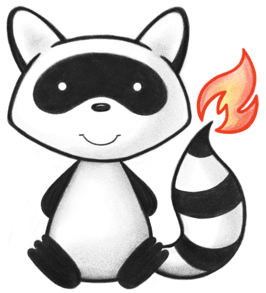
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.Date; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * A text note which also contains information about who made the statement and 045 * when. 046 */ 047@DatatypeDef(name = "Annotation") 048public class Annotation extends Type implements ICompositeType { 049 050 /** 051 * The individual responsible for making the annotation. 052 */ 053 @Child(name = "author", type = { Practitioner.class, Patient.class, RelatedPerson.class, Organization.class, 054 StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Individual responsible for the annotation", formalDefinition = "The individual responsible for making the annotation.") 056 protected Type author; 057 058 /** 059 * Indicates when this particular annotation was made. 060 */ 061 @Child(name = "time", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "When the annotation was made", formalDefinition = "Indicates when this particular annotation was made.") 063 protected DateTimeType time; 064 065 /** 066 * The text of the annotation in markdown format. 067 */ 068 @Child(name = "text", type = { MarkdownType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "The annotation - text content (as markdown)", formalDefinition = "The text of the annotation in markdown format.") 070 protected MarkdownType text; 071 072 private static final long serialVersionUID = 1324090545L; 073 074 /** 075 * Constructor 076 */ 077 public Annotation() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public Annotation(MarkdownType text) { 085 super(); 086 this.text = text; 087 } 088 089 /** 090 * @return {@link #author} (The individual responsible for making the 091 * annotation.) 092 */ 093 public Type getAuthor() { 094 return this.author; 095 } 096 097 /** 098 * @return {@link #author} (The individual responsible for making the 099 * annotation.) 100 */ 101 public Reference getAuthorReference() throws FHIRException { 102 if (this.author == null) 103 this.author = new Reference(); 104 if (!(this.author instanceof Reference)) 105 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.author.getClass().getName() 106 + " was encountered"); 107 return (Reference) this.author; 108 } 109 110 public boolean hasAuthorReference() { 111 return this != null && this.author instanceof Reference; 112 } 113 114 /** 115 * @return {@link #author} (The individual responsible for making the 116 * annotation.) 117 */ 118 public StringType getAuthorStringType() throws FHIRException { 119 if (this.author == null) 120 this.author = new StringType(); 121 if (!(this.author instanceof StringType)) 122 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.author.getClass().getName() 123 + " was encountered"); 124 return (StringType) this.author; 125 } 126 127 public boolean hasAuthorStringType() { 128 return this != null && this.author instanceof StringType; 129 } 130 131 public boolean hasAuthor() { 132 return this.author != null && !this.author.isEmpty(); 133 } 134 135 /** 136 * @param value {@link #author} (The individual responsible for making the 137 * annotation.) 138 */ 139 public Annotation setAuthor(Type value) { 140 if (value != null && !(value instanceof Reference || value instanceof StringType)) 141 throw new Error("Not the right type for Annotation.author[x]: " + value.fhirType()); 142 this.author = value; 143 return this; 144 } 145 146 /** 147 * @return {@link #time} (Indicates when this particular annotation was made.). 148 * This is the underlying object with id, value and extensions. The 149 * accessor "getTime" gives direct access to the value 150 */ 151 public DateTimeType getTimeElement() { 152 if (this.time == null) 153 if (Configuration.errorOnAutoCreate()) 154 throw new Error("Attempt to auto-create Annotation.time"); 155 else if (Configuration.doAutoCreate()) 156 this.time = new DateTimeType(); // bb 157 return this.time; 158 } 159 160 public boolean hasTimeElement() { 161 return this.time != null && !this.time.isEmpty(); 162 } 163 164 public boolean hasTime() { 165 return this.time != null && !this.time.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #time} (Indicates when this particular annotation was 170 * made.). This is the underlying object with id, value and 171 * extensions. The accessor "getTime" gives direct access to the 172 * value 173 */ 174 public Annotation setTimeElement(DateTimeType value) { 175 this.time = value; 176 return this; 177 } 178 179 /** 180 * @return Indicates when this particular annotation was made. 181 */ 182 public Date getTime() { 183 return this.time == null ? null : this.time.getValue(); 184 } 185 186 /** 187 * @param value Indicates when this particular annotation was made. 188 */ 189 public Annotation setTime(Date value) { 190 if (value == null) 191 this.time = null; 192 else { 193 if (this.time == null) 194 this.time = new DateTimeType(); 195 this.time.setValue(value); 196 } 197 return this; 198 } 199 200 /** 201 * @return {@link #text} (The text of the annotation in markdown format.). This 202 * is the underlying object with id, value and extensions. The accessor 203 * "getText" gives direct access to the value 204 */ 205 public MarkdownType getTextElement() { 206 if (this.text == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create Annotation.text"); 209 else if (Configuration.doAutoCreate()) 210 this.text = new MarkdownType(); // bb 211 return this.text; 212 } 213 214 public boolean hasTextElement() { 215 return this.text != null && !this.text.isEmpty(); 216 } 217 218 public boolean hasText() { 219 return this.text != null && !this.text.isEmpty(); 220 } 221 222 /** 223 * @param value {@link #text} (The text of the annotation in markdown format.). 224 * This is the underlying object with id, value and extensions. The 225 * accessor "getText" gives direct access to the value 226 */ 227 public Annotation setTextElement(MarkdownType value) { 228 this.text = value; 229 return this; 230 } 231 232 /** 233 * @return The text of the annotation in markdown format. 234 */ 235 public String getText() { 236 return this.text == null ? null : this.text.getValue(); 237 } 238 239 /** 240 * @param value The text of the annotation in markdown format. 241 */ 242 public Annotation setText(String value) { 243 if (this.text == null) 244 this.text = new MarkdownType(); 245 this.text.setValue(value); 246 return this; 247 } 248 249 protected void listChildren(List<Property> children) { 250 super.listChildren(children); 251 children.add(new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson|Organization)|string", 252 "The individual responsible for making the annotation.", 0, 1, author)); 253 children.add(new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, time)); 254 children.add(new Property("text", "markdown", "The text of the annotation in markdown format.", 0, 1, text)); 255 } 256 257 @Override 258 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 259 switch (_hash) { 260 case 1475597077: 261 /* author[x] */ return new Property("author[x]", 262 "Reference(Practitioner|Patient|RelatedPerson|Organization)|string", 263 "The individual responsible for making the annotation.", 0, 1, author); 264 case -1406328437: 265 /* author */ return new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson|Organization)|string", 266 "The individual responsible for making the annotation.", 0, 1, author); 267 case 305515008: 268 /* authorReference */ return new Property("author[x]", 269 "Reference(Practitioner|Patient|RelatedPerson|Organization)|string", 270 "The individual responsible for making the annotation.", 0, 1, author); 271 case 290249084: 272 /* authorString */ return new Property("author[x]", 273 "Reference(Practitioner|Patient|RelatedPerson|Organization)|string", 274 "The individual responsible for making the annotation.", 0, 1, author); 275 case 3560141: 276 /* time */ return new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 1, 277 time); 278 case 3556653: 279 /* text */ return new Property("text", "markdown", "The text of the annotation in markdown format.", 0, 1, text); 280 default: 281 return super.getNamedProperty(_hash, _name, _checkValid); 282 } 283 284 } 285 286 @Override 287 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 288 switch (hash) { 289 case -1406328437: 290 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Type 291 case 3560141: 292 /* time */ return this.time == null ? new Base[0] : new Base[] { this.time }; // DateTimeType 293 case 3556653: 294 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // MarkdownType 295 default: 296 return super.getProperty(hash, name, checkValid); 297 } 298 299 } 300 301 @Override 302 public Base setProperty(int hash, String name, Base value) throws FHIRException { 303 switch (hash) { 304 case -1406328437: // author 305 this.author = castToType(value); // Type 306 return value; 307 case 3560141: // time 308 this.time = castToDateTime(value); // DateTimeType 309 return value; 310 case 3556653: // text 311 this.text = castToMarkdown(value); // MarkdownType 312 return value; 313 default: 314 return super.setProperty(hash, name, value); 315 } 316 317 } 318 319 @Override 320 public Base setProperty(String name, Base value) throws FHIRException { 321 if (name.equals("author[x]")) { 322 this.author = castToType(value); // Type 323 } else if (name.equals("time")) { 324 this.time = castToDateTime(value); // DateTimeType 325 } else if (name.equals("text")) { 326 this.text = castToMarkdown(value); // MarkdownType 327 } else 328 return super.setProperty(name, value); 329 return value; 330 } 331 332 @Override 333 public void removeChild(String name, Base value) throws FHIRException { 334 if (name.equals("author[x]")) { 335 this.author = null; 336 } else if (name.equals("time")) { 337 this.time = null; 338 } else if (name.equals("text")) { 339 this.text = null; 340 } else 341 super.removeChild(name, value); 342 343 } 344 345 @Override 346 public Base makeProperty(int hash, String name) throws FHIRException { 347 switch (hash) { 348 case 1475597077: 349 return getAuthor(); 350 case -1406328437: 351 return getAuthor(); 352 case 3560141: 353 return getTimeElement(); 354 case 3556653: 355 return getTextElement(); 356 default: 357 return super.makeProperty(hash, name); 358 } 359 360 } 361 362 @Override 363 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 364 switch (hash) { 365 case -1406328437: 366 /* author */ return new String[] { "Reference", "string" }; 367 case 3560141: 368 /* time */ return new String[] { "dateTime" }; 369 case 3556653: 370 /* text */ return new String[] { "markdown" }; 371 default: 372 return super.getTypesForProperty(hash, name); 373 } 374 375 } 376 377 @Override 378 public Base addChild(String name) throws FHIRException { 379 if (name.equals("authorReference")) { 380 this.author = new Reference(); 381 return this.author; 382 } else if (name.equals("authorString")) { 383 this.author = new StringType(); 384 return this.author; 385 } else if (name.equals("time")) { 386 throw new FHIRException("Cannot call addChild on a singleton property Annotation.time"); 387 } else if (name.equals("text")) { 388 throw new FHIRException("Cannot call addChild on a singleton property Annotation.text"); 389 } else 390 return super.addChild(name); 391 } 392 393 public String fhirType() { 394 return "Annotation"; 395 396 } 397 398 public Annotation copy() { 399 Annotation dst = new Annotation(); 400 copyValues(dst); 401 return dst; 402 } 403 404 public void copyValues(Annotation dst) { 405 super.copyValues(dst); 406 dst.author = author == null ? null : author.copy(); 407 dst.time = time == null ? null : time.copy(); 408 dst.text = text == null ? null : text.copy(); 409 } 410 411 protected Annotation typedCopy() { 412 return copy(); 413 } 414 415 @Override 416 public boolean equalsDeep(Base other_) { 417 if (!super.equalsDeep(other_)) 418 return false; 419 if (!(other_ instanceof Annotation)) 420 return false; 421 Annotation o = (Annotation) other_; 422 return compareDeep(author, o.author, true) && compareDeep(time, o.time, true) && compareDeep(text, o.text, true); 423 } 424 425 @Override 426 public boolean equalsShallow(Base other_) { 427 if (!super.equalsShallow(other_)) 428 return false; 429 if (!(other_ instanceof Annotation)) 430 return false; 431 Annotation o = (Annotation) other_; 432 return compareValues(time, o.time, true) && compareValues(text, o.text, true); 433 } 434 435 public boolean isEmpty() { 436 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(author, time, text); 437 } 438 439}