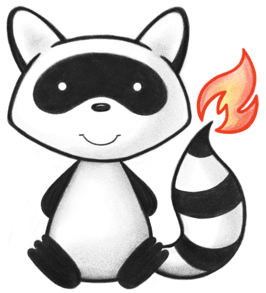
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * Base definition for all elements that are defined inside a resource - but not 046 * those in a data type. 047 */ 048@DatatypeDef(name = "BackboneElement") 049public abstract class BackboneElement extends Element implements IBaseBackboneElement { 050 051 /** 052 * May be used to represent additional information that is not part of the basic 053 * definition of the element and that modifies the understanding of the element 054 * in which it is contained and/or the understanding of the containing element's 055 * descendants. Usually modifier elements provide negation or qualification. To 056 * make the use of extensions safe and manageable, there is a strict set of 057 * governance applied to the definition and use of extensions. Though any 058 * implementer can define an extension, there is a set of requirements that 059 * SHALL be met as part of the definition of the extension. Applications 060 * processing a resource are required to check for modifier extensions. 061 * 062 * Modifier extensions SHALL NOT change the meaning of any elements on Resource 063 * or DomainResource (including cannot change the meaning of modifierExtension 064 * itself). 065 */ 066 @Child(name = "modifierExtension", type = { 067 Extension.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 068 @Description(shortDefinition = "Extensions that cannot be ignored even if unrecognized", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).") 069 protected List<Extension> modifierExtension; 070 071 private static final long serialVersionUID = -1431673179L; 072 073 /** 074 * Constructor 075 */ 076 public BackboneElement() { 077 super(); 078 } 079 080 /** 081 * @return {@link #modifierExtension} (May be used to represent additional 082 * information that is not part of the basic definition of the element 083 * and that modifies the understanding of the element in which it is 084 * contained and/or the understanding of the containing element's 085 * descendants. Usually modifier elements provide negation or 086 * qualification. To make the use of extensions safe and manageable, 087 * there is a strict set of governance applied to the definition and use 088 * of extensions. Though any implementer can define an extension, there 089 * is a set of requirements that SHALL be met as part of the definition 090 * of the extension. Applications processing a resource are required to 091 * check for modifier extensions. 092 * 093 * Modifier extensions SHALL NOT change the meaning of any elements on 094 * Resource or DomainResource (including cannot change the meaning of 095 * modifierExtension itself).) 096 */ 097 public List<Extension> getModifierExtension() { 098 if (this.modifierExtension == null) 099 this.modifierExtension = new ArrayList<Extension>(); 100 return this.modifierExtension; 101 } 102 103 /** 104 * @return Returns a reference to <code>this</code> for easy method chaining 105 */ 106 public BackboneElement setModifierExtension(List<Extension> theModifierExtension) { 107 this.modifierExtension = theModifierExtension; 108 return this; 109 } 110 111 public boolean hasModifierExtension() { 112 if (this.modifierExtension == null) 113 return false; 114 for (Extension item : this.modifierExtension) 115 if (!item.isEmpty()) 116 return true; 117 return false; 118 } 119 120 public Extension addModifierExtension() { // 3 121 Extension t = new Extension(); 122 if (this.modifierExtension == null) 123 this.modifierExtension = new ArrayList<Extension>(); 124 this.modifierExtension.add(t); 125 return t; 126 } 127 128 public BackboneElement addModifierExtension(Extension t) { // 3 129 if (t == null) 130 return this; 131 if (this.modifierExtension == null) 132 this.modifierExtension = new ArrayList<Extension>(); 133 this.modifierExtension.add(t); 134 return this; 135 } 136 137 /** 138 * @return The first repetition of repeating field {@link #modifierExtension}, 139 * creating it if it does not already exist 140 */ 141 public Extension getModifierExtensionFirstRep() { 142 if (getModifierExtension().isEmpty()) { 143 addModifierExtension(); 144 } 145 return getModifierExtension().get(0); 146 } 147 148 protected void listChildren(List<Property> children) { 149 super.listChildren(children); 150 children.add(new Property("modifierExtension", "Extension", 151 "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 152 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 153 } 154 155 @Override 156 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 157 switch (_hash) { 158 case -298878168: 159 /* modifierExtension */ return new Property("modifierExtension", "Extension", 160 "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 161 0, java.lang.Integer.MAX_VALUE, modifierExtension); 162 default: 163 return super.getNamedProperty(_hash, _name, _checkValid); 164 } 165 166 } 167 168 @Override 169 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 170 switch (hash) { 171 case -298878168: 172 /* modifierExtension */ return this.modifierExtension == null ? new Base[0] 173 : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 174 default: 175 return super.getProperty(hash, name, checkValid); 176 } 177 178 } 179 180 @Override 181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 182 switch (hash) { 183 case -298878168: // modifierExtension 184 this.getModifierExtension().add(castToExtension(value)); // Extension 185 return value; 186 default: 187 return super.setProperty(hash, name, value); 188 } 189 190 } 191 192 @Override 193 public Base setProperty(String name, Base value) throws FHIRException { 194 if (name.equals("modifierExtension")) { 195 this.getModifierExtension().add(castToExtension(value)); 196 } else 197 return super.setProperty(name, value); 198 return value; 199 } 200 201 @Override 202 public void removeChild(String name, Base value) throws FHIRException { 203 if (name.equals("modifierExtension")) { 204 this.getModifierExtension().remove(castToExtension(value)); 205 } else 206 super.removeChild(name, value); 207 208 } 209 210 @Override 211 public Base makeProperty(int hash, String name) throws FHIRException { 212 switch (hash) { 213 case -298878168: 214 return addModifierExtension(); 215 default: 216 return super.makeProperty(hash, name); 217 } 218 219 } 220 221 @Override 222 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 223 switch (hash) { 224 case -298878168: 225 /* modifierExtension */ return new String[] { "Extension" }; 226 default: 227 return super.getTypesForProperty(hash, name); 228 } 229 230 } 231 232 @Override 233 public Base addChild(String name) throws FHIRException { 234 if (name.equals("modifierExtension")) { 235 return addModifierExtension(); 236 } else 237 return super.addChild(name); 238 } 239 240 public String fhirType() { 241 return "BackboneElement"; 242 243 } 244 245 public abstract BackboneElement copy(); 246 247 public void copyValues(BackboneElement dst) { 248 super.copyValues(dst); 249 if (modifierExtension != null) { 250 dst.modifierExtension = new ArrayList<Extension>(); 251 for (Extension i : modifierExtension) 252 dst.modifierExtension.add(i.copy()); 253 } 254 ; 255 } 256 257 @Override 258 public boolean equalsDeep(Base other_) { 259 if (!super.equalsDeep(other_)) 260 return false; 261 if (!(other_ instanceof BackboneElement)) 262 return false; 263 BackboneElement o = (BackboneElement) other_; 264 return compareDeep(modifierExtension, o.modifierExtension, true); 265 } 266 267 @Override 268 public boolean equalsShallow(Base other_) { 269 if (!super.equalsShallow(other_)) 270 return false; 271 if (!(other_ instanceof BackboneElement)) 272 return false; 273 BackboneElement o = (BackboneElement) other_; 274 return true; 275 } 276 277 public boolean isEmpty() { 278 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifierExtension); 279 } 280 281 // added from java-adornments.txt: 282 283 public void checkNoModifiers(String noun, String verb) throws FHIRException { 284 if (hasModifierExtension()) { 285 throw new FHIRException("Found unknown Modifier Exceptions on " + noun + " doing " + verb); 286 } 287 288 } 289 290 public void addModifierExtension(String url, Type value) { 291 if (isDisallowExtensions()) 292 throw new Error("Extensions are not allowed in this context"); 293 Extension ex = new Extension(); 294 ex.setUrl(url); 295 ex.setValue(value); 296 getModifierExtension().add(ex); 297 } 298 299 300 @Override 301 public Extension getExtensionByUrl(String theUrl) { 302 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 303 ArrayList<Extension> retVal = new ArrayList<Extension>(); 304 Extension res = super.getExtensionByUrl(theUrl); 305 if (res != null) { 306 retVal.add(res); 307 } 308 for (Extension next : getModifierExtension()) { 309 if (theUrl.equals(next.getUrl())) { 310 retVal.add(next); 311 } 312 } 313 if (retVal.size() == 0) 314 return null; 315 else { 316 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 317 return retVal.get(0); 318 } 319 } 320 321 @Override 322 public void removeExtension(String theUrl) { 323 for (int i = getModifierExtension().size()-1; i >= 0; i--) { 324 if (theUrl.equals(getExtension().get(i).getUrl())) 325 getExtension().remove(i); 326 } 327 super.removeExtension(theUrl); 328 } 329 330 331 /** 332 * Returns an unmodifiable list containing all extensions on this element which 333 * match the given URL. 334 * 335 * @param theUrl The URL. Must not be blank or null. 336 * @return an unmodifiable list containing all extensions on this element which 337 * match the given URL 338 */ 339 @Override 340 public List<Extension> getExtensionsByUrl(String theUrl) { 341 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 342 ArrayList<Extension> retVal = new ArrayList<Extension>(); 343 retVal.addAll(super.getExtensionsByUrl(theUrl)); 344 for (Extension next : getModifierExtension()) { 345 if (theUrl.equals(next.getUrl())) { 346 retVal.add(next); 347 } 348 } 349 return java.util.Collections.unmodifiableList(retVal); 350 } 351 352 public void copyExtensions(org.hl7.fhir.r4.model.BackboneElement src, String... urls) { 353 super.copyExtensions(src,urls); 354 for (Extension e : src.getModifierExtension()) { 355 if (Utilities.existsInList(e.getUrl(), urls)) { 356 addModifierExtension(e.copy()); 357 } 358 } 359 } 360 361 public List<Extension> getExtensionsByUrl(String... theUrls) { 362 363 ArrayList<Extension> retVal = new ArrayList<>(); 364 for (Extension next : getModifierExtension()) { 365 if (Utilities.existsInList(next.getUrl(), theUrls)) { 366 retVal.add(next); 367 } 368 } 369 retVal.addAll(super.getExtensionsByUrl(theUrls)); 370 return java.util.Collections.unmodifiableList(retVal); 371 } 372 373 374 public boolean hasExtension(String... theUrls) { 375 for (Extension next : getModifierExtension()) { 376 if (Utilities.existsInList(next.getUrl(), theUrls)) { 377 return true; 378 } 379 } 380 return super.hasExtension(theUrls); 381 } 382 383 384 public boolean hasExtension(String theUrl) { 385 for (Extension ext : getModifierExtension()) { 386 if (theUrl.equals(ext.getUrl())) { 387 return true; 388 } 389 } 390 391 return super.hasExtension(theUrl); 392 } 393 394 395 public void copyNewExtensions(org.hl7.fhir.r4.model.BackboneElement src, String... urls) { 396 for (Extension e : src.getModifierExtension()) { 397 if (Utilities.existsInList(e.getUrl(), urls) && !!hasExtension(e.getUrl())) { 398 addExtension(e.copy()); 399 } 400 } 401 super.copyNewExtensions(src, urls); 402 } 403 // end addition 404 405}