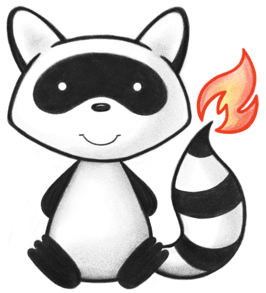
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * The resource ChargeItem describes the provision of healthcare provider 051 * products for a certain patient, therefore referring not only to the product, 052 * but containing in addition details of the provision, like date, time, amounts 053 * and participating organizations and persons. Main Usage of the ChargeItem is 054 * to enable the billing process and internal cost allocation. 055 */ 056@ResourceDef(name = "ChargeItem", profile = "http://hl7.org/fhir/StructureDefinition/ChargeItem") 057public class ChargeItem extends DomainResource { 058 059 public enum ChargeItemStatus { 060 /** 061 * The charge item has been entered, but the charged service is not yet 062 * complete, so it shall not be billed yet but might be used in the context of 063 * pre-authorization. 064 */ 065 PLANNED, 066 /** 067 * The charge item is ready for billing. 068 */ 069 BILLABLE, 070 /** 071 * The charge item has been determined to be not billable (e.g. due to rules 072 * associated with the billing code). 073 */ 074 NOTBILLABLE, 075 /** 076 * The processing of the charge was aborted. 077 */ 078 ABORTED, 079 /** 080 * The charge item has been billed (e.g. a billing engine has generated 081 * financial transactions by applying the associated ruled for the charge item 082 * to the context of the Encounter, and placed them into Claims/Invoices. 083 */ 084 BILLED, 085 /** 086 * The charge item has been entered in error and should not be processed for 087 * billing. 088 */ 089 ENTEREDINERROR, 090 /** 091 * The authoring system does not know which of the status values currently 092 * applies for this charge item Note: This concept is not to be used for "other" 093 * - one of the listed statuses is presumed to apply, it's just not known which 094 * one. 095 */ 096 UNKNOWN, 097 /** 098 * added to help the parsers with the generic types 099 */ 100 NULL; 101 102 public static ChargeItemStatus fromCode(String codeString) throws FHIRException { 103 if (codeString == null || "".equals(codeString)) 104 return null; 105 if ("planned".equals(codeString)) 106 return PLANNED; 107 if ("billable".equals(codeString)) 108 return BILLABLE; 109 if ("not-billable".equals(codeString)) 110 return NOTBILLABLE; 111 if ("aborted".equals(codeString)) 112 return ABORTED; 113 if ("billed".equals(codeString)) 114 return BILLED; 115 if ("entered-in-error".equals(codeString)) 116 return ENTEREDINERROR; 117 if ("unknown".equals(codeString)) 118 return UNKNOWN; 119 if (Configuration.isAcceptInvalidEnums()) 120 return null; 121 else 122 throw new FHIRException("Unknown ChargeItemStatus code '" + codeString + "'"); 123 } 124 125 public String toCode() { 126 switch (this) { 127 case PLANNED: 128 return "planned"; 129 case BILLABLE: 130 return "billable"; 131 case NOTBILLABLE: 132 return "not-billable"; 133 case ABORTED: 134 return "aborted"; 135 case BILLED: 136 return "billed"; 137 case ENTEREDINERROR: 138 return "entered-in-error"; 139 case UNKNOWN: 140 return "unknown"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getSystem() { 149 switch (this) { 150 case PLANNED: 151 return "http://hl7.org/fhir/chargeitem-status"; 152 case BILLABLE: 153 return "http://hl7.org/fhir/chargeitem-status"; 154 case NOTBILLABLE: 155 return "http://hl7.org/fhir/chargeitem-status"; 156 case ABORTED: 157 return "http://hl7.org/fhir/chargeitem-status"; 158 case BILLED: 159 return "http://hl7.org/fhir/chargeitem-status"; 160 case ENTEREDINERROR: 161 return "http://hl7.org/fhir/chargeitem-status"; 162 case UNKNOWN: 163 return "http://hl7.org/fhir/chargeitem-status"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getDefinition() { 172 switch (this) { 173 case PLANNED: 174 return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization."; 175 case BILLABLE: 176 return "The charge item is ready for billing."; 177 case NOTBILLABLE: 178 return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)."; 179 case ABORTED: 180 return "The processing of the charge was aborted."; 181 case BILLED: 182 return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices."; 183 case ENTEREDINERROR: 184 return "The charge item has been entered in error and should not be processed for billing."; 185 case UNKNOWN: 186 return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 187 case NULL: 188 return null; 189 default: 190 return "?"; 191 } 192 } 193 194 public String getDisplay() { 195 switch (this) { 196 case PLANNED: 197 return "Planned"; 198 case BILLABLE: 199 return "Billable"; 200 case NOTBILLABLE: 201 return "Not billable"; 202 case ABORTED: 203 return "Aborted"; 204 case BILLED: 205 return "Billed"; 206 case ENTEREDINERROR: 207 return "Entered in Error"; 208 case UNKNOWN: 209 return "Unknown"; 210 case NULL: 211 return null; 212 default: 213 return "?"; 214 } 215 } 216 } 217 218 public static class ChargeItemStatusEnumFactory implements EnumFactory<ChargeItemStatus> { 219 public ChargeItemStatus fromCode(String codeString) throws IllegalArgumentException { 220 if (codeString == null || "".equals(codeString)) 221 if (codeString == null || "".equals(codeString)) 222 return null; 223 if ("planned".equals(codeString)) 224 return ChargeItemStatus.PLANNED; 225 if ("billable".equals(codeString)) 226 return ChargeItemStatus.BILLABLE; 227 if ("not-billable".equals(codeString)) 228 return ChargeItemStatus.NOTBILLABLE; 229 if ("aborted".equals(codeString)) 230 return ChargeItemStatus.ABORTED; 231 if ("billed".equals(codeString)) 232 return ChargeItemStatus.BILLED; 233 if ("entered-in-error".equals(codeString)) 234 return ChargeItemStatus.ENTEREDINERROR; 235 if ("unknown".equals(codeString)) 236 return ChargeItemStatus.UNKNOWN; 237 throw new IllegalArgumentException("Unknown ChargeItemStatus code '" + codeString + "'"); 238 } 239 240 public Enumeration<ChargeItemStatus> fromType(PrimitiveType<?> code) throws FHIRException { 241 if (code == null) 242 return null; 243 if (code.isEmpty()) 244 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 245 String codeString = code.asStringValue(); 246 if (codeString == null || "".equals(codeString)) 247 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NULL, code); 248 if ("planned".equals(codeString)) 249 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.PLANNED, code); 250 if ("billable".equals(codeString)) 251 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLABLE, code); 252 if ("not-billable".equals(codeString)) 253 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.NOTBILLABLE, code); 254 if ("aborted".equals(codeString)) 255 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ABORTED, code); 256 if ("billed".equals(codeString)) 257 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.BILLED, code); 258 if ("entered-in-error".equals(codeString)) 259 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.ENTEREDINERROR, code); 260 if ("unknown".equals(codeString)) 261 return new Enumeration<ChargeItemStatus>(this, ChargeItemStatus.UNKNOWN, code); 262 throw new FHIRException("Unknown ChargeItemStatus code '" + codeString + "'"); 263 } 264 265 public String toCode(ChargeItemStatus code) { 266 if (code == ChargeItemStatus.NULL) 267 return null; 268 if (code == ChargeItemStatus.PLANNED) 269 return "planned"; 270 if (code == ChargeItemStatus.BILLABLE) 271 return "billable"; 272 if (code == ChargeItemStatus.NOTBILLABLE) 273 return "not-billable"; 274 if (code == ChargeItemStatus.ABORTED) 275 return "aborted"; 276 if (code == ChargeItemStatus.BILLED) 277 return "billed"; 278 if (code == ChargeItemStatus.ENTEREDINERROR) 279 return "entered-in-error"; 280 if (code == ChargeItemStatus.UNKNOWN) 281 return "unknown"; 282 return "?"; 283 } 284 285 public String toSystem(ChargeItemStatus code) { 286 return code.getSystem(); 287 } 288 } 289 290 @Block() 291 public static class ChargeItemPerformerComponent extends BackboneElement implements IBaseBackboneElement { 292 /** 293 * Describes the type of performance or participation(e.g. primary surgeon, 294 * anesthesiologiest, etc.). 295 */ 296 @Child(name = "function", type = { 297 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "What type of performance was done", formalDefinition = "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).") 299 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 300 protected CodeableConcept function; 301 302 /** 303 * The device, practitioner, etc. who performed or participated in the service. 304 */ 305 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 306 Patient.class, Device.class, 307 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 308 @Description(shortDefinition = "Individual who was performing", formalDefinition = "The device, practitioner, etc. who performed or participated in the service.") 309 protected Reference actor; 310 311 /** 312 * The actual object that is the target of the reference (The device, 313 * practitioner, etc. who performed or participated in the service.) 314 */ 315 protected Resource actorTarget; 316 317 private static final long serialVersionUID = 1424001049L; 318 319 /** 320 * Constructor 321 */ 322 public ChargeItemPerformerComponent() { 323 super(); 324 } 325 326 /** 327 * Constructor 328 */ 329 public ChargeItemPerformerComponent(Reference actor) { 330 super(); 331 this.actor = actor; 332 } 333 334 /** 335 * @return {@link #function} (Describes the type of performance or 336 * participation(e.g. primary surgeon, anesthesiologiest, etc.).) 337 */ 338 public CodeableConcept getFunction() { 339 if (this.function == null) 340 if (Configuration.errorOnAutoCreate()) 341 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.function"); 342 else if (Configuration.doAutoCreate()) 343 this.function = new CodeableConcept(); // cc 344 return this.function; 345 } 346 347 public boolean hasFunction() { 348 return this.function != null && !this.function.isEmpty(); 349 } 350 351 /** 352 * @param value {@link #function} (Describes the type of performance or 353 * participation(e.g. primary surgeon, anesthesiologiest, etc.).) 354 */ 355 public ChargeItemPerformerComponent setFunction(CodeableConcept value) { 356 this.function = value; 357 return this; 358 } 359 360 /** 361 * @return {@link #actor} (The device, practitioner, etc. who performed or 362 * participated in the service.) 363 */ 364 public Reference getActor() { 365 if (this.actor == null) 366 if (Configuration.errorOnAutoCreate()) 367 throw new Error("Attempt to auto-create ChargeItemPerformerComponent.actor"); 368 else if (Configuration.doAutoCreate()) 369 this.actor = new Reference(); // cc 370 return this.actor; 371 } 372 373 public boolean hasActor() { 374 return this.actor != null && !this.actor.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #actor} (The device, practitioner, etc. who performed or 379 * participated in the service.) 380 */ 381 public ChargeItemPerformerComponent setActor(Reference value) { 382 this.actor = value; 383 return this; 384 } 385 386 /** 387 * @return {@link #actor} The actual object that is the target of the reference. 388 * The reference library doesn't populate this, but you can use it to 389 * hold the resource if you resolve it. (The device, practitioner, etc. 390 * who performed or participated in the service.) 391 */ 392 public Resource getActorTarget() { 393 return this.actorTarget; 394 } 395 396 /** 397 * @param value {@link #actor} The actual object that is the target of the 398 * reference. The reference library doesn't use these, but you can 399 * use it to hold the resource if you resolve it. (The device, 400 * practitioner, etc. who performed or participated in the 401 * service.) 402 */ 403 public ChargeItemPerformerComponent setActorTarget(Resource value) { 404 this.actorTarget = value; 405 return this; 406 } 407 408 protected void listChildren(List<Property> children) { 409 super.listChildren(children); 410 children.add(new Property("function", "CodeableConcept", 411 "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, 412 function)); 413 children.add(new Property("actor", 414 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 415 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 416 } 417 418 @Override 419 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 420 switch (_hash) { 421 case 1380938712: 422 /* function */ return new Property("function", "CodeableConcept", 423 "Describes the type of performance or participation(e.g. primary surgeon, anesthesiologiest, etc.).", 0, 1, 424 function); 425 case 92645877: 426 /* actor */ return new Property("actor", 427 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 428 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 429 default: 430 return super.getNamedProperty(_hash, _name, _checkValid); 431 } 432 433 } 434 435 @Override 436 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 437 switch (hash) { 438 case 1380938712: 439 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 440 case 92645877: 441 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 442 default: 443 return super.getProperty(hash, name, checkValid); 444 } 445 446 } 447 448 @Override 449 public Base setProperty(int hash, String name, Base value) throws FHIRException { 450 switch (hash) { 451 case 1380938712: // function 452 this.function = castToCodeableConcept(value); // CodeableConcept 453 return value; 454 case 92645877: // actor 455 this.actor = castToReference(value); // Reference 456 return value; 457 default: 458 return super.setProperty(hash, name, value); 459 } 460 461 } 462 463 @Override 464 public Base setProperty(String name, Base value) throws FHIRException { 465 if (name.equals("function")) { 466 this.function = castToCodeableConcept(value); // CodeableConcept 467 } else if (name.equals("actor")) { 468 this.actor = castToReference(value); // Reference 469 } else 470 return super.setProperty(name, value); 471 return value; 472 } 473 474 @Override 475 public void removeChild(String name, Base value) throws FHIRException { 476 if (name.equals("function")) { 477 this.function = null; 478 } else if (name.equals("actor")) { 479 this.actor = null; 480 } else 481 super.removeChild(name, value); 482 483 } 484 485 @Override 486 public Base makeProperty(int hash, String name) throws FHIRException { 487 switch (hash) { 488 case 1380938712: 489 return getFunction(); 490 case 92645877: 491 return getActor(); 492 default: 493 return super.makeProperty(hash, name); 494 } 495 496 } 497 498 @Override 499 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 500 switch (hash) { 501 case 1380938712: 502 /* function */ return new String[] { "CodeableConcept" }; 503 case 92645877: 504 /* actor */ return new String[] { "Reference" }; 505 default: 506 return super.getTypesForProperty(hash, name); 507 } 508 509 } 510 511 @Override 512 public Base addChild(String name) throws FHIRException { 513 if (name.equals("function")) { 514 this.function = new CodeableConcept(); 515 return this.function; 516 } else if (name.equals("actor")) { 517 this.actor = new Reference(); 518 return this.actor; 519 } else 520 return super.addChild(name); 521 } 522 523 public ChargeItemPerformerComponent copy() { 524 ChargeItemPerformerComponent dst = new ChargeItemPerformerComponent(); 525 copyValues(dst); 526 return dst; 527 } 528 529 public void copyValues(ChargeItemPerformerComponent dst) { 530 super.copyValues(dst); 531 dst.function = function == null ? null : function.copy(); 532 dst.actor = actor == null ? null : actor.copy(); 533 } 534 535 @Override 536 public boolean equalsDeep(Base other_) { 537 if (!super.equalsDeep(other_)) 538 return false; 539 if (!(other_ instanceof ChargeItemPerformerComponent)) 540 return false; 541 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 542 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 543 } 544 545 @Override 546 public boolean equalsShallow(Base other_) { 547 if (!super.equalsShallow(other_)) 548 return false; 549 if (!(other_ instanceof ChargeItemPerformerComponent)) 550 return false; 551 ChargeItemPerformerComponent o = (ChargeItemPerformerComponent) other_; 552 return true; 553 } 554 555 public boolean isEmpty() { 556 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 557 } 558 559 public String fhirType() { 560 return "ChargeItem.performer"; 561 562 } 563 564 } 565 566 /** 567 * Identifiers assigned to this event performer or other systems. 568 */ 569 @Child(name = "identifier", type = { 570 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 571 @Description(shortDefinition = "Business Identifier for item", formalDefinition = "Identifiers assigned to this event performer or other systems.") 572 protected List<Identifier> identifier; 573 574 /** 575 * References the (external) source of pricing information, rules of application 576 * for the code this ChargeItem uses. 577 */ 578 @Child(name = "definitionUri", type = { 579 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 580 @Description(shortDefinition = "Defining information about the code of this charge item", formalDefinition = "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.") 581 protected List<UriType> definitionUri; 582 583 /** 584 * References the source of pricing information, rules of application for the 585 * code this ChargeItem uses. 586 */ 587 @Child(name = "definitionCanonical", type = { 588 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 589 @Description(shortDefinition = "Resource defining the code of this ChargeItem", formalDefinition = "References the source of pricing information, rules of application for the code this ChargeItem uses.") 590 protected List<CanonicalType> definitionCanonical; 591 592 /** 593 * The current state of the ChargeItem. 594 */ 595 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 596 @Description(shortDefinition = "planned | billable | not-billable | aborted | billed | entered-in-error | unknown", formalDefinition = "The current state of the ChargeItem.") 597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-status") 598 protected Enumeration<ChargeItemStatus> status; 599 600 /** 601 * ChargeItems can be grouped to larger ChargeItems covering the whole set. 602 */ 603 @Child(name = "partOf", type = { 604 ChargeItem.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 605 @Description(shortDefinition = "Part of referenced ChargeItem", formalDefinition = "ChargeItems can be grouped to larger ChargeItems covering the whole set.") 606 protected List<Reference> partOf; 607 /** 608 * The actual objects that are the target of the reference (ChargeItems can be 609 * grouped to larger ChargeItems covering the whole set.) 610 */ 611 protected List<ChargeItem> partOfTarget; 612 613 /** 614 * A code that identifies the charge, like a billing code. 615 */ 616 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 617 @Description(shortDefinition = "A code that identifies the charge, like a billing code", formalDefinition = "A code that identifies the charge, like a billing code.") 618 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 619 protected CodeableConcept code; 620 621 /** 622 * The individual or set of individuals the action is being or was performed on. 623 */ 624 @Child(name = "subject", type = { Patient.class, 625 Group.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 626 @Description(shortDefinition = "Individual service was done for/to", formalDefinition = "The individual or set of individuals the action is being or was performed on.") 627 protected Reference subject; 628 629 /** 630 * The actual object that is the target of the reference (The individual or set 631 * of individuals the action is being or was performed on.) 632 */ 633 protected Resource subjectTarget; 634 635 /** 636 * The encounter or episode of care that establishes the context for this event. 637 */ 638 @Child(name = "context", type = { Encounter.class, 639 EpisodeOfCare.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 640 @Description(shortDefinition = "Encounter / Episode associated with event", formalDefinition = "The encounter or episode of care that establishes the context for this event.") 641 protected Reference context; 642 643 /** 644 * The actual object that is the target of the reference (The encounter or 645 * episode of care that establishes the context for this event.) 646 */ 647 protected Resource contextTarget; 648 649 /** 650 * Date/time(s) or duration when the charged service was applied. 651 */ 652 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 653 Timing.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 654 @Description(shortDefinition = "When the charged service was applied", formalDefinition = "Date/time(s) or duration when the charged service was applied.") 655 protected Type occurrence; 656 657 /** 658 * Indicates who or what performed or participated in the charged service. 659 */ 660 @Child(name = "performer", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 661 @Description(shortDefinition = "Who performed charged service", formalDefinition = "Indicates who or what performed or participated in the charged service.") 662 protected List<ChargeItemPerformerComponent> performer; 663 664 /** 665 * The organization requesting the service. 666 */ 667 @Child(name = "performingOrganization", type = { 668 Organization.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 669 @Description(shortDefinition = "Organization providing the charged service", formalDefinition = "The organization requesting the service.") 670 protected Reference performingOrganization; 671 672 /** 673 * The actual object that is the target of the reference (The organization 674 * requesting the service.) 675 */ 676 protected Organization performingOrganizationTarget; 677 678 /** 679 * The organization performing the service. 680 */ 681 @Child(name = "requestingOrganization", type = { 682 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 683 @Description(shortDefinition = "Organization requesting the charged service", formalDefinition = "The organization performing the service.") 684 protected Reference requestingOrganization; 685 686 /** 687 * The actual object that is the target of the reference (The organization 688 * performing the service.) 689 */ 690 protected Organization requestingOrganizationTarget; 691 692 /** 693 * The financial cost center permits the tracking of charge attribution. 694 */ 695 @Child(name = "costCenter", type = { 696 Organization.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 697 @Description(shortDefinition = "Organization that has ownership of the (potential, future) revenue", formalDefinition = "The financial cost center permits the tracking of charge attribution.") 698 protected Reference costCenter; 699 700 /** 701 * The actual object that is the target of the reference (The financial cost 702 * center permits the tracking of charge attribution.) 703 */ 704 protected Organization costCenterTarget; 705 706 /** 707 * Quantity of which the charge item has been serviced. 708 */ 709 @Child(name = "quantity", type = { Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 710 @Description(shortDefinition = "Quantity of which the charge item has been serviced", formalDefinition = "Quantity of which the charge item has been serviced.") 711 protected Quantity quantity; 712 713 /** 714 * The anatomical location where the related service has been applied. 715 */ 716 @Child(name = "bodysite", type = { 717 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 718 @Description(shortDefinition = "Anatomical location, if relevant", formalDefinition = "The anatomical location where the related service has been applied.") 719 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 720 protected List<CodeableConcept> bodysite; 721 722 /** 723 * Factor overriding the factor determined by the rules associated with the 724 * code. 725 */ 726 @Child(name = "factorOverride", type = { 727 DecimalType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 728 @Description(shortDefinition = "Factor overriding the associated rules", formalDefinition = "Factor overriding the factor determined by the rules associated with the code.") 729 protected DecimalType factorOverride; 730 731 /** 732 * Total price of the charge overriding the list price associated with the code. 733 */ 734 @Child(name = "priceOverride", type = { 735 Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 736 @Description(shortDefinition = "Price overriding the associated rules", formalDefinition = "Total price of the charge overriding the list price associated with the code.") 737 protected Money priceOverride; 738 739 /** 740 * If the list price or the rule-based factor associated with the code is 741 * overridden, this attribute can capture a text to indicate the reason for this 742 * action. 743 */ 744 @Child(name = "overrideReason", type = { 745 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 746 @Description(shortDefinition = "Reason for overriding the list price/factor", formalDefinition = "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.") 747 protected StringType overrideReason; 748 749 /** 750 * The device, practitioner, etc. who entered the charge item. 751 */ 752 @Child(name = "enterer", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 753 Device.class, RelatedPerson.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 754 @Description(shortDefinition = "Individual who was entering", formalDefinition = "The device, practitioner, etc. who entered the charge item.") 755 protected Reference enterer; 756 757 /** 758 * The actual object that is the target of the reference (The device, 759 * practitioner, etc. who entered the charge item.) 760 */ 761 protected Resource entererTarget; 762 763 /** 764 * Date the charge item was entered. 765 */ 766 @Child(name = "enteredDate", type = { 767 DateTimeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 768 @Description(shortDefinition = "Date the charge item was entered", formalDefinition = "Date the charge item was entered.") 769 protected DateTimeType enteredDate; 770 771 /** 772 * Describes why the event occurred in coded or textual form. 773 */ 774 @Child(name = "reason", type = { 775 CodeableConcept.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 776 @Description(shortDefinition = "Why was the charged service rendered?", formalDefinition = "Describes why the event occurred in coded or textual form.") 777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/icd-10") 778 protected List<CodeableConcept> reason; 779 780 /** 781 * Indicated the rendered service that caused this charge. 782 */ 783 @Child(name = "service", type = { DiagnosticReport.class, ImagingStudy.class, Immunization.class, 784 MedicationAdministration.class, MedicationDispense.class, Observation.class, Procedure.class, 785 SupplyDelivery.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 786 @Description(shortDefinition = "Which rendered service is being charged?", formalDefinition = "Indicated the rendered service that caused this charge.") 787 protected List<Reference> service; 788 /** 789 * The actual objects that are the target of the reference (Indicated the 790 * rendered service that caused this charge.) 791 */ 792 protected List<Resource> serviceTarget; 793 794 /** 795 * Identifies the device, food, drug or other product being charged either by 796 * type code or reference to an instance. 797 */ 798 @Child(name = "product", type = { Device.class, Medication.class, Substance.class, 799 CodeableConcept.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 800 @Description(shortDefinition = "Product charged", formalDefinition = "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.") 801 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-kind") 802 protected Type product; 803 804 /** 805 * Account into which this ChargeItems belongs. 806 */ 807 @Child(name = "account", type = { 808 Account.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 809 @Description(shortDefinition = "Account to place this charge", formalDefinition = "Account into which this ChargeItems belongs.") 810 protected List<Reference> account; 811 /** 812 * The actual objects that are the target of the reference (Account into which 813 * this ChargeItems belongs.) 814 */ 815 protected List<Account> accountTarget; 816 817 /** 818 * Comments made about the event by the performer, subject or other 819 * participants. 820 */ 821 @Child(name = "note", type = { 822 Annotation.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 823 @Description(shortDefinition = "Comments made about the ChargeItem", formalDefinition = "Comments made about the event by the performer, subject or other participants.") 824 protected List<Annotation> note; 825 826 /** 827 * Further information supporting this charge. 828 */ 829 @Child(name = "supportingInformation", type = { 830 Reference.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 831 @Description(shortDefinition = "Further information supporting this charge", formalDefinition = "Further information supporting this charge.") 832 protected List<Reference> supportingInformation; 833 /** 834 * The actual objects that are the target of the reference (Further information 835 * supporting this charge.) 836 */ 837 protected List<Resource> supportingInformationTarget; 838 839 private static final long serialVersionUID = 1748644267L; 840 841 /** 842 * Constructor 843 */ 844 public ChargeItem() { 845 super(); 846 } 847 848 /** 849 * Constructor 850 */ 851 public ChargeItem(Enumeration<ChargeItemStatus> status, CodeableConcept code, Reference subject) { 852 super(); 853 this.status = status; 854 this.code = code; 855 this.subject = subject; 856 } 857 858 /** 859 * @return {@link #identifier} (Identifiers assigned to this event performer or 860 * other systems.) 861 */ 862 public List<Identifier> getIdentifier() { 863 if (this.identifier == null) 864 this.identifier = new ArrayList<Identifier>(); 865 return this.identifier; 866 } 867 868 /** 869 * @return Returns a reference to <code>this</code> for easy method chaining 870 */ 871 public ChargeItem setIdentifier(List<Identifier> theIdentifier) { 872 this.identifier = theIdentifier; 873 return this; 874 } 875 876 public boolean hasIdentifier() { 877 if (this.identifier == null) 878 return false; 879 for (Identifier item : this.identifier) 880 if (!item.isEmpty()) 881 return true; 882 return false; 883 } 884 885 public Identifier addIdentifier() { // 3 886 Identifier t = new Identifier(); 887 if (this.identifier == null) 888 this.identifier = new ArrayList<Identifier>(); 889 this.identifier.add(t); 890 return t; 891 } 892 893 public ChargeItem addIdentifier(Identifier t) { // 3 894 if (t == null) 895 return this; 896 if (this.identifier == null) 897 this.identifier = new ArrayList<Identifier>(); 898 this.identifier.add(t); 899 return this; 900 } 901 902 /** 903 * @return The first repetition of repeating field {@link #identifier}, creating 904 * it if it does not already exist 905 */ 906 public Identifier getIdentifierFirstRep() { 907 if (getIdentifier().isEmpty()) { 908 addIdentifier(); 909 } 910 return getIdentifier().get(0); 911 } 912 913 /** 914 * @return {@link #definitionUri} (References the (external) source of pricing 915 * information, rules of application for the code this ChargeItem uses.) 916 */ 917 public List<UriType> getDefinitionUri() { 918 if (this.definitionUri == null) 919 this.definitionUri = new ArrayList<UriType>(); 920 return this.definitionUri; 921 } 922 923 /** 924 * @return Returns a reference to <code>this</code> for easy method chaining 925 */ 926 public ChargeItem setDefinitionUri(List<UriType> theDefinitionUri) { 927 this.definitionUri = theDefinitionUri; 928 return this; 929 } 930 931 public boolean hasDefinitionUri() { 932 if (this.definitionUri == null) 933 return false; 934 for (UriType item : this.definitionUri) 935 if (!item.isEmpty()) 936 return true; 937 return false; 938 } 939 940 /** 941 * @return {@link #definitionUri} (References the (external) source of pricing 942 * information, rules of application for the code this ChargeItem uses.) 943 */ 944 public UriType addDefinitionUriElement() {// 2 945 UriType t = new UriType(); 946 if (this.definitionUri == null) 947 this.definitionUri = new ArrayList<UriType>(); 948 this.definitionUri.add(t); 949 return t; 950 } 951 952 /** 953 * @param value {@link #definitionUri} (References the (external) source of 954 * pricing information, rules of application for the code this 955 * ChargeItem uses.) 956 */ 957 public ChargeItem addDefinitionUri(String value) { // 1 958 UriType t = new UriType(); 959 t.setValue(value); 960 if (this.definitionUri == null) 961 this.definitionUri = new ArrayList<UriType>(); 962 this.definitionUri.add(t); 963 return this; 964 } 965 966 /** 967 * @param value {@link #definitionUri} (References the (external) source of 968 * pricing information, rules of application for the code this 969 * ChargeItem uses.) 970 */ 971 public boolean hasDefinitionUri(String value) { 972 if (this.definitionUri == null) 973 return false; 974 for (UriType v : this.definitionUri) 975 if (v.getValue().equals(value)) // uri 976 return true; 977 return false; 978 } 979 980 /** 981 * @return {@link #definitionCanonical} (References the source of pricing 982 * information, rules of application for the code this ChargeItem uses.) 983 */ 984 public List<CanonicalType> getDefinitionCanonical() { 985 if (this.definitionCanonical == null) 986 this.definitionCanonical = new ArrayList<CanonicalType>(); 987 return this.definitionCanonical; 988 } 989 990 /** 991 * @return Returns a reference to <code>this</code> for easy method chaining 992 */ 993 public ChargeItem setDefinitionCanonical(List<CanonicalType> theDefinitionCanonical) { 994 this.definitionCanonical = theDefinitionCanonical; 995 return this; 996 } 997 998 public boolean hasDefinitionCanonical() { 999 if (this.definitionCanonical == null) 1000 return false; 1001 for (CanonicalType item : this.definitionCanonical) 1002 if (!item.isEmpty()) 1003 return true; 1004 return false; 1005 } 1006 1007 /** 1008 * @return {@link #definitionCanonical} (References the source of pricing 1009 * information, rules of application for the code this ChargeItem uses.) 1010 */ 1011 public CanonicalType addDefinitionCanonicalElement() {// 2 1012 CanonicalType t = new CanonicalType(); 1013 if (this.definitionCanonical == null) 1014 this.definitionCanonical = new ArrayList<CanonicalType>(); 1015 this.definitionCanonical.add(t); 1016 return t; 1017 } 1018 1019 /** 1020 * @param value {@link #definitionCanonical} (References the source of pricing 1021 * information, rules of application for the code this ChargeItem 1022 * uses.) 1023 */ 1024 public ChargeItem addDefinitionCanonical(String value) { // 1 1025 CanonicalType t = new CanonicalType(); 1026 t.setValue(value); 1027 if (this.definitionCanonical == null) 1028 this.definitionCanonical = new ArrayList<CanonicalType>(); 1029 this.definitionCanonical.add(t); 1030 return this; 1031 } 1032 1033 /** 1034 * @param value {@link #definitionCanonical} (References the source of pricing 1035 * information, rules of application for the code this ChargeItem 1036 * uses.) 1037 */ 1038 public boolean hasDefinitionCanonical(String value) { 1039 if (this.definitionCanonical == null) 1040 return false; 1041 for (CanonicalType v : this.definitionCanonical) 1042 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 1043 return true; 1044 return false; 1045 } 1046 1047 /** 1048 * @return {@link #status} (The current state of the ChargeItem.). This is the 1049 * underlying object with id, value and extensions. The accessor 1050 * "getStatus" gives direct access to the value 1051 */ 1052 public Enumeration<ChargeItemStatus> getStatusElement() { 1053 if (this.status == null) 1054 if (Configuration.errorOnAutoCreate()) 1055 throw new Error("Attempt to auto-create ChargeItem.status"); 1056 else if (Configuration.doAutoCreate()) 1057 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); // bb 1058 return this.status; 1059 } 1060 1061 public boolean hasStatusElement() { 1062 return this.status != null && !this.status.isEmpty(); 1063 } 1064 1065 public boolean hasStatus() { 1066 return this.status != null && !this.status.isEmpty(); 1067 } 1068 1069 /** 1070 * @param value {@link #status} (The current state of the ChargeItem.). This is 1071 * the underlying object with id, value and extensions. The 1072 * accessor "getStatus" gives direct access to the value 1073 */ 1074 public ChargeItem setStatusElement(Enumeration<ChargeItemStatus> value) { 1075 this.status = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return The current state of the ChargeItem. 1081 */ 1082 public ChargeItemStatus getStatus() { 1083 return this.status == null ? null : this.status.getValue(); 1084 } 1085 1086 /** 1087 * @param value The current state of the ChargeItem. 1088 */ 1089 public ChargeItem setStatus(ChargeItemStatus value) { 1090 if (this.status == null) 1091 this.status = new Enumeration<ChargeItemStatus>(new ChargeItemStatusEnumFactory()); 1092 this.status.setValue(value); 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #partOf} (ChargeItems can be grouped to larger ChargeItems 1098 * covering the whole set.) 1099 */ 1100 public List<Reference> getPartOf() { 1101 if (this.partOf == null) 1102 this.partOf = new ArrayList<Reference>(); 1103 return this.partOf; 1104 } 1105 1106 /** 1107 * @return Returns a reference to <code>this</code> for easy method chaining 1108 */ 1109 public ChargeItem setPartOf(List<Reference> thePartOf) { 1110 this.partOf = thePartOf; 1111 return this; 1112 } 1113 1114 public boolean hasPartOf() { 1115 if (this.partOf == null) 1116 return false; 1117 for (Reference item : this.partOf) 1118 if (!item.isEmpty()) 1119 return true; 1120 return false; 1121 } 1122 1123 public Reference addPartOf() { // 3 1124 Reference t = new Reference(); 1125 if (this.partOf == null) 1126 this.partOf = new ArrayList<Reference>(); 1127 this.partOf.add(t); 1128 return t; 1129 } 1130 1131 public ChargeItem addPartOf(Reference t) { // 3 1132 if (t == null) 1133 return this; 1134 if (this.partOf == null) 1135 this.partOf = new ArrayList<Reference>(); 1136 this.partOf.add(t); 1137 return this; 1138 } 1139 1140 /** 1141 * @return The first repetition of repeating field {@link #partOf}, creating it 1142 * if it does not already exist 1143 */ 1144 public Reference getPartOfFirstRep() { 1145 if (getPartOf().isEmpty()) { 1146 addPartOf(); 1147 } 1148 return getPartOf().get(0); 1149 } 1150 1151 /** 1152 * @return {@link #code} (A code that identifies the charge, like a billing 1153 * code.) 1154 */ 1155 public CodeableConcept getCode() { 1156 if (this.code == null) 1157 if (Configuration.errorOnAutoCreate()) 1158 throw new Error("Attempt to auto-create ChargeItem.code"); 1159 else if (Configuration.doAutoCreate()) 1160 this.code = new CodeableConcept(); // cc 1161 return this.code; 1162 } 1163 1164 public boolean hasCode() { 1165 return this.code != null && !this.code.isEmpty(); 1166 } 1167 1168 /** 1169 * @param value {@link #code} (A code that identifies the charge, like a billing 1170 * code.) 1171 */ 1172 public ChargeItem setCode(CodeableConcept value) { 1173 this.code = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #subject} (The individual or set of individuals the action is 1179 * being or was performed on.) 1180 */ 1181 public Reference getSubject() { 1182 if (this.subject == null) 1183 if (Configuration.errorOnAutoCreate()) 1184 throw new Error("Attempt to auto-create ChargeItem.subject"); 1185 else if (Configuration.doAutoCreate()) 1186 this.subject = new Reference(); // cc 1187 return this.subject; 1188 } 1189 1190 public boolean hasSubject() { 1191 return this.subject != null && !this.subject.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #subject} (The individual or set of individuals the 1196 * action is being or was performed on.) 1197 */ 1198 public ChargeItem setSubject(Reference value) { 1199 this.subject = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return {@link #subject} The actual object that is the target of the 1205 * reference. The reference library doesn't populate this, but you can 1206 * use it to hold the resource if you resolve it. (The individual or set 1207 * of individuals the action is being or was performed on.) 1208 */ 1209 public Resource getSubjectTarget() { 1210 return this.subjectTarget; 1211 } 1212 1213 /** 1214 * @param value {@link #subject} The actual object that is the target of the 1215 * reference. The reference library doesn't use these, but you can 1216 * use it to hold the resource if you resolve it. (The individual 1217 * or set of individuals the action is being or was performed on.) 1218 */ 1219 public ChargeItem setSubjectTarget(Resource value) { 1220 this.subjectTarget = value; 1221 return this; 1222 } 1223 1224 /** 1225 * @return {@link #context} (The encounter or episode of care that establishes 1226 * the context for this event.) 1227 */ 1228 public Reference getContext() { 1229 if (this.context == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create ChargeItem.context"); 1232 else if (Configuration.doAutoCreate()) 1233 this.context = new Reference(); // cc 1234 return this.context; 1235 } 1236 1237 public boolean hasContext() { 1238 return this.context != null && !this.context.isEmpty(); 1239 } 1240 1241 /** 1242 * @param value {@link #context} (The encounter or episode of care that 1243 * establishes the context for this event.) 1244 */ 1245 public ChargeItem setContext(Reference value) { 1246 this.context = value; 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #context} The actual object that is the target of the 1252 * reference. The reference library doesn't populate this, but you can 1253 * use it to hold the resource if you resolve it. (The encounter or 1254 * episode of care that establishes the context for this event.) 1255 */ 1256 public Resource getContextTarget() { 1257 return this.contextTarget; 1258 } 1259 1260 /** 1261 * @param value {@link #context} The actual object that is the target of the 1262 * reference. The reference library doesn't use these, but you can 1263 * use it to hold the resource if you resolve it. (The encounter or 1264 * episode of care that establishes the context for this event.) 1265 */ 1266 public ChargeItem setContextTarget(Resource value) { 1267 this.contextTarget = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1273 * service was applied.) 1274 */ 1275 public Type getOccurrence() { 1276 return this.occurrence; 1277 } 1278 1279 /** 1280 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1281 * service was applied.) 1282 */ 1283 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1284 if (this.occurrence == null) 1285 this.occurrence = new DateTimeType(); 1286 if (!(this.occurrence instanceof DateTimeType)) 1287 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1288 + this.occurrence.getClass().getName() + " was encountered"); 1289 return (DateTimeType) this.occurrence; 1290 } 1291 1292 public boolean hasOccurrenceDateTimeType() { 1293 return this.occurrence instanceof DateTimeType; 1294 } 1295 1296 /** 1297 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1298 * service was applied.) 1299 */ 1300 public Period getOccurrencePeriod() throws FHIRException { 1301 if (this.occurrence == null) 1302 this.occurrence = new Period(); 1303 if (!(this.occurrence instanceof Period)) 1304 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1305 + " was encountered"); 1306 return (Period) this.occurrence; 1307 } 1308 1309 public boolean hasOccurrencePeriod() { 1310 return this.occurrence instanceof Period; 1311 } 1312 1313 /** 1314 * @return {@link #occurrence} (Date/time(s) or duration when the charged 1315 * service was applied.) 1316 */ 1317 public Timing getOccurrenceTiming() throws FHIRException { 1318 if (this.occurrence == null) 1319 this.occurrence = new Timing(); 1320 if (!(this.occurrence instanceof Timing)) 1321 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1322 + " was encountered"); 1323 return (Timing) this.occurrence; 1324 } 1325 1326 public boolean hasOccurrenceTiming() { 1327 return this.occurrence instanceof Timing; 1328 } 1329 1330 public boolean hasOccurrence() { 1331 return this.occurrence != null && !this.occurrence.isEmpty(); 1332 } 1333 1334 /** 1335 * @param value {@link #occurrence} (Date/time(s) or duration when the charged 1336 * service was applied.) 1337 */ 1338 public ChargeItem setOccurrence(Type value) { 1339 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1340 throw new Error("Not the right type for ChargeItem.occurrence[x]: " + value.fhirType()); 1341 this.occurrence = value; 1342 return this; 1343 } 1344 1345 /** 1346 * @return {@link #performer} (Indicates who or what performed or participated 1347 * in the charged service.) 1348 */ 1349 public List<ChargeItemPerformerComponent> getPerformer() { 1350 if (this.performer == null) 1351 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1352 return this.performer; 1353 } 1354 1355 /** 1356 * @return Returns a reference to <code>this</code> for easy method chaining 1357 */ 1358 public ChargeItem setPerformer(List<ChargeItemPerformerComponent> thePerformer) { 1359 this.performer = thePerformer; 1360 return this; 1361 } 1362 1363 public boolean hasPerformer() { 1364 if (this.performer == null) 1365 return false; 1366 for (ChargeItemPerformerComponent item : this.performer) 1367 if (!item.isEmpty()) 1368 return true; 1369 return false; 1370 } 1371 1372 public ChargeItemPerformerComponent addPerformer() { // 3 1373 ChargeItemPerformerComponent t = new ChargeItemPerformerComponent(); 1374 if (this.performer == null) 1375 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1376 this.performer.add(t); 1377 return t; 1378 } 1379 1380 public ChargeItem addPerformer(ChargeItemPerformerComponent t) { // 3 1381 if (t == null) 1382 return this; 1383 if (this.performer == null) 1384 this.performer = new ArrayList<ChargeItemPerformerComponent>(); 1385 this.performer.add(t); 1386 return this; 1387 } 1388 1389 /** 1390 * @return The first repetition of repeating field {@link #performer}, creating 1391 * it if it does not already exist 1392 */ 1393 public ChargeItemPerformerComponent getPerformerFirstRep() { 1394 if (getPerformer().isEmpty()) { 1395 addPerformer(); 1396 } 1397 return getPerformer().get(0); 1398 } 1399 1400 /** 1401 * @return {@link #performingOrganization} (The organization requesting the 1402 * service.) 1403 */ 1404 public Reference getPerformingOrganization() { 1405 if (this.performingOrganization == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1408 else if (Configuration.doAutoCreate()) 1409 this.performingOrganization = new Reference(); // cc 1410 return this.performingOrganization; 1411 } 1412 1413 public boolean hasPerformingOrganization() { 1414 return this.performingOrganization != null && !this.performingOrganization.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #performingOrganization} (The organization requesting the 1419 * service.) 1420 */ 1421 public ChargeItem setPerformingOrganization(Reference value) { 1422 this.performingOrganization = value; 1423 return this; 1424 } 1425 1426 /** 1427 * @return {@link #performingOrganization} The actual object that is the target 1428 * of the reference. The reference library doesn't populate this, but 1429 * you can use it to hold the resource if you resolve it. (The 1430 * organization requesting the service.) 1431 */ 1432 public Organization getPerformingOrganizationTarget() { 1433 if (this.performingOrganizationTarget == null) 1434 if (Configuration.errorOnAutoCreate()) 1435 throw new Error("Attempt to auto-create ChargeItem.performingOrganization"); 1436 else if (Configuration.doAutoCreate()) 1437 this.performingOrganizationTarget = new Organization(); // aa 1438 return this.performingOrganizationTarget; 1439 } 1440 1441 /** 1442 * @param value {@link #performingOrganization} The actual object that is the 1443 * target of the reference. The reference library doesn't use 1444 * these, but you can use it to hold the resource if you resolve 1445 * it. (The organization requesting the service.) 1446 */ 1447 public ChargeItem setPerformingOrganizationTarget(Organization value) { 1448 this.performingOrganizationTarget = value; 1449 return this; 1450 } 1451 1452 /** 1453 * @return {@link #requestingOrganization} (The organization performing the 1454 * service.) 1455 */ 1456 public Reference getRequestingOrganization() { 1457 if (this.requestingOrganization == null) 1458 if (Configuration.errorOnAutoCreate()) 1459 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1460 else if (Configuration.doAutoCreate()) 1461 this.requestingOrganization = new Reference(); // cc 1462 return this.requestingOrganization; 1463 } 1464 1465 public boolean hasRequestingOrganization() { 1466 return this.requestingOrganization != null && !this.requestingOrganization.isEmpty(); 1467 } 1468 1469 /** 1470 * @param value {@link #requestingOrganization} (The organization performing the 1471 * service.) 1472 */ 1473 public ChargeItem setRequestingOrganization(Reference value) { 1474 this.requestingOrganization = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return {@link #requestingOrganization} The actual object that is the target 1480 * of the reference. The reference library doesn't populate this, but 1481 * you can use it to hold the resource if you resolve it. (The 1482 * organization performing the service.) 1483 */ 1484 public Organization getRequestingOrganizationTarget() { 1485 if (this.requestingOrganizationTarget == null) 1486 if (Configuration.errorOnAutoCreate()) 1487 throw new Error("Attempt to auto-create ChargeItem.requestingOrganization"); 1488 else if (Configuration.doAutoCreate()) 1489 this.requestingOrganizationTarget = new Organization(); // aa 1490 return this.requestingOrganizationTarget; 1491 } 1492 1493 /** 1494 * @param value {@link #requestingOrganization} The actual object that is the 1495 * target of the reference. The reference library doesn't use 1496 * these, but you can use it to hold the resource if you resolve 1497 * it. (The organization performing the service.) 1498 */ 1499 public ChargeItem setRequestingOrganizationTarget(Organization value) { 1500 this.requestingOrganizationTarget = value; 1501 return this; 1502 } 1503 1504 /** 1505 * @return {@link #costCenter} (The financial cost center permits the tracking 1506 * of charge attribution.) 1507 */ 1508 public Reference getCostCenter() { 1509 if (this.costCenter == null) 1510 if (Configuration.errorOnAutoCreate()) 1511 throw new Error("Attempt to auto-create ChargeItem.costCenter"); 1512 else if (Configuration.doAutoCreate()) 1513 this.costCenter = new Reference(); // cc 1514 return this.costCenter; 1515 } 1516 1517 public boolean hasCostCenter() { 1518 return this.costCenter != null && !this.costCenter.isEmpty(); 1519 } 1520 1521 /** 1522 * @param value {@link #costCenter} (The financial cost center permits the 1523 * tracking of charge attribution.) 1524 */ 1525 public ChargeItem setCostCenter(Reference value) { 1526 this.costCenter = value; 1527 return this; 1528 } 1529 1530 /** 1531 * @return {@link #costCenter} The actual object that is the target of the 1532 * reference. The reference library doesn't populate this, but you can 1533 * use it to hold the resource if you resolve it. (The financial cost 1534 * center permits the tracking of charge attribution.) 1535 */ 1536 public Organization getCostCenterTarget() { 1537 if (this.costCenterTarget == null) 1538 if (Configuration.errorOnAutoCreate()) 1539 throw new Error("Attempt to auto-create ChargeItem.costCenter"); 1540 else if (Configuration.doAutoCreate()) 1541 this.costCenterTarget = new Organization(); // aa 1542 return this.costCenterTarget; 1543 } 1544 1545 /** 1546 * @param value {@link #costCenter} The actual object that is the target of the 1547 * reference. The reference library doesn't use these, but you can 1548 * use it to hold the resource if you resolve it. (The financial 1549 * cost center permits the tracking of charge attribution.) 1550 */ 1551 public ChargeItem setCostCenterTarget(Organization value) { 1552 this.costCenterTarget = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return {@link #quantity} (Quantity of which the charge item has been 1558 * serviced.) 1559 */ 1560 public Quantity getQuantity() { 1561 if (this.quantity == null) 1562 if (Configuration.errorOnAutoCreate()) 1563 throw new Error("Attempt to auto-create ChargeItem.quantity"); 1564 else if (Configuration.doAutoCreate()) 1565 this.quantity = new Quantity(); // cc 1566 return this.quantity; 1567 } 1568 1569 public boolean hasQuantity() { 1570 return this.quantity != null && !this.quantity.isEmpty(); 1571 } 1572 1573 /** 1574 * @param value {@link #quantity} (Quantity of which the charge item has been 1575 * serviced.) 1576 */ 1577 public ChargeItem setQuantity(Quantity value) { 1578 this.quantity = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #bodysite} (The anatomical location where the related service 1584 * has been applied.) 1585 */ 1586 public List<CodeableConcept> getBodysite() { 1587 if (this.bodysite == null) 1588 this.bodysite = new ArrayList<CodeableConcept>(); 1589 return this.bodysite; 1590 } 1591 1592 /** 1593 * @return Returns a reference to <code>this</code> for easy method chaining 1594 */ 1595 public ChargeItem setBodysite(List<CodeableConcept> theBodysite) { 1596 this.bodysite = theBodysite; 1597 return this; 1598 } 1599 1600 public boolean hasBodysite() { 1601 if (this.bodysite == null) 1602 return false; 1603 for (CodeableConcept item : this.bodysite) 1604 if (!item.isEmpty()) 1605 return true; 1606 return false; 1607 } 1608 1609 public CodeableConcept addBodysite() { // 3 1610 CodeableConcept t = new CodeableConcept(); 1611 if (this.bodysite == null) 1612 this.bodysite = new ArrayList<CodeableConcept>(); 1613 this.bodysite.add(t); 1614 return t; 1615 } 1616 1617 public ChargeItem addBodysite(CodeableConcept t) { // 3 1618 if (t == null) 1619 return this; 1620 if (this.bodysite == null) 1621 this.bodysite = new ArrayList<CodeableConcept>(); 1622 this.bodysite.add(t); 1623 return this; 1624 } 1625 1626 /** 1627 * @return The first repetition of repeating field {@link #bodysite}, creating 1628 * it if it does not already exist 1629 */ 1630 public CodeableConcept getBodysiteFirstRep() { 1631 if (getBodysite().isEmpty()) { 1632 addBodysite(); 1633 } 1634 return getBodysite().get(0); 1635 } 1636 1637 /** 1638 * @return {@link #factorOverride} (Factor overriding the factor determined by 1639 * the rules associated with the code.). This is the underlying object 1640 * with id, value and extensions. The accessor "getFactorOverride" gives 1641 * direct access to the value 1642 */ 1643 public DecimalType getFactorOverrideElement() { 1644 if (this.factorOverride == null) 1645 if (Configuration.errorOnAutoCreate()) 1646 throw new Error("Attempt to auto-create ChargeItem.factorOverride"); 1647 else if (Configuration.doAutoCreate()) 1648 this.factorOverride = new DecimalType(); // bb 1649 return this.factorOverride; 1650 } 1651 1652 public boolean hasFactorOverrideElement() { 1653 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1654 } 1655 1656 public boolean hasFactorOverride() { 1657 return this.factorOverride != null && !this.factorOverride.isEmpty(); 1658 } 1659 1660 /** 1661 * @param value {@link #factorOverride} (Factor overriding the factor determined 1662 * by the rules associated with the code.). This is the underlying 1663 * object with id, value and extensions. The accessor 1664 * "getFactorOverride" gives direct access to the value 1665 */ 1666 public ChargeItem setFactorOverrideElement(DecimalType value) { 1667 this.factorOverride = value; 1668 return this; 1669 } 1670 1671 /** 1672 * @return Factor overriding the factor determined by the rules associated with 1673 * the code. 1674 */ 1675 public BigDecimal getFactorOverride() { 1676 return this.factorOverride == null ? null : this.factorOverride.getValue(); 1677 } 1678 1679 /** 1680 * @param value Factor overriding the factor determined by the rules associated 1681 * with the code. 1682 */ 1683 public ChargeItem setFactorOverride(BigDecimal value) { 1684 if (value == null) 1685 this.factorOverride = null; 1686 else { 1687 if (this.factorOverride == null) 1688 this.factorOverride = new DecimalType(); 1689 this.factorOverride.setValue(value); 1690 } 1691 return this; 1692 } 1693 1694 /** 1695 * @param value Factor overriding the factor determined by the rules associated 1696 * with the code. 1697 */ 1698 public ChargeItem setFactorOverride(long value) { 1699 this.factorOverride = new DecimalType(); 1700 this.factorOverride.setValue(value); 1701 return this; 1702 } 1703 1704 /** 1705 * @param value Factor overriding the factor determined by the rules associated 1706 * with the code. 1707 */ 1708 public ChargeItem setFactorOverride(double value) { 1709 this.factorOverride = new DecimalType(); 1710 this.factorOverride.setValue(value); 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #priceOverride} (Total price of the charge overriding the list 1716 * price associated with the code.) 1717 */ 1718 public Money getPriceOverride() { 1719 if (this.priceOverride == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create ChargeItem.priceOverride"); 1722 else if (Configuration.doAutoCreate()) 1723 this.priceOverride = new Money(); // cc 1724 return this.priceOverride; 1725 } 1726 1727 public boolean hasPriceOverride() { 1728 return this.priceOverride != null && !this.priceOverride.isEmpty(); 1729 } 1730 1731 /** 1732 * @param value {@link #priceOverride} (Total price of the charge overriding the 1733 * list price associated with the code.) 1734 */ 1735 public ChargeItem setPriceOverride(Money value) { 1736 this.priceOverride = value; 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #overrideReason} (If the list price or the rule-based factor 1742 * associated with the code is overridden, this attribute can capture a 1743 * text to indicate the reason for this action.). This is the underlying 1744 * object with id, value and extensions. The accessor 1745 * "getOverrideReason" gives direct access to the value 1746 */ 1747 public StringType getOverrideReasonElement() { 1748 if (this.overrideReason == null) 1749 if (Configuration.errorOnAutoCreate()) 1750 throw new Error("Attempt to auto-create ChargeItem.overrideReason"); 1751 else if (Configuration.doAutoCreate()) 1752 this.overrideReason = new StringType(); // bb 1753 return this.overrideReason; 1754 } 1755 1756 public boolean hasOverrideReasonElement() { 1757 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1758 } 1759 1760 public boolean hasOverrideReason() { 1761 return this.overrideReason != null && !this.overrideReason.isEmpty(); 1762 } 1763 1764 /** 1765 * @param value {@link #overrideReason} (If the list price or the rule-based 1766 * factor associated with the code is overridden, this attribute 1767 * can capture a text to indicate the reason for this action.). 1768 * This is the underlying object with id, value and extensions. The 1769 * accessor "getOverrideReason" gives direct access to the value 1770 */ 1771 public ChargeItem setOverrideReasonElement(StringType value) { 1772 this.overrideReason = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return If the list price or the rule-based factor associated with the code 1778 * is overridden, this attribute can capture a text to indicate the 1779 * reason for this action. 1780 */ 1781 public String getOverrideReason() { 1782 return this.overrideReason == null ? null : this.overrideReason.getValue(); 1783 } 1784 1785 /** 1786 * @param value If the list price or the rule-based factor associated with the 1787 * code is overridden, this attribute can capture a text to 1788 * indicate the reason for this action. 1789 */ 1790 public ChargeItem setOverrideReason(String value) { 1791 if (Utilities.noString(value)) 1792 this.overrideReason = null; 1793 else { 1794 if (this.overrideReason == null) 1795 this.overrideReason = new StringType(); 1796 this.overrideReason.setValue(value); 1797 } 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #enterer} (The device, practitioner, etc. who entered the 1803 * charge item.) 1804 */ 1805 public Reference getEnterer() { 1806 if (this.enterer == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create ChargeItem.enterer"); 1809 else if (Configuration.doAutoCreate()) 1810 this.enterer = new Reference(); // cc 1811 return this.enterer; 1812 } 1813 1814 public boolean hasEnterer() { 1815 return this.enterer != null && !this.enterer.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #enterer} (The device, practitioner, etc. who entered the 1820 * charge item.) 1821 */ 1822 public ChargeItem setEnterer(Reference value) { 1823 this.enterer = value; 1824 return this; 1825 } 1826 1827 /** 1828 * @return {@link #enterer} The actual object that is the target of the 1829 * reference. The reference library doesn't populate this, but you can 1830 * use it to hold the resource if you resolve it. (The device, 1831 * practitioner, etc. who entered the charge item.) 1832 */ 1833 public Resource getEntererTarget() { 1834 return this.entererTarget; 1835 } 1836 1837 /** 1838 * @param value {@link #enterer} The actual object that is the target of the 1839 * reference. The reference library doesn't use these, but you can 1840 * use it to hold the resource if you resolve it. (The device, 1841 * practitioner, etc. who entered the charge item.) 1842 */ 1843 public ChargeItem setEntererTarget(Resource value) { 1844 this.entererTarget = value; 1845 return this; 1846 } 1847 1848 /** 1849 * @return {@link #enteredDate} (Date the charge item was entered.). This is the 1850 * underlying object with id, value and extensions. The accessor 1851 * "getEnteredDate" gives direct access to the value 1852 */ 1853 public DateTimeType getEnteredDateElement() { 1854 if (this.enteredDate == null) 1855 if (Configuration.errorOnAutoCreate()) 1856 throw new Error("Attempt to auto-create ChargeItem.enteredDate"); 1857 else if (Configuration.doAutoCreate()) 1858 this.enteredDate = new DateTimeType(); // bb 1859 return this.enteredDate; 1860 } 1861 1862 public boolean hasEnteredDateElement() { 1863 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1864 } 1865 1866 public boolean hasEnteredDate() { 1867 return this.enteredDate != null && !this.enteredDate.isEmpty(); 1868 } 1869 1870 /** 1871 * @param value {@link #enteredDate} (Date the charge item was entered.). This 1872 * is the underlying object with id, value and extensions. The 1873 * accessor "getEnteredDate" gives direct access to the value 1874 */ 1875 public ChargeItem setEnteredDateElement(DateTimeType value) { 1876 this.enteredDate = value; 1877 return this; 1878 } 1879 1880 /** 1881 * @return Date the charge item was entered. 1882 */ 1883 public Date getEnteredDate() { 1884 return this.enteredDate == null ? null : this.enteredDate.getValue(); 1885 } 1886 1887 /** 1888 * @param value Date the charge item was entered. 1889 */ 1890 public ChargeItem setEnteredDate(Date value) { 1891 if (value == null) 1892 this.enteredDate = null; 1893 else { 1894 if (this.enteredDate == null) 1895 this.enteredDate = new DateTimeType(); 1896 this.enteredDate.setValue(value); 1897 } 1898 return this; 1899 } 1900 1901 /** 1902 * @return {@link #reason} (Describes why the event occurred in coded or textual 1903 * form.) 1904 */ 1905 public List<CodeableConcept> getReason() { 1906 if (this.reason == null) 1907 this.reason = new ArrayList<CodeableConcept>(); 1908 return this.reason; 1909 } 1910 1911 /** 1912 * @return Returns a reference to <code>this</code> for easy method chaining 1913 */ 1914 public ChargeItem setReason(List<CodeableConcept> theReason) { 1915 this.reason = theReason; 1916 return this; 1917 } 1918 1919 public boolean hasReason() { 1920 if (this.reason == null) 1921 return false; 1922 for (CodeableConcept item : this.reason) 1923 if (!item.isEmpty()) 1924 return true; 1925 return false; 1926 } 1927 1928 public CodeableConcept addReason() { // 3 1929 CodeableConcept t = new CodeableConcept(); 1930 if (this.reason == null) 1931 this.reason = new ArrayList<CodeableConcept>(); 1932 this.reason.add(t); 1933 return t; 1934 } 1935 1936 public ChargeItem addReason(CodeableConcept t) { // 3 1937 if (t == null) 1938 return this; 1939 if (this.reason == null) 1940 this.reason = new ArrayList<CodeableConcept>(); 1941 this.reason.add(t); 1942 return this; 1943 } 1944 1945 /** 1946 * @return The first repetition of repeating field {@link #reason}, creating it 1947 * if it does not already exist 1948 */ 1949 public CodeableConcept getReasonFirstRep() { 1950 if (getReason().isEmpty()) { 1951 addReason(); 1952 } 1953 return getReason().get(0); 1954 } 1955 1956 /** 1957 * @return {@link #service} (Indicated the rendered service that caused this 1958 * charge.) 1959 */ 1960 public List<Reference> getService() { 1961 if (this.service == null) 1962 this.service = new ArrayList<Reference>(); 1963 return this.service; 1964 } 1965 1966 /** 1967 * @return Returns a reference to <code>this</code> for easy method chaining 1968 */ 1969 public ChargeItem setService(List<Reference> theService) { 1970 this.service = theService; 1971 return this; 1972 } 1973 1974 public boolean hasService() { 1975 if (this.service == null) 1976 return false; 1977 for (Reference item : this.service) 1978 if (!item.isEmpty()) 1979 return true; 1980 return false; 1981 } 1982 1983 public Reference addService() { // 3 1984 Reference t = new Reference(); 1985 if (this.service == null) 1986 this.service = new ArrayList<Reference>(); 1987 this.service.add(t); 1988 return t; 1989 } 1990 1991 public ChargeItem addService(Reference t) { // 3 1992 if (t == null) 1993 return this; 1994 if (this.service == null) 1995 this.service = new ArrayList<Reference>(); 1996 this.service.add(t); 1997 return this; 1998 } 1999 2000 /** 2001 * @return The first repetition of repeating field {@link #service}, creating it 2002 * if it does not already exist 2003 */ 2004 public Reference getServiceFirstRep() { 2005 if (getService().isEmpty()) { 2006 addService(); 2007 } 2008 return getService().get(0); 2009 } 2010 2011 /** 2012 * @return {@link #product} (Identifies the device, food, drug or other product 2013 * being charged either by type code or reference to an instance.) 2014 */ 2015 public Type getProduct() { 2016 return this.product; 2017 } 2018 2019 /** 2020 * @return {@link #product} (Identifies the device, food, drug or other product 2021 * being charged either by type code or reference to an instance.) 2022 */ 2023 public Reference getProductReference() throws FHIRException { 2024 if (this.product == null) 2025 this.product = new Reference(); 2026 if (!(this.product instanceof Reference)) 2027 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.product.getClass().getName() 2028 + " was encountered"); 2029 return (Reference) this.product; 2030 } 2031 2032 public boolean hasProductReference() { 2033 return this.product instanceof Reference; 2034 } 2035 2036 /** 2037 * @return {@link #product} (Identifies the device, food, drug or other product 2038 * being charged either by type code or reference to an instance.) 2039 */ 2040 public CodeableConcept getProductCodeableConcept() throws FHIRException { 2041 if (this.product == null) 2042 this.product = new CodeableConcept(); 2043 if (!(this.product instanceof CodeableConcept)) 2044 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2045 + this.product.getClass().getName() + " was encountered"); 2046 return (CodeableConcept) this.product; 2047 } 2048 2049 public boolean hasProductCodeableConcept() { 2050 return this.product instanceof CodeableConcept; 2051 } 2052 2053 public boolean hasProduct() { 2054 return this.product != null && !this.product.isEmpty(); 2055 } 2056 2057 /** 2058 * @param value {@link #product} (Identifies the device, food, drug or other 2059 * product being charged either by type code or reference to an 2060 * instance.) 2061 */ 2062 public ChargeItem setProduct(Type value) { 2063 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 2064 throw new Error("Not the right type for ChargeItem.product[x]: " + value.fhirType()); 2065 this.product = value; 2066 return this; 2067 } 2068 2069 /** 2070 * @return {@link #account} (Account into which this ChargeItems belongs.) 2071 */ 2072 public List<Reference> getAccount() { 2073 if (this.account == null) 2074 this.account = new ArrayList<Reference>(); 2075 return this.account; 2076 } 2077 2078 /** 2079 * @return Returns a reference to <code>this</code> for easy method chaining 2080 */ 2081 public ChargeItem setAccount(List<Reference> theAccount) { 2082 this.account = theAccount; 2083 return this; 2084 } 2085 2086 public boolean hasAccount() { 2087 if (this.account == null) 2088 return false; 2089 for (Reference item : this.account) 2090 if (!item.isEmpty()) 2091 return true; 2092 return false; 2093 } 2094 2095 public Reference addAccount() { // 3 2096 Reference t = new Reference(); 2097 if (this.account == null) 2098 this.account = new ArrayList<Reference>(); 2099 this.account.add(t); 2100 return t; 2101 } 2102 2103 public ChargeItem addAccount(Reference t) { // 3 2104 if (t == null) 2105 return this; 2106 if (this.account == null) 2107 this.account = new ArrayList<Reference>(); 2108 this.account.add(t); 2109 return this; 2110 } 2111 2112 /** 2113 * @return The first repetition of repeating field {@link #account}, creating it 2114 * if it does not already exist 2115 */ 2116 public Reference getAccountFirstRep() { 2117 if (getAccount().isEmpty()) { 2118 addAccount(); 2119 } 2120 return getAccount().get(0); 2121 } 2122 2123 /** 2124 * @return {@link #note} (Comments made about the event by the performer, 2125 * subject or other participants.) 2126 */ 2127 public List<Annotation> getNote() { 2128 if (this.note == null) 2129 this.note = new ArrayList<Annotation>(); 2130 return this.note; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public ChargeItem setNote(List<Annotation> theNote) { 2137 this.note = theNote; 2138 return this; 2139 } 2140 2141 public boolean hasNote() { 2142 if (this.note == null) 2143 return false; 2144 for (Annotation item : this.note) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 public Annotation addNote() { // 3 2151 Annotation t = new Annotation(); 2152 if (this.note == null) 2153 this.note = new ArrayList<Annotation>(); 2154 this.note.add(t); 2155 return t; 2156 } 2157 2158 public ChargeItem addNote(Annotation t) { // 3 2159 if (t == null) 2160 return this; 2161 if (this.note == null) 2162 this.note = new ArrayList<Annotation>(); 2163 this.note.add(t); 2164 return this; 2165 } 2166 2167 /** 2168 * @return The first repetition of repeating field {@link #note}, creating it if 2169 * it does not already exist 2170 */ 2171 public Annotation getNoteFirstRep() { 2172 if (getNote().isEmpty()) { 2173 addNote(); 2174 } 2175 return getNote().get(0); 2176 } 2177 2178 /** 2179 * @return {@link #supportingInformation} (Further information supporting this 2180 * charge.) 2181 */ 2182 public List<Reference> getSupportingInformation() { 2183 if (this.supportingInformation == null) 2184 this.supportingInformation = new ArrayList<Reference>(); 2185 return this.supportingInformation; 2186 } 2187 2188 /** 2189 * @return Returns a reference to <code>this</code> for easy method chaining 2190 */ 2191 public ChargeItem setSupportingInformation(List<Reference> theSupportingInformation) { 2192 this.supportingInformation = theSupportingInformation; 2193 return this; 2194 } 2195 2196 public boolean hasSupportingInformation() { 2197 if (this.supportingInformation == null) 2198 return false; 2199 for (Reference item : this.supportingInformation) 2200 if (!item.isEmpty()) 2201 return true; 2202 return false; 2203 } 2204 2205 public Reference addSupportingInformation() { // 3 2206 Reference t = new Reference(); 2207 if (this.supportingInformation == null) 2208 this.supportingInformation = new ArrayList<Reference>(); 2209 this.supportingInformation.add(t); 2210 return t; 2211 } 2212 2213 public ChargeItem addSupportingInformation(Reference t) { // 3 2214 if (t == null) 2215 return this; 2216 if (this.supportingInformation == null) 2217 this.supportingInformation = new ArrayList<Reference>(); 2218 this.supportingInformation.add(t); 2219 return this; 2220 } 2221 2222 /** 2223 * @return The first repetition of repeating field 2224 * {@link #supportingInformation}, creating it if it does not already 2225 * exist 2226 */ 2227 public Reference getSupportingInformationFirstRep() { 2228 if (getSupportingInformation().isEmpty()) { 2229 addSupportingInformation(); 2230 } 2231 return getSupportingInformation().get(0); 2232 } 2233 2234 protected void listChildren(List<Property> children) { 2235 super.listChildren(children); 2236 children.add(new Property("identifier", "Identifier", 2237 "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2238 children.add(new Property("definitionUri", "uri", 2239 "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 2240 0, java.lang.Integer.MAX_VALUE, definitionUri)); 2241 children.add(new Property("definitionCanonical", "canonical(ChargeItemDefinition)", 2242 "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, 2243 java.lang.Integer.MAX_VALUE, definitionCanonical)); 2244 children.add(new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status)); 2245 children.add(new Property("partOf", "Reference(ChargeItem)", 2246 "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, 2247 partOf)); 2248 children.add( 2249 new Property("code", "CodeableConcept", "A code that identifies the charge, like a billing code.", 0, 1, code)); 2250 children.add(new Property("subject", "Reference(Patient|Group)", 2251 "The individual or set of individuals the action is being or was performed on.", 0, 1, subject)); 2252 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 2253 "The encounter or episode of care that establishes the context for this event.", 0, 1, context)); 2254 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", 2255 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence)); 2256 children 2257 .add(new Property("performer", "", "Indicates who or what performed or participated in the charged service.", 0, 2258 java.lang.Integer.MAX_VALUE, performer)); 2259 children.add(new Property("performingOrganization", "Reference(Organization)", 2260 "The organization requesting the service.", 0, 1, performingOrganization)); 2261 children.add(new Property("requestingOrganization", "Reference(Organization)", 2262 "The organization performing the service.", 0, 1, requestingOrganization)); 2263 children.add(new Property("costCenter", "Reference(Organization)", 2264 "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter)); 2265 children.add( 2266 new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 0, 1, quantity)); 2267 children.add(new Property("bodysite", "CodeableConcept", 2268 "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, 2269 bodysite)); 2270 children.add(new Property("factorOverride", "decimal", 2271 "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride)); 2272 children.add(new Property("priceOverride", "Money", 2273 "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride)); 2274 children.add(new Property("overrideReason", "string", 2275 "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 2276 0, 1, overrideReason)); 2277 children.add( 2278 new Property("enterer", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 2279 "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer)); 2280 children.add(new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, enteredDate)); 2281 children.add(new Property("reason", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 2282 0, java.lang.Integer.MAX_VALUE, reason)); 2283 children.add(new Property("service", 2284 "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", 2285 "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service)); 2286 children.add(new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2287 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2288 0, 1, product)); 2289 children.add(new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 0, 2290 java.lang.Integer.MAX_VALUE, account)); 2291 children.add(new Property("note", "Annotation", 2292 "Comments made about the event by the performer, subject or other participants.", 0, 2293 java.lang.Integer.MAX_VALUE, note)); 2294 children.add(new Property("supportingInformation", "Reference(Any)", "Further information supporting this charge.", 2295 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2296 } 2297 2298 @Override 2299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2300 switch (_hash) { 2301 case -1618432855: 2302 /* identifier */ return new Property("identifier", "Identifier", 2303 "Identifiers assigned to this event performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 2304 case -1139428583: 2305 /* definitionUri */ return new Property("definitionUri", "uri", 2306 "References the (external) source of pricing information, rules of application for the code this ChargeItem uses.", 2307 0, java.lang.Integer.MAX_VALUE, definitionUri); 2308 case 933485793: 2309 /* definitionCanonical */ return new Property("definitionCanonical", "canonical(ChargeItemDefinition)", 2310 "References the source of pricing information, rules of application for the code this ChargeItem uses.", 0, 2311 java.lang.Integer.MAX_VALUE, definitionCanonical); 2312 case -892481550: 2313 /* status */ return new Property("status", "code", "The current state of the ChargeItem.", 0, 1, status); 2314 case -995410646: 2315 /* partOf */ return new Property("partOf", "Reference(ChargeItem)", 2316 "ChargeItems can be grouped to larger ChargeItems covering the whole set.", 0, java.lang.Integer.MAX_VALUE, 2317 partOf); 2318 case 3059181: 2319 /* code */ return new Property("code", "CodeableConcept", 2320 "A code that identifies the charge, like a billing code.", 0, 1, code); 2321 case -1867885268: 2322 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2323 "The individual or set of individuals the action is being or was performed on.", 0, 1, subject); 2324 case 951530927: 2325 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 2326 "The encounter or episode of care that establishes the context for this event.", 0, 1, context); 2327 case -2022646513: 2328 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2329 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2330 case 1687874001: 2331 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2332 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2333 case -298443636: 2334 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2335 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2336 case 1397156594: 2337 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2338 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2339 case 1515218299: 2340 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 2341 "Date/time(s) or duration when the charged service was applied.", 0, 1, occurrence); 2342 case 481140686: 2343 /* performer */ return new Property("performer", "", 2344 "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, 2345 performer); 2346 case 1273192628: 2347 /* performingOrganization */ return new Property("performingOrganization", "Reference(Organization)", 2348 "The organization requesting the service.", 0, 1, performingOrganization); 2349 case 1279054790: 2350 /* requestingOrganization */ return new Property("requestingOrganization", "Reference(Organization)", 2351 "The organization performing the service.", 0, 1, requestingOrganization); 2352 case -593192318: 2353 /* costCenter */ return new Property("costCenter", "Reference(Organization)", 2354 "The financial cost center permits the tracking of charge attribution.", 0, 1, costCenter); 2355 case -1285004149: 2356 /* quantity */ return new Property("quantity", "Quantity", "Quantity of which the charge item has been serviced.", 2357 0, 1, quantity); 2358 case 1703573481: 2359 /* bodysite */ return new Property("bodysite", "CodeableConcept", 2360 "The anatomical location where the related service has been applied.", 0, java.lang.Integer.MAX_VALUE, 2361 bodysite); 2362 case -451233221: 2363 /* factorOverride */ return new Property("factorOverride", "decimal", 2364 "Factor overriding the factor determined by the rules associated with the code.", 0, 1, factorOverride); 2365 case -216803275: 2366 /* priceOverride */ return new Property("priceOverride", "Money", 2367 "Total price of the charge overriding the list price associated with the code.", 0, 1, priceOverride); 2368 case -742878928: 2369 /* overrideReason */ return new Property("overrideReason", "string", 2370 "If the list price or the rule-based factor associated with the code is overridden, this attribute can capture a text to indicate the reason for this action.", 2371 0, 1, overrideReason); 2372 case -1591951995: 2373 /* enterer */ return new Property("enterer", 2374 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 2375 "The device, practitioner, etc. who entered the charge item.", 0, 1, enterer); 2376 case 555978181: 2377 /* enteredDate */ return new Property("enteredDate", "dateTime", "Date the charge item was entered.", 0, 1, 2378 enteredDate); 2379 case -934964668: 2380 /* reason */ return new Property("reason", "CodeableConcept", 2381 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 2382 case 1984153269: 2383 /* service */ return new Property("service", 2384 "Reference(DiagnosticReport|ImagingStudy|Immunization|MedicationAdministration|MedicationDispense|Observation|Procedure|SupplyDelivery)", 2385 "Indicated the rendered service that caused this charge.", 0, java.lang.Integer.MAX_VALUE, service); 2386 case 1753005361: 2387 /* product[x] */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2388 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2389 0, 1, product); 2390 case -309474065: 2391 /* product */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2392 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2393 0, 1, product); 2394 case -669667556: 2395 /* productReference */ return new Property("product[x]", "Reference(Device|Medication|Substance)|CodeableConcept", 2396 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2397 0, 1, product); 2398 case 906854066: 2399 /* productCodeableConcept */ return new Property("product[x]", 2400 "Reference(Device|Medication|Substance)|CodeableConcept", 2401 "Identifies the device, food, drug or other product being charged either by type code or reference to an instance.", 2402 0, 1, product); 2403 case -1177318867: 2404 /* account */ return new Property("account", "Reference(Account)", "Account into which this ChargeItems belongs.", 2405 0, java.lang.Integer.MAX_VALUE, account); 2406 case 3387378: 2407 /* note */ return new Property("note", "Annotation", 2408 "Comments made about the event by the performer, subject or other participants.", 0, 2409 java.lang.Integer.MAX_VALUE, note); 2410 case -1248768647: 2411 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2412 "Further information supporting this charge.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2413 default: 2414 return super.getNamedProperty(_hash, _name, _checkValid); 2415 } 2416 2417 } 2418 2419 @Override 2420 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2421 switch (hash) { 2422 case -1618432855: 2423 /* identifier */ return this.identifier == null ? new Base[0] 2424 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2425 case -1139428583: 2426 /* definitionUri */ return this.definitionUri == null ? new Base[0] 2427 : this.definitionUri.toArray(new Base[this.definitionUri.size()]); // UriType 2428 case 933485793: 2429 /* definitionCanonical */ return this.definitionCanonical == null ? new Base[0] 2430 : this.definitionCanonical.toArray(new Base[this.definitionCanonical.size()]); // CanonicalType 2431 case -892481550: 2432 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ChargeItemStatus> 2433 case -995410646: 2434 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2435 case 3059181: 2436 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2437 case -1867885268: 2438 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2439 case 951530927: 2440 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 2441 case 1687874001: 2442 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2443 case 481140686: 2444 /* performer */ return this.performer == null ? new Base[0] 2445 : this.performer.toArray(new Base[this.performer.size()]); // ChargeItemPerformerComponent 2446 case 1273192628: 2447 /* performingOrganization */ return this.performingOrganization == null ? new Base[0] 2448 : new Base[] { this.performingOrganization }; // Reference 2449 case 1279054790: 2450 /* requestingOrganization */ return this.requestingOrganization == null ? new Base[0] 2451 : new Base[] { this.requestingOrganization }; // Reference 2452 case -593192318: 2453 /* costCenter */ return this.costCenter == null ? new Base[0] : new Base[] { this.costCenter }; // Reference 2454 case -1285004149: 2455 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2456 case 1703573481: 2457 /* bodysite */ return this.bodysite == null ? new Base[0] : this.bodysite.toArray(new Base[this.bodysite.size()]); // CodeableConcept 2458 case -451233221: 2459 /* factorOverride */ return this.factorOverride == null ? new Base[0] : new Base[] { this.factorOverride }; // DecimalType 2460 case -216803275: 2461 /* priceOverride */ return this.priceOverride == null ? new Base[0] : new Base[] { this.priceOverride }; // Money 2462 case -742878928: 2463 /* overrideReason */ return this.overrideReason == null ? new Base[0] : new Base[] { this.overrideReason }; // StringType 2464 case -1591951995: 2465 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 2466 case 555978181: 2467 /* enteredDate */ return this.enteredDate == null ? new Base[0] : new Base[] { this.enteredDate }; // DateTimeType 2468 case -934964668: 2469 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 2470 case 1984153269: 2471 /* service */ return this.service == null ? new Base[0] : this.service.toArray(new Base[this.service.size()]); // Reference 2472 case -309474065: 2473 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // Type 2474 case -1177318867: 2475 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 2476 case 3387378: 2477 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2478 case -1248768647: 2479 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2480 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2481 default: 2482 return super.getProperty(hash, name, checkValid); 2483 } 2484 2485 } 2486 2487 @Override 2488 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2489 switch (hash) { 2490 case -1618432855: // identifier 2491 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2492 return value; 2493 case -1139428583: // definitionUri 2494 this.getDefinitionUri().add(castToUri(value)); // UriType 2495 return value; 2496 case 933485793: // definitionCanonical 2497 this.getDefinitionCanonical().add(castToCanonical(value)); // CanonicalType 2498 return value; 2499 case -892481550: // status 2500 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 2501 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 2502 return value; 2503 case -995410646: // partOf 2504 this.getPartOf().add(castToReference(value)); // Reference 2505 return value; 2506 case 3059181: // code 2507 this.code = castToCodeableConcept(value); // CodeableConcept 2508 return value; 2509 case -1867885268: // subject 2510 this.subject = castToReference(value); // Reference 2511 return value; 2512 case 951530927: // context 2513 this.context = castToReference(value); // Reference 2514 return value; 2515 case 1687874001: // occurrence 2516 this.occurrence = castToType(value); // Type 2517 return value; 2518 case 481140686: // performer 2519 this.getPerformer().add((ChargeItemPerformerComponent) value); // ChargeItemPerformerComponent 2520 return value; 2521 case 1273192628: // performingOrganization 2522 this.performingOrganization = castToReference(value); // Reference 2523 return value; 2524 case 1279054790: // requestingOrganization 2525 this.requestingOrganization = castToReference(value); // Reference 2526 return value; 2527 case -593192318: // costCenter 2528 this.costCenter = castToReference(value); // Reference 2529 return value; 2530 case -1285004149: // quantity 2531 this.quantity = castToQuantity(value); // Quantity 2532 return value; 2533 case 1703573481: // bodysite 2534 this.getBodysite().add(castToCodeableConcept(value)); // CodeableConcept 2535 return value; 2536 case -451233221: // factorOverride 2537 this.factorOverride = castToDecimal(value); // DecimalType 2538 return value; 2539 case -216803275: // priceOverride 2540 this.priceOverride = castToMoney(value); // Money 2541 return value; 2542 case -742878928: // overrideReason 2543 this.overrideReason = castToString(value); // StringType 2544 return value; 2545 case -1591951995: // enterer 2546 this.enterer = castToReference(value); // Reference 2547 return value; 2548 case 555978181: // enteredDate 2549 this.enteredDate = castToDateTime(value); // DateTimeType 2550 return value; 2551 case -934964668: // reason 2552 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 2553 return value; 2554 case 1984153269: // service 2555 this.getService().add(castToReference(value)); // Reference 2556 return value; 2557 case -309474065: // product 2558 this.product = castToType(value); // Type 2559 return value; 2560 case -1177318867: // account 2561 this.getAccount().add(castToReference(value)); // Reference 2562 return value; 2563 case 3387378: // note 2564 this.getNote().add(castToAnnotation(value)); // Annotation 2565 return value; 2566 case -1248768647: // supportingInformation 2567 this.getSupportingInformation().add(castToReference(value)); // Reference 2568 return value; 2569 default: 2570 return super.setProperty(hash, name, value); 2571 } 2572 2573 } 2574 2575 @Override 2576 public Base setProperty(String name, Base value) throws FHIRException { 2577 if (name.equals("identifier")) { 2578 this.getIdentifier().add(castToIdentifier(value)); 2579 } else if (name.equals("definitionUri")) { 2580 this.getDefinitionUri().add(castToUri(value)); 2581 } else if (name.equals("definitionCanonical")) { 2582 this.getDefinitionCanonical().add(castToCanonical(value)); 2583 } else if (name.equals("status")) { 2584 value = new ChargeItemStatusEnumFactory().fromType(castToCode(value)); 2585 this.status = (Enumeration) value; // Enumeration<ChargeItemStatus> 2586 } else if (name.equals("partOf")) { 2587 this.getPartOf().add(castToReference(value)); 2588 } else if (name.equals("code")) { 2589 this.code = castToCodeableConcept(value); // CodeableConcept 2590 } else if (name.equals("subject")) { 2591 this.subject = castToReference(value); // Reference 2592 } else if (name.equals("context")) { 2593 this.context = castToReference(value); // Reference 2594 } else if (name.equals("occurrence[x]")) { 2595 this.occurrence = castToType(value); // Type 2596 } else if (name.equals("performer")) { 2597 this.getPerformer().add((ChargeItemPerformerComponent) value); 2598 } else if (name.equals("performingOrganization")) { 2599 this.performingOrganization = castToReference(value); // Reference 2600 } else if (name.equals("requestingOrganization")) { 2601 this.requestingOrganization = castToReference(value); // Reference 2602 } else if (name.equals("costCenter")) { 2603 this.costCenter = castToReference(value); // Reference 2604 } else if (name.equals("quantity")) { 2605 this.quantity = castToQuantity(value); // Quantity 2606 } else if (name.equals("bodysite")) { 2607 this.getBodysite().add(castToCodeableConcept(value)); 2608 } else if (name.equals("factorOverride")) { 2609 this.factorOverride = castToDecimal(value); // DecimalType 2610 } else if (name.equals("priceOverride")) { 2611 this.priceOverride = castToMoney(value); // Money 2612 } else if (name.equals("overrideReason")) { 2613 this.overrideReason = castToString(value); // StringType 2614 } else if (name.equals("enterer")) { 2615 this.enterer = castToReference(value); // Reference 2616 } else if (name.equals("enteredDate")) { 2617 this.enteredDate = castToDateTime(value); // DateTimeType 2618 } else if (name.equals("reason")) { 2619 this.getReason().add(castToCodeableConcept(value)); 2620 } else if (name.equals("service")) { 2621 this.getService().add(castToReference(value)); 2622 } else if (name.equals("product[x]")) { 2623 this.product = castToType(value); // Type 2624 } else if (name.equals("account")) { 2625 this.getAccount().add(castToReference(value)); 2626 } else if (name.equals("note")) { 2627 this.getNote().add(castToAnnotation(value)); 2628 } else if (name.equals("supportingInformation")) { 2629 this.getSupportingInformation().add(castToReference(value)); 2630 } else 2631 return super.setProperty(name, value); 2632 return value; 2633 } 2634 2635 @Override 2636 public void removeChild(String name, Base value) throws FHIRException { 2637 if (name.equals("identifier")) { 2638 this.getIdentifier().remove(castToIdentifier(value)); 2639 } else if (name.equals("definitionUri")) { 2640 this.getDefinitionUri().remove(castToUri(value)); 2641 } else if (name.equals("definitionCanonical")) { 2642 this.getDefinitionCanonical().remove(castToCanonical(value)); 2643 } else if (name.equals("status")) { 2644 this.status = null; 2645 } else if (name.equals("partOf")) { 2646 this.getPartOf().remove(castToReference(value)); 2647 } else if (name.equals("code")) { 2648 this.code = null; 2649 } else if (name.equals("subject")) { 2650 this.subject = null; 2651 } else if (name.equals("context")) { 2652 this.context = null; 2653 } else if (name.equals("occurrence[x]")) { 2654 this.occurrence = null; 2655 } else if (name.equals("performer")) { 2656 this.getPerformer().remove((ChargeItemPerformerComponent) value); 2657 } else if (name.equals("performingOrganization")) { 2658 this.performingOrganization = null; 2659 } else if (name.equals("requestingOrganization")) { 2660 this.requestingOrganization = null; 2661 } else if (name.equals("costCenter")) { 2662 this.costCenter = null; 2663 } else if (name.equals("quantity")) { 2664 this.quantity = null; 2665 } else if (name.equals("bodysite")) { 2666 this.getBodysite().remove(castToCodeableConcept(value)); 2667 } else if (name.equals("factorOverride")) { 2668 this.factorOverride = null; 2669 } else if (name.equals("priceOverride")) { 2670 this.priceOverride = null; 2671 } else if (name.equals("overrideReason")) { 2672 this.overrideReason = null; 2673 } else if (name.equals("enterer")) { 2674 this.enterer = null; 2675 } else if (name.equals("enteredDate")) { 2676 this.enteredDate = null; 2677 } else if (name.equals("reason")) { 2678 this.getReason().remove(castToCodeableConcept(value)); 2679 } else if (name.equals("service")) { 2680 this.getService().remove(castToReference(value)); 2681 } else if (name.equals("product[x]")) { 2682 this.product = null; 2683 } else if (name.equals("account")) { 2684 this.getAccount().remove(castToReference(value)); 2685 } else if (name.equals("note")) { 2686 this.getNote().remove(castToAnnotation(value)); 2687 } else if (name.equals("supportingInformation")) { 2688 this.getSupportingInformation().remove(castToReference(value)); 2689 } else 2690 super.removeChild(name, value); 2691 2692 } 2693 2694 @Override 2695 public Base makeProperty(int hash, String name) throws FHIRException { 2696 switch (hash) { 2697 case -1618432855: 2698 return addIdentifier(); 2699 case -1139428583: 2700 return addDefinitionUriElement(); 2701 case 933485793: 2702 return addDefinitionCanonicalElement(); 2703 case -892481550: 2704 return getStatusElement(); 2705 case -995410646: 2706 return addPartOf(); 2707 case 3059181: 2708 return getCode(); 2709 case -1867885268: 2710 return getSubject(); 2711 case 951530927: 2712 return getContext(); 2713 case -2022646513: 2714 return getOccurrence(); 2715 case 1687874001: 2716 return getOccurrence(); 2717 case 481140686: 2718 return addPerformer(); 2719 case 1273192628: 2720 return getPerformingOrganization(); 2721 case 1279054790: 2722 return getRequestingOrganization(); 2723 case -593192318: 2724 return getCostCenter(); 2725 case -1285004149: 2726 return getQuantity(); 2727 case 1703573481: 2728 return addBodysite(); 2729 case -451233221: 2730 return getFactorOverrideElement(); 2731 case -216803275: 2732 return getPriceOverride(); 2733 case -742878928: 2734 return getOverrideReasonElement(); 2735 case -1591951995: 2736 return getEnterer(); 2737 case 555978181: 2738 return getEnteredDateElement(); 2739 case -934964668: 2740 return addReason(); 2741 case 1984153269: 2742 return addService(); 2743 case 1753005361: 2744 return getProduct(); 2745 case -309474065: 2746 return getProduct(); 2747 case -1177318867: 2748 return addAccount(); 2749 case 3387378: 2750 return addNote(); 2751 case -1248768647: 2752 return addSupportingInformation(); 2753 default: 2754 return super.makeProperty(hash, name); 2755 } 2756 2757 } 2758 2759 @Override 2760 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2761 switch (hash) { 2762 case -1618432855: 2763 /* identifier */ return new String[] { "Identifier" }; 2764 case -1139428583: 2765 /* definitionUri */ return new String[] { "uri" }; 2766 case 933485793: 2767 /* definitionCanonical */ return new String[] { "canonical" }; 2768 case -892481550: 2769 /* status */ return new String[] { "code" }; 2770 case -995410646: 2771 /* partOf */ return new String[] { "Reference" }; 2772 case 3059181: 2773 /* code */ return new String[] { "CodeableConcept" }; 2774 case -1867885268: 2775 /* subject */ return new String[] { "Reference" }; 2776 case 951530927: 2777 /* context */ return new String[] { "Reference" }; 2778 case 1687874001: 2779 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 2780 case 481140686: 2781 /* performer */ return new String[] {}; 2782 case 1273192628: 2783 /* performingOrganization */ return new String[] { "Reference" }; 2784 case 1279054790: 2785 /* requestingOrganization */ return new String[] { "Reference" }; 2786 case -593192318: 2787 /* costCenter */ return new String[] { "Reference" }; 2788 case -1285004149: 2789 /* quantity */ return new String[] { "Quantity" }; 2790 case 1703573481: 2791 /* bodysite */ return new String[] { "CodeableConcept" }; 2792 case -451233221: 2793 /* factorOverride */ return new String[] { "decimal" }; 2794 case -216803275: 2795 /* priceOverride */ return new String[] { "Money" }; 2796 case -742878928: 2797 /* overrideReason */ return new String[] { "string" }; 2798 case -1591951995: 2799 /* enterer */ return new String[] { "Reference" }; 2800 case 555978181: 2801 /* enteredDate */ return new String[] { "dateTime" }; 2802 case -934964668: 2803 /* reason */ return new String[] { "CodeableConcept" }; 2804 case 1984153269: 2805 /* service */ return new String[] { "Reference" }; 2806 case -309474065: 2807 /* product */ return new String[] { "Reference", "CodeableConcept" }; 2808 case -1177318867: 2809 /* account */ return new String[] { "Reference" }; 2810 case 3387378: 2811 /* note */ return new String[] { "Annotation" }; 2812 case -1248768647: 2813 /* supportingInformation */ return new String[] { "Reference" }; 2814 default: 2815 return super.getTypesForProperty(hash, name); 2816 } 2817 2818 } 2819 2820 @Override 2821 public Base addChild(String name) throws FHIRException { 2822 if (name.equals("identifier")) { 2823 return addIdentifier(); 2824 } else if (name.equals("definitionUri")) { 2825 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionUri"); 2826 } else if (name.equals("definitionCanonical")) { 2827 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.definitionCanonical"); 2828 } else if (name.equals("status")) { 2829 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.status"); 2830 } else if (name.equals("partOf")) { 2831 return addPartOf(); 2832 } else if (name.equals("code")) { 2833 this.code = new CodeableConcept(); 2834 return this.code; 2835 } else if (name.equals("subject")) { 2836 this.subject = new Reference(); 2837 return this.subject; 2838 } else if (name.equals("context")) { 2839 this.context = new Reference(); 2840 return this.context; 2841 } else if (name.equals("occurrenceDateTime")) { 2842 this.occurrence = new DateTimeType(); 2843 return this.occurrence; 2844 } else if (name.equals("occurrencePeriod")) { 2845 this.occurrence = new Period(); 2846 return this.occurrence; 2847 } else if (name.equals("occurrenceTiming")) { 2848 this.occurrence = new Timing(); 2849 return this.occurrence; 2850 } else if (name.equals("performer")) { 2851 return addPerformer(); 2852 } else if (name.equals("performingOrganization")) { 2853 this.performingOrganization = new Reference(); 2854 return this.performingOrganization; 2855 } else if (name.equals("requestingOrganization")) { 2856 this.requestingOrganization = new Reference(); 2857 return this.requestingOrganization; 2858 } else if (name.equals("costCenter")) { 2859 this.costCenter = new Reference(); 2860 return this.costCenter; 2861 } else if (name.equals("quantity")) { 2862 this.quantity = new Quantity(); 2863 return this.quantity; 2864 } else if (name.equals("bodysite")) { 2865 return addBodysite(); 2866 } else if (name.equals("factorOverride")) { 2867 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.factorOverride"); 2868 } else if (name.equals("priceOverride")) { 2869 this.priceOverride = new Money(); 2870 return this.priceOverride; 2871 } else if (name.equals("overrideReason")) { 2872 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.overrideReason"); 2873 } else if (name.equals("enterer")) { 2874 this.enterer = new Reference(); 2875 return this.enterer; 2876 } else if (name.equals("enteredDate")) { 2877 throw new FHIRException("Cannot call addChild on a singleton property ChargeItem.enteredDate"); 2878 } else if (name.equals("reason")) { 2879 return addReason(); 2880 } else if (name.equals("service")) { 2881 return addService(); 2882 } else if (name.equals("productReference")) { 2883 this.product = new Reference(); 2884 return this.product; 2885 } else if (name.equals("productCodeableConcept")) { 2886 this.product = new CodeableConcept(); 2887 return this.product; 2888 } else if (name.equals("account")) { 2889 return addAccount(); 2890 } else if (name.equals("note")) { 2891 return addNote(); 2892 } else if (name.equals("supportingInformation")) { 2893 return addSupportingInformation(); 2894 } else 2895 return super.addChild(name); 2896 } 2897 2898 public String fhirType() { 2899 return "ChargeItem"; 2900 2901 } 2902 2903 public ChargeItem copy() { 2904 ChargeItem dst = new ChargeItem(); 2905 copyValues(dst); 2906 return dst; 2907 } 2908 2909 public void copyValues(ChargeItem dst) { 2910 super.copyValues(dst); 2911 if (identifier != null) { 2912 dst.identifier = new ArrayList<Identifier>(); 2913 for (Identifier i : identifier) 2914 dst.identifier.add(i.copy()); 2915 } 2916 ; 2917 if (definitionUri != null) { 2918 dst.definitionUri = new ArrayList<UriType>(); 2919 for (UriType i : definitionUri) 2920 dst.definitionUri.add(i.copy()); 2921 } 2922 ; 2923 if (definitionCanonical != null) { 2924 dst.definitionCanonical = new ArrayList<CanonicalType>(); 2925 for (CanonicalType i : definitionCanonical) 2926 dst.definitionCanonical.add(i.copy()); 2927 } 2928 ; 2929 dst.status = status == null ? null : status.copy(); 2930 if (partOf != null) { 2931 dst.partOf = new ArrayList<Reference>(); 2932 for (Reference i : partOf) 2933 dst.partOf.add(i.copy()); 2934 } 2935 ; 2936 dst.code = code == null ? null : code.copy(); 2937 dst.subject = subject == null ? null : subject.copy(); 2938 dst.context = context == null ? null : context.copy(); 2939 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2940 if (performer != null) { 2941 dst.performer = new ArrayList<ChargeItemPerformerComponent>(); 2942 for (ChargeItemPerformerComponent i : performer) 2943 dst.performer.add(i.copy()); 2944 } 2945 ; 2946 dst.performingOrganization = performingOrganization == null ? null : performingOrganization.copy(); 2947 dst.requestingOrganization = requestingOrganization == null ? null : requestingOrganization.copy(); 2948 dst.costCenter = costCenter == null ? null : costCenter.copy(); 2949 dst.quantity = quantity == null ? null : quantity.copy(); 2950 if (bodysite != null) { 2951 dst.bodysite = new ArrayList<CodeableConcept>(); 2952 for (CodeableConcept i : bodysite) 2953 dst.bodysite.add(i.copy()); 2954 } 2955 ; 2956 dst.factorOverride = factorOverride == null ? null : factorOverride.copy(); 2957 dst.priceOverride = priceOverride == null ? null : priceOverride.copy(); 2958 dst.overrideReason = overrideReason == null ? null : overrideReason.copy(); 2959 dst.enterer = enterer == null ? null : enterer.copy(); 2960 dst.enteredDate = enteredDate == null ? null : enteredDate.copy(); 2961 if (reason != null) { 2962 dst.reason = new ArrayList<CodeableConcept>(); 2963 for (CodeableConcept i : reason) 2964 dst.reason.add(i.copy()); 2965 } 2966 ; 2967 if (service != null) { 2968 dst.service = new ArrayList<Reference>(); 2969 for (Reference i : service) 2970 dst.service.add(i.copy()); 2971 } 2972 ; 2973 dst.product = product == null ? null : product.copy(); 2974 if (account != null) { 2975 dst.account = new ArrayList<Reference>(); 2976 for (Reference i : account) 2977 dst.account.add(i.copy()); 2978 } 2979 ; 2980 if (note != null) { 2981 dst.note = new ArrayList<Annotation>(); 2982 for (Annotation i : note) 2983 dst.note.add(i.copy()); 2984 } 2985 ; 2986 if (supportingInformation != null) { 2987 dst.supportingInformation = new ArrayList<Reference>(); 2988 for (Reference i : supportingInformation) 2989 dst.supportingInformation.add(i.copy()); 2990 } 2991 ; 2992 } 2993 2994 protected ChargeItem typedCopy() { 2995 return copy(); 2996 } 2997 2998 @Override 2999 public boolean equalsDeep(Base other_) { 3000 if (!super.equalsDeep(other_)) 3001 return false; 3002 if (!(other_ instanceof ChargeItem)) 3003 return false; 3004 ChargeItem o = (ChargeItem) other_; 3005 return compareDeep(identifier, o.identifier, true) && compareDeep(definitionUri, o.definitionUri, true) 3006 && compareDeep(definitionCanonical, o.definitionCanonical, true) && compareDeep(status, o.status, true) 3007 && compareDeep(partOf, o.partOf, true) && compareDeep(code, o.code, true) 3008 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 3009 && compareDeep(occurrence, o.occurrence, true) && compareDeep(performer, o.performer, true) 3010 && compareDeep(performingOrganization, o.performingOrganization, true) 3011 && compareDeep(requestingOrganization, o.requestingOrganization, true) 3012 && compareDeep(costCenter, o.costCenter, true) && compareDeep(quantity, o.quantity, true) 3013 && compareDeep(bodysite, o.bodysite, true) && compareDeep(factorOverride, o.factorOverride, true) 3014 && compareDeep(priceOverride, o.priceOverride, true) && compareDeep(overrideReason, o.overrideReason, true) 3015 && compareDeep(enterer, o.enterer, true) && compareDeep(enteredDate, o.enteredDate, true) 3016 && compareDeep(reason, o.reason, true) && compareDeep(service, o.service, true) 3017 && compareDeep(product, o.product, true) && compareDeep(account, o.account, true) 3018 && compareDeep(note, o.note, true) && compareDeep(supportingInformation, o.supportingInformation, true); 3019 } 3020 3021 @Override 3022 public boolean equalsShallow(Base other_) { 3023 if (!super.equalsShallow(other_)) 3024 return false; 3025 if (!(other_ instanceof ChargeItem)) 3026 return false; 3027 ChargeItem o = (ChargeItem) other_; 3028 return compareValues(definitionUri, o.definitionUri, true) && compareValues(status, o.status, true) 3029 && compareValues(factorOverride, o.factorOverride, true) 3030 && compareValues(overrideReason, o.overrideReason, true) && compareValues(enteredDate, o.enteredDate, true); 3031 } 3032 3033 public boolean isEmpty() { 3034 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definitionUri, definitionCanonical, 3035 status, partOf, code, subject, context, occurrence, performer, performingOrganization, requestingOrganization, 3036 costCenter, quantity, bodysite, factorOverride, priceOverride, overrideReason, enterer, enteredDate, reason, 3037 service, product, account, note, supportingInformation); 3038 } 3039 3040 @Override 3041 public ResourceType getResourceType() { 3042 return ResourceType.ChargeItem; 3043 } 3044 3045 /** 3046 * Search parameter: <b>identifier</b> 3047 * <p> 3048 * Description: <b>Business Identifier for item</b><br> 3049 * Type: <b>token</b><br> 3050 * Path: <b>ChargeItem.identifier</b><br> 3051 * </p> 3052 */ 3053 @SearchParamDefinition(name = "identifier", path = "ChargeItem.identifier", description = "Business Identifier for item", type = "token") 3054 public static final String SP_IDENTIFIER = "identifier"; 3055 /** 3056 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3057 * <p> 3058 * Description: <b>Business Identifier for item</b><br> 3059 * Type: <b>token</b><br> 3060 * Path: <b>ChargeItem.identifier</b><br> 3061 * </p> 3062 */ 3063 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3064 SP_IDENTIFIER); 3065 3066 /** 3067 * Search parameter: <b>performing-organization</b> 3068 * <p> 3069 * Description: <b>Organization providing the charged service</b><br> 3070 * Type: <b>reference</b><br> 3071 * Path: <b>ChargeItem.performingOrganization</b><br> 3072 * </p> 3073 */ 3074 @SearchParamDefinition(name = "performing-organization", path = "ChargeItem.performingOrganization", description = "Organization providing the charged service", type = "reference", target = { 3075 Organization.class }) 3076 public static final String SP_PERFORMING_ORGANIZATION = "performing-organization"; 3077 /** 3078 * <b>Fluent Client</b> search parameter constant for 3079 * <b>performing-organization</b> 3080 * <p> 3081 * Description: <b>Organization providing the charged service</b><br> 3082 * Type: <b>reference</b><br> 3083 * Path: <b>ChargeItem.performingOrganization</b><br> 3084 * </p> 3085 */ 3086 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3087 SP_PERFORMING_ORGANIZATION); 3088 3089 /** 3090 * Constant for fluent queries to be used to add include statements. Specifies 3091 * the path value of "<b>ChargeItem:performing-organization</b>". 3092 */ 3093 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMING_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3094 "ChargeItem:performing-organization").toLocked(); 3095 3096 /** 3097 * Search parameter: <b>code</b> 3098 * <p> 3099 * Description: <b>A code that identifies the charge, like a billing 3100 * code</b><br> 3101 * Type: <b>token</b><br> 3102 * Path: <b>ChargeItem.code</b><br> 3103 * </p> 3104 */ 3105 @SearchParamDefinition(name = "code", path = "ChargeItem.code", description = "A code that identifies the charge, like a billing code", type = "token") 3106 public static final String SP_CODE = "code"; 3107 /** 3108 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3109 * <p> 3110 * Description: <b>A code that identifies the charge, like a billing 3111 * code</b><br> 3112 * Type: <b>token</b><br> 3113 * Path: <b>ChargeItem.code</b><br> 3114 * </p> 3115 */ 3116 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3117 SP_CODE); 3118 3119 /** 3120 * Search parameter: <b>quantity</b> 3121 * <p> 3122 * Description: <b>Quantity of which the charge item has been serviced</b><br> 3123 * Type: <b>quantity</b><br> 3124 * Path: <b>ChargeItem.quantity</b><br> 3125 * </p> 3126 */ 3127 @SearchParamDefinition(name = "quantity", path = "ChargeItem.quantity", description = "Quantity of which the charge item has been serviced", type = "quantity") 3128 public static final String SP_QUANTITY = "quantity"; 3129 /** 3130 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 3131 * <p> 3132 * Description: <b>Quantity of which the charge item has been serviced</b><br> 3133 * Type: <b>quantity</b><br> 3134 * Path: <b>ChargeItem.quantity</b><br> 3135 * </p> 3136 */ 3137 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3138 SP_QUANTITY); 3139 3140 /** 3141 * Search parameter: <b>subject</b> 3142 * <p> 3143 * Description: <b>Individual service was done for/to</b><br> 3144 * Type: <b>reference</b><br> 3145 * Path: <b>ChargeItem.subject</b><br> 3146 * </p> 3147 */ 3148 @SearchParamDefinition(name = "subject", path = "ChargeItem.subject", description = "Individual service was done for/to", type = "reference", providesMembershipIn = { 3149 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3150 public static final String SP_SUBJECT = "subject"; 3151 /** 3152 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3153 * <p> 3154 * Description: <b>Individual service was done for/to</b><br> 3155 * Type: <b>reference</b><br> 3156 * Path: <b>ChargeItem.subject</b><br> 3157 * </p> 3158 */ 3159 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3160 SP_SUBJECT); 3161 3162 /** 3163 * Constant for fluent queries to be used to add include statements. Specifies 3164 * the path value of "<b>ChargeItem:subject</b>". 3165 */ 3166 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3167 "ChargeItem:subject").toLocked(); 3168 3169 /** 3170 * Search parameter: <b>occurrence</b> 3171 * <p> 3172 * Description: <b>When the charged service was applied</b><br> 3173 * Type: <b>date</b><br> 3174 * Path: <b>ChargeItem.occurrence[x]</b><br> 3175 * </p> 3176 */ 3177 @SearchParamDefinition(name = "occurrence", path = "ChargeItem.occurrence", description = "When the charged service was applied", type = "date") 3178 public static final String SP_OCCURRENCE = "occurrence"; 3179 /** 3180 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3181 * <p> 3182 * Description: <b>When the charged service was applied</b><br> 3183 * Type: <b>date</b><br> 3184 * Path: <b>ChargeItem.occurrence[x]</b><br> 3185 * </p> 3186 */ 3187 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3188 SP_OCCURRENCE); 3189 3190 /** 3191 * Search parameter: <b>entered-date</b> 3192 * <p> 3193 * Description: <b>Date the charge item was entered</b><br> 3194 * Type: <b>date</b><br> 3195 * Path: <b>ChargeItem.enteredDate</b><br> 3196 * </p> 3197 */ 3198 @SearchParamDefinition(name = "entered-date", path = "ChargeItem.enteredDate", description = "Date the charge item was entered", type = "date") 3199 public static final String SP_ENTERED_DATE = "entered-date"; 3200 /** 3201 * <b>Fluent Client</b> search parameter constant for <b>entered-date</b> 3202 * <p> 3203 * Description: <b>Date the charge item was entered</b><br> 3204 * Type: <b>date</b><br> 3205 * Path: <b>ChargeItem.enteredDate</b><br> 3206 * </p> 3207 */ 3208 public static final ca.uhn.fhir.rest.gclient.DateClientParam ENTERED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3209 SP_ENTERED_DATE); 3210 3211 /** 3212 * Search parameter: <b>performer-function</b> 3213 * <p> 3214 * Description: <b>What type of performance was done</b><br> 3215 * Type: <b>token</b><br> 3216 * Path: <b>ChargeItem.performer.function</b><br> 3217 * </p> 3218 */ 3219 @SearchParamDefinition(name = "performer-function", path = "ChargeItem.performer.function", description = "What type of performance was done", type = "token") 3220 public static final String SP_PERFORMER_FUNCTION = "performer-function"; 3221 /** 3222 * <b>Fluent Client</b> search parameter constant for <b>performer-function</b> 3223 * <p> 3224 * Description: <b>What type of performance was done</b><br> 3225 * Type: <b>token</b><br> 3226 * Path: <b>ChargeItem.performer.function</b><br> 3227 * </p> 3228 */ 3229 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_FUNCTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3230 SP_PERFORMER_FUNCTION); 3231 3232 /** 3233 * Search parameter: <b>patient</b> 3234 * <p> 3235 * Description: <b>Individual service was done for/to</b><br> 3236 * Type: <b>reference</b><br> 3237 * Path: <b>ChargeItem.subject</b><br> 3238 * </p> 3239 */ 3240 @SearchParamDefinition(name = "patient", path = "ChargeItem.subject.where(resolve() is Patient)", description = "Individual service was done for/to", type = "reference", target = { 3241 Patient.class }) 3242 public static final String SP_PATIENT = "patient"; 3243 /** 3244 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3245 * <p> 3246 * Description: <b>Individual service was done for/to</b><br> 3247 * Type: <b>reference</b><br> 3248 * Path: <b>ChargeItem.subject</b><br> 3249 * </p> 3250 */ 3251 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3252 SP_PATIENT); 3253 3254 /** 3255 * Constant for fluent queries to be used to add include statements. Specifies 3256 * the path value of "<b>ChargeItem:patient</b>". 3257 */ 3258 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3259 "ChargeItem:patient").toLocked(); 3260 3261 /** 3262 * Search parameter: <b>factor-override</b> 3263 * <p> 3264 * Description: <b>Factor overriding the associated rules</b><br> 3265 * Type: <b>number</b><br> 3266 * Path: <b>ChargeItem.factorOverride</b><br> 3267 * </p> 3268 */ 3269 @SearchParamDefinition(name = "factor-override", path = "ChargeItem.factorOverride", description = "Factor overriding the associated rules", type = "number") 3270 public static final String SP_FACTOR_OVERRIDE = "factor-override"; 3271 /** 3272 * <b>Fluent Client</b> search parameter constant for <b>factor-override</b> 3273 * <p> 3274 * Description: <b>Factor overriding the associated rules</b><br> 3275 * Type: <b>number</b><br> 3276 * Path: <b>ChargeItem.factorOverride</b><br> 3277 * </p> 3278 */ 3279 public static final ca.uhn.fhir.rest.gclient.NumberClientParam FACTOR_OVERRIDE = new ca.uhn.fhir.rest.gclient.NumberClientParam( 3280 SP_FACTOR_OVERRIDE); 3281 3282 /** 3283 * Search parameter: <b>service</b> 3284 * <p> 3285 * Description: <b>Which rendered service is being charged?</b><br> 3286 * Type: <b>reference</b><br> 3287 * Path: <b>ChargeItem.service</b><br> 3288 * </p> 3289 */ 3290 @SearchParamDefinition(name = "service", path = "ChargeItem.service", description = "Which rendered service is being charged?", type = "reference", target = { 3291 DiagnosticReport.class, ImagingStudy.class, Immunization.class, MedicationAdministration.class, 3292 MedicationDispense.class, Observation.class, Procedure.class, SupplyDelivery.class }) 3293 public static final String SP_SERVICE = "service"; 3294 /** 3295 * <b>Fluent Client</b> search parameter constant for <b>service</b> 3296 * <p> 3297 * Description: <b>Which rendered service is being charged?</b><br> 3298 * Type: <b>reference</b><br> 3299 * Path: <b>ChargeItem.service</b><br> 3300 * </p> 3301 */ 3302 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3303 SP_SERVICE); 3304 3305 /** 3306 * Constant for fluent queries to be used to add include statements. Specifies 3307 * the path value of "<b>ChargeItem:service</b>". 3308 */ 3309 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include( 3310 "ChargeItem:service").toLocked(); 3311 3312 /** 3313 * Search parameter: <b>price-override</b> 3314 * <p> 3315 * Description: <b>Price overriding the associated rules</b><br> 3316 * Type: <b>quantity</b><br> 3317 * Path: <b>ChargeItem.priceOverride</b><br> 3318 * </p> 3319 */ 3320 @SearchParamDefinition(name = "price-override", path = "ChargeItem.priceOverride", description = "Price overriding the associated rules", type = "quantity") 3321 public static final String SP_PRICE_OVERRIDE = "price-override"; 3322 /** 3323 * <b>Fluent Client</b> search parameter constant for <b>price-override</b> 3324 * <p> 3325 * Description: <b>Price overriding the associated rules</b><br> 3326 * Type: <b>quantity</b><br> 3327 * Path: <b>ChargeItem.priceOverride</b><br> 3328 * </p> 3329 */ 3330 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PRICE_OVERRIDE = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3331 SP_PRICE_OVERRIDE); 3332 3333 /** 3334 * Search parameter: <b>context</b> 3335 * <p> 3336 * Description: <b>Encounter / Episode associated with event</b><br> 3337 * Type: <b>reference</b><br> 3338 * Path: <b>ChargeItem.context</b><br> 3339 * </p> 3340 */ 3341 @SearchParamDefinition(name = "context", path = "ChargeItem.context", description = "Encounter / Episode associated with event", type = "reference", providesMembershipIn = { 3342 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3343 EpisodeOfCare.class }) 3344 public static final String SP_CONTEXT = "context"; 3345 /** 3346 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3347 * <p> 3348 * Description: <b>Encounter / Episode associated with event</b><br> 3349 * Type: <b>reference</b><br> 3350 * Path: <b>ChargeItem.context</b><br> 3351 * </p> 3352 */ 3353 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3354 SP_CONTEXT); 3355 3356 /** 3357 * Constant for fluent queries to be used to add include statements. Specifies 3358 * the path value of "<b>ChargeItem:context</b>". 3359 */ 3360 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 3361 "ChargeItem:context").toLocked(); 3362 3363 /** 3364 * Search parameter: <b>enterer</b> 3365 * <p> 3366 * Description: <b>Individual who was entering</b><br> 3367 * Type: <b>reference</b><br> 3368 * Path: <b>ChargeItem.enterer</b><br> 3369 * </p> 3370 */ 3371 @SearchParamDefinition(name = "enterer", path = "ChargeItem.enterer", description = "Individual who was entering", type = "reference", providesMembershipIn = { 3372 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3373 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3374 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3375 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3376 public static final String SP_ENTERER = "enterer"; 3377 /** 3378 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 3379 * <p> 3380 * Description: <b>Individual who was entering</b><br> 3381 * Type: <b>reference</b><br> 3382 * Path: <b>ChargeItem.enterer</b><br> 3383 * </p> 3384 */ 3385 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3386 SP_ENTERER); 3387 3388 /** 3389 * Constant for fluent queries to be used to add include statements. Specifies 3390 * the path value of "<b>ChargeItem:enterer</b>". 3391 */ 3392 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include( 3393 "ChargeItem:enterer").toLocked(); 3394 3395 /** 3396 * Search parameter: <b>performer-actor</b> 3397 * <p> 3398 * Description: <b>Individual who was performing</b><br> 3399 * Type: <b>reference</b><br> 3400 * Path: <b>ChargeItem.performer.actor</b><br> 3401 * </p> 3402 */ 3403 @SearchParamDefinition(name = "performer-actor", path = "ChargeItem.performer.actor", description = "Individual who was performing", type = "reference", providesMembershipIn = { 3404 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3405 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3406 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3407 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3408 public static final String SP_PERFORMER_ACTOR = "performer-actor"; 3409 /** 3410 * <b>Fluent Client</b> search parameter constant for <b>performer-actor</b> 3411 * <p> 3412 * Description: <b>Individual who was performing</b><br> 3413 * Type: <b>reference</b><br> 3414 * Path: <b>ChargeItem.performer.actor</b><br> 3415 * </p> 3416 */ 3417 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER_ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3418 SP_PERFORMER_ACTOR); 3419 3420 /** 3421 * Constant for fluent queries to be used to add include statements. Specifies 3422 * the path value of "<b>ChargeItem:performer-actor</b>". 3423 */ 3424 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER_ACTOR = new ca.uhn.fhir.model.api.Include( 3425 "ChargeItem:performer-actor").toLocked(); 3426 3427 /** 3428 * Search parameter: <b>account</b> 3429 * <p> 3430 * Description: <b>Account to place this charge</b><br> 3431 * Type: <b>reference</b><br> 3432 * Path: <b>ChargeItem.account</b><br> 3433 * </p> 3434 */ 3435 @SearchParamDefinition(name = "account", path = "ChargeItem.account", description = "Account to place this charge", type = "reference", target = { 3436 Account.class }) 3437 public static final String SP_ACCOUNT = "account"; 3438 /** 3439 * <b>Fluent Client</b> search parameter constant for <b>account</b> 3440 * <p> 3441 * Description: <b>Account to place this charge</b><br> 3442 * Type: <b>reference</b><br> 3443 * Path: <b>ChargeItem.account</b><br> 3444 * </p> 3445 */ 3446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3447 SP_ACCOUNT); 3448 3449 /** 3450 * Constant for fluent queries to be used to add include statements. Specifies 3451 * the path value of "<b>ChargeItem:account</b>". 3452 */ 3453 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include( 3454 "ChargeItem:account").toLocked(); 3455 3456 /** 3457 * Search parameter: <b>requesting-organization</b> 3458 * <p> 3459 * Description: <b>Organization requesting the charged service</b><br> 3460 * Type: <b>reference</b><br> 3461 * Path: <b>ChargeItem.requestingOrganization</b><br> 3462 * </p> 3463 */ 3464 @SearchParamDefinition(name = "requesting-organization", path = "ChargeItem.requestingOrganization", description = "Organization requesting the charged service", type = "reference", target = { 3465 Organization.class }) 3466 public static final String SP_REQUESTING_ORGANIZATION = "requesting-organization"; 3467 /** 3468 * <b>Fluent Client</b> search parameter constant for 3469 * <b>requesting-organization</b> 3470 * <p> 3471 * Description: <b>Organization requesting the charged service</b><br> 3472 * Type: <b>reference</b><br> 3473 * Path: <b>ChargeItem.requestingOrganization</b><br> 3474 * </p> 3475 */ 3476 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTING_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3477 SP_REQUESTING_ORGANIZATION); 3478 3479 /** 3480 * Constant for fluent queries to be used to add include statements. Specifies 3481 * the path value of "<b>ChargeItem:requesting-organization</b>". 3482 */ 3483 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTING_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3484 "ChargeItem:requesting-organization").toLocked(); 3485 3486}