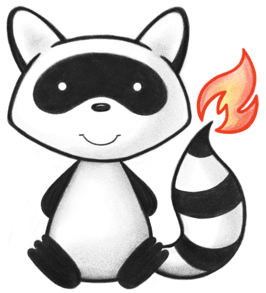
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * Record details about an anatomical structure. This resource may be used when 046 * a coded concept does not provide the necessary detail needed for the use 047 * case. 048 */ 049@ResourceDef(name = "BodyStructure", profile = "http://hl7.org/fhir/StructureDefinition/BodyStructure") 050public class BodyStructure extends DomainResource { 051 052 /** 053 * Identifier for this instance of the anatomical structure. 054 */ 055 @Child(name = "identifier", type = { 056 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 057 @Description(shortDefinition = "Bodystructure identifier", formalDefinition = "Identifier for this instance of the anatomical structure.") 058 protected List<Identifier> identifier; 059 060 /** 061 * Whether this body site is in active use. 062 */ 063 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 064 @Description(shortDefinition = "Whether this record is in active use", formalDefinition = "Whether this body site is in active use.") 065 protected BooleanType active; 066 067 /** 068 * The kind of structure being represented by the body structure at 069 * `BodyStructure.location`. This can define both normal and abnormal 070 * morphologies. 071 */ 072 @Child(name = "morphology", type = { 073 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 074 @Description(shortDefinition = "Kind of Structure", formalDefinition = "The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.") 075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/bodystructure-code") 076 protected CodeableConcept morphology; 077 078 /** 079 * The anatomical location or region of the specimen, lesion, or body structure. 080 */ 081 @Child(name = "location", type = { 082 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 083 @Description(shortDefinition = "Body site", formalDefinition = "The anatomical location or region of the specimen, lesion, or body structure.") 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 085 protected CodeableConcept location; 086 087 /** 088 * Qualifier to refine the anatomical location. These include qualifiers for 089 * laterality, relative location, directionality, number, and plane. 090 */ 091 @Child(name = "locationQualifier", type = { 092 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 093 @Description(shortDefinition = "Body site modifier", formalDefinition = "Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.") 094 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/bodystructure-relative-location") 095 protected List<CodeableConcept> locationQualifier; 096 097 /** 098 * A summary, characterization or explanation of the body structure. 099 */ 100 @Child(name = "description", type = { 101 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 102 @Description(shortDefinition = "Text description", formalDefinition = "A summary, characterization or explanation of the body structure.") 103 protected StringType description; 104 105 /** 106 * Image or images used to identify a location. 107 */ 108 @Child(name = "image", type = { 109 Attachment.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 110 @Description(shortDefinition = "Attached images", formalDefinition = "Image or images used to identify a location.") 111 protected List<Attachment> image; 112 113 /** 114 * The person to which the body site belongs. 115 */ 116 @Child(name = "patient", type = { Patient.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 117 @Description(shortDefinition = "Who this is about", formalDefinition = "The person to which the body site belongs.") 118 protected Reference patient; 119 120 /** 121 * The actual object that is the target of the reference (The person to which 122 * the body site belongs.) 123 */ 124 protected Patient patientTarget; 125 126 private static final long serialVersionUID = 1437500387L; 127 128 /** 129 * Constructor 130 */ 131 public BodyStructure() { 132 super(); 133 } 134 135 /** 136 * Constructor 137 */ 138 public BodyStructure(Reference patient) { 139 super(); 140 this.patient = patient; 141 } 142 143 /** 144 * @return {@link #identifier} (Identifier for this instance of the anatomical 145 * structure.) 146 */ 147 public List<Identifier> getIdentifier() { 148 if (this.identifier == null) 149 this.identifier = new ArrayList<Identifier>(); 150 return this.identifier; 151 } 152 153 /** 154 * @return Returns a reference to <code>this</code> for easy method chaining 155 */ 156 public BodyStructure setIdentifier(List<Identifier> theIdentifier) { 157 this.identifier = theIdentifier; 158 return this; 159 } 160 161 public boolean hasIdentifier() { 162 if (this.identifier == null) 163 return false; 164 for (Identifier item : this.identifier) 165 if (!item.isEmpty()) 166 return true; 167 return false; 168 } 169 170 public Identifier addIdentifier() { // 3 171 Identifier t = new Identifier(); 172 if (this.identifier == null) 173 this.identifier = new ArrayList<Identifier>(); 174 this.identifier.add(t); 175 return t; 176 } 177 178 public BodyStructure addIdentifier(Identifier t) { // 3 179 if (t == null) 180 return this; 181 if (this.identifier == null) 182 this.identifier = new ArrayList<Identifier>(); 183 this.identifier.add(t); 184 return this; 185 } 186 187 /** 188 * @return The first repetition of repeating field {@link #identifier}, creating 189 * it if it does not already exist 190 */ 191 public Identifier getIdentifierFirstRep() { 192 if (getIdentifier().isEmpty()) { 193 addIdentifier(); 194 } 195 return getIdentifier().get(0); 196 } 197 198 /** 199 * @return {@link #active} (Whether this body site is in active use.). This is 200 * the underlying object with id, value and extensions. The accessor 201 * "getActive" gives direct access to the value 202 */ 203 public BooleanType getActiveElement() { 204 if (this.active == null) 205 if (Configuration.errorOnAutoCreate()) 206 throw new Error("Attempt to auto-create BodyStructure.active"); 207 else if (Configuration.doAutoCreate()) 208 this.active = new BooleanType(); // bb 209 return this.active; 210 } 211 212 public boolean hasActiveElement() { 213 return this.active != null && !this.active.isEmpty(); 214 } 215 216 public boolean hasActive() { 217 return this.active != null && !this.active.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #active} (Whether this body site is in active use.). This 222 * is the underlying object with id, value and extensions. The 223 * accessor "getActive" gives direct access to the value 224 */ 225 public BodyStructure setActiveElement(BooleanType value) { 226 this.active = value; 227 return this; 228 } 229 230 /** 231 * @return Whether this body site is in active use. 232 */ 233 public boolean getActive() { 234 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 235 } 236 237 /** 238 * @param value Whether this body site is in active use. 239 */ 240 public BodyStructure setActive(boolean value) { 241 if (this.active == null) 242 this.active = new BooleanType(); 243 this.active.setValue(value); 244 return this; 245 } 246 247 /** 248 * @return {@link #morphology} (The kind of structure being represented by the 249 * body structure at `BodyStructure.location`. This can define both 250 * normal and abnormal morphologies.) 251 */ 252 public CodeableConcept getMorphology() { 253 if (this.morphology == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create BodyStructure.morphology"); 256 else if (Configuration.doAutoCreate()) 257 this.morphology = new CodeableConcept(); // cc 258 return this.morphology; 259 } 260 261 public boolean hasMorphology() { 262 return this.morphology != null && !this.morphology.isEmpty(); 263 } 264 265 /** 266 * @param value {@link #morphology} (The kind of structure being represented by 267 * the body structure at `BodyStructure.location`. This can define 268 * both normal and abnormal morphologies.) 269 */ 270 public BodyStructure setMorphology(CodeableConcept value) { 271 this.morphology = value; 272 return this; 273 } 274 275 /** 276 * @return {@link #location} (The anatomical location or region of the specimen, 277 * lesion, or body structure.) 278 */ 279 public CodeableConcept getLocation() { 280 if (this.location == null) 281 if (Configuration.errorOnAutoCreate()) 282 throw new Error("Attempt to auto-create BodyStructure.location"); 283 else if (Configuration.doAutoCreate()) 284 this.location = new CodeableConcept(); // cc 285 return this.location; 286 } 287 288 public boolean hasLocation() { 289 return this.location != null && !this.location.isEmpty(); 290 } 291 292 /** 293 * @param value {@link #location} (The anatomical location or region of the 294 * specimen, lesion, or body structure.) 295 */ 296 public BodyStructure setLocation(CodeableConcept value) { 297 this.location = value; 298 return this; 299 } 300 301 /** 302 * @return {@link #locationQualifier} (Qualifier to refine the anatomical 303 * location. These include qualifiers for laterality, relative location, 304 * directionality, number, and plane.) 305 */ 306 public List<CodeableConcept> getLocationQualifier() { 307 if (this.locationQualifier == null) 308 this.locationQualifier = new ArrayList<CodeableConcept>(); 309 return this.locationQualifier; 310 } 311 312 /** 313 * @return Returns a reference to <code>this</code> for easy method chaining 314 */ 315 public BodyStructure setLocationQualifier(List<CodeableConcept> theLocationQualifier) { 316 this.locationQualifier = theLocationQualifier; 317 return this; 318 } 319 320 public boolean hasLocationQualifier() { 321 if (this.locationQualifier == null) 322 return false; 323 for (CodeableConcept item : this.locationQualifier) 324 if (!item.isEmpty()) 325 return true; 326 return false; 327 } 328 329 public CodeableConcept addLocationQualifier() { // 3 330 CodeableConcept t = new CodeableConcept(); 331 if (this.locationQualifier == null) 332 this.locationQualifier = new ArrayList<CodeableConcept>(); 333 this.locationQualifier.add(t); 334 return t; 335 } 336 337 public BodyStructure addLocationQualifier(CodeableConcept t) { // 3 338 if (t == null) 339 return this; 340 if (this.locationQualifier == null) 341 this.locationQualifier = new ArrayList<CodeableConcept>(); 342 this.locationQualifier.add(t); 343 return this; 344 } 345 346 /** 347 * @return The first repetition of repeating field {@link #locationQualifier}, 348 * creating it if it does not already exist 349 */ 350 public CodeableConcept getLocationQualifierFirstRep() { 351 if (getLocationQualifier().isEmpty()) { 352 addLocationQualifier(); 353 } 354 return getLocationQualifier().get(0); 355 } 356 357 /** 358 * @return {@link #description} (A summary, characterization or explanation of 359 * the body structure.). This is the underlying object with id, value 360 * and extensions. The accessor "getDescription" gives direct access to 361 * the value 362 */ 363 public StringType getDescriptionElement() { 364 if (this.description == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create BodyStructure.description"); 367 else if (Configuration.doAutoCreate()) 368 this.description = new StringType(); // bb 369 return this.description; 370 } 371 372 public boolean hasDescriptionElement() { 373 return this.description != null && !this.description.isEmpty(); 374 } 375 376 public boolean hasDescription() { 377 return this.description != null && !this.description.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #description} (A summary, characterization or explanation 382 * of the body structure.). This is the underlying object with id, 383 * value and extensions. The accessor "getDescription" gives direct 384 * access to the value 385 */ 386 public BodyStructure setDescriptionElement(StringType value) { 387 this.description = value; 388 return this; 389 } 390 391 /** 392 * @return A summary, characterization or explanation of the body structure. 393 */ 394 public String getDescription() { 395 return this.description == null ? null : this.description.getValue(); 396 } 397 398 /** 399 * @param value A summary, characterization or explanation of the body 400 * structure. 401 */ 402 public BodyStructure setDescription(String value) { 403 if (Utilities.noString(value)) 404 this.description = null; 405 else { 406 if (this.description == null) 407 this.description = new StringType(); 408 this.description.setValue(value); 409 } 410 return this; 411 } 412 413 /** 414 * @return {@link #image} (Image or images used to identify a location.) 415 */ 416 public List<Attachment> getImage() { 417 if (this.image == null) 418 this.image = new ArrayList<Attachment>(); 419 return this.image; 420 } 421 422 /** 423 * @return Returns a reference to <code>this</code> for easy method chaining 424 */ 425 public BodyStructure setImage(List<Attachment> theImage) { 426 this.image = theImage; 427 return this; 428 } 429 430 public boolean hasImage() { 431 if (this.image == null) 432 return false; 433 for (Attachment item : this.image) 434 if (!item.isEmpty()) 435 return true; 436 return false; 437 } 438 439 public Attachment addImage() { // 3 440 Attachment t = new Attachment(); 441 if (this.image == null) 442 this.image = new ArrayList<Attachment>(); 443 this.image.add(t); 444 return t; 445 } 446 447 public BodyStructure addImage(Attachment t) { // 3 448 if (t == null) 449 return this; 450 if (this.image == null) 451 this.image = new ArrayList<Attachment>(); 452 this.image.add(t); 453 return this; 454 } 455 456 /** 457 * @return The first repetition of repeating field {@link #image}, creating it 458 * if it does not already exist 459 */ 460 public Attachment getImageFirstRep() { 461 if (getImage().isEmpty()) { 462 addImage(); 463 } 464 return getImage().get(0); 465 } 466 467 /** 468 * @return {@link #patient} (The person to which the body site belongs.) 469 */ 470 public Reference getPatient() { 471 if (this.patient == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create BodyStructure.patient"); 474 else if (Configuration.doAutoCreate()) 475 this.patient = new Reference(); // cc 476 return this.patient; 477 } 478 479 public boolean hasPatient() { 480 return this.patient != null && !this.patient.isEmpty(); 481 } 482 483 /** 484 * @param value {@link #patient} (The person to which the body site belongs.) 485 */ 486 public BodyStructure setPatient(Reference value) { 487 this.patient = value; 488 return this; 489 } 490 491 /** 492 * @return {@link #patient} The actual object that is the target of the 493 * reference. The reference library doesn't populate this, but you can 494 * use it to hold the resource if you resolve it. (The person to which 495 * the body site belongs.) 496 */ 497 public Patient getPatientTarget() { 498 if (this.patientTarget == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create BodyStructure.patient"); 501 else if (Configuration.doAutoCreate()) 502 this.patientTarget = new Patient(); // aa 503 return this.patientTarget; 504 } 505 506 /** 507 * @param value {@link #patient} The actual object that is the target of the 508 * reference. The reference library doesn't use these, but you can 509 * use it to hold the resource if you resolve it. (The person to 510 * which the body site belongs.) 511 */ 512 public BodyStructure setPatientTarget(Patient value) { 513 this.patientTarget = value; 514 return this; 515 } 516 517 protected void listChildren(List<Property> children) { 518 super.listChildren(children); 519 children.add(new Property("identifier", "Identifier", "Identifier for this instance of the anatomical structure.", 520 0, java.lang.Integer.MAX_VALUE, identifier)); 521 children.add(new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active)); 522 children.add(new Property("morphology", "CodeableConcept", 523 "The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.", 524 0, 1, morphology)); 525 children.add(new Property("location", "CodeableConcept", 526 "The anatomical location or region of the specimen, lesion, or body structure.", 0, 1, location)); 527 children.add(new Property("locationQualifier", "CodeableConcept", 528 "Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.", 529 0, java.lang.Integer.MAX_VALUE, locationQualifier)); 530 children.add(new Property("description", "string", 531 "A summary, characterization or explanation of the body structure.", 0, 1, description)); 532 children.add(new Property("image", "Attachment", "Image or images used to identify a location.", 0, 533 java.lang.Integer.MAX_VALUE, image)); 534 children.add( 535 new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 1, patient)); 536 } 537 538 @Override 539 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 540 switch (_hash) { 541 case -1618432855: 542 /* identifier */ return new Property("identifier", "Identifier", 543 "Identifier for this instance of the anatomical structure.", 0, java.lang.Integer.MAX_VALUE, identifier); 544 case -1422950650: 545 /* active */ return new Property("active", "boolean", "Whether this body site is in active use.", 0, 1, active); 546 case 1807231644: 547 /* morphology */ return new Property("morphology", "CodeableConcept", 548 "The kind of structure being represented by the body structure at `BodyStructure.location`. This can define both normal and abnormal morphologies.", 549 0, 1, morphology); 550 case 1901043637: 551 /* location */ return new Property("location", "CodeableConcept", 552 "The anatomical location or region of the specimen, lesion, or body structure.", 0, 1, location); 553 case 433081461: 554 /* locationQualifier */ return new Property("locationQualifier", "CodeableConcept", 555 "Qualifier to refine the anatomical location. These include qualifiers for laterality, relative location, directionality, number, and plane.", 556 0, java.lang.Integer.MAX_VALUE, locationQualifier); 557 case -1724546052: 558 /* description */ return new Property("description", "string", 559 "A summary, characterization or explanation of the body structure.", 0, 1, description); 560 case 100313435: 561 /* image */ return new Property("image", "Attachment", "Image or images used to identify a location.", 0, 562 java.lang.Integer.MAX_VALUE, image); 563 case -791418107: 564 /* patient */ return new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 565 0, 1, patient); 566 default: 567 return super.getNamedProperty(_hash, _name, _checkValid); 568 } 569 570 } 571 572 @Override 573 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 574 switch (hash) { 575 case -1618432855: 576 /* identifier */ return this.identifier == null ? new Base[0] 577 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 578 case -1422950650: 579 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 580 case 1807231644: 581 /* morphology */ return this.morphology == null ? new Base[0] : new Base[] { this.morphology }; // CodeableConcept 582 case 1901043637: 583 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // CodeableConcept 584 case 433081461: 585 /* locationQualifier */ return this.locationQualifier == null ? new Base[0] 586 : this.locationQualifier.toArray(new Base[this.locationQualifier.size()]); // CodeableConcept 587 case -1724546052: 588 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 589 case 100313435: 590 /* image */ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 591 case -791418107: 592 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 593 default: 594 return super.getProperty(hash, name, checkValid); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(int hash, String name, Base value) throws FHIRException { 601 switch (hash) { 602 case -1618432855: // identifier 603 this.getIdentifier().add(castToIdentifier(value)); // Identifier 604 return value; 605 case -1422950650: // active 606 this.active = castToBoolean(value); // BooleanType 607 return value; 608 case 1807231644: // morphology 609 this.morphology = castToCodeableConcept(value); // CodeableConcept 610 return value; 611 case 1901043637: // location 612 this.location = castToCodeableConcept(value); // CodeableConcept 613 return value; 614 case 433081461: // locationQualifier 615 this.getLocationQualifier().add(castToCodeableConcept(value)); // CodeableConcept 616 return value; 617 case -1724546052: // description 618 this.description = castToString(value); // StringType 619 return value; 620 case 100313435: // image 621 this.getImage().add(castToAttachment(value)); // Attachment 622 return value; 623 case -791418107: // patient 624 this.patient = castToReference(value); // Reference 625 return value; 626 default: 627 return super.setProperty(hash, name, value); 628 } 629 630 } 631 632 @Override 633 public Base setProperty(String name, Base value) throws FHIRException { 634 if (name.equals("identifier")) { 635 this.getIdentifier().add(castToIdentifier(value)); 636 } else if (name.equals("active")) { 637 this.active = castToBoolean(value); // BooleanType 638 } else if (name.equals("morphology")) { 639 this.morphology = castToCodeableConcept(value); // CodeableConcept 640 } else if (name.equals("location")) { 641 this.location = castToCodeableConcept(value); // CodeableConcept 642 } else if (name.equals("locationQualifier")) { 643 this.getLocationQualifier().add(castToCodeableConcept(value)); 644 } else if (name.equals("description")) { 645 this.description = castToString(value); // StringType 646 } else if (name.equals("image")) { 647 this.getImage().add(castToAttachment(value)); 648 } else if (name.equals("patient")) { 649 this.patient = castToReference(value); // Reference 650 } else 651 return super.setProperty(name, value); 652 return value; 653 } 654 655 @Override 656 public void removeChild(String name, Base value) throws FHIRException { 657 if (name.equals("identifier")) { 658 this.getIdentifier().remove(castToIdentifier(value)); 659 } else if (name.equals("active")) { 660 this.active = null; 661 } else if (name.equals("morphology")) { 662 this.morphology = null; 663 } else if (name.equals("location")) { 664 this.location = null; 665 } else if (name.equals("locationQualifier")) { 666 this.getLocationQualifier().remove(castToCodeableConcept(value)); 667 } else if (name.equals("description")) { 668 this.description = null; 669 } else if (name.equals("image")) { 670 this.getImage().remove(castToAttachment(value)); 671 } else if (name.equals("patient")) { 672 this.patient = null; 673 } else 674 super.removeChild(name, value); 675 676 } 677 678 @Override 679 public Base makeProperty(int hash, String name) throws FHIRException { 680 switch (hash) { 681 case -1618432855: 682 return addIdentifier(); 683 case -1422950650: 684 return getActiveElement(); 685 case 1807231644: 686 return getMorphology(); 687 case 1901043637: 688 return getLocation(); 689 case 433081461: 690 return addLocationQualifier(); 691 case -1724546052: 692 return getDescriptionElement(); 693 case 100313435: 694 return addImage(); 695 case -791418107: 696 return getPatient(); 697 default: 698 return super.makeProperty(hash, name); 699 } 700 701 } 702 703 @Override 704 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 705 switch (hash) { 706 case -1618432855: 707 /* identifier */ return new String[] { "Identifier" }; 708 case -1422950650: 709 /* active */ return new String[] { "boolean" }; 710 case 1807231644: 711 /* morphology */ return new String[] { "CodeableConcept" }; 712 case 1901043637: 713 /* location */ return new String[] { "CodeableConcept" }; 714 case 433081461: 715 /* locationQualifier */ return new String[] { "CodeableConcept" }; 716 case -1724546052: 717 /* description */ return new String[] { "string" }; 718 case 100313435: 719 /* image */ return new String[] { "Attachment" }; 720 case -791418107: 721 /* patient */ return new String[] { "Reference" }; 722 default: 723 return super.getTypesForProperty(hash, name); 724 } 725 726 } 727 728 @Override 729 public Base addChild(String name) throws FHIRException { 730 if (name.equals("identifier")) { 731 return addIdentifier(); 732 } else if (name.equals("active")) { 733 throw new FHIRException("Cannot call addChild on a singleton property BodyStructure.active"); 734 } else if (name.equals("morphology")) { 735 this.morphology = new CodeableConcept(); 736 return this.morphology; 737 } else if (name.equals("location")) { 738 this.location = new CodeableConcept(); 739 return this.location; 740 } else if (name.equals("locationQualifier")) { 741 return addLocationQualifier(); 742 } else if (name.equals("description")) { 743 throw new FHIRException("Cannot call addChild on a singleton property BodyStructure.description"); 744 } else if (name.equals("image")) { 745 return addImage(); 746 } else if (name.equals("patient")) { 747 this.patient = new Reference(); 748 return this.patient; 749 } else 750 return super.addChild(name); 751 } 752 753 public String fhirType() { 754 return "BodyStructure"; 755 756 } 757 758 public BodyStructure copy() { 759 BodyStructure dst = new BodyStructure(); 760 copyValues(dst); 761 return dst; 762 } 763 764 public void copyValues(BodyStructure dst) { 765 super.copyValues(dst); 766 if (identifier != null) { 767 dst.identifier = new ArrayList<Identifier>(); 768 for (Identifier i : identifier) 769 dst.identifier.add(i.copy()); 770 } 771 ; 772 dst.active = active == null ? null : active.copy(); 773 dst.morphology = morphology == null ? null : morphology.copy(); 774 dst.location = location == null ? null : location.copy(); 775 if (locationQualifier != null) { 776 dst.locationQualifier = new ArrayList<CodeableConcept>(); 777 for (CodeableConcept i : locationQualifier) 778 dst.locationQualifier.add(i.copy()); 779 } 780 ; 781 dst.description = description == null ? null : description.copy(); 782 if (image != null) { 783 dst.image = new ArrayList<Attachment>(); 784 for (Attachment i : image) 785 dst.image.add(i.copy()); 786 } 787 ; 788 dst.patient = patient == null ? null : patient.copy(); 789 } 790 791 protected BodyStructure typedCopy() { 792 return copy(); 793 } 794 795 @Override 796 public boolean equalsDeep(Base other_) { 797 if (!super.equalsDeep(other_)) 798 return false; 799 if (!(other_ instanceof BodyStructure)) 800 return false; 801 BodyStructure o = (BodyStructure) other_; 802 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 803 && compareDeep(morphology, o.morphology, true) && compareDeep(location, o.location, true) 804 && compareDeep(locationQualifier, o.locationQualifier, true) && compareDeep(description, o.description, true) 805 && compareDeep(image, o.image, true) && compareDeep(patient, o.patient, true); 806 } 807 808 @Override 809 public boolean equalsShallow(Base other_) { 810 if (!super.equalsShallow(other_)) 811 return false; 812 if (!(other_ instanceof BodyStructure)) 813 return false; 814 BodyStructure o = (BodyStructure) other_; 815 return compareValues(active, o.active, true) && compareValues(description, o.description, true); 816 } 817 818 public boolean isEmpty() { 819 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, morphology, location, 820 locationQualifier, description, image, patient); 821 } 822 823 @Override 824 public ResourceType getResourceType() { 825 return ResourceType.BodyStructure; 826 } 827 828 /** 829 * Search parameter: <b>identifier</b> 830 * <p> 831 * Description: <b>Bodystructure identifier</b><br> 832 * Type: <b>token</b><br> 833 * Path: <b>BodyStructure.identifier</b><br> 834 * </p> 835 */ 836 @SearchParamDefinition(name = "identifier", path = "BodyStructure.identifier", description = "Bodystructure identifier", type = "token") 837 public static final String SP_IDENTIFIER = "identifier"; 838 /** 839 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 840 * <p> 841 * Description: <b>Bodystructure identifier</b><br> 842 * Type: <b>token</b><br> 843 * Path: <b>BodyStructure.identifier</b><br> 844 * </p> 845 */ 846 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 847 SP_IDENTIFIER); 848 849 /** 850 * Search parameter: <b>morphology</b> 851 * <p> 852 * Description: <b>Kind of Structure</b><br> 853 * Type: <b>token</b><br> 854 * Path: <b>BodyStructure.morphology</b><br> 855 * </p> 856 */ 857 @SearchParamDefinition(name = "morphology", path = "BodyStructure.morphology", description = "Kind of Structure", type = "token") 858 public static final String SP_MORPHOLOGY = "morphology"; 859 /** 860 * <b>Fluent Client</b> search parameter constant for <b>morphology</b> 861 * <p> 862 * Description: <b>Kind of Structure</b><br> 863 * Type: <b>token</b><br> 864 * Path: <b>BodyStructure.morphology</b><br> 865 * </p> 866 */ 867 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MORPHOLOGY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 868 SP_MORPHOLOGY); 869 870 /** 871 * Search parameter: <b>patient</b> 872 * <p> 873 * Description: <b>Who this is about</b><br> 874 * Type: <b>reference</b><br> 875 * Path: <b>BodyStructure.patient</b><br> 876 * </p> 877 */ 878 @SearchParamDefinition(name = "patient", path = "BodyStructure.patient", description = "Who this is about", type = "reference", providesMembershipIn = { 879 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 880 public static final String SP_PATIENT = "patient"; 881 /** 882 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 883 * <p> 884 * Description: <b>Who this is about</b><br> 885 * Type: <b>reference</b><br> 886 * Path: <b>BodyStructure.patient</b><br> 887 * </p> 888 */ 889 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 890 SP_PATIENT); 891 892 /** 893 * Constant for fluent queries to be used to add include statements. Specifies 894 * the path value of "<b>BodyStructure:patient</b>". 895 */ 896 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 897 "BodyStructure:patient").toLocked(); 898 899 /** 900 * Search parameter: <b>location</b> 901 * <p> 902 * Description: <b>Body site</b><br> 903 * Type: <b>token</b><br> 904 * Path: <b>BodyStructure.location</b><br> 905 * </p> 906 */ 907 @SearchParamDefinition(name = "location", path = "BodyStructure.location", description = "Body site", type = "token") 908 public static final String SP_LOCATION = "location"; 909 /** 910 * <b>Fluent Client</b> search parameter constant for <b>location</b> 911 * <p> 912 * Description: <b>Body site</b><br> 913 * Type: <b>token</b><br> 914 * Path: <b>BodyStructure.location</b><br> 915 * </p> 916 */ 917 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOCATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 918 SP_LOCATION); 919 920}