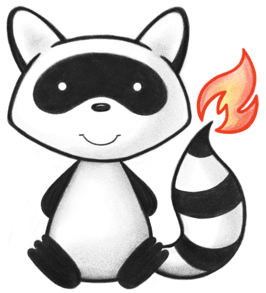
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The Care Team includes all the people and organizations who plan to 048 * participate in the coordination and delivery of care for a patient. 049 */ 050@ResourceDef(name = "CareTeam", profile = "http://hl7.org/fhir/StructureDefinition/CareTeam") 051public class CareTeam extends DomainResource { 052 053 public enum CareTeamStatus { 054 /** 055 * The care team has been drafted and proposed, but not yet participating in the 056 * coordination and delivery of patient care. 057 */ 058 PROPOSED, 059 /** 060 * The care team is currently participating in the coordination and delivery of 061 * care. 062 */ 063 ACTIVE, 064 /** 065 * The care team is temporarily on hold or suspended and not participating in 066 * the coordination and delivery of care. 067 */ 068 SUSPENDED, 069 /** 070 * The care team was, but is no longer, participating in the coordination and 071 * delivery of care. 072 */ 073 INACTIVE, 074 /** 075 * The care team should have never existed. 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static CareTeamStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("proposed".equals(codeString)) 087 return PROPOSED; 088 if ("active".equals(codeString)) 089 return ACTIVE; 090 if ("suspended".equals(codeString)) 091 return SUSPENDED; 092 if ("inactive".equals(codeString)) 093 return INACTIVE; 094 if ("entered-in-error".equals(codeString)) 095 return ENTEREDINERROR; 096 if (Configuration.isAcceptInvalidEnums()) 097 return null; 098 else 099 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 100 } 101 102 public String toCode() { 103 switch (this) { 104 case PROPOSED: 105 return "proposed"; 106 case ACTIVE: 107 return "active"; 108 case SUSPENDED: 109 return "suspended"; 110 case INACTIVE: 111 return "inactive"; 112 case ENTEREDINERROR: 113 return "entered-in-error"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 switch (this) { 123 case PROPOSED: 124 return "http://hl7.org/fhir/care-team-status"; 125 case ACTIVE: 126 return "http://hl7.org/fhir/care-team-status"; 127 case SUSPENDED: 128 return "http://hl7.org/fhir/care-team-status"; 129 case INACTIVE: 130 return "http://hl7.org/fhir/care-team-status"; 131 case ENTEREDINERROR: 132 return "http://hl7.org/fhir/care-team-status"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case PROPOSED: 143 return "The care team has been drafted and proposed, but not yet participating in the coordination and delivery of patient care."; 144 case ACTIVE: 145 return "The care team is currently participating in the coordination and delivery of care."; 146 case SUSPENDED: 147 return "The care team is temporarily on hold or suspended and not participating in the coordination and delivery of care."; 148 case INACTIVE: 149 return "The care team was, but is no longer, participating in the coordination and delivery of care."; 150 case ENTEREDINERROR: 151 return "The care team should have never existed."; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDisplay() { 160 switch (this) { 161 case PROPOSED: 162 return "Proposed"; 163 case ACTIVE: 164 return "Active"; 165 case SUSPENDED: 166 return "Suspended"; 167 case INACTIVE: 168 return "Inactive"; 169 case ENTEREDINERROR: 170 return "Entered in Error"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 } 178 179 public static class CareTeamStatusEnumFactory implements EnumFactory<CareTeamStatus> { 180 public CareTeamStatus fromCode(String codeString) throws IllegalArgumentException { 181 if (codeString == null || "".equals(codeString)) 182 if (codeString == null || "".equals(codeString)) 183 return null; 184 if ("proposed".equals(codeString)) 185 return CareTeamStatus.PROPOSED; 186 if ("active".equals(codeString)) 187 return CareTeamStatus.ACTIVE; 188 if ("suspended".equals(codeString)) 189 return CareTeamStatus.SUSPENDED; 190 if ("inactive".equals(codeString)) 191 return CareTeamStatus.INACTIVE; 192 if ("entered-in-error".equals(codeString)) 193 return CareTeamStatus.ENTEREDINERROR; 194 throw new IllegalArgumentException("Unknown CareTeamStatus code '" + codeString + "'"); 195 } 196 197 public Enumeration<CareTeamStatus> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.NULL, code); 205 if ("proposed".equals(codeString)) 206 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.PROPOSED, code); 207 if ("active".equals(codeString)) 208 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ACTIVE, code); 209 if ("suspended".equals(codeString)) 210 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.SUSPENDED, code); 211 if ("inactive".equals(codeString)) 212 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.INACTIVE, code); 213 if ("entered-in-error".equals(codeString)) 214 return new Enumeration<CareTeamStatus>(this, CareTeamStatus.ENTEREDINERROR, code); 215 throw new FHIRException("Unknown CareTeamStatus code '" + codeString + "'"); 216 } 217 218 public String toCode(CareTeamStatus code) { 219 if (code == CareTeamStatus.NULL) 220 return null; 221 if (code == CareTeamStatus.PROPOSED) 222 return "proposed"; 223 if (code == CareTeamStatus.ACTIVE) 224 return "active"; 225 if (code == CareTeamStatus.SUSPENDED) 226 return "suspended"; 227 if (code == CareTeamStatus.INACTIVE) 228 return "inactive"; 229 if (code == CareTeamStatus.ENTEREDINERROR) 230 return "entered-in-error"; 231 return "?"; 232 } 233 234 public String toSystem(CareTeamStatus code) { 235 return code.getSystem(); 236 } 237 } 238 239 @Block() 240 public static class CareTeamParticipantComponent extends BackboneElement implements IBaseBackboneElement { 241 /** 242 * Indicates specific responsibility of an individual within the care team, such 243 * as "Primary care physician", "Trained social worker counselor", "Caregiver", 244 * etc. 245 */ 246 @Child(name = "role", type = { 247 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 248 @Description(shortDefinition = "Type of involvement", formalDefinition = "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.") 249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participant-role") 250 protected List<CodeableConcept> role; 251 252 /** 253 * The specific person or organization who is participating/expected to 254 * participate in the care team. 255 */ 256 @Child(name = "member", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 257 Organization.class, CareTeam.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 258 @Description(shortDefinition = "Who is involved", formalDefinition = "The specific person or organization who is participating/expected to participate in the care team.") 259 protected Reference member; 260 261 /** 262 * The actual object that is the target of the reference (The specific person or 263 * organization who is participating/expected to participate in the care team.) 264 */ 265 protected Resource memberTarget; 266 267 /** 268 * The organization of the practitioner. 269 */ 270 @Child(name = "onBehalfOf", type = { 271 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 272 @Description(shortDefinition = "Organization of the practitioner", formalDefinition = "The organization of the practitioner.") 273 protected Reference onBehalfOf; 274 275 /** 276 * The actual object that is the target of the reference (The organization of 277 * the practitioner.) 278 */ 279 protected Organization onBehalfOfTarget; 280 281 /** 282 * Indicates when the specific member or organization did (or is intended to) 283 * come into effect and end. 284 */ 285 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 286 @Description(shortDefinition = "Time period of participant", formalDefinition = "Indicates when the specific member or organization did (or is intended to) come into effect and end.") 287 protected Period period; 288 289 private static final long serialVersionUID = -575634410L; 290 291 /** 292 * Constructor 293 */ 294 public CareTeamParticipantComponent() { 295 super(); 296 } 297 298 /** 299 * @return {@link #role} (Indicates specific responsibility of an individual 300 * within the care team, such as "Primary care physician", "Trained 301 * social worker counselor", "Caregiver", etc.) 302 */ 303 public List<CodeableConcept> getRole() { 304 if (this.role == null) 305 this.role = new ArrayList<CodeableConcept>(); 306 return this.role; 307 } 308 309 /** 310 * @return Returns a reference to <code>this</code> for easy method chaining 311 */ 312 public CareTeamParticipantComponent setRole(List<CodeableConcept> theRole) { 313 this.role = theRole; 314 return this; 315 } 316 317 public boolean hasRole() { 318 if (this.role == null) 319 return false; 320 for (CodeableConcept item : this.role) 321 if (!item.isEmpty()) 322 return true; 323 return false; 324 } 325 326 public CodeableConcept addRole() { // 3 327 CodeableConcept t = new CodeableConcept(); 328 if (this.role == null) 329 this.role = new ArrayList<CodeableConcept>(); 330 this.role.add(t); 331 return t; 332 } 333 334 public CareTeamParticipantComponent addRole(CodeableConcept t) { // 3 335 if (t == null) 336 return this; 337 if (this.role == null) 338 this.role = new ArrayList<CodeableConcept>(); 339 this.role.add(t); 340 return this; 341 } 342 343 /** 344 * @return The first repetition of repeating field {@link #role}, creating it if 345 * it does not already exist 346 */ 347 public CodeableConcept getRoleFirstRep() { 348 if (getRole().isEmpty()) { 349 addRole(); 350 } 351 return getRole().get(0); 352 } 353 354 /** 355 * @return {@link #member} (The specific person or organization who is 356 * participating/expected to participate in the care team.) 357 */ 358 public Reference getMember() { 359 if (this.member == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create CareTeamParticipantComponent.member"); 362 else if (Configuration.doAutoCreate()) 363 this.member = new Reference(); // cc 364 return this.member; 365 } 366 367 public boolean hasMember() { 368 return this.member != null && !this.member.isEmpty(); 369 } 370 371 /** 372 * @param value {@link #member} (The specific person or organization who is 373 * participating/expected to participate in the care team.) 374 */ 375 public CareTeamParticipantComponent setMember(Reference value) { 376 this.member = value; 377 return this; 378 } 379 380 /** 381 * @return {@link #member} The actual object that is the target of the 382 * reference. The reference library doesn't populate this, but you can 383 * use it to hold the resource if you resolve it. (The specific person 384 * or organization who is participating/expected to participate in the 385 * care team.) 386 */ 387 public Resource getMemberTarget() { 388 return this.memberTarget; 389 } 390 391 /** 392 * @param value {@link #member} The actual object that is the target of the 393 * reference. The reference library doesn't use these, but you can 394 * use it to hold the resource if you resolve it. (The specific 395 * person or organization who is participating/expected to 396 * participate in the care team.) 397 */ 398 public CareTeamParticipantComponent setMemberTarget(Resource value) { 399 this.memberTarget = value; 400 return this; 401 } 402 403 /** 404 * @return {@link #onBehalfOf} (The organization of the practitioner.) 405 */ 406 public Reference getOnBehalfOf() { 407 if (this.onBehalfOf == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 410 else if (Configuration.doAutoCreate()) 411 this.onBehalfOf = new Reference(); // cc 412 return this.onBehalfOf; 413 } 414 415 public boolean hasOnBehalfOf() { 416 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #onBehalfOf} (The organization of the practitioner.) 421 */ 422 public CareTeamParticipantComponent setOnBehalfOf(Reference value) { 423 this.onBehalfOf = value; 424 return this; 425 } 426 427 /** 428 * @return {@link #onBehalfOf} The actual object that is the target of the 429 * reference. The reference library doesn't populate this, but you can 430 * use it to hold the resource if you resolve it. (The organization of 431 * the practitioner.) 432 */ 433 public Organization getOnBehalfOfTarget() { 434 if (this.onBehalfOfTarget == null) 435 if (Configuration.errorOnAutoCreate()) 436 throw new Error("Attempt to auto-create CareTeamParticipantComponent.onBehalfOf"); 437 else if (Configuration.doAutoCreate()) 438 this.onBehalfOfTarget = new Organization(); // aa 439 return this.onBehalfOfTarget; 440 } 441 442 /** 443 * @param value {@link #onBehalfOf} The actual object that is the target of the 444 * reference. The reference library doesn't use these, but you can 445 * use it to hold the resource if you resolve it. (The organization 446 * of the practitioner.) 447 */ 448 public CareTeamParticipantComponent setOnBehalfOfTarget(Organization value) { 449 this.onBehalfOfTarget = value; 450 return this; 451 } 452 453 /** 454 * @return {@link #period} (Indicates when the specific member or organization 455 * did (or is intended to) come into effect and end.) 456 */ 457 public Period getPeriod() { 458 if (this.period == null) 459 if (Configuration.errorOnAutoCreate()) 460 throw new Error("Attempt to auto-create CareTeamParticipantComponent.period"); 461 else if (Configuration.doAutoCreate()) 462 this.period = new Period(); // cc 463 return this.period; 464 } 465 466 public boolean hasPeriod() { 467 return this.period != null && !this.period.isEmpty(); 468 } 469 470 /** 471 * @param value {@link #period} (Indicates when the specific member or 472 * organization did (or is intended to) come into effect and end.) 473 */ 474 public CareTeamParticipantComponent setPeriod(Period value) { 475 this.period = value; 476 return this; 477 } 478 479 protected void listChildren(List<Property> children) { 480 super.listChildren(children); 481 children.add(new Property("role", "CodeableConcept", 482 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 483 0, java.lang.Integer.MAX_VALUE, role)); 484 children.add( 485 new Property("member", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 486 "The specific person or organization who is participating/expected to participate in the care team.", 0, 487 1, member)); 488 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization of the practitioner.", 0, 1, 489 onBehalfOf)); 490 children.add(new Property("period", "Period", 491 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 1, 492 period)); 493 } 494 495 @Override 496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 497 switch (_hash) { 498 case 3506294: 499 /* role */ return new Property("role", "CodeableConcept", 500 "Indicates specific responsibility of an individual within the care team, such as \"Primary care physician\", \"Trained social worker counselor\", \"Caregiver\", etc.", 501 0, java.lang.Integer.MAX_VALUE, role); 502 case -1077769574: 503 /* member */ return new Property("member", 504 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Organization|CareTeam)", 505 "The specific person or organization who is participating/expected to participate in the care team.", 0, 1, 506 member); 507 case -14402964: 508 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization)", 509 "The organization of the practitioner.", 0, 1, onBehalfOf); 510 case -991726143: 511 /* period */ return new Property("period", "Period", 512 "Indicates when the specific member or organization did (or is intended to) come into effect and end.", 0, 513 1, period); 514 default: 515 return super.getNamedProperty(_hash, _name, _checkValid); 516 } 517 518 } 519 520 @Override 521 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 522 switch (hash) { 523 case 3506294: 524 /* role */ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 525 case -1077769574: 526 /* member */ return this.member == null ? new Base[0] : new Base[] { this.member }; // Reference 527 case -14402964: 528 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 529 case -991726143: 530 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 531 default: 532 return super.getProperty(hash, name, checkValid); 533 } 534 535 } 536 537 @Override 538 public Base setProperty(int hash, String name, Base value) throws FHIRException { 539 switch (hash) { 540 case 3506294: // role 541 this.getRole().add(castToCodeableConcept(value)); // CodeableConcept 542 return value; 543 case -1077769574: // member 544 this.member = castToReference(value); // Reference 545 return value; 546 case -14402964: // onBehalfOf 547 this.onBehalfOf = castToReference(value); // Reference 548 return value; 549 case -991726143: // period 550 this.period = castToPeriod(value); // Period 551 return value; 552 default: 553 return super.setProperty(hash, name, value); 554 } 555 556 } 557 558 @Override 559 public Base setProperty(String name, Base value) throws FHIRException { 560 if (name.equals("role")) { 561 this.getRole().add(castToCodeableConcept(value)); 562 } else if (name.equals("member")) { 563 this.member = castToReference(value); // Reference 564 } else if (name.equals("onBehalfOf")) { 565 this.onBehalfOf = castToReference(value); // Reference 566 } else if (name.equals("period")) { 567 this.period = castToPeriod(value); // Period 568 } else 569 return super.setProperty(name, value); 570 return value; 571 } 572 573 @Override 574 public void removeChild(String name, Base value) throws FHIRException { 575 if (name.equals("role")) { 576 this.getRole().remove(castToCodeableConcept(value)); 577 } else if (name.equals("member")) { 578 this.member = null; 579 } else if (name.equals("onBehalfOf")) { 580 this.onBehalfOf = null; 581 } else if (name.equals("period")) { 582 this.period = null; 583 } else 584 super.removeChild(name, value); 585 586 } 587 588 @Override 589 public Base makeProperty(int hash, String name) throws FHIRException { 590 switch (hash) { 591 case 3506294: 592 return addRole(); 593 case -1077769574: 594 return getMember(); 595 case -14402964: 596 return getOnBehalfOf(); 597 case -991726143: 598 return getPeriod(); 599 default: 600 return super.makeProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 607 switch (hash) { 608 case 3506294: 609 /* role */ return new String[] { "CodeableConcept" }; 610 case -1077769574: 611 /* member */ return new String[] { "Reference" }; 612 case -14402964: 613 /* onBehalfOf */ return new String[] { "Reference" }; 614 case -991726143: 615 /* period */ return new String[] { "Period" }; 616 default: 617 return super.getTypesForProperty(hash, name); 618 } 619 620 } 621 622 @Override 623 public Base addChild(String name) throws FHIRException { 624 if (name.equals("role")) { 625 return addRole(); 626 } else if (name.equals("member")) { 627 this.member = new Reference(); 628 return this.member; 629 } else if (name.equals("onBehalfOf")) { 630 this.onBehalfOf = new Reference(); 631 return this.onBehalfOf; 632 } else if (name.equals("period")) { 633 this.period = new Period(); 634 return this.period; 635 } else 636 return super.addChild(name); 637 } 638 639 public CareTeamParticipantComponent copy() { 640 CareTeamParticipantComponent dst = new CareTeamParticipantComponent(); 641 copyValues(dst); 642 return dst; 643 } 644 645 public void copyValues(CareTeamParticipantComponent dst) { 646 super.copyValues(dst); 647 if (role != null) { 648 dst.role = new ArrayList<CodeableConcept>(); 649 for (CodeableConcept i : role) 650 dst.role.add(i.copy()); 651 } 652 ; 653 dst.member = member == null ? null : member.copy(); 654 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 655 dst.period = period == null ? null : period.copy(); 656 } 657 658 @Override 659 public boolean equalsDeep(Base other_) { 660 if (!super.equalsDeep(other_)) 661 return false; 662 if (!(other_ instanceof CareTeamParticipantComponent)) 663 return false; 664 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 665 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true) 666 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(period, o.period, true); 667 } 668 669 @Override 670 public boolean equalsShallow(Base other_) { 671 if (!super.equalsShallow(other_)) 672 return false; 673 if (!(other_ instanceof CareTeamParticipantComponent)) 674 return false; 675 CareTeamParticipantComponent o = (CareTeamParticipantComponent) other_; 676 return true; 677 } 678 679 public boolean isEmpty() { 680 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, member, onBehalfOf, period); 681 } 682 683 public String fhirType() { 684 return "CareTeam.participant"; 685 686 } 687 688 } 689 690 /** 691 * Business identifiers assigned to this care team by the performer or other 692 * systems which remain constant as the resource is updated and propagates from 693 * server to server. 694 */ 695 @Child(name = "identifier", type = { 696 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 697 @Description(shortDefinition = "External Ids for this team", formalDefinition = "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 698 protected List<Identifier> identifier; 699 700 /** 701 * Indicates the current state of the care team. 702 */ 703 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 704 @Description(shortDefinition = "proposed | active | suspended | inactive | entered-in-error", formalDefinition = "Indicates the current state of the care team.") 705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-status") 706 protected Enumeration<CareTeamStatus> status; 707 708 /** 709 * Identifies what kind of team. This is to support differentiation between 710 * multiple co-existing teams, such as care plan team, episode of care team, 711 * longitudinal care team. 712 */ 713 @Child(name = "category", type = { 714 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 715 @Description(shortDefinition = "Type of team", formalDefinition = "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.") 716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/care-team-category") 717 protected List<CodeableConcept> category; 718 719 /** 720 * A label for human use intended to distinguish like teams. E.g. the "red" vs. 721 * "green" trauma teams. 722 */ 723 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 724 @Description(shortDefinition = "Name of the team, such as crisis assessment team", formalDefinition = "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.") 725 protected StringType name; 726 727 /** 728 * Identifies the patient or group whose intended care is handled by the team. 729 */ 730 @Child(name = "subject", type = { Patient.class, 731 Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 732 @Description(shortDefinition = "Who care team is for", formalDefinition = "Identifies the patient or group whose intended care is handled by the team.") 733 protected Reference subject; 734 735 /** 736 * The actual object that is the target of the reference (Identifies the patient 737 * or group whose intended care is handled by the team.) 738 */ 739 protected Resource subjectTarget; 740 741 /** 742 * The Encounter during which this CareTeam was created or to which the creation 743 * of this record is tightly associated. 744 */ 745 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 746 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.") 747 protected Reference encounter; 748 749 /** 750 * The actual object that is the target of the reference (The Encounter during 751 * which this CareTeam was created or to which the creation of this record is 752 * tightly associated.) 753 */ 754 protected Encounter encounterTarget; 755 756 /** 757 * Indicates when the team did (or is intended to) come into effect and end. 758 */ 759 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 760 @Description(shortDefinition = "Time period team covers", formalDefinition = "Indicates when the team did (or is intended to) come into effect and end.") 761 protected Period period; 762 763 /** 764 * Identifies all people and organizations who are expected to be involved in 765 * the care team. 766 */ 767 @Child(name = "participant", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 768 @Description(shortDefinition = "Members of the team", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care team.") 769 protected List<CareTeamParticipantComponent> participant; 770 771 /** 772 * Describes why the care team exists. 773 */ 774 @Child(name = "reasonCode", type = { 775 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 776 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Describes why the care team exists.") 777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 778 protected List<CodeableConcept> reasonCode; 779 780 /** 781 * Condition(s) that this care team addresses. 782 */ 783 @Child(name = "reasonReference", type = { 784 Condition.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 785 @Description(shortDefinition = "Why the care team exists", formalDefinition = "Condition(s) that this care team addresses.") 786 protected List<Reference> reasonReference; 787 /** 788 * The actual objects that are the target of the reference (Condition(s) that 789 * this care team addresses.) 790 */ 791 protected List<Condition> reasonReferenceTarget; 792 793 /** 794 * The organization responsible for the care team. 795 */ 796 @Child(name = "managingOrganization", type = { 797 Organization.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 798 @Description(shortDefinition = "Organization responsible for the care team", formalDefinition = "The organization responsible for the care team.") 799 protected List<Reference> managingOrganization; 800 /** 801 * The actual objects that are the target of the reference (The organization 802 * responsible for the care team.) 803 */ 804 protected List<Organization> managingOrganizationTarget; 805 806 /** 807 * A central contact detail for the care team (that applies to all members). 808 */ 809 @Child(name = "telecom", type = { 810 ContactPoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 811 @Description(shortDefinition = "A contact detail for the care team (that applies to all members)", formalDefinition = "A central contact detail for the care team (that applies to all members).") 812 protected List<ContactPoint> telecom; 813 814 /** 815 * Comments made about the CareTeam. 816 */ 817 @Child(name = "note", type = { 818 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 819 @Description(shortDefinition = "Comments made about the CareTeam", formalDefinition = "Comments made about the CareTeam.") 820 protected List<Annotation> note; 821 822 private static final long serialVersionUID = 1793069286L; 823 824 /** 825 * Constructor 826 */ 827 public CareTeam() { 828 super(); 829 } 830 831 /** 832 * @return {@link #identifier} (Business identifiers assigned to this care team 833 * by the performer or other systems which remain constant as the 834 * resource is updated and propagates from server to server.) 835 */ 836 public List<Identifier> getIdentifier() { 837 if (this.identifier == null) 838 this.identifier = new ArrayList<Identifier>(); 839 return this.identifier; 840 } 841 842 /** 843 * @return Returns a reference to <code>this</code> for easy method chaining 844 */ 845 public CareTeam setIdentifier(List<Identifier> theIdentifier) { 846 this.identifier = theIdentifier; 847 return this; 848 } 849 850 public boolean hasIdentifier() { 851 if (this.identifier == null) 852 return false; 853 for (Identifier item : this.identifier) 854 if (!item.isEmpty()) 855 return true; 856 return false; 857 } 858 859 public Identifier addIdentifier() { // 3 860 Identifier t = new Identifier(); 861 if (this.identifier == null) 862 this.identifier = new ArrayList<Identifier>(); 863 this.identifier.add(t); 864 return t; 865 } 866 867 public CareTeam addIdentifier(Identifier t) { // 3 868 if (t == null) 869 return this; 870 if (this.identifier == null) 871 this.identifier = new ArrayList<Identifier>(); 872 this.identifier.add(t); 873 return this; 874 } 875 876 /** 877 * @return The first repetition of repeating field {@link #identifier}, creating 878 * it if it does not already exist 879 */ 880 public Identifier getIdentifierFirstRep() { 881 if (getIdentifier().isEmpty()) { 882 addIdentifier(); 883 } 884 return getIdentifier().get(0); 885 } 886 887 /** 888 * @return {@link #status} (Indicates the current state of the care team.). This 889 * is the underlying object with id, value and extensions. The accessor 890 * "getStatus" gives direct access to the value 891 */ 892 public Enumeration<CareTeamStatus> getStatusElement() { 893 if (this.status == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create CareTeam.status"); 896 else if (Configuration.doAutoCreate()) 897 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); // bb 898 return this.status; 899 } 900 901 public boolean hasStatusElement() { 902 return this.status != null && !this.status.isEmpty(); 903 } 904 905 public boolean hasStatus() { 906 return this.status != null && !this.status.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #status} (Indicates the current state of the care team.). 911 * This is the underlying object with id, value and extensions. The 912 * accessor "getStatus" gives direct access to the value 913 */ 914 public CareTeam setStatusElement(Enumeration<CareTeamStatus> value) { 915 this.status = value; 916 return this; 917 } 918 919 /** 920 * @return Indicates the current state of the care team. 921 */ 922 public CareTeamStatus getStatus() { 923 return this.status == null ? null : this.status.getValue(); 924 } 925 926 /** 927 * @param value Indicates the current state of the care team. 928 */ 929 public CareTeam setStatus(CareTeamStatus value) { 930 if (value == null) 931 this.status = null; 932 else { 933 if (this.status == null) 934 this.status = new Enumeration<CareTeamStatus>(new CareTeamStatusEnumFactory()); 935 this.status.setValue(value); 936 } 937 return this; 938 } 939 940 /** 941 * @return {@link #category} (Identifies what kind of team. This is to support 942 * differentiation between multiple co-existing teams, such as care plan 943 * team, episode of care team, longitudinal care team.) 944 */ 945 public List<CodeableConcept> getCategory() { 946 if (this.category == null) 947 this.category = new ArrayList<CodeableConcept>(); 948 return this.category; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public CareTeam setCategory(List<CodeableConcept> theCategory) { 955 this.category = theCategory; 956 return this; 957 } 958 959 public boolean hasCategory() { 960 if (this.category == null) 961 return false; 962 for (CodeableConcept item : this.category) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public CodeableConcept addCategory() { // 3 969 CodeableConcept t = new CodeableConcept(); 970 if (this.category == null) 971 this.category = new ArrayList<CodeableConcept>(); 972 this.category.add(t); 973 return t; 974 } 975 976 public CareTeam addCategory(CodeableConcept t) { // 3 977 if (t == null) 978 return this; 979 if (this.category == null) 980 this.category = new ArrayList<CodeableConcept>(); 981 this.category.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #category}, creating 987 * it if it does not already exist 988 */ 989 public CodeableConcept getCategoryFirstRep() { 990 if (getCategory().isEmpty()) { 991 addCategory(); 992 } 993 return getCategory().get(0); 994 } 995 996 /** 997 * @return {@link #name} (A label for human use intended to distinguish like 998 * teams. E.g. the "red" vs. "green" trauma teams.). This is the 999 * underlying object with id, value and extensions. The accessor 1000 * "getName" gives direct access to the value 1001 */ 1002 public StringType getNameElement() { 1003 if (this.name == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create CareTeam.name"); 1006 else if (Configuration.doAutoCreate()) 1007 this.name = new StringType(); // bb 1008 return this.name; 1009 } 1010 1011 public boolean hasNameElement() { 1012 return this.name != null && !this.name.isEmpty(); 1013 } 1014 1015 public boolean hasName() { 1016 return this.name != null && !this.name.isEmpty(); 1017 } 1018 1019 /** 1020 * @param value {@link #name} (A label for human use intended to distinguish 1021 * like teams. E.g. the "red" vs. "green" trauma teams.). This is 1022 * the underlying object with id, value and extensions. The 1023 * accessor "getName" gives direct access to the value 1024 */ 1025 public CareTeam setNameElement(StringType value) { 1026 this.name = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return A label for human use intended to distinguish like teams. E.g. the 1032 * "red" vs. "green" trauma teams. 1033 */ 1034 public String getName() { 1035 return this.name == null ? null : this.name.getValue(); 1036 } 1037 1038 /** 1039 * @param value A label for human use intended to distinguish like teams. E.g. 1040 * the "red" vs. "green" trauma teams. 1041 */ 1042 public CareTeam setName(String value) { 1043 if (Utilities.noString(value)) 1044 this.name = null; 1045 else { 1046 if (this.name == null) 1047 this.name = new StringType(); 1048 this.name.setValue(value); 1049 } 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #subject} (Identifies the patient or group whose intended care 1055 * is handled by the team.) 1056 */ 1057 public Reference getSubject() { 1058 if (this.subject == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create CareTeam.subject"); 1061 else if (Configuration.doAutoCreate()) 1062 this.subject = new Reference(); // cc 1063 return this.subject; 1064 } 1065 1066 public boolean hasSubject() { 1067 return this.subject != null && !this.subject.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #subject} (Identifies the patient or group whose intended 1072 * care is handled by the team.) 1073 */ 1074 public CareTeam setSubject(Reference value) { 1075 this.subject = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #subject} The actual object that is the target of the 1081 * reference. The reference library doesn't populate this, but you can 1082 * use it to hold the resource if you resolve it. (Identifies the 1083 * patient or group whose intended care is handled by the team.) 1084 */ 1085 public Resource getSubjectTarget() { 1086 return this.subjectTarget; 1087 } 1088 1089 /** 1090 * @param value {@link #subject} The actual object that is the target of the 1091 * reference. The reference library doesn't use these, but you can 1092 * use it to hold the resource if you resolve it. (Identifies the 1093 * patient or group whose intended care is handled by the team.) 1094 */ 1095 public CareTeam setSubjectTarget(Resource value) { 1096 this.subjectTarget = value; 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #encounter} (The Encounter during which this CareTeam was 1102 * created or to which the creation of this record is tightly 1103 * associated.) 1104 */ 1105 public Reference getEncounter() { 1106 if (this.encounter == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create CareTeam.encounter"); 1109 else if (Configuration.doAutoCreate()) 1110 this.encounter = new Reference(); // cc 1111 return this.encounter; 1112 } 1113 1114 public boolean hasEncounter() { 1115 return this.encounter != null && !this.encounter.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #encounter} (The Encounter during which this CareTeam was 1120 * created or to which the creation of this record is tightly 1121 * associated.) 1122 */ 1123 public CareTeam setEncounter(Reference value) { 1124 this.encounter = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #encounter} The actual object that is the target of the 1130 * reference. The reference library doesn't populate this, but you can 1131 * use it to hold the resource if you resolve it. (The Encounter during 1132 * which this CareTeam was created or to which the creation of this 1133 * record is tightly associated.) 1134 */ 1135 public Encounter getEncounterTarget() { 1136 if (this.encounterTarget == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create CareTeam.encounter"); 1139 else if (Configuration.doAutoCreate()) 1140 this.encounterTarget = new Encounter(); // aa 1141 return this.encounterTarget; 1142 } 1143 1144 /** 1145 * @param value {@link #encounter} The actual object that is the target of the 1146 * reference. The reference library doesn't use these, but you can 1147 * use it to hold the resource if you resolve it. (The Encounter 1148 * during which this CareTeam was created or to which the creation 1149 * of this record is tightly associated.) 1150 */ 1151 public CareTeam setEncounterTarget(Encounter value) { 1152 this.encounterTarget = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #period} (Indicates when the team did (or is intended to) come 1158 * into effect and end.) 1159 */ 1160 public Period getPeriod() { 1161 if (this.period == null) 1162 if (Configuration.errorOnAutoCreate()) 1163 throw new Error("Attempt to auto-create CareTeam.period"); 1164 else if (Configuration.doAutoCreate()) 1165 this.period = new Period(); // cc 1166 return this.period; 1167 } 1168 1169 public boolean hasPeriod() { 1170 return this.period != null && !this.period.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #period} (Indicates when the team did (or is intended to) 1175 * come into effect and end.) 1176 */ 1177 public CareTeam setPeriod(Period value) { 1178 this.period = value; 1179 return this; 1180 } 1181 1182 /** 1183 * @return {@link #participant} (Identifies all people and organizations who are 1184 * expected to be involved in the care team.) 1185 */ 1186 public List<CareTeamParticipantComponent> getParticipant() { 1187 if (this.participant == null) 1188 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1189 return this.participant; 1190 } 1191 1192 /** 1193 * @return Returns a reference to <code>this</code> for easy method chaining 1194 */ 1195 public CareTeam setParticipant(List<CareTeamParticipantComponent> theParticipant) { 1196 this.participant = theParticipant; 1197 return this; 1198 } 1199 1200 public boolean hasParticipant() { 1201 if (this.participant == null) 1202 return false; 1203 for (CareTeamParticipantComponent item : this.participant) 1204 if (!item.isEmpty()) 1205 return true; 1206 return false; 1207 } 1208 1209 public CareTeamParticipantComponent addParticipant() { // 3 1210 CareTeamParticipantComponent t = new CareTeamParticipantComponent(); 1211 if (this.participant == null) 1212 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1213 this.participant.add(t); 1214 return t; 1215 } 1216 1217 public CareTeam addParticipant(CareTeamParticipantComponent t) { // 3 1218 if (t == null) 1219 return this; 1220 if (this.participant == null) 1221 this.participant = new ArrayList<CareTeamParticipantComponent>(); 1222 this.participant.add(t); 1223 return this; 1224 } 1225 1226 /** 1227 * @return The first repetition of repeating field {@link #participant}, 1228 * creating it if it does not already exist 1229 */ 1230 public CareTeamParticipantComponent getParticipantFirstRep() { 1231 if (getParticipant().isEmpty()) { 1232 addParticipant(); 1233 } 1234 return getParticipant().get(0); 1235 } 1236 1237 /** 1238 * @return {@link #reasonCode} (Describes why the care team exists.) 1239 */ 1240 public List<CodeableConcept> getReasonCode() { 1241 if (this.reasonCode == null) 1242 this.reasonCode = new ArrayList<CodeableConcept>(); 1243 return this.reasonCode; 1244 } 1245 1246 /** 1247 * @return Returns a reference to <code>this</code> for easy method chaining 1248 */ 1249 public CareTeam setReasonCode(List<CodeableConcept> theReasonCode) { 1250 this.reasonCode = theReasonCode; 1251 return this; 1252 } 1253 1254 public boolean hasReasonCode() { 1255 if (this.reasonCode == null) 1256 return false; 1257 for (CodeableConcept item : this.reasonCode) 1258 if (!item.isEmpty()) 1259 return true; 1260 return false; 1261 } 1262 1263 public CodeableConcept addReasonCode() { // 3 1264 CodeableConcept t = new CodeableConcept(); 1265 if (this.reasonCode == null) 1266 this.reasonCode = new ArrayList<CodeableConcept>(); 1267 this.reasonCode.add(t); 1268 return t; 1269 } 1270 1271 public CareTeam addReasonCode(CodeableConcept t) { // 3 1272 if (t == null) 1273 return this; 1274 if (this.reasonCode == null) 1275 this.reasonCode = new ArrayList<CodeableConcept>(); 1276 this.reasonCode.add(t); 1277 return this; 1278 } 1279 1280 /** 1281 * @return The first repetition of repeating field {@link #reasonCode}, creating 1282 * it if it does not already exist 1283 */ 1284 public CodeableConcept getReasonCodeFirstRep() { 1285 if (getReasonCode().isEmpty()) { 1286 addReasonCode(); 1287 } 1288 return getReasonCode().get(0); 1289 } 1290 1291 /** 1292 * @return {@link #reasonReference} (Condition(s) that this care team 1293 * addresses.) 1294 */ 1295 public List<Reference> getReasonReference() { 1296 if (this.reasonReference == null) 1297 this.reasonReference = new ArrayList<Reference>(); 1298 return this.reasonReference; 1299 } 1300 1301 /** 1302 * @return Returns a reference to <code>this</code> for easy method chaining 1303 */ 1304 public CareTeam setReasonReference(List<Reference> theReasonReference) { 1305 this.reasonReference = theReasonReference; 1306 return this; 1307 } 1308 1309 public boolean hasReasonReference() { 1310 if (this.reasonReference == null) 1311 return false; 1312 for (Reference item : this.reasonReference) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public Reference addReasonReference() { // 3 1319 Reference t = new Reference(); 1320 if (this.reasonReference == null) 1321 this.reasonReference = new ArrayList<Reference>(); 1322 this.reasonReference.add(t); 1323 return t; 1324 } 1325 1326 public CareTeam addReasonReference(Reference t) { // 3 1327 if (t == null) 1328 return this; 1329 if (this.reasonReference == null) 1330 this.reasonReference = new ArrayList<Reference>(); 1331 this.reasonReference.add(t); 1332 return this; 1333 } 1334 1335 /** 1336 * @return The first repetition of repeating field {@link #reasonReference}, 1337 * creating it if it does not already exist 1338 */ 1339 public Reference getReasonReferenceFirstRep() { 1340 if (getReasonReference().isEmpty()) { 1341 addReasonReference(); 1342 } 1343 return getReasonReference().get(0); 1344 } 1345 1346 /** 1347 * @return {@link #managingOrganization} (The organization responsible for the 1348 * care team.) 1349 */ 1350 public List<Reference> getManagingOrganization() { 1351 if (this.managingOrganization == null) 1352 this.managingOrganization = new ArrayList<Reference>(); 1353 return this.managingOrganization; 1354 } 1355 1356 /** 1357 * @return Returns a reference to <code>this</code> for easy method chaining 1358 */ 1359 public CareTeam setManagingOrganization(List<Reference> theManagingOrganization) { 1360 this.managingOrganization = theManagingOrganization; 1361 return this; 1362 } 1363 1364 public boolean hasManagingOrganization() { 1365 if (this.managingOrganization == null) 1366 return false; 1367 for (Reference item : this.managingOrganization) 1368 if (!item.isEmpty()) 1369 return true; 1370 return false; 1371 } 1372 1373 public Reference addManagingOrganization() { // 3 1374 Reference t = new Reference(); 1375 if (this.managingOrganization == null) 1376 this.managingOrganization = new ArrayList<Reference>(); 1377 this.managingOrganization.add(t); 1378 return t; 1379 } 1380 1381 public CareTeam addManagingOrganization(Reference t) { // 3 1382 if (t == null) 1383 return this; 1384 if (this.managingOrganization == null) 1385 this.managingOrganization = new ArrayList<Reference>(); 1386 this.managingOrganization.add(t); 1387 return this; 1388 } 1389 1390 /** 1391 * @return The first repetition of repeating field 1392 * {@link #managingOrganization}, creating it if it does not already 1393 * exist 1394 */ 1395 public Reference getManagingOrganizationFirstRep() { 1396 if (getManagingOrganization().isEmpty()) { 1397 addManagingOrganization(); 1398 } 1399 return getManagingOrganization().get(0); 1400 } 1401 1402 /** 1403 * @return {@link #telecom} (A central contact detail for the care team (that 1404 * applies to all members).) 1405 */ 1406 public List<ContactPoint> getTelecom() { 1407 if (this.telecom == null) 1408 this.telecom = new ArrayList<ContactPoint>(); 1409 return this.telecom; 1410 } 1411 1412 /** 1413 * @return Returns a reference to <code>this</code> for easy method chaining 1414 */ 1415 public CareTeam setTelecom(List<ContactPoint> theTelecom) { 1416 this.telecom = theTelecom; 1417 return this; 1418 } 1419 1420 public boolean hasTelecom() { 1421 if (this.telecom == null) 1422 return false; 1423 for (ContactPoint item : this.telecom) 1424 if (!item.isEmpty()) 1425 return true; 1426 return false; 1427 } 1428 1429 public ContactPoint addTelecom() { // 3 1430 ContactPoint t = new ContactPoint(); 1431 if (this.telecom == null) 1432 this.telecom = new ArrayList<ContactPoint>(); 1433 this.telecom.add(t); 1434 return t; 1435 } 1436 1437 public CareTeam addTelecom(ContactPoint t) { // 3 1438 if (t == null) 1439 return this; 1440 if (this.telecom == null) 1441 this.telecom = new ArrayList<ContactPoint>(); 1442 this.telecom.add(t); 1443 return this; 1444 } 1445 1446 /** 1447 * @return The first repetition of repeating field {@link #telecom}, creating it 1448 * if it does not already exist 1449 */ 1450 public ContactPoint getTelecomFirstRep() { 1451 if (getTelecom().isEmpty()) { 1452 addTelecom(); 1453 } 1454 return getTelecom().get(0); 1455 } 1456 1457 /** 1458 * @return {@link #note} (Comments made about the CareTeam.) 1459 */ 1460 public List<Annotation> getNote() { 1461 if (this.note == null) 1462 this.note = new ArrayList<Annotation>(); 1463 return this.note; 1464 } 1465 1466 /** 1467 * @return Returns a reference to <code>this</code> for easy method chaining 1468 */ 1469 public CareTeam setNote(List<Annotation> theNote) { 1470 this.note = theNote; 1471 return this; 1472 } 1473 1474 public boolean hasNote() { 1475 if (this.note == null) 1476 return false; 1477 for (Annotation item : this.note) 1478 if (!item.isEmpty()) 1479 return true; 1480 return false; 1481 } 1482 1483 public Annotation addNote() { // 3 1484 Annotation t = new Annotation(); 1485 if (this.note == null) 1486 this.note = new ArrayList<Annotation>(); 1487 this.note.add(t); 1488 return t; 1489 } 1490 1491 public CareTeam addNote(Annotation t) { // 3 1492 if (t == null) 1493 return this; 1494 if (this.note == null) 1495 this.note = new ArrayList<Annotation>(); 1496 this.note.add(t); 1497 return this; 1498 } 1499 1500 /** 1501 * @return The first repetition of repeating field {@link #note}, creating it if 1502 * it does not already exist 1503 */ 1504 public Annotation getNoteFirstRep() { 1505 if (getNote().isEmpty()) { 1506 addNote(); 1507 } 1508 return getNote().get(0); 1509 } 1510 1511 protected void listChildren(List<Property> children) { 1512 super.listChildren(children); 1513 children.add(new Property("identifier", "Identifier", 1514 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1515 0, java.lang.Integer.MAX_VALUE, identifier)); 1516 children.add(new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status)); 1517 children.add(new Property("category", "CodeableConcept", 1518 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1519 0, java.lang.Integer.MAX_VALUE, category)); 1520 children.add(new Property("name", "string", 1521 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1, 1522 name)); 1523 children.add(new Property("subject", "Reference(Patient|Group)", 1524 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject)); 1525 children.add(new Property("encounter", "Reference(Encounter)", 1526 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1527 0, 1, encounter)); 1528 children.add(new Property("period", "Period", 1529 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period)); 1530 children.add(new Property("participant", "", 1531 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1532 java.lang.Integer.MAX_VALUE, participant)); 1533 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1534 java.lang.Integer.MAX_VALUE, reasonCode)); 1535 children.add(new Property("reasonReference", "Reference(Condition)", "Condition(s) that this care team addresses.", 1536 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1537 children.add(new Property("managingOrganization", "Reference(Organization)", 1538 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization)); 1539 children.add(new Property("telecom", "ContactPoint", 1540 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1541 telecom)); 1542 children.add( 1543 new Property("note", "Annotation", "Comments made about the CareTeam.", 0, java.lang.Integer.MAX_VALUE, note)); 1544 } 1545 1546 @Override 1547 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1548 switch (_hash) { 1549 case -1618432855: 1550 /* identifier */ return new Property("identifier", "Identifier", 1551 "Business identifiers assigned to this care team by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1552 0, java.lang.Integer.MAX_VALUE, identifier); 1553 case -892481550: 1554 /* status */ return new Property("status", "code", "Indicates the current state of the care team.", 0, 1, status); 1555 case 50511102: 1556 /* category */ return new Property("category", "CodeableConcept", 1557 "Identifies what kind of team. This is to support differentiation between multiple co-existing teams, such as care plan team, episode of care team, longitudinal care team.", 1558 0, java.lang.Integer.MAX_VALUE, category); 1559 case 3373707: 1560 /* name */ return new Property("name", "string", 1561 "A label for human use intended to distinguish like teams. E.g. the \"red\" vs. \"green\" trauma teams.", 0, 1562 1, name); 1563 case -1867885268: 1564 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1565 "Identifies the patient or group whose intended care is handled by the team.", 0, 1, subject); 1566 case 1524132147: 1567 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1568 "The Encounter during which this CareTeam was created or to which the creation of this record is tightly associated.", 1569 0, 1, encounter); 1570 case -991726143: 1571 /* period */ return new Property("period", "Period", 1572 "Indicates when the team did (or is intended to) come into effect and end.", 0, 1, period); 1573 case 767422259: 1574 /* participant */ return new Property("participant", "", 1575 "Identifies all people and organizations who are expected to be involved in the care team.", 0, 1576 java.lang.Integer.MAX_VALUE, participant); 1577 case 722137681: 1578 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", "Describes why the care team exists.", 0, 1579 java.lang.Integer.MAX_VALUE, reasonCode); 1580 case -1146218137: 1581 /* reasonReference */ return new Property("reasonReference", "Reference(Condition)", 1582 "Condition(s) that this care team addresses.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1583 case -2058947787: 1584 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1585 "The organization responsible for the care team.", 0, java.lang.Integer.MAX_VALUE, managingOrganization); 1586 case -1429363305: 1587 /* telecom */ return new Property("telecom", "ContactPoint", 1588 "A central contact detail for the care team (that applies to all members).", 0, java.lang.Integer.MAX_VALUE, 1589 telecom); 1590 case 3387378: 1591 /* note */ return new Property("note", "Annotation", "Comments made about the CareTeam.", 0, 1592 java.lang.Integer.MAX_VALUE, note); 1593 default: 1594 return super.getNamedProperty(_hash, _name, _checkValid); 1595 } 1596 1597 } 1598 1599 @Override 1600 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1601 switch (hash) { 1602 case -1618432855: 1603 /* identifier */ return this.identifier == null ? new Base[0] 1604 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1605 case -892481550: 1606 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CareTeamStatus> 1607 case 50511102: 1608 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1609 case 3373707: 1610 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1611 case -1867885268: 1612 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1613 case 1524132147: 1614 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1615 case -991726143: 1616 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1617 case 767422259: 1618 /* participant */ return this.participant == null ? new Base[0] 1619 : this.participant.toArray(new Base[this.participant.size()]); // CareTeamParticipantComponent 1620 case 722137681: 1621 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1622 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1623 case -1146218137: 1624 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1625 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1626 case -2058947787: 1627 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1628 : this.managingOrganization.toArray(new Base[this.managingOrganization.size()]); // Reference 1629 case -1429363305: 1630 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1631 case 3387378: 1632 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1633 default: 1634 return super.getProperty(hash, name, checkValid); 1635 } 1636 1637 } 1638 1639 @Override 1640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1641 switch (hash) { 1642 case -1618432855: // identifier 1643 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1644 return value; 1645 case -892481550: // status 1646 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1647 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1648 return value; 1649 case 50511102: // category 1650 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1651 return value; 1652 case 3373707: // name 1653 this.name = castToString(value); // StringType 1654 return value; 1655 case -1867885268: // subject 1656 this.subject = castToReference(value); // Reference 1657 return value; 1658 case 1524132147: // encounter 1659 this.encounter = castToReference(value); // Reference 1660 return value; 1661 case -991726143: // period 1662 this.period = castToPeriod(value); // Period 1663 return value; 1664 case 767422259: // participant 1665 this.getParticipant().add((CareTeamParticipantComponent) value); // CareTeamParticipantComponent 1666 return value; 1667 case 722137681: // reasonCode 1668 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1669 return value; 1670 case -1146218137: // reasonReference 1671 this.getReasonReference().add(castToReference(value)); // Reference 1672 return value; 1673 case -2058947787: // managingOrganization 1674 this.getManagingOrganization().add(castToReference(value)); // Reference 1675 return value; 1676 case -1429363305: // telecom 1677 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1678 return value; 1679 case 3387378: // note 1680 this.getNote().add(castToAnnotation(value)); // Annotation 1681 return value; 1682 default: 1683 return super.setProperty(hash, name, value); 1684 } 1685 1686 } 1687 1688 @Override 1689 public Base setProperty(String name, Base value) throws FHIRException { 1690 if (name.equals("identifier")) { 1691 this.getIdentifier().add(castToIdentifier(value)); 1692 } else if (name.equals("status")) { 1693 value = new CareTeamStatusEnumFactory().fromType(castToCode(value)); 1694 this.status = (Enumeration) value; // Enumeration<CareTeamStatus> 1695 } else if (name.equals("category")) { 1696 this.getCategory().add(castToCodeableConcept(value)); 1697 } else if (name.equals("name")) { 1698 this.name = castToString(value); // StringType 1699 } else if (name.equals("subject")) { 1700 this.subject = castToReference(value); // Reference 1701 } else if (name.equals("encounter")) { 1702 this.encounter = castToReference(value); // Reference 1703 } else if (name.equals("period")) { 1704 this.period = castToPeriod(value); // Period 1705 } else if (name.equals("participant")) { 1706 this.getParticipant().add((CareTeamParticipantComponent) value); 1707 } else if (name.equals("reasonCode")) { 1708 this.getReasonCode().add(castToCodeableConcept(value)); 1709 } else if (name.equals("reasonReference")) { 1710 this.getReasonReference().add(castToReference(value)); 1711 } else if (name.equals("managingOrganization")) { 1712 this.getManagingOrganization().add(castToReference(value)); 1713 } else if (name.equals("telecom")) { 1714 this.getTelecom().add(castToContactPoint(value)); 1715 } else if (name.equals("note")) { 1716 this.getNote().add(castToAnnotation(value)); 1717 } else 1718 return super.setProperty(name, value); 1719 return value; 1720 } 1721 1722 @Override 1723 public void removeChild(String name, Base value) throws FHIRException { 1724 if (name.equals("identifier")) { 1725 this.getIdentifier().remove(castToIdentifier(value)); 1726 } else if (name.equals("status")) { 1727 this.status = null; 1728 } else if (name.equals("category")) { 1729 this.getCategory().remove(castToCodeableConcept(value)); 1730 } else if (name.equals("name")) { 1731 this.name = null; 1732 } else if (name.equals("subject")) { 1733 this.subject = null; 1734 } else if (name.equals("encounter")) { 1735 this.encounter = null; 1736 } else if (name.equals("period")) { 1737 this.period = null; 1738 } else if (name.equals("participant")) { 1739 this.getParticipant().remove((CareTeamParticipantComponent) value); 1740 } else if (name.equals("reasonCode")) { 1741 this.getReasonCode().remove(castToCodeableConcept(value)); 1742 } else if (name.equals("reasonReference")) { 1743 this.getReasonReference().remove(castToReference(value)); 1744 } else if (name.equals("managingOrganization")) { 1745 this.getManagingOrganization().remove(castToReference(value)); 1746 } else if (name.equals("telecom")) { 1747 this.getTelecom().remove(castToContactPoint(value)); 1748 } else if (name.equals("note")) { 1749 this.getNote().remove(castToAnnotation(value)); 1750 } else 1751 super.removeChild(name, value); 1752 1753 } 1754 1755 @Override 1756 public Base makeProperty(int hash, String name) throws FHIRException { 1757 switch (hash) { 1758 case -1618432855: 1759 return addIdentifier(); 1760 case -892481550: 1761 return getStatusElement(); 1762 case 50511102: 1763 return addCategory(); 1764 case 3373707: 1765 return getNameElement(); 1766 case -1867885268: 1767 return getSubject(); 1768 case 1524132147: 1769 return getEncounter(); 1770 case -991726143: 1771 return getPeriod(); 1772 case 767422259: 1773 return addParticipant(); 1774 case 722137681: 1775 return addReasonCode(); 1776 case -1146218137: 1777 return addReasonReference(); 1778 case -2058947787: 1779 return addManagingOrganization(); 1780 case -1429363305: 1781 return addTelecom(); 1782 case 3387378: 1783 return addNote(); 1784 default: 1785 return super.makeProperty(hash, name); 1786 } 1787 1788 } 1789 1790 @Override 1791 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1792 switch (hash) { 1793 case -1618432855: 1794 /* identifier */ return new String[] { "Identifier" }; 1795 case -892481550: 1796 /* status */ return new String[] { "code" }; 1797 case 50511102: 1798 /* category */ return new String[] { "CodeableConcept" }; 1799 case 3373707: 1800 /* name */ return new String[] { "string" }; 1801 case -1867885268: 1802 /* subject */ return new String[] { "Reference" }; 1803 case 1524132147: 1804 /* encounter */ return new String[] { "Reference" }; 1805 case -991726143: 1806 /* period */ return new String[] { "Period" }; 1807 case 767422259: 1808 /* participant */ return new String[] {}; 1809 case 722137681: 1810 /* reasonCode */ return new String[] { "CodeableConcept" }; 1811 case -1146218137: 1812 /* reasonReference */ return new String[] { "Reference" }; 1813 case -2058947787: 1814 /* managingOrganization */ return new String[] { "Reference" }; 1815 case -1429363305: 1816 /* telecom */ return new String[] { "ContactPoint" }; 1817 case 3387378: 1818 /* note */ return new String[] { "Annotation" }; 1819 default: 1820 return super.getTypesForProperty(hash, name); 1821 } 1822 1823 } 1824 1825 @Override 1826 public Base addChild(String name) throws FHIRException { 1827 if (name.equals("identifier")) { 1828 return addIdentifier(); 1829 } else if (name.equals("status")) { 1830 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.status"); 1831 } else if (name.equals("category")) { 1832 return addCategory(); 1833 } else if (name.equals("name")) { 1834 throw new FHIRException("Cannot call addChild on a singleton property CareTeam.name"); 1835 } else if (name.equals("subject")) { 1836 this.subject = new Reference(); 1837 return this.subject; 1838 } else if (name.equals("encounter")) { 1839 this.encounter = new Reference(); 1840 return this.encounter; 1841 } else if (name.equals("period")) { 1842 this.period = new Period(); 1843 return this.period; 1844 } else if (name.equals("participant")) { 1845 return addParticipant(); 1846 } else if (name.equals("reasonCode")) { 1847 return addReasonCode(); 1848 } else if (name.equals("reasonReference")) { 1849 return addReasonReference(); 1850 } else if (name.equals("managingOrganization")) { 1851 return addManagingOrganization(); 1852 } else if (name.equals("telecom")) { 1853 return addTelecom(); 1854 } else if (name.equals("note")) { 1855 return addNote(); 1856 } else 1857 return super.addChild(name); 1858 } 1859 1860 public String fhirType() { 1861 return "CareTeam"; 1862 1863 } 1864 1865 public CareTeam copy() { 1866 CareTeam dst = new CareTeam(); 1867 copyValues(dst); 1868 return dst; 1869 } 1870 1871 public void copyValues(CareTeam dst) { 1872 super.copyValues(dst); 1873 if (identifier != null) { 1874 dst.identifier = new ArrayList<Identifier>(); 1875 for (Identifier i : identifier) 1876 dst.identifier.add(i.copy()); 1877 } 1878 ; 1879 dst.status = status == null ? null : status.copy(); 1880 if (category != null) { 1881 dst.category = new ArrayList<CodeableConcept>(); 1882 for (CodeableConcept i : category) 1883 dst.category.add(i.copy()); 1884 } 1885 ; 1886 dst.name = name == null ? null : name.copy(); 1887 dst.subject = subject == null ? null : subject.copy(); 1888 dst.encounter = encounter == null ? null : encounter.copy(); 1889 dst.period = period == null ? null : period.copy(); 1890 if (participant != null) { 1891 dst.participant = new ArrayList<CareTeamParticipantComponent>(); 1892 for (CareTeamParticipantComponent i : participant) 1893 dst.participant.add(i.copy()); 1894 } 1895 ; 1896 if (reasonCode != null) { 1897 dst.reasonCode = new ArrayList<CodeableConcept>(); 1898 for (CodeableConcept i : reasonCode) 1899 dst.reasonCode.add(i.copy()); 1900 } 1901 ; 1902 if (reasonReference != null) { 1903 dst.reasonReference = new ArrayList<Reference>(); 1904 for (Reference i : reasonReference) 1905 dst.reasonReference.add(i.copy()); 1906 } 1907 ; 1908 if (managingOrganization != null) { 1909 dst.managingOrganization = new ArrayList<Reference>(); 1910 for (Reference i : managingOrganization) 1911 dst.managingOrganization.add(i.copy()); 1912 } 1913 ; 1914 if (telecom != null) { 1915 dst.telecom = new ArrayList<ContactPoint>(); 1916 for (ContactPoint i : telecom) 1917 dst.telecom.add(i.copy()); 1918 } 1919 ; 1920 if (note != null) { 1921 dst.note = new ArrayList<Annotation>(); 1922 for (Annotation i : note) 1923 dst.note.add(i.copy()); 1924 } 1925 ; 1926 } 1927 1928 protected CareTeam typedCopy() { 1929 return copy(); 1930 } 1931 1932 @Override 1933 public boolean equalsDeep(Base other_) { 1934 if (!super.equalsDeep(other_)) 1935 return false; 1936 if (!(other_ instanceof CareTeam)) 1937 return false; 1938 CareTeam o = (CareTeam) other_; 1939 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1940 && compareDeep(category, o.category, true) && compareDeep(name, o.name, true) 1941 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1942 && compareDeep(period, o.period, true) && compareDeep(participant, o.participant, true) 1943 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1944 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(telecom, o.telecom, true) 1945 && compareDeep(note, o.note, true); 1946 } 1947 1948 @Override 1949 public boolean equalsShallow(Base other_) { 1950 if (!super.equalsShallow(other_)) 1951 return false; 1952 if (!(other_ instanceof CareTeam)) 1953 return false; 1954 CareTeam o = (CareTeam) other_; 1955 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 1956 } 1957 1958 public boolean isEmpty() { 1959 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, name, subject, 1960 encounter, period, participant, reasonCode, reasonReference, managingOrganization, telecom, note); 1961 } 1962 1963 @Override 1964 public ResourceType getResourceType() { 1965 return ResourceType.CareTeam; 1966 } 1967 1968 /** 1969 * Search parameter: <b>date</b> 1970 * <p> 1971 * Description: <b>Time period team covers</b><br> 1972 * Type: <b>date</b><br> 1973 * Path: <b>CareTeam.period</b><br> 1974 * </p> 1975 */ 1976 @SearchParamDefinition(name = "date", path = "CareTeam.period", description = "Time period team covers", type = "date") 1977 public static final String SP_DATE = "date"; 1978 /** 1979 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1980 * <p> 1981 * Description: <b>Time period team covers</b><br> 1982 * Type: <b>date</b><br> 1983 * Path: <b>CareTeam.period</b><br> 1984 * </p> 1985 */ 1986 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1987 SP_DATE); 1988 1989 /** 1990 * Search parameter: <b>identifier</b> 1991 * <p> 1992 * Description: <b>External Ids for this team</b><br> 1993 * Type: <b>token</b><br> 1994 * Path: <b>CareTeam.identifier</b><br> 1995 * </p> 1996 */ 1997 @SearchParamDefinition(name = "identifier", path = "CareTeam.identifier", description = "External Ids for this team", type = "token") 1998 public static final String SP_IDENTIFIER = "identifier"; 1999 /** 2000 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2001 * <p> 2002 * Description: <b>External Ids for this team</b><br> 2003 * Type: <b>token</b><br> 2004 * Path: <b>CareTeam.identifier</b><br> 2005 * </p> 2006 */ 2007 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2008 SP_IDENTIFIER); 2009 2010 /** 2011 * Search parameter: <b>patient</b> 2012 * <p> 2013 * Description: <b>Who care team is for</b><br> 2014 * Type: <b>reference</b><br> 2015 * Path: <b>CareTeam.subject</b><br> 2016 * </p> 2017 */ 2018 @SearchParamDefinition(name = "patient", path = "CareTeam.subject.where(resolve() is Patient)", description = "Who care team is for", type = "reference", providesMembershipIn = { 2019 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2020 public static final String SP_PATIENT = "patient"; 2021 /** 2022 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2023 * <p> 2024 * Description: <b>Who care team is for</b><br> 2025 * Type: <b>reference</b><br> 2026 * Path: <b>CareTeam.subject</b><br> 2027 * </p> 2028 */ 2029 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2030 SP_PATIENT); 2031 2032 /** 2033 * Constant for fluent queries to be used to add include statements. Specifies 2034 * the path value of "<b>CareTeam:patient</b>". 2035 */ 2036 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2037 "CareTeam:patient").toLocked(); 2038 2039 /** 2040 * Search parameter: <b>subject</b> 2041 * <p> 2042 * Description: <b>Who care team is for</b><br> 2043 * Type: <b>reference</b><br> 2044 * Path: <b>CareTeam.subject</b><br> 2045 * </p> 2046 */ 2047 @SearchParamDefinition(name = "subject", path = "CareTeam.subject", description = "Who care team is for", type = "reference", target = { 2048 Group.class, Patient.class }) 2049 public static final String SP_SUBJECT = "subject"; 2050 /** 2051 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2052 * <p> 2053 * Description: <b>Who care team is for</b><br> 2054 * Type: <b>reference</b><br> 2055 * Path: <b>CareTeam.subject</b><br> 2056 * </p> 2057 */ 2058 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2059 SP_SUBJECT); 2060 2061 /** 2062 * Constant for fluent queries to be used to add include statements. Specifies 2063 * the path value of "<b>CareTeam:subject</b>". 2064 */ 2065 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2066 "CareTeam:subject").toLocked(); 2067 2068 /** 2069 * Search parameter: <b>encounter</b> 2070 * <p> 2071 * Description: <b>Encounter created as part of</b><br> 2072 * Type: <b>reference</b><br> 2073 * Path: <b>CareTeam.encounter</b><br> 2074 * </p> 2075 */ 2076 @SearchParamDefinition(name = "encounter", path = "CareTeam.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2077 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2078 public static final String SP_ENCOUNTER = "encounter"; 2079 /** 2080 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2081 * <p> 2082 * Description: <b>Encounter created as part of</b><br> 2083 * Type: <b>reference</b><br> 2084 * Path: <b>CareTeam.encounter</b><br> 2085 * </p> 2086 */ 2087 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2088 SP_ENCOUNTER); 2089 2090 /** 2091 * Constant for fluent queries to be used to add include statements. Specifies 2092 * the path value of "<b>CareTeam:encounter</b>". 2093 */ 2094 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2095 "CareTeam:encounter").toLocked(); 2096 2097 /** 2098 * Search parameter: <b>category</b> 2099 * <p> 2100 * Description: <b>Type of team</b><br> 2101 * Type: <b>token</b><br> 2102 * Path: <b>CareTeam.category</b><br> 2103 * </p> 2104 */ 2105 @SearchParamDefinition(name = "category", path = "CareTeam.category", description = "Type of team", type = "token") 2106 public static final String SP_CATEGORY = "category"; 2107 /** 2108 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2109 * <p> 2110 * Description: <b>Type of team</b><br> 2111 * Type: <b>token</b><br> 2112 * Path: <b>CareTeam.category</b><br> 2113 * </p> 2114 */ 2115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2116 SP_CATEGORY); 2117 2118 /** 2119 * Search parameter: <b>participant</b> 2120 * <p> 2121 * Description: <b>Who is involved</b><br> 2122 * Type: <b>reference</b><br> 2123 * Path: <b>CareTeam.participant.member</b><br> 2124 * </p> 2125 */ 2126 @SearchParamDefinition(name = "participant", path = "CareTeam.participant.member", description = "Who is involved", type = "reference", providesMembershipIn = { 2127 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2128 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2129 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, 2130 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2131 public static final String SP_PARTICIPANT = "participant"; 2132 /** 2133 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2134 * <p> 2135 * Description: <b>Who is involved</b><br> 2136 * Type: <b>reference</b><br> 2137 * Path: <b>CareTeam.participant.member</b><br> 2138 * </p> 2139 */ 2140 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2141 SP_PARTICIPANT); 2142 2143 /** 2144 * Constant for fluent queries to be used to add include statements. Specifies 2145 * the path value of "<b>CareTeam:participant</b>". 2146 */ 2147 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 2148 "CareTeam:participant").toLocked(); 2149 2150 /** 2151 * Search parameter: <b>status</b> 2152 * <p> 2153 * Description: <b>proposed | active | suspended | inactive | 2154 * entered-in-error</b><br> 2155 * Type: <b>token</b><br> 2156 * Path: <b>CareTeam.status</b><br> 2157 * </p> 2158 */ 2159 @SearchParamDefinition(name = "status", path = "CareTeam.status", description = "proposed | active | suspended | inactive | entered-in-error", type = "token") 2160 public static final String SP_STATUS = "status"; 2161 /** 2162 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2163 * <p> 2164 * Description: <b>proposed | active | suspended | inactive | 2165 * entered-in-error</b><br> 2166 * Type: <b>token</b><br> 2167 * Path: <b>CareTeam.status</b><br> 2168 * </p> 2169 */ 2170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2171 SP_STATUS); 2172 2173}