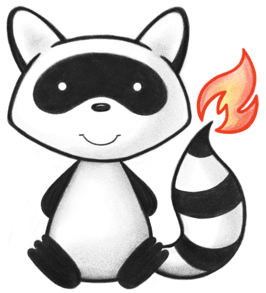
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Collections; 035import java.util.Comparator; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * The CodeSystem resource is used to declare the existence of and describe a 054 * code system or code system supplement and its key properties, and optionally 055 * define a part or all of its content. 056 */ 057@ResourceDef(name = "CodeSystem", profile = "http://hl7.org/fhir/StructureDefinition/CodeSystem") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 059 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "caseSensitive", "valueSet", 060 "hierarchyMeaning", "compositional", "versionNeeded", "content", "supplements", "count", "filter", "property", 061 "concept" }) 062public class CodeSystem extends MetadataResource { 063 064 public enum CodeSystemHierarchyMeaning { 065 /** 066 * No particular relationship between the concepts can be assumed, except what 067 * can be determined by inspection of the definitions of the elements (possible 068 * reasons to use this: importing from a source where this is not defined, or 069 * where various parts of the hierarchy have different meanings). 070 */ 071 GROUPEDBY, 072 /** 073 * A hierarchy where the child concepts have an IS-A relationship with the 074 * parents - that is, all the properties of the parent are also true for its 075 * child concepts. Not that is-a is a property of the concepts, so additional 076 * subsumption relationships may be defined using properties or the 077 * [subsumes](extension-codesystem-subsumes.html) extension. 078 */ 079 ISA, 080 /** 081 * Child elements list the individual parts of a composite whole (e.g. body 082 * site). 083 */ 084 PARTOF, 085 /** 086 * Child concepts in the hierarchy may have only one parent, and there is a 087 * presumption that the code system is a "closed world" meaning all things must 088 * be in the hierarchy. This results in concepts such as "not otherwise 089 * classified.". 090 */ 091 CLASSIFIEDWITH, 092 /** 093 * added to help the parsers with the generic types 094 */ 095 NULL; 096 097 public static CodeSystemHierarchyMeaning fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("grouped-by".equals(codeString)) 101 return GROUPEDBY; 102 if ("is-a".equals(codeString)) 103 return ISA; 104 if ("part-of".equals(codeString)) 105 return PARTOF; 106 if ("classified-with".equals(codeString)) 107 return CLASSIFIEDWITH; 108 if (Configuration.isAcceptInvalidEnums()) 109 return null; 110 else 111 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '" + codeString + "'"); 112 } 113 114 public String toCode() { 115 switch (this) { 116 case GROUPEDBY: 117 return "grouped-by"; 118 case ISA: 119 return "is-a"; 120 case PARTOF: 121 return "part-of"; 122 case CLASSIFIEDWITH: 123 return "classified-with"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getSystem() { 132 switch (this) { 133 case GROUPEDBY: 134 return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 135 case ISA: 136 return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 137 case PARTOF: 138 return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 139 case CLASSIFIEDWITH: 140 return "http://hl7.org/fhir/codesystem-hierarchy-meaning"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getDefinition() { 149 switch (this) { 150 case GROUPEDBY: 151 return "No particular relationship between the concepts can be assumed, except what can be determined by inspection of the definitions of the elements (possible reasons to use this: importing from a source where this is not defined, or where various parts of the hierarchy have different meanings)."; 152 case ISA: 153 return "A hierarchy where the child concepts have an IS-A relationship with the parents - that is, all the properties of the parent are also true for its child concepts. Not that is-a is a property of the concepts, so additional subsumption relationships may be defined using properties or the [subsumes](extension-codesystem-subsumes.html) extension."; 154 case PARTOF: 155 return "Child elements list the individual parts of a composite whole (e.g. body site)."; 156 case CLASSIFIEDWITH: 157 return "Child concepts in the hierarchy may have only one parent, and there is a presumption that the code system is a \"closed world\" meaning all things must be in the hierarchy. This results in concepts such as \"not otherwise classified.\"."; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDisplay() { 166 switch (this) { 167 case GROUPEDBY: 168 return "Grouped By"; 169 case ISA: 170 return "Is-A"; 171 case PARTOF: 172 return "Part Of"; 173 case CLASSIFIEDWITH: 174 return "Classified With"; 175 case NULL: 176 return null; 177 default: 178 return "?"; 179 } 180 } 181 } 182 183 public static class CodeSystemHierarchyMeaningEnumFactory implements EnumFactory<CodeSystemHierarchyMeaning> { 184 public CodeSystemHierarchyMeaning fromCode(String codeString) throws IllegalArgumentException { 185 if (codeString == null || "".equals(codeString)) 186 if (codeString == null || "".equals(codeString)) 187 return null; 188 if ("grouped-by".equals(codeString)) 189 return CodeSystemHierarchyMeaning.GROUPEDBY; 190 if ("is-a".equals(codeString)) 191 return CodeSystemHierarchyMeaning.ISA; 192 if ("part-of".equals(codeString)) 193 return CodeSystemHierarchyMeaning.PARTOF; 194 if ("classified-with".equals(codeString)) 195 return CodeSystemHierarchyMeaning.CLASSIFIEDWITH; 196 throw new IllegalArgumentException("Unknown CodeSystemHierarchyMeaning code '" + codeString + "'"); 197 } 198 199 public Enumeration<CodeSystemHierarchyMeaning> fromType(PrimitiveType<?> code) throws FHIRException { 200 if (code == null) 201 return null; 202 if (code.isEmpty()) 203 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.NULL, code); 204 String codeString = code.asStringValue(); 205 if (codeString == null || "".equals(codeString)) 206 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.NULL, code); 207 if ("grouped-by".equals(codeString)) 208 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.GROUPEDBY, code); 209 if ("is-a".equals(codeString)) 210 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.ISA, code); 211 if ("part-of".equals(codeString)) 212 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.PARTOF, code); 213 if ("classified-with".equals(codeString)) 214 return new Enumeration<CodeSystemHierarchyMeaning>(this, CodeSystemHierarchyMeaning.CLASSIFIEDWITH, code); 215 throw new FHIRException("Unknown CodeSystemHierarchyMeaning code '" + codeString + "'"); 216 } 217 218 public String toCode(CodeSystemHierarchyMeaning code) { 219 if (code == CodeSystemHierarchyMeaning.NULL) 220 return null; 221 if (code == CodeSystemHierarchyMeaning.GROUPEDBY) 222 return "grouped-by"; 223 if (code == CodeSystemHierarchyMeaning.ISA) 224 return "is-a"; 225 if (code == CodeSystemHierarchyMeaning.PARTOF) 226 return "part-of"; 227 if (code == CodeSystemHierarchyMeaning.CLASSIFIEDWITH) 228 return "classified-with"; 229 return "?"; 230 } 231 232 public String toSystem(CodeSystemHierarchyMeaning code) { 233 return code.getSystem(); 234 } 235 } 236 237 public enum CodeSystemContentMode { 238 /** 239 * None of the concepts defined by the code system are included in the code 240 * system resource. 241 */ 242 NOTPRESENT, 243 /** 244 * A few representative concepts are included in the code system resource. There 245 * is no useful intent in the subset choice and there's no process to make it 246 * workable: it's not intended to be workable. 247 */ 248 EXAMPLE, 249 /** 250 * A subset of the code system concepts are included in the code system 251 * resource. This is a curated subset released for a specific purpose under the 252 * governance of the code system steward, and that the intent, bounds and 253 * consequences of the fragmentation are clearly defined in the fragment or the 254 * code system documentation. Fragments are also known as partitions. 255 */ 256 FRAGMENT, 257 /** 258 * All the concepts defined by the code system are included in the code system 259 * resource. 260 */ 261 COMPLETE, 262 /** 263 * The resource doesn't define any new concepts; it just provides additional 264 * designations and properties to another code system. 265 */ 266 SUPPLEMENT, 267 /** 268 * added to help the parsers with the generic types 269 */ 270 NULL; 271 272 public static CodeSystemContentMode fromCode(String codeString) throws FHIRException { 273 if (codeString == null || "".equals(codeString)) 274 return null; 275 if ("not-present".equals(codeString)) 276 return NOTPRESENT; 277 if ("example".equals(codeString)) 278 return EXAMPLE; 279 if ("fragment".equals(codeString)) 280 return FRAGMENT; 281 if ("complete".equals(codeString)) 282 return COMPLETE; 283 if ("supplement".equals(codeString)) 284 return SUPPLEMENT; 285 if (Configuration.isAcceptInvalidEnums()) 286 return null; 287 else 288 throw new FHIRException("Unknown CodeSystemContentMode code '" + codeString + "'"); 289 } 290 291 public String toCode() { 292 switch (this) { 293 case NOTPRESENT: 294 return "not-present"; 295 case EXAMPLE: 296 return "example"; 297 case FRAGMENT: 298 return "fragment"; 299 case COMPLETE: 300 return "complete"; 301 case SUPPLEMENT: 302 return "supplement"; 303 case NULL: 304 return null; 305 default: 306 return "?"; 307 } 308 } 309 310 public String getSystem() { 311 switch (this) { 312 case NOTPRESENT: 313 return "http://hl7.org/fhir/codesystem-content-mode"; 314 case EXAMPLE: 315 return "http://hl7.org/fhir/codesystem-content-mode"; 316 case FRAGMENT: 317 return "http://hl7.org/fhir/codesystem-content-mode"; 318 case COMPLETE: 319 return "http://hl7.org/fhir/codesystem-content-mode"; 320 case SUPPLEMENT: 321 return "http://hl7.org/fhir/codesystem-content-mode"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 329 public String getDefinition() { 330 switch (this) { 331 case NOTPRESENT: 332 return "None of the concepts defined by the code system are included in the code system resource."; 333 case EXAMPLE: 334 return "A few representative concepts are included in the code system resource. There is no useful intent in the subset choice and there's no process to make it workable: it's not intended to be workable."; 335 case FRAGMENT: 336 return "A subset of the code system concepts are included in the code system resource. This is a curated subset released for a specific purpose under the governance of the code system steward, and that the intent, bounds and consequences of the fragmentation are clearly defined in the fragment or the code system documentation. Fragments are also known as partitions."; 337 case COMPLETE: 338 return "All the concepts defined by the code system are included in the code system resource."; 339 case SUPPLEMENT: 340 return "The resource doesn't define any new concepts; it just provides additional designations and properties to another code system."; 341 case NULL: 342 return null; 343 default: 344 return "?"; 345 } 346 } 347 348 public String getDisplay() { 349 switch (this) { 350 case NOTPRESENT: 351 return "Not Present"; 352 case EXAMPLE: 353 return "Example"; 354 case FRAGMENT: 355 return "Fragment"; 356 case COMPLETE: 357 return "Complete"; 358 case SUPPLEMENT: 359 return "Supplement"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 } 367 368 public static class CodeSystemContentModeEnumFactory implements EnumFactory<CodeSystemContentMode> { 369 public CodeSystemContentMode fromCode(String codeString) throws IllegalArgumentException { 370 if (codeString == null || "".equals(codeString)) 371 if (codeString == null || "".equals(codeString)) 372 return null; 373 if ("not-present".equals(codeString)) 374 return CodeSystemContentMode.NOTPRESENT; 375 if ("example".equals(codeString)) 376 return CodeSystemContentMode.EXAMPLE; 377 if ("fragment".equals(codeString)) 378 return CodeSystemContentMode.FRAGMENT; 379 if ("complete".equals(codeString)) 380 return CodeSystemContentMode.COMPLETE; 381 if ("supplement".equals(codeString)) 382 return CodeSystemContentMode.SUPPLEMENT; 383 throw new IllegalArgumentException("Unknown CodeSystemContentMode code '" + codeString + "'"); 384 } 385 386 public Enumeration<CodeSystemContentMode> fromType(PrimitiveType<?> code) throws FHIRException { 387 if (code == null) 388 return null; 389 if (code.isEmpty()) 390 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.NULL, code); 391 String codeString = code.asStringValue(); 392 if (codeString == null || "".equals(codeString)) 393 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.NULL, code); 394 if ("not-present".equals(codeString)) 395 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.NOTPRESENT, code); 396 if ("example".equals(codeString)) 397 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.EXAMPLE, code); 398 if ("fragment".equals(codeString)) 399 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.FRAGMENT, code); 400 if ("complete".equals(codeString)) 401 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.COMPLETE, code); 402 if ("supplement".equals(codeString)) 403 return new Enumeration<CodeSystemContentMode>(this, CodeSystemContentMode.SUPPLEMENT, code); 404 throw new FHIRException("Unknown CodeSystemContentMode code '" + codeString + "'"); 405 } 406 407 public String toCode(CodeSystemContentMode code) { 408 if (code == CodeSystemContentMode.NULL) 409 return null; 410 if (code == CodeSystemContentMode.NOTPRESENT) 411 return "not-present"; 412 if (code == CodeSystemContentMode.EXAMPLE) 413 return "example"; 414 if (code == CodeSystemContentMode.FRAGMENT) 415 return "fragment"; 416 if (code == CodeSystemContentMode.COMPLETE) 417 return "complete"; 418 if (code == CodeSystemContentMode.SUPPLEMENT) 419 return "supplement"; 420 return "?"; 421 } 422 423 public String toSystem(CodeSystemContentMode code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum FilterOperator { 429 /** 430 * The specified property of the code equals the provided value. 431 */ 432 EQUAL, 433 /** 434 * Includes all concept ids that have a transitive is-a relationship with the 435 * concept Id provided as the value, including the provided concept itself 436 * (include descendant codes and self). 437 */ 438 ISA, 439 /** 440 * Includes all concept ids that have a transitive is-a relationship with the 441 * concept Id provided as the value, excluding the provided concept itself i.e. 442 * include descendant codes only). 443 */ 444 DESCENDENTOF, 445 /** 446 * The specified property of the code does not have an is-a relationship with 447 * the provided value. 448 */ 449 ISNOTA, 450 /** 451 * The specified property of the code matches the regex specified in the 452 * provided value. 453 */ 454 REGEX, 455 /** 456 * The specified property of the code is in the set of codes or concepts 457 * specified in the provided value (comma separated list). 458 */ 459 IN, 460 /** 461 * The specified property of the code is not in the set of codes or concepts 462 * specified in the provided value (comma separated list). 463 */ 464 NOTIN, 465 /** 466 * Includes all concept ids that have a transitive is-a relationship from the 467 * concept Id provided as the value, including the provided concept itself (i.e. 468 * include ancestor codes and self). 469 */ 470 GENERALIZES, 471 /** 472 * The specified property of the code has at least one value (if the specified 473 * value is true; if the specified value is false, then matches when the 474 * specified property of the code has no values). 475 */ 476 EXISTS, 477 /** 478 * added to help the parsers with the generic types 479 */ 480 NULL; 481 482 public static FilterOperator fromCode(String codeString) throws FHIRException { 483 if (codeString == null || "".equals(codeString)) 484 return null; 485 if ("=".equals(codeString)) 486 return EQUAL; 487 if ("is-a".equals(codeString)) 488 return ISA; 489 if ("descendent-of".equals(codeString)) 490 return DESCENDENTOF; 491 if ("is-not-a".equals(codeString)) 492 return ISNOTA; 493 if ("regex".equals(codeString)) 494 return REGEX; 495 if ("in".equals(codeString)) 496 return IN; 497 if ("not-in".equals(codeString)) 498 return NOTIN; 499 if ("generalizes".equals(codeString)) 500 return GENERALIZES; 501 if ("exists".equals(codeString)) 502 return EXISTS; 503 if (Configuration.isAcceptInvalidEnums()) 504 return null; 505 else 506 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 507 } 508 509 public String toCode() { 510 switch (this) { 511 case EQUAL: 512 return "="; 513 case ISA: 514 return "is-a"; 515 case DESCENDENTOF: 516 return "descendent-of"; 517 case ISNOTA: 518 return "is-not-a"; 519 case REGEX: 520 return "regex"; 521 case IN: 522 return "in"; 523 case NOTIN: 524 return "not-in"; 525 case GENERALIZES: 526 return "generalizes"; 527 case EXISTS: 528 return "exists"; 529 case NULL: 530 return null; 531 default: 532 return "?"; 533 } 534 } 535 536 public String getSystem() { 537 switch (this) { 538 case EQUAL: 539 return "http://hl7.org/fhir/filter-operator"; 540 case ISA: 541 return "http://hl7.org/fhir/filter-operator"; 542 case DESCENDENTOF: 543 return "http://hl7.org/fhir/filter-operator"; 544 case ISNOTA: 545 return "http://hl7.org/fhir/filter-operator"; 546 case REGEX: 547 return "http://hl7.org/fhir/filter-operator"; 548 case IN: 549 return "http://hl7.org/fhir/filter-operator"; 550 case NOTIN: 551 return "http://hl7.org/fhir/filter-operator"; 552 case GENERALIZES: 553 return "http://hl7.org/fhir/filter-operator"; 554 case EXISTS: 555 return "http://hl7.org/fhir/filter-operator"; 556 case NULL: 557 return null; 558 default: 559 return "?"; 560 } 561 } 562 563 public String getDefinition() { 564 switch (this) { 565 case EQUAL: 566 return "The specified property of the code equals the provided value."; 567 case ISA: 568 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (include descendant codes and self)."; 569 case DESCENDENTOF: 570 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself i.e. include descendant codes only)."; 571 case ISNOTA: 572 return "The specified property of the code does not have an is-a relationship with the provided value."; 573 case REGEX: 574 return "The specified property of the code matches the regex specified in the provided value."; 575 case IN: 576 return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 577 case NOTIN: 578 return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 579 case GENERALIZES: 580 return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (i.e. include ancestor codes and self)."; 581 case EXISTS: 582 return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)."; 583 case NULL: 584 return null; 585 default: 586 return "?"; 587 } 588 } 589 590 public String getDisplay() { 591 switch (this) { 592 case EQUAL: 593 return "Equals"; 594 case ISA: 595 return "Is A (by subsumption)"; 596 case DESCENDENTOF: 597 return "Descendent Of (by subsumption)"; 598 case ISNOTA: 599 return "Not (Is A) (by subsumption)"; 600 case REGEX: 601 return "Regular Expression"; 602 case IN: 603 return "In Set"; 604 case NOTIN: 605 return "Not in Set"; 606 case GENERALIZES: 607 return "Generalizes (by Subsumption)"; 608 case EXISTS: 609 return "Exists"; 610 case NULL: 611 return null; 612 default: 613 return "?"; 614 } 615 } 616 } 617 618 public static class FilterOperatorEnumFactory implements EnumFactory<FilterOperator> { 619 public FilterOperator fromCode(String codeString) throws IllegalArgumentException { 620 if (codeString == null || "".equals(codeString)) 621 if (codeString == null || "".equals(codeString)) 622 return null; 623 if ("=".equals(codeString)) 624 return FilterOperator.EQUAL; 625 if ("is-a".equals(codeString)) 626 return FilterOperator.ISA; 627 if ("descendent-of".equals(codeString)) 628 return FilterOperator.DESCENDENTOF; 629 if ("is-not-a".equals(codeString)) 630 return FilterOperator.ISNOTA; 631 if ("regex".equals(codeString)) 632 return FilterOperator.REGEX; 633 if ("in".equals(codeString)) 634 return FilterOperator.IN; 635 if ("not-in".equals(codeString)) 636 return FilterOperator.NOTIN; 637 if ("generalizes".equals(codeString)) 638 return FilterOperator.GENERALIZES; 639 if ("exists".equals(codeString)) 640 return FilterOperator.EXISTS; 641 throw new IllegalArgumentException("Unknown FilterOperator code '" + codeString + "'"); 642 } 643 644 public Enumeration<FilterOperator> fromType(PrimitiveType<?> code) throws FHIRException { 645 if (code == null) 646 return null; 647 if (code.isEmpty()) 648 return new Enumeration<FilterOperator>(this, FilterOperator.NULL, code); 649 String codeString = code.asStringValue(); 650 if (codeString == null || "".equals(codeString)) 651 return new Enumeration<FilterOperator>(this, FilterOperator.NULL, code); 652 if ("=".equals(codeString)) 653 return new Enumeration<FilterOperator>(this, FilterOperator.EQUAL, code); 654 if ("is-a".equals(codeString)) 655 return new Enumeration<FilterOperator>(this, FilterOperator.ISA, code); 656 if ("descendent-of".equals(codeString)) 657 return new Enumeration<FilterOperator>(this, FilterOperator.DESCENDENTOF, code); 658 if ("is-not-a".equals(codeString)) 659 return new Enumeration<FilterOperator>(this, FilterOperator.ISNOTA, code); 660 if ("regex".equals(codeString)) 661 return new Enumeration<FilterOperator>(this, FilterOperator.REGEX, code); 662 if ("in".equals(codeString)) 663 return new Enumeration<FilterOperator>(this, FilterOperator.IN, code); 664 if ("not-in".equals(codeString)) 665 return new Enumeration<FilterOperator>(this, FilterOperator.NOTIN, code); 666 if ("generalizes".equals(codeString)) 667 return new Enumeration<FilterOperator>(this, FilterOperator.GENERALIZES, code); 668 if ("exists".equals(codeString)) 669 return new Enumeration<FilterOperator>(this, FilterOperator.EXISTS, code); 670 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 671 } 672 673 public String toCode(FilterOperator code) { 674 if (code == FilterOperator.NULL) 675 return null; 676 if (code == FilterOperator.EQUAL) 677 return "="; 678 if (code == FilterOperator.ISA) 679 return "is-a"; 680 if (code == FilterOperator.DESCENDENTOF) 681 return "descendent-of"; 682 if (code == FilterOperator.ISNOTA) 683 return "is-not-a"; 684 if (code == FilterOperator.REGEX) 685 return "regex"; 686 if (code == FilterOperator.IN) 687 return "in"; 688 if (code == FilterOperator.NOTIN) 689 return "not-in"; 690 if (code == FilterOperator.GENERALIZES) 691 return "generalizes"; 692 if (code == FilterOperator.EXISTS) 693 return "exists"; 694 return "?"; 695 } 696 697 public String toSystem(FilterOperator code) { 698 return code.getSystem(); 699 } 700 } 701 702 public enum PropertyType { 703 /** 704 * The property value is a code that identifies a concept defined in the code 705 * system. 706 */ 707 CODE, 708 /** 709 * The property value is a code defined in an external code system. This may be 710 * used for translations, but is not the intent. 711 */ 712 CODING, 713 /** 714 * The property value is a string. 715 */ 716 STRING, 717 /** 718 * The property value is a string (often used to assign ranking values to 719 * concepts for supporting score assessments). 720 */ 721 INTEGER, 722 /** 723 * The property value is a boolean true | false. 724 */ 725 BOOLEAN, 726 /** 727 * The property is a date or a date + time. 728 */ 729 DATETIME, 730 /** 731 * The property value is a decimal number. 732 */ 733 DECIMAL, 734 /** 735 * added to help the parsers with the generic types 736 */ 737 NULL; 738 739 public static PropertyType fromCode(String codeString) throws FHIRException { 740 if (codeString == null || "".equals(codeString)) 741 return null; 742 if ("code".equals(codeString)) 743 return CODE; 744 if ("Coding".equals(codeString)) 745 return CODING; 746 if ("string".equals(codeString)) 747 return STRING; 748 if ("integer".equals(codeString)) 749 return INTEGER; 750 if ("boolean".equals(codeString)) 751 return BOOLEAN; 752 if ("dateTime".equals(codeString)) 753 return DATETIME; 754 if ("decimal".equals(codeString)) 755 return DECIMAL; 756 if (Configuration.isAcceptInvalidEnums()) 757 return null; 758 else 759 throw new FHIRException("Unknown PropertyType code '" + codeString + "'"); 760 } 761 762 public String toCode() { 763 switch (this) { 764 case CODE: 765 return "code"; 766 case CODING: 767 return "Coding"; 768 case STRING: 769 return "string"; 770 case INTEGER: 771 return "integer"; 772 case BOOLEAN: 773 return "boolean"; 774 case DATETIME: 775 return "dateTime"; 776 case DECIMAL: 777 return "decimal"; 778 case NULL: 779 return null; 780 default: 781 return "?"; 782 } 783 } 784 785 public String getSystem() { 786 switch (this) { 787 case CODE: 788 return "http://hl7.org/fhir/concept-property-type"; 789 case CODING: 790 return "http://hl7.org/fhir/concept-property-type"; 791 case STRING: 792 return "http://hl7.org/fhir/concept-property-type"; 793 case INTEGER: 794 return "http://hl7.org/fhir/concept-property-type"; 795 case BOOLEAN: 796 return "http://hl7.org/fhir/concept-property-type"; 797 case DATETIME: 798 return "http://hl7.org/fhir/concept-property-type"; 799 case DECIMAL: 800 return "http://hl7.org/fhir/concept-property-type"; 801 case NULL: 802 return null; 803 default: 804 return "?"; 805 } 806 } 807 808 public String getDefinition() { 809 switch (this) { 810 case CODE: 811 return "The property value is a code that identifies a concept defined in the code system."; 812 case CODING: 813 return "The property value is a code defined in an external code system. This may be used for translations, but is not the intent."; 814 case STRING: 815 return "The property value is a string."; 816 case INTEGER: 817 return "The property value is a string (often used to assign ranking values to concepts for supporting score assessments)."; 818 case BOOLEAN: 819 return "The property value is a boolean true | false."; 820 case DATETIME: 821 return "The property is a date or a date + time."; 822 case DECIMAL: 823 return "The property value is a decimal number."; 824 case NULL: 825 return null; 826 default: 827 return "?"; 828 } 829 } 830 831 public String getDisplay() { 832 switch (this) { 833 case CODE: 834 return "code (internal reference)"; 835 case CODING: 836 return "Coding (external reference)"; 837 case STRING: 838 return "string"; 839 case INTEGER: 840 return "integer"; 841 case BOOLEAN: 842 return "boolean"; 843 case DATETIME: 844 return "dateTime"; 845 case DECIMAL: 846 return "decimal"; 847 case NULL: 848 return null; 849 default: 850 return "?"; 851 } 852 } 853 } 854 855 public static class PropertyTypeEnumFactory implements EnumFactory<PropertyType> { 856 public PropertyType fromCode(String codeString) throws IllegalArgumentException { 857 if (codeString == null || "".equals(codeString)) 858 if (codeString == null || "".equals(codeString)) 859 return null; 860 if ("code".equals(codeString)) 861 return PropertyType.CODE; 862 if ("Coding".equals(codeString)) 863 return PropertyType.CODING; 864 if ("string".equals(codeString)) 865 return PropertyType.STRING; 866 if ("integer".equals(codeString)) 867 return PropertyType.INTEGER; 868 if ("boolean".equals(codeString)) 869 return PropertyType.BOOLEAN; 870 if ("dateTime".equals(codeString)) 871 return PropertyType.DATETIME; 872 if ("decimal".equals(codeString)) 873 return PropertyType.DECIMAL; 874 throw new IllegalArgumentException("Unknown PropertyType code '" + codeString + "'"); 875 } 876 877 public Enumeration<PropertyType> fromType(PrimitiveType<?> code) throws FHIRException { 878 if (code == null) 879 return null; 880 if (code.isEmpty()) 881 return new Enumeration<PropertyType>(this, PropertyType.NULL, code); 882 String codeString = code.asStringValue(); 883 if (codeString == null || "".equals(codeString)) 884 return new Enumeration<PropertyType>(this, PropertyType.NULL, code); 885 if ("code".equals(codeString)) 886 return new Enumeration<PropertyType>(this, PropertyType.CODE, code); 887 if ("Coding".equals(codeString)) 888 return new Enumeration<PropertyType>(this, PropertyType.CODING, code); 889 if ("string".equals(codeString)) 890 return new Enumeration<PropertyType>(this, PropertyType.STRING, code); 891 if ("integer".equals(codeString)) 892 return new Enumeration<PropertyType>(this, PropertyType.INTEGER, code); 893 if ("boolean".equals(codeString)) 894 return new Enumeration<PropertyType>(this, PropertyType.BOOLEAN, code); 895 if ("dateTime".equals(codeString)) 896 return new Enumeration<PropertyType>(this, PropertyType.DATETIME, code); 897 if ("decimal".equals(codeString)) 898 return new Enumeration<PropertyType>(this, PropertyType.DECIMAL, code); 899 throw new FHIRException("Unknown PropertyType code '" + codeString + "'"); 900 } 901 902 public String toCode(PropertyType code) { 903 if (code == PropertyType.NULL) 904 return null; 905 if (code == PropertyType.CODE) 906 return "code"; 907 if (code == PropertyType.CODING) 908 return "Coding"; 909 if (code == PropertyType.STRING) 910 return "string"; 911 if (code == PropertyType.INTEGER) 912 return "integer"; 913 if (code == PropertyType.BOOLEAN) 914 return "boolean"; 915 if (code == PropertyType.DATETIME) 916 return "dateTime"; 917 if (code == PropertyType.DECIMAL) 918 return "decimal"; 919 return "?"; 920 } 921 922 public String toSystem(PropertyType code) { 923 return code.getSystem(); 924 } 925 } 926 927 @Block() 928 public static class CodeSystemFilterComponent extends BackboneElement implements IBaseBackboneElement { 929 /** 930 * The code that identifies this filter when it is used as a filter in 931 * [[[ValueSet]]].compose.include.filter. 932 */ 933 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 934 @Description(shortDefinition = "Code that identifies the filter", formalDefinition = "The code that identifies this filter when it is used as a filter in [[[ValueSet]]].compose.include.filter.") 935 protected CodeType code; 936 937 /** 938 * A description of how or why the filter is used. 939 */ 940 @Child(name = "description", type = { 941 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 942 @Description(shortDefinition = "How or why the filter is used", formalDefinition = "A description of how or why the filter is used.") 943 protected StringType description; 944 945 /** 946 * A list of operators that can be used with the filter. 947 */ 948 @Child(name = "operator", type = { 949 CodeType.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 950 @Description(shortDefinition = "= | is-a | descendent-of | is-not-a | regex | in | not-in | generalizes | exists", formalDefinition = "A list of operators that can be used with the filter.") 951 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/filter-operator") 952 protected List<Enumeration<FilterOperator>> operator; 953 954 /** 955 * A description of what the value for the filter should be. 956 */ 957 @Child(name = "value", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 958 @Description(shortDefinition = "What to use for the value", formalDefinition = "A description of what the value for the filter should be.") 959 protected StringType value; 960 961 private static final long serialVersionUID = -1087409836L; 962 963 /** 964 * Constructor 965 */ 966 public CodeSystemFilterComponent() { 967 super(); 968 } 969 970 /** 971 * Constructor 972 */ 973 public CodeSystemFilterComponent(CodeType code, StringType value) { 974 super(); 975 this.code = code; 976 this.value = value; 977 } 978 979 /** 980 * @return {@link #code} (The code that identifies this filter when it is used 981 * as a filter in [[[ValueSet]]].compose.include.filter.). This is the 982 * underlying object with id, value and extensions. The accessor 983 * "getCode" gives direct access to the value 984 */ 985 public CodeType getCodeElement() { 986 if (this.code == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create CodeSystemFilterComponent.code"); 989 else if (Configuration.doAutoCreate()) 990 this.code = new CodeType(); // bb 991 return this.code; 992 } 993 994 public boolean hasCodeElement() { 995 return this.code != null && !this.code.isEmpty(); 996 } 997 998 public boolean hasCode() { 999 return this.code != null && !this.code.isEmpty(); 1000 } 1001 1002 /** 1003 * @param value {@link #code} (The code that identifies this filter when it is 1004 * used as a filter in [[[ValueSet]]].compose.include.filter.). 1005 * This is the underlying object with id, value and extensions. The 1006 * accessor "getCode" gives direct access to the value 1007 */ 1008 public CodeSystemFilterComponent setCodeElement(CodeType value) { 1009 this.code = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return The code that identifies this filter when it is used as a filter in 1015 * [[[ValueSet]]].compose.include.filter. 1016 */ 1017 public String getCode() { 1018 return this.code == null ? null : this.code.getValue(); 1019 } 1020 1021 /** 1022 * @param value The code that identifies this filter when it is used as a filter 1023 * in [[[ValueSet]]].compose.include.filter. 1024 */ 1025 public CodeSystemFilterComponent setCode(String value) { 1026 if (this.code == null) 1027 this.code = new CodeType(); 1028 this.code.setValue(value); 1029 return this; 1030 } 1031 1032 /** 1033 * @return {@link #description} (A description of how or why the filter is 1034 * used.). This is the underlying object with id, value and extensions. 1035 * The accessor "getDescription" gives direct access to the value 1036 */ 1037 public StringType getDescriptionElement() { 1038 if (this.description == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create CodeSystemFilterComponent.description"); 1041 else if (Configuration.doAutoCreate()) 1042 this.description = new StringType(); // bb 1043 return this.description; 1044 } 1045 1046 public boolean hasDescriptionElement() { 1047 return this.description != null && !this.description.isEmpty(); 1048 } 1049 1050 public boolean hasDescription() { 1051 return this.description != null && !this.description.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #description} (A description of how or why the filter is 1056 * used.). This is the underlying object with id, value and 1057 * extensions. The accessor "getDescription" gives direct access to 1058 * the value 1059 */ 1060 public CodeSystemFilterComponent setDescriptionElement(StringType value) { 1061 this.description = value; 1062 return this; 1063 } 1064 1065 /** 1066 * @return A description of how or why the filter is used. 1067 */ 1068 public String getDescription() { 1069 return this.description == null ? null : this.description.getValue(); 1070 } 1071 1072 /** 1073 * @param value A description of how or why the filter is used. 1074 */ 1075 public CodeSystemFilterComponent setDescription(String value) { 1076 if (Utilities.noString(value)) 1077 this.description = null; 1078 else { 1079 if (this.description == null) 1080 this.description = new StringType(); 1081 this.description.setValue(value); 1082 } 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #operator} (A list of operators that can be used with the 1088 * filter.) 1089 */ 1090 public List<Enumeration<FilterOperator>> getOperator() { 1091 if (this.operator == null) 1092 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 1093 return this.operator; 1094 } 1095 1096 /** 1097 * @return Returns a reference to <code>this</code> for easy method chaining 1098 */ 1099 public CodeSystemFilterComponent setOperator(List<Enumeration<FilterOperator>> theOperator) { 1100 this.operator = theOperator; 1101 return this; 1102 } 1103 1104 public boolean hasOperator() { 1105 if (this.operator == null) 1106 return false; 1107 for (Enumeration<FilterOperator> item : this.operator) 1108 if (!item.isEmpty()) 1109 return true; 1110 return false; 1111 } 1112 1113 /** 1114 * @return {@link #operator} (A list of operators that can be used with the 1115 * filter.) 1116 */ 1117 public Enumeration<FilterOperator> addOperatorElement() {// 2 1118 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 1119 if (this.operator == null) 1120 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 1121 this.operator.add(t); 1122 return t; 1123 } 1124 1125 /** 1126 * @param value {@link #operator} (A list of operators that can be used with the 1127 * filter.) 1128 */ 1129 public CodeSystemFilterComponent addOperator(FilterOperator value) { // 1 1130 Enumeration<FilterOperator> t = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 1131 t.setValue(value); 1132 if (this.operator == null) 1133 this.operator = new ArrayList<Enumeration<FilterOperator>>(); 1134 this.operator.add(t); 1135 return this; 1136 } 1137 1138 /** 1139 * @param value {@link #operator} (A list of operators that can be used with the 1140 * filter.) 1141 */ 1142 public boolean hasOperator(FilterOperator value) { 1143 if (this.operator == null) 1144 return false; 1145 for (Enumeration<FilterOperator> v : this.operator) 1146 if (v.getValue().equals(value)) // code 1147 return true; 1148 return false; 1149 } 1150 1151 /** 1152 * @return {@link #value} (A description of what the value for the filter should 1153 * be.). This is the underlying object with id, value and extensions. 1154 * The accessor "getValue" gives direct access to the value 1155 */ 1156 public StringType getValueElement() { 1157 if (this.value == null) 1158 if (Configuration.errorOnAutoCreate()) 1159 throw new Error("Attempt to auto-create CodeSystemFilterComponent.value"); 1160 else if (Configuration.doAutoCreate()) 1161 this.value = new StringType(); // bb 1162 return this.value; 1163 } 1164 1165 public boolean hasValueElement() { 1166 return this.value != null && !this.value.isEmpty(); 1167 } 1168 1169 public boolean hasValue() { 1170 return this.value != null && !this.value.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #value} (A description of what the value for the filter 1175 * should be.). This is the underlying object with id, value and 1176 * extensions. The accessor "getValue" gives direct access to the 1177 * value 1178 */ 1179 public CodeSystemFilterComponent setValueElement(StringType value) { 1180 this.value = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return A description of what the value for the filter should be. 1186 */ 1187 public String getValue() { 1188 return this.value == null ? null : this.value.getValue(); 1189 } 1190 1191 /** 1192 * @param value A description of what the value for the filter should be. 1193 */ 1194 public CodeSystemFilterComponent setValue(String value) { 1195 if (this.value == null) 1196 this.value = new StringType(); 1197 this.value.setValue(value); 1198 return this; 1199 } 1200 1201 protected void listChildren(List<Property> children) { 1202 super.listChildren(children); 1203 children.add(new Property("code", "code", 1204 "The code that identifies this filter when it is used as a filter in [[[ValueSet]]].compose.include.filter.", 1205 0, 1, code)); 1206 children.add( 1207 new Property("description", "string", "A description of how or why the filter is used.", 0, 1, description)); 1208 children.add(new Property("operator", "code", "A list of operators that can be used with the filter.", 0, 1209 java.lang.Integer.MAX_VALUE, operator)); 1210 children.add( 1211 new Property("value", "string", "A description of what the value for the filter should be.", 0, 1, value)); 1212 } 1213 1214 @Override 1215 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1216 switch (_hash) { 1217 case 3059181: 1218 /* code */ return new Property("code", "code", 1219 "The code that identifies this filter when it is used as a filter in [[[ValueSet]]].compose.include.filter.", 1220 0, 1, code); 1221 case -1724546052: 1222 /* description */ return new Property("description", "string", 1223 "A description of how or why the filter is used.", 0, 1, description); 1224 case -500553564: 1225 /* operator */ return new Property("operator", "code", "A list of operators that can be used with the filter.", 1226 0, java.lang.Integer.MAX_VALUE, operator); 1227 case 111972721: 1228 /* value */ return new Property("value", "string", "A description of what the value for the filter should be.", 1229 0, 1, value); 1230 default: 1231 return super.getNamedProperty(_hash, _name, _checkValid); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1238 switch (hash) { 1239 case 3059181: 1240 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1241 case -1724546052: 1242 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1243 case -500553564: 1244 /* operator */ return this.operator == null ? new Base[0] 1245 : this.operator.toArray(new Base[this.operator.size()]); // Enumeration<FilterOperator> 1246 case 111972721: 1247 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 1248 default: 1249 return super.getProperty(hash, name, checkValid); 1250 } 1251 1252 } 1253 1254 @Override 1255 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1256 switch (hash) { 1257 case 3059181: // code 1258 this.code = castToCode(value); // CodeType 1259 return value; 1260 case -1724546052: // description 1261 this.description = castToString(value); // StringType 1262 return value; 1263 case -500553564: // operator 1264 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 1265 this.getOperator().add((Enumeration) value); // Enumeration<FilterOperator> 1266 return value; 1267 case 111972721: // value 1268 this.value = castToString(value); // StringType 1269 return value; 1270 default: 1271 return super.setProperty(hash, name, value); 1272 } 1273 1274 } 1275 1276 @Override 1277 public Base setProperty(String name, Base value) throws FHIRException { 1278 if (name.equals("code")) { 1279 this.code = castToCode(value); // CodeType 1280 } else if (name.equals("description")) { 1281 this.description = castToString(value); // StringType 1282 } else if (name.equals("operator")) { 1283 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 1284 this.getOperator().add((Enumeration) value); 1285 } else if (name.equals("value")) { 1286 this.value = castToString(value); // StringType 1287 } else 1288 return super.setProperty(name, value); 1289 return value; 1290 } 1291 1292 @Override 1293 public void removeChild(String name, Base value) throws FHIRException { 1294 if (name.equals("code")) { 1295 this.code = null; 1296 } else if (name.equals("description")) { 1297 this.description = null; 1298 } else if (name.equals("operator")) { 1299 this.getOperator().remove((Enumeration) value); 1300 } else if (name.equals("value")) { 1301 this.value = null; 1302 } else 1303 super.removeChild(name, value); 1304 1305 } 1306 1307 @Override 1308 public Base makeProperty(int hash, String name) throws FHIRException { 1309 switch (hash) { 1310 case 3059181: 1311 return getCodeElement(); 1312 case -1724546052: 1313 return getDescriptionElement(); 1314 case -500553564: 1315 return addOperatorElement(); 1316 case 111972721: 1317 return getValueElement(); 1318 default: 1319 return super.makeProperty(hash, name); 1320 } 1321 1322 } 1323 1324 @Override 1325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1326 switch (hash) { 1327 case 3059181: 1328 /* code */ return new String[] { "code" }; 1329 case -1724546052: 1330 /* description */ return new String[] { "string" }; 1331 case -500553564: 1332 /* operator */ return new String[] { "code" }; 1333 case 111972721: 1334 /* value */ return new String[] { "string" }; 1335 default: 1336 return super.getTypesForProperty(hash, name); 1337 } 1338 1339 } 1340 1341 @Override 1342 public Base addChild(String name) throws FHIRException { 1343 if (name.equals("code")) { 1344 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 1345 } else if (name.equals("description")) { 1346 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 1347 } else if (name.equals("operator")) { 1348 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.operator"); 1349 } else if (name.equals("value")) { 1350 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.value"); 1351 } else 1352 return super.addChild(name); 1353 } 1354 1355 public CodeSystemFilterComponent copy() { 1356 CodeSystemFilterComponent dst = new CodeSystemFilterComponent(); 1357 copyValues(dst); 1358 return dst; 1359 } 1360 1361 public void copyValues(CodeSystemFilterComponent dst) { 1362 super.copyValues(dst); 1363 dst.code = code == null ? null : code.copy(); 1364 dst.description = description == null ? null : description.copy(); 1365 if (operator != null) { 1366 dst.operator = new ArrayList<Enumeration<FilterOperator>>(); 1367 for (Enumeration<FilterOperator> i : operator) 1368 dst.operator.add(i.copy()); 1369 } 1370 ; 1371 dst.value = value == null ? null : value.copy(); 1372 } 1373 1374 @Override 1375 public boolean equalsDeep(Base other_) { 1376 if (!super.equalsDeep(other_)) 1377 return false; 1378 if (!(other_ instanceof CodeSystemFilterComponent)) 1379 return false; 1380 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 1381 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1382 && compareDeep(operator, o.operator, true) && compareDeep(value, o.value, true); 1383 } 1384 1385 @Override 1386 public boolean equalsShallow(Base other_) { 1387 if (!super.equalsShallow(other_)) 1388 return false; 1389 if (!(other_ instanceof CodeSystemFilterComponent)) 1390 return false; 1391 CodeSystemFilterComponent o = (CodeSystemFilterComponent) other_; 1392 return compareValues(code, o.code, true) && compareValues(description, o.description, true) 1393 && compareValues(operator, o.operator, true) && compareValues(value, o.value, true); 1394 } 1395 1396 public boolean isEmpty() { 1397 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, operator, value); 1398 } 1399 1400 public String fhirType() { 1401 return "CodeSystem.filter"; 1402 1403 } 1404 1405 } 1406 1407 @Block() 1408 public static class PropertyComponent extends BackboneElement implements IBaseBackboneElement { 1409 /** 1410 * A code that is used to identify the property. The code is used internally (in 1411 * CodeSystem.concept.property.code) and also externally, such as in property 1412 * filters. 1413 */ 1414 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1415 @Description(shortDefinition = "Identifies the property on the concepts, and when referred to in operations", formalDefinition = "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.") 1416 protected CodeType code; 1417 1418 /** 1419 * Reference to the formal meaning of the property. One possible source of 1420 * meaning is the [Concept Properties](codesystem-concept-properties.html) code 1421 * system. 1422 */ 1423 @Child(name = "uri", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1424 @Description(shortDefinition = "Formal identifier for the property", formalDefinition = "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.") 1425 protected UriType uri; 1426 1427 /** 1428 * A description of the property- why it is defined, and how its value might be 1429 * used. 1430 */ 1431 @Child(name = "description", type = { 1432 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1433 @Description(shortDefinition = "Why the property is defined, and/or what it conveys", formalDefinition = "A description of the property- why it is defined, and how its value might be used.") 1434 protected StringType description; 1435 1436 /** 1437 * The type of the property value. Properties of type "code" contain a code 1438 * defined by the code system (e.g. a reference to another defined concept). 1439 */ 1440 @Child(name = "type", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1441 @Description(shortDefinition = "code | Coding | string | integer | boolean | dateTime | decimal", formalDefinition = "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept).") 1442 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/concept-property-type") 1443 protected Enumeration<PropertyType> type; 1444 1445 private static final long serialVersionUID = -1810713373L; 1446 1447 /** 1448 * Constructor 1449 */ 1450 public PropertyComponent() { 1451 super(); 1452 } 1453 1454 /** 1455 * Constructor 1456 */ 1457 public PropertyComponent(CodeType code, Enumeration<PropertyType> type) { 1458 super(); 1459 this.code = code; 1460 this.type = type; 1461 } 1462 1463 /** 1464 * @return {@link #code} (A code that is used to identify the property. The code 1465 * is used internally (in CodeSystem.concept.property.code) and also 1466 * externally, such as in property filters.). This is the underlying 1467 * object with id, value and extensions. The accessor "getCode" gives 1468 * direct access to the value 1469 */ 1470 public CodeType getCodeElement() { 1471 if (this.code == null) 1472 if (Configuration.errorOnAutoCreate()) 1473 throw new Error("Attempt to auto-create PropertyComponent.code"); 1474 else if (Configuration.doAutoCreate()) 1475 this.code = new CodeType(); // bb 1476 return this.code; 1477 } 1478 1479 public boolean hasCodeElement() { 1480 return this.code != null && !this.code.isEmpty(); 1481 } 1482 1483 public boolean hasCode() { 1484 return this.code != null && !this.code.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #code} (A code that is used to identify the property. The 1489 * code is used internally (in CodeSystem.concept.property.code) 1490 * and also externally, such as in property filters.). This is the 1491 * underlying object with id, value and extensions. The accessor 1492 * "getCode" gives direct access to the value 1493 */ 1494 public PropertyComponent setCodeElement(CodeType value) { 1495 this.code = value; 1496 return this; 1497 } 1498 1499 /** 1500 * @return A code that is used to identify the property. The code is used 1501 * internally (in CodeSystem.concept.property.code) and also externally, 1502 * such as in property filters. 1503 */ 1504 public String getCode() { 1505 return this.code == null ? null : this.code.getValue(); 1506 } 1507 1508 /** 1509 * @param value A code that is used to identify the property. The code is used 1510 * internally (in CodeSystem.concept.property.code) and also 1511 * externally, such as in property filters. 1512 */ 1513 public PropertyComponent setCode(String value) { 1514 if (this.code == null) 1515 this.code = new CodeType(); 1516 this.code.setValue(value); 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #uri} (Reference to the formal meaning of the property. One 1522 * possible source of meaning is the [Concept 1523 * Properties](codesystem-concept-properties.html) code system.). This 1524 * is the underlying object with id, value and extensions. The accessor 1525 * "getUri" gives direct access to the value 1526 */ 1527 public UriType getUriElement() { 1528 if (this.uri == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create PropertyComponent.uri"); 1531 else if (Configuration.doAutoCreate()) 1532 this.uri = new UriType(); // bb 1533 return this.uri; 1534 } 1535 1536 public boolean hasUriElement() { 1537 return this.uri != null && !this.uri.isEmpty(); 1538 } 1539 1540 public boolean hasUri() { 1541 return this.uri != null && !this.uri.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #uri} (Reference to the formal meaning of the property. 1546 * One possible source of meaning is the [Concept 1547 * Properties](codesystem-concept-properties.html) code system.). 1548 * This is the underlying object with id, value and extensions. The 1549 * accessor "getUri" gives direct access to the value 1550 */ 1551 public PropertyComponent setUriElement(UriType value) { 1552 this.uri = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return Reference to the formal meaning of the property. One possible source 1558 * of meaning is the [Concept 1559 * Properties](codesystem-concept-properties.html) code system. 1560 */ 1561 public String getUri() { 1562 return this.uri == null ? null : this.uri.getValue(); 1563 } 1564 1565 /** 1566 * @param value Reference to the formal meaning of the property. One possible 1567 * source of meaning is the [Concept 1568 * Properties](codesystem-concept-properties.html) code system. 1569 */ 1570 public PropertyComponent setUri(String value) { 1571 if (Utilities.noString(value)) 1572 this.uri = null; 1573 else { 1574 if (this.uri == null) 1575 this.uri = new UriType(); 1576 this.uri.setValue(value); 1577 } 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #description} (A description of the property- why it is 1583 * defined, and how its value might be used.). This is the underlying 1584 * object with id, value and extensions. The accessor "getDescription" 1585 * gives direct access to the value 1586 */ 1587 public StringType getDescriptionElement() { 1588 if (this.description == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create PropertyComponent.description"); 1591 else if (Configuration.doAutoCreate()) 1592 this.description = new StringType(); // bb 1593 return this.description; 1594 } 1595 1596 public boolean hasDescriptionElement() { 1597 return this.description != null && !this.description.isEmpty(); 1598 } 1599 1600 public boolean hasDescription() { 1601 return this.description != null && !this.description.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #description} (A description of the property- why it is 1606 * defined, and how its value might be used.). This is the 1607 * underlying object with id, value and extensions. The accessor 1608 * "getDescription" gives direct access to the value 1609 */ 1610 public PropertyComponent setDescriptionElement(StringType value) { 1611 this.description = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return A description of the property- why it is defined, and how its value 1617 * might be used. 1618 */ 1619 public String getDescription() { 1620 return this.description == null ? null : this.description.getValue(); 1621 } 1622 1623 /** 1624 * @param value A description of the property- why it is defined, and how its 1625 * value might be used. 1626 */ 1627 public PropertyComponent setDescription(String value) { 1628 if (Utilities.noString(value)) 1629 this.description = null; 1630 else { 1631 if (this.description == null) 1632 this.description = new StringType(); 1633 this.description.setValue(value); 1634 } 1635 return this; 1636 } 1637 1638 /** 1639 * @return {@link #type} (The type of the property value. Properties of type 1640 * "code" contain a code defined by the code system (e.g. a reference to 1641 * another defined concept).). This is the underlying object with id, 1642 * value and extensions. The accessor "getType" gives direct access to 1643 * the value 1644 */ 1645 public Enumeration<PropertyType> getTypeElement() { 1646 if (this.type == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create PropertyComponent.type"); 1649 else if (Configuration.doAutoCreate()) 1650 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); // bb 1651 return this.type; 1652 } 1653 1654 public boolean hasTypeElement() { 1655 return this.type != null && !this.type.isEmpty(); 1656 } 1657 1658 public boolean hasType() { 1659 return this.type != null && !this.type.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #type} (The type of the property value. Properties of 1664 * type "code" contain a code defined by the code system (e.g. a 1665 * reference to another defined concept).). This is the underlying 1666 * object with id, value and extensions. The accessor "getType" 1667 * gives direct access to the value 1668 */ 1669 public PropertyComponent setTypeElement(Enumeration<PropertyType> value) { 1670 this.type = value; 1671 return this; 1672 } 1673 1674 /** 1675 * @return The type of the property value. Properties of type "code" contain a 1676 * code defined by the code system (e.g. a reference to another defined 1677 * concept). 1678 */ 1679 public PropertyType getType() { 1680 return this.type == null ? null : this.type.getValue(); 1681 } 1682 1683 /** 1684 * @param value The type of the property value. Properties of type "code" 1685 * contain a code defined by the code system (e.g. a reference to 1686 * another defined concept). 1687 */ 1688 public PropertyComponent setType(PropertyType value) { 1689 if (this.type == null) 1690 this.type = new Enumeration<PropertyType>(new PropertyTypeEnumFactory()); 1691 this.type.setValue(value); 1692 return this; 1693 } 1694 1695 protected void listChildren(List<Property> children) { 1696 super.listChildren(children); 1697 children.add(new Property("code", "code", 1698 "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 1699 0, 1, code)); 1700 children.add(new Property("uri", "uri", 1701 "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 1702 0, 1, uri)); 1703 children.add(new Property("description", "string", 1704 "A description of the property- why it is defined, and how its value might be used.", 0, 1, description)); 1705 children.add(new Property("type", "code", 1706 "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept).", 1707 0, 1, type)); 1708 } 1709 1710 @Override 1711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1712 switch (_hash) { 1713 case 3059181: 1714 /* code */ return new Property("code", "code", 1715 "A code that is used to identify the property. The code is used internally (in CodeSystem.concept.property.code) and also externally, such as in property filters.", 1716 0, 1, code); 1717 case 116076: 1718 /* uri */ return new Property("uri", "uri", 1719 "Reference to the formal meaning of the property. One possible source of meaning is the [Concept Properties](codesystem-concept-properties.html) code system.", 1720 0, 1, uri); 1721 case -1724546052: 1722 /* description */ return new Property("description", "string", 1723 "A description of the property- why it is defined, and how its value might be used.", 0, 1, description); 1724 case 3575610: 1725 /* type */ return new Property("type", "code", 1726 "The type of the property value. Properties of type \"code\" contain a code defined by the code system (e.g. a reference to another defined concept).", 1727 0, 1, type); 1728 default: 1729 return super.getNamedProperty(_hash, _name, _checkValid); 1730 } 1731 1732 } 1733 1734 @Override 1735 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1736 switch (hash) { 1737 case 3059181: 1738 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1739 case 116076: 1740 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // UriType 1741 case -1724546052: 1742 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1743 case 3575610: 1744 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<PropertyType> 1745 default: 1746 return super.getProperty(hash, name, checkValid); 1747 } 1748 1749 } 1750 1751 @Override 1752 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1753 switch (hash) { 1754 case 3059181: // code 1755 this.code = castToCode(value); // CodeType 1756 return value; 1757 case 116076: // uri 1758 this.uri = castToUri(value); // UriType 1759 return value; 1760 case -1724546052: // description 1761 this.description = castToString(value); // StringType 1762 return value; 1763 case 3575610: // type 1764 value = new PropertyTypeEnumFactory().fromType(castToCode(value)); 1765 this.type = (Enumeration) value; // Enumeration<PropertyType> 1766 return value; 1767 default: 1768 return super.setProperty(hash, name, value); 1769 } 1770 1771 } 1772 1773 @Override 1774 public Base setProperty(String name, Base value) throws FHIRException { 1775 if (name.equals("code")) { 1776 this.code = castToCode(value); // CodeType 1777 } else if (name.equals("uri")) { 1778 this.uri = castToUri(value); // UriType 1779 } else if (name.equals("description")) { 1780 this.description = castToString(value); // StringType 1781 } else if (name.equals("type")) { 1782 value = new PropertyTypeEnumFactory().fromType(castToCode(value)); 1783 this.type = (Enumeration) value; // Enumeration<PropertyType> 1784 } else 1785 return super.setProperty(name, value); 1786 return value; 1787 } 1788 1789 @Override 1790 public void removeChild(String name, Base value) throws FHIRException { 1791 if (name.equals("code")) { 1792 this.code = null; 1793 } else if (name.equals("uri")) { 1794 this.uri = null; 1795 } else if (name.equals("description")) { 1796 this.description = null; 1797 } else if (name.equals("type")) { 1798 this.type = null; 1799 } else 1800 super.removeChild(name, value); 1801 1802 } 1803 1804 @Override 1805 public Base makeProperty(int hash, String name) throws FHIRException { 1806 switch (hash) { 1807 case 3059181: 1808 return getCodeElement(); 1809 case 116076: 1810 return getUriElement(); 1811 case -1724546052: 1812 return getDescriptionElement(); 1813 case 3575610: 1814 return getTypeElement(); 1815 default: 1816 return super.makeProperty(hash, name); 1817 } 1818 1819 } 1820 1821 @Override 1822 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1823 switch (hash) { 1824 case 3059181: 1825 /* code */ return new String[] { "code" }; 1826 case 116076: 1827 /* uri */ return new String[] { "uri" }; 1828 case -1724546052: 1829 /* description */ return new String[] { "string" }; 1830 case 3575610: 1831 /* type */ return new String[] { "code" }; 1832 default: 1833 return super.getTypesForProperty(hash, name); 1834 } 1835 1836 } 1837 1838 @Override 1839 public Base addChild(String name) throws FHIRException { 1840 if (name.equals("code")) { 1841 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 1842 } else if (name.equals("uri")) { 1843 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.uri"); 1844 } else if (name.equals("description")) { 1845 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 1846 } else if (name.equals("type")) { 1847 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.type"); 1848 } else 1849 return super.addChild(name); 1850 } 1851 1852 public PropertyComponent copy() { 1853 PropertyComponent dst = new PropertyComponent(); 1854 copyValues(dst); 1855 return dst; 1856 } 1857 1858 public void copyValues(PropertyComponent dst) { 1859 super.copyValues(dst); 1860 dst.code = code == null ? null : code.copy(); 1861 dst.uri = uri == null ? null : uri.copy(); 1862 dst.description = description == null ? null : description.copy(); 1863 dst.type = type == null ? null : type.copy(); 1864 } 1865 1866 @Override 1867 public boolean equalsDeep(Base other_) { 1868 if (!super.equalsDeep(other_)) 1869 return false; 1870 if (!(other_ instanceof PropertyComponent)) 1871 return false; 1872 PropertyComponent o = (PropertyComponent) other_; 1873 return compareDeep(code, o.code, true) && compareDeep(uri, o.uri, true) 1874 && compareDeep(description, o.description, true) && compareDeep(type, o.type, true); 1875 } 1876 1877 @Override 1878 public boolean equalsShallow(Base other_) { 1879 if (!super.equalsShallow(other_)) 1880 return false; 1881 if (!(other_ instanceof PropertyComponent)) 1882 return false; 1883 PropertyComponent o = (PropertyComponent) other_; 1884 return compareValues(code, o.code, true) && compareValues(uri, o.uri, true) 1885 && compareValues(description, o.description, true) && compareValues(type, o.type, true); 1886 } 1887 1888 public boolean isEmpty() { 1889 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, uri, description, type); 1890 } 1891 1892 public String fhirType() { 1893 return "CodeSystem.property"; 1894 1895 } 1896 1897 } 1898 1899 @Block() 1900 public static class ConceptDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 1901 /** 1902 * A code - a text symbol - that uniquely identifies the concept within the code 1903 * system. 1904 */ 1905 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1906 @Description(shortDefinition = "Code that identifies concept", formalDefinition = "A code - a text symbol - that uniquely identifies the concept within the code system.") 1907 protected CodeType code; 1908 1909 /** 1910 * A human readable string that is the recommended default way to present this 1911 * concept to a user. 1912 */ 1913 @Child(name = "display", type = { 1914 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1915 @Description(shortDefinition = "Text to display to the user", formalDefinition = "A human readable string that is the recommended default way to present this concept to a user.") 1916 protected StringType display; 1917 1918 /** 1919 * The formal definition of the concept. The code system resource does not make 1920 * formal definitions required, because of the prevalence of legacy systems. 1921 * However, they are highly recommended, as without them there is no formal 1922 * meaning associated with the concept. 1923 */ 1924 @Child(name = "definition", type = { 1925 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1926 @Description(shortDefinition = "Formal definition", formalDefinition = "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.") 1927 protected StringType definition; 1928 1929 /** 1930 * Additional representations for the concept - other languages, aliases, 1931 * specialized purposes, used for particular purposes, etc. 1932 */ 1933 @Child(name = "designation", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1934 @Description(shortDefinition = "Additional representations for the concept", formalDefinition = "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.") 1935 protected List<ConceptDefinitionDesignationComponent> designation; 1936 1937 /** 1938 * A property value for this concept. 1939 */ 1940 @Child(name = "property", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1941 @Description(shortDefinition = "Property value for the concept", formalDefinition = "A property value for this concept.") 1942 protected List<ConceptPropertyComponent> property; 1943 1944 /** 1945 * Defines children of a concept to produce a hierarchy of concepts. The nature 1946 * of the relationships is variable (is-a/contains/categorizes) - see 1947 * hierarchyMeaning. 1948 */ 1949 @Child(name = "concept", type = { 1950 ConceptDefinitionComponent.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1951 @Description(shortDefinition = "Child Concepts (is-a/contains/categorizes)", formalDefinition = "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.") 1952 protected List<ConceptDefinitionComponent> concept; 1953 1954 private static final long serialVersionUID = 878320988L; 1955 1956 /** 1957 * Constructor 1958 */ 1959 public ConceptDefinitionComponent() { 1960 super(); 1961 } 1962 1963 /** 1964 * Constructor 1965 */ 1966 public ConceptDefinitionComponent(CodeType code) { 1967 super(); 1968 this.code = code; 1969 } 1970 1971 /** 1972 * @return {@link #code} (A code - a text symbol - that uniquely identifies the 1973 * concept within the code system.). This is the underlying object with 1974 * id, value and extensions. The accessor "getCode" gives direct access 1975 * to the value 1976 */ 1977 public CodeType getCodeElement() { 1978 if (this.code == null) 1979 if (Configuration.errorOnAutoCreate()) 1980 throw new Error("Attempt to auto-create ConceptDefinitionComponent.code"); 1981 else if (Configuration.doAutoCreate()) 1982 this.code = new CodeType(); // bb 1983 return this.code; 1984 } 1985 1986 public boolean hasCodeElement() { 1987 return this.code != null && !this.code.isEmpty(); 1988 } 1989 1990 public boolean hasCode() { 1991 return this.code != null && !this.code.isEmpty(); 1992 } 1993 1994 /** 1995 * @param value {@link #code} (A code - a text symbol - that uniquely identifies 1996 * the concept within the code system.). This is the underlying 1997 * object with id, value and extensions. The accessor "getCode" 1998 * gives direct access to the value 1999 */ 2000 public ConceptDefinitionComponent setCodeElement(CodeType value) { 2001 this.code = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return A code - a text symbol - that uniquely identifies the concept within 2007 * the code system. 2008 */ 2009 public String getCode() { 2010 return this.code == null ? null : this.code.getValue(); 2011 } 2012 2013 /** 2014 * @param value A code - a text symbol - that uniquely identifies the concept 2015 * within the code system. 2016 */ 2017 public ConceptDefinitionComponent setCode(String value) { 2018 if (this.code == null) 2019 this.code = new CodeType(); 2020 this.code.setValue(value); 2021 return this; 2022 } 2023 2024 /** 2025 * @return {@link #display} (A human readable string that is the recommended 2026 * default way to present this concept to a user.). This is the 2027 * underlying object with id, value and extensions. The accessor 2028 * "getDisplay" gives direct access to the value 2029 */ 2030 public StringType getDisplayElement() { 2031 if (this.display == null) 2032 if (Configuration.errorOnAutoCreate()) 2033 throw new Error("Attempt to auto-create ConceptDefinitionComponent.display"); 2034 else if (Configuration.doAutoCreate()) 2035 this.display = new StringType(); // bb 2036 return this.display; 2037 } 2038 2039 public boolean hasDisplayElement() { 2040 return this.display != null && !this.display.isEmpty(); 2041 } 2042 2043 public boolean hasDisplay() { 2044 return this.display != null && !this.display.isEmpty(); 2045 } 2046 2047 /** 2048 * @param value {@link #display} (A human readable string that is the 2049 * recommended default way to present this concept to a user.). 2050 * This is the underlying object with id, value and extensions. The 2051 * accessor "getDisplay" gives direct access to the value 2052 */ 2053 public ConceptDefinitionComponent setDisplayElement(StringType value) { 2054 this.display = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return A human readable string that is the recommended default way to 2060 * present this concept to a user. 2061 */ 2062 public String getDisplay() { 2063 return this.display == null ? null : this.display.getValue(); 2064 } 2065 2066 /** 2067 * @param value A human readable string that is the recommended default way to 2068 * present this concept to a user. 2069 */ 2070 public ConceptDefinitionComponent setDisplay(String value) { 2071 if (Utilities.noString(value)) 2072 this.display = null; 2073 else { 2074 if (this.display == null) 2075 this.display = new StringType(); 2076 this.display.setValue(value); 2077 } 2078 return this; 2079 } 2080 2081 /** 2082 * @return {@link #definition} (The formal definition of the concept. The code 2083 * system resource does not make formal definitions required, because of 2084 * the prevalence of legacy systems. However, they are highly 2085 * recommended, as without them there is no formal meaning associated 2086 * with the concept.). This is the underlying object with id, value and 2087 * extensions. The accessor "getDefinition" gives direct access to the 2088 * value 2089 */ 2090 public StringType getDefinitionElement() { 2091 if (this.definition == null) 2092 if (Configuration.errorOnAutoCreate()) 2093 throw new Error("Attempt to auto-create ConceptDefinitionComponent.definition"); 2094 else if (Configuration.doAutoCreate()) 2095 this.definition = new StringType(); // bb 2096 return this.definition; 2097 } 2098 2099 public boolean hasDefinitionElement() { 2100 return this.definition != null && !this.definition.isEmpty(); 2101 } 2102 2103 public boolean hasDefinition() { 2104 return this.definition != null && !this.definition.isEmpty(); 2105 } 2106 2107 /** 2108 * @param value {@link #definition} (The formal definition of the concept. The 2109 * code system resource does not make formal definitions required, 2110 * because of the prevalence of legacy systems. However, they are 2111 * highly recommended, as without them there is no formal meaning 2112 * associated with the concept.). This is the underlying object 2113 * with id, value and extensions. The accessor "getDefinition" 2114 * gives direct access to the value 2115 */ 2116 public ConceptDefinitionComponent setDefinitionElement(StringType value) { 2117 this.definition = value; 2118 return this; 2119 } 2120 2121 /** 2122 * @return The formal definition of the concept. The code system resource does 2123 * not make formal definitions required, because of the prevalence of 2124 * legacy systems. However, they are highly recommended, as without them 2125 * there is no formal meaning associated with the concept. 2126 */ 2127 public String getDefinition() { 2128 return this.definition == null ? null : this.definition.getValue(); 2129 } 2130 2131 /** 2132 * @param value The formal definition of the concept. The code system resource 2133 * does not make formal definitions required, because of the 2134 * prevalence of legacy systems. However, they are highly 2135 * recommended, as without them there is no formal meaning 2136 * associated with the concept. 2137 */ 2138 public ConceptDefinitionComponent setDefinition(String value) { 2139 if (Utilities.noString(value)) 2140 this.definition = null; 2141 else { 2142 if (this.definition == null) 2143 this.definition = new StringType(); 2144 this.definition.setValue(value); 2145 } 2146 return this; 2147 } 2148 2149 /** 2150 * @return {@link #designation} (Additional representations for the concept - 2151 * other languages, aliases, specialized purposes, used for particular 2152 * purposes, etc.) 2153 */ 2154 public List<ConceptDefinitionDesignationComponent> getDesignation() { 2155 if (this.designation == null) 2156 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2157 return this.designation; 2158 } 2159 2160 /** 2161 * @return Returns a reference to <code>this</code> for easy method chaining 2162 */ 2163 public ConceptDefinitionComponent setDesignation(List<ConceptDefinitionDesignationComponent> theDesignation) { 2164 this.designation = theDesignation; 2165 return this; 2166 } 2167 2168 public boolean hasDesignation() { 2169 if (this.designation == null) 2170 return false; 2171 for (ConceptDefinitionDesignationComponent item : this.designation) 2172 if (!item.isEmpty()) 2173 return true; 2174 return false; 2175 } 2176 2177 public ConceptDefinitionDesignationComponent addDesignation() { // 3 2178 ConceptDefinitionDesignationComponent t = new ConceptDefinitionDesignationComponent(); 2179 if (this.designation == null) 2180 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2181 this.designation.add(t); 2182 return t; 2183 } 2184 2185 public ConceptDefinitionComponent addDesignation(ConceptDefinitionDesignationComponent t) { // 3 2186 if (t == null) 2187 return this; 2188 if (this.designation == null) 2189 this.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2190 this.designation.add(t); 2191 return this; 2192 } 2193 2194 /** 2195 * @return The first repetition of repeating field {@link #designation}, 2196 * creating it if it does not already exist 2197 */ 2198 public ConceptDefinitionDesignationComponent getDesignationFirstRep() { 2199 if (getDesignation().isEmpty()) { 2200 addDesignation(); 2201 } 2202 return getDesignation().get(0); 2203 } 2204 2205 /** 2206 * @return {@link #property} (A property value for this concept.) 2207 */ 2208 public List<ConceptPropertyComponent> getProperty() { 2209 if (this.property == null) 2210 this.property = new ArrayList<ConceptPropertyComponent>(); 2211 return this.property; 2212 } 2213 2214 /** 2215 * @return Returns a reference to <code>this</code> for easy method chaining 2216 */ 2217 public ConceptDefinitionComponent setProperty(List<ConceptPropertyComponent> theProperty) { 2218 this.property = theProperty; 2219 return this; 2220 } 2221 2222 public boolean hasProperty() { 2223 if (this.property == null) 2224 return false; 2225 for (ConceptPropertyComponent item : this.property) 2226 if (!item.isEmpty()) 2227 return true; 2228 return false; 2229 } 2230 2231 public ConceptPropertyComponent addProperty() { // 3 2232 ConceptPropertyComponent t = new ConceptPropertyComponent(); 2233 if (this.property == null) 2234 this.property = new ArrayList<ConceptPropertyComponent>(); 2235 this.property.add(t); 2236 return t; 2237 } 2238 2239 public ConceptDefinitionComponent addProperty(ConceptPropertyComponent t) { // 3 2240 if (t == null) 2241 return this; 2242 if (this.property == null) 2243 this.property = new ArrayList<ConceptPropertyComponent>(); 2244 this.property.add(t); 2245 return this; 2246 } 2247 2248 /** 2249 * @return The first repetition of repeating field {@link #property}, creating 2250 * it if it does not already exist 2251 */ 2252 public ConceptPropertyComponent getPropertyFirstRep() { 2253 if (getProperty().isEmpty()) { 2254 addProperty(); 2255 } 2256 return getProperty().get(0); 2257 } 2258 2259 /** 2260 * @return {@link #concept} (Defines children of a concept to produce a 2261 * hierarchy of concepts. The nature of the relationships is variable 2262 * (is-a/contains/categorizes) - see hierarchyMeaning.) 2263 */ 2264 public List<ConceptDefinitionComponent> getConcept() { 2265 if (this.concept == null) 2266 this.concept = new ArrayList<ConceptDefinitionComponent>(); 2267 return this.concept; 2268 } 2269 2270 /** 2271 * @return Returns a reference to <code>this</code> for easy method chaining 2272 */ 2273 public ConceptDefinitionComponent setConcept(List<ConceptDefinitionComponent> theConcept) { 2274 this.concept = theConcept; 2275 return this; 2276 } 2277 2278 public boolean hasConcept() { 2279 if (this.concept == null) 2280 return false; 2281 for (ConceptDefinitionComponent item : this.concept) 2282 if (!item.isEmpty()) 2283 return true; 2284 return false; 2285 } 2286 2287 public ConceptDefinitionComponent addConcept() { // 3 2288 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 2289 if (this.concept == null) 2290 this.concept = new ArrayList<ConceptDefinitionComponent>(); 2291 this.concept.add(t); 2292 return t; 2293 } 2294 2295 public ConceptDefinitionComponent addConcept(ConceptDefinitionComponent t) { // 3 2296 if (t == null) 2297 return this; 2298 if (this.concept == null) 2299 this.concept = new ArrayList<ConceptDefinitionComponent>(); 2300 this.concept.add(t); 2301 return this; 2302 } 2303 2304 /** 2305 * @return The first repetition of repeating field {@link #concept}, creating it 2306 * if it does not already exist 2307 */ 2308 public ConceptDefinitionComponent getConceptFirstRep() { 2309 if (getConcept().isEmpty()) { 2310 addConcept(); 2311 } 2312 return getConcept().get(0); 2313 } 2314 2315 protected void listChildren(List<Property> children) { 2316 super.listChildren(children); 2317 children.add(new Property("code", "code", 2318 "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code)); 2319 children.add(new Property("display", "string", 2320 "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, 2321 display)); 2322 children.add(new Property("definition", "string", 2323 "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 2324 0, 1, definition)); 2325 children.add(new Property("designation", "", 2326 "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 2327 0, java.lang.Integer.MAX_VALUE, designation)); 2328 children.add( 2329 new Property("property", "", "A property value for this concept.", 0, java.lang.Integer.MAX_VALUE, property)); 2330 children.add(new Property("concept", "@CodeSystem.concept", 2331 "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 2332 0, java.lang.Integer.MAX_VALUE, concept)); 2333 } 2334 2335 @Override 2336 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2337 switch (_hash) { 2338 case 3059181: 2339 /* code */ return new Property("code", "code", 2340 "A code - a text symbol - that uniquely identifies the concept within the code system.", 0, 1, code); 2341 case 1671764162: 2342 /* display */ return new Property("display", "string", 2343 "A human readable string that is the recommended default way to present this concept to a user.", 0, 1, 2344 display); 2345 case -1014418093: 2346 /* definition */ return new Property("definition", "string", 2347 "The formal definition of the concept. The code system resource does not make formal definitions required, because of the prevalence of legacy systems. However, they are highly recommended, as without them there is no formal meaning associated with the concept.", 2348 0, 1, definition); 2349 case -900931593: 2350 /* designation */ return new Property("designation", "", 2351 "Additional representations for the concept - other languages, aliases, specialized purposes, used for particular purposes, etc.", 2352 0, java.lang.Integer.MAX_VALUE, designation); 2353 case -993141291: 2354 /* property */ return new Property("property", "", "A property value for this concept.", 0, 2355 java.lang.Integer.MAX_VALUE, property); 2356 case 951024232: 2357 /* concept */ return new Property("concept", "@CodeSystem.concept", 2358 "Defines children of a concept to produce a hierarchy of concepts. The nature of the relationships is variable (is-a/contains/categorizes) - see hierarchyMeaning.", 2359 0, java.lang.Integer.MAX_VALUE, concept); 2360 default: 2361 return super.getNamedProperty(_hash, _name, _checkValid); 2362 } 2363 2364 } 2365 2366 @Override 2367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2368 switch (hash) { 2369 case 3059181: 2370 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 2371 case 1671764162: 2372 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 2373 case -1014418093: 2374 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // StringType 2375 case -900931593: 2376 /* designation */ return this.designation == null ? new Base[0] 2377 : this.designation.toArray(new Base[this.designation.size()]); // ConceptDefinitionDesignationComponent 2378 case -993141291: 2379 /* property */ return this.property == null ? new Base[0] 2380 : this.property.toArray(new Base[this.property.size()]); // ConceptPropertyComponent 2381 case 951024232: 2382 /* concept */ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 2383 default: 2384 return super.getProperty(hash, name, checkValid); 2385 } 2386 2387 } 2388 2389 @Override 2390 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2391 switch (hash) { 2392 case 3059181: // code 2393 this.code = castToCode(value); // CodeType 2394 return value; 2395 case 1671764162: // display 2396 this.display = castToString(value); // StringType 2397 return value; 2398 case -1014418093: // definition 2399 this.definition = castToString(value); // StringType 2400 return value; 2401 case -900931593: // designation 2402 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); // ConceptDefinitionDesignationComponent 2403 return value; 2404 case -993141291: // property 2405 this.getProperty().add((ConceptPropertyComponent) value); // ConceptPropertyComponent 2406 return value; 2407 case 951024232: // concept 2408 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 2409 return value; 2410 default: 2411 return super.setProperty(hash, name, value); 2412 } 2413 2414 } 2415 2416 @Override 2417 public Base setProperty(String name, Base value) throws FHIRException { 2418 if (name.equals("code")) { 2419 this.code = castToCode(value); // CodeType 2420 } else if (name.equals("display")) { 2421 this.display = castToString(value); // StringType 2422 } else if (name.equals("definition")) { 2423 this.definition = castToString(value); // StringType 2424 } else if (name.equals("designation")) { 2425 this.getDesignation().add((ConceptDefinitionDesignationComponent) value); 2426 } else if (name.equals("property")) { 2427 this.getProperty().add((ConceptPropertyComponent) value); 2428 } else if (name.equals("concept")) { 2429 this.getConcept().add((ConceptDefinitionComponent) value); 2430 } else 2431 return super.setProperty(name, value); 2432 return value; 2433 } 2434 2435 @Override 2436 public void removeChild(String name, Base value) throws FHIRException { 2437 if (name.equals("code")) { 2438 this.code = null; 2439 } else if (name.equals("display")) { 2440 this.display = null; 2441 } else if (name.equals("definition")) { 2442 this.definition = null; 2443 } else if (name.equals("designation")) { 2444 this.getDesignation().remove((ConceptDefinitionDesignationComponent) value); 2445 } else if (name.equals("property")) { 2446 this.getProperty().remove((ConceptPropertyComponent) value); 2447 } else if (name.equals("concept")) { 2448 this.getConcept().remove((ConceptDefinitionComponent) value); 2449 } else 2450 super.removeChild(name, value); 2451 2452 } 2453 2454 @Override 2455 public Base makeProperty(int hash, String name) throws FHIRException { 2456 switch (hash) { 2457 case 3059181: 2458 return getCodeElement(); 2459 case 1671764162: 2460 return getDisplayElement(); 2461 case -1014418093: 2462 return getDefinitionElement(); 2463 case -900931593: 2464 return addDesignation(); 2465 case -993141291: 2466 return addProperty(); 2467 case 951024232: 2468 return addConcept(); 2469 default: 2470 return super.makeProperty(hash, name); 2471 } 2472 2473 } 2474 2475 @Override 2476 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2477 switch (hash) { 2478 case 3059181: 2479 /* code */ return new String[] { "code" }; 2480 case 1671764162: 2481 /* display */ return new String[] { "string" }; 2482 case -1014418093: 2483 /* definition */ return new String[] { "string" }; 2484 case -900931593: 2485 /* designation */ return new String[] {}; 2486 case -993141291: 2487 /* property */ return new String[] {}; 2488 case 951024232: 2489 /* concept */ return new String[] { "@CodeSystem.concept" }; 2490 default: 2491 return super.getTypesForProperty(hash, name); 2492 } 2493 2494 } 2495 2496 @Override 2497 public Base addChild(String name) throws FHIRException { 2498 if (name.equals("code")) { 2499 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 2500 } else if (name.equals("display")) { 2501 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.display"); 2502 } else if (name.equals("definition")) { 2503 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.definition"); 2504 } else if (name.equals("designation")) { 2505 return addDesignation(); 2506 } else if (name.equals("property")) { 2507 return addProperty(); 2508 } else if (name.equals("concept")) { 2509 return addConcept(); 2510 } else 2511 return super.addChild(name); 2512 } 2513 2514 public ConceptDefinitionComponent copy() { 2515 ConceptDefinitionComponent dst = new ConceptDefinitionComponent(); 2516 copyValues(dst); 2517 return dst; 2518 } 2519 2520 public void copyValues(ConceptDefinitionComponent dst) { 2521 super.copyValues(dst); 2522 dst.code = code == null ? null : code.copy(); 2523 dst.display = display == null ? null : display.copy(); 2524 dst.definition = definition == null ? null : definition.copy(); 2525 if (designation != null) { 2526 dst.designation = new ArrayList<ConceptDefinitionDesignationComponent>(); 2527 for (ConceptDefinitionDesignationComponent i : designation) 2528 dst.designation.add(i.copy()); 2529 } 2530 ; 2531 if (property != null) { 2532 dst.property = new ArrayList<ConceptPropertyComponent>(); 2533 for (ConceptPropertyComponent i : property) 2534 dst.property.add(i.copy()); 2535 } 2536 ; 2537 if (concept != null) { 2538 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 2539 for (ConceptDefinitionComponent i : concept) 2540 dst.concept.add(i.copy()); 2541 } 2542 ; 2543 } 2544 2545 @Override 2546 public boolean equalsDeep(Base other_) { 2547 if (!super.equalsDeep(other_)) 2548 return false; 2549 if (!(other_ instanceof ConceptDefinitionComponent)) 2550 return false; 2551 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 2552 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 2553 && compareDeep(definition, o.definition, true) && compareDeep(designation, o.designation, true) 2554 && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true); 2555 } 2556 2557 @Override 2558 public boolean equalsShallow(Base other_) { 2559 if (!super.equalsShallow(other_)) 2560 return false; 2561 if (!(other_ instanceof ConceptDefinitionComponent)) 2562 return false; 2563 ConceptDefinitionComponent o = (ConceptDefinitionComponent) other_; 2564 return compareValues(code, o.code, true) && compareValues(display, o.display, true) 2565 && compareValues(definition, o.definition, true); 2566 } 2567 2568 public boolean isEmpty() { 2569 return super.isEmpty() 2570 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, definition, designation, property, concept); 2571 } 2572 2573 public String fhirType() { 2574 return "CodeSystem.concept"; 2575 2576 } 2577 2578 public ConceptPropertyComponent forceProperty(String code) { 2579 for (ConceptPropertyComponent prop : getProperty()) { 2580 if (code.equals(prop.getCode())) { 2581 return prop; 2582 } 2583 } 2584 return addProperty().setCode(code); 2585 } 2586 2587 } 2588 2589 @Block() 2590 public static class ConceptDefinitionDesignationComponent extends BackboneElement implements IBaseBackboneElement { 2591 /** 2592 * The language this designation is defined for. 2593 */ 2594 @Child(name = "language", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2595 @Description(shortDefinition = "Human language of the designation", formalDefinition = "The language this designation is defined for.") 2596 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 2597 protected CodeType language; 2598 2599 /** 2600 * A code that details how this designation would be used. 2601 */ 2602 @Child(name = "use", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2603 @Description(shortDefinition = "Details how this designation would be used", formalDefinition = "A code that details how this designation would be used.") 2604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/designation-use") 2605 protected Coding use; 2606 2607 /** 2608 * The text value for this designation. 2609 */ 2610 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 2611 @Description(shortDefinition = "The text value for this designation", formalDefinition = "The text value for this designation.") 2612 protected StringType value; 2613 2614 private static final long serialVersionUID = 1515662414L; 2615 2616 /** 2617 * Constructor 2618 */ 2619 public ConceptDefinitionDesignationComponent() { 2620 super(); 2621 } 2622 2623 /** 2624 * Constructor 2625 */ 2626 public ConceptDefinitionDesignationComponent(StringType value) { 2627 super(); 2628 this.value = value; 2629 } 2630 2631 /** 2632 * @return {@link #language} (The language this designation is defined for.). 2633 * This is the underlying object with id, value and extensions. The 2634 * accessor "getLanguage" gives direct access to the value 2635 */ 2636 public CodeType getLanguageElement() { 2637 if (this.language == null) 2638 if (Configuration.errorOnAutoCreate()) 2639 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.language"); 2640 else if (Configuration.doAutoCreate()) 2641 this.language = new CodeType(); // bb 2642 return this.language; 2643 } 2644 2645 public boolean hasLanguageElement() { 2646 return this.language != null && !this.language.isEmpty(); 2647 } 2648 2649 public boolean hasLanguage() { 2650 return this.language != null && !this.language.isEmpty(); 2651 } 2652 2653 /** 2654 * @param value {@link #language} (The language this designation is defined 2655 * for.). This is the underlying object with id, value and 2656 * extensions. The accessor "getLanguage" gives direct access to 2657 * the value 2658 */ 2659 public ConceptDefinitionDesignationComponent setLanguageElement(CodeType value) { 2660 this.language = value; 2661 return this; 2662 } 2663 2664 /** 2665 * @return The language this designation is defined for. 2666 */ 2667 public String getLanguage() { 2668 return this.language == null ? null : this.language.getValue(); 2669 } 2670 2671 /** 2672 * @param value The language this designation is defined for. 2673 */ 2674 public ConceptDefinitionDesignationComponent setLanguage(String value) { 2675 if (Utilities.noString(value)) 2676 this.language = null; 2677 else { 2678 if (this.language == null) 2679 this.language = new CodeType(); 2680 this.language.setValue(value); 2681 } 2682 return this; 2683 } 2684 2685 /** 2686 * @return {@link #use} (A code that details how this designation would be 2687 * used.) 2688 */ 2689 public Coding getUse() { 2690 if (this.use == null) 2691 if (Configuration.errorOnAutoCreate()) 2692 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.use"); 2693 else if (Configuration.doAutoCreate()) 2694 this.use = new Coding(); // cc 2695 return this.use; 2696 } 2697 2698 public boolean hasUse() { 2699 return this.use != null && !this.use.isEmpty(); 2700 } 2701 2702 /** 2703 * @param value {@link #use} (A code that details how this designation would be 2704 * used.) 2705 */ 2706 public ConceptDefinitionDesignationComponent setUse(Coding value) { 2707 this.use = value; 2708 return this; 2709 } 2710 2711 /** 2712 * @return {@link #value} (The text value for this designation.). This is the 2713 * underlying object with id, value and extensions. The accessor 2714 * "getValue" gives direct access to the value 2715 */ 2716 public StringType getValueElement() { 2717 if (this.value == null) 2718 if (Configuration.errorOnAutoCreate()) 2719 throw new Error("Attempt to auto-create ConceptDefinitionDesignationComponent.value"); 2720 else if (Configuration.doAutoCreate()) 2721 this.value = new StringType(); // bb 2722 return this.value; 2723 } 2724 2725 public boolean hasValueElement() { 2726 return this.value != null && !this.value.isEmpty(); 2727 } 2728 2729 public boolean hasValue() { 2730 return this.value != null && !this.value.isEmpty(); 2731 } 2732 2733 /** 2734 * @param value {@link #value} (The text value for this designation.). This is 2735 * the underlying object with id, value and extensions. The 2736 * accessor "getValue" gives direct access to the value 2737 */ 2738 public ConceptDefinitionDesignationComponent setValueElement(StringType value) { 2739 this.value = value; 2740 return this; 2741 } 2742 2743 /** 2744 * @return The text value for this designation. 2745 */ 2746 public String getValue() { 2747 return this.value == null ? null : this.value.getValue(); 2748 } 2749 2750 /** 2751 * @param value The text value for this designation. 2752 */ 2753 public ConceptDefinitionDesignationComponent setValue(String value) { 2754 if (this.value == null) 2755 this.value = new StringType(); 2756 this.value.setValue(value); 2757 return this; 2758 } 2759 2760 protected void listChildren(List<Property> children) { 2761 super.listChildren(children); 2762 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 2763 children.add(new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use)); 2764 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 2765 } 2766 2767 @Override 2768 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2769 switch (_hash) { 2770 case -1613589672: 2771 /* language */ return new Property("language", "code", "The language this designation is defined for.", 0, 1, 2772 language); 2773 case 116103: 2774 /* use */ return new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, 2775 use); 2776 case 111972721: 2777 /* value */ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 2778 default: 2779 return super.getNamedProperty(_hash, _name, _checkValid); 2780 } 2781 2782 } 2783 2784 @Override 2785 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2786 switch (hash) { 2787 case -1613589672: 2788 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 2789 case 116103: 2790 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Coding 2791 case 111972721: 2792 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2793 default: 2794 return super.getProperty(hash, name, checkValid); 2795 } 2796 2797 } 2798 2799 @Override 2800 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2801 switch (hash) { 2802 case -1613589672: // language 2803 this.language = castToCode(value); // CodeType 2804 return value; 2805 case 116103: // use 2806 this.use = castToCoding(value); // Coding 2807 return value; 2808 case 111972721: // value 2809 this.value = castToString(value); // StringType 2810 return value; 2811 default: 2812 return super.setProperty(hash, name, value); 2813 } 2814 2815 } 2816 2817 @Override 2818 public Base setProperty(String name, Base value) throws FHIRException { 2819 if (name.equals("language")) { 2820 this.language = castToCode(value); // CodeType 2821 } else if (name.equals("use")) { 2822 this.use = castToCoding(value); // Coding 2823 } else if (name.equals("value")) { 2824 this.value = castToString(value); // StringType 2825 } else 2826 return super.setProperty(name, value); 2827 return value; 2828 } 2829 2830 @Override 2831 public void removeChild(String name, Base value) throws FHIRException { 2832 if (name.equals("language")) { 2833 this.language = null; 2834 } else if (name.equals("use")) { 2835 this.use = null; 2836 } else if (name.equals("value")) { 2837 this.value = null; 2838 } else 2839 super.removeChild(name, value); 2840 2841 } 2842 2843 @Override 2844 public Base makeProperty(int hash, String name) throws FHIRException { 2845 switch (hash) { 2846 case -1613589672: 2847 return getLanguageElement(); 2848 case 116103: 2849 return getUse(); 2850 case 111972721: 2851 return getValueElement(); 2852 default: 2853 return super.makeProperty(hash, name); 2854 } 2855 2856 } 2857 2858 @Override 2859 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2860 switch (hash) { 2861 case -1613589672: 2862 /* language */ return new String[] { "code" }; 2863 case 116103: 2864 /* use */ return new String[] { "Coding" }; 2865 case 111972721: 2866 /* value */ return new String[] { "string" }; 2867 default: 2868 return super.getTypesForProperty(hash, name); 2869 } 2870 2871 } 2872 2873 @Override 2874 public Base addChild(String name) throws FHIRException { 2875 if (name.equals("language")) { 2876 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.language"); 2877 } else if (name.equals("use")) { 2878 this.use = new Coding(); 2879 return this.use; 2880 } else if (name.equals("value")) { 2881 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.value"); 2882 } else 2883 return super.addChild(name); 2884 } 2885 2886 public ConceptDefinitionDesignationComponent copy() { 2887 ConceptDefinitionDesignationComponent dst = new ConceptDefinitionDesignationComponent(); 2888 copyValues(dst); 2889 return dst; 2890 } 2891 2892 public void copyValues(ConceptDefinitionDesignationComponent dst) { 2893 super.copyValues(dst); 2894 dst.language = language == null ? null : language.copy(); 2895 dst.use = use == null ? null : use.copy(); 2896 dst.value = value == null ? null : value.copy(); 2897 } 2898 2899 @Override 2900 public boolean equalsDeep(Base other_) { 2901 if (!super.equalsDeep(other_)) 2902 return false; 2903 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2904 return false; 2905 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2906 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) 2907 && compareDeep(value, o.value, true); 2908 } 2909 2910 @Override 2911 public boolean equalsShallow(Base other_) { 2912 if (!super.equalsShallow(other_)) 2913 return false; 2914 if (!(other_ instanceof ConceptDefinitionDesignationComponent)) 2915 return false; 2916 ConceptDefinitionDesignationComponent o = (ConceptDefinitionDesignationComponent) other_; 2917 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 2918 } 2919 2920 public boolean isEmpty() { 2921 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, value); 2922 } 2923 2924 public String fhirType() { 2925 return "CodeSystem.concept.designation"; 2926 2927 } 2928 2929 } 2930 2931 @Block() 2932 public static class ConceptPropertyComponent extends BackboneElement implements IBaseBackboneElement { 2933 /** 2934 * A code that is a reference to CodeSystem.property.code. 2935 */ 2936 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2937 @Description(shortDefinition = "Reference to CodeSystem.property.code", formalDefinition = "A code that is a reference to CodeSystem.property.code.") 2938 protected CodeType code; 2939 2940 /** 2941 * The value of this property. 2942 */ 2943 @Child(name = "value", type = { CodeType.class, Coding.class, StringType.class, IntegerType.class, 2944 BooleanType.class, DateTimeType.class, 2945 DecimalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2946 @Description(shortDefinition = "Value of the property for this concept", formalDefinition = "The value of this property.") 2947 protected Type value; 2948 2949 private static final long serialVersionUID = 1742812311L; 2950 2951 /** 2952 * Constructor 2953 */ 2954 public ConceptPropertyComponent() { 2955 super(); 2956 } 2957 2958 /** 2959 * Constructor 2960 */ 2961 public ConceptPropertyComponent(CodeType code, Type value) { 2962 super(); 2963 this.code = code; 2964 this.value = value; 2965 } 2966 2967 /** 2968 * @return {@link #code} (A code that is a reference to 2969 * CodeSystem.property.code.). This is the underlying object with id, 2970 * value and extensions. The accessor "getCode" gives direct access to 2971 * the value 2972 */ 2973 public CodeType getCodeElement() { 2974 if (this.code == null) 2975 if (Configuration.errorOnAutoCreate()) 2976 throw new Error("Attempt to auto-create ConceptPropertyComponent.code"); 2977 else if (Configuration.doAutoCreate()) 2978 this.code = new CodeType(); // bb 2979 return this.code; 2980 } 2981 2982 public boolean hasCodeElement() { 2983 return this.code != null && !this.code.isEmpty(); 2984 } 2985 2986 public boolean hasCode() { 2987 return this.code != null && !this.code.isEmpty(); 2988 } 2989 2990 /** 2991 * @param value {@link #code} (A code that is a reference to 2992 * CodeSystem.property.code.). This is the underlying object with 2993 * id, value and extensions. The accessor "getCode" gives direct 2994 * access to the value 2995 */ 2996 public ConceptPropertyComponent setCodeElement(CodeType value) { 2997 this.code = value; 2998 return this; 2999 } 3000 3001 /** 3002 * @return A code that is a reference to CodeSystem.property.code. 3003 */ 3004 public String getCode() { 3005 return this.code == null ? null : this.code.getValue(); 3006 } 3007 3008 /** 3009 * @param value A code that is a reference to CodeSystem.property.code. 3010 */ 3011 public ConceptPropertyComponent setCode(String value) { 3012 if (this.code == null) 3013 this.code = new CodeType(); 3014 this.code.setValue(value); 3015 return this; 3016 } 3017 3018 /** 3019 * @return {@link #value} (The value of this property.) 3020 */ 3021 public Type getValue() { 3022 return this.value; 3023 } 3024 3025 /** 3026 * @return {@link #value} (The value of this property.) 3027 */ 3028 public CodeType getValueCodeType() throws FHIRException { 3029 if (this.value == null) 3030 this.value = new CodeType(); 3031 if (!(this.value instanceof CodeType)) 3032 throw new FHIRException("Type mismatch: the type CodeType was expected, but " + this.value.getClass().getName() 3033 + " was encountered"); 3034 return (CodeType) this.value; 3035 } 3036 3037 public boolean hasValueCodeType() { 3038 return this != null && this.value instanceof CodeType; 3039 } 3040 3041 /** 3042 * @return {@link #value} (The value of this property.) 3043 */ 3044 public Coding getValueCoding() throws FHIRException { 3045 if (this.value == null) 3046 this.value = new Coding(); 3047 if (!(this.value instanceof Coding)) 3048 throw new FHIRException( 3049 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 3050 return (Coding) this.value; 3051 } 3052 3053 public boolean hasValueCoding() { 3054 return this != null && this.value instanceof Coding; 3055 } 3056 3057 /** 3058 * @return {@link #value} (The value of this property.) 3059 */ 3060 public StringType getValueStringType() throws FHIRException { 3061 if (this.value == null) 3062 this.value = new StringType(); 3063 if (!(this.value instanceof StringType)) 3064 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3065 + this.value.getClass().getName() + " was encountered"); 3066 return (StringType) this.value; 3067 } 3068 3069 public boolean hasValueStringType() { 3070 return this != null && this.value instanceof StringType; 3071 } 3072 3073 /** 3074 * @return {@link #value} (The value of this property.) 3075 */ 3076 public IntegerType getValueIntegerType() throws FHIRException { 3077 if (this.value == null) 3078 this.value = new IntegerType(); 3079 if (!(this.value instanceof IntegerType)) 3080 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 3081 + this.value.getClass().getName() + " was encountered"); 3082 return (IntegerType) this.value; 3083 } 3084 3085 public boolean hasValueIntegerType() { 3086 return this != null && this.value instanceof IntegerType; 3087 } 3088 3089 /** 3090 * @return {@link #value} (The value of this property.) 3091 */ 3092 public BooleanType getValueBooleanType() throws FHIRException { 3093 if (this.value == null) 3094 this.value = new BooleanType(); 3095 if (!(this.value instanceof BooleanType)) 3096 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 3097 + this.value.getClass().getName() + " was encountered"); 3098 return (BooleanType) this.value; 3099 } 3100 3101 public boolean hasValueBooleanType() { 3102 return this != null && this.value instanceof BooleanType; 3103 } 3104 3105 /** 3106 * @return {@link #value} (The value of this property.) 3107 */ 3108 public DateTimeType getValueDateTimeType() throws FHIRException { 3109 if (this.value == null) 3110 this.value = new DateTimeType(); 3111 if (!(this.value instanceof DateTimeType)) 3112 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3113 + this.value.getClass().getName() + " was encountered"); 3114 return (DateTimeType) this.value; 3115 } 3116 3117 public boolean hasValueDateTimeType() { 3118 return this != null && this.value instanceof DateTimeType; 3119 } 3120 3121 /** 3122 * @return {@link #value} (The value of this property.) 3123 */ 3124 public DecimalType getValueDecimalType() throws FHIRException { 3125 if (this.value == null) 3126 this.value = new DecimalType(); 3127 if (!(this.value instanceof DecimalType)) 3128 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 3129 + this.value.getClass().getName() + " was encountered"); 3130 return (DecimalType) this.value; 3131 } 3132 3133 public boolean hasValueDecimalType() { 3134 return this != null && this.value instanceof DecimalType; 3135 } 3136 3137 public boolean hasValue() { 3138 return this.value != null && !this.value.isEmpty(); 3139 } 3140 3141 /** 3142 * @param value {@link #value} (The value of this property.) 3143 */ 3144 public ConceptPropertyComponent setValue(Type value) { 3145 if (value != null && !(value instanceof CodeType || value instanceof Coding || value instanceof StringType 3146 || value instanceof IntegerType || value instanceof BooleanType || value instanceof DateTimeType 3147 || value instanceof DecimalType)) 3148 throw new Error("Not the right type for CodeSystem.concept.property.value[x]: " + value.fhirType()); 3149 this.value = value; 3150 return this; 3151 } 3152 3153 protected void listChildren(List<Property> children) { 3154 super.listChildren(children); 3155 children.add(new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, code)); 3156 children.add(new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3157 "The value of this property.", 0, 1, value)); 3158 } 3159 3160 @Override 3161 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3162 switch (_hash) { 3163 case 3059181: 3164 /* code */ return new Property("code", "code", "A code that is a reference to CodeSystem.property.code.", 0, 1, 3165 code); 3166 case -1410166417: 3167 /* value[x] */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3168 "The value of this property.", 0, 1, value); 3169 case 111972721: 3170 /* value */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3171 "The value of this property.", 0, 1, value); 3172 case -766209282: 3173 /* valueCode */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3174 "The value of this property.", 0, 1, value); 3175 case -1887705029: 3176 /* valueCoding */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3177 "The value of this property.", 0, 1, value); 3178 case -1424603934: 3179 /* valueString */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3180 "The value of this property.", 0, 1, value); 3181 case -1668204915: 3182 /* valueInteger */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3183 "The value of this property.", 0, 1, value); 3184 case 733421943: 3185 /* valueBoolean */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3186 "The value of this property.", 0, 1, value); 3187 case 1047929900: 3188 /* valueDateTime */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3189 "The value of this property.", 0, 1, value); 3190 case -2083993440: 3191 /* valueDecimal */ return new Property("value[x]", "code|Coding|string|integer|boolean|dateTime|decimal", 3192 "The value of this property.", 0, 1, value); 3193 default: 3194 return super.getNamedProperty(_hash, _name, _checkValid); 3195 } 3196 3197 } 3198 3199 @Override 3200 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3201 switch (hash) { 3202 case 3059181: 3203 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 3204 case 111972721: 3205 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3206 default: 3207 return super.getProperty(hash, name, checkValid); 3208 } 3209 3210 } 3211 3212 @Override 3213 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3214 switch (hash) { 3215 case 3059181: // code 3216 this.code = castToCode(value); // CodeType 3217 return value; 3218 case 111972721: // value 3219 this.value = castToType(value); // Type 3220 return value; 3221 default: 3222 return super.setProperty(hash, name, value); 3223 } 3224 3225 } 3226 3227 @Override 3228 public Base setProperty(String name, Base value) throws FHIRException { 3229 if (name.equals("code")) { 3230 this.code = castToCode(value); // CodeType 3231 } else if (name.equals("value[x]")) { 3232 this.value = castToType(value); // Type 3233 } else 3234 return super.setProperty(name, value); 3235 return value; 3236 } 3237 3238 @Override 3239 public void removeChild(String name, Base value) throws FHIRException { 3240 if (name.equals("code")) { 3241 this.code = null; 3242 } else if (name.equals("value[x]")) { 3243 this.value = null; 3244 } else 3245 super.removeChild(name, value); 3246 3247 } 3248 3249 @Override 3250 public Base makeProperty(int hash, String name) throws FHIRException { 3251 switch (hash) { 3252 case 3059181: 3253 return getCodeElement(); 3254 case -1410166417: 3255 return getValue(); 3256 case 111972721: 3257 return getValue(); 3258 default: 3259 return super.makeProperty(hash, name); 3260 } 3261 3262 } 3263 3264 @Override 3265 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3266 switch (hash) { 3267 case 3059181: 3268 /* code */ return new String[] { "code" }; 3269 case 111972721: 3270 /* value */ return new String[] { "code", "Coding", "string", "integer", "boolean", "dateTime", "decimal" }; 3271 default: 3272 return super.getTypesForProperty(hash, name); 3273 } 3274 3275 } 3276 3277 @Override 3278 public Base addChild(String name) throws FHIRException { 3279 if (name.equals("code")) { 3280 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.code"); 3281 } else if (name.equals("valueCode")) { 3282 this.value = new CodeType(); 3283 return this.value; 3284 } else if (name.equals("valueCoding")) { 3285 this.value = new Coding(); 3286 return this.value; 3287 } else if (name.equals("valueString")) { 3288 this.value = new StringType(); 3289 return this.value; 3290 } else if (name.equals("valueInteger")) { 3291 this.value = new IntegerType(); 3292 return this.value; 3293 } else if (name.equals("valueBoolean")) { 3294 this.value = new BooleanType(); 3295 return this.value; 3296 } else if (name.equals("valueDateTime")) { 3297 this.value = new DateTimeType(); 3298 return this.value; 3299 } else if (name.equals("valueDecimal")) { 3300 this.value = new DecimalType(); 3301 return this.value; 3302 } else 3303 return super.addChild(name); 3304 } 3305 3306 public ConceptPropertyComponent copy() { 3307 ConceptPropertyComponent dst = new ConceptPropertyComponent(); 3308 copyValues(dst); 3309 return dst; 3310 } 3311 3312 public void copyValues(ConceptPropertyComponent dst) { 3313 super.copyValues(dst); 3314 dst.code = code == null ? null : code.copy(); 3315 dst.value = value == null ? null : value.copy(); 3316 } 3317 3318 @Override 3319 public boolean equalsDeep(Base other_) { 3320 if (!super.equalsDeep(other_)) 3321 return false; 3322 if (!(other_ instanceof ConceptPropertyComponent)) 3323 return false; 3324 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 3325 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 3326 } 3327 3328 @Override 3329 public boolean equalsShallow(Base other_) { 3330 if (!super.equalsShallow(other_)) 3331 return false; 3332 if (!(other_ instanceof ConceptPropertyComponent)) 3333 return false; 3334 ConceptPropertyComponent o = (ConceptPropertyComponent) other_; 3335 return compareValues(code, o.code, true); 3336 } 3337 3338 public boolean isEmpty() { 3339 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 3340 } 3341 3342 public String fhirType() { 3343 return "CodeSystem.concept.property"; 3344 3345 } 3346 3347 } 3348 3349 /** 3350 * A formal identifier that is used to identify this code system when it is 3351 * represented in other formats, or referenced in a specification, model, design 3352 * or an instance. 3353 */ 3354 @Child(name = "identifier", type = { 3355 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3356 @Description(shortDefinition = "Additional identifier for the code system (business identifier)", formalDefinition = "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.") 3357 protected List<Identifier> identifier; 3358 3359 /** 3360 * Explanation of why this code system is needed and why it has been designed as 3361 * it has. 3362 */ 3363 @Child(name = "purpose", type = { 3364 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3365 @Description(shortDefinition = "Why this code system is defined", formalDefinition = "Explanation of why this code system is needed and why it has been designed as it has.") 3366 protected MarkdownType purpose; 3367 3368 /** 3369 * A copyright statement relating to the code system and/or its contents. 3370 * Copyright statements are generally legal restrictions on the use and 3371 * publishing of the code system. 3372 */ 3373 @Child(name = "copyright", type = { 3374 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3375 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.") 3376 protected MarkdownType copyright; 3377 3378 /** 3379 * If code comparison is case sensitive when codes within this system are 3380 * compared to each other. 3381 */ 3382 @Child(name = "caseSensitive", type = { 3383 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3384 @Description(shortDefinition = "If code comparison is case sensitive", formalDefinition = "If code comparison is case sensitive when codes within this system are compared to each other.") 3385 protected BooleanType caseSensitive; 3386 3387 /** 3388 * Canonical reference to the value set that contains the entire code system. 3389 */ 3390 @Child(name = "valueSet", type = { 3391 CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3392 @Description(shortDefinition = "Canonical reference to the value set with entire code system", formalDefinition = "Canonical reference to the value set that contains the entire code system.") 3393 protected CanonicalType valueSet; 3394 3395 /** 3396 * The meaning of the hierarchy of concepts as represented in this resource. 3397 */ 3398 @Child(name = "hierarchyMeaning", type = { 3399 CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3400 @Description(shortDefinition = "grouped-by | is-a | part-of | classified-with", formalDefinition = "The meaning of the hierarchy of concepts as represented in this resource.") 3401 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/codesystem-hierarchy-meaning") 3402 protected Enumeration<CodeSystemHierarchyMeaning> hierarchyMeaning; 3403 3404 /** 3405 * The code system defines a compositional (post-coordination) grammar. 3406 */ 3407 @Child(name = "compositional", type = { 3408 BooleanType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3409 @Description(shortDefinition = "If code system defines a compositional grammar", formalDefinition = "The code system defines a compositional (post-coordination) grammar.") 3410 protected BooleanType compositional; 3411 3412 /** 3413 * This flag is used to signify that the code system does not commit to concept 3414 * permanence across versions. If true, a version must be specified when 3415 * referencing this code system. 3416 */ 3417 @Child(name = "versionNeeded", type = { 3418 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3419 @Description(shortDefinition = "If definitions are not stable", formalDefinition = "This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.") 3420 protected BooleanType versionNeeded; 3421 3422 /** 3423 * The extent of the content of the code system (the concepts and codes it 3424 * defines) are represented in this resource instance. 3425 */ 3426 @Child(name = "content", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 3427 @Description(shortDefinition = "not-present | example | fragment | complete | supplement", formalDefinition = "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.") 3428 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/codesystem-content-mode") 3429 protected Enumeration<CodeSystemContentMode> content; 3430 3431 /** 3432 * The canonical URL of the code system that this code system supplement is 3433 * adding designations and properties to. 3434 */ 3435 @Child(name = "supplements", type = { 3436 CanonicalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3437 @Description(shortDefinition = "Canonical URL of Code System this adds designations and properties to", formalDefinition = "The canonical URL of the code system that this code system supplement is adding designations and properties to.") 3438 protected CanonicalType supplements; 3439 3440 /** 3441 * The total number of concepts defined by the code system. Where the code 3442 * system has a compositional grammar, the basis of this count is defined by the 3443 * system steward. 3444 */ 3445 @Child(name = "count", type = { 3446 UnsignedIntType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 3447 @Description(shortDefinition = "Total concepts in the code system", formalDefinition = "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.") 3448 protected UnsignedIntType count; 3449 3450 /** 3451 * A filter that can be used in a value set compose statement when selecting 3452 * concepts using a filter. 3453 */ 3454 @Child(name = "filter", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3455 @Description(shortDefinition = "Filter that can be used in a value set", formalDefinition = "A filter that can be used in a value set compose statement when selecting concepts using a filter.") 3456 protected List<CodeSystemFilterComponent> filter; 3457 3458 /** 3459 * A property defines an additional slot through which additional information 3460 * can be provided about a concept. 3461 */ 3462 @Child(name = "property", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3463 @Description(shortDefinition = "Additional information supplied about each concept", formalDefinition = "A property defines an additional slot through which additional information can be provided about a concept.") 3464 protected List<PropertyComponent> property; 3465 3466 /** 3467 * Concepts that are in the code system. The concept definitions are inherently 3468 * hierarchical, but the definitions must be consulted to determine what the 3469 * meanings of the hierarchical relationships are. 3470 */ 3471 @Child(name = "concept", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3472 @Description(shortDefinition = "Concepts in the code system", formalDefinition = "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.") 3473 protected List<ConceptDefinitionComponent> concept; 3474 3475 private static final long serialVersionUID = -1735124584L; 3476 3477 /** 3478 * Constructor 3479 */ 3480 public CodeSystem() { 3481 super(); 3482 } 3483 3484 /** 3485 * Constructor 3486 */ 3487 public CodeSystem(Enumeration<PublicationStatus> status, Enumeration<CodeSystemContentMode> content) { 3488 super(); 3489 this.status = status; 3490 this.content = content; 3491 } 3492 3493 /** 3494 * @return {@link #url} (An absolute URI that is used to identify this code 3495 * system when it is referenced in a specification, model, design or an 3496 * instance; also called its canonical identifier. This SHOULD be 3497 * globally unique and SHOULD be a literal address at which at which an 3498 * authoritative instance of this code system is (or will be) published. 3499 * This URL can be the target of a canonical reference. It SHALL remain 3500 * the same when the code system is stored on different servers. This is 3501 * used in [Coding](datatypes.html#Coding).system.). This is the 3502 * underlying object with id, value and extensions. The accessor 3503 * "getUrl" gives direct access to the value 3504 */ 3505 public UriType getUrlElement() { 3506 if (this.url == null) 3507 if (Configuration.errorOnAutoCreate()) 3508 throw new Error("Attempt to auto-create CodeSystem.url"); 3509 else if (Configuration.doAutoCreate()) 3510 this.url = new UriType(); // bb 3511 return this.url; 3512 } 3513 3514 public boolean hasUrlElement() { 3515 return this.url != null && !this.url.isEmpty(); 3516 } 3517 3518 public boolean hasUrl() { 3519 return this.url != null && !this.url.isEmpty(); 3520 } 3521 3522 /** 3523 * @param value {@link #url} (An absolute URI that is used to identify this code 3524 * system when it is referenced in a specification, model, design 3525 * or an instance; also called its canonical identifier. This 3526 * SHOULD be globally unique and SHOULD be a literal address at 3527 * which at which an authoritative instance of this code system is 3528 * (or will be) published. This URL can be the target of a 3529 * canonical reference. It SHALL remain the same when the code 3530 * system is stored on different servers. This is used in 3531 * [Coding](datatypes.html#Coding).system.). This is the underlying 3532 * object with id, value and extensions. The accessor "getUrl" 3533 * gives direct access to the value 3534 */ 3535 public CodeSystem setUrlElement(UriType value) { 3536 this.url = value; 3537 return this; 3538 } 3539 3540 /** 3541 * @return An absolute URI that is used to identify this code system when it is 3542 * referenced in a specification, model, design or an instance; also 3543 * called its canonical identifier. This SHOULD be globally unique and 3544 * SHOULD be a literal address at which at which an authoritative 3545 * instance of this code system is (or will be) published. This URL can 3546 * be the target of a canonical reference. It SHALL remain the same when 3547 * the code system is stored on different servers. This is used in 3548 * [Coding](datatypes.html#Coding).system. 3549 */ 3550 public String getUrl() { 3551 return this.url == null ? null : this.url.getValue(); 3552 } 3553 3554 /** 3555 * @param value An absolute URI that is used to identify this code system when 3556 * it is referenced in a specification, model, design or an 3557 * instance; also called its canonical identifier. This SHOULD be 3558 * globally unique and SHOULD be a literal address at which at 3559 * which an authoritative instance of this code system is (or will 3560 * be) published. This URL can be the target of a canonical 3561 * reference. It SHALL remain the same when the code system is 3562 * stored on different servers. This is used in 3563 * [Coding](datatypes.html#Coding).system. 3564 */ 3565 public CodeSystem setUrl(String value) { 3566 if (Utilities.noString(value)) 3567 this.url = null; 3568 else { 3569 if (this.url == null) 3570 this.url = new UriType(); 3571 this.url.setValue(value); 3572 } 3573 return this; 3574 } 3575 3576 /** 3577 * @return {@link #identifier} (A formal identifier that is used to identify 3578 * this code system when it is represented in other formats, or 3579 * referenced in a specification, model, design or an instance.) 3580 */ 3581 public List<Identifier> getIdentifier() { 3582 if (this.identifier == null) 3583 this.identifier = new ArrayList<Identifier>(); 3584 return this.identifier; 3585 } 3586 3587 /** 3588 * @return Returns a reference to <code>this</code> for easy method chaining 3589 */ 3590 public CodeSystem setIdentifier(List<Identifier> theIdentifier) { 3591 this.identifier = theIdentifier; 3592 return this; 3593 } 3594 3595 public boolean hasIdentifier() { 3596 if (this.identifier == null) 3597 return false; 3598 for (Identifier item : this.identifier) 3599 if (!item.isEmpty()) 3600 return true; 3601 return false; 3602 } 3603 3604 public Identifier addIdentifier() { // 3 3605 Identifier t = new Identifier(); 3606 if (this.identifier == null) 3607 this.identifier = new ArrayList<Identifier>(); 3608 this.identifier.add(t); 3609 return t; 3610 } 3611 3612 public CodeSystem addIdentifier(Identifier t) { // 3 3613 if (t == null) 3614 return this; 3615 if (this.identifier == null) 3616 this.identifier = new ArrayList<Identifier>(); 3617 this.identifier.add(t); 3618 return this; 3619 } 3620 3621 /** 3622 * @return The first repetition of repeating field {@link #identifier}, creating 3623 * it if it does not already exist 3624 */ 3625 public Identifier getIdentifierFirstRep() { 3626 if (getIdentifier().isEmpty()) { 3627 addIdentifier(); 3628 } 3629 return getIdentifier().get(0); 3630 } 3631 3632 /** 3633 * @return {@link #version} (The identifier that is used to identify this 3634 * version of the code system when it is referenced in a specification, 3635 * model, design or instance. This is an arbitrary value managed by the 3636 * code system author and is not expected to be globally unique. For 3637 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 3638 * is not available. There is also no expectation that versions can be 3639 * placed in a lexicographical sequence. This is used in 3640 * [Coding](datatypes.html#Coding).version.). This is the underlying 3641 * object with id, value and extensions. The accessor "getVersion" gives 3642 * direct access to the value 3643 */ 3644 public StringType getVersionElement() { 3645 if (this.version == null) 3646 if (Configuration.errorOnAutoCreate()) 3647 throw new Error("Attempt to auto-create CodeSystem.version"); 3648 else if (Configuration.doAutoCreate()) 3649 this.version = new StringType(); // bb 3650 return this.version; 3651 } 3652 3653 public boolean hasVersionElement() { 3654 return this.version != null && !this.version.isEmpty(); 3655 } 3656 3657 public boolean hasVersion() { 3658 return this.version != null && !this.version.isEmpty(); 3659 } 3660 3661 /** 3662 * @param value {@link #version} (The identifier that is used to identify this 3663 * version of the code system when it is referenced in a 3664 * specification, model, design or instance. This is an arbitrary 3665 * value managed by the code system author and is not expected to 3666 * be globally unique. For example, it might be a timestamp (e.g. 3667 * yyyymmdd) if a managed version is not available. There is also 3668 * no expectation that versions can be placed in a lexicographical 3669 * sequence. This is used in 3670 * [Coding](datatypes.html#Coding).version.). This is the 3671 * underlying object with id, value and extensions. The accessor 3672 * "getVersion" gives direct access to the value 3673 */ 3674 public CodeSystem setVersionElement(StringType value) { 3675 this.version = value; 3676 return this; 3677 } 3678 3679 /** 3680 * @return The identifier that is used to identify this version of the code 3681 * system when it is referenced in a specification, model, design or 3682 * instance. This is an arbitrary value managed by the code system 3683 * author and is not expected to be globally unique. For example, it 3684 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 3685 * available. There is also no expectation that versions can be placed 3686 * in a lexicographical sequence. This is used in 3687 * [Coding](datatypes.html#Coding).version. 3688 */ 3689 public String getVersion() { 3690 return this.version == null ? null : this.version.getValue(); 3691 } 3692 3693 /** 3694 * @param value The identifier that is used to identify this version of the code 3695 * system when it is referenced in a specification, model, design 3696 * or instance. This is an arbitrary value managed by the code 3697 * system author and is not expected to be globally unique. For 3698 * example, it might be a timestamp (e.g. yyyymmdd) if a managed 3699 * version is not available. There is also no expectation that 3700 * versions can be placed in a lexicographical sequence. This is 3701 * used in [Coding](datatypes.html#Coding).version. 3702 */ 3703 public CodeSystem setVersion(String value) { 3704 if (Utilities.noString(value)) 3705 this.version = null; 3706 else { 3707 if (this.version == null) 3708 this.version = new StringType(); 3709 this.version.setValue(value); 3710 } 3711 return this; 3712 } 3713 3714 /** 3715 * @return {@link #name} (A natural language name identifying the code system. 3716 * This name should be usable as an identifier for the module by machine 3717 * processing applications such as code generation.). This is the 3718 * underlying object with id, value and extensions. The accessor 3719 * "getName" gives direct access to the value 3720 */ 3721 public StringType getNameElement() { 3722 if (this.name == null) 3723 if (Configuration.errorOnAutoCreate()) 3724 throw new Error("Attempt to auto-create CodeSystem.name"); 3725 else if (Configuration.doAutoCreate()) 3726 this.name = new StringType(); // bb 3727 return this.name; 3728 } 3729 3730 public boolean hasNameElement() { 3731 return this.name != null && !this.name.isEmpty(); 3732 } 3733 3734 public boolean hasName() { 3735 return this.name != null && !this.name.isEmpty(); 3736 } 3737 3738 /** 3739 * @param value {@link #name} (A natural language name identifying the code 3740 * system. This name should be usable as an identifier for the 3741 * module by machine processing applications such as code 3742 * generation.). This is the underlying object with id, value and 3743 * extensions. The accessor "getName" gives direct access to the 3744 * value 3745 */ 3746 public CodeSystem setNameElement(StringType value) { 3747 this.name = value; 3748 return this; 3749 } 3750 3751 /** 3752 * @return A natural language name identifying the code system. This name should 3753 * be usable as an identifier for the module by machine processing 3754 * applications such as code generation. 3755 */ 3756 public String getName() { 3757 return this.name == null ? null : this.name.getValue(); 3758 } 3759 3760 /** 3761 * @param value A natural language name identifying the code system. This name 3762 * should be usable as an identifier for the module by machine 3763 * processing applications such as code generation. 3764 */ 3765 public CodeSystem setName(String value) { 3766 if (Utilities.noString(value)) 3767 this.name = null; 3768 else { 3769 if (this.name == null) 3770 this.name = new StringType(); 3771 this.name.setValue(value); 3772 } 3773 return this; 3774 } 3775 3776 /** 3777 * @return {@link #title} (A short, descriptive, user-friendly title for the 3778 * code system.). This is the underlying object with id, value and 3779 * extensions. The accessor "getTitle" gives direct access to the value 3780 */ 3781 public StringType getTitleElement() { 3782 if (this.title == null) 3783 if (Configuration.errorOnAutoCreate()) 3784 throw new Error("Attempt to auto-create CodeSystem.title"); 3785 else if (Configuration.doAutoCreate()) 3786 this.title = new StringType(); // bb 3787 return this.title; 3788 } 3789 3790 public boolean hasTitleElement() { 3791 return this.title != null && !this.title.isEmpty(); 3792 } 3793 3794 public boolean hasTitle() { 3795 return this.title != null && !this.title.isEmpty(); 3796 } 3797 3798 /** 3799 * @param value {@link #title} (A short, descriptive, user-friendly title for 3800 * the code system.). This is the underlying object with id, value 3801 * and extensions. The accessor "getTitle" gives direct access to 3802 * the value 3803 */ 3804 public CodeSystem setTitleElement(StringType value) { 3805 this.title = value; 3806 return this; 3807 } 3808 3809 /** 3810 * @return A short, descriptive, user-friendly title for the code system. 3811 */ 3812 public String getTitle() { 3813 return this.title == null ? null : this.title.getValue(); 3814 } 3815 3816 /** 3817 * @param value A short, descriptive, user-friendly title for the code system. 3818 */ 3819 public CodeSystem setTitle(String value) { 3820 if (Utilities.noString(value)) 3821 this.title = null; 3822 else { 3823 if (this.title == null) 3824 this.title = new StringType(); 3825 this.title.setValue(value); 3826 } 3827 return this; 3828 } 3829 3830 /** 3831 * @return {@link #status} (The date (and optionally time) when the code system 3832 * resource was created or revised.). This is the underlying object with 3833 * id, value and extensions. The accessor "getStatus" gives direct 3834 * access to the value 3835 */ 3836 public Enumeration<PublicationStatus> getStatusElement() { 3837 if (this.status == null) 3838 if (Configuration.errorOnAutoCreate()) 3839 throw new Error("Attempt to auto-create CodeSystem.status"); 3840 else if (Configuration.doAutoCreate()) 3841 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3842 return this.status; 3843 } 3844 3845 public boolean hasStatusElement() { 3846 return this.status != null && !this.status.isEmpty(); 3847 } 3848 3849 public boolean hasStatus() { 3850 return this.status != null && !this.status.isEmpty(); 3851 } 3852 3853 /** 3854 * @param value {@link #status} (The date (and optionally time) when the code 3855 * system resource was created or revised.). This is the underlying 3856 * object with id, value and extensions. The accessor "getStatus" 3857 * gives direct access to the value 3858 */ 3859 public CodeSystem setStatusElement(Enumeration<PublicationStatus> value) { 3860 this.status = value; 3861 return this; 3862 } 3863 3864 /** 3865 * @return The date (and optionally time) when the code system resource was 3866 * created or revised. 3867 */ 3868 public PublicationStatus getStatus() { 3869 return this.status == null ? null : this.status.getValue(); 3870 } 3871 3872 /** 3873 * @param value The date (and optionally time) when the code system resource was 3874 * created or revised. 3875 */ 3876 public CodeSystem setStatus(PublicationStatus value) { 3877 if (this.status == null) 3878 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3879 this.status.setValue(value); 3880 return this; 3881 } 3882 3883 /** 3884 * @return {@link #experimental} (A Boolean value to indicate that this code 3885 * system is authored for testing purposes (or 3886 * education/evaluation/marketing) and is not intended to be used for 3887 * genuine usage.). This is the underlying object with id, value and 3888 * extensions. The accessor "getExperimental" gives direct access to the 3889 * value 3890 */ 3891 public BooleanType getExperimentalElement() { 3892 if (this.experimental == null) 3893 if (Configuration.errorOnAutoCreate()) 3894 throw new Error("Attempt to auto-create CodeSystem.experimental"); 3895 else if (Configuration.doAutoCreate()) 3896 this.experimental = new BooleanType(); // bb 3897 return this.experimental; 3898 } 3899 3900 public boolean hasExperimentalElement() { 3901 return this.experimental != null && !this.experimental.isEmpty(); 3902 } 3903 3904 public boolean hasExperimental() { 3905 return this.experimental != null && !this.experimental.isEmpty(); 3906 } 3907 3908 /** 3909 * @param value {@link #experimental} (A Boolean value to indicate that this 3910 * code system is authored for testing purposes (or 3911 * education/evaluation/marketing) and is not intended to be used 3912 * for genuine usage.). This is the underlying object with id, 3913 * value and extensions. The accessor "getExperimental" gives 3914 * direct access to the value 3915 */ 3916 public CodeSystem setExperimentalElement(BooleanType value) { 3917 this.experimental = value; 3918 return this; 3919 } 3920 3921 /** 3922 * @return A Boolean value to indicate that this code system is authored for 3923 * testing purposes (or education/evaluation/marketing) and is not 3924 * intended to be used for genuine usage. 3925 */ 3926 public boolean getExperimental() { 3927 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3928 } 3929 3930 /** 3931 * @param value A Boolean value to indicate that this code system is authored 3932 * for testing purposes (or education/evaluation/marketing) and is 3933 * not intended to be used for genuine usage. 3934 */ 3935 public CodeSystem setExperimental(boolean value) { 3936 if (this.experimental == null) 3937 this.experimental = new BooleanType(); 3938 this.experimental.setValue(value); 3939 return this; 3940 } 3941 3942 /** 3943 * @return {@link #date} (The date (and optionally time) when the code system 3944 * was published. The date must change when the business version changes 3945 * and it must change if the status code changes. In addition, it should 3946 * change when the substantive content of the code system changes.). 3947 * This is the underlying object with id, value and extensions. The 3948 * accessor "getDate" gives direct access to the value 3949 */ 3950 public DateTimeType getDateElement() { 3951 if (this.date == null) 3952 if (Configuration.errorOnAutoCreate()) 3953 throw new Error("Attempt to auto-create CodeSystem.date"); 3954 else if (Configuration.doAutoCreate()) 3955 this.date = new DateTimeType(); // bb 3956 return this.date; 3957 } 3958 3959 public boolean hasDateElement() { 3960 return this.date != null && !this.date.isEmpty(); 3961 } 3962 3963 public boolean hasDate() { 3964 return this.date != null && !this.date.isEmpty(); 3965 } 3966 3967 /** 3968 * @param value {@link #date} (The date (and optionally time) when the code 3969 * system was published. The date must change when the business 3970 * version changes and it must change if the status code changes. 3971 * In addition, it should change when the substantive content of 3972 * the code system changes.). This is the underlying object with 3973 * id, value and extensions. The accessor "getDate" gives direct 3974 * access to the value 3975 */ 3976 public CodeSystem setDateElement(DateTimeType value) { 3977 this.date = value; 3978 return this; 3979 } 3980 3981 /** 3982 * @return The date (and optionally time) when the code system was published. 3983 * The date must change when the business version changes and it must 3984 * change if the status code changes. In addition, it should change when 3985 * the substantive content of the code system changes. 3986 */ 3987 public Date getDate() { 3988 return this.date == null ? null : this.date.getValue(); 3989 } 3990 3991 /** 3992 * @param value The date (and optionally time) when the code system was 3993 * published. The date must change when the business version 3994 * changes and it must change if the status code changes. In 3995 * addition, it should change when the substantive content of the 3996 * code system changes. 3997 */ 3998 public CodeSystem setDate(Date value) { 3999 if (value == null) 4000 this.date = null; 4001 else { 4002 if (this.date == null) 4003 this.date = new DateTimeType(); 4004 this.date.setValue(value); 4005 } 4006 return this; 4007 } 4008 4009 /** 4010 * @return {@link #publisher} (The name of the organization or individual that 4011 * published the code system.). This is the underlying object with id, 4012 * value and extensions. The accessor "getPublisher" gives direct access 4013 * to the value 4014 */ 4015 public StringType getPublisherElement() { 4016 if (this.publisher == null) 4017 if (Configuration.errorOnAutoCreate()) 4018 throw new Error("Attempt to auto-create CodeSystem.publisher"); 4019 else if (Configuration.doAutoCreate()) 4020 this.publisher = new StringType(); // bb 4021 return this.publisher; 4022 } 4023 4024 public boolean hasPublisherElement() { 4025 return this.publisher != null && !this.publisher.isEmpty(); 4026 } 4027 4028 public boolean hasPublisher() { 4029 return this.publisher != null && !this.publisher.isEmpty(); 4030 } 4031 4032 /** 4033 * @param value {@link #publisher} (The name of the organization or individual 4034 * that published the code system.). This is the underlying object 4035 * with id, value and extensions. The accessor "getPublisher" gives 4036 * direct access to the value 4037 */ 4038 public CodeSystem setPublisherElement(StringType value) { 4039 this.publisher = value; 4040 return this; 4041 } 4042 4043 /** 4044 * @return The name of the organization or individual that published the code 4045 * system. 4046 */ 4047 public String getPublisher() { 4048 return this.publisher == null ? null : this.publisher.getValue(); 4049 } 4050 4051 /** 4052 * @param value The name of the organization or individual that published the 4053 * code system. 4054 */ 4055 public CodeSystem setPublisher(String value) { 4056 if (Utilities.noString(value)) 4057 this.publisher = null; 4058 else { 4059 if (this.publisher == null) 4060 this.publisher = new StringType(); 4061 this.publisher.setValue(value); 4062 } 4063 return this; 4064 } 4065 4066 /** 4067 * @return {@link #contact} (Contact details to assist a user in finding and 4068 * communicating with the publisher.) 4069 */ 4070 public List<ContactDetail> getContact() { 4071 if (this.contact == null) 4072 this.contact = new ArrayList<ContactDetail>(); 4073 return this.contact; 4074 } 4075 4076 /** 4077 * @return Returns a reference to <code>this</code> for easy method chaining 4078 */ 4079 public CodeSystem setContact(List<ContactDetail> theContact) { 4080 this.contact = theContact; 4081 return this; 4082 } 4083 4084 public boolean hasContact() { 4085 if (this.contact == null) 4086 return false; 4087 for (ContactDetail item : this.contact) 4088 if (!item.isEmpty()) 4089 return true; 4090 return false; 4091 } 4092 4093 public ContactDetail addContact() { // 3 4094 ContactDetail t = new ContactDetail(); 4095 if (this.contact == null) 4096 this.contact = new ArrayList<ContactDetail>(); 4097 this.contact.add(t); 4098 return t; 4099 } 4100 4101 public CodeSystem addContact(ContactDetail t) { // 3 4102 if (t == null) 4103 return this; 4104 if (this.contact == null) 4105 this.contact = new ArrayList<ContactDetail>(); 4106 this.contact.add(t); 4107 return this; 4108 } 4109 4110 /** 4111 * @return The first repetition of repeating field {@link #contact}, creating it 4112 * if it does not already exist 4113 */ 4114 public ContactDetail getContactFirstRep() { 4115 if (getContact().isEmpty()) { 4116 addContact(); 4117 } 4118 return getContact().get(0); 4119 } 4120 4121 /** 4122 * @return {@link #description} (A free text natural language description of the 4123 * code system from a consumer's perspective.). This is the underlying 4124 * object with id, value and extensions. The accessor "getDescription" 4125 * gives direct access to the value 4126 */ 4127 public MarkdownType getDescriptionElement() { 4128 if (this.description == null) 4129 if (Configuration.errorOnAutoCreate()) 4130 throw new Error("Attempt to auto-create CodeSystem.description"); 4131 else if (Configuration.doAutoCreate()) 4132 this.description = new MarkdownType(); // bb 4133 return this.description; 4134 } 4135 4136 public boolean hasDescriptionElement() { 4137 return this.description != null && !this.description.isEmpty(); 4138 } 4139 4140 public boolean hasDescription() { 4141 return this.description != null && !this.description.isEmpty(); 4142 } 4143 4144 /** 4145 * @param value {@link #description} (A free text natural language description 4146 * of the code system from a consumer's perspective.). This is the 4147 * underlying object with id, value and extensions. The accessor 4148 * "getDescription" gives direct access to the value 4149 */ 4150 public CodeSystem setDescriptionElement(MarkdownType value) { 4151 this.description = value; 4152 return this; 4153 } 4154 4155 /** 4156 * @return A free text natural language description of the code system from a 4157 * consumer's perspective. 4158 */ 4159 public String getDescription() { 4160 return this.description == null ? null : this.description.getValue(); 4161 } 4162 4163 /** 4164 * @param value A free text natural language description of the code system from 4165 * a consumer's perspective. 4166 */ 4167 public CodeSystem setDescription(String value) { 4168 if (value == null) 4169 this.description = null; 4170 else { 4171 if (this.description == null) 4172 this.description = new MarkdownType(); 4173 this.description.setValue(value); 4174 } 4175 return this; 4176 } 4177 4178 /** 4179 * @return {@link #useContext} (The content was developed with a focus and 4180 * intent of supporting the contexts that are listed. These contexts may 4181 * be general categories (gender, age, ...) or may be references to 4182 * specific programs (insurance plans, studies, ...) and may be used to 4183 * assist with indexing and searching for appropriate code system 4184 * instances.) 4185 */ 4186 public List<UsageContext> getUseContext() { 4187 if (this.useContext == null) 4188 this.useContext = new ArrayList<UsageContext>(); 4189 return this.useContext; 4190 } 4191 4192 /** 4193 * @return Returns a reference to <code>this</code> for easy method chaining 4194 */ 4195 public CodeSystem setUseContext(List<UsageContext> theUseContext) { 4196 this.useContext = theUseContext; 4197 return this; 4198 } 4199 4200 public boolean hasUseContext() { 4201 if (this.useContext == null) 4202 return false; 4203 for (UsageContext item : this.useContext) 4204 if (!item.isEmpty()) 4205 return true; 4206 return false; 4207 } 4208 4209 public UsageContext addUseContext() { // 3 4210 UsageContext t = new UsageContext(); 4211 if (this.useContext == null) 4212 this.useContext = new ArrayList<UsageContext>(); 4213 this.useContext.add(t); 4214 return t; 4215 } 4216 4217 public CodeSystem addUseContext(UsageContext t) { // 3 4218 if (t == null) 4219 return this; 4220 if (this.useContext == null) 4221 this.useContext = new ArrayList<UsageContext>(); 4222 this.useContext.add(t); 4223 return this; 4224 } 4225 4226 /** 4227 * @return The first repetition of repeating field {@link #useContext}, creating 4228 * it if it does not already exist 4229 */ 4230 public UsageContext getUseContextFirstRep() { 4231 if (getUseContext().isEmpty()) { 4232 addUseContext(); 4233 } 4234 return getUseContext().get(0); 4235 } 4236 4237 /** 4238 * @return {@link #jurisdiction} (A legal or geographic region in which the code 4239 * system is intended to be used.) 4240 */ 4241 public List<CodeableConcept> getJurisdiction() { 4242 if (this.jurisdiction == null) 4243 this.jurisdiction = new ArrayList<CodeableConcept>(); 4244 return this.jurisdiction; 4245 } 4246 4247 /** 4248 * @return Returns a reference to <code>this</code> for easy method chaining 4249 */ 4250 public CodeSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 4251 this.jurisdiction = theJurisdiction; 4252 return this; 4253 } 4254 4255 public boolean hasJurisdiction() { 4256 if (this.jurisdiction == null) 4257 return false; 4258 for (CodeableConcept item : this.jurisdiction) 4259 if (!item.isEmpty()) 4260 return true; 4261 return false; 4262 } 4263 4264 public CodeableConcept addJurisdiction() { // 3 4265 CodeableConcept t = new CodeableConcept(); 4266 if (this.jurisdiction == null) 4267 this.jurisdiction = new ArrayList<CodeableConcept>(); 4268 this.jurisdiction.add(t); 4269 return t; 4270 } 4271 4272 public CodeSystem addJurisdiction(CodeableConcept t) { // 3 4273 if (t == null) 4274 return this; 4275 if (this.jurisdiction == null) 4276 this.jurisdiction = new ArrayList<CodeableConcept>(); 4277 this.jurisdiction.add(t); 4278 return this; 4279 } 4280 4281 /** 4282 * @return The first repetition of repeating field {@link #jurisdiction}, 4283 * creating it if it does not already exist 4284 */ 4285 public CodeableConcept getJurisdictionFirstRep() { 4286 if (getJurisdiction().isEmpty()) { 4287 addJurisdiction(); 4288 } 4289 return getJurisdiction().get(0); 4290 } 4291 4292 /** 4293 * @return {@link #purpose} (Explanation of why this code system is needed and 4294 * why it has been designed as it has.). This is the underlying object 4295 * with id, value and extensions. The accessor "getPurpose" gives direct 4296 * access to the value 4297 */ 4298 public MarkdownType getPurposeElement() { 4299 if (this.purpose == null) 4300 if (Configuration.errorOnAutoCreate()) 4301 throw new Error("Attempt to auto-create CodeSystem.purpose"); 4302 else if (Configuration.doAutoCreate()) 4303 this.purpose = new MarkdownType(); // bb 4304 return this.purpose; 4305 } 4306 4307 public boolean hasPurposeElement() { 4308 return this.purpose != null && !this.purpose.isEmpty(); 4309 } 4310 4311 public boolean hasPurpose() { 4312 return this.purpose != null && !this.purpose.isEmpty(); 4313 } 4314 4315 /** 4316 * @param value {@link #purpose} (Explanation of why this code system is needed 4317 * and why it has been designed as it has.). This is the underlying 4318 * object with id, value and extensions. The accessor "getPurpose" 4319 * gives direct access to the value 4320 */ 4321 public CodeSystem setPurposeElement(MarkdownType value) { 4322 this.purpose = value; 4323 return this; 4324 } 4325 4326 /** 4327 * @return Explanation of why this code system is needed and why it has been 4328 * designed as it has. 4329 */ 4330 public String getPurpose() { 4331 return this.purpose == null ? null : this.purpose.getValue(); 4332 } 4333 4334 /** 4335 * @param value Explanation of why this code system is needed and why it has 4336 * been designed as it has. 4337 */ 4338 public CodeSystem setPurpose(String value) { 4339 if (value == null) 4340 this.purpose = null; 4341 else { 4342 if (this.purpose == null) 4343 this.purpose = new MarkdownType(); 4344 this.purpose.setValue(value); 4345 } 4346 return this; 4347 } 4348 4349 /** 4350 * @return {@link #copyright} (A copyright statement relating to the code system 4351 * and/or its contents. Copyright statements are generally legal 4352 * restrictions on the use and publishing of the code system.). This is 4353 * the underlying object with id, value and extensions. The accessor 4354 * "getCopyright" gives direct access to the value 4355 */ 4356 public MarkdownType getCopyrightElement() { 4357 if (this.copyright == null) 4358 if (Configuration.errorOnAutoCreate()) 4359 throw new Error("Attempt to auto-create CodeSystem.copyright"); 4360 else if (Configuration.doAutoCreate()) 4361 this.copyright = new MarkdownType(); // bb 4362 return this.copyright; 4363 } 4364 4365 public boolean hasCopyrightElement() { 4366 return this.copyright != null && !this.copyright.isEmpty(); 4367 } 4368 4369 public boolean hasCopyright() { 4370 return this.copyright != null && !this.copyright.isEmpty(); 4371 } 4372 4373 /** 4374 * @param value {@link #copyright} (A copyright statement relating to the code 4375 * system and/or its contents. Copyright statements are generally 4376 * legal restrictions on the use and publishing of the code 4377 * system.). This is the underlying object with id, value and 4378 * extensions. The accessor "getCopyright" gives direct access to 4379 * the value 4380 */ 4381 public CodeSystem setCopyrightElement(MarkdownType value) { 4382 this.copyright = value; 4383 return this; 4384 } 4385 4386 /** 4387 * @return A copyright statement relating to the code system and/or its 4388 * contents. Copyright statements are generally legal restrictions on 4389 * the use and publishing of the code system. 4390 */ 4391 public String getCopyright() { 4392 return this.copyright == null ? null : this.copyright.getValue(); 4393 } 4394 4395 /** 4396 * @param value A copyright statement relating to the code system and/or its 4397 * contents. Copyright statements are generally legal restrictions 4398 * on the use and publishing of the code system. 4399 */ 4400 public CodeSystem setCopyright(String value) { 4401 if (value == null) 4402 this.copyright = null; 4403 else { 4404 if (this.copyright == null) 4405 this.copyright = new MarkdownType(); 4406 this.copyright.setValue(value); 4407 } 4408 return this; 4409 } 4410 4411 /** 4412 * @return {@link #caseSensitive} (If code comparison is case sensitive when 4413 * codes within this system are compared to each other.). This is the 4414 * underlying object with id, value and extensions. The accessor 4415 * "getCaseSensitive" gives direct access to the value 4416 */ 4417 public BooleanType getCaseSensitiveElement() { 4418 if (this.caseSensitive == null) 4419 if (Configuration.errorOnAutoCreate()) 4420 throw new Error("Attempt to auto-create CodeSystem.caseSensitive"); 4421 else if (Configuration.doAutoCreate()) 4422 this.caseSensitive = new BooleanType(); // bb 4423 return this.caseSensitive; 4424 } 4425 4426 public boolean hasCaseSensitiveElement() { 4427 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 4428 } 4429 4430 public boolean hasCaseSensitive() { 4431 return this.caseSensitive != null && !this.caseSensitive.isEmpty(); 4432 } 4433 4434 /** 4435 * @param value {@link #caseSensitive} (If code comparison is case sensitive 4436 * when codes within this system are compared to each other.). This 4437 * is the underlying object with id, value and extensions. The 4438 * accessor "getCaseSensitive" gives direct access to the value 4439 */ 4440 public CodeSystem setCaseSensitiveElement(BooleanType value) { 4441 this.caseSensitive = value; 4442 return this; 4443 } 4444 4445 /** 4446 * @return If code comparison is case sensitive when codes within this system 4447 * are compared to each other. 4448 */ 4449 public boolean getCaseSensitive() { 4450 return this.caseSensitive == null || this.caseSensitive.isEmpty() ? false : this.caseSensitive.getValue(); 4451 } 4452 4453 /** 4454 * @param value If code comparison is case sensitive when codes within this 4455 * system are compared to each other. 4456 */ 4457 public CodeSystem setCaseSensitive(boolean value) { 4458 if (this.caseSensitive == null) 4459 this.caseSensitive = new BooleanType(); 4460 this.caseSensitive.setValue(value); 4461 return this; 4462 } 4463 4464 /** 4465 * @return {@link #valueSet} (Canonical reference to the value set that contains 4466 * the entire code system.). This is the underlying object with id, 4467 * value and extensions. The accessor "getValueSet" gives direct access 4468 * to the value 4469 */ 4470 public CanonicalType getValueSetElement() { 4471 if (this.valueSet == null) 4472 if (Configuration.errorOnAutoCreate()) 4473 throw new Error("Attempt to auto-create CodeSystem.valueSet"); 4474 else if (Configuration.doAutoCreate()) 4475 this.valueSet = new CanonicalType(); // bb 4476 return this.valueSet; 4477 } 4478 4479 public boolean hasValueSetElement() { 4480 return this.valueSet != null && !this.valueSet.isEmpty(); 4481 } 4482 4483 public boolean hasValueSet() { 4484 return this.valueSet != null && !this.valueSet.isEmpty(); 4485 } 4486 4487 /** 4488 * @param value {@link #valueSet} (Canonical reference to the value set that 4489 * contains the entire code system.). This is the underlying object 4490 * with id, value and extensions. The accessor "getValueSet" gives 4491 * direct access to the value 4492 */ 4493 public CodeSystem setValueSetElement(CanonicalType value) { 4494 this.valueSet = value; 4495 return this; 4496 } 4497 4498 /** 4499 * @return Canonical reference to the value set that contains the entire code 4500 * system. 4501 */ 4502 public String getValueSet() { 4503 return this.valueSet == null ? null : this.valueSet.getValue(); 4504 } 4505 4506 /** 4507 * @param value Canonical reference to the value set that contains the entire 4508 * code system. 4509 */ 4510 public CodeSystem setValueSet(String value) { 4511 if (Utilities.noString(value)) 4512 this.valueSet = null; 4513 else { 4514 if (this.valueSet == null) 4515 this.valueSet = new CanonicalType(); 4516 this.valueSet.setValue(value); 4517 } 4518 return this; 4519 } 4520 4521 /** 4522 * @return {@link #hierarchyMeaning} (The meaning of the hierarchy of concepts 4523 * as represented in this resource.). This is the underlying object with 4524 * id, value and extensions. The accessor "getHierarchyMeaning" gives 4525 * direct access to the value 4526 */ 4527 public Enumeration<CodeSystemHierarchyMeaning> getHierarchyMeaningElement() { 4528 if (this.hierarchyMeaning == null) 4529 if (Configuration.errorOnAutoCreate()) 4530 throw new Error("Attempt to auto-create CodeSystem.hierarchyMeaning"); 4531 else if (Configuration.doAutoCreate()) 4532 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>( 4533 new CodeSystemHierarchyMeaningEnumFactory()); // bb 4534 return this.hierarchyMeaning; 4535 } 4536 4537 public boolean hasHierarchyMeaningElement() { 4538 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 4539 } 4540 4541 public boolean hasHierarchyMeaning() { 4542 return this.hierarchyMeaning != null && !this.hierarchyMeaning.isEmpty(); 4543 } 4544 4545 /** 4546 * @param value {@link #hierarchyMeaning} (The meaning of the hierarchy of 4547 * concepts as represented in this resource.). This is the 4548 * underlying object with id, value and extensions. The accessor 4549 * "getHierarchyMeaning" gives direct access to the value 4550 */ 4551 public CodeSystem setHierarchyMeaningElement(Enumeration<CodeSystemHierarchyMeaning> value) { 4552 this.hierarchyMeaning = value; 4553 return this; 4554 } 4555 4556 /** 4557 * @return The meaning of the hierarchy of concepts as represented in this 4558 * resource. 4559 */ 4560 public CodeSystemHierarchyMeaning getHierarchyMeaning() { 4561 return this.hierarchyMeaning == null ? null : this.hierarchyMeaning.getValue(); 4562 } 4563 4564 /** 4565 * @param value The meaning of the hierarchy of concepts as represented in this 4566 * resource. 4567 */ 4568 public CodeSystem setHierarchyMeaning(CodeSystemHierarchyMeaning value) { 4569 if (value == null) 4570 this.hierarchyMeaning = null; 4571 else { 4572 if (this.hierarchyMeaning == null) 4573 this.hierarchyMeaning = new Enumeration<CodeSystemHierarchyMeaning>( 4574 new CodeSystemHierarchyMeaningEnumFactory()); 4575 this.hierarchyMeaning.setValue(value); 4576 } 4577 return this; 4578 } 4579 4580 /** 4581 * @return {@link #compositional} (The code system defines a compositional 4582 * (post-coordination) grammar.). This is the underlying object with id, 4583 * value and extensions. The accessor "getCompositional" gives direct 4584 * access to the value 4585 */ 4586 public BooleanType getCompositionalElement() { 4587 if (this.compositional == null) 4588 if (Configuration.errorOnAutoCreate()) 4589 throw new Error("Attempt to auto-create CodeSystem.compositional"); 4590 else if (Configuration.doAutoCreate()) 4591 this.compositional = new BooleanType(); // bb 4592 return this.compositional; 4593 } 4594 4595 public boolean hasCompositionalElement() { 4596 return this.compositional != null && !this.compositional.isEmpty(); 4597 } 4598 4599 public boolean hasCompositional() { 4600 return this.compositional != null && !this.compositional.isEmpty(); 4601 } 4602 4603 /** 4604 * @param value {@link #compositional} (The code system defines a compositional 4605 * (post-coordination) grammar.). This is the underlying object 4606 * with id, value and extensions. The accessor "getCompositional" 4607 * gives direct access to the value 4608 */ 4609 public CodeSystem setCompositionalElement(BooleanType value) { 4610 this.compositional = value; 4611 return this; 4612 } 4613 4614 /** 4615 * @return The code system defines a compositional (post-coordination) grammar. 4616 */ 4617 public boolean getCompositional() { 4618 return this.compositional == null || this.compositional.isEmpty() ? false : this.compositional.getValue(); 4619 } 4620 4621 /** 4622 * @param value The code system defines a compositional (post-coordination) 4623 * grammar. 4624 */ 4625 public CodeSystem setCompositional(boolean value) { 4626 if (this.compositional == null) 4627 this.compositional = new BooleanType(); 4628 this.compositional.setValue(value); 4629 return this; 4630 } 4631 4632 /** 4633 * @return {@link #versionNeeded} (This flag is used to signify that the code 4634 * system does not commit to concept permanence across versions. If 4635 * true, a version must be specified when referencing this code 4636 * system.). This is the underlying object with id, value and 4637 * extensions. The accessor "getVersionNeeded" gives direct access to 4638 * the value 4639 */ 4640 public BooleanType getVersionNeededElement() { 4641 if (this.versionNeeded == null) 4642 if (Configuration.errorOnAutoCreate()) 4643 throw new Error("Attempt to auto-create CodeSystem.versionNeeded"); 4644 else if (Configuration.doAutoCreate()) 4645 this.versionNeeded = new BooleanType(); // bb 4646 return this.versionNeeded; 4647 } 4648 4649 public boolean hasVersionNeededElement() { 4650 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 4651 } 4652 4653 public boolean hasVersionNeeded() { 4654 return this.versionNeeded != null && !this.versionNeeded.isEmpty(); 4655 } 4656 4657 /** 4658 * @param value {@link #versionNeeded} (This flag is used to signify that the 4659 * code system does not commit to concept permanence across 4660 * versions. If true, a version must be specified when referencing 4661 * this code system.). This is the underlying object with id, value 4662 * and extensions. The accessor "getVersionNeeded" gives direct 4663 * access to the value 4664 */ 4665 public CodeSystem setVersionNeededElement(BooleanType value) { 4666 this.versionNeeded = value; 4667 return this; 4668 } 4669 4670 /** 4671 * @return This flag is used to signify that the code system does not commit to 4672 * concept permanence across versions. If true, a version must be 4673 * specified when referencing this code system. 4674 */ 4675 public boolean getVersionNeeded() { 4676 return this.versionNeeded == null || this.versionNeeded.isEmpty() ? false : this.versionNeeded.getValue(); 4677 } 4678 4679 /** 4680 * @param value This flag is used to signify that the code system does not 4681 * commit to concept permanence across versions. If true, a version 4682 * must be specified when referencing this code system. 4683 */ 4684 public CodeSystem setVersionNeeded(boolean value) { 4685 if (this.versionNeeded == null) 4686 this.versionNeeded = new BooleanType(); 4687 this.versionNeeded.setValue(value); 4688 return this; 4689 } 4690 4691 /** 4692 * @return {@link #content} (The extent of the content of the code system (the 4693 * concepts and codes it defines) are represented in this resource 4694 * instance.). This is the underlying object with id, value and 4695 * extensions. The accessor "getContent" gives direct access to the 4696 * value 4697 */ 4698 public Enumeration<CodeSystemContentMode> getContentElement() { 4699 if (this.content == null) 4700 if (Configuration.errorOnAutoCreate()) 4701 throw new Error("Attempt to auto-create CodeSystem.content"); 4702 else if (Configuration.doAutoCreate()) 4703 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); // bb 4704 return this.content; 4705 } 4706 4707 public boolean hasContentElement() { 4708 return this.content != null && !this.content.isEmpty(); 4709 } 4710 4711 public boolean hasContent() { 4712 return this.content != null && !this.content.isEmpty(); 4713 } 4714 4715 /** 4716 * @param value {@link #content} (The extent of the content of the code system 4717 * (the concepts and codes it defines) are represented in this 4718 * resource instance.). This is the underlying object with id, 4719 * value and extensions. The accessor "getContent" gives direct 4720 * access to the value 4721 */ 4722 public CodeSystem setContentElement(Enumeration<CodeSystemContentMode> value) { 4723 this.content = value; 4724 return this; 4725 } 4726 4727 /** 4728 * @return The extent of the content of the code system (the concepts and codes 4729 * it defines) are represented in this resource instance. 4730 */ 4731 public CodeSystemContentMode getContent() { 4732 return this.content == null ? null : this.content.getValue(); 4733 } 4734 4735 /** 4736 * @param value The extent of the content of the code system (the concepts and 4737 * codes it defines) are represented in this resource instance. 4738 */ 4739 public CodeSystem setContent(CodeSystemContentMode value) { 4740 if (this.content == null) 4741 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); 4742 this.content.setValue(value); 4743 return this; 4744 } 4745 4746 /** 4747 * @return {@link #supplements} (The canonical URL of the code system that this 4748 * code system supplement is adding designations and properties to.). 4749 * This is the underlying object with id, value and extensions. The 4750 * accessor "getSupplements" gives direct access to the value 4751 */ 4752 public CanonicalType getSupplementsElement() { 4753 if (this.supplements == null) 4754 if (Configuration.errorOnAutoCreate()) 4755 throw new Error("Attempt to auto-create CodeSystem.supplements"); 4756 else if (Configuration.doAutoCreate()) 4757 this.supplements = new CanonicalType(); // bb 4758 return this.supplements; 4759 } 4760 4761 public boolean hasSupplementsElement() { 4762 return this.supplements != null && !this.supplements.isEmpty(); 4763 } 4764 4765 public boolean hasSupplements() { 4766 return this.supplements != null && !this.supplements.isEmpty(); 4767 } 4768 4769 /** 4770 * @param value {@link #supplements} (The canonical URL of the code system that 4771 * this code system supplement is adding designations and 4772 * properties to.). This is the underlying object with id, value 4773 * and extensions. The accessor "getSupplements" gives direct 4774 * access to the value 4775 */ 4776 public CodeSystem setSupplementsElement(CanonicalType value) { 4777 this.supplements = value; 4778 return this; 4779 } 4780 4781 /** 4782 * @return The canonical URL of the code system that this code system supplement 4783 * is adding designations and properties to. 4784 */ 4785 public String getSupplements() { 4786 return this.supplements == null ? null : this.supplements.getValue(); 4787 } 4788 4789 /** 4790 * @param value The canonical URL of the code system that this code system 4791 * supplement is adding designations and properties to. 4792 */ 4793 public CodeSystem setSupplements(String value) { 4794 if (Utilities.noString(value)) 4795 this.supplements = null; 4796 else { 4797 if (this.supplements == null) 4798 this.supplements = new CanonicalType(); 4799 this.supplements.setValue(value); 4800 } 4801 return this; 4802 } 4803 4804 /** 4805 * @return {@link #count} (The total number of concepts defined by the code 4806 * system. Where the code system has a compositional grammar, the basis 4807 * of this count is defined by the system steward.). This is the 4808 * underlying object with id, value and extensions. The accessor 4809 * "getCount" gives direct access to the value 4810 */ 4811 public UnsignedIntType getCountElement() { 4812 if (this.count == null) 4813 if (Configuration.errorOnAutoCreate()) 4814 throw new Error("Attempt to auto-create CodeSystem.count"); 4815 else if (Configuration.doAutoCreate()) 4816 this.count = new UnsignedIntType(); // bb 4817 return this.count; 4818 } 4819 4820 public boolean hasCountElement() { 4821 return this.count != null && !this.count.isEmpty(); 4822 } 4823 4824 public boolean hasCount() { 4825 return this.count != null && !this.count.isEmpty(); 4826 } 4827 4828 /** 4829 * @param value {@link #count} (The total number of concepts defined by the code 4830 * system. Where the code system has a compositional grammar, the 4831 * basis of this count is defined by the system steward.). This is 4832 * the underlying object with id, value and extensions. The 4833 * accessor "getCount" gives direct access to the value 4834 */ 4835 public CodeSystem setCountElement(UnsignedIntType value) { 4836 this.count = value; 4837 return this; 4838 } 4839 4840 /** 4841 * @return The total number of concepts defined by the code system. Where the 4842 * code system has a compositional grammar, the basis of this count is 4843 * defined by the system steward. 4844 */ 4845 public int getCount() { 4846 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 4847 } 4848 4849 /** 4850 * @param value The total number of concepts defined by the code system. Where 4851 * the code system has a compositional grammar, the basis of this 4852 * count is defined by the system steward. 4853 */ 4854 public CodeSystem setCount(int value) { 4855 if (this.count == null) 4856 this.count = new UnsignedIntType(); 4857 this.count.setValue(value); 4858 return this; 4859 } 4860 4861 /** 4862 * @return {@link #filter} (A filter that can be used in a value set compose 4863 * statement when selecting concepts using a filter.) 4864 */ 4865 public List<CodeSystemFilterComponent> getFilter() { 4866 if (this.filter == null) 4867 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4868 return this.filter; 4869 } 4870 4871 /** 4872 * @return Returns a reference to <code>this</code> for easy method chaining 4873 */ 4874 public CodeSystem setFilter(List<CodeSystemFilterComponent> theFilter) { 4875 this.filter = theFilter; 4876 return this; 4877 } 4878 4879 public boolean hasFilter() { 4880 if (this.filter == null) 4881 return false; 4882 for (CodeSystemFilterComponent item : this.filter) 4883 if (!item.isEmpty()) 4884 return true; 4885 return false; 4886 } 4887 4888 public CodeSystemFilterComponent addFilter() { // 3 4889 CodeSystemFilterComponent t = new CodeSystemFilterComponent(); 4890 if (this.filter == null) 4891 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4892 this.filter.add(t); 4893 return t; 4894 } 4895 4896 public CodeSystem addFilter(CodeSystemFilterComponent t) { // 3 4897 if (t == null) 4898 return this; 4899 if (this.filter == null) 4900 this.filter = new ArrayList<CodeSystemFilterComponent>(); 4901 this.filter.add(t); 4902 return this; 4903 } 4904 4905 /** 4906 * @return The first repetition of repeating field {@link #filter}, creating it 4907 * if it does not already exist 4908 */ 4909 public CodeSystemFilterComponent getFilterFirstRep() { 4910 if (getFilter().isEmpty()) { 4911 addFilter(); 4912 } 4913 return getFilter().get(0); 4914 } 4915 4916 /** 4917 * @return {@link #property} (A property defines an additional slot through 4918 * which additional information can be provided about a concept.) 4919 */ 4920 public List<PropertyComponent> getProperty() { 4921 if (this.property == null) 4922 this.property = new ArrayList<PropertyComponent>(); 4923 return this.property; 4924 } 4925 4926 /** 4927 * @return Returns a reference to <code>this</code> for easy method chaining 4928 */ 4929 public CodeSystem setProperty(List<PropertyComponent> theProperty) { 4930 this.property = theProperty; 4931 return this; 4932 } 4933 4934 public boolean hasProperty() { 4935 if (this.property == null) 4936 return false; 4937 for (PropertyComponent item : this.property) 4938 if (!item.isEmpty()) 4939 return true; 4940 return false; 4941 } 4942 4943 public PropertyComponent addProperty() { // 3 4944 PropertyComponent t = new PropertyComponent(); 4945 if (this.property == null) 4946 this.property = new ArrayList<PropertyComponent>(); 4947 this.property.add(t); 4948 return t; 4949 } 4950 4951 public CodeSystem addProperty(PropertyComponent t) { // 3 4952 if (t == null) 4953 return this; 4954 if (this.property == null) 4955 this.property = new ArrayList<PropertyComponent>(); 4956 this.property.add(t); 4957 return this; 4958 } 4959 4960 /** 4961 * @return The first repetition of repeating field {@link #property}, creating 4962 * it if it does not already exist 4963 */ 4964 public PropertyComponent getPropertyFirstRep() { 4965 if (getProperty().isEmpty()) { 4966 addProperty(); 4967 } 4968 return getProperty().get(0); 4969 } 4970 4971 /** 4972 * @return {@link #concept} (Concepts that are in the code system. The concept 4973 * definitions are inherently hierarchical, but the definitions must be 4974 * consulted to determine what the meanings of the hierarchical 4975 * relationships are.) 4976 */ 4977 public List<ConceptDefinitionComponent> getConcept() { 4978 if (this.concept == null) 4979 this.concept = new ArrayList<ConceptDefinitionComponent>(); 4980 return this.concept; 4981 } 4982 4983 /** 4984 * @return Returns a reference to <code>this</code> for easy method chaining 4985 */ 4986 public CodeSystem setConcept(List<ConceptDefinitionComponent> theConcept) { 4987 this.concept = theConcept; 4988 return this; 4989 } 4990 4991 public boolean hasConcept() { 4992 if (this.concept == null) 4993 return false; 4994 for (ConceptDefinitionComponent item : this.concept) 4995 if (!item.isEmpty()) 4996 return true; 4997 return false; 4998 } 4999 5000 public ConceptDefinitionComponent addConcept() { // 3 5001 ConceptDefinitionComponent t = new ConceptDefinitionComponent(); 5002 if (this.concept == null) 5003 this.concept = new ArrayList<ConceptDefinitionComponent>(); 5004 this.concept.add(t); 5005 return t; 5006 } 5007 5008 public CodeSystem addConcept(ConceptDefinitionComponent t) { // 3 5009 if (t == null) 5010 return this; 5011 if (this.concept == null) 5012 this.concept = new ArrayList<ConceptDefinitionComponent>(); 5013 this.concept.add(t); 5014 return this; 5015 } 5016 5017 /** 5018 * @return The first repetition of repeating field {@link #concept}, creating it 5019 * if it does not already exist 5020 */ 5021 public ConceptDefinitionComponent getConceptFirstRep() { 5022 if (getConcept().isEmpty()) { 5023 addConcept(); 5024 } 5025 return getConcept().get(0); 5026 } 5027 5028 protected void listChildren(List<Property> children) { 5029 super.listChildren(children); 5030 children.add(new Property("url", "uri", 5031 "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.", 5032 0, 1, url)); 5033 children.add(new Property("identifier", "Identifier", 5034 "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5035 0, java.lang.Integer.MAX_VALUE, identifier)); 5036 children.add(new Property("version", "string", 5037 "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.", 5038 0, 1, version)); 5039 children.add(new Property("name", "string", 5040 "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5041 0, 1, name)); 5042 children.add( 5043 new Property("title", "string", "A short, descriptive, user-friendly title for the code system.", 0, 1, title)); 5044 children.add(new Property("status", "code", 5045 "The date (and optionally time) when the code system resource was created or revised.", 0, 1, status)); 5046 children.add(new Property("experimental", "boolean", 5047 "A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5048 0, 1, experimental)); 5049 children.add(new Property("date", "dateTime", 5050 "The date (and optionally time) when the code system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 5051 0, 1, date)); 5052 children.add(new Property("publisher", "string", 5053 "The name of the organization or individual that published the code system.", 0, 1, publisher)); 5054 children.add(new Property("contact", "ContactDetail", 5055 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5056 java.lang.Integer.MAX_VALUE, contact)); 5057 children.add(new Property("description", "markdown", 5058 "A free text natural language description of the code system from a consumer's perspective.", 0, 1, 5059 description)); 5060 children.add(new Property("useContext", "UsageContext", 5061 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances.", 5062 0, java.lang.Integer.MAX_VALUE, useContext)); 5063 children.add(new Property("jurisdiction", "CodeableConcept", 5064 "A legal or geographic region in which the code system is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 5065 jurisdiction)); 5066 children.add(new Property("purpose", "markdown", 5067 "Explanation of why this code system is needed and why it has been designed as it has.", 0, 1, purpose)); 5068 children.add(new Property("copyright", "markdown", 5069 "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 5070 0, 1, copyright)); 5071 children.add(new Property("caseSensitive", "boolean", 5072 "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, 5073 caseSensitive)); 5074 children.add(new Property("valueSet", "canonical(ValueSet)", 5075 "Canonical reference to the value set that contains the entire code system.", 0, 1, valueSet)); 5076 children.add(new Property("hierarchyMeaning", "code", 5077 "The meaning of the hierarchy of concepts as represented in this resource.", 0, 1, hierarchyMeaning)); 5078 children.add(new Property("compositional", "boolean", 5079 "The code system defines a compositional (post-coordination) grammar.", 0, 1, compositional)); 5080 children.add(new Property("versionNeeded", "boolean", 5081 "This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.", 5082 0, 1, versionNeeded)); 5083 children.add(new Property("content", "code", 5084 "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 5085 0, 1, content)); 5086 children.add(new Property("supplements", "canonical(CodeSystem)", 5087 "The canonical URL of the code system that this code system supplement is adding designations and properties to.", 5088 0, 1, supplements)); 5089 children.add(new Property("count", "unsignedInt", 5090 "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.", 5091 0, 1, count)); 5092 children.add(new Property("filter", "", 5093 "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, 5094 java.lang.Integer.MAX_VALUE, filter)); 5095 children.add(new Property("property", "", 5096 "A property defines an additional slot through which additional information can be provided about a concept.", 5097 0, java.lang.Integer.MAX_VALUE, property)); 5098 children.add(new Property("concept", "", 5099 "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.", 5100 0, java.lang.Integer.MAX_VALUE, concept)); 5101 } 5102 5103 @Override 5104 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5105 switch (_hash) { 5106 case 116079: 5107 /* url */ return new Property("url", "uri", 5108 "An absolute URI that is used to identify this code system when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this code system is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the code system is stored on different servers. This is used in [Coding](datatypes.html#Coding).system.", 5109 0, 1, url); 5110 case -1618432855: 5111 /* identifier */ return new Property("identifier", "Identifier", 5112 "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5113 0, java.lang.Integer.MAX_VALUE, identifier); 5114 case 351608024: 5115 /* version */ return new Property("version", "string", 5116 "The identifier that is used to identify this version of the code system when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the code system author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. This is used in [Coding](datatypes.html#Coding).version.", 5117 0, 1, version); 5118 case 3373707: 5119 /* name */ return new Property("name", "string", 5120 "A natural language name identifying the code system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5121 0, 1, name); 5122 case 110371416: 5123 /* title */ return new Property("title", "string", 5124 "A short, descriptive, user-friendly title for the code system.", 0, 1, title); 5125 case -892481550: 5126 /* status */ return new Property("status", "code", 5127 "The date (and optionally time) when the code system resource was created or revised.", 0, 1, status); 5128 case -404562712: 5129 /* experimental */ return new Property("experimental", "boolean", 5130 "A Boolean value to indicate that this code system is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5131 0, 1, experimental); 5132 case 3076014: 5133 /* date */ return new Property("date", "dateTime", 5134 "The date (and optionally time) when the code system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the code system changes.", 5135 0, 1, date); 5136 case 1447404028: 5137 /* publisher */ return new Property("publisher", "string", 5138 "The name of the organization or individual that published the code system.", 0, 1, publisher); 5139 case 951526432: 5140 /* contact */ return new Property("contact", "ContactDetail", 5141 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5142 java.lang.Integer.MAX_VALUE, contact); 5143 case -1724546052: 5144 /* description */ return new Property("description", "markdown", 5145 "A free text natural language description of the code system from a consumer's perspective.", 0, 1, 5146 description); 5147 case -669707736: 5148 /* useContext */ return new Property("useContext", "UsageContext", 5149 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate code system instances.", 5150 0, java.lang.Integer.MAX_VALUE, useContext); 5151 case -507075711: 5152 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 5153 "A legal or geographic region in which the code system is intended to be used.", 0, 5154 java.lang.Integer.MAX_VALUE, jurisdiction); 5155 case -220463842: 5156 /* purpose */ return new Property("purpose", "markdown", 5157 "Explanation of why this code system is needed and why it has been designed as it has.", 0, 1, purpose); 5158 case 1522889671: 5159 /* copyright */ return new Property("copyright", "markdown", 5160 "A copyright statement relating to the code system and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the code system.", 5161 0, 1, copyright); 5162 case -35616442: 5163 /* caseSensitive */ return new Property("caseSensitive", "boolean", 5164 "If code comparison is case sensitive when codes within this system are compared to each other.", 0, 1, 5165 caseSensitive); 5166 case -1410174671: 5167 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 5168 "Canonical reference to the value set that contains the entire code system.", 0, 1, valueSet); 5169 case 1913078280: 5170 /* hierarchyMeaning */ return new Property("hierarchyMeaning", "code", 5171 "The meaning of the hierarchy of concepts as represented in this resource.", 0, 1, hierarchyMeaning); 5172 case 1248023381: 5173 /* compositional */ return new Property("compositional", "boolean", 5174 "The code system defines a compositional (post-coordination) grammar.", 0, 1, compositional); 5175 case 617270957: 5176 /* versionNeeded */ return new Property("versionNeeded", "boolean", 5177 "This flag is used to signify that the code system does not commit to concept permanence across versions. If true, a version must be specified when referencing this code system.", 5178 0, 1, versionNeeded); 5179 case 951530617: 5180 /* content */ return new Property("content", "code", 5181 "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 5182 0, 1, content); 5183 case -596951334: 5184 /* supplements */ return new Property("supplements", "canonical(CodeSystem)", 5185 "The canonical URL of the code system that this code system supplement is adding designations and properties to.", 5186 0, 1, supplements); 5187 case 94851343: 5188 /* count */ return new Property("count", "unsignedInt", 5189 "The total number of concepts defined by the code system. Where the code system has a compositional grammar, the basis of this count is defined by the system steward.", 5190 0, 1, count); 5191 case -1274492040: 5192 /* filter */ return new Property("filter", "", 5193 "A filter that can be used in a value set compose statement when selecting concepts using a filter.", 0, 5194 java.lang.Integer.MAX_VALUE, filter); 5195 case -993141291: 5196 /* property */ return new Property("property", "", 5197 "A property defines an additional slot through which additional information can be provided about a concept.", 5198 0, java.lang.Integer.MAX_VALUE, property); 5199 case 951024232: 5200 /* concept */ return new Property("concept", "", 5201 "Concepts that are in the code system. The concept definitions are inherently hierarchical, but the definitions must be consulted to determine what the meanings of the hierarchical relationships are.", 5202 0, java.lang.Integer.MAX_VALUE, concept); 5203 default: 5204 return super.getNamedProperty(_hash, _name, _checkValid); 5205 } 5206 5207 } 5208 5209 @Override 5210 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5211 switch (hash) { 5212 case 116079: 5213 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5214 case -1618432855: 5215 /* identifier */ return this.identifier == null ? new Base[0] 5216 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5217 case 351608024: 5218 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5219 case 3373707: 5220 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5221 case 110371416: 5222 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5223 case -892481550: 5224 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5225 case -404562712: 5226 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5227 case 3076014: 5228 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5229 case 1447404028: 5230 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5231 case 951526432: 5232 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5233 case -1724546052: 5234 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5235 case -669707736: 5236 /* useContext */ return this.useContext == null ? new Base[0] 5237 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5238 case -507075711: 5239 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5240 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5241 case -220463842: 5242 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5243 case 1522889671: 5244 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5245 case -35616442: 5246 /* caseSensitive */ return this.caseSensitive == null ? new Base[0] : new Base[] { this.caseSensitive }; // BooleanType 5247 case -1410174671: 5248 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 5249 case 1913078280: 5250 /* hierarchyMeaning */ return this.hierarchyMeaning == null ? new Base[0] : new Base[] { this.hierarchyMeaning }; // Enumeration<CodeSystemHierarchyMeaning> 5251 case 1248023381: 5252 /* compositional */ return this.compositional == null ? new Base[0] : new Base[] { this.compositional }; // BooleanType 5253 case 617270957: 5254 /* versionNeeded */ return this.versionNeeded == null ? new Base[0] : new Base[] { this.versionNeeded }; // BooleanType 5255 case 951530617: 5256 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Enumeration<CodeSystemContentMode> 5257 case -596951334: 5258 /* supplements */ return this.supplements == null ? new Base[0] : new Base[] { this.supplements }; // CanonicalType 5259 case 94851343: 5260 /* count */ return this.count == null ? new Base[0] : new Base[] { this.count }; // UnsignedIntType 5261 case -1274492040: 5262 /* filter */ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // CodeSystemFilterComponent 5263 case -993141291: 5264 /* property */ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // PropertyComponent 5265 case 951024232: 5266 /* concept */ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptDefinitionComponent 5267 default: 5268 return super.getProperty(hash, name, checkValid); 5269 } 5270 5271 } 5272 5273 @Override 5274 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5275 switch (hash) { 5276 case 116079: // url 5277 this.url = castToUri(value); // UriType 5278 return value; 5279 case -1618432855: // identifier 5280 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5281 return value; 5282 case 351608024: // version 5283 this.version = castToString(value); // StringType 5284 return value; 5285 case 3373707: // name 5286 this.name = castToString(value); // StringType 5287 return value; 5288 case 110371416: // title 5289 this.title = castToString(value); // StringType 5290 return value; 5291 case -892481550: // status 5292 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5293 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5294 return value; 5295 case -404562712: // experimental 5296 this.experimental = castToBoolean(value); // BooleanType 5297 return value; 5298 case 3076014: // date 5299 this.date = castToDateTime(value); // DateTimeType 5300 return value; 5301 case 1447404028: // publisher 5302 this.publisher = castToString(value); // StringType 5303 return value; 5304 case 951526432: // contact 5305 this.getContact().add(castToContactDetail(value)); // ContactDetail 5306 return value; 5307 case -1724546052: // description 5308 this.description = castToMarkdown(value); // MarkdownType 5309 return value; 5310 case -669707736: // useContext 5311 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5312 return value; 5313 case -507075711: // jurisdiction 5314 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5315 return value; 5316 case -220463842: // purpose 5317 this.purpose = castToMarkdown(value); // MarkdownType 5318 return value; 5319 case 1522889671: // copyright 5320 this.copyright = castToMarkdown(value); // MarkdownType 5321 return value; 5322 case -35616442: // caseSensitive 5323 this.caseSensitive = castToBoolean(value); // BooleanType 5324 return value; 5325 case -1410174671: // valueSet 5326 this.valueSet = castToCanonical(value); // CanonicalType 5327 return value; 5328 case 1913078280: // hierarchyMeaning 5329 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(castToCode(value)); 5330 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 5331 return value; 5332 case 1248023381: // compositional 5333 this.compositional = castToBoolean(value); // BooleanType 5334 return value; 5335 case 617270957: // versionNeeded 5336 this.versionNeeded = castToBoolean(value); // BooleanType 5337 return value; 5338 case 951530617: // content 5339 value = new CodeSystemContentModeEnumFactory().fromType(castToCode(value)); 5340 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 5341 return value; 5342 case -596951334: // supplements 5343 this.supplements = castToCanonical(value); // CanonicalType 5344 return value; 5345 case 94851343: // count 5346 this.count = castToUnsignedInt(value); // UnsignedIntType 5347 return value; 5348 case -1274492040: // filter 5349 this.getFilter().add((CodeSystemFilterComponent) value); // CodeSystemFilterComponent 5350 return value; 5351 case -993141291: // property 5352 this.getProperty().add((PropertyComponent) value); // PropertyComponent 5353 return value; 5354 case 951024232: // concept 5355 this.getConcept().add((ConceptDefinitionComponent) value); // ConceptDefinitionComponent 5356 return value; 5357 default: 5358 return super.setProperty(hash, name, value); 5359 } 5360 5361 } 5362 5363 @Override 5364 public Base setProperty(String name, Base value) throws FHIRException { 5365 if (name.equals("url")) { 5366 this.url = castToUri(value); // UriType 5367 } else if (name.equals("identifier")) { 5368 this.getIdentifier().add(castToIdentifier(value)); 5369 } else if (name.equals("version")) { 5370 this.version = castToString(value); // StringType 5371 } else if (name.equals("name")) { 5372 this.name = castToString(value); // StringType 5373 } else if (name.equals("title")) { 5374 this.title = castToString(value); // StringType 5375 } else if (name.equals("status")) { 5376 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5377 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5378 } else if (name.equals("experimental")) { 5379 this.experimental = castToBoolean(value); // BooleanType 5380 } else if (name.equals("date")) { 5381 this.date = castToDateTime(value); // DateTimeType 5382 } else if (name.equals("publisher")) { 5383 this.publisher = castToString(value); // StringType 5384 } else if (name.equals("contact")) { 5385 this.getContact().add(castToContactDetail(value)); 5386 } else if (name.equals("description")) { 5387 this.description = castToMarkdown(value); // MarkdownType 5388 } else if (name.equals("useContext")) { 5389 this.getUseContext().add(castToUsageContext(value)); 5390 } else if (name.equals("jurisdiction")) { 5391 this.getJurisdiction().add(castToCodeableConcept(value)); 5392 } else if (name.equals("purpose")) { 5393 this.purpose = castToMarkdown(value); // MarkdownType 5394 } else if (name.equals("copyright")) { 5395 this.copyright = castToMarkdown(value); // MarkdownType 5396 } else if (name.equals("caseSensitive")) { 5397 this.caseSensitive = castToBoolean(value); // BooleanType 5398 } else if (name.equals("valueSet")) { 5399 this.valueSet = castToCanonical(value); // CanonicalType 5400 } else if (name.equals("hierarchyMeaning")) { 5401 value = new CodeSystemHierarchyMeaningEnumFactory().fromType(castToCode(value)); 5402 this.hierarchyMeaning = (Enumeration) value; // Enumeration<CodeSystemHierarchyMeaning> 5403 } else if (name.equals("compositional")) { 5404 this.compositional = castToBoolean(value); // BooleanType 5405 } else if (name.equals("versionNeeded")) { 5406 this.versionNeeded = castToBoolean(value); // BooleanType 5407 } else if (name.equals("content")) { 5408 value = new CodeSystemContentModeEnumFactory().fromType(castToCode(value)); 5409 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 5410 } else if (name.equals("supplements")) { 5411 this.supplements = castToCanonical(value); // CanonicalType 5412 } else if (name.equals("count")) { 5413 this.count = castToUnsignedInt(value); // UnsignedIntType 5414 } else if (name.equals("filter")) { 5415 this.getFilter().add((CodeSystemFilterComponent) value); 5416 } else if (name.equals("property")) { 5417 this.getProperty().add((PropertyComponent) value); 5418 } else if (name.equals("concept")) { 5419 this.getConcept().add((ConceptDefinitionComponent) value); 5420 } else 5421 return super.setProperty(name, value); 5422 return value; 5423 } 5424 5425 @Override 5426 public void removeChild(String name, Base value) throws FHIRException { 5427 if (name.equals("url")) { 5428 this.url = null; 5429 } else if (name.equals("identifier")) { 5430 this.getIdentifier().remove(castToIdentifier(value)); 5431 } else if (name.equals("version")) { 5432 this.version = null; 5433 } else if (name.equals("name")) { 5434 this.name = null; 5435 } else if (name.equals("title")) { 5436 this.title = null; 5437 } else if (name.equals("status")) { 5438 this.status = null; 5439 } else if (name.equals("experimental")) { 5440 this.experimental = null; 5441 } else if (name.equals("date")) { 5442 this.date = null; 5443 } else if (name.equals("publisher")) { 5444 this.publisher = null; 5445 } else if (name.equals("contact")) { 5446 this.getContact().remove(castToContactDetail(value)); 5447 } else if (name.equals("description")) { 5448 this.description = null; 5449 } else if (name.equals("useContext")) { 5450 this.getUseContext().remove(castToUsageContext(value)); 5451 } else if (name.equals("jurisdiction")) { 5452 this.getJurisdiction().remove(castToCodeableConcept(value)); 5453 } else if (name.equals("purpose")) { 5454 this.purpose = null; 5455 } else if (name.equals("copyright")) { 5456 this.copyright = null; 5457 } else if (name.equals("caseSensitive")) { 5458 this.caseSensitive = null; 5459 } else if (name.equals("valueSet")) { 5460 this.valueSet = null; 5461 } else if (name.equals("hierarchyMeaning")) { 5462 this.hierarchyMeaning = null; 5463 } else if (name.equals("compositional")) { 5464 this.compositional = null; 5465 } else if (name.equals("versionNeeded")) { 5466 this.versionNeeded = null; 5467 } else if (name.equals("content")) { 5468 this.content = null; 5469 } else if (name.equals("supplements")) { 5470 this.supplements = null; 5471 } else if (name.equals("count")) { 5472 this.count = null; 5473 } else if (name.equals("filter")) { 5474 this.getFilter().remove((CodeSystemFilterComponent) value); 5475 } else if (name.equals("property")) { 5476 this.getProperty().remove((PropertyComponent) value); 5477 } else if (name.equals("concept")) { 5478 this.getConcept().remove((ConceptDefinitionComponent) value); 5479 } else 5480 super.removeChild(name, value); 5481 5482 } 5483 5484 @Override 5485 public Base makeProperty(int hash, String name) throws FHIRException { 5486 switch (hash) { 5487 case 116079: 5488 return getUrlElement(); 5489 case -1618432855: 5490 return addIdentifier(); 5491 case 351608024: 5492 return getVersionElement(); 5493 case 3373707: 5494 return getNameElement(); 5495 case 110371416: 5496 return getTitleElement(); 5497 case -892481550: 5498 return getStatusElement(); 5499 case -404562712: 5500 return getExperimentalElement(); 5501 case 3076014: 5502 return getDateElement(); 5503 case 1447404028: 5504 return getPublisherElement(); 5505 case 951526432: 5506 return addContact(); 5507 case -1724546052: 5508 return getDescriptionElement(); 5509 case -669707736: 5510 return addUseContext(); 5511 case -507075711: 5512 return addJurisdiction(); 5513 case -220463842: 5514 return getPurposeElement(); 5515 case 1522889671: 5516 return getCopyrightElement(); 5517 case -35616442: 5518 return getCaseSensitiveElement(); 5519 case -1410174671: 5520 return getValueSetElement(); 5521 case 1913078280: 5522 return getHierarchyMeaningElement(); 5523 case 1248023381: 5524 return getCompositionalElement(); 5525 case 617270957: 5526 return getVersionNeededElement(); 5527 case 951530617: 5528 return getContentElement(); 5529 case -596951334: 5530 return getSupplementsElement(); 5531 case 94851343: 5532 return getCountElement(); 5533 case -1274492040: 5534 return addFilter(); 5535 case -993141291: 5536 return addProperty(); 5537 case 951024232: 5538 return addConcept(); 5539 default: 5540 return super.makeProperty(hash, name); 5541 } 5542 5543 } 5544 5545 @Override 5546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5547 switch (hash) { 5548 case 116079: 5549 /* url */ return new String[] { "uri" }; 5550 case -1618432855: 5551 /* identifier */ return new String[] { "Identifier" }; 5552 case 351608024: 5553 /* version */ return new String[] { "string" }; 5554 case 3373707: 5555 /* name */ return new String[] { "string" }; 5556 case 110371416: 5557 /* title */ return new String[] { "string" }; 5558 case -892481550: 5559 /* status */ return new String[] { "code" }; 5560 case -404562712: 5561 /* experimental */ return new String[] { "boolean" }; 5562 case 3076014: 5563 /* date */ return new String[] { "dateTime" }; 5564 case 1447404028: 5565 /* publisher */ return new String[] { "string" }; 5566 case 951526432: 5567 /* contact */ return new String[] { "ContactDetail" }; 5568 case -1724546052: 5569 /* description */ return new String[] { "markdown" }; 5570 case -669707736: 5571 /* useContext */ return new String[] { "UsageContext" }; 5572 case -507075711: 5573 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5574 case -220463842: 5575 /* purpose */ return new String[] { "markdown" }; 5576 case 1522889671: 5577 /* copyright */ return new String[] { "markdown" }; 5578 case -35616442: 5579 /* caseSensitive */ return new String[] { "boolean" }; 5580 case -1410174671: 5581 /* valueSet */ return new String[] { "canonical" }; 5582 case 1913078280: 5583 /* hierarchyMeaning */ return new String[] { "code" }; 5584 case 1248023381: 5585 /* compositional */ return new String[] { "boolean" }; 5586 case 617270957: 5587 /* versionNeeded */ return new String[] { "boolean" }; 5588 case 951530617: 5589 /* content */ return new String[] { "code" }; 5590 case -596951334: 5591 /* supplements */ return new String[] { "canonical" }; 5592 case 94851343: 5593 /* count */ return new String[] { "unsignedInt" }; 5594 case -1274492040: 5595 /* filter */ return new String[] {}; 5596 case -993141291: 5597 /* property */ return new String[] {}; 5598 case 951024232: 5599 /* concept */ return new String[] {}; 5600 default: 5601 return super.getTypesForProperty(hash, name); 5602 } 5603 5604 } 5605 5606 @Override 5607 public Base addChild(String name) throws FHIRException { 5608 if (name.equals("url")) { 5609 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.url"); 5610 } else if (name.equals("identifier")) { 5611 return addIdentifier(); 5612 } else if (name.equals("version")) { 5613 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.version"); 5614 } else if (name.equals("name")) { 5615 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.name"); 5616 } else if (name.equals("title")) { 5617 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.title"); 5618 } else if (name.equals("status")) { 5619 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.status"); 5620 } else if (name.equals("experimental")) { 5621 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.experimental"); 5622 } else if (name.equals("date")) { 5623 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.date"); 5624 } else if (name.equals("publisher")) { 5625 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.publisher"); 5626 } else if (name.equals("contact")) { 5627 return addContact(); 5628 } else if (name.equals("description")) { 5629 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.description"); 5630 } else if (name.equals("useContext")) { 5631 return addUseContext(); 5632 } else if (name.equals("jurisdiction")) { 5633 return addJurisdiction(); 5634 } else if (name.equals("purpose")) { 5635 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.purpose"); 5636 } else if (name.equals("copyright")) { 5637 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.copyright"); 5638 } else if (name.equals("caseSensitive")) { 5639 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.caseSensitive"); 5640 } else if (name.equals("valueSet")) { 5641 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.valueSet"); 5642 } else if (name.equals("hierarchyMeaning")) { 5643 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.hierarchyMeaning"); 5644 } else if (name.equals("compositional")) { 5645 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.compositional"); 5646 } else if (name.equals("versionNeeded")) { 5647 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.versionNeeded"); 5648 } else if (name.equals("content")) { 5649 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.content"); 5650 } else if (name.equals("supplements")) { 5651 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.supplements"); 5652 } else if (name.equals("count")) { 5653 throw new FHIRException("Cannot call addChild on a singleton property CodeSystem.count"); 5654 } else if (name.equals("filter")) { 5655 return addFilter(); 5656 } else if (name.equals("property")) { 5657 return addProperty(); 5658 } else if (name.equals("concept")) { 5659 return addConcept(); 5660 } else 5661 return super.addChild(name); 5662 } 5663 5664 public String fhirType() { 5665 return "CodeSystem"; 5666 5667 } 5668 5669 public CodeSystem copy() { 5670 CodeSystem dst = new CodeSystem(); 5671 copyValues(dst); 5672 return dst; 5673 } 5674 5675 public void copyValues(CodeSystem dst) { 5676 super.copyValues(dst); 5677 dst.url = url == null ? null : url.copy(); 5678 if (identifier != null) { 5679 dst.identifier = new ArrayList<Identifier>(); 5680 for (Identifier i : identifier) 5681 dst.identifier.add(i.copy()); 5682 } 5683 ; 5684 dst.version = version == null ? null : version.copy(); 5685 dst.name = name == null ? null : name.copy(); 5686 dst.title = title == null ? null : title.copy(); 5687 dst.status = status == null ? null : status.copy(); 5688 dst.experimental = experimental == null ? null : experimental.copy(); 5689 dst.date = date == null ? null : date.copy(); 5690 dst.publisher = publisher == null ? null : publisher.copy(); 5691 if (contact != null) { 5692 dst.contact = new ArrayList<ContactDetail>(); 5693 for (ContactDetail i : contact) 5694 dst.contact.add(i.copy()); 5695 } 5696 ; 5697 dst.description = description == null ? null : description.copy(); 5698 if (useContext != null) { 5699 dst.useContext = new ArrayList<UsageContext>(); 5700 for (UsageContext i : useContext) 5701 dst.useContext.add(i.copy()); 5702 } 5703 ; 5704 if (jurisdiction != null) { 5705 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5706 for (CodeableConcept i : jurisdiction) 5707 dst.jurisdiction.add(i.copy()); 5708 } 5709 ; 5710 dst.purpose = purpose == null ? null : purpose.copy(); 5711 dst.copyright = copyright == null ? null : copyright.copy(); 5712 dst.caseSensitive = caseSensitive == null ? null : caseSensitive.copy(); 5713 dst.valueSet = valueSet == null ? null : valueSet.copy(); 5714 dst.hierarchyMeaning = hierarchyMeaning == null ? null : hierarchyMeaning.copy(); 5715 dst.compositional = compositional == null ? null : compositional.copy(); 5716 dst.versionNeeded = versionNeeded == null ? null : versionNeeded.copy(); 5717 dst.content = content == null ? null : content.copy(); 5718 dst.supplements = supplements == null ? null : supplements.copy(); 5719 dst.count = count == null ? null : count.copy(); 5720 if (filter != null) { 5721 dst.filter = new ArrayList<CodeSystemFilterComponent>(); 5722 for (CodeSystemFilterComponent i : filter) 5723 dst.filter.add(i.copy()); 5724 } 5725 ; 5726 if (property != null) { 5727 dst.property = new ArrayList<PropertyComponent>(); 5728 for (PropertyComponent i : property) 5729 dst.property.add(i.copy()); 5730 } 5731 ; 5732 if (concept != null) { 5733 dst.concept = new ArrayList<ConceptDefinitionComponent>(); 5734 for (ConceptDefinitionComponent i : concept) 5735 dst.concept.add(i.copy()); 5736 } 5737 ; 5738 } 5739 5740 protected CodeSystem typedCopy() { 5741 return copy(); 5742 } 5743 5744 @Override 5745 public boolean equalsDeep(Base other_) { 5746 if (!super.equalsDeep(other_)) 5747 return false; 5748 if (!(other_ instanceof CodeSystem)) 5749 return false; 5750 CodeSystem o = (CodeSystem) other_; 5751 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 5752 && compareDeep(copyright, o.copyright, true) && compareDeep(caseSensitive, o.caseSensitive, true) 5753 && compareDeep(valueSet, o.valueSet, true) && compareDeep(hierarchyMeaning, o.hierarchyMeaning, true) 5754 && compareDeep(compositional, o.compositional, true) && compareDeep(versionNeeded, o.versionNeeded, true) 5755 && compareDeep(content, o.content, true) && compareDeep(supplements, o.supplements, true) 5756 && compareDeep(count, o.count, true) && compareDeep(filter, o.filter, true) 5757 && compareDeep(property, o.property, true) && compareDeep(concept, o.concept, true); 5758 } 5759 5760 @Override 5761 public boolean equalsShallow(Base other_) { 5762 if (!super.equalsShallow(other_)) 5763 return false; 5764 if (!(other_ instanceof CodeSystem)) 5765 return false; 5766 CodeSystem o = (CodeSystem) other_; 5767 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 5768 && compareValues(caseSensitive, o.caseSensitive, true) 5769 && compareValues(hierarchyMeaning, o.hierarchyMeaning, true) 5770 && compareValues(compositional, o.compositional, true) && compareValues(versionNeeded, o.versionNeeded, true) 5771 && compareValues(content, o.content, true) && compareValues(count, o.count, true); 5772 } 5773 5774 public boolean isEmpty() { 5775 return super.isEmpty() 5776 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, caseSensitive, valueSet, 5777 hierarchyMeaning, compositional, versionNeeded, content, supplements, count, filter, property, concept); 5778 } 5779 5780 @Override 5781 public ResourceType getResourceType() { 5782 return ResourceType.CodeSystem; 5783 } 5784 5785 /** 5786 * Search parameter: <b>date</b> 5787 * <p> 5788 * Description: <b>The code system publication date</b><br> 5789 * Type: <b>date</b><br> 5790 * Path: <b>CodeSystem.date</b><br> 5791 * </p> 5792 */ 5793 @SearchParamDefinition(name = "date", path = "CodeSystem.date", description = "The code system publication date", type = "date") 5794 public static final String SP_DATE = "date"; 5795 /** 5796 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5797 * <p> 5798 * Description: <b>The code system publication date</b><br> 5799 * Type: <b>date</b><br> 5800 * Path: <b>CodeSystem.date</b><br> 5801 * </p> 5802 */ 5803 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5804 SP_DATE); 5805 5806 /** 5807 * Search parameter: <b>identifier</b> 5808 * <p> 5809 * Description: <b>External identifier for the code system</b><br> 5810 * Type: <b>token</b><br> 5811 * Path: <b>CodeSystem.identifier</b><br> 5812 * </p> 5813 */ 5814 @SearchParamDefinition(name = "identifier", path = "CodeSystem.identifier", description = "External identifier for the code system", type = "token") 5815 public static final String SP_IDENTIFIER = "identifier"; 5816 /** 5817 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5818 * <p> 5819 * Description: <b>External identifier for the code system</b><br> 5820 * Type: <b>token</b><br> 5821 * Path: <b>CodeSystem.identifier</b><br> 5822 * </p> 5823 */ 5824 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5825 SP_IDENTIFIER); 5826 5827 /** 5828 * Search parameter: <b>code</b> 5829 * <p> 5830 * Description: <b>A code defined in the code system</b><br> 5831 * Type: <b>token</b><br> 5832 * Path: <b>CodeSystem.concept.code</b><br> 5833 * </p> 5834 */ 5835 @SearchParamDefinition(name = "code", path = "CodeSystem.concept.code", description = "A code defined in the code system", type = "token") 5836 public static final String SP_CODE = "code"; 5837 /** 5838 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5839 * <p> 5840 * Description: <b>A code defined in the code system</b><br> 5841 * Type: <b>token</b><br> 5842 * Path: <b>CodeSystem.concept.code</b><br> 5843 * </p> 5844 */ 5845 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5846 SP_CODE); 5847 5848 /** 5849 * Search parameter: <b>context-type-value</b> 5850 * <p> 5851 * Description: <b>A use context type and value assigned to the code 5852 * system</b><br> 5853 * Type: <b>composite</b><br> 5854 * Path: <b></b><br> 5855 * </p> 5856 */ 5857 @SearchParamDefinition(name = "context-type-value", path = "CodeSystem.useContext", description = "A use context type and value assigned to the code system", type = "composite", compositeOf = { 5858 "context-type", "context" }) 5859 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5860 /** 5861 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5862 * <p> 5863 * Description: <b>A use context type and value assigned to the code 5864 * system</b><br> 5865 * Type: <b>composite</b><br> 5866 * Path: <b></b><br> 5867 * </p> 5868 */ 5869 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5870 SP_CONTEXT_TYPE_VALUE); 5871 5872 /** 5873 * Search parameter: <b>content-mode</b> 5874 * <p> 5875 * Description: <b>not-present | example | fragment | complete | 5876 * supplement</b><br> 5877 * Type: <b>token</b><br> 5878 * Path: <b>CodeSystem.content</b><br> 5879 * </p> 5880 */ 5881 @SearchParamDefinition(name = "content-mode", path = "CodeSystem.content", description = "not-present | example | fragment | complete | supplement", type = "token") 5882 public static final String SP_CONTENT_MODE = "content-mode"; 5883 /** 5884 * <b>Fluent Client</b> search parameter constant for <b>content-mode</b> 5885 * <p> 5886 * Description: <b>not-present | example | fragment | complete | 5887 * supplement</b><br> 5888 * Type: <b>token</b><br> 5889 * Path: <b>CodeSystem.content</b><br> 5890 * </p> 5891 */ 5892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_MODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5893 SP_CONTENT_MODE); 5894 5895 /** 5896 * Search parameter: <b>jurisdiction</b> 5897 * <p> 5898 * Description: <b>Intended jurisdiction for the code system</b><br> 5899 * Type: <b>token</b><br> 5900 * Path: <b>CodeSystem.jurisdiction</b><br> 5901 * </p> 5902 */ 5903 @SearchParamDefinition(name = "jurisdiction", path = "CodeSystem.jurisdiction", description = "Intended jurisdiction for the code system", type = "token") 5904 public static final String SP_JURISDICTION = "jurisdiction"; 5905 /** 5906 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5907 * <p> 5908 * Description: <b>Intended jurisdiction for the code system</b><br> 5909 * Type: <b>token</b><br> 5910 * Path: <b>CodeSystem.jurisdiction</b><br> 5911 * </p> 5912 */ 5913 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5914 SP_JURISDICTION); 5915 5916 /** 5917 * Search parameter: <b>description</b> 5918 * <p> 5919 * Description: <b>The description of the code system</b><br> 5920 * Type: <b>string</b><br> 5921 * Path: <b>CodeSystem.description</b><br> 5922 * </p> 5923 */ 5924 @SearchParamDefinition(name = "description", path = "CodeSystem.description", description = "The description of the code system", type = "string") 5925 public static final String SP_DESCRIPTION = "description"; 5926 /** 5927 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5928 * <p> 5929 * Description: <b>The description of the code system</b><br> 5930 * Type: <b>string</b><br> 5931 * Path: <b>CodeSystem.description</b><br> 5932 * </p> 5933 */ 5934 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5935 SP_DESCRIPTION); 5936 5937 /** 5938 * Search parameter: <b>context-type</b> 5939 * <p> 5940 * Description: <b>A type of use context assigned to the code system</b><br> 5941 * Type: <b>token</b><br> 5942 * Path: <b>CodeSystem.useContext.code</b><br> 5943 * </p> 5944 */ 5945 @SearchParamDefinition(name = "context-type", path = "CodeSystem.useContext.code", description = "A type of use context assigned to the code system", type = "token") 5946 public static final String SP_CONTEXT_TYPE = "context-type"; 5947 /** 5948 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5949 * <p> 5950 * Description: <b>A type of use context assigned to the code system</b><br> 5951 * Type: <b>token</b><br> 5952 * Path: <b>CodeSystem.useContext.code</b><br> 5953 * </p> 5954 */ 5955 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5956 SP_CONTEXT_TYPE); 5957 5958 /** 5959 * Search parameter: <b>language</b> 5960 * <p> 5961 * Description: <b>A language in which a designation is provided</b><br> 5962 * Type: <b>token</b><br> 5963 * Path: <b>CodeSystem.concept.designation.language</b><br> 5964 * </p> 5965 */ 5966 @SearchParamDefinition(name = "language", path = "CodeSystem.concept.designation.language", description = "A language in which a designation is provided", type = "token") 5967 public static final String SP_LANGUAGE = "language"; 5968 /** 5969 * <b>Fluent Client</b> search parameter constant for <b>language</b> 5970 * <p> 5971 * Description: <b>A language in which a designation is provided</b><br> 5972 * Type: <b>token</b><br> 5973 * Path: <b>CodeSystem.concept.designation.language</b><br> 5974 * </p> 5975 */ 5976 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5977 SP_LANGUAGE); 5978 5979 /** 5980 * Search parameter: <b>title</b> 5981 * <p> 5982 * Description: <b>The human-friendly name of the code system</b><br> 5983 * Type: <b>string</b><br> 5984 * Path: <b>CodeSystem.title</b><br> 5985 * </p> 5986 */ 5987 @SearchParamDefinition(name = "title", path = "CodeSystem.title", description = "The human-friendly name of the code system", type = "string") 5988 public static final String SP_TITLE = "title"; 5989 /** 5990 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5991 * <p> 5992 * Description: <b>The human-friendly name of the code system</b><br> 5993 * Type: <b>string</b><br> 5994 * Path: <b>CodeSystem.title</b><br> 5995 * </p> 5996 */ 5997 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5998 SP_TITLE); 5999 6000 /** 6001 * Search parameter: <b>version</b> 6002 * <p> 6003 * Description: <b>The business version of the code system</b><br> 6004 * Type: <b>token</b><br> 6005 * Path: <b>CodeSystem.version</b><br> 6006 * </p> 6007 */ 6008 @SearchParamDefinition(name = "version", path = "CodeSystem.version", description = "The business version of the code system", type = "token") 6009 public static final String SP_VERSION = "version"; 6010 /** 6011 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6012 * <p> 6013 * Description: <b>The business version of the code system</b><br> 6014 * Type: <b>token</b><br> 6015 * Path: <b>CodeSystem.version</b><br> 6016 * </p> 6017 */ 6018 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6019 SP_VERSION); 6020 6021 /** 6022 * Search parameter: <b>url</b> 6023 * <p> 6024 * Description: <b>The uri that identifies the code system</b><br> 6025 * Type: <b>uri</b><br> 6026 * Path: <b>CodeSystem.url</b><br> 6027 * </p> 6028 */ 6029 @SearchParamDefinition(name = "url", path = "CodeSystem.url", description = "The uri that identifies the code system", type = "uri") 6030 public static final String SP_URL = "url"; 6031 /** 6032 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6033 * <p> 6034 * Description: <b>The uri that identifies the code system</b><br> 6035 * Type: <b>uri</b><br> 6036 * Path: <b>CodeSystem.url</b><br> 6037 * </p> 6038 */ 6039 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6040 6041 /** 6042 * Search parameter: <b>context-quantity</b> 6043 * <p> 6044 * Description: <b>A quantity- or range-valued use context assigned to the code 6045 * system</b><br> 6046 * Type: <b>quantity</b><br> 6047 * Path: <b>CodeSystem.useContext.valueQuantity, 6048 * CodeSystem.useContext.valueRange</b><br> 6049 * </p> 6050 */ 6051 @SearchParamDefinition(name = "context-quantity", path = "(CodeSystem.useContext.value as Quantity) | (CodeSystem.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the code system", type = "quantity") 6052 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6053 /** 6054 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6055 * <p> 6056 * Description: <b>A quantity- or range-valued use context assigned to the code 6057 * system</b><br> 6058 * Type: <b>quantity</b><br> 6059 * Path: <b>CodeSystem.useContext.valueQuantity, 6060 * CodeSystem.useContext.valueRange</b><br> 6061 * </p> 6062 */ 6063 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6064 SP_CONTEXT_QUANTITY); 6065 6066 /** 6067 * Search parameter: <b>supplements</b> 6068 * <p> 6069 * Description: <b>Find code system supplements for the referenced code 6070 * system</b><br> 6071 * Type: <b>reference</b><br> 6072 * Path: <b>CodeSystem.supplements</b><br> 6073 * </p> 6074 */ 6075 @SearchParamDefinition(name = "supplements", path = "CodeSystem.supplements", description = "Find code system supplements for the referenced code system", type = "reference", target = { 6076 CodeSystem.class }) 6077 public static final String SP_SUPPLEMENTS = "supplements"; 6078 /** 6079 * <b>Fluent Client</b> search parameter constant for <b>supplements</b> 6080 * <p> 6081 * Description: <b>Find code system supplements for the referenced code 6082 * system</b><br> 6083 * Type: <b>reference</b><br> 6084 * Path: <b>CodeSystem.supplements</b><br> 6085 * </p> 6086 */ 6087 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLEMENTS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6088 SP_SUPPLEMENTS); 6089 6090 /** 6091 * Constant for fluent queries to be used to add include statements. Specifies 6092 * the path value of "<b>CodeSystem:supplements</b>". 6093 */ 6094 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLEMENTS = new ca.uhn.fhir.model.api.Include( 6095 "CodeSystem:supplements").toLocked(); 6096 6097 /** 6098 * Search parameter: <b>system</b> 6099 * <p> 6100 * Description: <b>The system for any codes defined by this code system (same as 6101 * 'url')</b><br> 6102 * Type: <b>uri</b><br> 6103 * Path: <b>CodeSystem.url</b><br> 6104 * </p> 6105 */ 6106 @SearchParamDefinition(name = "system", path = "CodeSystem.url", description = "The system for any codes defined by this code system (same as 'url')", type = "uri") 6107 public static final String SP_SYSTEM = "system"; 6108 /** 6109 * <b>Fluent Client</b> search parameter constant for <b>system</b> 6110 * <p> 6111 * Description: <b>The system for any codes defined by this code system (same as 6112 * 'url')</b><br> 6113 * Type: <b>uri</b><br> 6114 * Path: <b>CodeSystem.url</b><br> 6115 * </p> 6116 */ 6117 public static final ca.uhn.fhir.rest.gclient.UriClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.UriClientParam( 6118 SP_SYSTEM); 6119 6120 /** 6121 * Search parameter: <b>name</b> 6122 * <p> 6123 * Description: <b>Computationally friendly name of the code system</b><br> 6124 * Type: <b>string</b><br> 6125 * Path: <b>CodeSystem.name</b><br> 6126 * </p> 6127 */ 6128 @SearchParamDefinition(name = "name", path = "CodeSystem.name", description = "Computationally friendly name of the code system", type = "string") 6129 public static final String SP_NAME = "name"; 6130 /** 6131 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6132 * <p> 6133 * Description: <b>Computationally friendly name of the code system</b><br> 6134 * Type: <b>string</b><br> 6135 * Path: <b>CodeSystem.name</b><br> 6136 * </p> 6137 */ 6138 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6139 SP_NAME); 6140 6141 /** 6142 * Search parameter: <b>context</b> 6143 * <p> 6144 * Description: <b>A use context assigned to the code system</b><br> 6145 * Type: <b>token</b><br> 6146 * Path: <b>CodeSystem.useContext.valueCodeableConcept</b><br> 6147 * </p> 6148 */ 6149 @SearchParamDefinition(name = "context", path = "(CodeSystem.useContext.value as CodeableConcept)", description = "A use context assigned to the code system", type = "token") 6150 public static final String SP_CONTEXT = "context"; 6151 /** 6152 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6153 * <p> 6154 * Description: <b>A use context assigned to the code system</b><br> 6155 * Type: <b>token</b><br> 6156 * Path: <b>CodeSystem.useContext.valueCodeableConcept</b><br> 6157 * </p> 6158 */ 6159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6160 SP_CONTEXT); 6161 6162 /** 6163 * Search parameter: <b>publisher</b> 6164 * <p> 6165 * Description: <b>Name of the publisher of the code system</b><br> 6166 * Type: <b>string</b><br> 6167 * Path: <b>CodeSystem.publisher</b><br> 6168 * </p> 6169 */ 6170 @SearchParamDefinition(name = "publisher", path = "CodeSystem.publisher", description = "Name of the publisher of the code system", type = "string") 6171 public static final String SP_PUBLISHER = "publisher"; 6172 /** 6173 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6174 * <p> 6175 * Description: <b>Name of the publisher of the code system</b><br> 6176 * Type: <b>string</b><br> 6177 * Path: <b>CodeSystem.publisher</b><br> 6178 * </p> 6179 */ 6180 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6181 SP_PUBLISHER); 6182 6183 /** 6184 * Search parameter: <b>context-type-quantity</b> 6185 * <p> 6186 * Description: <b>A use context type and quantity- or range-based value 6187 * assigned to the code system</b><br> 6188 * Type: <b>composite</b><br> 6189 * Path: <b></b><br> 6190 * </p> 6191 */ 6192 @SearchParamDefinition(name = "context-type-quantity", path = "CodeSystem.useContext", description = "A use context type and quantity- or range-based value assigned to the code system", type = "composite", compositeOf = { 6193 "context-type", "context-quantity" }) 6194 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6195 /** 6196 * <b>Fluent Client</b> search parameter constant for 6197 * <b>context-type-quantity</b> 6198 * <p> 6199 * Description: <b>A use context type and quantity- or range-based value 6200 * assigned to the code system</b><br> 6201 * Type: <b>composite</b><br> 6202 * Path: <b></b><br> 6203 * </p> 6204 */ 6205 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6206 SP_CONTEXT_TYPE_QUANTITY); 6207 6208 /** 6209 * Search parameter: <b>status</b> 6210 * <p> 6211 * Description: <b>The current status of the code system</b><br> 6212 * Type: <b>token</b><br> 6213 * Path: <b>CodeSystem.status</b><br> 6214 * </p> 6215 */ 6216 @SearchParamDefinition(name = "status", path = "CodeSystem.status", description = "The current status of the code system", type = "token") 6217 public static final String SP_STATUS = "status"; 6218 /** 6219 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6220 * <p> 6221 * Description: <b>The current status of the code system</b><br> 6222 * Type: <b>token</b><br> 6223 * Path: <b>CodeSystem.status</b><br> 6224 * </p> 6225 */ 6226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6227 SP_STATUS); 6228 6229// added from java-adornments.txt: 6230 public PropertyComponent getProperty(String code) { 6231 for (PropertyComponent pd : getProperty()) { 6232 if (pd.getCode().equalsIgnoreCase(code)) 6233 return pd; 6234 } 6235 return null; 6236 } 6237 6238 public class ConceptDefinitionComponentSorter implements Comparator<ConceptDefinitionComponent> { 6239 @Override 6240 public int compare(ConceptDefinitionComponent l, ConceptDefinitionComponent r) { 6241 return l.getCode().compareTo(r.getCode()); 6242 } 6243 } 6244 6245 public void sort() { 6246 sort(getConcept(), new ConceptDefinitionComponentSorter()); 6247 } 6248 6249 public void sort(List<ConceptDefinitionComponent> list, Comparator<ConceptDefinitionComponent> comp) { 6250 Collections.sort(list, comp); 6251 for (ConceptDefinitionComponent def : list) { 6252 if (def.hasConcept()) { 6253 sort(def.getConcept(), comp); 6254 } 6255 } 6256 } 6257 6258 public void sort(Comparator<ConceptDefinitionComponent> comp) { 6259 sort(getConcept(), comp); 6260 } 6261 6262// end addition 6263 6264}