.causality; 283 } 284 285 /** 286 * @return Returns a reference to <code>this</code> for easy method chaining 287 */ 288 public AdverseEventSuspectEntityComponent setCausality( 289 List<AdverseEventSuspectEntityCausalityComponent> theCausality) { 290 this.causality = theCausality; 291 return this; 292 } 293 294 public boolean hasCausality() { 295 if (this.causality == null) 296 return false; 297 for (AdverseEventSuspectEntityCausalityComponent item : this.causality) 298 if (!item.isEmpty()) 299 return true; 300 return false; 301 } 302 303 public AdverseEventSuspectEntityCausalityComponent addCausality() { // 3 304 AdverseEventSuspectEntityCausalityComponent t = new AdverseEventSuspectEntityCausalityComponent(); 305 if (this.causality == null) 306 this.causality = new ArrayList<AdverseEventSuspectEntityCausalityComponent>(); 307 this.causality.add(t); 308 return t; 309 } 310 311 public AdverseEventSuspectEntityComponent addCausality(AdverseEventSuspectEntityCausalityComponent t) { // 3 312 if (t == null) 313 return this; 314 if (this.causality == null) 315 this.causality = new ArrayList<AdverseEventSuspectEntityCausalityComponent>(); 316 this.causality.add(t); 317 return this; 318 } 319 320 /** 321 * @return The first repetition of repeating field {@link #causality}, creating 322 * it if it does not already exist 323 */ 324 public AdverseEventSuspectEntityCausalityComponent getCausalityFirstRep() { 325 if (getCausality().isEmpty()) { 326 addCausality(); 327 } 328 return getCausality().get(0); 329 } 330 331 protected void listChildren(List<Property> children) { 332 super.listChildren(children); 333 children.add(new Property("instance", 334 "Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device)", 335 "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 336 0, 1, instance)); 337 children.add(new Property("causality", "", "Information on the possible cause of the event.", 0, 338 java.lang.Integer.MAX_VALUE, causality)); 339 } 340 341 @Override 342 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 343 switch (_hash) { 344 case 555127957: 345 /* instance */ return new Property("instance", 346 "Reference(Immunization|Procedure|Substance|Medication|MedicationAdministration|MedicationStatement|Device)", 347 "Identifies the actual instance of what caused the adverse event. May be a substance, medication, medication administration, medication statement or a device.", 348 0, 1, instance); 349 case -1446450521: 350 /* causality */ return new Property("causality", "", "Information on the possible cause of the event.", 0, 351 java.lang.Integer.MAX_VALUE, causality); 352 default: 353 return super.getNamedProperty(_hash, _name, _checkValid); 354 } 355 356 } 357 358 @Override 359 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 360 switch (hash) { 361 case 555127957: 362 /* instance */ return this.instance == null ? new Base[0] : new Base[] { this.instance }; // Reference 363 case -1446450521: 364 /* causality */ return this.causality == null ? new Base[0] 365 : this.causality.toArray(new Base[this.causality.size()]); // AdverseEventSuspectEntityCausalityComponent 366 default: 367 return super.getProperty(hash, name, checkValid); 368 } 369 370 } 371 372 @Override 373 public Base setProperty(int hash, String name, Base value) throws FHIRException { 374 switch (hash) { 375 case 555127957: // instance 376 this.instance = castToReference(value); // Reference 377 return value; 378 case -1446450521: // causality 379 this.getCausality().add((AdverseEventSuspectEntityCausalityComponent) value); // AdverseEventSuspectEntityCausalityComponent 380 return value; 381 default: 382 return super.setProperty(hash, name, value); 383 } 384 385 } 386 387 @Override 388 public Base setProperty(String name, Base value) throws FHIRException { 389 if (name.equals("instance")) { 390 this.instance = castToReference(value); // Reference 391 } else if (name.equals("causality")) { 392 this.getCausality().add((AdverseEventSuspectEntityCausalityComponent) value); 393 } else 394 return super.setProperty(name, value); 395 return value; 396 } 397 398 @Override 399 public void removeChild(String name, Base value) throws FHIRException { 400 if (name.equals("instance")) { 401 this.instance = null; 402 } else if (name.equals("causality")) { 403 this.getCausality().remove((AdverseEventSuspectEntityCausalityComponent) value); 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case 555127957: 413 return getInstance(); 414 case -1446450521: 415 return addCausality(); 416 default: 417 return super.makeProperty(hash, name); 418 } 419 420 } 421 422 @Override 423 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 424 switch (hash) { 425 case 555127957: 426 /* instance */ return new String[] { "Reference" }; 427 case -1446450521: 428 /* causality */ return new String[] {}; 429 default: 430 return super.getTypesForProperty(hash, name); 431 } 432 433 } 434 435 @Override 436 public Base addChild(String name) throws FHIRException { 437 if (name.equals("instance")) { 438 this.instance = new Reference(); 439 return this.instance; 440 } else if (name.equals("causality")) { 441 return addCausality(); 442 } else 443 return super.addChild(name); 444 } 445 446 public AdverseEventSuspectEntityComponent copy() { 447 AdverseEventSuspectEntityComponent dst = new AdverseEventSuspectEntityComponent(); 448 copyValues(dst); 449 return dst; 450 } 451 452 public void copyValues(AdverseEventSuspectEntityComponent dst) { 453 super.copyValues(dst); 454 dst.instance = instance == null ? null : instance.copy(); 455 if (causality != null) { 456 dst.causality = new ArrayList<AdverseEventSuspectEntityCausalityComponent>(); 457 for (AdverseEventSuspectEntityCausalityComponent i : causality) 458 dst.causality.add(i.copy()); 459 } 460 ; 461 } 462 463 @Override 464 public boolean equalsDeep(Base other_) { 465 if (!super.equalsDeep(other_)) 466 return false; 467 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 468 return false; 469 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 470 return compareDeep(instance, o.instance, true) && compareDeep(causality, o.causality, true); 471 } 472 473 @Override 474 public boolean equalsShallow(Base other_) { 475 if (!super.equalsShallow(other_)) 476 return false; 477 if (!(other_ instanceof AdverseEventSuspectEntityComponent)) 478 return false; 479 AdverseEventSuspectEntityComponent o = (AdverseEventSuspectEntityComponent) other_; 480 return true; 481 } 482 483 public boolean isEmpty() { 484 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instance, causality); 485 } 486 487 public String fhirType() { 488 return "AdverseEvent.suspectEntity"; 489 490 } 491 492 } 493 494 @Block() 495 public static class AdverseEventSuspectEntityCausalityComponent extends BackboneElement 496 implements IBaseBackboneElement { 497 /** 498 * Assessment of if the entity caused the event. 499 */ 500 @Child(name = "assessment", type = { 501 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 502 @Description(shortDefinition = "Assessment of if the entity caused the event", formalDefinition = "Assessment of if the entity caused the event.") 503 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-causality-assess") 504 protected CodeableConcept assessment; 505 506 /** 507 * AdverseEvent.suspectEntity.causalityProductRelatedness. 508 */ 509 @Child(name = "productRelatedness", type = { 510 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 511 @Description(shortDefinition = "AdverseEvent.suspectEntity.causalityProductRelatedness", formalDefinition = "AdverseEvent.suspectEntity.causalityProductRelatedness.") 512 protected StringType productRelatedness; 513 514 /** 515 * AdverseEvent.suspectEntity.causalityAuthor. 516 */ 517 @Child(name = "author", type = { Practitioner.class, 518 PractitionerRole.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 519 @Description(shortDefinition = "AdverseEvent.suspectEntity.causalityAuthor", formalDefinition = "AdverseEvent.suspectEntity.causalityAuthor.") 520 protected Reference author; 521 522 /** 523 * The actual object that is the target of the reference 524 * (AdverseEvent.suspectEntity.causalityAuthor.) 525 */ 526 protected Resource authorTarget; 527 528 /** 529 * ProbabilityScale | Bayesian | Checklist. 530 */ 531 @Child(name = "method", type = { 532 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 533 @Description(shortDefinition = "ProbabilityScale | Bayesian | Checklist", formalDefinition = "ProbabilityScale | Bayesian | Checklist.") 534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-causality-method") 535 protected CodeableConcept method; 536 537 private static final long serialVersionUID = -1847234837L; 538 539 /** 540 * Constructor 541 */ 542 public AdverseEventSuspectEntityCausalityComponent() { 543 super(); 544 } 545 546 /** 547 * @return {@link #assessment} (Assessment of if the entity caused the event.) 548 */ 549 public CodeableConcept getAssessment() { 550 if (this.assessment == null) 551 if (Configuration.errorOnAutoCreate()) 552 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.assessment"); 553 else if (Configuration.doAutoCreate()) 554 this.assessment = new CodeableConcept(); // cc 555 return this.assessment; 556 } 557 558 public boolean hasAssessment() { 559 return this.assessment != null && !this.assessment.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #assessment} (Assessment of if the entity caused the 564 * event.) 565 */ 566 public AdverseEventSuspectEntityCausalityComponent setAssessment(CodeableConcept value) { 567 this.assessment = value; 568 return this; 569 } 570 571 /** 572 * @return {@link #productRelatedness} 573 * (AdverseEvent.suspectEntity.causalityProductRelatedness.). This is 574 * the underlying object with id, value and extensions. The accessor 575 * "getProductRelatedness" gives direct access to the value 576 */ 577 public StringType getProductRelatednessElement() { 578 if (this.productRelatedness == null) 579 if (Configuration.errorOnAutoCreate()) 580 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.productRelatedness"); 581 else if (Configuration.doAutoCreate()) 582 this.productRelatedness = new StringType(); // bb 583 return this.productRelatedness; 584 } 585 586 public boolean hasProductRelatednessElement() { 587 return this.productRelatedness != null && !this.productRelatedness.isEmpty(); 588 } 589 590 public boolean hasProductRelatedness() { 591 return this.productRelatedness != null && !this.productRelatedness.isEmpty(); 592 } 593 594 /** 595 * @param value {@link #productRelatedness} 596 * (AdverseEvent.suspectEntity.causalityProductRelatedness.). This 597 * is the underlying object with id, value and extensions. The 598 * accessor "getProductRelatedness" gives direct access to the 599 * value 600 */ 601 public AdverseEventSuspectEntityCausalityComponent setProductRelatednessElement(StringType value) { 602 this.productRelatedness = value; 603 return this; 604 } 605 606 /** 607 * @return AdverseEvent.suspectEntity.causalityProductRelatedness. 608 */ 609 public String getProductRelatedness() { 610 return this.productRelatedness == null ? null : this.productRelatedness.getValue(); 611 } 612 613 /** 614 * @param value AdverseEvent.suspectEntity.causalityProductRelatedness. 615 */ 616 public AdverseEventSuspectEntityCausalityComponent setProductRelatedness(String value) { 617 if (Utilities.noString(value)) 618 this.productRelatedness = null; 619 else { 620 if (this.productRelatedness == null) 621 this.productRelatedness = new StringType(); 622 this.productRelatedness.setValue(value); 623 } 624 return this; 625 } 626 627 /** 628 * @return {@link #author} (AdverseEvent.suspectEntity.causalityAuthor.) 629 */ 630 public Reference getAuthor() { 631 if (this.author == null) 632 if (Configuration.errorOnAutoCreate()) 633 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.author"); 634 else if (Configuration.doAutoCreate()) 635 this.author = new Reference(); // cc 636 return this.author; 637 } 638 639 public boolean hasAuthor() { 640 return this.author != null && !this.author.isEmpty(); 641 } 642 643 /** 644 * @param value {@link #author} (AdverseEvent.suspectEntity.causalityAuthor.) 645 */ 646 public AdverseEventSuspectEntityCausalityComponent setAuthor(Reference value) { 647 this.author = value; 648 return this; 649 } 650 651 /** 652 * @return {@link #author} The actual object that is the target of the 653 * reference. The reference library doesn't populate this, but you can 654 * use it to hold the resource if you resolve it. 655 * (AdverseEvent.suspectEntity.causalityAuthor.) 656 */ 657 public Resource getAuthorTarget() { 658 return this.authorTarget; 659 } 660 661 /** 662 * @param value {@link #author} The actual object that is the target of the 663 * reference. The reference library doesn't use these, but you can 664 * use it to hold the resource if you resolve it. 665 * (AdverseEvent.suspectEntity.causalityAuthor.) 666 */ 667 public AdverseEventSuspectEntityCausalityComponent setAuthorTarget(Resource value) { 668 this.authorTarget = value; 669 return this; 670 } 671 672 /** 673 * @return {@link #method} (ProbabilityScale | Bayesian | Checklist.) 674 */ 675 public CodeableConcept getMethod() { 676 if (this.method == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create AdverseEventSuspectEntityCausalityComponent.method"); 679 else if (Configuration.doAutoCreate()) 680 this.method = new CodeableConcept(); // cc 681 return this.method; 682 } 683 684 public boolean hasMethod() { 685 return this.method != null && !this.method.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #method} (ProbabilityScale | Bayesian | Checklist.) 690 */ 691 public AdverseEventSuspectEntityCausalityComponent setMethod(CodeableConcept value) { 692 this.method = value; 693 return this; 694 } 695 696 protected void listChildren(List<Property> children) { 697 super.listChildren(children); 698 children.add(new Property("assessment", "CodeableConcept", "Assessment of if the entity caused the event.", 0, 1, 699 assessment)); 700 children.add(new Property("productRelatedness", "string", 701 "AdverseEvent.suspectEntity.causalityProductRelatedness.", 0, 1, productRelatedness)); 702 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", 703 "AdverseEvent.suspectEntity.causalityAuthor.", 0, 1, author)); 704 children.add(new Property("method", "CodeableConcept", "ProbabilityScale | Bayesian | Checklist.", 0, 1, method)); 705 } 706 707 @Override 708 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 709 switch (_hash) { 710 case 2119382722: 711 /* assessment */ return new Property("assessment", "CodeableConcept", 712 "Assessment of if the entity caused the event.", 0, 1, assessment); 713 case 1824577683: 714 /* productRelatedness */ return new Property("productRelatedness", "string", 715 "AdverseEvent.suspectEntity.causalityProductRelatedness.", 0, 1, productRelatedness); 716 case -1406328437: 717 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole)", 718 "AdverseEvent.suspectEntity.causalityAuthor.", 0, 1, author); 719 case -1077554975: 720 /* method */ return new Property("method", "CodeableConcept", "ProbabilityScale | Bayesian | Checklist.", 0, 1, 721 method); 722 default: 723 return super.getNamedProperty(_hash, _name, _checkValid); 724 } 725 726 } 727 728 @Override 729 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 730 switch (hash) { 731 case 2119382722: 732 /* assessment */ return this.assessment == null ? new Base[0] : new Base[] { this.assessment }; // CodeableConcept 733 case 1824577683: 734 /* productRelatedness */ return this.productRelatedness == null ? new Base[0] 735 : new Base[] { this.productRelatedness }; // StringType 736 case -1406328437: 737 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 738 case -1077554975: 739 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 740 default: 741 return super.getProperty(hash, name, checkValid); 742 } 743 744 } 745 746 @Override 747 public Base setProperty(int hash, String name, Base value) throws FHIRException { 748 switch (hash) { 749 case 2119382722: // assessment 750 this.assessment = castToCodeableConcept(value); // CodeableConcept 751 return value; 752 case 1824577683: // productRelatedness 753 this.productRelatedness = castToString(value); // StringType 754 return value; 755 case -1406328437: // author 756 this.author = castToReference(value); // Reference 757 return value; 758 case -1077554975: // method 759 this.method = castToCodeableConcept(value); // CodeableConcept 760 return value; 761 default: 762 return super.setProperty(hash, name, value); 763 } 764 765 } 766 767 @Override 768 public Base setProperty(String name, Base value) throws FHIRException { 769 if (name.equals("assessment")) { 770 this.assessment = castToCodeableConcept(value); // CodeableConcept 771 } else if (name.equals("productRelatedness")) { 772 this.productRelatedness = castToString(value); // StringType 773 } else if (name.equals("author")) { 774 this.author = castToReference(value); // Reference 775 } else if (name.equals("method")) { 776 this.method = castToCodeableConcept(value); // CodeableConcept 777 } else 778 return super.setProperty(name, value); 779 return value; 780 } 781 782 @Override 783 public void removeChild(String name, Base value) throws FHIRException { 784 if (name.equals("assessment")) { 785 this.assessment = null; 786 } else if (name.equals("productRelatedness")) { 787 this.productRelatedness = null; 788 } else if (name.equals("author")) { 789 this.author = null; 790 } else if (name.equals("method")) { 791 this.method = null; 792 } else 793 super.removeChild(name, value); 794 795 } 796 797 @Override 798 public Base makeProperty(int hash, String name) throws FHIRException { 799 switch (hash) { 800 case 2119382722: 801 return getAssessment(); 802 case 1824577683: 803 return getProductRelatednessElement(); 804 case -1406328437: 805 return getAuthor(); 806 case -1077554975: 807 return getMethod(); 808 default: 809 return super.makeProperty(hash, name); 810 } 811 812 } 813 814 @Override 815 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 816 switch (hash) { 817 case 2119382722: 818 /* assessment */ return new String[] { "CodeableConcept" }; 819 case 1824577683: 820 /* productRelatedness */ return new String[] { "string" }; 821 case -1406328437: 822 /* author */ return new String[] { "Reference" }; 823 case -1077554975: 824 /* method */ return new String[] { "CodeableConcept" }; 825 default: 826 return super.getTypesForProperty(hash, name); 827 } 828 829 } 830 831 @Override 832 public Base addChild(String name) throws FHIRException { 833 if (name.equals("assessment")) { 834 this.assessment = new CodeableConcept(); 835 return this.assessment; 836 } else if (name.equals("productRelatedness")) { 837 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.productRelatedness"); 838 } else if (name.equals("author")) { 839 this.author = new Reference(); 840 return this.author; 841 } else if (name.equals("method")) { 842 this.method = new CodeableConcept(); 843 return this.method; 844 } else 845 return super.addChild(name); 846 } 847 848 public AdverseEventSuspectEntityCausalityComponent copy() { 849 AdverseEventSuspectEntityCausalityComponent dst = new AdverseEventSuspectEntityCausalityComponent(); 850 copyValues(dst); 851 return dst; 852 } 853 854 public void copyValues(AdverseEventSuspectEntityCausalityComponent dst) { 855 super.copyValues(dst); 856 dst.assessment = assessment == null ? null : assessment.copy(); 857 dst.productRelatedness = productRelatedness == null ? null : productRelatedness.copy(); 858 dst.author = author == null ? null : author.copy(); 859 dst.method = method == null ? null : method.copy(); 860 } 861 862 @Override 863 public boolean equalsDeep(Base other_) { 864 if (!super.equalsDeep(other_)) 865 return false; 866 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 867 return false; 868 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 869 return compareDeep(assessment, o.assessment, true) && compareDeep(productRelatedness, o.productRelatedness, true) 870 && compareDeep(author, o.author, true) && compareDeep(method, o.method, true); 871 } 872 873 @Override 874 public boolean equalsShallow(Base other_) { 875 if (!super.equalsShallow(other_)) 876 return false; 877 if (!(other_ instanceof AdverseEventSuspectEntityCausalityComponent)) 878 return false; 879 AdverseEventSuspectEntityCausalityComponent o = (AdverseEventSuspectEntityCausalityComponent) other_; 880 return compareValues(productRelatedness, o.productRelatedness, true); 881 } 882 883 public boolean isEmpty() { 884 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(assessment, productRelatedness, author, method); 885 } 886 887 public String fhirType() { 888 return "AdverseEvent.suspectEntity.causality"; 889 890 } 891 892 } 893 894 /** 895 * Business identifiers assigned to this adverse event by the performer or other 896 * systems which remain constant as the resource is updated and propagates from 897 * server to server. 898 */ 899 @Child(name = "identifier", type = { 900 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 901 @Description(shortDefinition = "Business identifier for the event", formalDefinition = "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 902 protected Identifier identifier; 903 904 /** 905 * Whether the event actually happened, or just had the potential to. Note that 906 * this is independent of whether anyone was affected or harmed or how severely. 907 */ 908 @Child(name = "actuality", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 909 @Description(shortDefinition = "actual | potential", formalDefinition = "Whether the event actually happened, or just had the potential to. Note that this is independent of whether anyone was affected or harmed or how severely.") 910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-actuality") 911 protected Enumeration<AdverseEventActuality> actuality; 912 913 /** 914 * The overall type of event, intended for search and filtering purposes. 915 */ 916 @Child(name = "category", type = { 917 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 918 @Description(shortDefinition = "product-problem | product-quality | product-use-error | wrong-dose | incorrect-prescribing-information | wrong-technique | wrong-route-of-administration | wrong-rate | wrong-duration | wrong-time | expired-drug | medical-device-use-error | problem-different-manufacturer | unsafe-physical-environment", formalDefinition = "The overall type of event, intended for search and filtering purposes.") 919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-category") 920 protected List<CodeableConcept> category; 921 922 /** 923 * This element defines the specific type of event that occurred or that was 924 * prevented from occurring. 925 */ 926 @Child(name = "event", type = { 927 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 928 @Description(shortDefinition = "Type of the event itself in relation to the subject", formalDefinition = "This element defines the specific type of event that occurred or that was prevented from occurring.") 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-type") 930 protected CodeableConcept event; 931 932 /** 933 * This subject or group impacted by the event. 934 */ 935 @Child(name = "subject", type = { Patient.class, Group.class, Practitioner.class, 936 RelatedPerson.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 937 @Description(shortDefinition = "Subject impacted by event", formalDefinition = "This subject or group impacted by the event.") 938 protected Reference subject; 939 940 /** 941 * The actual object that is the target of the reference (This subject or group 942 * impacted by the event.) 943 */ 944 protected Resource subjectTarget; 945 946 /** 947 * The Encounter during which AdverseEvent was created or to which the creation 948 * of this record is tightly associated. 949 */ 950 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 951 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which AdverseEvent was created or to which the creation of this record is tightly associated.") 952 protected Reference encounter; 953 954 /** 955 * The actual object that is the target of the reference (The Encounter during 956 * which AdverseEvent was created or to which the creation of this record is 957 * tightly associated.) 958 */ 959 protected Encounter encounterTarget; 960 961 /** 962 * The date (and perhaps time) when the adverse event occurred. 963 */ 964 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 965 @Description(shortDefinition = "When the event occurred", formalDefinition = "The date (and perhaps time) when the adverse event occurred.") 966 protected DateTimeType date; 967 968 /** 969 * Estimated or actual date the AdverseEvent began, in the opinion of the 970 * reporter. 971 */ 972 @Child(name = "detected", type = { 973 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 974 @Description(shortDefinition = "When the event was detected", formalDefinition = "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.") 975 protected DateTimeType detected; 976 977 /** 978 * The date on which the existence of the AdverseEvent was first recorded. 979 */ 980 @Child(name = "recordedDate", type = { 981 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 982 @Description(shortDefinition = "When the event was recorded", formalDefinition = "The date on which the existence of the AdverseEvent was first recorded.") 983 protected DateTimeType recordedDate; 984 985 /** 986 * Includes information about the reaction that occurred as a result of exposure 987 * to a substance (for example, a drug or a chemical). 988 */ 989 @Child(name = "resultingCondition", type = { 990 Condition.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 991 @Description(shortDefinition = "Effect on the subject due to this event", formalDefinition = "Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).") 992 protected List<Reference> resultingCondition; 993 /** 994 * The actual objects that are the target of the reference (Includes information 995 * about the reaction that occurred as a result of exposure to a substance (for 996 * example, a drug or a chemical).) 997 */ 998 protected List<Condition> resultingConditionTarget; 999 1000 /** 1001 * The information about where the adverse event occurred. 1002 */ 1003 @Child(name = "location", type = { Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1004 @Description(shortDefinition = "Location where adverse event occurred", formalDefinition = "The information about where the adverse event occurred.") 1005 protected Reference location; 1006 1007 /** 1008 * The actual object that is the target of the reference (The information about 1009 * where the adverse event occurred.) 1010 */ 1011 protected Location locationTarget; 1012 1013 /** 1014 * Assessment whether this event was of real importance. 1015 */ 1016 @Child(name = "seriousness", type = { 1017 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1018 @Description(shortDefinition = "Seriousness of the event", formalDefinition = "Assessment whether this event was of real importance.") 1019 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-seriousness") 1020 protected CodeableConcept seriousness; 1021 1022 /** 1023 * Describes the severity of the adverse event, in relation to the subject. 1024 * Contrast to AdverseEvent.seriousness - a severe rash might not be serious, 1025 * but a mild heart problem is. 1026 */ 1027 @Child(name = "severity", type = { 1028 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1029 @Description(shortDefinition = "mild | moderate | severe", formalDefinition = "Describes the severity of the adverse event, in relation to the subject. Contrast to AdverseEvent.seriousness - a severe rash might not be serious, but a mild heart problem is.") 1030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-severity") 1031 protected CodeableConcept severity; 1032 1033 /** 1034 * Describes the type of outcome from the adverse event. 1035 */ 1036 @Child(name = "outcome", type = { 1037 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1038 @Description(shortDefinition = "resolved | recovering | ongoing | resolvedWithSequelae | fatal | unknown", formalDefinition = "Describes the type of outcome from the adverse event.") 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adverse-event-outcome") 1040 protected CodeableConcept outcome; 1041 1042 /** 1043 * Information on who recorded the adverse event. May be the patient or a 1044 * practitioner. 1045 */ 1046 @Child(name = "recorder", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1047 RelatedPerson.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1048 @Description(shortDefinition = "Who recorded the adverse event", formalDefinition = "Information on who recorded the adverse event. May be the patient or a practitioner.") 1049 protected Reference recorder; 1050 1051 /** 1052 * The actual object that is the target of the reference (Information on who 1053 * recorded the adverse event. May be the patient or a practitioner.) 1054 */ 1055 protected Resource recorderTarget; 1056 1057 /** 1058 * Parties that may or should contribute or have contributed information to the 1059 * adverse event, which can consist of one or more activities. Such information 1060 * includes information leading to the decision to perform the activity and how 1061 * to perform the activity (e.g. consultant), information that the activity 1062 * itself seeks to reveal (e.g. informant of clinical history), or information 1063 * about what activity was performed (e.g. informant witness). 1064 */ 1065 @Child(name = "contributor", type = { Practitioner.class, PractitionerRole.class, 1066 Device.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1067 @Description(shortDefinition = "Who was involved in the adverse event or the potential adverse event", formalDefinition = "Parties that may or should contribute or have contributed information to the adverse event, which can consist of one or more activities. Such information includes information leading to the decision to perform the activity and how to perform the activity (e.g. consultant), information that the activity itself seeks to reveal (e.g. informant of clinical history), or information about what activity was performed (e.g. informant witness).") 1068 protected List<Reference> contributor; 1069 /** 1070 * The actual objects that are the target of the reference (Parties that may or 1071 * should contribute or have contributed information to the adverse event, which 1072 * can consist of one or more activities. Such information includes information 1073 * leading to the decision to perform the activity and how to perform the 1074 * activity (e.g. consultant), information that the activity itself seeks to 1075 * reveal (e.g. informant of clinical history), or information about what 1076 * activity was performed (e.g. informant witness).) 1077 */ 1078 protected List<Resource> contributorTarget; 1079 1080 /** 1081 * Describes the entity that is suspected to have caused the adverse event. 1082 */ 1083 @Child(name = "suspectEntity", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1084 @Description(shortDefinition = "The suspected agent causing the adverse event", formalDefinition = "Describes the entity that is suspected to have caused the adverse event.") 1085 protected List<AdverseEventSuspectEntityComponent> suspectEntity; 1086 1087 /** 1088 * AdverseEvent.subjectMedicalHistory. 1089 */ 1090 @Child(name = "subjectMedicalHistory", type = { Condition.class, Observation.class, AllergyIntolerance.class, 1091 FamilyMemberHistory.class, Immunization.class, Procedure.class, Media.class, 1092 DocumentReference.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1093 @Description(shortDefinition = "AdverseEvent.subjectMedicalHistory", formalDefinition = "AdverseEvent.subjectMedicalHistory.") 1094 protected List<Reference> subjectMedicalHistory; 1095 /** 1096 * The actual objects that are the target of the reference 1097 * (AdverseEvent.subjectMedicalHistory.) 1098 */ 1099 protected List<Resource> subjectMedicalHistoryTarget; 1100 1101 /** 1102 * AdverseEvent.referenceDocument. 1103 */ 1104 @Child(name = "referenceDocument", type = { 1105 DocumentReference.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1106 @Description(shortDefinition = "AdverseEvent.referenceDocument", formalDefinition = "AdverseEvent.referenceDocument.") 1107 protected List<Reference> referenceDocument; 1108 /** 1109 * The actual objects that are the target of the reference 1110 * (AdverseEvent.referenceDocument.) 1111 */ 1112 protected List<DocumentReference> referenceDocumentTarget; 1113 1114 /** 1115 * AdverseEvent.study. 1116 */ 1117 @Child(name = "study", type = { 1118 ResearchStudy.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1119 @Description(shortDefinition = "AdverseEvent.study", formalDefinition = "AdverseEvent.study.") 1120 protected List<Reference> study; 1121 /** 1122 * The actual objects that are the target of the reference (AdverseEvent.study.) 1123 */ 1124 protected List<ResearchStudy> studyTarget; 1125 1126 private static final long serialVersionUID = -2055195281L; 1127 1128 /** 1129 * Constructor 1130 */ 1131 public AdverseEvent() { 1132 super(); 1133 } 1134 1135 /** 1136 * Constructor 1137 */ 1138 public AdverseEvent(Enumeration<AdverseEventActuality> actuality, Reference subject) { 1139 super(); 1140 this.actuality = actuality; 1141 this.subject = subject; 1142 } 1143 1144 /** 1145 * @return {@link #identifier} (Business identifiers assigned to this adverse 1146 * event by the performer or other systems which remain constant as the 1147 * resource is updated and propagates from server to server.) 1148 */ 1149 public Identifier getIdentifier() { 1150 if (this.identifier == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create AdverseEvent.identifier"); 1153 else if (Configuration.doAutoCreate()) 1154 this.identifier = new Identifier(); // cc 1155 return this.identifier; 1156 } 1157 1158 public boolean hasIdentifier() { 1159 return this.identifier != null && !this.identifier.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #identifier} (Business identifiers assigned to this 1164 * adverse event by the performer or other systems which remain 1165 * constant as the resource is updated and propagates from server 1166 * to server.) 1167 */ 1168 public AdverseEvent setIdentifier(Identifier value) { 1169 this.identifier = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #actuality} (Whether the event actually happened, or just had 1175 * the potential to. Note that this is independent of whether anyone was 1176 * affected or harmed or how severely.). This is the underlying object 1177 * with id, value and extensions. The accessor "getActuality" gives 1178 * direct access to the value 1179 */ 1180 public Enumeration<AdverseEventActuality> getActualityElement() { 1181 if (this.actuality == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create AdverseEvent.actuality"); 1184 else if (Configuration.doAutoCreate()) 1185 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); // bb 1186 return this.actuality; 1187 } 1188 1189 public boolean hasActualityElement() { 1190 return this.actuality != null && !this.actuality.isEmpty(); 1191 } 1192 1193 public boolean hasActuality() { 1194 return this.actuality != null && !this.actuality.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #actuality} (Whether the event actually happened, or just 1199 * had the potential to. Note that this is independent of whether 1200 * anyone was affected or harmed or how severely.). This is the 1201 * underlying object with id, value and extensions. The accessor 1202 * "getActuality" gives direct access to the value 1203 */ 1204 public AdverseEvent setActualityElement(Enumeration<AdverseEventActuality> value) { 1205 this.actuality = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return Whether the event actually happened, or just had the potential to. 1211 * Note that this is independent of whether anyone was affected or 1212 * harmed or how severely. 1213 */ 1214 public AdverseEventActuality getActuality() { 1215 return this.actuality == null ? null : this.actuality.getValue(); 1216 } 1217 1218 /** 1219 * @param value Whether the event actually happened, or just had the potential 1220 * to. Note that this is independent of whether anyone was affected 1221 * or harmed or how severely. 1222 */ 1223 public AdverseEvent setActuality(AdverseEventActuality value) { 1224 if (this.actuality == null) 1225 this.actuality = new Enumeration<AdverseEventActuality>(new AdverseEventActualityEnumFactory()); 1226 this.actuality.setValue(value); 1227 return this; 1228 } 1229 1230 /** 1231 * @return {@link #category} (The overall type of event, intended for search and 1232 * filtering purposes.) 1233 */ 1234 public List<CodeableConcept> getCategory() { 1235 if (this.category == null) 1236 this.category = new ArrayList<CodeableConcept>(); 1237 return this.category; 1238 } 1239 1240 /** 1241 * @return Returns a reference to <code>this</code> for easy method chaining 1242 */ 1243 public AdverseEvent setCategory(List<CodeableConcept> theCategory) { 1244 this.category = theCategory; 1245 return this; 1246 } 1247 1248 public boolean hasCategory() { 1249 if (this.category == null) 1250 return false; 1251 for (CodeableConcept item : this.category) 1252 if (!item.isEmpty()) 1253 return true; 1254 return false; 1255 } 1256 1257 public CodeableConcept addCategory() { // 3 1258 CodeableConcept t = new CodeableConcept(); 1259 if (this.category == null) 1260 this.category = new ArrayList<CodeableConcept>(); 1261 this.category.add(t); 1262 return t; 1263 } 1264 1265 public AdverseEvent addCategory(CodeableConcept t) { // 3 1266 if (t == null) 1267 return this; 1268 if (this.category == null) 1269 this.category = new ArrayList<CodeableConcept>(); 1270 this.category.add(t); 1271 return this; 1272 } 1273 1274 /** 1275 * @return The first repetition of repeating field {@link #category}, creating 1276 * it if it does not already exist 1277 */ 1278 public CodeableConcept getCategoryFirstRep() { 1279 if (getCategory().isEmpty()) { 1280 addCategory(); 1281 } 1282 return getCategory().get(0); 1283 } 1284 1285 /** 1286 * @return {@link #event} (This element defines the specific type of event that 1287 * occurred or that was prevented from occurring.) 1288 */ 1289 public CodeableConcept getEvent() { 1290 if (this.event == null) 1291 if (Configuration.errorOnAutoCreate()) 1292 throw new Error("Attempt to auto-create AdverseEvent.event"); 1293 else if (Configuration.doAutoCreate()) 1294 this.event = new CodeableConcept(); // cc 1295 return this.event; 1296 } 1297 1298 public boolean hasEvent() { 1299 return this.event != null && !this.event.isEmpty(); 1300 } 1301 1302 /** 1303 * @param value {@link #event} (This element defines the specific type of event 1304 * that occurred or that was prevented from occurring.) 1305 */ 1306 public AdverseEvent setEvent(CodeableConcept value) { 1307 this.event = value; 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #subject} (This subject or group impacted by the event.) 1313 */ 1314 public Reference getSubject() { 1315 if (this.subject == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create AdverseEvent.subject"); 1318 else if (Configuration.doAutoCreate()) 1319 this.subject = new Reference(); // cc 1320 return this.subject; 1321 } 1322 1323 public boolean hasSubject() { 1324 return this.subject != null && !this.subject.isEmpty(); 1325 } 1326 1327 /** 1328 * @param value {@link #subject} (This subject or group impacted by the event.) 1329 */ 1330 public AdverseEvent setSubject(Reference value) { 1331 this.subject = value; 1332 return this; 1333 } 1334 1335 /** 1336 * @return {@link #subject} The actual object that is the target of the 1337 * reference. The reference library doesn't populate this, but you can 1338 * use it to hold the resource if you resolve it. (This subject or group 1339 * impacted by the event.) 1340 */ 1341 public Resource getSubjectTarget() { 1342 return this.subjectTarget; 1343 } 1344 1345 /** 1346 * @param value {@link #subject} The actual object that is the target of the 1347 * reference. The reference library doesn't use these, but you can 1348 * use it to hold the resource if you resolve it. (This subject or 1349 * group impacted by the event.) 1350 */ 1351 public AdverseEvent setSubjectTarget(Resource value) { 1352 this.subjectTarget = value; 1353 return this; 1354 } 1355 1356 /** 1357 * @return {@link #encounter} (The Encounter during which AdverseEvent was 1358 * created or to which the creation of this record is tightly 1359 * associated.) 1360 */ 1361 public Reference getEncounter() { 1362 if (this.encounter == null) 1363 if (Configuration.errorOnAutoCreate()) 1364 throw new Error("Attempt to auto-create AdverseEvent.encounter"); 1365 else if (Configuration.doAutoCreate()) 1366 this.encounter = new Reference(); // cc 1367 return this.encounter; 1368 } 1369 1370 public boolean hasEncounter() { 1371 return this.encounter != null && !this.encounter.isEmpty(); 1372 } 1373 1374 /** 1375 * @param value {@link #encounter} (The Encounter during which AdverseEvent was 1376 * created or to which the creation of this record is tightly 1377 * associated.) 1378 */ 1379 public AdverseEvent setEncounter(Reference value) { 1380 this.encounter = value; 1381 return this; 1382 } 1383 1384 /** 1385 * @return {@link #encounter} The actual object that is the target of the 1386 * reference. The reference library doesn't populate this, but you can 1387 * use it to hold the resource if you resolve it. (The Encounter during 1388 * which AdverseEvent was created or to which the creation of this 1389 * record is tightly associated.) 1390 */ 1391 public Encounter getEncounterTarget() { 1392 if (this.encounterTarget == null) 1393 if (Configuration.errorOnAutoCreate()) 1394 throw new Error("Attempt to auto-create AdverseEvent.encounter"); 1395 else if (Configuration.doAutoCreate()) 1396 this.encounterTarget = new Encounter(); // aa 1397 return this.encounterTarget; 1398 } 1399 1400 /** 1401 * @param value {@link #encounter} The actual object that is the target of the 1402 * reference. The reference library doesn't use these, but you can 1403 * use it to hold the resource if you resolve it. (The Encounter 1404 * during which AdverseEvent was created or to which the creation 1405 * of this record is tightly associated.) 1406 */ 1407 public AdverseEvent setEncounterTarget(Encounter value) { 1408 this.encounterTarget = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return {@link #date} (The date (and perhaps time) when the adverse event 1414 * occurred.). This is the underlying object with id, value and 1415 * extensions. The accessor "getDate" gives direct access to the value 1416 */ 1417 public DateTimeType getDateElement() { 1418 if (this.date == null) 1419 if (Configuration.errorOnAutoCreate()) 1420 throw new Error("Attempt to auto-create AdverseEvent.date"); 1421 else if (Configuration.doAutoCreate()) 1422 this.date = new DateTimeType(); // bb 1423 return this.date; 1424 } 1425 1426 public boolean hasDateElement() { 1427 return this.date != null && !this.date.isEmpty(); 1428 } 1429 1430 public boolean hasDate() { 1431 return this.date != null && !this.date.isEmpty(); 1432 } 1433 1434 /** 1435 * @param value {@link #date} (The date (and perhaps time) when the adverse 1436 * event occurred.). This is the underlying object with id, value 1437 * and extensions. The accessor "getDate" gives direct access to 1438 * the value 1439 */ 1440 public AdverseEvent setDateElement(DateTimeType value) { 1441 this.date = value; 1442 return this; 1443 } 1444 1445 /** 1446 * @return The date (and perhaps time) when the adverse event occurred. 1447 */ 1448 public Date getDate() { 1449 return this.date == null ? null : this.date.getValue(); 1450 } 1451 1452 /** 1453 * @param value The date (and perhaps time) when the adverse event occurred. 1454 */ 1455 public AdverseEvent setDate(Date value) { 1456 if (value == null) 1457 this.date = null; 1458 else { 1459 if (this.date == null) 1460 this.date = new DateTimeType(); 1461 this.date.setValue(value); 1462 } 1463 return this; 1464 } 1465 1466 /** 1467 * @return {@link #detected} (Estimated or actual date the AdverseEvent began, 1468 * in the opinion of the reporter.). This is the underlying object with 1469 * id, value and extensions. The accessor "getDetected" gives direct 1470 * access to the value 1471 */ 1472 public DateTimeType getDetectedElement() { 1473 if (this.detected == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create AdverseEvent.detected"); 1476 else if (Configuration.doAutoCreate()) 1477 this.detected = new DateTimeType(); // bb 1478 return this.detected; 1479 } 1480 1481 public boolean hasDetectedElement() { 1482 return this.detected != null && !this.detected.isEmpty(); 1483 } 1484 1485 public boolean hasDetected() { 1486 return this.detected != null && !this.detected.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #detected} (Estimated or actual date the AdverseEvent 1491 * began, in the opinion of the reporter.). This is the underlying 1492 * object with id, value and extensions. The accessor "getDetected" 1493 * gives direct access to the value 1494 */ 1495 public AdverseEvent setDetectedElement(DateTimeType value) { 1496 this.detected = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return Estimated or actual date the AdverseEvent began, in the opinion of 1502 * the reporter. 1503 */ 1504 public Date getDetected() { 1505 return this.detected == null ? null : this.detected.getValue(); 1506 } 1507 1508 /** 1509 * @param value Estimated or actual date the AdverseEvent began, in the opinion 1510 * of the reporter. 1511 */ 1512 public AdverseEvent setDetected(Date value) { 1513 if (value == null) 1514 this.detected = null; 1515 else { 1516 if (this.detected == null) 1517 this.detected = new DateTimeType(); 1518 this.detected.setValue(value); 1519 } 1520 return this; 1521 } 1522 1523 /** 1524 * @return {@link #recordedDate} (The date on which the existence of the 1525 * AdverseEvent was first recorded.). This is the underlying object with 1526 * id, value and extensions. The accessor "getRecordedDate" gives direct 1527 * access to the value 1528 */ 1529 public DateTimeType getRecordedDateElement() { 1530 if (this.recordedDate == null) 1531 if (Configuration.errorOnAutoCreate()) 1532 throw new Error("Attempt to auto-create AdverseEvent.recordedDate"); 1533 else if (Configuration.doAutoCreate()) 1534 this.recordedDate = new DateTimeType(); // bb 1535 return this.recordedDate; 1536 } 1537 1538 public boolean hasRecordedDateElement() { 1539 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1540 } 1541 1542 public boolean hasRecordedDate() { 1543 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #recordedDate} (The date on which the existence of the 1548 * AdverseEvent was first recorded.). This is the underlying object 1549 * with id, value and extensions. The accessor "getRecordedDate" 1550 * gives direct access to the value 1551 */ 1552 public AdverseEvent setRecordedDateElement(DateTimeType value) { 1553 this.recordedDate = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return The date on which the existence of the AdverseEvent was first 1559 * recorded. 1560 */ 1561 public Date getRecordedDate() { 1562 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1563 } 1564 1565 /** 1566 * @param value The date on which the existence of the AdverseEvent was first 1567 * recorded. 1568 */ 1569 public AdverseEvent setRecordedDate(Date value) { 1570 if (value == null) 1571 this.recordedDate = null; 1572 else { 1573 if (this.recordedDate == null) 1574 this.recordedDate = new DateTimeType(); 1575 this.recordedDate.setValue(value); 1576 } 1577 return this; 1578 } 1579 1580 /** 1581 * @return {@link #resultingCondition} (Includes information about the reaction 1582 * that occurred as a result of exposure to a substance (for example, a 1583 * drug or a chemical).) 1584 */ 1585 public List<Reference> getResultingCondition() { 1586 if (this.resultingCondition == null) 1587 this.resultingCondition = new ArrayList<Reference>(); 1588 return this.resultingCondition; 1589 } 1590 1591 /** 1592 * @return Returns a reference to <code>this</code> for easy method chaining 1593 */ 1594 public AdverseEvent setResultingCondition(List<Reference> theResultingCondition) { 1595 this.resultingCondition = theResultingCondition; 1596 return this; 1597 } 1598 1599 public boolean hasResultingCondition() { 1600 if (this.resultingCondition == null) 1601 return false; 1602 for (Reference item : this.resultingCondition) 1603 if (!item.isEmpty()) 1604 return true; 1605 return false; 1606 } 1607 1608 public Reference addResultingCondition() { // 3 1609 Reference t = new Reference(); 1610 if (this.resultingCondition == null) 1611 this.resultingCondition = new ArrayList<Reference>(); 1612 this.resultingCondition.add(t); 1613 return t; 1614 } 1615 1616 public AdverseEvent addResultingCondition(Reference t) { // 3 1617 if (t == null) 1618 return this; 1619 if (this.resultingCondition == null) 1620 this.resultingCondition = new ArrayList<Reference>(); 1621 this.resultingCondition.add(t); 1622 return this; 1623 } 1624 1625 /** 1626 * @return The first repetition of repeating field {@link #resultingCondition}, 1627 * creating it if it does not already exist 1628 */ 1629 public Reference getResultingConditionFirstRep() { 1630 if (getResultingCondition().isEmpty()) { 1631 addResultingCondition(); 1632 } 1633 return getResultingCondition().get(0); 1634 } 1635 1636 /** 1637 * @deprecated Use Reference#setResource(IBaseResource) instead 1638 */ 1639 @Deprecated 1640 public List<Condition> getResultingConditionTarget() { 1641 if (this.resultingConditionTarget == null) 1642 this.resultingConditionTarget = new ArrayList<Condition>(); 1643 return this.resultingConditionTarget; 1644 } 1645 1646 /** 1647 * @deprecated Use Reference#setResource(IBaseResource) instead 1648 */ 1649 @Deprecated 1650 public Condition addResultingConditionTarget() { 1651 Condition r = new Condition(); 1652 if (this.resultingConditionTarget == null) 1653 this.resultingConditionTarget = new ArrayList<Condition>(); 1654 this.resultingConditionTarget.add(r); 1655 return r; 1656 } 1657 1658 /** 1659 * @return {@link #location} (The information about where the adverse event 1660 * occurred.) 1661 */ 1662 public Reference getLocation() { 1663 if (this.location == null) 1664 if (Configuration.errorOnAutoCreate()) 1665 throw new Error("Attempt to auto-create AdverseEvent.location"); 1666 else if (Configuration.doAutoCreate()) 1667 this.location = new Reference(); // cc 1668 return this.location; 1669 } 1670 1671 public boolean hasLocation() { 1672 return this.location != null && !this.location.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #location} (The information about where the adverse event 1677 * occurred.) 1678 */ 1679 public AdverseEvent setLocation(Reference value) { 1680 this.location = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #location} The actual object that is the target of the 1686 * reference. The reference library doesn't populate this, but you can 1687 * use it to hold the resource if you resolve it. (The information about 1688 * where the adverse event occurred.) 1689 */ 1690 public Location getLocationTarget() { 1691 if (this.locationTarget == null) 1692 if (Configuration.errorOnAutoCreate()) 1693 throw new Error("Attempt to auto-create AdverseEvent.location"); 1694 else if (Configuration.doAutoCreate()) 1695 this.locationTarget = new Location(); // aa 1696 return this.locationTarget; 1697 } 1698 1699 /** 1700 * @param value {@link #location} The actual object that is the target of the 1701 * reference. The reference library doesn't use these, but you can 1702 * use it to hold the resource if you resolve it. (The information 1703 * about where the adverse event occurred.) 1704 */ 1705 public AdverseEvent setLocationTarget(Location value) { 1706 this.locationTarget = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return {@link #seriousness} (Assessment whether this event was of real 1712 * importance.) 1713 */ 1714 public CodeableConcept getSeriousness() { 1715 if (this.seriousness == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create AdverseEvent.seriousness"); 1718 else if (Configuration.doAutoCreate()) 1719 this.seriousness = new CodeableConcept(); // cc 1720 return this.seriousness; 1721 } 1722 1723 public boolean hasSeriousness() { 1724 return this.seriousness != null && !this.seriousness.isEmpty(); 1725 } 1726 1727 /** 1728 * @param value {@link #seriousness} (Assessment whether this event was of real 1729 * importance.) 1730 */ 1731 public AdverseEvent setSeriousness(CodeableConcept value) { 1732 this.seriousness = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #severity} (Describes the severity of the adverse event, in 1738 * relation to the subject. Contrast to AdverseEvent.seriousness - a 1739 * severe rash might not be serious, but a mild heart problem is.) 1740 */ 1741 public CodeableConcept getSeverity() { 1742 if (this.severity == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create AdverseEvent.severity"); 1745 else if (Configuration.doAutoCreate()) 1746 this.severity = new CodeableConcept(); // cc 1747 return this.severity; 1748 } 1749 1750 public boolean hasSeverity() { 1751 return this.severity != null && !this.severity.isEmpty(); 1752 } 1753 1754 /** 1755 * @param value {@link #severity} (Describes the severity of the adverse event, 1756 * in relation to the subject. Contrast to AdverseEvent.seriousness 1757 * - a severe rash might not be serious, but a mild heart problem 1758 * is.) 1759 */ 1760 public AdverseEvent setSeverity(CodeableConcept value) { 1761 this.severity = value; 1762 return this; 1763 } 1764 1765 /** 1766 * @return {@link #outcome} (Describes the type of outcome from the adverse 1767 * event.) 1768 */ 1769 public CodeableConcept getOutcome() { 1770 if (this.outcome == null) 1771 if (Configuration.errorOnAutoCreate()) 1772 throw new Error("Attempt to auto-create AdverseEvent.outcome"); 1773 else if (Configuration.doAutoCreate()) 1774 this.outcome = new CodeableConcept(); // cc 1775 return this.outcome; 1776 } 1777 1778 public boolean hasOutcome() { 1779 return this.outcome != null && !this.outcome.isEmpty(); 1780 } 1781 1782 /** 1783 * @param value {@link #outcome} (Describes the type of outcome from the adverse 1784 * event.) 1785 */ 1786 public AdverseEvent setOutcome(CodeableConcept value) { 1787 this.outcome = value; 1788 return this; 1789 } 1790 1791 /** 1792 * @return {@link #recorder} (Information on who recorded the adverse event. May 1793 * be the patient or a practitioner.) 1794 */ 1795 public Reference getRecorder() { 1796 if (this.recorder == null) 1797 if (Configuration.errorOnAutoCreate()) 1798 throw new Error("Attempt to auto-create AdverseEvent.recorder"); 1799 else if (Configuration.doAutoCreate()) 1800 this.recorder = new Reference(); // cc 1801 return this.recorder; 1802 } 1803 1804 public boolean hasRecorder() { 1805 return this.recorder != null && !this.recorder.isEmpty(); 1806 } 1807 1808 /** 1809 * @param value {@link #recorder} (Information on who recorded the adverse 1810 * event. May be the patient or a practitioner.) 1811 */ 1812 public AdverseEvent setRecorder(Reference value) { 1813 this.recorder = value; 1814 return this; 1815 } 1816 1817 /** 1818 * @return {@link #recorder} The actual object that is the target of the 1819 * reference. The reference library doesn't populate this, but you can 1820 * use it to hold the resource if you resolve it. (Information on who 1821 * recorded the adverse event. May be the patient or a practitioner.) 1822 */ 1823 public Resource getRecorderTarget() { 1824 return this.recorderTarget; 1825 } 1826 1827 /** 1828 * @param value {@link #recorder} The actual object that is the target of the 1829 * reference. The reference library doesn't use these, but you can 1830 * use it to hold the resource if you resolve it. (Information on 1831 * who recorded the adverse event. May be the patient or a 1832 * practitioner.) 1833 */ 1834 public AdverseEvent setRecorderTarget(Resource value) { 1835 this.recorderTarget = value; 1836 return this; 1837 } 1838 1839 /** 1840 * @return {@link #contributor} (Parties that may or should contribute or have 1841 * contributed information to the adverse event, which can consist of 1842 * one or more activities. Such information includes information leading 1843 * to the decision to perform the activity and how to perform the 1844 * activity (e.g. consultant), information that the activity itself 1845 * seeks to reveal (e.g. informant of clinical history), or information 1846 * about what activity was performed (e.g. informant witness).) 1847 */ 1848 public List<Reference> getContributor() { 1849 if (this.contributor == null) 1850 this.contributor = new ArrayList<Reference>(); 1851 return this.contributor; 1852 } 1853 1854 /** 1855 * @return Returns a reference to <code>this</code> for easy method chaining 1856 */ 1857 public AdverseEvent setContributor(List<Reference> theContributor) { 1858 this.contributor = theContributor; 1859 return this; 1860 } 1861 1862 public boolean hasContributor() { 1863 if (this.contributor == null) 1864 return false; 1865 for (Reference item : this.contributor) 1866 if (!item.isEmpty()) 1867 return true; 1868 return false; 1869 } 1870 1871 public Reference addContributor() { // 3 1872 Reference t = new Reference(); 1873 if (this.contributor == null) 1874 this.contributor = new ArrayList<Reference>(); 1875 this.contributor.add(t); 1876 return t; 1877 } 1878 1879 public AdverseEvent addContributor(Reference t) { // 3 1880 if (t == null) 1881 return this; 1882 if (this.contributor == null) 1883 this.contributor = new ArrayList<Reference>(); 1884 this.contributor.add(t); 1885 return this; 1886 } 1887 1888 /** 1889 * @return The first repetition of repeating field {@link #contributor}, 1890 * creating it if it does not already exist 1891 */ 1892 public Reference getContributorFirstRep() { 1893 if (getContributor().isEmpty()) { 1894 addContributor(); 1895 } 1896 return getContributor().get(0); 1897 } 1898 1899 /** 1900 * @deprecated Use Reference#setResource(IBaseResource) instead 1901 */ 1902 @Deprecated 1903 public List<Resource> getContributorTarget() { 1904 if (this.contributorTarget == null) 1905 this.contributorTarget = new ArrayList<Resource>(); 1906 return this.contributorTarget; 1907 } 1908 1909 /** 1910 * @return {@link #suspectEntity} (Describes the entity that is suspected to 1911 * have caused the adverse event.) 1912 */ 1913 public List<AdverseEventSuspectEntityComponent> getSuspectEntity() { 1914 if (this.suspectEntity == null) 1915 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1916 return this.suspectEntity; 1917 } 1918 1919 /** 1920 * @return Returns a reference to <code>this</code> for easy method chaining 1921 */ 1922 public AdverseEvent setSuspectEntity(List<AdverseEventSuspectEntityComponent> theSuspectEntity) { 1923 this.suspectEntity = theSuspectEntity; 1924 return this; 1925 } 1926 1927 public boolean hasSuspectEntity() { 1928 if (this.suspectEntity == null) 1929 return false; 1930 for (AdverseEventSuspectEntityComponent item : this.suspectEntity) 1931 if (!item.isEmpty()) 1932 return true; 1933 return false; 1934 } 1935 1936 public AdverseEventSuspectEntityComponent addSuspectEntity() { // 3 1937 AdverseEventSuspectEntityComponent t = new AdverseEventSuspectEntityComponent(); 1938 if (this.suspectEntity == null) 1939 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1940 this.suspectEntity.add(t); 1941 return t; 1942 } 1943 1944 public AdverseEvent addSuspectEntity(AdverseEventSuspectEntityComponent t) { // 3 1945 if (t == null) 1946 return this; 1947 if (this.suspectEntity == null) 1948 this.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 1949 this.suspectEntity.add(t); 1950 return this; 1951 } 1952 1953 /** 1954 * @return The first repetition of repeating field {@link #suspectEntity}, 1955 * creating it if it does not already exist 1956 */ 1957 public AdverseEventSuspectEntityComponent getSuspectEntityFirstRep() { 1958 if (getSuspectEntity().isEmpty()) { 1959 addSuspectEntity(); 1960 } 1961 return getSuspectEntity().get(0); 1962 } 1963 1964 /** 1965 * @return {@link #subjectMedicalHistory} (AdverseEvent.subjectMedicalHistory.) 1966 */ 1967 public List<Reference> getSubjectMedicalHistory() { 1968 if (this.subjectMedicalHistory == null) 1969 this.subjectMedicalHistory = new ArrayList<Reference>(); 1970 return this.subjectMedicalHistory; 1971 } 1972 1973 /** 1974 * @return Returns a reference to <code>this</code> for easy method chaining 1975 */ 1976 public AdverseEvent setSubjectMedicalHistory(List<Reference> theSubjectMedicalHistory) { 1977 this.subjectMedicalHistory = theSubjectMedicalHistory; 1978 return this; 1979 } 1980 1981 public boolean hasSubjectMedicalHistory() { 1982 if (this.subjectMedicalHistory == null) 1983 return false; 1984 for (Reference item : this.subjectMedicalHistory) 1985 if (!item.isEmpty()) 1986 return true; 1987 return false; 1988 } 1989 1990 public Reference addSubjectMedicalHistory() { // 3 1991 Reference t = new Reference(); 1992 if (this.subjectMedicalHistory == null) 1993 this.subjectMedicalHistory = new ArrayList<Reference>(); 1994 this.subjectMedicalHistory.add(t); 1995 return t; 1996 } 1997 1998 public AdverseEvent addSubjectMedicalHistory(Reference t) { // 3 1999 if (t == null) 2000 return this; 2001 if (this.subjectMedicalHistory == null) 2002 this.subjectMedicalHistory = new ArrayList<Reference>(); 2003 this.subjectMedicalHistory.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @return The first repetition of repeating field 2009 * {@link #subjectMedicalHistory}, creating it if it does not already 2010 * exist 2011 */ 2012 public Reference getSubjectMedicalHistoryFirstRep() { 2013 if (getSubjectMedicalHistory().isEmpty()) { 2014 addSubjectMedicalHistory(); 2015 } 2016 return getSubjectMedicalHistory().get(0); 2017 } 2018 2019 /** 2020 * @deprecated Use Reference#setResource(IBaseResource) instead 2021 */ 2022 @Deprecated 2023 public List<Resource> getSubjectMedicalHistoryTarget() { 2024 if (this.subjectMedicalHistoryTarget == null) 2025 this.subjectMedicalHistoryTarget = new ArrayList<Resource>(); 2026 return this.subjectMedicalHistoryTarget; 2027 } 2028 2029 /** 2030 * @return {@link #referenceDocument} (AdverseEvent.referenceDocument.) 2031 */ 2032 public List<Reference> getReferenceDocument() { 2033 if (this.referenceDocument == null) 2034 this.referenceDocument = new ArrayList<Reference>(); 2035 return this.referenceDocument; 2036 } 2037 2038 /** 2039 * @return Returns a reference to <code>this</code> for easy method chaining 2040 */ 2041 public AdverseEvent setReferenceDocument(List<Reference> theReferenceDocument) { 2042 this.referenceDocument = theReferenceDocument; 2043 return this; 2044 } 2045 2046 public boolean hasReferenceDocument() { 2047 if (this.referenceDocument == null) 2048 return false; 2049 for (Reference item : this.referenceDocument) 2050 if (!item.isEmpty()) 2051 return true; 2052 return false; 2053 } 2054 2055 public Reference addReferenceDocument() { // 3 2056 Reference t = new Reference(); 2057 if (this.referenceDocument == null) 2058 this.referenceDocument = new ArrayList<Reference>(); 2059 this.referenceDocument.add(t); 2060 return t; 2061 } 2062 2063 public AdverseEvent addReferenceDocument(Reference t) { // 3 2064 if (t == null) 2065 return this; 2066 if (this.referenceDocument == null) 2067 this.referenceDocument = new ArrayList<Reference>(); 2068 this.referenceDocument.add(t); 2069 return this; 2070 } 2071 2072 /** 2073 * @return The first repetition of repeating field {@link #referenceDocument}, 2074 * creating it if it does not already exist 2075 */ 2076 public Reference getReferenceDocumentFirstRep() { 2077 if (getReferenceDocument().isEmpty()) { 2078 addReferenceDocument(); 2079 } 2080 return getReferenceDocument().get(0); 2081 } 2082 2083 /** 2084 * @deprecated Use Reference#setResource(IBaseResource) instead 2085 */ 2086 @Deprecated 2087 public List<DocumentReference> getReferenceDocumentTarget() { 2088 if (this.referenceDocumentTarget == null) 2089 this.referenceDocumentTarget = new ArrayList<DocumentReference>(); 2090 return this.referenceDocumentTarget; 2091 } 2092 2093 /** 2094 * @deprecated Use Reference#setResource(IBaseResource) instead 2095 */ 2096 @Deprecated 2097 public DocumentReference addReferenceDocumentTarget() { 2098 DocumentReference r = new DocumentReference(); 2099 if (this.referenceDocumentTarget == null) 2100 this.referenceDocumentTarget = new ArrayList<DocumentReference>(); 2101 this.referenceDocumentTarget.add(r); 2102 return r; 2103 } 2104 2105 /** 2106 * @return {@link #study} (AdverseEvent.study.) 2107 */ 2108 public List<Reference> getStudy() { 2109 if (this.study == null) 2110 this.study = new ArrayList<Reference>(); 2111 return this.study; 2112 } 2113 2114 /** 2115 * @return Returns a reference to <code>this</code> for easy method chaining 2116 */ 2117 public AdverseEvent setStudy(List<Reference> theStudy) { 2118 this.study = theStudy; 2119 return this; 2120 } 2121 2122 public boolean hasStudy() { 2123 if (this.study == null) 2124 return false; 2125 for (Reference item : this.study) 2126 if (!item.isEmpty()) 2127 return true; 2128 return false; 2129 } 2130 2131 public Reference addStudy() { // 3 2132 Reference t = new Reference(); 2133 if (this.study == null) 2134 this.study = new ArrayList<Reference>(); 2135 this.study.add(t); 2136 return t; 2137 } 2138 2139 public AdverseEvent addStudy(Reference t) { // 3 2140 if (t == null) 2141 return this; 2142 if (this.study == null) 2143 this.study = new ArrayList<Reference>(); 2144 this.study.add(t); 2145 return this; 2146 } 2147 2148 /** 2149 * @return The first repetition of repeating field {@link #study}, creating it 2150 * if it does not already exist 2151 */ 2152 public Reference getStudyFirstRep() { 2153 if (getStudy().isEmpty()) { 2154 addStudy(); 2155 } 2156 return getStudy().get(0); 2157 } 2158 2159 /** 2160 * @deprecated Use Reference#setResource(IBaseResource) instead 2161 */ 2162 @Deprecated 2163 public List<ResearchStudy> getStudyTarget() { 2164 if (this.studyTarget == null) 2165 this.studyTarget = new ArrayList<ResearchStudy>(); 2166 return this.studyTarget; 2167 } 2168 2169 /** 2170 * @deprecated Use Reference#setResource(IBaseResource) instead 2171 */ 2172 @Deprecated 2173 public ResearchStudy addStudyTarget() { 2174 ResearchStudy r = new ResearchStudy(); 2175 if (this.studyTarget == null) 2176 this.studyTarget = new ArrayList<ResearchStudy>(); 2177 this.studyTarget.add(r); 2178 return r; 2179 } 2180 2181 protected void listChildren(List<Property> children) { 2182 super.listChildren(children); 2183 children.add(new Property("identifier", "Identifier", 2184 "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2185 0, 1, identifier)); 2186 children.add(new Property("actuality", "code", 2187 "Whether the event actually happened, or just had the potential to. Note that this is independent of whether anyone was affected or harmed or how severely.", 2188 0, 1, actuality)); 2189 children.add(new Property("category", "CodeableConcept", 2190 "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, 2191 category)); 2192 children.add(new Property("event", "CodeableConcept", 2193 "This element defines the specific type of event that occurred or that was prevented from occurring.", 0, 1, 2194 event)); 2195 children.add(new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson)", 2196 "This subject or group impacted by the event.", 0, 1, subject)); 2197 children.add(new Property("encounter", "Reference(Encounter)", 2198 "The Encounter during which AdverseEvent was created or to which the creation of this record is tightly associated.", 2199 0, 1, encounter)); 2200 children.add( 2201 new Property("date", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 0, 1, date)); 2202 children.add(new Property("detected", "dateTime", 2203 "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected)); 2204 children.add(new Property("recordedDate", "dateTime", 2205 "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate)); 2206 children.add(new Property("resultingCondition", "Reference(Condition)", 2207 "Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).", 2208 0, java.lang.Integer.MAX_VALUE, resultingCondition)); 2209 children.add(new Property("location", "Reference(Location)", 2210 "The information about where the adverse event occurred.", 0, 1, location)); 2211 children.add(new Property("seriousness", "CodeableConcept", "Assessment whether this event was of real importance.", 2212 0, 1, seriousness)); 2213 children.add(new Property("severity", "CodeableConcept", 2214 "Describes the severity of the adverse event, in relation to the subject. Contrast to AdverseEvent.seriousness - a severe rash might not be serious, but a mild heart problem is.", 2215 0, 1, severity)); 2216 children.add(new Property("outcome", "CodeableConcept", "Describes the type of outcome from the adverse event.", 0, 2217 1, outcome)); 2218 children.add(new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2219 "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder)); 2220 children.add(new Property("contributor", "Reference(Practitioner|PractitionerRole|Device)", 2221 "Parties that may or should contribute or have contributed information to the adverse event, which can consist of one or more activities. Such information includes information leading to the decision to perform the activity and how to perform the activity (e.g. consultant), information that the activity itself seeks to reveal (e.g. informant of clinical history), or information about what activity was performed (e.g. informant witness).", 2222 0, java.lang.Integer.MAX_VALUE, contributor)); 2223 children.add( 2224 new Property("suspectEntity", "", "Describes the entity that is suspected to have caused the adverse event.", 0, 2225 java.lang.Integer.MAX_VALUE, suspectEntity)); 2226 children.add(new Property("subjectMedicalHistory", 2227 "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Media|DocumentReference)", 2228 "AdverseEvent.subjectMedicalHistory.", 0, java.lang.Integer.MAX_VALUE, subjectMedicalHistory)); 2229 children.add(new Property("referenceDocument", "Reference(DocumentReference)", "AdverseEvent.referenceDocument.", 0, 2230 java.lang.Integer.MAX_VALUE, referenceDocument)); 2231 children.add(new Property("study", "Reference(ResearchStudy)", "AdverseEvent.study.", 0, 2232 java.lang.Integer.MAX_VALUE, study)); 2233 } 2234 2235 @Override 2236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2237 switch (_hash) { 2238 case -1618432855: 2239 /* identifier */ return new Property("identifier", "Identifier", 2240 "Business identifiers assigned to this adverse event by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2241 0, 1, identifier); 2242 case 528866400: 2243 /* actuality */ return new Property("actuality", "code", 2244 "Whether the event actually happened, or just had the potential to. Note that this is independent of whether anyone was affected or harmed or how severely.", 2245 0, 1, actuality); 2246 case 50511102: 2247 /* category */ return new Property("category", "CodeableConcept", 2248 "The overall type of event, intended for search and filtering purposes.", 0, java.lang.Integer.MAX_VALUE, 2249 category); 2250 case 96891546: 2251 /* event */ return new Property("event", "CodeableConcept", 2252 "This element defines the specific type of event that occurred or that was prevented from occurring.", 0, 1, 2253 event); 2254 case -1867885268: 2255 /* subject */ return new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson)", 2256 "This subject or group impacted by the event.", 0, 1, subject); 2257 case 1524132147: 2258 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2259 "The Encounter during which AdverseEvent was created or to which the creation of this record is tightly associated.", 2260 0, 1, encounter); 2261 case 3076014: 2262 /* date */ return new Property("date", "dateTime", "The date (and perhaps time) when the adverse event occurred.", 2263 0, 1, date); 2264 case 1048254082: 2265 /* detected */ return new Property("detected", "dateTime", 2266 "Estimated or actual date the AdverseEvent began, in the opinion of the reporter.", 0, 1, detected); 2267 case -1952893826: 2268 /* recordedDate */ return new Property("recordedDate", "dateTime", 2269 "The date on which the existence of the AdverseEvent was first recorded.", 0, 1, recordedDate); 2270 case -830261258: 2271 /* resultingCondition */ return new Property("resultingCondition", "Reference(Condition)", 2272 "Includes information about the reaction that occurred as a result of exposure to a substance (for example, a drug or a chemical).", 2273 0, java.lang.Integer.MAX_VALUE, resultingCondition); 2274 case 1901043637: 2275 /* location */ return new Property("location", "Reference(Location)", 2276 "The information about where the adverse event occurred.", 0, 1, location); 2277 case -1551003909: 2278 /* seriousness */ return new Property("seriousness", "CodeableConcept", 2279 "Assessment whether this event was of real importance.", 0, 1, seriousness); 2280 case 1478300413: 2281 /* severity */ return new Property("severity", "CodeableConcept", 2282 "Describes the severity of the adverse event, in relation to the subject. Contrast to AdverseEvent.seriousness - a severe rash might not be serious, but a mild heart problem is.", 2283 0, 1, severity); 2284 case -1106507950: 2285 /* outcome */ return new Property("outcome", "CodeableConcept", 2286 "Describes the type of outcome from the adverse event.", 0, 1, outcome); 2287 case -799233858: 2288 /* recorder */ return new Property("recorder", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2289 "Information on who recorded the adverse event. May be the patient or a practitioner.", 0, 1, recorder); 2290 case -1895276325: 2291 /* contributor */ return new Property("contributor", "Reference(Practitioner|PractitionerRole|Device)", 2292 "Parties that may or should contribute or have contributed information to the adverse event, which can consist of one or more activities. Such information includes information leading to the decision to perform the activity and how to perform the activity (e.g. consultant), information that the activity itself seeks to reveal (e.g. informant of clinical history), or information about what activity was performed (e.g. informant witness).", 2293 0, java.lang.Integer.MAX_VALUE, contributor); 2294 case -1957422662: 2295 /* suspectEntity */ return new Property("suspectEntity", "", 2296 "Describes the entity that is suspected to have caused the adverse event.", 0, java.lang.Integer.MAX_VALUE, 2297 suspectEntity); 2298 case -1685245681: 2299 /* subjectMedicalHistory */ return new Property("subjectMedicalHistory", 2300 "Reference(Condition|Observation|AllergyIntolerance|FamilyMemberHistory|Immunization|Procedure|Media|DocumentReference)", 2301 "AdverseEvent.subjectMedicalHistory.", 0, java.lang.Integer.MAX_VALUE, subjectMedicalHistory); 2302 case 1013971334: 2303 /* referenceDocument */ return new Property("referenceDocument", "Reference(DocumentReference)", 2304 "AdverseEvent.referenceDocument.", 0, java.lang.Integer.MAX_VALUE, referenceDocument); 2305 case 109776329: 2306 /* study */ return new Property("study", "Reference(ResearchStudy)", "AdverseEvent.study.", 0, 2307 java.lang.Integer.MAX_VALUE, study); 2308 default: 2309 return super.getNamedProperty(_hash, _name, _checkValid); 2310 } 2311 2312 } 2313 2314 @Override 2315 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2316 switch (hash) { 2317 case -1618432855: 2318 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2319 case 528866400: 2320 /* actuality */ return this.actuality == null ? new Base[0] : new Base[] { this.actuality }; // Enumeration<AdverseEventActuality> 2321 case 50511102: 2322 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2323 case 96891546: 2324 /* event */ return this.event == null ? new Base[0] : new Base[] { this.event }; // CodeableConcept 2325 case -1867885268: 2326 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2327 case 1524132147: 2328 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2329 case 3076014: 2330 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2331 case 1048254082: 2332 /* detected */ return this.detected == null ? new Base[0] : new Base[] { this.detected }; // DateTimeType 2333 case -1952893826: 2334 /* recordedDate */ return this.recordedDate == null ? new Base[0] : new Base[] { this.recordedDate }; // DateTimeType 2335 case -830261258: 2336 /* resultingCondition */ return this.resultingCondition == null ? new Base[0] 2337 : this.resultingCondition.toArray(new Base[this.resultingCondition.size()]); // Reference 2338 case 1901043637: 2339 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2340 case -1551003909: 2341 /* seriousness */ return this.seriousness == null ? new Base[0] : new Base[] { this.seriousness }; // CodeableConcept 2342 case 1478300413: 2343 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // CodeableConcept 2344 case -1106507950: 2345 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // CodeableConcept 2346 case -799233858: 2347 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 2348 case -1895276325: 2349 /* contributor */ return this.contributor == null ? new Base[0] 2350 : this.contributor.toArray(new Base[this.contributor.size()]); // Reference 2351 case -1957422662: 2352 /* suspectEntity */ return this.suspectEntity == null ? new Base[0] 2353 : this.suspectEntity.toArray(new Base[this.suspectEntity.size()]); // AdverseEventSuspectEntityComponent 2354 case -1685245681: 2355 /* subjectMedicalHistory */ return this.subjectMedicalHistory == null ? new Base[0] 2356 : this.subjectMedicalHistory.toArray(new Base[this.subjectMedicalHistory.size()]); // Reference 2357 case 1013971334: 2358 /* referenceDocument */ return this.referenceDocument == null ? new Base[0] 2359 : this.referenceDocument.toArray(new Base[this.referenceDocument.size()]); // Reference 2360 case 109776329: 2361 /* study */ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // Reference 2362 default: 2363 return super.getProperty(hash, name, checkValid); 2364 } 2365 2366 } 2367 2368 @Override 2369 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2370 switch (hash) { 2371 case -1618432855: // identifier 2372 this.identifier = castToIdentifier(value); // Identifier 2373 return value; 2374 case 528866400: // actuality 2375 value = new AdverseEventActualityEnumFactory().fromType(castToCode(value)); 2376 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 2377 return value; 2378 case 50511102: // category 2379 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2380 return value; 2381 case 96891546: // event 2382 this.event = castToCodeableConcept(value); // CodeableConcept 2383 return value; 2384 case -1867885268: // subject 2385 this.subject = castToReference(value); // Reference 2386 return value; 2387 case 1524132147: // encounter 2388 this.encounter = castToReference(value); // Reference 2389 return value; 2390 case 3076014: // date 2391 this.date = castToDateTime(value); // DateTimeType 2392 return value; 2393 case 1048254082: // detected 2394 this.detected = castToDateTime(value); // DateTimeType 2395 return value; 2396 case -1952893826: // recordedDate 2397 this.recordedDate = castToDateTime(value); // DateTimeType 2398 return value; 2399 case -830261258: // resultingCondition 2400 this.getResultingCondition().add(castToReference(value)); // Reference 2401 return value; 2402 case 1901043637: // location 2403 this.location = castToReference(value); // Reference 2404 return value; 2405 case -1551003909: // seriousness 2406 this.seriousness = castToCodeableConcept(value); // CodeableConcept 2407 return value; 2408 case 1478300413: // severity 2409 this.severity = castToCodeableConcept(value); // CodeableConcept 2410 return value; 2411 case -1106507950: // outcome 2412 this.outcome = castToCodeableConcept(value); // CodeableConcept 2413 return value; 2414 case -799233858: // recorder 2415 this.recorder = castToReference(value); // Reference 2416 return value; 2417 case -1895276325: // contributor 2418 this.getContributor().add(castToReference(value)); // Reference 2419 return value; 2420 case -1957422662: // suspectEntity 2421 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); // AdverseEventSuspectEntityComponent 2422 return value; 2423 case -1685245681: // subjectMedicalHistory 2424 this.getSubjectMedicalHistory().add(castToReference(value)); // Reference 2425 return value; 2426 case 1013971334: // referenceDocument 2427 this.getReferenceDocument().add(castToReference(value)); // Reference 2428 return value; 2429 case 109776329: // study 2430 this.getStudy().add(castToReference(value)); // Reference 2431 return value; 2432 default: 2433 return super.setProperty(hash, name, value); 2434 } 2435 2436 } 2437 2438 @Override 2439 public Base setProperty(String name, Base value) throws FHIRException { 2440 if (name.equals("identifier")) { 2441 this.identifier = castToIdentifier(value); // Identifier 2442 } else if (name.equals("actuality")) { 2443 value = new AdverseEventActualityEnumFactory().fromType(castToCode(value)); 2444 this.actuality = (Enumeration) value; // Enumeration<AdverseEventActuality> 2445 } else if (name.equals("category")) { 2446 this.getCategory().add(castToCodeableConcept(value)); 2447 } else if (name.equals("event")) { 2448 this.event = castToCodeableConcept(value); // CodeableConcept 2449 } else if (name.equals("subject")) { 2450 this.subject = castToReference(value); // Reference 2451 } else if (name.equals("encounter")) { 2452 this.encounter = castToReference(value); // Reference 2453 } else if (name.equals("date")) { 2454 this.date = castToDateTime(value); // DateTimeType 2455 } else if (name.equals("detected")) { 2456 this.detected = castToDateTime(value); // DateTimeType 2457 } else if (name.equals("recordedDate")) { 2458 this.recordedDate = castToDateTime(value); // DateTimeType 2459 } else if (name.equals("resultingCondition")) { 2460 this.getResultingCondition().add(castToReference(value)); 2461 } else if (name.equals("location")) { 2462 this.location = castToReference(value); // Reference 2463 } else if (name.equals("seriousness")) { 2464 this.seriousness = castToCodeableConcept(value); // CodeableConcept 2465 } else if (name.equals("severity")) { 2466 this.severity = castToCodeableConcept(value); // CodeableConcept 2467 } else if (name.equals("outcome")) { 2468 this.outcome = castToCodeableConcept(value); // CodeableConcept 2469 } else if (name.equals("recorder")) { 2470 this.recorder = castToReference(value); // Reference 2471 } else if (name.equals("contributor")) { 2472 this.getContributor().add(castToReference(value)); 2473 } else if (name.equals("suspectEntity")) { 2474 this.getSuspectEntity().add((AdverseEventSuspectEntityComponent) value); 2475 } else if (name.equals("subjectMedicalHistory")) { 2476 this.getSubjectMedicalHistory().add(castToReference(value)); 2477 } else if (name.equals("referenceDocument")) { 2478 this.getReferenceDocument().add(castToReference(value)); 2479 } else if (name.equals("study")) { 2480 this.getStudy().add(castToReference(value)); 2481 } else 2482 return super.setProperty(name, value); 2483 return value; 2484 } 2485 2486 @Override 2487 public void removeChild(String name, Base value) throws FHIRException { 2488 if (name.equals("identifier")) { 2489 this.identifier = null; 2490 } else if (name.equals("actuality")) { 2491 this.actuality = null; 2492 } else if (name.equals("category")) { 2493 this.getCategory().remove(castToCodeableConcept(value)); 2494 } else if (name.equals("event")) { 2495 this.event = null; 2496 } else if (name.equals("subject")) { 2497 this.subject = null; 2498 } else if (name.equals("encounter")) { 2499 this.encounter = null; 2500 } else if (name.equals("date")) { 2501 this.date = null; 2502 } else if (name.equals("detected")) { 2503 this.detected = null; 2504 } else if (name.equals("recordedDate")) { 2505 this.recordedDate = null; 2506 } else if (name.equals("resultingCondition")) { 2507 this.getResultingCondition().remove(castToReference(value)); 2508 } else if (name.equals("location")) { 2509 this.location = null; 2510 } else if (name.equals("seriousness")) { 2511 this.seriousness = null; 2512 } else if (name.equals("severity")) { 2513 this.severity = null; 2514 } else if (name.equals("outcome")) { 2515 this.outcome = null; 2516 } else if (name.equals("recorder")) { 2517 this.recorder = null; 2518 } else if (name.equals("contributor")) { 2519 this.getContributor().remove(castToReference(value)); 2520 } else if (name.equals("suspectEntity")) { 2521 this.getSuspectEntity().remove((AdverseEventSuspectEntityComponent) value); 2522 } else if (name.equals("subjectMedicalHistory")) { 2523 this.getSubjectMedicalHistory().remove(castToReference(value)); 2524 } else if (name.equals("referenceDocument")) { 2525 this.getReferenceDocument().remove(castToReference(value)); 2526 } else if (name.equals("study")) { 2527 this.getStudy().remove(castToReference(value)); 2528 } else 2529 super.removeChild(name, value); 2530 2531 } 2532 2533 @Override 2534 public Base makeProperty(int hash, String name) throws FHIRException { 2535 switch (hash) { 2536 case -1618432855: 2537 return getIdentifier(); 2538 case 528866400: 2539 return getActualityElement(); 2540 case 50511102: 2541 return addCategory(); 2542 case 96891546: 2543 return getEvent(); 2544 case -1867885268: 2545 return getSubject(); 2546 case 1524132147: 2547 return getEncounter(); 2548 case 3076014: 2549 return getDateElement(); 2550 case 1048254082: 2551 return getDetectedElement(); 2552 case -1952893826: 2553 return getRecordedDateElement(); 2554 case -830261258: 2555 return addResultingCondition(); 2556 case 1901043637: 2557 return getLocation(); 2558 case -1551003909: 2559 return getSeriousness(); 2560 case 1478300413: 2561 return getSeverity(); 2562 case -1106507950: 2563 return getOutcome(); 2564 case -799233858: 2565 return getRecorder(); 2566 case -1895276325: 2567 return addContributor(); 2568 case -1957422662: 2569 return addSuspectEntity(); 2570 case -1685245681: 2571 return addSubjectMedicalHistory(); 2572 case 1013971334: 2573 return addReferenceDocument(); 2574 case 109776329: 2575 return addStudy(); 2576 default: 2577 return super.makeProperty(hash, name); 2578 } 2579 2580 } 2581 2582 @Override 2583 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2584 switch (hash) { 2585 case -1618432855: 2586 /* identifier */ return new String[] { "Identifier" }; 2587 case 528866400: 2588 /* actuality */ return new String[] { "code" }; 2589 case 50511102: 2590 /* category */ return new String[] { "CodeableConcept" }; 2591 case 96891546: 2592 /* event */ return new String[] { "CodeableConcept" }; 2593 case -1867885268: 2594 /* subject */ return new String[] { "Reference" }; 2595 case 1524132147: 2596 /* encounter */ return new String[] { "Reference" }; 2597 case 3076014: 2598 /* date */ return new String[] { "dateTime" }; 2599 case 1048254082: 2600 /* detected */ return new String[] { "dateTime" }; 2601 case -1952893826: 2602 /* recordedDate */ return new String[] { "dateTime" }; 2603 case -830261258: 2604 /* resultingCondition */ return new String[] { "Reference" }; 2605 case 1901043637: 2606 /* location */ return new String[] { "Reference" }; 2607 case -1551003909: 2608 /* seriousness */ return new String[] { "CodeableConcept" }; 2609 case 1478300413: 2610 /* severity */ return new String[] { "CodeableConcept" }; 2611 case -1106507950: 2612 /* outcome */ return new String[] { "CodeableConcept" }; 2613 case -799233858: 2614 /* recorder */ return new String[] { "Reference" }; 2615 case -1895276325: 2616 /* contributor */ return new String[] { "Reference" }; 2617 case -1957422662: 2618 /* suspectEntity */ return new String[] {}; 2619 case -1685245681: 2620 /* subjectMedicalHistory */ return new String[] { "Reference" }; 2621 case 1013971334: 2622 /* referenceDocument */ return new String[] { "Reference" }; 2623 case 109776329: 2624 /* study */ return new String[] { "Reference" }; 2625 default: 2626 return super.getTypesForProperty(hash, name); 2627 } 2628 2629 } 2630 2631 @Override 2632 public Base addChild(String name) throws FHIRException { 2633 if (name.equals("identifier")) { 2634 this.identifier = new Identifier(); 2635 return this.identifier; 2636 } else if (name.equals("actuality")) { 2637 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.actuality"); 2638 } else if (name.equals("category")) { 2639 return addCategory(); 2640 } else if (name.equals("event")) { 2641 this.event = new CodeableConcept(); 2642 return this.event; 2643 } else if (name.equals("subject")) { 2644 this.subject = new Reference(); 2645 return this.subject; 2646 } else if (name.equals("encounter")) { 2647 this.encounter = new Reference(); 2648 return this.encounter; 2649 } else if (name.equals("date")) { 2650 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.date"); 2651 } else if (name.equals("detected")) { 2652 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.detected"); 2653 } else if (name.equals("recordedDate")) { 2654 throw new FHIRException("Cannot call addChild on a singleton property AdverseEvent.recordedDate"); 2655 } else if (name.equals("resultingCondition")) { 2656 return addResultingCondition(); 2657 } else if (name.equals("location")) { 2658 this.location = new Reference(); 2659 return this.location; 2660 } else if (name.equals("seriousness")) { 2661 this.seriousness = new CodeableConcept(); 2662 return this.seriousness; 2663 } else if (name.equals("severity")) { 2664 this.severity = new CodeableConcept(); 2665 return this.severity; 2666 } else if (name.equals("outcome")) { 2667 this.outcome = new CodeableConcept(); 2668 return this.outcome; 2669 } else if (name.equals("recorder")) { 2670 this.recorder = new Reference(); 2671 return this.recorder; 2672 } else if (name.equals("contributor")) { 2673 return addContributor(); 2674 } else if (name.equals("suspectEntity")) { 2675 return addSuspectEntity(); 2676 } else if (name.equals("subjectMedicalHistory")) { 2677 return addSubjectMedicalHistory(); 2678 } else if (name.equals("referenceDocument")) { 2679 return addReferenceDocument(); 2680 } else if (name.equals("study")) { 2681 return addStudy(); 2682 } else 2683 return super.addChild(name); 2684 } 2685 2686 public String fhirType() { 2687 return "AdverseEvent"; 2688 2689 } 2690 2691 public AdverseEvent copy() { 2692 AdverseEvent dst = new AdverseEvent(); 2693 copyValues(dst); 2694 return dst; 2695 } 2696 2697 public void copyValues(AdverseEvent dst) { 2698 super.copyValues(dst); 2699 dst.identifier = identifier == null ? null : identifier.copy(); 2700 dst.actuality = actuality == null ? null : actuality.copy(); 2701 if (category != null) { 2702 dst.category = new ArrayList<CodeableConcept>(); 2703 for (CodeableConcept i : category) 2704 dst.category.add(i.copy()); 2705 } 2706 ; 2707 dst.event = event == null ? null : event.copy(); 2708 dst.subject = subject == null ? null : subject.copy(); 2709 dst.encounter = encounter == null ? null : encounter.copy(); 2710 dst.date = date == null ? null : date.copy(); 2711 dst.detected = detected == null ? null : detected.copy(); 2712 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2713 if (resultingCondition != null) { 2714 dst.resultingCondition = new ArrayList<Reference>(); 2715 for (Reference i : resultingCondition) 2716 dst.resultingCondition.add(i.copy()); 2717 } 2718 ; 2719 dst.location = location == null ? null : location.copy(); 2720 dst.seriousness = seriousness == null ? null : seriousness.copy(); 2721 dst.severity = severity == null ? null : severity.copy(); 2722 dst.outcome = outcome == null ? null : outcome.copy(); 2723 dst.recorder = recorder == null ? null : recorder.copy(); 2724 if (contributor != null) { 2725 dst.contributor = new ArrayList<Reference>(); 2726 for (Reference i : contributor) 2727 dst.contributor.add(i.copy()); 2728 } 2729 ; 2730 if (suspectEntity != null) { 2731 dst.suspectEntity = new ArrayList<AdverseEventSuspectEntityComponent>(); 2732 for (AdverseEventSuspectEntityComponent i : suspectEntity) 2733 dst.suspectEntity.add(i.copy()); 2734 } 2735 ; 2736 if (subjectMedicalHistory != null) { 2737 dst.subjectMedicalHistory = new ArrayList<Reference>(); 2738 for (Reference i : subjectMedicalHistory) 2739 dst.subjectMedicalHistory.add(i.copy()); 2740 } 2741 ; 2742 if (referenceDocument != null) { 2743 dst.referenceDocument = new ArrayList<Reference>(); 2744 for (Reference i : referenceDocument) 2745 dst.referenceDocument.add(i.copy()); 2746 } 2747 ; 2748 if (study != null) { 2749 dst.study = new ArrayList<Reference>(); 2750 for (Reference i : study) 2751 dst.study.add(i.copy()); 2752 } 2753 ; 2754 } 2755 2756 protected AdverseEvent typedCopy() { 2757 return copy(); 2758 } 2759 2760 @Override 2761 public boolean equalsDeep(Base other_) { 2762 if (!super.equalsDeep(other_)) 2763 return false; 2764 if (!(other_ instanceof AdverseEvent)) 2765 return false; 2766 AdverseEvent o = (AdverseEvent) other_; 2767 return compareDeep(identifier, o.identifier, true) && compareDeep(actuality, o.actuality, true) 2768 && compareDeep(category, o.category, true) && compareDeep(event, o.event, true) 2769 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2770 && compareDeep(date, o.date, true) && compareDeep(detected, o.detected, true) 2771 && compareDeep(recordedDate, o.recordedDate, true) 2772 && compareDeep(resultingCondition, o.resultingCondition, true) && compareDeep(location, o.location, true) 2773 && compareDeep(seriousness, o.seriousness, true) && compareDeep(severity, o.severity, true) 2774 && compareDeep(outcome, o.outcome, true) && compareDeep(recorder, o.recorder, true) 2775 && compareDeep(contributor, o.contributor, true) && compareDeep(suspectEntity, o.suspectEntity, true) 2776 && compareDeep(subjectMedicalHistory, o.subjectMedicalHistory, true) 2777 && compareDeep(referenceDocument, o.referenceDocument, true) && compareDeep(study, o.study, true); 2778 } 2779 2780 @Override 2781 public boolean equalsShallow(Base other_) { 2782 if (!super.equalsShallow(other_)) 2783 return false; 2784 if (!(other_ instanceof AdverseEvent)) 2785 return false; 2786 AdverseEvent o = (AdverseEvent) other_; 2787 return compareValues(actuality, o.actuality, true) && compareValues(date, o.date, true) 2788 && compareValues(detected, o.detected, true) && compareValues(recordedDate, o.recordedDate, true); 2789 } 2790 2791 public boolean isEmpty() { 2792 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, actuality, category, event, subject, 2793 encounter, date, detected, recordedDate, resultingCondition, location, seriousness, severity, outcome, recorder, 2794 contributor, suspectEntity, subjectMedicalHistory, referenceDocument, study); 2795 } 2796 2797 @Override 2798 public ResourceType getResourceType() { 2799 return ResourceType.AdverseEvent; 2800 } 2801 2802 /** 2803 * Search parameter: <b>date</b> 2804 * <p> 2805 * Description: <b>When the event occurred</b><br> 2806 * Type: <b>date</b><br> 2807 * Path: <b>AdverseEvent.date</b><br> 2808 * </p> 2809 */ 2810 @SearchParamDefinition(name = "date", path = "AdverseEvent.date", description = "When the event occurred", type = "date") 2811 public static final String SP_DATE = "date"; 2812 /** 2813 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2814 * <p> 2815 * Description: <b>When the event occurred</b><br> 2816 * Type: <b>date</b><br> 2817 * Path: <b>AdverseEvent.date</b><br> 2818 * </p> 2819 */ 2820 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2821 SP_DATE); 2822 2823 /** 2824 * Search parameter: <b>severity</b> 2825 * <p> 2826 * Description: <b>mild | moderate | severe</b><br> 2827 * Type: <b>token</b><br> 2828 * Path: <b>AdverseEvent.severity</b><br> 2829 * </p> 2830 */ 2831 @SearchParamDefinition(name = "severity", path = "AdverseEvent.severity", description = "mild | moderate | severe", type = "token") 2832 public static final String SP_SEVERITY = "severity"; 2833 /** 2834 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2835 * <p> 2836 * Description: <b>mild | moderate | severe</b><br> 2837 * Type: <b>token</b><br> 2838 * Path: <b>AdverseEvent.severity</b><br> 2839 * </p> 2840 */ 2841 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2842 SP_SEVERITY); 2843 2844 /** 2845 * Search parameter: <b>recorder</b> 2846 * <p> 2847 * Description: <b>Who recorded the adverse event</b><br> 2848 * Type: <b>reference</b><br> 2849 * Path: <b>AdverseEvent.recorder</b><br> 2850 * </p> 2851 */ 2852 @SearchParamDefinition(name = "recorder", path = "AdverseEvent.recorder", description = "Who recorded the adverse event", type = "reference", providesMembershipIn = { 2853 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2854 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2855 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2856 public static final String SP_RECORDER = "recorder"; 2857 /** 2858 * <b>Fluent Client</b> search parameter constant for <b>recorder</b> 2859 * <p> 2860 * Description: <b>Who recorded the adverse event</b><br> 2861 * Type: <b>reference</b><br> 2862 * Path: <b>AdverseEvent.recorder</b><br> 2863 * </p> 2864 */ 2865 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECORDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2866 SP_RECORDER); 2867 2868 /** 2869 * Constant for fluent queries to be used to add include statements. Specifies 2870 * the path value of "<b>AdverseEvent:recorder</b>". 2871 */ 2872 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECORDER = new ca.uhn.fhir.model.api.Include( 2873 "AdverseEvent:recorder").toLocked(); 2874 2875 /** 2876 * Search parameter: <b>study</b> 2877 * <p> 2878 * Description: <b>AdverseEvent.study</b><br> 2879 * Type: <b>reference</b><br> 2880 * Path: <b>AdverseEvent.study</b><br> 2881 * </p> 2882 */ 2883 @SearchParamDefinition(name = "study", path = "AdverseEvent.study", description = "AdverseEvent.study", type = "reference", target = { 2884 ResearchStudy.class }) 2885 public static final String SP_STUDY = "study"; 2886 /** 2887 * <b>Fluent Client</b> search parameter constant for <b>study</b> 2888 * <p> 2889 * Description: <b>AdverseEvent.study</b><br> 2890 * Type: <b>reference</b><br> 2891 * Path: <b>AdverseEvent.study</b><br> 2892 * </p> 2893 */ 2894 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2895 SP_STUDY); 2896 2897 /** 2898 * Constant for fluent queries to be used to add include statements. Specifies 2899 * the path value of "<b>AdverseEvent:study</b>". 2900 */ 2901 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include( 2902 "AdverseEvent:study").toLocked(); 2903 2904 /** 2905 * Search parameter: <b>actuality</b> 2906 * <p> 2907 * Description: <b>actual | potential</b><br> 2908 * Type: <b>token</b><br> 2909 * Path: <b>AdverseEvent.actuality</b><br> 2910 * </p> 2911 */ 2912 @SearchParamDefinition(name = "actuality", path = "AdverseEvent.actuality", description = "actual | potential", type = "token") 2913 public static final String SP_ACTUALITY = "actuality"; 2914 /** 2915 * <b>Fluent Client</b> search parameter constant for <b>actuality</b> 2916 * <p> 2917 * Description: <b>actual | potential</b><br> 2918 * Type: <b>token</b><br> 2919 * Path: <b>AdverseEvent.actuality</b><br> 2920 * </p> 2921 */ 2922 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2923 SP_ACTUALITY); 2924 2925 /** 2926 * Search parameter: <b>seriousness</b> 2927 * <p> 2928 * Description: <b>Seriousness of the event</b><br> 2929 * Type: <b>token</b><br> 2930 * Path: <b>AdverseEvent.seriousness</b><br> 2931 * </p> 2932 */ 2933 @SearchParamDefinition(name = "seriousness", path = "AdverseEvent.seriousness", description = "Seriousness of the event", type = "token") 2934 public static final String SP_SERIOUSNESS = "seriousness"; 2935 /** 2936 * <b>Fluent Client</b> search parameter constant for <b>seriousness</b> 2937 * <p> 2938 * Description: <b>Seriousness of the event</b><br> 2939 * Type: <b>token</b><br> 2940 * Path: <b>AdverseEvent.seriousness</b><br> 2941 * </p> 2942 */ 2943 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIOUSNESS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2944 SP_SERIOUSNESS); 2945 2946 /** 2947 * Search parameter: <b>subject</b> 2948 * <p> 2949 * Description: <b>Subject impacted by event</b><br> 2950 * Type: <b>reference</b><br> 2951 * Path: <b>AdverseEvent.subject</b><br> 2952 * </p> 2953 */ 2954 @SearchParamDefinition(name = "subject", path = "AdverseEvent.subject", description = "Subject impacted by event", type = "reference", providesMembershipIn = { 2955 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class, 2956 Practitioner.class, RelatedPerson.class }) 2957 public static final String SP_SUBJECT = "subject"; 2958 /** 2959 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2960 * <p> 2961 * Description: <b>Subject impacted by event</b><br> 2962 * Type: <b>reference</b><br> 2963 * Path: <b>AdverseEvent.subject</b><br> 2964 * </p> 2965 */ 2966 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2967 SP_SUBJECT); 2968 2969 /** 2970 * Constant for fluent queries to be used to add include statements. Specifies 2971 * the path value of "<b>AdverseEvent:subject</b>". 2972 */ 2973 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2974 "AdverseEvent:subject").toLocked(); 2975 2976 /** 2977 * Search parameter: <b>resultingcondition</b> 2978 * <p> 2979 * Description: <b>Effect on the subject due to this event</b><br> 2980 * Type: <b>reference</b><br> 2981 * Path: <b>AdverseEvent.resultingCondition</b><br> 2982 * </p> 2983 */ 2984 @SearchParamDefinition(name = "resultingcondition", path = "AdverseEvent.resultingCondition", description = "Effect on the subject due to this event", type = "reference", target = { 2985 Condition.class }) 2986 public static final String SP_RESULTINGCONDITION = "resultingcondition"; 2987 /** 2988 * <b>Fluent Client</b> search parameter constant for <b>resultingcondition</b> 2989 * <p> 2990 * Description: <b>Effect on the subject due to this event</b><br> 2991 * Type: <b>reference</b><br> 2992 * Path: <b>AdverseEvent.resultingCondition</b><br> 2993 * </p> 2994 */ 2995 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTINGCONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2996 SP_RESULTINGCONDITION); 2997 2998 /** 2999 * Constant for fluent queries to be used to add include statements. Specifies 3000 * the path value of "<b>AdverseEvent:resultingcondition</b>". 3001 */ 3002 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTINGCONDITION = new ca.uhn.fhir.model.api.Include( 3003 "AdverseEvent:resultingcondition").toLocked(); 3004 3005 /** 3006 * Search parameter: <b>substance</b> 3007 * <p> 3008 * Description: <b>Refers to the specific entity that caused the adverse 3009 * event</b><br> 3010 * Type: <b>reference</b><br> 3011 * Path: <b>AdverseEvent.suspectEntity.instance</b><br> 3012 * </p> 3013 */ 3014 @SearchParamDefinition(name = "substance", path = "AdverseEvent.suspectEntity.instance", description = "Refers to the specific entity that caused the adverse event", type = "reference", target = { 3015 Device.class, Immunization.class, Medication.class, MedicationAdministration.class, MedicationStatement.class, 3016 Procedure.class, Substance.class }) 3017 public static final String SP_SUBSTANCE = "substance"; 3018 /** 3019 * <b>Fluent Client</b> search parameter constant for <b>substance</b> 3020 * <p> 3021 * Description: <b>Refers to the specific entity that caused the adverse 3022 * event</b><br> 3023 * Type: <b>reference</b><br> 3024 * Path: <b>AdverseEvent.suspectEntity.instance</b><br> 3025 * </p> 3026 */ 3027 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3028 SP_SUBSTANCE); 3029 3030 /** 3031 * Constant for fluent queries to be used to add include statements. Specifies 3032 * the path value of "<b>AdverseEvent:substance</b>". 3033 */ 3034 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE = new ca.uhn.fhir.model.api.Include( 3035 "AdverseEvent:substance").toLocked(); 3036 3037 /** 3038 * Search parameter: <b>location</b> 3039 * <p> 3040 * Description: <b>Location where adverse event occurred</b><br> 3041 * Type: <b>reference</b><br> 3042 * Path: <b>AdverseEvent.location</b><br> 3043 * </p> 3044 */ 3045 @SearchParamDefinition(name = "location", path = "AdverseEvent.location", description = "Location where adverse event occurred", type = "reference", target = { 3046 Location.class }) 3047 public static final String SP_LOCATION = "location"; 3048 /** 3049 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3050 * <p> 3051 * Description: <b>Location where adverse event occurred</b><br> 3052 * Type: <b>reference</b><br> 3053 * Path: <b>AdverseEvent.location</b><br> 3054 * </p> 3055 */ 3056 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3057 SP_LOCATION); 3058 3059 /** 3060 * Constant for fluent queries to be used to add include statements. Specifies 3061 * the path value of "<b>AdverseEvent:location</b>". 3062 */ 3063 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 3064 "AdverseEvent:location").toLocked(); 3065 3066 /** 3067 * Search parameter: <b>category</b> 3068 * <p> 3069 * Description: <b>product-problem | product-quality | product-use-error | 3070 * wrong-dose | incorrect-prescribing-information | wrong-technique | 3071 * wrong-route-of-administration | wrong-rate | wrong-duration | wrong-time | 3072 * expired-drug | medical-device-use-error | problem-different-manufacturer | 3073 * unsafe-physical-environment</b><br> 3074 * Type: <b>token</b><br> 3075 * Path: <b>AdverseEvent.category</b><br> 3076 * </p> 3077 */ 3078 @SearchParamDefinition(name = "category", path = "AdverseEvent.category", description = "product-problem | product-quality | product-use-error | wrong-dose | incorrect-prescribing-information | wrong-technique | wrong-route-of-administration | wrong-rate | wrong-duration | wrong-time | expired-drug | medical-device-use-error | problem-different-manufacturer | unsafe-physical-environment", type = "token") 3079 public static final String SP_CATEGORY = "category"; 3080 /** 3081 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3082 * <p> 3083 * Description: <b>product-problem | product-quality | product-use-error | 3084 * wrong-dose | incorrect-prescribing-information | wrong-technique | 3085 * wrong-route-of-administration | wrong-rate | wrong-duration | wrong-time | 3086 * expired-drug | medical-device-use-error | problem-different-manufacturer | 3087 * unsafe-physical-environment</b><br> 3088 * Type: <b>token</b><br> 3089 * Path: <b>AdverseEvent.category</b><br> 3090 * </p> 3091 */ 3092 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3093 SP_CATEGORY); 3094 3095 /** 3096 * Search parameter: <b>event</b> 3097 * <p> 3098 * Description: <b>Type of the event itself in relation to the subject</b><br> 3099 * Type: <b>token</b><br> 3100 * Path: <b>AdverseEvent.event</b><br> 3101 * </p> 3102 */ 3103 @SearchParamDefinition(name = "event", path = "AdverseEvent.event", description = "Type of the event itself in relation to the subject", type = "token") 3104 public static final String SP_EVENT = "event"; 3105 /** 3106 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3107 * <p> 3108 * Description: <b>Type of the event itself in relation to the subject</b><br> 3109 * Type: <b>token</b><br> 3110 * Path: <b>AdverseEvent.event</b><br> 3111 * </p> 3112 */ 3113 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3114 SP_EVENT); 3115 3116}