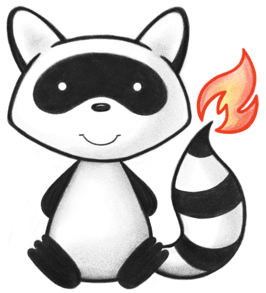
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The ChargeItemDefinition resource provides the properties that apply to the 054 * (billing) codes necessary to calculate costs and prices. The properties may 055 * differ largely depending on type and realm, therefore this resource gives 056 * only a rough structure and requires profiling for each type of billing code 057 * system. 058 */ 059@ResourceDef(name = "ChargeItemDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ChargeItemDefinition") 060@ChildOrder(names = { "url", "identifier", "version", "title", "derivedFromUri", "partOf", "replaces", "status", 061 "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "copyright", 062 "approvalDate", "lastReviewDate", "effectivePeriod", "code", "instance", "applicability", "propertyGroup" }) 063public class ChargeItemDefinition extends MetadataResource { 064 065 public enum ChargeItemDefinitionPriceComponentType { 066 /** 067 * the amount is the base price used for calculating the total price before 068 * applying surcharges, discount or taxes. 069 */ 070 BASE, 071 /** 072 * the amount is a surcharge applied on the base price. 073 */ 074 SURCHARGE, 075 /** 076 * the amount is a deduction applied on the base price. 077 */ 078 DEDUCTION, 079 /** 080 * the amount is a discount applied on the base price. 081 */ 082 DISCOUNT, 083 /** 084 * the amount is the tax component of the total price. 085 */ 086 TAX, 087 /** 088 * the amount is of informational character, it has not been applied in the 089 * calculation of the total price. 090 */ 091 INFORMATIONAL, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 097 public static ChargeItemDefinitionPriceComponentType fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("base".equals(codeString)) 101 return BASE; 102 if ("surcharge".equals(codeString)) 103 return SURCHARGE; 104 if ("deduction".equals(codeString)) 105 return DEDUCTION; 106 if ("discount".equals(codeString)) 107 return DISCOUNT; 108 if ("tax".equals(codeString)) 109 return TAX; 110 if ("informational".equals(codeString)) 111 return INFORMATIONAL; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case BASE: 121 return "base"; 122 case SURCHARGE: 123 return "surcharge"; 124 case DEDUCTION: 125 return "deduction"; 126 case DISCOUNT: 127 return "discount"; 128 case TAX: 129 return "tax"; 130 case INFORMATIONAL: 131 return "informational"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getSystem() { 140 switch (this) { 141 case BASE: 142 return "http://hl7.org/fhir/invoice-priceComponentType"; 143 case SURCHARGE: 144 return "http://hl7.org/fhir/invoice-priceComponentType"; 145 case DEDUCTION: 146 return "http://hl7.org/fhir/invoice-priceComponentType"; 147 case DISCOUNT: 148 return "http://hl7.org/fhir/invoice-priceComponentType"; 149 case TAX: 150 return "http://hl7.org/fhir/invoice-priceComponentType"; 151 case INFORMATIONAL: 152 return "http://hl7.org/fhir/invoice-priceComponentType"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDefinition() { 161 switch (this) { 162 case BASE: 163 return "the amount is the base price used for calculating the total price before applying surcharges, discount or taxes."; 164 case SURCHARGE: 165 return "the amount is a surcharge applied on the base price."; 166 case DEDUCTION: 167 return "the amount is a deduction applied on the base price."; 168 case DISCOUNT: 169 return "the amount is a discount applied on the base price."; 170 case TAX: 171 return "the amount is the tax component of the total price."; 172 case INFORMATIONAL: 173 return "the amount is of informational character, it has not been applied in the calculation of the total price."; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case BASE: 184 return "base price"; 185 case SURCHARGE: 186 return "surcharge"; 187 case DEDUCTION: 188 return "deduction"; 189 case DISCOUNT: 190 return "discount"; 191 case TAX: 192 return "tax"; 193 case INFORMATIONAL: 194 return "informational"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 } 202 203 public static class ChargeItemDefinitionPriceComponentTypeEnumFactory 204 implements EnumFactory<ChargeItemDefinitionPriceComponentType> { 205 public ChargeItemDefinitionPriceComponentType fromCode(String codeString) throws IllegalArgumentException { 206 if (codeString == null || "".equals(codeString)) 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("base".equals(codeString)) 210 return ChargeItemDefinitionPriceComponentType.BASE; 211 if ("surcharge".equals(codeString)) 212 return ChargeItemDefinitionPriceComponentType.SURCHARGE; 213 if ("deduction".equals(codeString)) 214 return ChargeItemDefinitionPriceComponentType.DEDUCTION; 215 if ("discount".equals(codeString)) 216 return ChargeItemDefinitionPriceComponentType.DISCOUNT; 217 if ("tax".equals(codeString)) 218 return ChargeItemDefinitionPriceComponentType.TAX; 219 if ("informational".equals(codeString)) 220 return ChargeItemDefinitionPriceComponentType.INFORMATIONAL; 221 throw new IllegalArgumentException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 222 } 223 224 public Enumeration<ChargeItemDefinitionPriceComponentType> fromType(PrimitiveType<?> code) throws FHIRException { 225 if (code == null) 226 return null; 227 if (code.isEmpty()) 228 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 229 ChargeItemDefinitionPriceComponentType.NULL, code); 230 String codeString = code.asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 233 ChargeItemDefinitionPriceComponentType.NULL, code); 234 if ("base".equals(codeString)) 235 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 236 ChargeItemDefinitionPriceComponentType.BASE, code); 237 if ("surcharge".equals(codeString)) 238 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 239 ChargeItemDefinitionPriceComponentType.SURCHARGE, code); 240 if ("deduction".equals(codeString)) 241 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 242 ChargeItemDefinitionPriceComponentType.DEDUCTION, code); 243 if ("discount".equals(codeString)) 244 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 245 ChargeItemDefinitionPriceComponentType.DISCOUNT, code); 246 if ("tax".equals(codeString)) 247 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, ChargeItemDefinitionPriceComponentType.TAX, 248 code); 249 if ("informational".equals(codeString)) 250 return new Enumeration<ChargeItemDefinitionPriceComponentType>(this, 251 ChargeItemDefinitionPriceComponentType.INFORMATIONAL, code); 252 throw new FHIRException("Unknown ChargeItemDefinitionPriceComponentType code '" + codeString + "'"); 253 } 254 255 public String toCode(ChargeItemDefinitionPriceComponentType code) { 256 if (code == ChargeItemDefinitionPriceComponentType.NULL) 257 return null; 258 if (code == ChargeItemDefinitionPriceComponentType.BASE) 259 return "base"; 260 if (code == ChargeItemDefinitionPriceComponentType.SURCHARGE) 261 return "surcharge"; 262 if (code == ChargeItemDefinitionPriceComponentType.DEDUCTION) 263 return "deduction"; 264 if (code == ChargeItemDefinitionPriceComponentType.DISCOUNT) 265 return "discount"; 266 if (code == ChargeItemDefinitionPriceComponentType.TAX) 267 return "tax"; 268 if (code == ChargeItemDefinitionPriceComponentType.INFORMATIONAL) 269 return "informational"; 270 return "?"; 271 } 272 273 public String toSystem(ChargeItemDefinitionPriceComponentType code) { 274 return code.getSystem(); 275 } 276 } 277 278 @Block() 279 public static class ChargeItemDefinitionApplicabilityComponent extends BackboneElement 280 implements IBaseBackboneElement { 281 /** 282 * A brief, natural language description of the condition that effectively 283 * communicates the intended semantics. 284 */ 285 @Child(name = "description", type = { 286 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 287 @Description(shortDefinition = "Natural language description of the condition", formalDefinition = "A brief, natural language description of the condition that effectively communicates the intended semantics.") 288 protected StringType description; 289 290 /** 291 * The media type of the language for the expression, e.g. "text/cql" for 292 * Clinical Query Language expressions or "text/fhirpath" for FHIRPath 293 * expressions. 294 */ 295 @Child(name = "language", type = { 296 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 297 @Description(shortDefinition = "Language of the expression", formalDefinition = "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.") 298 protected StringType language; 299 300 /** 301 * An expression that returns true or false, indicating whether the condition is 302 * satisfied. When using FHIRPath expressions, the %context environment variable 303 * must be replaced at runtime with the ChargeItem resource to which this 304 * definition is applied. 305 */ 306 @Child(name = "expression", type = { 307 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 308 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.") 309 protected StringType expression; 310 311 private static final long serialVersionUID = 1354288281L; 312 313 /** 314 * Constructor 315 */ 316 public ChargeItemDefinitionApplicabilityComponent() { 317 super(); 318 } 319 320 /** 321 * @return {@link #description} (A brief, natural language description of the 322 * condition that effectively communicates the intended semantics.). 323 * This is the underlying object with id, value and extensions. The 324 * accessor "getDescription" gives direct access to the value 325 */ 326 public StringType getDescriptionElement() { 327 if (this.description == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.description"); 330 else if (Configuration.doAutoCreate()) 331 this.description = new StringType(); // bb 332 return this.description; 333 } 334 335 public boolean hasDescriptionElement() { 336 return this.description != null && !this.description.isEmpty(); 337 } 338 339 public boolean hasDescription() { 340 return this.description != null && !this.description.isEmpty(); 341 } 342 343 /** 344 * @param value {@link #description} (A brief, natural language description of 345 * the condition that effectively communicates the intended 346 * semantics.). This is the underlying object with id, value and 347 * extensions. The accessor "getDescription" gives direct access to 348 * the value 349 */ 350 public ChargeItemDefinitionApplicabilityComponent setDescriptionElement(StringType value) { 351 this.description = value; 352 return this; 353 } 354 355 /** 356 * @return A brief, natural language description of the condition that 357 * effectively communicates the intended semantics. 358 */ 359 public String getDescription() { 360 return this.description == null ? null : this.description.getValue(); 361 } 362 363 /** 364 * @param value A brief, natural language description of the condition that 365 * effectively communicates the intended semantics. 366 */ 367 public ChargeItemDefinitionApplicabilityComponent setDescription(String value) { 368 if (Utilities.noString(value)) 369 this.description = null; 370 else { 371 if (this.description == null) 372 this.description = new StringType(); 373 this.description.setValue(value); 374 } 375 return this; 376 } 377 378 /** 379 * @return {@link #language} (The media type of the language for the expression, 380 * e.g. "text/cql" for Clinical Query Language expressions or 381 * "text/fhirpath" for FHIRPath expressions.). This is the underlying 382 * object with id, value and extensions. The accessor "getLanguage" 383 * gives direct access to the value 384 */ 385 public StringType getLanguageElement() { 386 if (this.language == null) 387 if (Configuration.errorOnAutoCreate()) 388 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.language"); 389 else if (Configuration.doAutoCreate()) 390 this.language = new StringType(); // bb 391 return this.language; 392 } 393 394 public boolean hasLanguageElement() { 395 return this.language != null && !this.language.isEmpty(); 396 } 397 398 public boolean hasLanguage() { 399 return this.language != null && !this.language.isEmpty(); 400 } 401 402 /** 403 * @param value {@link #language} (The media type of the language for the 404 * expression, e.g. "text/cql" for Clinical Query Language 405 * expressions or "text/fhirpath" for FHIRPath expressions.). This 406 * is the underlying object with id, value and extensions. The 407 * accessor "getLanguage" gives direct access to the value 408 */ 409 public ChargeItemDefinitionApplicabilityComponent setLanguageElement(StringType value) { 410 this.language = value; 411 return this; 412 } 413 414 /** 415 * @return The media type of the language for the expression, e.g. "text/cql" 416 * for Clinical Query Language expressions or "text/fhirpath" for 417 * FHIRPath expressions. 418 */ 419 public String getLanguage() { 420 return this.language == null ? null : this.language.getValue(); 421 } 422 423 /** 424 * @param value The media type of the language for the expression, e.g. 425 * "text/cql" for Clinical Query Language expressions or 426 * "text/fhirpath" for FHIRPath expressions. 427 */ 428 public ChargeItemDefinitionApplicabilityComponent setLanguage(String value) { 429 if (Utilities.noString(value)) 430 this.language = null; 431 else { 432 if (this.language == null) 433 this.language = new StringType(); 434 this.language.setValue(value); 435 } 436 return this; 437 } 438 439 /** 440 * @return {@link #expression} (An expression that returns true or false, 441 * indicating whether the condition is satisfied. When using FHIRPath 442 * expressions, the %context environment variable must be replaced at 443 * runtime with the ChargeItem resource to which this definition is 444 * applied.). This is the underlying object with id, value and 445 * extensions. The accessor "getExpression" gives direct access to the 446 * value 447 */ 448 public StringType getExpressionElement() { 449 if (this.expression == null) 450 if (Configuration.errorOnAutoCreate()) 451 throw new Error("Attempt to auto-create ChargeItemDefinitionApplicabilityComponent.expression"); 452 else if (Configuration.doAutoCreate()) 453 this.expression = new StringType(); // bb 454 return this.expression; 455 } 456 457 public boolean hasExpressionElement() { 458 return this.expression != null && !this.expression.isEmpty(); 459 } 460 461 public boolean hasExpression() { 462 return this.expression != null && !this.expression.isEmpty(); 463 } 464 465 /** 466 * @param value {@link #expression} (An expression that returns true or false, 467 * indicating whether the condition is satisfied. When using 468 * FHIRPath expressions, the %context environment variable must be 469 * replaced at runtime with the ChargeItem resource to which this 470 * definition is applied.). This is the underlying object with id, 471 * value and extensions. The accessor "getExpression" gives direct 472 * access to the value 473 */ 474 public ChargeItemDefinitionApplicabilityComponent setExpressionElement(StringType value) { 475 this.expression = value; 476 return this; 477 } 478 479 /** 480 * @return An expression that returns true or false, indicating whether the 481 * condition is satisfied. When using FHIRPath expressions, the %context 482 * environment variable must be replaced at runtime with the ChargeItem 483 * resource to which this definition is applied. 484 */ 485 public String getExpression() { 486 return this.expression == null ? null : this.expression.getValue(); 487 } 488 489 /** 490 * @param value An expression that returns true or false, indicating whether the 491 * condition is satisfied. When using FHIRPath expressions, the 492 * %context environment variable must be replaced at runtime with 493 * the ChargeItem resource to which this definition is applied. 494 */ 495 public ChargeItemDefinitionApplicabilityComponent setExpression(String value) { 496 if (Utilities.noString(value)) 497 this.expression = null; 498 else { 499 if (this.expression == null) 500 this.expression = new StringType(); 501 this.expression.setValue(value); 502 } 503 return this; 504 } 505 506 protected void listChildren(List<Property> children) { 507 super.listChildren(children); 508 children.add(new Property("description", "string", 509 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 510 0, 1, description)); 511 children.add(new Property("language", "string", 512 "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.", 513 0, 1, language)); 514 children.add(new Property("expression", "string", 515 "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 516 0, 1, expression)); 517 } 518 519 @Override 520 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 521 switch (_hash) { 522 case -1724546052: 523 /* description */ return new Property("description", "string", 524 "A brief, natural language description of the condition that effectively communicates the intended semantics.", 525 0, 1, description); 526 case -1613589672: 527 /* language */ return new Property("language", "string", 528 "The media type of the language for the expression, e.g. \"text/cql\" for Clinical Query Language expressions or \"text/fhirpath\" for FHIRPath expressions.", 529 0, 1, language); 530 case -1795452264: 531 /* expression */ return new Property("expression", "string", 532 "An expression that returns true or false, indicating whether the condition is satisfied. When using FHIRPath expressions, the %context environment variable must be replaced at runtime with the ChargeItem resource to which this definition is applied.", 533 0, 1, expression); 534 default: 535 return super.getNamedProperty(_hash, _name, _checkValid); 536 } 537 538 } 539 540 @Override 541 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 542 switch (hash) { 543 case -1724546052: 544 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 545 case -1613589672: 546 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // StringType 547 case -1795452264: 548 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 549 default: 550 return super.getProperty(hash, name, checkValid); 551 } 552 553 } 554 555 @Override 556 public Base setProperty(int hash, String name, Base value) throws FHIRException { 557 switch (hash) { 558 case -1724546052: // description 559 this.description = castToString(value); // StringType 560 return value; 561 case -1613589672: // language 562 this.language = castToString(value); // StringType 563 return value; 564 case -1795452264: // expression 565 this.expression = castToString(value); // StringType 566 return value; 567 default: 568 return super.setProperty(hash, name, value); 569 } 570 571 } 572 573 @Override 574 public Base setProperty(String name, Base value) throws FHIRException { 575 if (name.equals("description")) { 576 this.description = castToString(value); // StringType 577 } else if (name.equals("language")) { 578 this.language = castToString(value); // StringType 579 } else if (name.equals("expression")) { 580 this.expression = castToString(value); // StringType 581 } else 582 return super.setProperty(name, value); 583 return value; 584 } 585 586 @Override 587 public void removeChild(String name, Base value) throws FHIRException { 588 if (name.equals("description")) { 589 this.description = null; 590 } else if (name.equals("language")) { 591 this.language = null; 592 } else if (name.equals("expression")) { 593 this.expression = null; 594 } else 595 super.removeChild(name, value); 596 597 } 598 599 @Override 600 public Base makeProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case -1724546052: 603 return getDescriptionElement(); 604 case -1613589672: 605 return getLanguageElement(); 606 case -1795452264: 607 return getExpressionElement(); 608 default: 609 return super.makeProperty(hash, name); 610 } 611 612 } 613 614 @Override 615 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 616 switch (hash) { 617 case -1724546052: 618 /* description */ return new String[] { "string" }; 619 case -1613589672: 620 /* language */ return new String[] { "string" }; 621 case -1795452264: 622 /* expression */ return new String[] { "string" }; 623 default: 624 return super.getTypesForProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public Base addChild(String name) throws FHIRException { 631 if (name.equals("description")) { 632 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 633 } else if (name.equals("language")) { 634 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.language"); 635 } else if (name.equals("expression")) { 636 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.expression"); 637 } else 638 return super.addChild(name); 639 } 640 641 public ChargeItemDefinitionApplicabilityComponent copy() { 642 ChargeItemDefinitionApplicabilityComponent dst = new ChargeItemDefinitionApplicabilityComponent(); 643 copyValues(dst); 644 return dst; 645 } 646 647 public void copyValues(ChargeItemDefinitionApplicabilityComponent dst) { 648 super.copyValues(dst); 649 dst.description = description == null ? null : description.copy(); 650 dst.language = language == null ? null : language.copy(); 651 dst.expression = expression == null ? null : expression.copy(); 652 } 653 654 @Override 655 public boolean equalsDeep(Base other_) { 656 if (!super.equalsDeep(other_)) 657 return false; 658 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 659 return false; 660 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 661 return compareDeep(description, o.description, true) && compareDeep(language, o.language, true) 662 && compareDeep(expression, o.expression, true); 663 } 664 665 @Override 666 public boolean equalsShallow(Base other_) { 667 if (!super.equalsShallow(other_)) 668 return false; 669 if (!(other_ instanceof ChargeItemDefinitionApplicabilityComponent)) 670 return false; 671 ChargeItemDefinitionApplicabilityComponent o = (ChargeItemDefinitionApplicabilityComponent) other_; 672 return compareValues(description, o.description, true) && compareValues(language, o.language, true) 673 && compareValues(expression, o.expression, true); 674 } 675 676 public boolean isEmpty() { 677 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, language, expression); 678 } 679 680 public String fhirType() { 681 return "ChargeItemDefinition.applicability"; 682 683 } 684 685 } 686 687 @Block() 688 public static class ChargeItemDefinitionPropertyGroupComponent extends BackboneElement 689 implements IBaseBackboneElement { 690 /** 691 * Expressions that describe applicability criteria for the priceComponent. 692 */ 693 @Child(name = "applicability", type = { 694 ChargeItemDefinitionApplicabilityComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 695 @Description(shortDefinition = "Conditions under which the priceComponent is applicable", formalDefinition = "Expressions that describe applicability criteria for the priceComponent.") 696 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 697 698 /** 699 * The price for a ChargeItem may be calculated as a base price with 700 * surcharges/deductions that apply in certain conditions. A 701 * ChargeItemDefinition resource that defines the prices, factors and conditions 702 * that apply to a billing code is currently under development. The 703 * priceComponent element can be used to offer transparency to the recipient of 704 * the Invoice of how the prices have been calculated. 705 */ 706 @Child(name = "priceComponent", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 707 @Description(shortDefinition = "Components of total line item price", formalDefinition = "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.") 708 protected List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> priceComponent; 709 710 private static final long serialVersionUID = 1723436176L; 711 712 /** 713 * Constructor 714 */ 715 public ChargeItemDefinitionPropertyGroupComponent() { 716 super(); 717 } 718 719 /** 720 * @return {@link #applicability} (Expressions that describe applicability 721 * criteria for the priceComponent.) 722 */ 723 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 724 if (this.applicability == null) 725 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 726 return this.applicability; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public ChargeItemDefinitionPropertyGroupComponent setApplicability( 733 List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 734 this.applicability = theApplicability; 735 return this; 736 } 737 738 public boolean hasApplicability() { 739 if (this.applicability == null) 740 return false; 741 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 742 if (!item.isEmpty()) 743 return true; 744 return false; 745 } 746 747 public ChargeItemDefinitionApplicabilityComponent addApplicability() { // 3 748 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 749 if (this.applicability == null) 750 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 751 this.applicability.add(t); 752 return t; 753 } 754 755 public ChargeItemDefinitionPropertyGroupComponent addApplicability(ChargeItemDefinitionApplicabilityComponent t) { // 3 756 if (t == null) 757 return this; 758 if (this.applicability == null) 759 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 760 this.applicability.add(t); 761 return this; 762 } 763 764 /** 765 * @return The first repetition of repeating field {@link #applicability}, 766 * creating it if it does not already exist 767 */ 768 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 769 if (getApplicability().isEmpty()) { 770 addApplicability(); 771 } 772 return getApplicability().get(0); 773 } 774 775 /** 776 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated 777 * as a base price with surcharges/deductions that apply in certain 778 * conditions. A ChargeItemDefinition resource that defines the prices, 779 * factors and conditions that apply to a billing code is currently 780 * under development. The priceComponent element can be used to offer 781 * transparency to the recipient of the Invoice of how the prices have 782 * been calculated.) 783 */ 784 public List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> getPriceComponent() { 785 if (this.priceComponent == null) 786 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 787 return this.priceComponent; 788 } 789 790 /** 791 * @return Returns a reference to <code>this</code> for easy method chaining 792 */ 793 public ChargeItemDefinitionPropertyGroupComponent setPriceComponent( 794 List<ChargeItemDefinitionPropertyGroupPriceComponentComponent> thePriceComponent) { 795 this.priceComponent = thePriceComponent; 796 return this; 797 } 798 799 public boolean hasPriceComponent() { 800 if (this.priceComponent == null) 801 return false; 802 for (ChargeItemDefinitionPropertyGroupPriceComponentComponent item : this.priceComponent) 803 if (!item.isEmpty()) 804 return true; 805 return false; 806 } 807 808 public ChargeItemDefinitionPropertyGroupPriceComponentComponent addPriceComponent() { // 3 809 ChargeItemDefinitionPropertyGroupPriceComponentComponent t = new ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 810 if (this.priceComponent == null) 811 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 812 this.priceComponent.add(t); 813 return t; 814 } 815 816 public ChargeItemDefinitionPropertyGroupComponent addPriceComponent( 817 ChargeItemDefinitionPropertyGroupPriceComponentComponent t) { // 3 818 if (t == null) 819 return this; 820 if (this.priceComponent == null) 821 this.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 822 this.priceComponent.add(t); 823 return this; 824 } 825 826 /** 827 * @return The first repetition of repeating field {@link #priceComponent}, 828 * creating it if it does not already exist 829 */ 830 public ChargeItemDefinitionPropertyGroupPriceComponentComponent getPriceComponentFirstRep() { 831 if (getPriceComponent().isEmpty()) { 832 addPriceComponent(); 833 } 834 return getPriceComponent().get(0); 835 } 836 837 protected void listChildren(List<Property> children) { 838 super.listChildren(children); 839 children.add(new Property("applicability", "@ChargeItemDefinition.applicability", 840 "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, 841 applicability)); 842 children.add(new Property("priceComponent", "", 843 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 844 0, java.lang.Integer.MAX_VALUE, priceComponent)); 845 } 846 847 @Override 848 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 849 switch (_hash) { 850 case -1526770491: 851 /* applicability */ return new Property("applicability", "@ChargeItemDefinition.applicability", 852 "Expressions that describe applicability criteria for the priceComponent.", 0, java.lang.Integer.MAX_VALUE, 853 applicability); 854 case 1219095988: 855 /* priceComponent */ return new Property("priceComponent", "", 856 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the prices have been calculated.", 857 0, java.lang.Integer.MAX_VALUE, priceComponent); 858 default: 859 return super.getNamedProperty(_hash, _name, _checkValid); 860 } 861 862 } 863 864 @Override 865 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 866 switch (hash) { 867 case -1526770491: 868 /* applicability */ return this.applicability == null ? new Base[0] 869 : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 870 case 1219095988: 871 /* priceComponent */ return this.priceComponent == null ? new Base[0] 872 : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // ChargeItemDefinitionPropertyGroupPriceComponentComponent 873 default: 874 return super.getProperty(hash, name, checkValid); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 881 switch (hash) { 882 case -1526770491: // applicability 883 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 884 return value; 885 case 1219095988: // priceComponent 886 this.getPriceComponent().add((ChargeItemDefinitionPropertyGroupPriceComponentComponent) value); // ChargeItemDefinitionPropertyGroupPriceComponentComponent 887 return value; 888 default: 889 return super.setProperty(hash, name, value); 890 } 891 892 } 893 894 @Override 895 public Base setProperty(String name, Base value) throws FHIRException { 896 if (name.equals("applicability")) { 897 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 898 } else if (name.equals("priceComponent")) { 899 this.getPriceComponent().add((ChargeItemDefinitionPropertyGroupPriceComponentComponent) value); 900 } else 901 return super.setProperty(name, value); 902 return value; 903 } 904 905 @Override 906 public void removeChild(String name, Base value) throws FHIRException { 907 if (name.equals("applicability")) { 908 this.getApplicability().remove((ChargeItemDefinitionApplicabilityComponent) value); 909 } else if (name.equals("priceComponent")) { 910 this.getPriceComponent().remove((ChargeItemDefinitionPropertyGroupPriceComponentComponent) value); 911 } else 912 super.removeChild(name, value); 913 914 } 915 916 @Override 917 public Base makeProperty(int hash, String name) throws FHIRException { 918 switch (hash) { 919 case -1526770491: 920 return addApplicability(); 921 case 1219095988: 922 return addPriceComponent(); 923 default: 924 return super.makeProperty(hash, name); 925 } 926 927 } 928 929 @Override 930 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 931 switch (hash) { 932 case -1526770491: 933 /* applicability */ return new String[] { "@ChargeItemDefinition.applicability" }; 934 case 1219095988: 935 /* priceComponent */ return new String[] {}; 936 default: 937 return super.getTypesForProperty(hash, name); 938 } 939 940 } 941 942 @Override 943 public Base addChild(String name) throws FHIRException { 944 if (name.equals("applicability")) { 945 return addApplicability(); 946 } else if (name.equals("priceComponent")) { 947 return addPriceComponent(); 948 } else 949 return super.addChild(name); 950 } 951 952 public ChargeItemDefinitionPropertyGroupComponent copy() { 953 ChargeItemDefinitionPropertyGroupComponent dst = new ChargeItemDefinitionPropertyGroupComponent(); 954 copyValues(dst); 955 return dst; 956 } 957 958 public void copyValues(ChargeItemDefinitionPropertyGroupComponent dst) { 959 super.copyValues(dst); 960 if (applicability != null) { 961 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 962 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 963 dst.applicability.add(i.copy()); 964 } 965 ; 966 if (priceComponent != null) { 967 dst.priceComponent = new ArrayList<ChargeItemDefinitionPropertyGroupPriceComponentComponent>(); 968 for (ChargeItemDefinitionPropertyGroupPriceComponentComponent i : priceComponent) 969 dst.priceComponent.add(i.copy()); 970 } 971 ; 972 } 973 974 @Override 975 public boolean equalsDeep(Base other_) { 976 if (!super.equalsDeep(other_)) 977 return false; 978 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 979 return false; 980 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 981 return compareDeep(applicability, o.applicability, true) && compareDeep(priceComponent, o.priceComponent, true); 982 } 983 984 @Override 985 public boolean equalsShallow(Base other_) { 986 if (!super.equalsShallow(other_)) 987 return false; 988 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupComponent)) 989 return false; 990 ChargeItemDefinitionPropertyGroupComponent o = (ChargeItemDefinitionPropertyGroupComponent) other_; 991 return true; 992 } 993 994 public boolean isEmpty() { 995 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(applicability, priceComponent); 996 } 997 998 public String fhirType() { 999 return "ChargeItemDefinition.propertyGroup"; 1000 1001 } 1002 1003 } 1004 1005 @Block() 1006 public static class ChargeItemDefinitionPropertyGroupPriceComponentComponent extends BackboneElement 1007 implements IBaseBackboneElement { 1008 /** 1009 * This code identifies the type of the component. 1010 */ 1011 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1012 @Description(shortDefinition = "base | surcharge | deduction | discount | tax | informational", formalDefinition = "This code identifies the type of the component.") 1013 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/invoice-priceComponentType") 1014 protected Enumeration<ChargeItemDefinitionPriceComponentType> type; 1015 1016 /** 1017 * A code that identifies the component. Codes may be used to differentiate 1018 * between kinds of taxes, surcharges, discounts etc. 1019 */ 1020 @Child(name = "code", type = { 1021 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1022 @Description(shortDefinition = "Code identifying the specific component", formalDefinition = "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.") 1023 protected CodeableConcept code; 1024 1025 /** 1026 * The factor that has been applied on the base price for calculating this 1027 * component. 1028 */ 1029 @Child(name = "factor", type = { 1030 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1031 @Description(shortDefinition = "Factor used for calculating this component", formalDefinition = "The factor that has been applied on the base price for calculating this component.") 1032 protected DecimalType factor; 1033 1034 /** 1035 * The amount calculated for this component. 1036 */ 1037 @Child(name = "amount", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1038 @Description(shortDefinition = "Monetary amount associated with this component", formalDefinition = "The amount calculated for this component.") 1039 protected Money amount; 1040 1041 private static final long serialVersionUID = -841451335L; 1042 1043 /** 1044 * Constructor 1045 */ 1046 public ChargeItemDefinitionPropertyGroupPriceComponentComponent() { 1047 super(); 1048 } 1049 1050 /** 1051 * Constructor 1052 */ 1053 public ChargeItemDefinitionPropertyGroupPriceComponentComponent( 1054 Enumeration<ChargeItemDefinitionPriceComponentType> type) { 1055 super(); 1056 this.type = type; 1057 } 1058 1059 /** 1060 * @return {@link #type} (This code identifies the type of the component.). This 1061 * is the underlying object with id, value and extensions. The accessor 1062 * "getType" gives direct access to the value 1063 */ 1064 public Enumeration<ChargeItemDefinitionPriceComponentType> getTypeElement() { 1065 if (this.type == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.type"); 1068 else if (Configuration.doAutoCreate()) 1069 this.type = new Enumeration<ChargeItemDefinitionPriceComponentType>( 1070 new ChargeItemDefinitionPriceComponentTypeEnumFactory()); // bb 1071 return this.type; 1072 } 1073 1074 public boolean hasTypeElement() { 1075 return this.type != null && !this.type.isEmpty(); 1076 } 1077 1078 public boolean hasType() { 1079 return this.type != null && !this.type.isEmpty(); 1080 } 1081 1082 /** 1083 * @param value {@link #type} (This code identifies the type of the component.). 1084 * This is the underlying object with id, value and extensions. The 1085 * accessor "getType" gives direct access to the value 1086 */ 1087 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setTypeElement( 1088 Enumeration<ChargeItemDefinitionPriceComponentType> value) { 1089 this.type = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return This code identifies the type of the component. 1095 */ 1096 public ChargeItemDefinitionPriceComponentType getType() { 1097 return this.type == null ? null : this.type.getValue(); 1098 } 1099 1100 /** 1101 * @param value This code identifies the type of the component. 1102 */ 1103 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setType( 1104 ChargeItemDefinitionPriceComponentType value) { 1105 if (this.type == null) 1106 this.type = new Enumeration<ChargeItemDefinitionPriceComponentType>( 1107 new ChargeItemDefinitionPriceComponentTypeEnumFactory()); 1108 this.type.setValue(value); 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #code} (A code that identifies the component. Codes may be 1114 * used to differentiate between kinds of taxes, surcharges, discounts 1115 * etc.) 1116 */ 1117 public CodeableConcept getCode() { 1118 if (this.code == null) 1119 if (Configuration.errorOnAutoCreate()) 1120 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.code"); 1121 else if (Configuration.doAutoCreate()) 1122 this.code = new CodeableConcept(); // cc 1123 return this.code; 1124 } 1125 1126 public boolean hasCode() { 1127 return this.code != null && !this.code.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #code} (A code that identifies the component. Codes may 1132 * be used to differentiate between kinds of taxes, surcharges, 1133 * discounts etc.) 1134 */ 1135 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setCode(CodeableConcept value) { 1136 this.code = value; 1137 return this; 1138 } 1139 1140 /** 1141 * @return {@link #factor} (The factor that has been applied on the base price 1142 * for calculating this component.). This is the underlying object with 1143 * id, value and extensions. The accessor "getFactor" gives direct 1144 * access to the value 1145 */ 1146 public DecimalType getFactorElement() { 1147 if (this.factor == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.factor"); 1150 else if (Configuration.doAutoCreate()) 1151 this.factor = new DecimalType(); // bb 1152 return this.factor; 1153 } 1154 1155 public boolean hasFactorElement() { 1156 return this.factor != null && !this.factor.isEmpty(); 1157 } 1158 1159 public boolean hasFactor() { 1160 return this.factor != null && !this.factor.isEmpty(); 1161 } 1162 1163 /** 1164 * @param value {@link #factor} (The factor that has been applied on the base 1165 * price for calculating this component.). This is the underlying 1166 * object with id, value and extensions. The accessor "getFactor" 1167 * gives direct access to the value 1168 */ 1169 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactorElement(DecimalType value) { 1170 this.factor = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return The factor that has been applied on the base price for calculating 1176 * this component. 1177 */ 1178 public BigDecimal getFactor() { 1179 return this.factor == null ? null : this.factor.getValue(); 1180 } 1181 1182 /** 1183 * @param value The factor that has been applied on the base price for 1184 * calculating this component. 1185 */ 1186 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(BigDecimal value) { 1187 if (value == null) 1188 this.factor = null; 1189 else { 1190 if (this.factor == null) 1191 this.factor = new DecimalType(); 1192 this.factor.setValue(value); 1193 } 1194 return this; 1195 } 1196 1197 /** 1198 * @param value The factor that has been applied on the base price for 1199 * calculating this component. 1200 */ 1201 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(long value) { 1202 this.factor = new DecimalType(); 1203 this.factor.setValue(value); 1204 return this; 1205 } 1206 1207 /** 1208 * @param value The factor that has been applied on the base price for 1209 * calculating this component. 1210 */ 1211 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setFactor(double value) { 1212 this.factor = new DecimalType(); 1213 this.factor.setValue(value); 1214 return this; 1215 } 1216 1217 /** 1218 * @return {@link #amount} (The amount calculated for this component.) 1219 */ 1220 public Money getAmount() { 1221 if (this.amount == null) 1222 if (Configuration.errorOnAutoCreate()) 1223 throw new Error("Attempt to auto-create ChargeItemDefinitionPropertyGroupPriceComponentComponent.amount"); 1224 else if (Configuration.doAutoCreate()) 1225 this.amount = new Money(); // cc 1226 return this.amount; 1227 } 1228 1229 public boolean hasAmount() { 1230 return this.amount != null && !this.amount.isEmpty(); 1231 } 1232 1233 /** 1234 * @param value {@link #amount} (The amount calculated for this component.) 1235 */ 1236 public ChargeItemDefinitionPropertyGroupPriceComponentComponent setAmount(Money value) { 1237 this.amount = value; 1238 return this; 1239 } 1240 1241 protected void listChildren(List<Property> children) { 1242 super.listChildren(children); 1243 children.add(new Property("type", "code", "This code identifies the type of the component.", 0, 1, type)); 1244 children.add(new Property("code", "CodeableConcept", 1245 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1246 0, 1, code)); 1247 children.add(new Property("factor", "decimal", 1248 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor)); 1249 children.add(new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount)); 1250 } 1251 1252 @Override 1253 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1254 switch (_hash) { 1255 case 3575610: 1256 /* type */ return new Property("type", "code", "This code identifies the type of the component.", 0, 1, type); 1257 case 3059181: 1258 /* code */ return new Property("code", "CodeableConcept", 1259 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1260 0, 1, code); 1261 case -1282148017: 1262 /* factor */ return new Property("factor", "decimal", 1263 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor); 1264 case -1413853096: 1265 /* amount */ return new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount); 1266 default: 1267 return super.getNamedProperty(_hash, _name, _checkValid); 1268 } 1269 1270 } 1271 1272 @Override 1273 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1274 switch (hash) { 1275 case 3575610: 1276 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ChargeItemDefinitionPriceComponentType> 1277 case 3059181: 1278 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1279 case -1282148017: 1280 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 1281 case -1413853096: 1282 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1283 default: 1284 return super.getProperty(hash, name, checkValid); 1285 } 1286 1287 } 1288 1289 @Override 1290 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1291 switch (hash) { 1292 case 3575610: // type 1293 value = new ChargeItemDefinitionPriceComponentTypeEnumFactory().fromType(castToCode(value)); 1294 this.type = (Enumeration) value; // Enumeration<ChargeItemDefinitionPriceComponentType> 1295 return value; 1296 case 3059181: // code 1297 this.code = castToCodeableConcept(value); // CodeableConcept 1298 return value; 1299 case -1282148017: // factor 1300 this.factor = castToDecimal(value); // DecimalType 1301 return value; 1302 case -1413853096: // amount 1303 this.amount = castToMoney(value); // Money 1304 return value; 1305 default: 1306 return super.setProperty(hash, name, value); 1307 } 1308 1309 } 1310 1311 @Override 1312 public Base setProperty(String name, Base value) throws FHIRException { 1313 if (name.equals("type")) { 1314 value = new ChargeItemDefinitionPriceComponentTypeEnumFactory().fromType(castToCode(value)); 1315 this.type = (Enumeration) value; // Enumeration<ChargeItemDefinitionPriceComponentType> 1316 } else if (name.equals("code")) { 1317 this.code = castToCodeableConcept(value); // CodeableConcept 1318 } else if (name.equals("factor")) { 1319 this.factor = castToDecimal(value); // DecimalType 1320 } else if (name.equals("amount")) { 1321 this.amount = castToMoney(value); // Money 1322 } else 1323 return super.setProperty(name, value); 1324 return value; 1325 } 1326 1327 @Override 1328 public void removeChild(String name, Base value) throws FHIRException { 1329 if (name.equals("type")) { 1330 this.type = null; 1331 } else if (name.equals("code")) { 1332 this.code = null; 1333 } else if (name.equals("factor")) { 1334 this.factor = null; 1335 } else if (name.equals("amount")) { 1336 this.amount = null; 1337 } else 1338 super.removeChild(name, value); 1339 1340 } 1341 1342 @Override 1343 public Base makeProperty(int hash, String name) throws FHIRException { 1344 switch (hash) { 1345 case 3575610: 1346 return getTypeElement(); 1347 case 3059181: 1348 return getCode(); 1349 case -1282148017: 1350 return getFactorElement(); 1351 case -1413853096: 1352 return getAmount(); 1353 default: 1354 return super.makeProperty(hash, name); 1355 } 1356 1357 } 1358 1359 @Override 1360 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1361 switch (hash) { 1362 case 3575610: 1363 /* type */ return new String[] { "code" }; 1364 case 3059181: 1365 /* code */ return new String[] { "CodeableConcept" }; 1366 case -1282148017: 1367 /* factor */ return new String[] { "decimal" }; 1368 case -1413853096: 1369 /* amount */ return new String[] { "Money" }; 1370 default: 1371 return super.getTypesForProperty(hash, name); 1372 } 1373 1374 } 1375 1376 @Override 1377 public Base addChild(String name) throws FHIRException { 1378 if (name.equals("type")) { 1379 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.type"); 1380 } else if (name.equals("code")) { 1381 this.code = new CodeableConcept(); 1382 return this.code; 1383 } else if (name.equals("factor")) { 1384 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.factor"); 1385 } else if (name.equals("amount")) { 1386 this.amount = new Money(); 1387 return this.amount; 1388 } else 1389 return super.addChild(name); 1390 } 1391 1392 public ChargeItemDefinitionPropertyGroupPriceComponentComponent copy() { 1393 ChargeItemDefinitionPropertyGroupPriceComponentComponent dst = new ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 1394 copyValues(dst); 1395 return dst; 1396 } 1397 1398 public void copyValues(ChargeItemDefinitionPropertyGroupPriceComponentComponent dst) { 1399 super.copyValues(dst); 1400 dst.type = type == null ? null : type.copy(); 1401 dst.code = code == null ? null : code.copy(); 1402 dst.factor = factor == null ? null : factor.copy(); 1403 dst.amount = amount == null ? null : amount.copy(); 1404 } 1405 1406 @Override 1407 public boolean equalsDeep(Base other_) { 1408 if (!super.equalsDeep(other_)) 1409 return false; 1410 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupPriceComponentComponent)) 1411 return false; 1412 ChargeItemDefinitionPropertyGroupPriceComponentComponent o = (ChargeItemDefinitionPropertyGroupPriceComponentComponent) other_; 1413 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(factor, o.factor, true) 1414 && compareDeep(amount, o.amount, true); 1415 } 1416 1417 @Override 1418 public boolean equalsShallow(Base other_) { 1419 if (!super.equalsShallow(other_)) 1420 return false; 1421 if (!(other_ instanceof ChargeItemDefinitionPropertyGroupPriceComponentComponent)) 1422 return false; 1423 ChargeItemDefinitionPropertyGroupPriceComponentComponent o = (ChargeItemDefinitionPropertyGroupPriceComponentComponent) other_; 1424 return compareValues(type, o.type, true) && compareValues(factor, o.factor, true); 1425 } 1426 1427 public boolean isEmpty() { 1428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, factor, amount); 1429 } 1430 1431 public String fhirType() { 1432 return "ChargeItemDefinition.propertyGroup.priceComponent"; 1433 1434 } 1435 1436 } 1437 1438 /** 1439 * A formal identifier that is used to identify this charge item definition when 1440 * it is represented in other formats, or referenced in a specification, model, 1441 * design or an instance. 1442 */ 1443 @Child(name = "identifier", type = { 1444 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1445 @Description(shortDefinition = "Additional identifier for the charge item definition", formalDefinition = "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1446 protected List<Identifier> identifier; 1447 1448 /** 1449 * The URL pointing to an externally-defined charge item definition that is 1450 * adhered to in whole or in part by this definition. 1451 */ 1452 @Child(name = "derivedFromUri", type = { 1453 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1454 @Description(shortDefinition = "Underlying externally-defined charge item definition", formalDefinition = "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.") 1455 protected List<UriType> derivedFromUri; 1456 1457 /** 1458 * A larger definition of which this particular definition is a component or 1459 * step. 1460 */ 1461 @Child(name = "partOf", type = { 1462 CanonicalType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1463 @Description(shortDefinition = "A larger definition of which this particular definition is a component or step", formalDefinition = "A larger definition of which this particular definition is a component or step.") 1464 protected List<CanonicalType> partOf; 1465 1466 /** 1467 * As new versions of a protocol or guideline are defined, allows identification 1468 * of what versions are replaced by a new instance. 1469 */ 1470 @Child(name = "replaces", type = { 1471 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1472 @Description(shortDefinition = "Completed or terminated request(s) whose function is taken by this new request", formalDefinition = "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.") 1473 protected List<CanonicalType> replaces; 1474 1475 /** 1476 * A copyright statement relating to the charge item definition and/or its 1477 * contents. Copyright statements are generally legal restrictions on the use 1478 * and publishing of the charge item definition. 1479 */ 1480 @Child(name = "copyright", type = { 1481 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1482 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.") 1483 protected MarkdownType copyright; 1484 1485 /** 1486 * The date on which the resource content was approved by the publisher. 1487 * Approval happens once when the content is officially approved for usage. 1488 */ 1489 @Child(name = "approvalDate", type = { 1490 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1491 @Description(shortDefinition = "When the charge item definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1492 protected DateType approvalDate; 1493 1494 /** 1495 * The date on which the resource content was last reviewed. Review happens 1496 * periodically after approval but does not change the original approval date. 1497 */ 1498 @Child(name = "lastReviewDate", type = { 1499 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1500 @Description(shortDefinition = "When the charge item definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1501 protected DateType lastReviewDate; 1502 1503 /** 1504 * The period during which the charge item definition content was or is planned 1505 * to be in active use. 1506 */ 1507 @Child(name = "effectivePeriod", type = { 1508 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1509 @Description(shortDefinition = "When the charge item definition is expected to be used", formalDefinition = "The period during which the charge item definition content was or is planned to be in active use.") 1510 protected Period effectivePeriod; 1511 1512 /** 1513 * The defined billing details in this resource pertain to the given billing 1514 * code. 1515 */ 1516 @Child(name = "code", type = { CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1517 @Description(shortDefinition = "Billing codes or product types this definition applies to", formalDefinition = "The defined billing details in this resource pertain to the given billing code.") 1518 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chargeitem-billingcodes") 1519 protected CodeableConcept code; 1520 1521 /** 1522 * The defined billing details in this resource pertain to the given product 1523 * instance(s). 1524 */ 1525 @Child(name = "instance", type = { Medication.class, Substance.class, 1526 Device.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1527 @Description(shortDefinition = "Instances this definition applies to", formalDefinition = "The defined billing details in this resource pertain to the given product instance(s).") 1528 protected List<Reference> instance; 1529 /** 1530 * The actual objects that are the target of the reference (The defined billing 1531 * details in this resource pertain to the given product instance(s).) 1532 */ 1533 protected List<Resource> instanceTarget; 1534 1535 /** 1536 * Expressions that describe applicability criteria for the billing code. 1537 */ 1538 @Child(name = "applicability", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1539 @Description(shortDefinition = "Whether or not the billing code is applicable", formalDefinition = "Expressions that describe applicability criteria for the billing code.") 1540 protected List<ChargeItemDefinitionApplicabilityComponent> applicability; 1541 1542 /** 1543 * Group of properties which are applicable under the same conditions. If no 1544 * applicability rules are established for the group, then all properties always 1545 * apply. 1546 */ 1547 @Child(name = "propertyGroup", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1548 @Description(shortDefinition = "Group of properties which are applicable under the same conditions", formalDefinition = "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.") 1549 protected List<ChargeItemDefinitionPropertyGroupComponent> propertyGroup; 1550 1551 private static final long serialVersionUID = -583681330L; 1552 1553 /** 1554 * Constructor 1555 */ 1556 public ChargeItemDefinition() { 1557 super(); 1558 } 1559 1560 /** 1561 * Constructor 1562 */ 1563 public ChargeItemDefinition(UriType url, Enumeration<PublicationStatus> status) { 1564 super(); 1565 this.url = url; 1566 this.status = status; 1567 } 1568 1569 /** 1570 * @return {@link #url} (An absolute URI that is used to identify this charge 1571 * item definition when it is referenced in a specification, model, 1572 * design or an instance; also called its canonical identifier. This 1573 * SHOULD be globally unique and SHOULD be a literal address at which at 1574 * which an authoritative instance of this charge item definition is (or 1575 * will be) published. This URL can be the target of a canonical 1576 * reference. It SHALL remain the same when the charge item definition 1577 * is stored on different servers.). This is the underlying object with 1578 * id, value and extensions. The accessor "getUrl" gives direct access 1579 * to the value 1580 */ 1581 public UriType getUrlElement() { 1582 if (this.url == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create ChargeItemDefinition.url"); 1585 else if (Configuration.doAutoCreate()) 1586 this.url = new UriType(); // bb 1587 return this.url; 1588 } 1589 1590 public boolean hasUrlElement() { 1591 return this.url != null && !this.url.isEmpty(); 1592 } 1593 1594 public boolean hasUrl() { 1595 return this.url != null && !this.url.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #url} (An absolute URI that is used to identify this 1600 * charge item definition when it is referenced in a specification, 1601 * model, design or an instance; also called its canonical 1602 * identifier. This SHOULD be globally unique and SHOULD be a 1603 * literal address at which at which an authoritative instance of 1604 * this charge item definition is (or will be) published. This URL 1605 * can be the target of a canonical reference. It SHALL remain the 1606 * same when the charge item definition is stored on different 1607 * servers.). This is the underlying object with id, value and 1608 * extensions. The accessor "getUrl" gives direct access to the 1609 * value 1610 */ 1611 public ChargeItemDefinition setUrlElement(UriType value) { 1612 this.url = value; 1613 return this; 1614 } 1615 1616 /** 1617 * @return An absolute URI that is used to identify this charge item definition 1618 * when it is referenced in a specification, model, design or an 1619 * instance; also called its canonical identifier. This SHOULD be 1620 * globally unique and SHOULD be a literal address at which at which an 1621 * authoritative instance of this charge item definition is (or will be) 1622 * published. This URL can be the target of a canonical reference. It 1623 * SHALL remain the same when the charge item definition is stored on 1624 * different servers. 1625 */ 1626 public String getUrl() { 1627 return this.url == null ? null : this.url.getValue(); 1628 } 1629 1630 /** 1631 * @param value An absolute URI that is used to identify this charge item 1632 * definition when it is referenced in a specification, model, 1633 * design or an instance; also called its canonical identifier. 1634 * This SHOULD be globally unique and SHOULD be a literal address 1635 * at which at which an authoritative instance of this charge item 1636 * definition is (or will be) published. This URL can be the target 1637 * of a canonical reference. It SHALL remain the same when the 1638 * charge item definition is stored on different servers. 1639 */ 1640 public ChargeItemDefinition setUrl(String value) { 1641 if (this.url == null) 1642 this.url = new UriType(); 1643 this.url.setValue(value); 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #identifier} (A formal identifier that is used to identify 1649 * this charge item definition when it is represented in other formats, 1650 * or referenced in a specification, model, design or an instance.) 1651 */ 1652 public List<Identifier> getIdentifier() { 1653 if (this.identifier == null) 1654 this.identifier = new ArrayList<Identifier>(); 1655 return this.identifier; 1656 } 1657 1658 /** 1659 * @return Returns a reference to <code>this</code> for easy method chaining 1660 */ 1661 public ChargeItemDefinition setIdentifier(List<Identifier> theIdentifier) { 1662 this.identifier = theIdentifier; 1663 return this; 1664 } 1665 1666 public boolean hasIdentifier() { 1667 if (this.identifier == null) 1668 return false; 1669 for (Identifier item : this.identifier) 1670 if (!item.isEmpty()) 1671 return true; 1672 return false; 1673 } 1674 1675 public Identifier addIdentifier() { // 3 1676 Identifier t = new Identifier(); 1677 if (this.identifier == null) 1678 this.identifier = new ArrayList<Identifier>(); 1679 this.identifier.add(t); 1680 return t; 1681 } 1682 1683 public ChargeItemDefinition addIdentifier(Identifier t) { // 3 1684 if (t == null) 1685 return this; 1686 if (this.identifier == null) 1687 this.identifier = new ArrayList<Identifier>(); 1688 this.identifier.add(t); 1689 return this; 1690 } 1691 1692 /** 1693 * @return The first repetition of repeating field {@link #identifier}, creating 1694 * it if it does not already exist 1695 */ 1696 public Identifier getIdentifierFirstRep() { 1697 if (getIdentifier().isEmpty()) { 1698 addIdentifier(); 1699 } 1700 return getIdentifier().get(0); 1701 } 1702 1703 /** 1704 * @return {@link #version} (The identifier that is used to identify this 1705 * version of the charge item definition when it is referenced in a 1706 * specification, model, design or instance. This is an arbitrary value 1707 * managed by the charge item definition author and is not expected to 1708 * be globally unique. For example, it might be a timestamp (e.g. 1709 * yyyymmdd) if a managed version is not available. There is also no 1710 * expectation that versions can be placed in a lexicographical 1711 * sequence. To provide a version consistent with the Decision Support 1712 * Service specification, use the format Major.Minor.Revision (e.g. 1713 * 1.0.0). For more information on versioning knowledge assets, refer to 1714 * the Decision Support Service specification. Note that a version is 1715 * required for non-experimental active assets.). This is the underlying 1716 * object with id, value and extensions. The accessor "getVersion" gives 1717 * direct access to the value 1718 */ 1719 public StringType getVersionElement() { 1720 if (this.version == null) 1721 if (Configuration.errorOnAutoCreate()) 1722 throw new Error("Attempt to auto-create ChargeItemDefinition.version"); 1723 else if (Configuration.doAutoCreate()) 1724 this.version = new StringType(); // bb 1725 return this.version; 1726 } 1727 1728 public boolean hasVersionElement() { 1729 return this.version != null && !this.version.isEmpty(); 1730 } 1731 1732 public boolean hasVersion() { 1733 return this.version != null && !this.version.isEmpty(); 1734 } 1735 1736 /** 1737 * @param value {@link #version} (The identifier that is used to identify this 1738 * version of the charge item definition when it is referenced in a 1739 * specification, model, design or instance. This is an arbitrary 1740 * value managed by the charge item definition author and is not 1741 * expected to be globally unique. For example, it might be a 1742 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1743 * There is also no expectation that versions can be placed in a 1744 * lexicographical sequence. To provide a version consistent with 1745 * the Decision Support Service specification, use the format 1746 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1747 * versioning knowledge assets, refer to the Decision Support 1748 * Service specification. Note that a version is required for 1749 * non-experimental active assets.). This is the underlying object 1750 * with id, value and extensions. The accessor "getVersion" gives 1751 * direct access to the value 1752 */ 1753 public ChargeItemDefinition setVersionElement(StringType value) { 1754 this.version = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return The identifier that is used to identify this version of the charge 1760 * item definition when it is referenced in a specification, model, 1761 * design or instance. This is an arbitrary value managed by the charge 1762 * item definition author and is not expected to be globally unique. For 1763 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 1764 * is not available. There is also no expectation that versions can be 1765 * placed in a lexicographical sequence. To provide a version consistent 1766 * with the Decision Support Service specification, use the format 1767 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 1768 * knowledge assets, refer to the Decision Support Service 1769 * specification. Note that a version is required for non-experimental 1770 * active assets. 1771 */ 1772 public String getVersion() { 1773 return this.version == null ? null : this.version.getValue(); 1774 } 1775 1776 /** 1777 * @param value The identifier that is used to identify this version of the 1778 * charge item definition when it is referenced in a specification, 1779 * model, design or instance. This is an arbitrary value managed by 1780 * the charge item definition author and is not expected to be 1781 * globally unique. For example, it might be a timestamp (e.g. 1782 * yyyymmdd) if a managed version is not available. There is also 1783 * no expectation that versions can be placed in a lexicographical 1784 * sequence. To provide a version consistent with the Decision 1785 * Support Service specification, use the format 1786 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1787 * versioning knowledge assets, refer to the Decision Support 1788 * Service specification. Note that a version is required for 1789 * non-experimental active assets. 1790 */ 1791 public ChargeItemDefinition setVersion(String value) { 1792 if (Utilities.noString(value)) 1793 this.version = null; 1794 else { 1795 if (this.version == null) 1796 this.version = new StringType(); 1797 this.version.setValue(value); 1798 } 1799 return this; 1800 } 1801 1802 /** 1803 * @return {@link #title} (A short, descriptive, user-friendly title for the 1804 * charge item definition.). This is the underlying object with id, 1805 * value and extensions. The accessor "getTitle" gives direct access to 1806 * the value 1807 */ 1808 public StringType getTitleElement() { 1809 if (this.title == null) 1810 if (Configuration.errorOnAutoCreate()) 1811 throw new Error("Attempt to auto-create ChargeItemDefinition.title"); 1812 else if (Configuration.doAutoCreate()) 1813 this.title = new StringType(); // bb 1814 return this.title; 1815 } 1816 1817 public boolean hasTitleElement() { 1818 return this.title != null && !this.title.isEmpty(); 1819 } 1820 1821 public boolean hasTitle() { 1822 return this.title != null && !this.title.isEmpty(); 1823 } 1824 1825 /** 1826 * @param value {@link #title} (A short, descriptive, user-friendly title for 1827 * the charge item definition.). This is the underlying object with 1828 * id, value and extensions. The accessor "getTitle" gives direct 1829 * access to the value 1830 */ 1831 public ChargeItemDefinition setTitleElement(StringType value) { 1832 this.title = value; 1833 return this; 1834 } 1835 1836 /** 1837 * @return A short, descriptive, user-friendly title for the charge item 1838 * definition. 1839 */ 1840 public String getTitle() { 1841 return this.title == null ? null : this.title.getValue(); 1842 } 1843 1844 /** 1845 * @param value A short, descriptive, user-friendly title for the charge item 1846 * definition. 1847 */ 1848 public ChargeItemDefinition setTitle(String value) { 1849 if (Utilities.noString(value)) 1850 this.title = null; 1851 else { 1852 if (this.title == null) 1853 this.title = new StringType(); 1854 this.title.setValue(value); 1855 } 1856 return this; 1857 } 1858 1859 /** 1860 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined 1861 * charge item definition that is adhered to in whole or in part by this 1862 * definition.) 1863 */ 1864 public List<UriType> getDerivedFromUri() { 1865 if (this.derivedFromUri == null) 1866 this.derivedFromUri = new ArrayList<UriType>(); 1867 return this.derivedFromUri; 1868 } 1869 1870 /** 1871 * @return Returns a reference to <code>this</code> for easy method chaining 1872 */ 1873 public ChargeItemDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 1874 this.derivedFromUri = theDerivedFromUri; 1875 return this; 1876 } 1877 1878 public boolean hasDerivedFromUri() { 1879 if (this.derivedFromUri == null) 1880 return false; 1881 for (UriType item : this.derivedFromUri) 1882 if (!item.isEmpty()) 1883 return true; 1884 return false; 1885 } 1886 1887 /** 1888 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined 1889 * charge item definition that is adhered to in whole or in part by this 1890 * definition.) 1891 */ 1892 public UriType addDerivedFromUriElement() {// 2 1893 UriType t = new UriType(); 1894 if (this.derivedFromUri == null) 1895 this.derivedFromUri = new ArrayList<UriType>(); 1896 this.derivedFromUri.add(t); 1897 return t; 1898 } 1899 1900 /** 1901 * @param value {@link #derivedFromUri} (The URL pointing to an 1902 * externally-defined charge item definition that is adhered to in 1903 * whole or in part by this definition.) 1904 */ 1905 public ChargeItemDefinition addDerivedFromUri(String value) { // 1 1906 UriType t = new UriType(); 1907 t.setValue(value); 1908 if (this.derivedFromUri == null) 1909 this.derivedFromUri = new ArrayList<UriType>(); 1910 this.derivedFromUri.add(t); 1911 return this; 1912 } 1913 1914 /** 1915 * @param value {@link #derivedFromUri} (The URL pointing to an 1916 * externally-defined charge item definition that is adhered to in 1917 * whole or in part by this definition.) 1918 */ 1919 public boolean hasDerivedFromUri(String value) { 1920 if (this.derivedFromUri == null) 1921 return false; 1922 for (UriType v : this.derivedFromUri) 1923 if (v.getValue().equals(value)) // uri 1924 return true; 1925 return false; 1926 } 1927 1928 /** 1929 * @return {@link #partOf} (A larger definition of which this particular 1930 * definition is a component or step.) 1931 */ 1932 public List<CanonicalType> getPartOf() { 1933 if (this.partOf == null) 1934 this.partOf = new ArrayList<CanonicalType>(); 1935 return this.partOf; 1936 } 1937 1938 /** 1939 * @return Returns a reference to <code>this</code> for easy method chaining 1940 */ 1941 public ChargeItemDefinition setPartOf(List<CanonicalType> thePartOf) { 1942 this.partOf = thePartOf; 1943 return this; 1944 } 1945 1946 public boolean hasPartOf() { 1947 if (this.partOf == null) 1948 return false; 1949 for (CanonicalType item : this.partOf) 1950 if (!item.isEmpty()) 1951 return true; 1952 return false; 1953 } 1954 1955 /** 1956 * @return {@link #partOf} (A larger definition of which this particular 1957 * definition is a component or step.) 1958 */ 1959 public CanonicalType addPartOfElement() {// 2 1960 CanonicalType t = new CanonicalType(); 1961 if (this.partOf == null) 1962 this.partOf = new ArrayList<CanonicalType>(); 1963 this.partOf.add(t); 1964 return t; 1965 } 1966 1967 /** 1968 * @param value {@link #partOf} (A larger definition of which this particular 1969 * definition is a component or step.) 1970 */ 1971 public ChargeItemDefinition addPartOf(String value) { // 1 1972 CanonicalType t = new CanonicalType(); 1973 t.setValue(value); 1974 if (this.partOf == null) 1975 this.partOf = new ArrayList<CanonicalType>(); 1976 this.partOf.add(t); 1977 return this; 1978 } 1979 1980 /** 1981 * @param value {@link #partOf} (A larger definition of which this particular 1982 * definition is a component or step.) 1983 */ 1984 public boolean hasPartOf(String value) { 1985 if (this.partOf == null) 1986 return false; 1987 for (CanonicalType v : this.partOf) 1988 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 1989 return true; 1990 return false; 1991 } 1992 1993 /** 1994 * @return {@link #replaces} (As new versions of a protocol or guideline are 1995 * defined, allows identification of what versions are replaced by a new 1996 * instance.) 1997 */ 1998 public List<CanonicalType> getReplaces() { 1999 if (this.replaces == null) 2000 this.replaces = new ArrayList<CanonicalType>(); 2001 return this.replaces; 2002 } 2003 2004 /** 2005 * @return Returns a reference to <code>this</code> for easy method chaining 2006 */ 2007 public ChargeItemDefinition setReplaces(List<CanonicalType> theReplaces) { 2008 this.replaces = theReplaces; 2009 return this; 2010 } 2011 2012 public boolean hasReplaces() { 2013 if (this.replaces == null) 2014 return false; 2015 for (CanonicalType item : this.replaces) 2016 if (!item.isEmpty()) 2017 return true; 2018 return false; 2019 } 2020 2021 /** 2022 * @return {@link #replaces} (As new versions of a protocol or guideline are 2023 * defined, allows identification of what versions are replaced by a new 2024 * instance.) 2025 */ 2026 public CanonicalType addReplacesElement() {// 2 2027 CanonicalType t = new CanonicalType(); 2028 if (this.replaces == null) 2029 this.replaces = new ArrayList<CanonicalType>(); 2030 this.replaces.add(t); 2031 return t; 2032 } 2033 2034 /** 2035 * @param value {@link #replaces} (As new versions of a protocol or guideline 2036 * are defined, allows identification of what versions are replaced 2037 * by a new instance.) 2038 */ 2039 public ChargeItemDefinition addReplaces(String value) { // 1 2040 CanonicalType t = new CanonicalType(); 2041 t.setValue(value); 2042 if (this.replaces == null) 2043 this.replaces = new ArrayList<CanonicalType>(); 2044 this.replaces.add(t); 2045 return this; 2046 } 2047 2048 /** 2049 * @param value {@link #replaces} (As new versions of a protocol or guideline 2050 * are defined, allows identification of what versions are replaced 2051 * by a new instance.) 2052 */ 2053 public boolean hasReplaces(String value) { 2054 if (this.replaces == null) 2055 return false; 2056 for (CanonicalType v : this.replaces) 2057 if (v.getValue().equals(value)) // canonical(ChargeItemDefinition) 2058 return true; 2059 return false; 2060 } 2061 2062 /** 2063 * @return {@link #status} (The current state of the ChargeItemDefinition.). 2064 * This is the underlying object with id, value and extensions. The 2065 * accessor "getStatus" gives direct access to the value 2066 */ 2067 public Enumeration<PublicationStatus> getStatusElement() { 2068 if (this.status == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create ChargeItemDefinition.status"); 2071 else if (Configuration.doAutoCreate()) 2072 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2073 return this.status; 2074 } 2075 2076 public boolean hasStatusElement() { 2077 return this.status != null && !this.status.isEmpty(); 2078 } 2079 2080 public boolean hasStatus() { 2081 return this.status != null && !this.status.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #status} (The current state of the 2086 * ChargeItemDefinition.). This is the underlying object with id, 2087 * value and extensions. The accessor "getStatus" gives direct 2088 * access to the value 2089 */ 2090 public ChargeItemDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2091 this.status = value; 2092 return this; 2093 } 2094 2095 /** 2096 * @return The current state of the ChargeItemDefinition. 2097 */ 2098 public PublicationStatus getStatus() { 2099 return this.status == null ? null : this.status.getValue(); 2100 } 2101 2102 /** 2103 * @param value The current state of the ChargeItemDefinition. 2104 */ 2105 public ChargeItemDefinition setStatus(PublicationStatus value) { 2106 if (this.status == null) 2107 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2108 this.status.setValue(value); 2109 return this; 2110 } 2111 2112 /** 2113 * @return {@link #experimental} (A Boolean value to indicate that this charge 2114 * item definition is authored for testing purposes (or 2115 * education/evaluation/marketing) and is not intended to be used for 2116 * genuine usage.). This is the underlying object with id, value and 2117 * extensions. The accessor "getExperimental" gives direct access to the 2118 * value 2119 */ 2120 public BooleanType getExperimentalElement() { 2121 if (this.experimental == null) 2122 if (Configuration.errorOnAutoCreate()) 2123 throw new Error("Attempt to auto-create ChargeItemDefinition.experimental"); 2124 else if (Configuration.doAutoCreate()) 2125 this.experimental = new BooleanType(); // bb 2126 return this.experimental; 2127 } 2128 2129 public boolean hasExperimentalElement() { 2130 return this.experimental != null && !this.experimental.isEmpty(); 2131 } 2132 2133 public boolean hasExperimental() { 2134 return this.experimental != null && !this.experimental.isEmpty(); 2135 } 2136 2137 /** 2138 * @param value {@link #experimental} (A Boolean value to indicate that this 2139 * charge item definition is authored for testing purposes (or 2140 * education/evaluation/marketing) and is not intended to be used 2141 * for genuine usage.). This is the underlying object with id, 2142 * value and extensions. The accessor "getExperimental" gives 2143 * direct access to the value 2144 */ 2145 public ChargeItemDefinition setExperimentalElement(BooleanType value) { 2146 this.experimental = value; 2147 return this; 2148 } 2149 2150 /** 2151 * @return A Boolean value to indicate that this charge item definition is 2152 * authored for testing purposes (or education/evaluation/marketing) and 2153 * is not intended to be used for genuine usage. 2154 */ 2155 public boolean getExperimental() { 2156 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2157 } 2158 2159 /** 2160 * @param value A Boolean value to indicate that this charge item definition is 2161 * authored for testing purposes (or 2162 * education/evaluation/marketing) and is not intended to be used 2163 * for genuine usage. 2164 */ 2165 public ChargeItemDefinition setExperimental(boolean value) { 2166 if (this.experimental == null) 2167 this.experimental = new BooleanType(); 2168 this.experimental.setValue(value); 2169 return this; 2170 } 2171 2172 /** 2173 * @return {@link #date} (The date (and optionally time) when the charge item 2174 * definition was published. The date must change when the business 2175 * version changes and it must change if the status code changes. In 2176 * addition, it should change when the substantive content of the charge 2177 * item definition changes.). This is the underlying object with id, 2178 * value and extensions. The accessor "getDate" gives direct access to 2179 * the value 2180 */ 2181 public DateTimeType getDateElement() { 2182 if (this.date == null) 2183 if (Configuration.errorOnAutoCreate()) 2184 throw new Error("Attempt to auto-create ChargeItemDefinition.date"); 2185 else if (Configuration.doAutoCreate()) 2186 this.date = new DateTimeType(); // bb 2187 return this.date; 2188 } 2189 2190 public boolean hasDateElement() { 2191 return this.date != null && !this.date.isEmpty(); 2192 } 2193 2194 public boolean hasDate() { 2195 return this.date != null && !this.date.isEmpty(); 2196 } 2197 2198 /** 2199 * @param value {@link #date} (The date (and optionally time) when the charge 2200 * item definition was published. The date must change when the 2201 * business version changes and it must change if the status code 2202 * changes. In addition, it should change when the substantive 2203 * content of the charge item definition changes.). This is the 2204 * underlying object with id, value and extensions. The accessor 2205 * "getDate" gives direct access to the value 2206 */ 2207 public ChargeItemDefinition setDateElement(DateTimeType value) { 2208 this.date = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return The date (and optionally time) when the charge item definition was 2214 * published. The date must change when the business version changes and 2215 * it must change if the status code changes. In addition, it should 2216 * change when the substantive content of the charge item definition 2217 * changes. 2218 */ 2219 public Date getDate() { 2220 return this.date == null ? null : this.date.getValue(); 2221 } 2222 2223 /** 2224 * @param value The date (and optionally time) when the charge item definition 2225 * was published. The date must change when the business version 2226 * changes and it must change if the status code changes. In 2227 * addition, it should change when the substantive content of the 2228 * charge item definition changes. 2229 */ 2230 public ChargeItemDefinition setDate(Date value) { 2231 if (value == null) 2232 this.date = null; 2233 else { 2234 if (this.date == null) 2235 this.date = new DateTimeType(); 2236 this.date.setValue(value); 2237 } 2238 return this; 2239 } 2240 2241 /** 2242 * @return {@link #publisher} (The name of the organization or individual that 2243 * published the charge item definition.). This is the underlying object 2244 * with id, value and extensions. The accessor "getPublisher" gives 2245 * direct access to the value 2246 */ 2247 public StringType getPublisherElement() { 2248 if (this.publisher == null) 2249 if (Configuration.errorOnAutoCreate()) 2250 throw new Error("Attempt to auto-create ChargeItemDefinition.publisher"); 2251 else if (Configuration.doAutoCreate()) 2252 this.publisher = new StringType(); // bb 2253 return this.publisher; 2254 } 2255 2256 public boolean hasPublisherElement() { 2257 return this.publisher != null && !this.publisher.isEmpty(); 2258 } 2259 2260 public boolean hasPublisher() { 2261 return this.publisher != null && !this.publisher.isEmpty(); 2262 } 2263 2264 /** 2265 * @param value {@link #publisher} (The name of the organization or individual 2266 * that published the charge item definition.). This is the 2267 * underlying object with id, value and extensions. The accessor 2268 * "getPublisher" gives direct access to the value 2269 */ 2270 public ChargeItemDefinition setPublisherElement(StringType value) { 2271 this.publisher = value; 2272 return this; 2273 } 2274 2275 /** 2276 * @return The name of the organization or individual that published the charge 2277 * item definition. 2278 */ 2279 public String getPublisher() { 2280 return this.publisher == null ? null : this.publisher.getValue(); 2281 } 2282 2283 /** 2284 * @param value The name of the organization or individual that published the 2285 * charge item definition. 2286 */ 2287 public ChargeItemDefinition setPublisher(String value) { 2288 if (Utilities.noString(value)) 2289 this.publisher = null; 2290 else { 2291 if (this.publisher == null) 2292 this.publisher = new StringType(); 2293 this.publisher.setValue(value); 2294 } 2295 return this; 2296 } 2297 2298 /** 2299 * @return {@link #contact} (Contact details to assist a user in finding and 2300 * communicating with the publisher.) 2301 */ 2302 public List<ContactDetail> getContact() { 2303 if (this.contact == null) 2304 this.contact = new ArrayList<ContactDetail>(); 2305 return this.contact; 2306 } 2307 2308 /** 2309 * @return Returns a reference to <code>this</code> for easy method chaining 2310 */ 2311 public ChargeItemDefinition setContact(List<ContactDetail> theContact) { 2312 this.contact = theContact; 2313 return this; 2314 } 2315 2316 public boolean hasContact() { 2317 if (this.contact == null) 2318 return false; 2319 for (ContactDetail item : this.contact) 2320 if (!item.isEmpty()) 2321 return true; 2322 return false; 2323 } 2324 2325 public ContactDetail addContact() { // 3 2326 ContactDetail t = new ContactDetail(); 2327 if (this.contact == null) 2328 this.contact = new ArrayList<ContactDetail>(); 2329 this.contact.add(t); 2330 return t; 2331 } 2332 2333 public ChargeItemDefinition addContact(ContactDetail t) { // 3 2334 if (t == null) 2335 return this; 2336 if (this.contact == null) 2337 this.contact = new ArrayList<ContactDetail>(); 2338 this.contact.add(t); 2339 return this; 2340 } 2341 2342 /** 2343 * @return The first repetition of repeating field {@link #contact}, creating it 2344 * if it does not already exist 2345 */ 2346 public ContactDetail getContactFirstRep() { 2347 if (getContact().isEmpty()) { 2348 addContact(); 2349 } 2350 return getContact().get(0); 2351 } 2352 2353 /** 2354 * @return {@link #description} (A free text natural language description of the 2355 * charge item definition from a consumer's perspective.). This is the 2356 * underlying object with id, value and extensions. The accessor 2357 * "getDescription" gives direct access to the value 2358 */ 2359 public MarkdownType getDescriptionElement() { 2360 if (this.description == null) 2361 if (Configuration.errorOnAutoCreate()) 2362 throw new Error("Attempt to auto-create ChargeItemDefinition.description"); 2363 else if (Configuration.doAutoCreate()) 2364 this.description = new MarkdownType(); // bb 2365 return this.description; 2366 } 2367 2368 public boolean hasDescriptionElement() { 2369 return this.description != null && !this.description.isEmpty(); 2370 } 2371 2372 public boolean hasDescription() { 2373 return this.description != null && !this.description.isEmpty(); 2374 } 2375 2376 /** 2377 * @param value {@link #description} (A free text natural language description 2378 * of the charge item definition from a consumer's perspective.). 2379 * This is the underlying object with id, value and extensions. The 2380 * accessor "getDescription" gives direct access to the value 2381 */ 2382 public ChargeItemDefinition setDescriptionElement(MarkdownType value) { 2383 this.description = value; 2384 return this; 2385 } 2386 2387 /** 2388 * @return A free text natural language description of the charge item 2389 * definition from a consumer's perspective. 2390 */ 2391 public String getDescription() { 2392 return this.description == null ? null : this.description.getValue(); 2393 } 2394 2395 /** 2396 * @param value A free text natural language description of the charge item 2397 * definition from a consumer's perspective. 2398 */ 2399 public ChargeItemDefinition setDescription(String value) { 2400 if (value == null) 2401 this.description = null; 2402 else { 2403 if (this.description == null) 2404 this.description = new MarkdownType(); 2405 this.description.setValue(value); 2406 } 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #useContext} (The content was developed with a focus and 2412 * intent of supporting the contexts that are listed. These contexts may 2413 * be general categories (gender, age, ...) or may be references to 2414 * specific programs (insurance plans, studies, ...) and may be used to 2415 * assist with indexing and searching for appropriate charge item 2416 * definition instances.) 2417 */ 2418 public List<UsageContext> getUseContext() { 2419 if (this.useContext == null) 2420 this.useContext = new ArrayList<UsageContext>(); 2421 return this.useContext; 2422 } 2423 2424 /** 2425 * @return Returns a reference to <code>this</code> for easy method chaining 2426 */ 2427 public ChargeItemDefinition setUseContext(List<UsageContext> theUseContext) { 2428 this.useContext = theUseContext; 2429 return this; 2430 } 2431 2432 public boolean hasUseContext() { 2433 if (this.useContext == null) 2434 return false; 2435 for (UsageContext item : this.useContext) 2436 if (!item.isEmpty()) 2437 return true; 2438 return false; 2439 } 2440 2441 public UsageContext addUseContext() { // 3 2442 UsageContext t = new UsageContext(); 2443 if (this.useContext == null) 2444 this.useContext = new ArrayList<UsageContext>(); 2445 this.useContext.add(t); 2446 return t; 2447 } 2448 2449 public ChargeItemDefinition addUseContext(UsageContext t) { // 3 2450 if (t == null) 2451 return this; 2452 if (this.useContext == null) 2453 this.useContext = new ArrayList<UsageContext>(); 2454 this.useContext.add(t); 2455 return this; 2456 } 2457 2458 /** 2459 * @return The first repetition of repeating field {@link #useContext}, creating 2460 * it if it does not already exist 2461 */ 2462 public UsageContext getUseContextFirstRep() { 2463 if (getUseContext().isEmpty()) { 2464 addUseContext(); 2465 } 2466 return getUseContext().get(0); 2467 } 2468 2469 /** 2470 * @return {@link #jurisdiction} (A legal or geographic region in which the 2471 * charge item definition is intended to be used.) 2472 */ 2473 public List<CodeableConcept> getJurisdiction() { 2474 if (this.jurisdiction == null) 2475 this.jurisdiction = new ArrayList<CodeableConcept>(); 2476 return this.jurisdiction; 2477 } 2478 2479 /** 2480 * @return Returns a reference to <code>this</code> for easy method chaining 2481 */ 2482 public ChargeItemDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2483 this.jurisdiction = theJurisdiction; 2484 return this; 2485 } 2486 2487 public boolean hasJurisdiction() { 2488 if (this.jurisdiction == null) 2489 return false; 2490 for (CodeableConcept item : this.jurisdiction) 2491 if (!item.isEmpty()) 2492 return true; 2493 return false; 2494 } 2495 2496 public CodeableConcept addJurisdiction() { // 3 2497 CodeableConcept t = new CodeableConcept(); 2498 if (this.jurisdiction == null) 2499 this.jurisdiction = new ArrayList<CodeableConcept>(); 2500 this.jurisdiction.add(t); 2501 return t; 2502 } 2503 2504 public ChargeItemDefinition addJurisdiction(CodeableConcept t) { // 3 2505 if (t == null) 2506 return this; 2507 if (this.jurisdiction == null) 2508 this.jurisdiction = new ArrayList<CodeableConcept>(); 2509 this.jurisdiction.add(t); 2510 return this; 2511 } 2512 2513 /** 2514 * @return The first repetition of repeating field {@link #jurisdiction}, 2515 * creating it if it does not already exist 2516 */ 2517 public CodeableConcept getJurisdictionFirstRep() { 2518 if (getJurisdiction().isEmpty()) { 2519 addJurisdiction(); 2520 } 2521 return getJurisdiction().get(0); 2522 } 2523 2524 /** 2525 * @return {@link #copyright} (A copyright statement relating to the charge item 2526 * definition and/or its contents. Copyright statements are generally 2527 * legal restrictions on the use and publishing of the charge item 2528 * definition.). This is the underlying object with id, value and 2529 * extensions. The accessor "getCopyright" gives direct access to the 2530 * value 2531 */ 2532 public MarkdownType getCopyrightElement() { 2533 if (this.copyright == null) 2534 if (Configuration.errorOnAutoCreate()) 2535 throw new Error("Attempt to auto-create ChargeItemDefinition.copyright"); 2536 else if (Configuration.doAutoCreate()) 2537 this.copyright = new MarkdownType(); // bb 2538 return this.copyright; 2539 } 2540 2541 public boolean hasCopyrightElement() { 2542 return this.copyright != null && !this.copyright.isEmpty(); 2543 } 2544 2545 public boolean hasCopyright() { 2546 return this.copyright != null && !this.copyright.isEmpty(); 2547 } 2548 2549 /** 2550 * @param value {@link #copyright} (A copyright statement relating to the charge 2551 * item definition and/or its contents. Copyright statements are 2552 * generally legal restrictions on the use and publishing of the 2553 * charge item definition.). This is the underlying object with id, 2554 * value and extensions. The accessor "getCopyright" gives direct 2555 * access to the value 2556 */ 2557 public ChargeItemDefinition setCopyrightElement(MarkdownType value) { 2558 this.copyright = value; 2559 return this; 2560 } 2561 2562 /** 2563 * @return A copyright statement relating to the charge item definition and/or 2564 * its contents. Copyright statements are generally legal restrictions 2565 * on the use and publishing of the charge item definition. 2566 */ 2567 public String getCopyright() { 2568 return this.copyright == null ? null : this.copyright.getValue(); 2569 } 2570 2571 /** 2572 * @param value A copyright statement relating to the charge item definition 2573 * and/or its contents. Copyright statements are generally legal 2574 * restrictions on the use and publishing of the charge item 2575 * definition. 2576 */ 2577 public ChargeItemDefinition setCopyright(String value) { 2578 if (value == null) 2579 this.copyright = null; 2580 else { 2581 if (this.copyright == null) 2582 this.copyright = new MarkdownType(); 2583 this.copyright.setValue(value); 2584 } 2585 return this; 2586 } 2587 2588 /** 2589 * @return {@link #approvalDate} (The date on which the resource content was 2590 * approved by the publisher. Approval happens once when the content is 2591 * officially approved for usage.). This is the underlying object with 2592 * id, value and extensions. The accessor "getApprovalDate" gives direct 2593 * access to the value 2594 */ 2595 public DateType getApprovalDateElement() { 2596 if (this.approvalDate == null) 2597 if (Configuration.errorOnAutoCreate()) 2598 throw new Error("Attempt to auto-create ChargeItemDefinition.approvalDate"); 2599 else if (Configuration.doAutoCreate()) 2600 this.approvalDate = new DateType(); // bb 2601 return this.approvalDate; 2602 } 2603 2604 public boolean hasApprovalDateElement() { 2605 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2606 } 2607 2608 public boolean hasApprovalDate() { 2609 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2610 } 2611 2612 /** 2613 * @param value {@link #approvalDate} (The date on which the resource content 2614 * was approved by the publisher. Approval happens once when the 2615 * content is officially approved for usage.). This is the 2616 * underlying object with id, value and extensions. The accessor 2617 * "getApprovalDate" gives direct access to the value 2618 */ 2619 public ChargeItemDefinition setApprovalDateElement(DateType value) { 2620 this.approvalDate = value; 2621 return this; 2622 } 2623 2624 /** 2625 * @return The date on which the resource content was approved by the publisher. 2626 * Approval happens once when the content is officially approved for 2627 * usage. 2628 */ 2629 public Date getApprovalDate() { 2630 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2631 } 2632 2633 /** 2634 * @param value The date on which the resource content was approved by the 2635 * publisher. Approval happens once when the content is officially 2636 * approved for usage. 2637 */ 2638 public ChargeItemDefinition setApprovalDate(Date value) { 2639 if (value == null) 2640 this.approvalDate = null; 2641 else { 2642 if (this.approvalDate == null) 2643 this.approvalDate = new DateType(); 2644 this.approvalDate.setValue(value); 2645 } 2646 return this; 2647 } 2648 2649 /** 2650 * @return {@link #lastReviewDate} (The date on which the resource content was 2651 * last reviewed. Review happens periodically after approval but does 2652 * not change the original approval date.). This is the underlying 2653 * object with id, value and extensions. The accessor 2654 * "getLastReviewDate" gives direct access to the value 2655 */ 2656 public DateType getLastReviewDateElement() { 2657 if (this.lastReviewDate == null) 2658 if (Configuration.errorOnAutoCreate()) 2659 throw new Error("Attempt to auto-create ChargeItemDefinition.lastReviewDate"); 2660 else if (Configuration.doAutoCreate()) 2661 this.lastReviewDate = new DateType(); // bb 2662 return this.lastReviewDate; 2663 } 2664 2665 public boolean hasLastReviewDateElement() { 2666 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2667 } 2668 2669 public boolean hasLastReviewDate() { 2670 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2671 } 2672 2673 /** 2674 * @param value {@link #lastReviewDate} (The date on which the resource content 2675 * was last reviewed. Review happens periodically after approval 2676 * but does not change the original approval date.). This is the 2677 * underlying object with id, value and extensions. The accessor 2678 * "getLastReviewDate" gives direct access to the value 2679 */ 2680 public ChargeItemDefinition setLastReviewDateElement(DateType value) { 2681 this.lastReviewDate = value; 2682 return this; 2683 } 2684 2685 /** 2686 * @return The date on which the resource content was last reviewed. Review 2687 * happens periodically after approval but does not change the original 2688 * approval date. 2689 */ 2690 public Date getLastReviewDate() { 2691 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2692 } 2693 2694 /** 2695 * @param value The date on which the resource content was last reviewed. Review 2696 * happens periodically after approval but does not change the 2697 * original approval date. 2698 */ 2699 public ChargeItemDefinition setLastReviewDate(Date value) { 2700 if (value == null) 2701 this.lastReviewDate = null; 2702 else { 2703 if (this.lastReviewDate == null) 2704 this.lastReviewDate = new DateType(); 2705 this.lastReviewDate.setValue(value); 2706 } 2707 return this; 2708 } 2709 2710 /** 2711 * @return {@link #effectivePeriod} (The period during which the charge item 2712 * definition content was or is planned to be in active use.) 2713 */ 2714 public Period getEffectivePeriod() { 2715 if (this.effectivePeriod == null) 2716 if (Configuration.errorOnAutoCreate()) 2717 throw new Error("Attempt to auto-create ChargeItemDefinition.effectivePeriod"); 2718 else if (Configuration.doAutoCreate()) 2719 this.effectivePeriod = new Period(); // cc 2720 return this.effectivePeriod; 2721 } 2722 2723 public boolean hasEffectivePeriod() { 2724 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #effectivePeriod} (The period during which the charge 2729 * item definition content was or is planned to be in active use.) 2730 */ 2731 public ChargeItemDefinition setEffectivePeriod(Period value) { 2732 this.effectivePeriod = value; 2733 return this; 2734 } 2735 2736 /** 2737 * @return {@link #code} (The defined billing details in this resource pertain 2738 * to the given billing code.) 2739 */ 2740 public CodeableConcept getCode() { 2741 if (this.code == null) 2742 if (Configuration.errorOnAutoCreate()) 2743 throw new Error("Attempt to auto-create ChargeItemDefinition.code"); 2744 else if (Configuration.doAutoCreate()) 2745 this.code = new CodeableConcept(); // cc 2746 return this.code; 2747 } 2748 2749 public boolean hasCode() { 2750 return this.code != null && !this.code.isEmpty(); 2751 } 2752 2753 /** 2754 * @param value {@link #code} (The defined billing details in this resource 2755 * pertain to the given billing code.) 2756 */ 2757 public ChargeItemDefinition setCode(CodeableConcept value) { 2758 this.code = value; 2759 return this; 2760 } 2761 2762 /** 2763 * @return {@link #instance} (The defined billing details in this resource 2764 * pertain to the given product instance(s).) 2765 */ 2766 public List<Reference> getInstance() { 2767 if (this.instance == null) 2768 this.instance = new ArrayList<Reference>(); 2769 return this.instance; 2770 } 2771 2772 /** 2773 * @return Returns a reference to <code>this</code> for easy method chaining 2774 */ 2775 public ChargeItemDefinition setInstance(List<Reference> theInstance) { 2776 this.instance = theInstance; 2777 return this; 2778 } 2779 2780 public boolean hasInstance() { 2781 if (this.instance == null) 2782 return false; 2783 for (Reference item : this.instance) 2784 if (!item.isEmpty()) 2785 return true; 2786 return false; 2787 } 2788 2789 public Reference addInstance() { // 3 2790 Reference t = new Reference(); 2791 if (this.instance == null) 2792 this.instance = new ArrayList<Reference>(); 2793 this.instance.add(t); 2794 return t; 2795 } 2796 2797 public ChargeItemDefinition addInstance(Reference t) { // 3 2798 if (t == null) 2799 return this; 2800 if (this.instance == null) 2801 this.instance = new ArrayList<Reference>(); 2802 this.instance.add(t); 2803 return this; 2804 } 2805 2806 /** 2807 * @return The first repetition of repeating field {@link #instance}, creating 2808 * it if it does not already exist 2809 */ 2810 public Reference getInstanceFirstRep() { 2811 if (getInstance().isEmpty()) { 2812 addInstance(); 2813 } 2814 return getInstance().get(0); 2815 } 2816 2817 /** 2818 * @deprecated Use Reference#setResource(IBaseResource) instead 2819 */ 2820 @Deprecated 2821 public List<Resource> getInstanceTarget() { 2822 if (this.instanceTarget == null) 2823 this.instanceTarget = new ArrayList<Resource>(); 2824 return this.instanceTarget; 2825 } 2826 2827 /** 2828 * @return {@link #applicability} (Expressions that describe applicability 2829 * criteria for the billing code.) 2830 */ 2831 public List<ChargeItemDefinitionApplicabilityComponent> getApplicability() { 2832 if (this.applicability == null) 2833 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2834 return this.applicability; 2835 } 2836 2837 /** 2838 * @return Returns a reference to <code>this</code> for easy method chaining 2839 */ 2840 public ChargeItemDefinition setApplicability(List<ChargeItemDefinitionApplicabilityComponent> theApplicability) { 2841 this.applicability = theApplicability; 2842 return this; 2843 } 2844 2845 public boolean hasApplicability() { 2846 if (this.applicability == null) 2847 return false; 2848 for (ChargeItemDefinitionApplicabilityComponent item : this.applicability) 2849 if (!item.isEmpty()) 2850 return true; 2851 return false; 2852 } 2853 2854 public ChargeItemDefinitionApplicabilityComponent addApplicability() { // 3 2855 ChargeItemDefinitionApplicabilityComponent t = new ChargeItemDefinitionApplicabilityComponent(); 2856 if (this.applicability == null) 2857 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2858 this.applicability.add(t); 2859 return t; 2860 } 2861 2862 public ChargeItemDefinition addApplicability(ChargeItemDefinitionApplicabilityComponent t) { // 3 2863 if (t == null) 2864 return this; 2865 if (this.applicability == null) 2866 this.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 2867 this.applicability.add(t); 2868 return this; 2869 } 2870 2871 /** 2872 * @return The first repetition of repeating field {@link #applicability}, 2873 * creating it if it does not already exist 2874 */ 2875 public ChargeItemDefinitionApplicabilityComponent getApplicabilityFirstRep() { 2876 if (getApplicability().isEmpty()) { 2877 addApplicability(); 2878 } 2879 return getApplicability().get(0); 2880 } 2881 2882 /** 2883 * @return {@link #propertyGroup} (Group of properties which are applicable 2884 * under the same conditions. If no applicability rules are established 2885 * for the group, then all properties always apply.) 2886 */ 2887 public List<ChargeItemDefinitionPropertyGroupComponent> getPropertyGroup() { 2888 if (this.propertyGroup == null) 2889 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2890 return this.propertyGroup; 2891 } 2892 2893 /** 2894 * @return Returns a reference to <code>this</code> for easy method chaining 2895 */ 2896 public ChargeItemDefinition setPropertyGroup(List<ChargeItemDefinitionPropertyGroupComponent> thePropertyGroup) { 2897 this.propertyGroup = thePropertyGroup; 2898 return this; 2899 } 2900 2901 public boolean hasPropertyGroup() { 2902 if (this.propertyGroup == null) 2903 return false; 2904 for (ChargeItemDefinitionPropertyGroupComponent item : this.propertyGroup) 2905 if (!item.isEmpty()) 2906 return true; 2907 return false; 2908 } 2909 2910 public ChargeItemDefinitionPropertyGroupComponent addPropertyGroup() { // 3 2911 ChargeItemDefinitionPropertyGroupComponent t = new ChargeItemDefinitionPropertyGroupComponent(); 2912 if (this.propertyGroup == null) 2913 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2914 this.propertyGroup.add(t); 2915 return t; 2916 } 2917 2918 public ChargeItemDefinition addPropertyGroup(ChargeItemDefinitionPropertyGroupComponent t) { // 3 2919 if (t == null) 2920 return this; 2921 if (this.propertyGroup == null) 2922 this.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 2923 this.propertyGroup.add(t); 2924 return this; 2925 } 2926 2927 /** 2928 * @return The first repetition of repeating field {@link #propertyGroup}, 2929 * creating it if it does not already exist 2930 */ 2931 public ChargeItemDefinitionPropertyGroupComponent getPropertyGroupFirstRep() { 2932 if (getPropertyGroup().isEmpty()) { 2933 addPropertyGroup(); 2934 } 2935 return getPropertyGroup().get(0); 2936 } 2937 2938 protected void listChildren(List<Property> children) { 2939 super.listChildren(children); 2940 children.add(new Property("url", "uri", 2941 "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 2942 0, 1, url)); 2943 children.add(new Property("identifier", "Identifier", 2944 "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2945 0, java.lang.Integer.MAX_VALUE, identifier)); 2946 children.add(new Property("version", "string", 2947 "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 2948 0, 1, version)); 2949 children.add(new Property("title", "string", 2950 "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title)); 2951 children.add(new Property("derivedFromUri", "uri", 2952 "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 2953 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 2954 children.add(new Property("partOf", "canonical(ChargeItemDefinition)", 2955 "A larger definition of which this particular definition is a component or step.", 0, 2956 java.lang.Integer.MAX_VALUE, partOf)); 2957 children.add(new Property("replaces", "canonical(ChargeItemDefinition)", 2958 "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 2959 0, java.lang.Integer.MAX_VALUE, replaces)); 2960 children.add(new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, status)); 2961 children.add(new Property("experimental", "boolean", 2962 "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2963 0, 1, experimental)); 2964 children.add(new Property("date", "dateTime", 2965 "The date (and optionally time) when the charge item definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 2966 0, 1, date)); 2967 children.add(new Property("publisher", "string", 2968 "The name of the organization or individual that published the charge item definition.", 0, 1, publisher)); 2969 children.add(new Property("contact", "ContactDetail", 2970 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2971 java.lang.Integer.MAX_VALUE, contact)); 2972 children.add(new Property("description", "markdown", 2973 "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, 2974 description)); 2975 children.add(new Property("useContext", "UsageContext", 2976 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 2977 0, java.lang.Integer.MAX_VALUE, useContext)); 2978 children.add(new Property("jurisdiction", "CodeableConcept", 2979 "A legal or geographic region in which the charge item definition is intended to be used.", 0, 2980 java.lang.Integer.MAX_VALUE, jurisdiction)); 2981 children.add(new Property("copyright", "markdown", 2982 "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 2983 0, 1, copyright)); 2984 children.add(new Property("approvalDate", "date", 2985 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2986 0, 1, approvalDate)); 2987 children.add(new Property("lastReviewDate", "date", 2988 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2989 0, 1, lastReviewDate)); 2990 children.add(new Property("effectivePeriod", "Period", 2991 "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, 2992 effectivePeriod)); 2993 children.add(new Property("code", "CodeableConcept", 2994 "The defined billing details in this resource pertain to the given billing code.", 0, 1, code)); 2995 children.add(new Property("instance", "Reference(Medication|Substance|Device)", 2996 "The defined billing details in this resource pertain to the given product instance(s).", 0, 2997 java.lang.Integer.MAX_VALUE, instance)); 2998 children 2999 .add(new Property("applicability", "", "Expressions that describe applicability criteria for the billing code.", 3000 0, java.lang.Integer.MAX_VALUE, applicability)); 3001 children.add(new Property("propertyGroup", "", 3002 "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 3003 0, java.lang.Integer.MAX_VALUE, propertyGroup)); 3004 } 3005 3006 @Override 3007 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3008 switch (_hash) { 3009 case 116079: 3010 /* url */ return new Property("url", "uri", 3011 "An absolute URI that is used to identify this charge item definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this charge item definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the charge item definition is stored on different servers.", 3012 0, 1, url); 3013 case -1618432855: 3014 /* identifier */ return new Property("identifier", "Identifier", 3015 "A formal identifier that is used to identify this charge item definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3016 0, java.lang.Integer.MAX_VALUE, identifier); 3017 case 351608024: 3018 /* version */ return new Property("version", "string", 3019 "The identifier that is used to identify this version of the charge item definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the charge item definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active assets.", 3020 0, 1, version); 3021 case 110371416: 3022 /* title */ return new Property("title", "string", 3023 "A short, descriptive, user-friendly title for the charge item definition.", 0, 1, title); 3024 case -1076333435: 3025 /* derivedFromUri */ return new Property("derivedFromUri", "uri", 3026 "The URL pointing to an externally-defined charge item definition that is adhered to in whole or in part by this definition.", 3027 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 3028 case -995410646: 3029 /* partOf */ return new Property("partOf", "canonical(ChargeItemDefinition)", 3030 "A larger definition of which this particular definition is a component or step.", 0, 3031 java.lang.Integer.MAX_VALUE, partOf); 3032 case -430332865: 3033 /* replaces */ return new Property("replaces", "canonical(ChargeItemDefinition)", 3034 "As new versions of a protocol or guideline are defined, allows identification of what versions are replaced by a new instance.", 3035 0, java.lang.Integer.MAX_VALUE, replaces); 3036 case -892481550: 3037 /* status */ return new Property("status", "code", "The current state of the ChargeItemDefinition.", 0, 1, 3038 status); 3039 case -404562712: 3040 /* experimental */ return new Property("experimental", "boolean", 3041 "A Boolean value to indicate that this charge item definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3042 0, 1, experimental); 3043 case 3076014: 3044 /* date */ return new Property("date", "dateTime", 3045 "The date (and optionally time) when the charge item definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the charge item definition changes.", 3046 0, 1, date); 3047 case 1447404028: 3048 /* publisher */ return new Property("publisher", "string", 3049 "The name of the organization or individual that published the charge item definition.", 0, 1, publisher); 3050 case 951526432: 3051 /* contact */ return new Property("contact", "ContactDetail", 3052 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3053 java.lang.Integer.MAX_VALUE, contact); 3054 case -1724546052: 3055 /* description */ return new Property("description", "markdown", 3056 "A free text natural language description of the charge item definition from a consumer's perspective.", 0, 1, 3057 description); 3058 case -669707736: 3059 /* useContext */ return new Property("useContext", "UsageContext", 3060 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate charge item definition instances.", 3061 0, java.lang.Integer.MAX_VALUE, useContext); 3062 case -507075711: 3063 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3064 "A legal or geographic region in which the charge item definition is intended to be used.", 0, 3065 java.lang.Integer.MAX_VALUE, jurisdiction); 3066 case 1522889671: 3067 /* copyright */ return new Property("copyright", "markdown", 3068 "A copyright statement relating to the charge item definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the charge item definition.", 3069 0, 1, copyright); 3070 case 223539345: 3071 /* approvalDate */ return new Property("approvalDate", "date", 3072 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3073 0, 1, approvalDate); 3074 case -1687512484: 3075 /* lastReviewDate */ return new Property("lastReviewDate", "date", 3076 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3077 0, 1, lastReviewDate); 3078 case -403934648: 3079 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 3080 "The period during which the charge item definition content was or is planned to be in active use.", 0, 1, 3081 effectivePeriod); 3082 case 3059181: 3083 /* code */ return new Property("code", "CodeableConcept", 3084 "The defined billing details in this resource pertain to the given billing code.", 0, 1, code); 3085 case 555127957: 3086 /* instance */ return new Property("instance", "Reference(Medication|Substance|Device)", 3087 "The defined billing details in this resource pertain to the given product instance(s).", 0, 3088 java.lang.Integer.MAX_VALUE, instance); 3089 case -1526770491: 3090 /* applicability */ return new Property("applicability", "", 3091 "Expressions that describe applicability criteria for the billing code.", 0, java.lang.Integer.MAX_VALUE, 3092 applicability); 3093 case -1041594966: 3094 /* propertyGroup */ return new Property("propertyGroup", "", 3095 "Group of properties which are applicable under the same conditions. If no applicability rules are established for the group, then all properties always apply.", 3096 0, java.lang.Integer.MAX_VALUE, propertyGroup); 3097 default: 3098 return super.getNamedProperty(_hash, _name, _checkValid); 3099 } 3100 3101 } 3102 3103 @Override 3104 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3105 switch (hash) { 3106 case 116079: 3107 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3108 case -1618432855: 3109 /* identifier */ return this.identifier == null ? new Base[0] 3110 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3111 case 351608024: 3112 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3113 case 110371416: 3114 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3115 case -1076333435: 3116 /* derivedFromUri */ return this.derivedFromUri == null ? new Base[0] 3117 : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 3118 case -995410646: 3119 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // CanonicalType 3120 case -430332865: 3121 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 3122 case -892481550: 3123 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3124 case -404562712: 3125 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3126 case 3076014: 3127 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3128 case 1447404028: 3129 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3130 case 951526432: 3131 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3132 case -1724546052: 3133 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3134 case -669707736: 3135 /* useContext */ return this.useContext == null ? new Base[0] 3136 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3137 case -507075711: 3138 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3139 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3140 case 1522889671: 3141 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 3142 case 223539345: 3143 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 3144 case -1687512484: 3145 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 3146 case -403934648: 3147 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 3148 case 3059181: 3149 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3150 case 555127957: 3151 /* instance */ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // Reference 3152 case -1526770491: 3153 /* applicability */ return this.applicability == null ? new Base[0] 3154 : this.applicability.toArray(new Base[this.applicability.size()]); // ChargeItemDefinitionApplicabilityComponent 3155 case -1041594966: 3156 /* propertyGroup */ return this.propertyGroup == null ? new Base[0] 3157 : this.propertyGroup.toArray(new Base[this.propertyGroup.size()]); // ChargeItemDefinitionPropertyGroupComponent 3158 default: 3159 return super.getProperty(hash, name, checkValid); 3160 } 3161 3162 } 3163 3164 @Override 3165 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3166 switch (hash) { 3167 case 116079: // url 3168 this.url = castToUri(value); // UriType 3169 return value; 3170 case -1618432855: // identifier 3171 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3172 return value; 3173 case 351608024: // version 3174 this.version = castToString(value); // StringType 3175 return value; 3176 case 110371416: // title 3177 this.title = castToString(value); // StringType 3178 return value; 3179 case -1076333435: // derivedFromUri 3180 this.getDerivedFromUri().add(castToUri(value)); // UriType 3181 return value; 3182 case -995410646: // partOf 3183 this.getPartOf().add(castToCanonical(value)); // CanonicalType 3184 return value; 3185 case -430332865: // replaces 3186 this.getReplaces().add(castToCanonical(value)); // CanonicalType 3187 return value; 3188 case -892481550: // status 3189 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3190 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3191 return value; 3192 case -404562712: // experimental 3193 this.experimental = castToBoolean(value); // BooleanType 3194 return value; 3195 case 3076014: // date 3196 this.date = castToDateTime(value); // DateTimeType 3197 return value; 3198 case 1447404028: // publisher 3199 this.publisher = castToString(value); // StringType 3200 return value; 3201 case 951526432: // contact 3202 this.getContact().add(castToContactDetail(value)); // ContactDetail 3203 return value; 3204 case -1724546052: // description 3205 this.description = castToMarkdown(value); // MarkdownType 3206 return value; 3207 case -669707736: // useContext 3208 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3209 return value; 3210 case -507075711: // jurisdiction 3211 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3212 return value; 3213 case 1522889671: // copyright 3214 this.copyright = castToMarkdown(value); // MarkdownType 3215 return value; 3216 case 223539345: // approvalDate 3217 this.approvalDate = castToDate(value); // DateType 3218 return value; 3219 case -1687512484: // lastReviewDate 3220 this.lastReviewDate = castToDate(value); // DateType 3221 return value; 3222 case -403934648: // effectivePeriod 3223 this.effectivePeriod = castToPeriod(value); // Period 3224 return value; 3225 case 3059181: // code 3226 this.code = castToCodeableConcept(value); // CodeableConcept 3227 return value; 3228 case 555127957: // instance 3229 this.getInstance().add(castToReference(value)); // Reference 3230 return value; 3231 case -1526770491: // applicability 3232 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); // ChargeItemDefinitionApplicabilityComponent 3233 return value; 3234 case -1041594966: // propertyGroup 3235 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); // ChargeItemDefinitionPropertyGroupComponent 3236 return value; 3237 default: 3238 return super.setProperty(hash, name, value); 3239 } 3240 3241 } 3242 3243 @Override 3244 public Base setProperty(String name, Base value) throws FHIRException { 3245 if (name.equals("url")) { 3246 this.url = castToUri(value); // UriType 3247 } else if (name.equals("identifier")) { 3248 this.getIdentifier().add(castToIdentifier(value)); 3249 } else if (name.equals("version")) { 3250 this.version = castToString(value); // StringType 3251 } else if (name.equals("title")) { 3252 this.title = castToString(value); // StringType 3253 } else if (name.equals("derivedFromUri")) { 3254 this.getDerivedFromUri().add(castToUri(value)); 3255 } else if (name.equals("partOf")) { 3256 this.getPartOf().add(castToCanonical(value)); 3257 } else if (name.equals("replaces")) { 3258 this.getReplaces().add(castToCanonical(value)); 3259 } else if (name.equals("status")) { 3260 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3261 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3262 } else if (name.equals("experimental")) { 3263 this.experimental = castToBoolean(value); // BooleanType 3264 } else if (name.equals("date")) { 3265 this.date = castToDateTime(value); // DateTimeType 3266 } else if (name.equals("publisher")) { 3267 this.publisher = castToString(value); // StringType 3268 } else if (name.equals("contact")) { 3269 this.getContact().add(castToContactDetail(value)); 3270 } else if (name.equals("description")) { 3271 this.description = castToMarkdown(value); // MarkdownType 3272 } else if (name.equals("useContext")) { 3273 this.getUseContext().add(castToUsageContext(value)); 3274 } else if (name.equals("jurisdiction")) { 3275 this.getJurisdiction().add(castToCodeableConcept(value)); 3276 } else if (name.equals("copyright")) { 3277 this.copyright = castToMarkdown(value); // MarkdownType 3278 } else if (name.equals("approvalDate")) { 3279 this.approvalDate = castToDate(value); // DateType 3280 } else if (name.equals("lastReviewDate")) { 3281 this.lastReviewDate = castToDate(value); // DateType 3282 } else if (name.equals("effectivePeriod")) { 3283 this.effectivePeriod = castToPeriod(value); // Period 3284 } else if (name.equals("code")) { 3285 this.code = castToCodeableConcept(value); // CodeableConcept 3286 } else if (name.equals("instance")) { 3287 this.getInstance().add(castToReference(value)); 3288 } else if (name.equals("applicability")) { 3289 this.getApplicability().add((ChargeItemDefinitionApplicabilityComponent) value); 3290 } else if (name.equals("propertyGroup")) { 3291 this.getPropertyGroup().add((ChargeItemDefinitionPropertyGroupComponent) value); 3292 } else 3293 return super.setProperty(name, value); 3294 return value; 3295 } 3296 3297 @Override 3298 public void removeChild(String name, Base value) throws FHIRException { 3299 if (name.equals("url")) { 3300 this.url = null; 3301 } else if (name.equals("identifier")) { 3302 this.getIdentifier().remove(castToIdentifier(value)); 3303 } else if (name.equals("version")) { 3304 this.version = null; 3305 } else if (name.equals("title")) { 3306 this.title = null; 3307 } else if (name.equals("derivedFromUri")) { 3308 this.getDerivedFromUri().remove(castToUri(value)); 3309 } else if (name.equals("partOf")) { 3310 this.getPartOf().remove(castToCanonical(value)); 3311 } else if (name.equals("replaces")) { 3312 this.getReplaces().remove(castToCanonical(value)); 3313 } else if (name.equals("status")) { 3314 this.status = null; 3315 } else if (name.equals("experimental")) { 3316 this.experimental = null; 3317 } else if (name.equals("date")) { 3318 this.date = null; 3319 } else if (name.equals("publisher")) { 3320 this.publisher = null; 3321 } else if (name.equals("contact")) { 3322 this.getContact().remove(castToContactDetail(value)); 3323 } else if (name.equals("description")) { 3324 this.description = null; 3325 } else if (name.equals("useContext")) { 3326 this.getUseContext().remove(castToUsageContext(value)); 3327 } else if (name.equals("jurisdiction")) { 3328 this.getJurisdiction().remove(castToCodeableConcept(value)); 3329 } else if (name.equals("copyright")) { 3330 this.copyright = null; 3331 } else if (name.equals("approvalDate")) { 3332 this.approvalDate = null; 3333 } else if (name.equals("lastReviewDate")) { 3334 this.lastReviewDate = null; 3335 } else if (name.equals("effectivePeriod")) { 3336 this.effectivePeriod = null; 3337 } else if (name.equals("code")) { 3338 this.code = null; 3339 } else if (name.equals("instance")) { 3340 this.getInstance().remove(castToReference(value)); 3341 } else if (name.equals("applicability")) { 3342 this.getApplicability().remove((ChargeItemDefinitionApplicabilityComponent) value); 3343 } else if (name.equals("propertyGroup")) { 3344 this.getPropertyGroup().remove((ChargeItemDefinitionPropertyGroupComponent) value); 3345 } else 3346 super.removeChild(name, value); 3347 3348 } 3349 3350 @Override 3351 public Base makeProperty(int hash, String name) throws FHIRException { 3352 switch (hash) { 3353 case 116079: 3354 return getUrlElement(); 3355 case -1618432855: 3356 return addIdentifier(); 3357 case 351608024: 3358 return getVersionElement(); 3359 case 110371416: 3360 return getTitleElement(); 3361 case -1076333435: 3362 return addDerivedFromUriElement(); 3363 case -995410646: 3364 return addPartOfElement(); 3365 case -430332865: 3366 return addReplacesElement(); 3367 case -892481550: 3368 return getStatusElement(); 3369 case -404562712: 3370 return getExperimentalElement(); 3371 case 3076014: 3372 return getDateElement(); 3373 case 1447404028: 3374 return getPublisherElement(); 3375 case 951526432: 3376 return addContact(); 3377 case -1724546052: 3378 return getDescriptionElement(); 3379 case -669707736: 3380 return addUseContext(); 3381 case -507075711: 3382 return addJurisdiction(); 3383 case 1522889671: 3384 return getCopyrightElement(); 3385 case 223539345: 3386 return getApprovalDateElement(); 3387 case -1687512484: 3388 return getLastReviewDateElement(); 3389 case -403934648: 3390 return getEffectivePeriod(); 3391 case 3059181: 3392 return getCode(); 3393 case 555127957: 3394 return addInstance(); 3395 case -1526770491: 3396 return addApplicability(); 3397 case -1041594966: 3398 return addPropertyGroup(); 3399 default: 3400 return super.makeProperty(hash, name); 3401 } 3402 3403 } 3404 3405 @Override 3406 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3407 switch (hash) { 3408 case 116079: 3409 /* url */ return new String[] { "uri" }; 3410 case -1618432855: 3411 /* identifier */ return new String[] { "Identifier" }; 3412 case 351608024: 3413 /* version */ return new String[] { "string" }; 3414 case 110371416: 3415 /* title */ return new String[] { "string" }; 3416 case -1076333435: 3417 /* derivedFromUri */ return new String[] { "uri" }; 3418 case -995410646: 3419 /* partOf */ return new String[] { "canonical" }; 3420 case -430332865: 3421 /* replaces */ return new String[] { "canonical" }; 3422 case -892481550: 3423 /* status */ return new String[] { "code" }; 3424 case -404562712: 3425 /* experimental */ return new String[] { "boolean" }; 3426 case 3076014: 3427 /* date */ return new String[] { "dateTime" }; 3428 case 1447404028: 3429 /* publisher */ return new String[] { "string" }; 3430 case 951526432: 3431 /* contact */ return new String[] { "ContactDetail" }; 3432 case -1724546052: 3433 /* description */ return new String[] { "markdown" }; 3434 case -669707736: 3435 /* useContext */ return new String[] { "UsageContext" }; 3436 case -507075711: 3437 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3438 case 1522889671: 3439 /* copyright */ return new String[] { "markdown" }; 3440 case 223539345: 3441 /* approvalDate */ return new String[] { "date" }; 3442 case -1687512484: 3443 /* lastReviewDate */ return new String[] { "date" }; 3444 case -403934648: 3445 /* effectivePeriod */ return new String[] { "Period" }; 3446 case 3059181: 3447 /* code */ return new String[] { "CodeableConcept" }; 3448 case 555127957: 3449 /* instance */ return new String[] { "Reference" }; 3450 case -1526770491: 3451 /* applicability */ return new String[] {}; 3452 case -1041594966: 3453 /* propertyGroup */ return new String[] {}; 3454 default: 3455 return super.getTypesForProperty(hash, name); 3456 } 3457 3458 } 3459 3460 @Override 3461 public Base addChild(String name) throws FHIRException { 3462 if (name.equals("url")) { 3463 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.url"); 3464 } else if (name.equals("identifier")) { 3465 return addIdentifier(); 3466 } else if (name.equals("version")) { 3467 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.version"); 3468 } else if (name.equals("title")) { 3469 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.title"); 3470 } else if (name.equals("derivedFromUri")) { 3471 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.derivedFromUri"); 3472 } else if (name.equals("partOf")) { 3473 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.partOf"); 3474 } else if (name.equals("replaces")) { 3475 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.replaces"); 3476 } else if (name.equals("status")) { 3477 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.status"); 3478 } else if (name.equals("experimental")) { 3479 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.experimental"); 3480 } else if (name.equals("date")) { 3481 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.date"); 3482 } else if (name.equals("publisher")) { 3483 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.publisher"); 3484 } else if (name.equals("contact")) { 3485 return addContact(); 3486 } else if (name.equals("description")) { 3487 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.description"); 3488 } else if (name.equals("useContext")) { 3489 return addUseContext(); 3490 } else if (name.equals("jurisdiction")) { 3491 return addJurisdiction(); 3492 } else if (name.equals("copyright")) { 3493 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.copyright"); 3494 } else if (name.equals("approvalDate")) { 3495 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.approvalDate"); 3496 } else if (name.equals("lastReviewDate")) { 3497 throw new FHIRException("Cannot call addChild on a singleton property ChargeItemDefinition.lastReviewDate"); 3498 } else if (name.equals("effectivePeriod")) { 3499 this.effectivePeriod = new Period(); 3500 return this.effectivePeriod; 3501 } else if (name.equals("code")) { 3502 this.code = new CodeableConcept(); 3503 return this.code; 3504 } else if (name.equals("instance")) { 3505 return addInstance(); 3506 } else if (name.equals("applicability")) { 3507 return addApplicability(); 3508 } else if (name.equals("propertyGroup")) { 3509 return addPropertyGroup(); 3510 } else 3511 return super.addChild(name); 3512 } 3513 3514 public String fhirType() { 3515 return "ChargeItemDefinition"; 3516 3517 } 3518 3519 public ChargeItemDefinition copy() { 3520 ChargeItemDefinition dst = new ChargeItemDefinition(); 3521 copyValues(dst); 3522 return dst; 3523 } 3524 3525 public void copyValues(ChargeItemDefinition dst) { 3526 super.copyValues(dst); 3527 dst.url = url == null ? null : url.copy(); 3528 if (identifier != null) { 3529 dst.identifier = new ArrayList<Identifier>(); 3530 for (Identifier i : identifier) 3531 dst.identifier.add(i.copy()); 3532 } 3533 ; 3534 dst.version = version == null ? null : version.copy(); 3535 dst.title = title == null ? null : title.copy(); 3536 if (derivedFromUri != null) { 3537 dst.derivedFromUri = new ArrayList<UriType>(); 3538 for (UriType i : derivedFromUri) 3539 dst.derivedFromUri.add(i.copy()); 3540 } 3541 ; 3542 if (partOf != null) { 3543 dst.partOf = new ArrayList<CanonicalType>(); 3544 for (CanonicalType i : partOf) 3545 dst.partOf.add(i.copy()); 3546 } 3547 ; 3548 if (replaces != null) { 3549 dst.replaces = new ArrayList<CanonicalType>(); 3550 for (CanonicalType i : replaces) 3551 dst.replaces.add(i.copy()); 3552 } 3553 ; 3554 dst.status = status == null ? null : status.copy(); 3555 dst.experimental = experimental == null ? null : experimental.copy(); 3556 dst.date = date == null ? null : date.copy(); 3557 dst.publisher = publisher == null ? null : publisher.copy(); 3558 if (contact != null) { 3559 dst.contact = new ArrayList<ContactDetail>(); 3560 for (ContactDetail i : contact) 3561 dst.contact.add(i.copy()); 3562 } 3563 ; 3564 dst.description = description == null ? null : description.copy(); 3565 if (useContext != null) { 3566 dst.useContext = new ArrayList<UsageContext>(); 3567 for (UsageContext i : useContext) 3568 dst.useContext.add(i.copy()); 3569 } 3570 ; 3571 if (jurisdiction != null) { 3572 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3573 for (CodeableConcept i : jurisdiction) 3574 dst.jurisdiction.add(i.copy()); 3575 } 3576 ; 3577 dst.copyright = copyright == null ? null : copyright.copy(); 3578 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3579 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3580 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3581 dst.code = code == null ? null : code.copy(); 3582 if (instance != null) { 3583 dst.instance = new ArrayList<Reference>(); 3584 for (Reference i : instance) 3585 dst.instance.add(i.copy()); 3586 } 3587 ; 3588 if (applicability != null) { 3589 dst.applicability = new ArrayList<ChargeItemDefinitionApplicabilityComponent>(); 3590 for (ChargeItemDefinitionApplicabilityComponent i : applicability) 3591 dst.applicability.add(i.copy()); 3592 } 3593 ; 3594 if (propertyGroup != null) { 3595 dst.propertyGroup = new ArrayList<ChargeItemDefinitionPropertyGroupComponent>(); 3596 for (ChargeItemDefinitionPropertyGroupComponent i : propertyGroup) 3597 dst.propertyGroup.add(i.copy()); 3598 } 3599 ; 3600 } 3601 3602 protected ChargeItemDefinition typedCopy() { 3603 return copy(); 3604 } 3605 3606 @Override 3607 public boolean equalsDeep(Base other_) { 3608 if (!super.equalsDeep(other_)) 3609 return false; 3610 if (!(other_ instanceof ChargeItemDefinition)) 3611 return false; 3612 ChargeItemDefinition o = (ChargeItemDefinition) other_; 3613 return compareDeep(identifier, o.identifier, true) && compareDeep(derivedFromUri, o.derivedFromUri, true) 3614 && compareDeep(partOf, o.partOf, true) && compareDeep(replaces, o.replaces, true) 3615 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 3616 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 3617 && compareDeep(code, o.code, true) && compareDeep(instance, o.instance, true) 3618 && compareDeep(applicability, o.applicability, true) && compareDeep(propertyGroup, o.propertyGroup, true); 3619 } 3620 3621 @Override 3622 public boolean equalsShallow(Base other_) { 3623 if (!super.equalsShallow(other_)) 3624 return false; 3625 if (!(other_ instanceof ChargeItemDefinition)) 3626 return false; 3627 ChargeItemDefinition o = (ChargeItemDefinition) other_; 3628 return compareValues(derivedFromUri, o.derivedFromUri, true) && compareValues(copyright, o.copyright, true) 3629 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 3630 } 3631 3632 public boolean isEmpty() { 3633 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, derivedFromUri, partOf, replaces, 3634 copyright, approvalDate, lastReviewDate, effectivePeriod, code, instance, applicability, propertyGroup); 3635 } 3636 3637 @Override 3638 public ResourceType getResourceType() { 3639 return ResourceType.ChargeItemDefinition; 3640 } 3641 3642 /** 3643 * Search parameter: <b>date</b> 3644 * <p> 3645 * Description: <b>The charge item definition publication date</b><br> 3646 * Type: <b>date</b><br> 3647 * Path: <b>ChargeItemDefinition.date</b><br> 3648 * </p> 3649 */ 3650 @SearchParamDefinition(name = "date", path = "ChargeItemDefinition.date", description = "The charge item definition publication date", type = "date") 3651 public static final String SP_DATE = "date"; 3652 /** 3653 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3654 * <p> 3655 * Description: <b>The charge item definition publication date</b><br> 3656 * Type: <b>date</b><br> 3657 * Path: <b>ChargeItemDefinition.date</b><br> 3658 * </p> 3659 */ 3660 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3661 SP_DATE); 3662 3663 /** 3664 * Search parameter: <b>identifier</b> 3665 * <p> 3666 * Description: <b>External identifier for the charge item definition</b><br> 3667 * Type: <b>token</b><br> 3668 * Path: <b>ChargeItemDefinition.identifier</b><br> 3669 * </p> 3670 */ 3671 @SearchParamDefinition(name = "identifier", path = "ChargeItemDefinition.identifier", description = "External identifier for the charge item definition", type = "token") 3672 public static final String SP_IDENTIFIER = "identifier"; 3673 /** 3674 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3675 * <p> 3676 * Description: <b>External identifier for the charge item definition</b><br> 3677 * Type: <b>token</b><br> 3678 * Path: <b>ChargeItemDefinition.identifier</b><br> 3679 * </p> 3680 */ 3681 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3682 SP_IDENTIFIER); 3683 3684 /** 3685 * Search parameter: <b>context-type-value</b> 3686 * <p> 3687 * Description: <b>A use context type and value assigned to the charge item 3688 * definition</b><br> 3689 * Type: <b>composite</b><br> 3690 * Path: <b></b><br> 3691 * </p> 3692 */ 3693 @SearchParamDefinition(name = "context-type-value", path = "ChargeItemDefinition.useContext", description = "A use context type and value assigned to the charge item definition", type = "composite", compositeOf = { 3694 "context-type", "context" }) 3695 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3696 /** 3697 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3698 * <p> 3699 * Description: <b>A use context type and value assigned to the charge item 3700 * definition</b><br> 3701 * Type: <b>composite</b><br> 3702 * Path: <b></b><br> 3703 * </p> 3704 */ 3705 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3706 SP_CONTEXT_TYPE_VALUE); 3707 3708 /** 3709 * Search parameter: <b>jurisdiction</b> 3710 * <p> 3711 * Description: <b>Intended jurisdiction for the charge item definition</b><br> 3712 * Type: <b>token</b><br> 3713 * Path: <b>ChargeItemDefinition.jurisdiction</b><br> 3714 * </p> 3715 */ 3716 @SearchParamDefinition(name = "jurisdiction", path = "ChargeItemDefinition.jurisdiction", description = "Intended jurisdiction for the charge item definition", type = "token") 3717 public static final String SP_JURISDICTION = "jurisdiction"; 3718 /** 3719 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3720 * <p> 3721 * Description: <b>Intended jurisdiction for the charge item definition</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>ChargeItemDefinition.jurisdiction</b><br> 3724 * </p> 3725 */ 3726 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3727 SP_JURISDICTION); 3728 3729 /** 3730 * Search parameter: <b>description</b> 3731 * <p> 3732 * Description: <b>The description of the charge item definition</b><br> 3733 * Type: <b>string</b><br> 3734 * Path: <b>ChargeItemDefinition.description</b><br> 3735 * </p> 3736 */ 3737 @SearchParamDefinition(name = "description", path = "ChargeItemDefinition.description", description = "The description of the charge item definition", type = "string") 3738 public static final String SP_DESCRIPTION = "description"; 3739 /** 3740 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3741 * <p> 3742 * Description: <b>The description of the charge item definition</b><br> 3743 * Type: <b>string</b><br> 3744 * Path: <b>ChargeItemDefinition.description</b><br> 3745 * </p> 3746 */ 3747 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3748 SP_DESCRIPTION); 3749 3750 /** 3751 * Search parameter: <b>context-type</b> 3752 * <p> 3753 * Description: <b>A type of use context assigned to the charge item 3754 * definition</b><br> 3755 * Type: <b>token</b><br> 3756 * Path: <b>ChargeItemDefinition.useContext.code</b><br> 3757 * </p> 3758 */ 3759 @SearchParamDefinition(name = "context-type", path = "ChargeItemDefinition.useContext.code", description = "A type of use context assigned to the charge item definition", type = "token") 3760 public static final String SP_CONTEXT_TYPE = "context-type"; 3761 /** 3762 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3763 * <p> 3764 * Description: <b>A type of use context assigned to the charge item 3765 * definition</b><br> 3766 * Type: <b>token</b><br> 3767 * Path: <b>ChargeItemDefinition.useContext.code</b><br> 3768 * </p> 3769 */ 3770 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3771 SP_CONTEXT_TYPE); 3772 3773 /** 3774 * Search parameter: <b>title</b> 3775 * <p> 3776 * Description: <b>The human-friendly name of the charge item definition</b><br> 3777 * Type: <b>string</b><br> 3778 * Path: <b>ChargeItemDefinition.title</b><br> 3779 * </p> 3780 */ 3781 @SearchParamDefinition(name = "title", path = "ChargeItemDefinition.title", description = "The human-friendly name of the charge item definition", type = "string") 3782 public static final String SP_TITLE = "title"; 3783 /** 3784 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3785 * <p> 3786 * Description: <b>The human-friendly name of the charge item definition</b><br> 3787 * Type: <b>string</b><br> 3788 * Path: <b>ChargeItemDefinition.title</b><br> 3789 * </p> 3790 */ 3791 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3792 SP_TITLE); 3793 3794 /** 3795 * Search parameter: <b>version</b> 3796 * <p> 3797 * Description: <b>The business version of the charge item definition</b><br> 3798 * Type: <b>token</b><br> 3799 * Path: <b>ChargeItemDefinition.version</b><br> 3800 * </p> 3801 */ 3802 @SearchParamDefinition(name = "version", path = "ChargeItemDefinition.version", description = "The business version of the charge item definition", type = "token") 3803 public static final String SP_VERSION = "version"; 3804 /** 3805 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3806 * <p> 3807 * Description: <b>The business version of the charge item definition</b><br> 3808 * Type: <b>token</b><br> 3809 * Path: <b>ChargeItemDefinition.version</b><br> 3810 * </p> 3811 */ 3812 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3813 SP_VERSION); 3814 3815 /** 3816 * Search parameter: <b>url</b> 3817 * <p> 3818 * Description: <b>The uri that identifies the charge item definition</b><br> 3819 * Type: <b>uri</b><br> 3820 * Path: <b>ChargeItemDefinition.url</b><br> 3821 * </p> 3822 */ 3823 @SearchParamDefinition(name = "url", path = "ChargeItemDefinition.url", description = "The uri that identifies the charge item definition", type = "uri") 3824 public static final String SP_URL = "url"; 3825 /** 3826 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3827 * <p> 3828 * Description: <b>The uri that identifies the charge item definition</b><br> 3829 * Type: <b>uri</b><br> 3830 * Path: <b>ChargeItemDefinition.url</b><br> 3831 * </p> 3832 */ 3833 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3834 3835 /** 3836 * Search parameter: <b>context-quantity</b> 3837 * <p> 3838 * Description: <b>A quantity- or range-valued use context assigned to the 3839 * charge item definition</b><br> 3840 * Type: <b>quantity</b><br> 3841 * Path: <b>ChargeItemDefinition.useContext.valueQuantity, 3842 * ChargeItemDefinition.useContext.valueRange</b><br> 3843 * </p> 3844 */ 3845 @SearchParamDefinition(name = "context-quantity", path = "(ChargeItemDefinition.useContext.value as Quantity) | (ChargeItemDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the charge item definition", type = "quantity") 3846 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3847 /** 3848 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3849 * <p> 3850 * Description: <b>A quantity- or range-valued use context assigned to the 3851 * charge item definition</b><br> 3852 * Type: <b>quantity</b><br> 3853 * Path: <b>ChargeItemDefinition.useContext.valueQuantity, 3854 * ChargeItemDefinition.useContext.valueRange</b><br> 3855 * </p> 3856 */ 3857 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3858 SP_CONTEXT_QUANTITY); 3859 3860 /** 3861 * Search parameter: <b>effective</b> 3862 * <p> 3863 * Description: <b>The time during which the charge item definition is intended 3864 * to be in use</b><br> 3865 * Type: <b>date</b><br> 3866 * Path: <b>ChargeItemDefinition.effectivePeriod</b><br> 3867 * </p> 3868 */ 3869 @SearchParamDefinition(name = "effective", path = "ChargeItemDefinition.effectivePeriod", description = "The time during which the charge item definition is intended to be in use", type = "date") 3870 public static final String SP_EFFECTIVE = "effective"; 3871 /** 3872 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3873 * <p> 3874 * Description: <b>The time during which the charge item definition is intended 3875 * to be in use</b><br> 3876 * Type: <b>date</b><br> 3877 * Path: <b>ChargeItemDefinition.effectivePeriod</b><br> 3878 * </p> 3879 */ 3880 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3881 SP_EFFECTIVE); 3882 3883 /** 3884 * Search parameter: <b>context</b> 3885 * <p> 3886 * Description: <b>A use context assigned to the charge item definition</b><br> 3887 * Type: <b>token</b><br> 3888 * Path: <b>ChargeItemDefinition.useContext.valueCodeableConcept</b><br> 3889 * </p> 3890 */ 3891 @SearchParamDefinition(name = "context", path = "(ChargeItemDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the charge item definition", type = "token") 3892 public static final String SP_CONTEXT = "context"; 3893 /** 3894 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3895 * <p> 3896 * Description: <b>A use context assigned to the charge item definition</b><br> 3897 * Type: <b>token</b><br> 3898 * Path: <b>ChargeItemDefinition.useContext.valueCodeableConcept</b><br> 3899 * </p> 3900 */ 3901 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3902 SP_CONTEXT); 3903 3904 /** 3905 * Search parameter: <b>publisher</b> 3906 * <p> 3907 * Description: <b>Name of the publisher of the charge item definition</b><br> 3908 * Type: <b>string</b><br> 3909 * Path: <b>ChargeItemDefinition.publisher</b><br> 3910 * </p> 3911 */ 3912 @SearchParamDefinition(name = "publisher", path = "ChargeItemDefinition.publisher", description = "Name of the publisher of the charge item definition", type = "string") 3913 public static final String SP_PUBLISHER = "publisher"; 3914 /** 3915 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3916 * <p> 3917 * Description: <b>Name of the publisher of the charge item definition</b><br> 3918 * Type: <b>string</b><br> 3919 * Path: <b>ChargeItemDefinition.publisher</b><br> 3920 * </p> 3921 */ 3922 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3923 SP_PUBLISHER); 3924 3925 /** 3926 * Search parameter: <b>context-type-quantity</b> 3927 * <p> 3928 * Description: <b>A use context type and quantity- or range-based value 3929 * assigned to the charge item definition</b><br> 3930 * Type: <b>composite</b><br> 3931 * Path: <b></b><br> 3932 * </p> 3933 */ 3934 @SearchParamDefinition(name = "context-type-quantity", path = "ChargeItemDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the charge item definition", type = "composite", compositeOf = { 3935 "context-type", "context-quantity" }) 3936 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3937 /** 3938 * <b>Fluent Client</b> search parameter constant for 3939 * <b>context-type-quantity</b> 3940 * <p> 3941 * Description: <b>A use context type and quantity- or range-based value 3942 * assigned to the charge item definition</b><br> 3943 * Type: <b>composite</b><br> 3944 * Path: <b></b><br> 3945 * </p> 3946 */ 3947 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3948 SP_CONTEXT_TYPE_QUANTITY); 3949 3950 /** 3951 * Search parameter: <b>status</b> 3952 * <p> 3953 * Description: <b>The current status of the charge item definition</b><br> 3954 * Type: <b>token</b><br> 3955 * Path: <b>ChargeItemDefinition.status</b><br> 3956 * </p> 3957 */ 3958 @SearchParamDefinition(name = "status", path = "ChargeItemDefinition.status", description = "The current status of the charge item definition", type = "token") 3959 public static final String SP_STATUS = "status"; 3960 /** 3961 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3962 * <p> 3963 * Description: <b>The current status of the charge item definition</b><br> 3964 * Type: <b>token</b><br> 3965 * Path: <b>ChargeItemDefinition.status</b><br> 3966 * </p> 3967 */ 3968 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3969 SP_STATUS); 3970 3971}