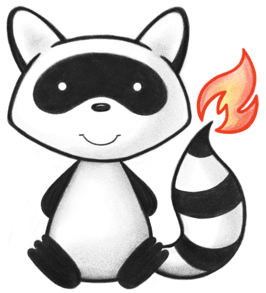
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039 040/** 041 * A duration of time during which an organism (or a process) has existed. 042 */ 043@DatatypeDef(name = "Age") 044public class Age extends Quantity implements ICompositeType { 045 046 private static final long serialVersionUID = 0L; 047 048 /** 049 * Constructor 050 */ 051 public Age() { 052 super(); 053 } 054 055 protected void listChildren(List<Property> children) { 056 super.listChildren(children); 057 } 058 059 @Override 060 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 061 switch (_hash) { 062 default: 063 return super.getNamedProperty(_hash, _name, _checkValid); 064 } 065 066 } 067 068 @Override 069 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 070 switch (hash) { 071 default: 072 return super.getProperty(hash, name, checkValid); 073 } 074 075 } 076 077 @Override 078 public Base setProperty(int hash, String name, Base value) throws FHIRException { 079 switch (hash) { 080 default: 081 return super.setProperty(hash, name, value); 082 } 083 084 } 085 086 @Override 087 public Base setProperty(String name, Base value) throws FHIRException { 088 return super.setProperty(name, value); 089 } 090 091 @Override 092 public void removeChild(String name, Base value) throws FHIRException { 093 super.removeChild(name, value); 094 } 095 096 @Override 097 public Base makeProperty(int hash, String name) throws FHIRException { 098 switch (hash) { 099 default: 100 return super.makeProperty(hash, name); 101 } 102 103 } 104 105 @Override 106 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 107 switch (hash) { 108 default: 109 return super.getTypesForProperty(hash, name); 110 } 111 112 } 113 114 @Override 115 public Base addChild(String name) throws FHIRException { 116 return super.addChild(name); 117 } 118 119 public String fhirType() { 120 return "Age"; 121 122 } 123 124 public Age copy() { 125 Age dst = new Age(); 126 copyValues(dst); 127 return dst; 128 } 129 130 public void copyValues(Age dst) { 131 super.copyValues(dst); 132 } 133 134 protected Age typedCopy() { 135 return copy(); 136 } 137 138 @Override 139 public boolean equalsDeep(Base other_) { 140 if (!super.equalsDeep(other_)) 141 return false; 142 if (!(other_ instanceof Age)) 143 return false; 144 Age o = (Age) other_; 145 return true; 146 } 147 148 @Override 149 public boolean equalsShallow(Base other_) { 150 if (!super.equalsShallow(other_)) 151 return false; 152 if (!(other_ instanceof Age)) 153 return false; 154 Age o = (Age) other_; 155 return true; 156 } 157 158 public boolean isEmpty() { 159 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 160 } 161 162}