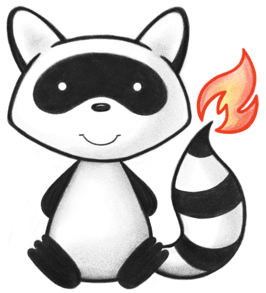
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.instance.model.api.IAnyResource; 033import org.hl7.fhir.instance.model.api.IBaseReference; 034import org.hl7.fhir.instance.model.api.IBaseResource; 035import org.hl7.fhir.instance.model.api.ICompositeType; 036import org.hl7.fhir.instance.model.api.IIdType; 037 038public abstract class BaseReference extends Type implements IBaseReference, ICompositeType { 039 040 /** 041 * This is not a part of the "wire format" resource, but can be changed/accessed 042 * by parsers 043 */ 044 private transient IBaseResource resource; 045 046 public BaseReference(String theReference) { 047 setReference(theReference); 048 } 049 050 public BaseReference(IIdType theReference) { 051 if (theReference != null) { 052 setReference(theReference.getValue()); 053 } else { 054 setReference(null); 055 } 056 } 057 058 public BaseReference(IAnyResource theResource) { 059 resource = theResource; 060 } 061 062 public BaseReference() { 063 } 064 065 /** 066 * Retrieves the actual resource referenced by this reference. Note that the 067 * resource itself is not a part of the FHIR "wire format" and is never 068 * transmitted or receieved inline, but this property may be changed/accessed by 069 * parsers. 070 */ 071 public IBaseResource getResource() { 072 return resource; 073 } 074 075 @Override 076 public IIdType getReferenceElement() { 077 return new IdType(getReference()); 078 } 079 080 abstract String getReference(); 081 082 /** 083 * Sets the actual resource referenced by this reference. Note that the resource 084 * itself is not a part of the FHIR "wire format" and is never transmitted or 085 * receieved inline, but this property may be changed/accessed by parsers. 086 */ 087 public IBaseReference setResource(IBaseResource theResource) { 088 resource = theResource; 089 return this; 090 } 091 092 @Override 093 public boolean isEmpty() { 094 return resource == null && super.isEmpty(); 095 } 096 097 @Override 098 public void copyValues(Element dst) { 099 super.copyValues(dst); 100 if (resource != null && dst instanceof BaseReference) { 101 ((BaseReference) dst).setResource(resource); 102 } 103 } 104 105}